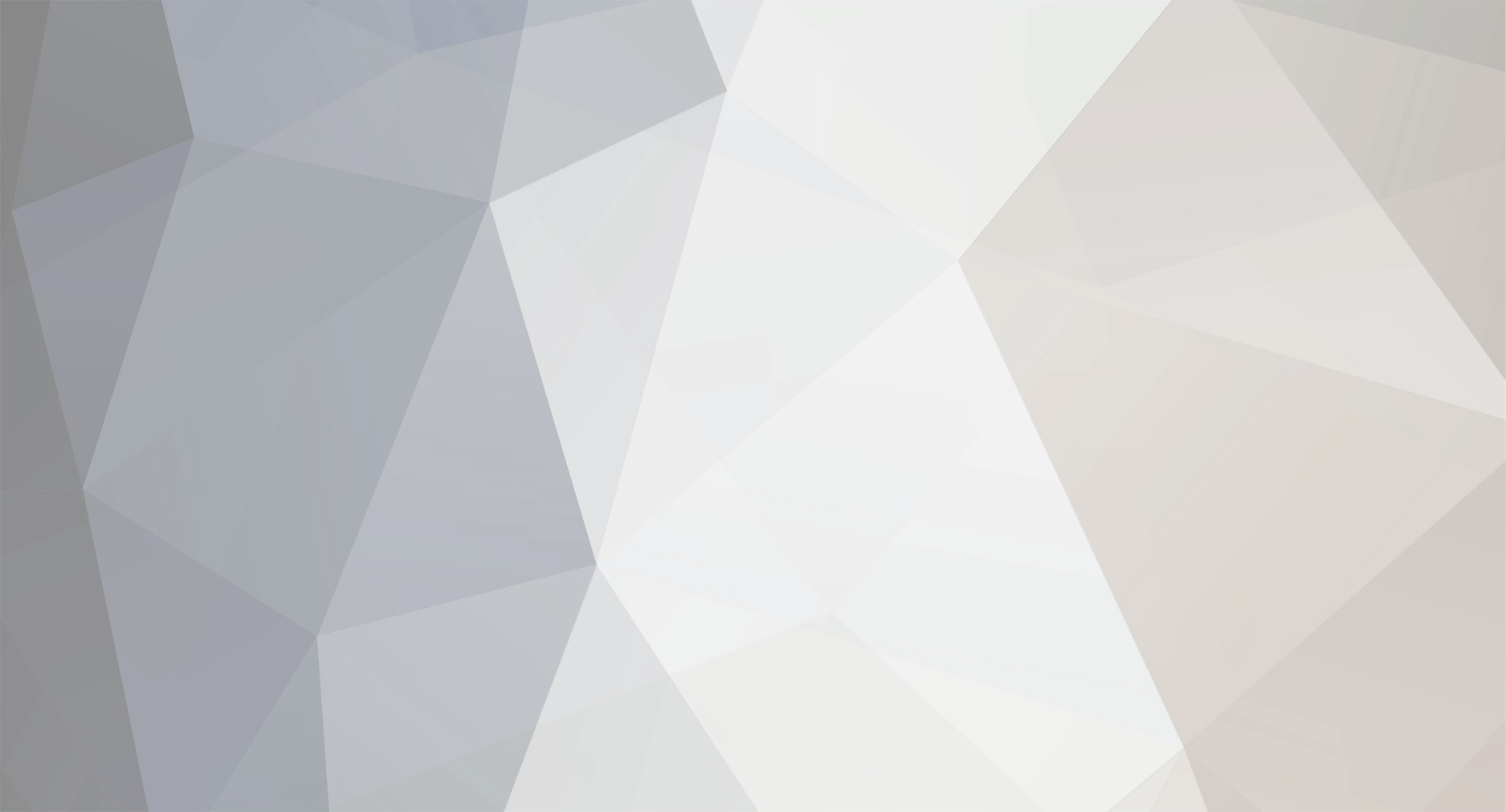
Big_Bad_E
-
Posts
312 -
Joined
-
Last visited
-
Days Won
1
Posts posted by Big_Bad_E
-
-
I have a slot, it has a texuture, the slot appears, but not the texture.
