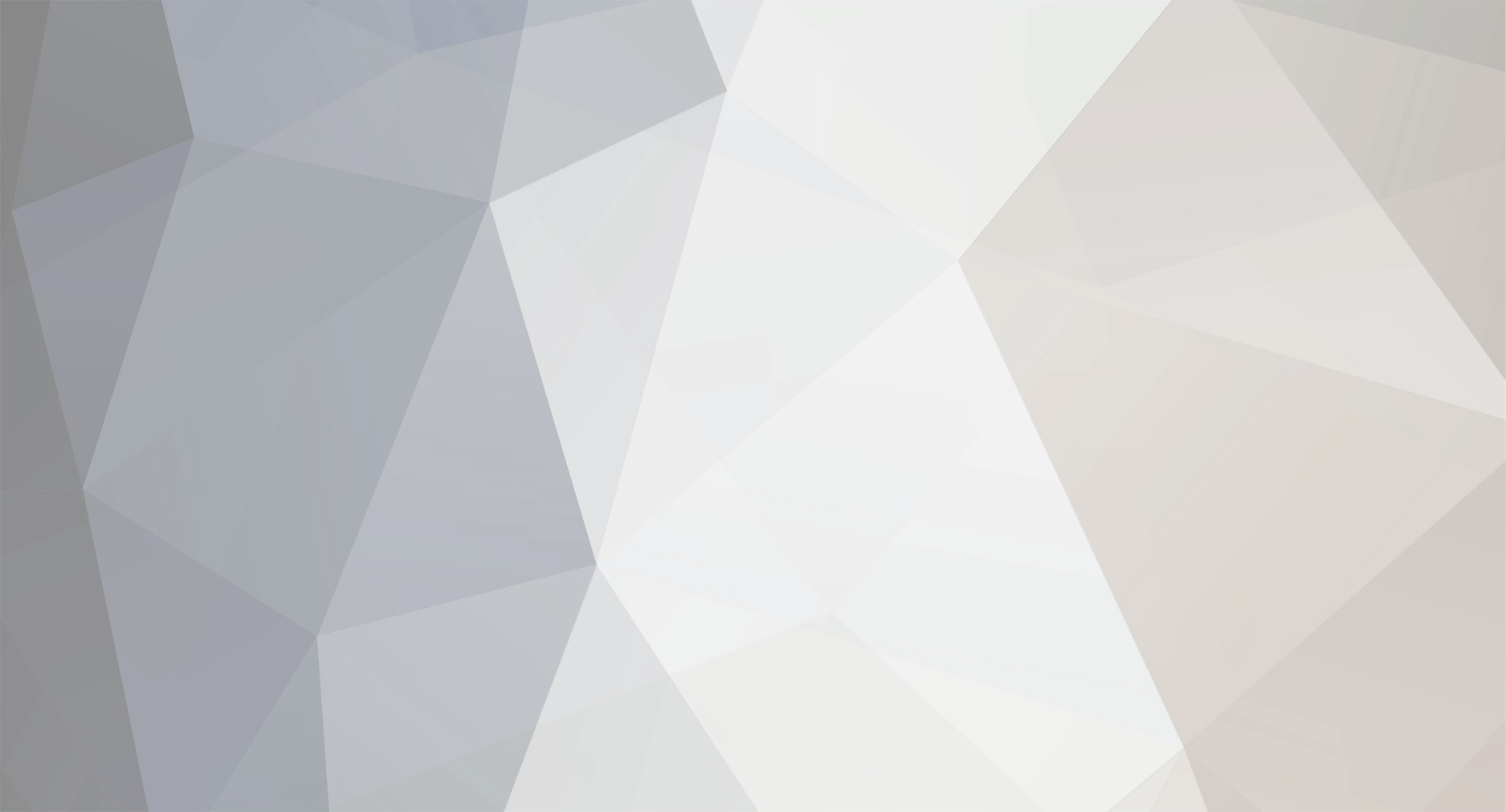
Electric
-
Posts
50 -
Joined
-
Last visited
Posts posted by Electric
-
-
Awesome, setRotationPoint works great in moving each block's position. Thanks for the help, Jabelar!
-
My model consists of a center that I would like to rotate and spin independently of the rest of the model. Right now, it's able to spin using rotateAngleY and values from a capability that stores an increasing int every tick after a player interacts with the entity. I would like to have the center similarly move up and down as well.
Here is my long model file:
-
I have been able to successfully rotate specific parts of my custom entity, using Block.rotateAngleY within this method of the model class:
public void setLivingAnimations(EntityLivingBase entityIn, float limbSwing, float limbSwingAmount, float partialTickTime)
Now I'm trying to figure out how to move specific parts up and down. Using the same method above, I tried using Block.offsetY, but it has absolutely no effect. Any ideas on how I can translate specific sections of my entity?
-
Yes, how do I add the slot and how can I override the inventory Gui with a custom one?
-
So I've fixed up the capability itself and it attaches to the player. Could someone go into more detail on how to add the extra slot itself to the inventory?
-
Any suggestions?
-
I'm attempting to use capabilities to create 2 custom inventory slots for every player. So far I've attached the capability to the player with an event handler, but I don't know what to put in the capability provider's "return capability" method. My code is as follows, any help would be appreciated.
Event Handler:
@SubscribeEvent public void attachEntityCapabilities( AttachCapabilitiesEvent<Entity> event) { if ( !( event.getObject() instanceof EntityPlayer ) ) { return ; } event.addCapability(CapabilityHandler.INV, new CustomInvProvider()); }
Non-working Capability provider:
@Override public boolean hasCapability(@Nonnull Capability<?> capability, @Nullable EnumFacing facing) { return capability == CapabilityHandler.INV; } @Override public <T> T getCapability(Capability<T> capability, EnumFacing facing) { if (capability == CapabilityItemHandler.ITEM_HANDLER_CAPABILITY) { return (T) inventory; } return super.getCapability(capability, facing); }
I don't understand what to return in the capability provider for hasCapability and getCapability. Any solutions?
-
I am interested in this too
-
I've done the toBytes and fromBytes. I'm just not sure how to copy it to the client-side instance, since there isn't much documentation on it.
-
1 hour ago, Draco18s said:
You need to synchronize the capability from the server to the client yourself.
Okay, now I'm confused on what goes in this method. How can I send the player capability data?
@Override public IMessage onMessage(MessageUpdateClientTardis message, MessageContext ctx) { EntityPlayerMP serverPlayer = ctx.getServerHandler().player; serverPlayer.getServerWorld().addScheduledTask(() -> { }); return null; }
-
Hello,
I’m trying to store a per-entity int using capabilities, which seems to be working. The problem I have is accessing them form the model file. entityIn.getcapability seems to only return the default capability values I’ve set. Any suggestions?
-
Can capabilities be used to store data in a plain .txt or .json file, instead of using NBT data?
-
On 9/14/2017 at 3:18 PM, diesieben07 said:
For example you do not need a separate interface and implementation class, you can just make a class that extends ItemStackHandler and use that as your capability.
So I'm not sure I completely follow you. I've created a class that extends ItemStackHandler, but I'm not sure what goes in there since I'm not creating an interface class. And since I don't need a capability provider, what would I put in the second parameter of event#addCapability()?
@SubscribeEvent public void attachCapability(AttachCapabilitiesEvent<Entity> event) { if (event.getObject() instanceof EntityPlayer) { event.addCapability(INV_SLOT,); } }
-
Hello,
My goal is to create a custom inventory slot, next to the already existing armor slots. I'm not entirely sure how to accomplish this, but I've read some things online about forge capabilities. It seems like the best way to go, except there doesn't seem to be much information on it. Could anyone help get me started?
-
1
-
-
I have currently called entityIn#addTag and playerIn#addTag to store little bits of data for specific players and entities in my mod, but after closing and relaunching the game, the tags disappear. Is there a way to save these tags, and restore them upon each relaunch of Minecraft?
-
6 hours ago, V0idWa1k3r said:
Block13.rotateAngleX = Math.toRadians((float)entityIn.ticksExisted / 2);
Additionally models are singletons. If you change a value of a fieldin your model it will apply to all your entities. I am talking about this:
Thank you so much! Now I've got the beginning of an animation, but is there a way to reset the entityIn#ticksExisted on each right click, so that the animation will begin at the same point each time?
And oops, I didn't realize this makes each of my entities do the same animation at the same time. How could I have the animation apply only to the entity being interacted with? I'm guessing it has something to do with entityIn#getEntityId, but I'm not sure. Does everything I do in the mob's model class affect each of my custom mobs?
-
So I'm wondering how I can make my mob do sort of a key frame animation. Right now I've got a simple rotation:
public void setRotationAngles(float limbSwing, float limbSwingAmount, float ageInTicks, float netHeadYaw, float headPitch, float scaleFactor, Entity entityIn) { if (playerRightClicked) { Block13.rotateAngleX = 2F; } else { Block13.rotateAngleX = 0F; } }
I'm hoping to somehow make it so each tick causes the Block13 to be rotated 0.5 more. Is there an easy way to accomplish this?
-
So I have created a custom mob, with its own model and rotations, but these rotations only activate while the mob is walking/wandering. How can I have the mob perform them while it is standing still? Right now I've just got some test rotations:
public void setRotationAngles(float par1, float par2, float par3, float par4, float par5, float par6, Entity entitypar7) { this.Block26.rotateAngleX = -1.5F * this.custommotion(par1, 13.0F) * par2; this.Block13.rotateAngleX = 1.5F * this.custommotion(par1, 13.0F) * par2; this.Block13.rotateAngleY = 0.0F; }
So for starters, I'd like block26 to constantly do its rotations, while the mob is standing still. Is there a way to accomplish this?
-
I have directly loaded an .obj model into the game using ModelLoaderRegistry#getModel. As this does not reference a blockstate file, I am unable to tell it to flip-v, which is necessary since I have exported this model from blender. Is there any way around this?
-
So my .obj file was exported from blender, and has the field mtllib itemsonicscrewdriver.mtl which seems to work, since it does give information on the .mtl file in the console log. Section of my .obj file:
Spoiler# Blender v2.78 (sub 0) OBJ File: '' # www.blender.org mtllib itemsonicscrewdriver.mtl o SonicScrewdriverReal v 0.125000 5.000000 0.125001 v -0.125000 -0.000000 0.125000
And I already did have the map_Kd operationbluebox:items/sonicscrewdrivertexture in my .mtl file. Is this problematic to have the texture applied in both the .mtl file and the .json blockstate file? I tried running the mod once with only the texture defined in the .json, and once with it only defined in the .mtl, but neither worked. My .mtl file:
Spoiler# Blender MTL File: 'None' # Material Count: 1 newmtl itemsonicscrewdriver Ns 96.078431 Ka 1.000000 1.000000 1.000000 Kd 0.640000 0.640000 0.640000 Ks 0.500000 0.500000 0.500000 Ke 0.000000 0.000000 0.000000 Ni 1.000000 d 1.000000 illum 2 map_Kd operationbluebox:items/sonicscrewdrivertexture
And I'm trying to register this model as an item only, but I'm also trying to have it use a blockstate file in order to add rotations and other information to the model.
I'm pretty certain that the model isn't working because I've done something wrong with the .json in the blockstates folder, but I'm not entirely sure.
-
3 minutes ago, Matryoshika said:
There's no other errors in the client_log?
I'm not sure why, but there are absolutely no errors in the log.
-
-
10 hours ago, Matryoshika said:
You have to call OBJLoader.INSTANCE.addDomain(MODID) before you register you models
I have done so in my ClientProxy, so I'm sure this can't be the issue ... unless I did it wrong somehow.
@Override public void preInit(FMLPreInitializationEvent event) { super.preInit(event); OBJLoader.INSTANCE.addDomain(OperationBlueBox.MODID); } @SubscribeEvent public static void registerModels(ModelRegistryEvent event) { ModBlocks.initModels(); ModItems.initModels(); }
I also find it weird that after running the Minecraft client, this shows in my console, which makes it seem as though it has loaded the .obj model, even though it does not show up in-game:
[FML]: OBJLoader.MaterialLibrary: key 'Ns' (model: 'operationbluebox:models/block/itemsonicscrewdriver.mtl') is not currently supported, skipping [10:28:08] [main/INFO] [FML]: OBJModel: A color has already been defined for material 'itemsonicscrewdriver' in 'operationbluebox:models/block/itemsonicscrewdriver.mtl'. The color defined by key 'Ks' will not be applied! [10:28:08] [main/INFO] [FML]: OBJLoader.MaterialLibrary: key 'Ke' (model: 'operationbluebox:models/block/itemsonicscrewdriver.mtl') is not currently supported, skipping [10:28:08] [main/INFO] [FML]: OBJLoader.MaterialLibrary: key 'Ni' (model: 'operationbluebox:models/block/itemsonicscrewdriver.mtl') is not currently supported, skipping [10:28:08] [main/INFO] [FML]: OBJLoader.MaterialLibrary: key 'illum' (model: 'operationbluebox:models/block/itemsonicscrewdriver.mtl') is not currently supported, skipping
-
Hello,
I'm attempting to create a mod with blocks and items rendered using .obj files. I have been able to successfully render a block from an .obj file, but rendering an item is proving to be much more difficult, and it relentlessly shows up as the pink and black texture in game. I cannot figure out what I am doing wrong.
Here is my item's class:
public ItemSonicScrewdriver() { setRegistryName("itemsonicscrewdriver"); setUnlocalizedName(OperationBlueBox.MODID + ".itemsonicscrewdriver"); } @SideOnly(Side.CLIENT) public void initModel() { ModelLoader.setCustomModelResourceLocation(this, 0, new ModelResourceLocation(getRegistryName(), "inventory")); }
And here's how I've registered it in my ModItems class, which is then used in my ClientProxy:
@GameRegistry.ObjectHolder("operationbluebox:itemsonicscrewdriver") public static ItemSonicScrewdriver itemSonicScrewdriver; @SideOnly(Side.CLIENT) public static void initModels() { itemSonicScrewdriver.initModel(); }
I've also tried using someone's block states file that seemed to work for them:
Spoiler{ "forge_marker": 1, "defaults": { "textures": { "#itemsonicscrewdriver": "operationbluebox:items/sonicscrewdrivertexture" }, "model": "operationbluebox:itemsonicscrewdriver.obj" }, "variants": { "normal": [{}], "inventory": [{ "transform": { "firstperson": { "translation": [0.1, 0, -0.3], "rotation": [ { "y": 130}, {"x": 0}, {"z": 0} ], "scale": 0.2 }, "thirdperson": { "translation": [0.02, 0.05, -0.05], "rotation": [ { "y": 0}, {"x": -92}, {"z": 0} ], "scale": 0.07 }, "gui": { "translation": [0, -0.1, 0], "scale": 0.16 } } }] } }
And I have made sure to place the .obj and .mtl files in my models/block folder. All help is appreciated.
[1.12.2] MultiPart Entity Interaction Help
in Modder Support
Posted
By following the EnderDragon's format, I have successfully created a multipart entity, complete with several different bounding boxes. After a player right clicks the entity, I would like to be able to detect which bounding box is being interacted with, but I am not sure if this is possible. IEntityMultiPart does supply the following method, which detects the bounding box, but it is only triggered with a left click. Any ideas?