GUI:
public class BWGui extends GuiContainer { private final int BUTTON_X = 20; private final int BUTTON_Y = 20; private static final ResourceLocation GUI_BACKGROUND = new ResourceLocation("enhancedbows", "textures/gui/workbench_gui.png"); private static final ResourceLocation GUI_WIDGETS = new ResourceLocation("enhancedbows", "textures/gui/workbench_widgets.png"); BWContainer container; public BWGui(Container inventorySlotsIn) { super(inventorySlotsIn); container = (BWContainer) inventorySlotsIn; } @Override public void initGui() { super.initGui(); buttonList.clear(); int id = 1; for(int i = 0; i < BowTypes.values().length; i++) { buttonList.add(new GuiButtonImage(id, BUTTON_X*((id-1)/8)+Minecraft.getMinecraft().displayWidth/10, BUTTON_Y*((id-1)%8)+Minecraft.getMinecraft().displayHeight/20, 25, 25, 64*((id-1)/8), 64*((id-1)%8), 1, GUI_WIDGETS)); id += 1; } container.updateType(); } @Override protected void actionPerformed(GuiButton button) { container.updateType(); } @Override protected void drawGuiContainerBackgroundLayer(float partialTicks, int mouseX, int mouseY) { drawDefaultBackground(); Minecraft.getMinecraft().getTextureManager().bindTexture(GUI_BACKGROUND); drawTexturedModalRect(this.guiLeft/3*2, this.guiTop/4, 0, 0, 256, 256); } @Override protected void drawGuiContainerForegroundLayer(int mouseX, int mouseY) { } }
Container:
public class BWContainer extends Container { private TEBowWorkbench tile; private ItemStackHandler handler; public BowTypes type = null; BWContainer(InventoryPlayer inventory, TEBowWorkbench tile) { this.tile = tile; for(BowTypes type : BowTypes.values()) { if(type.ordinal() == tile.getTab()) { this.type = type; } } if(type == null) type = BowTypes.SHORTBOW; int i; for (i = 0; i < 3; ++i) { for (int j = 0; j < 9; ++j) { addSlotToContainer(new Slot(inventory, j + i * 9 + 9, Minecraft.getMinecraft().displayWidth/10 + j * 18, Minecraft.getMinecraft().displayHeight/10 + i * 18)); } } // add hotbar slots for (i = 0; i < 9; ++i) { addSlotToContainer(new Slot(inventory, i, Minecraft.getMinecraft().displayWidth/10 + i * 18, Minecraft.getMinecraft().displayHeight/10)); } } public void updateType() { int i = 0; if(type == null) type = BowTypes.SHORTBOW; if(handler != null) for(int i2 = 0; i2 < handler.getSlots(); i2++) inventorySlots.remove(i2); handler = new ItemStackHandler(type.materials.length); } @Override @ParametersAreNonnullByDefault public boolean canInteractWith(EntityPlayer playerIn) { return true; } @Override @MethodsReturnNonnullByDefault public ItemStack transferStackInSlot(EntityPlayer playerIn, int slotIndex) { ItemStack itemStack1 = null; Slot slot = inventorySlots.get(slotIndex); if (slot != null && slot.getHasStack()) { ItemStack itemStack2 = slot.getStack(); itemStack1 = itemStack2.copy(); // player inventory slots if (slotIndex >= 9 && slotIndex < 36) { if (!mergeItemStack(itemStack2, 9, 35, false)) return null; // hotbar slots } else if (slotIndex < 9 && !mergeItemStack(itemStack2, 2, 28, false)) { if (!mergeItemStack(itemStack2, 0, 8, false)) return null; } else if (!mergeItemStack(itemStack2, 0, 35, false)) { return null; } if (itemStack2.getCount() == 0) { slot.putStack(ItemStack.EMPTY); } else { slot.onSlotChanged(); } if (itemStack2.getCount() == itemStack1.getCount()) { return null; } } return itemStack1; } }
Misc enums are simple and I know the resource locations are correct, there are no errors in console.
I'm 99% sure I am doing a ton of things wrong with the positioning, but right now I am just focusing on getting the background textures to work.
-
7 hours ago, Cadiboo said:
Post your new logs please, are you sure your drawing your texture with the correct size at the correct coordinates?
Look at the post, I figured it out. Spaceboy was right.
-
Now the texture is still blank, but I don't get a file not found error.
-
I just copy pasted all the files from another mod and it worked for some reason... .-.
-
-
32 minutes ago, DaemonUmbra said:
There are .gradle folders in your project workspace and your user directory, try deleting those, note that next time you run anything Gradle related everything it had cached previously will need to be re-downloaded.
Also I am not 100% sure this will work.
I would also delete your build folder.
I deleted everything in the folder when I tried the 3 times
-
Just now, DaemonUmbra said:
Have you tried cleaning the gradle cache?
How is that done? That will probably fix everything.
-
3 minutes ago, DaemonUmbra said:
Exactly how did you start coding this project without Gradle?
It's not without gradle, but I copy pasted another mod I made, and changed the src files, which obviously was dumb and caused problems.
-
1 minute ago, DaemonUmbra said:
Can you post your code to GitHub so I can test it on my end?
The code works, it was working until I tried to get gradle working properly, but to test I've tested a different mod I know works and no dice.
-
Bump because I'd like to continue modding, and I can't do pretty much anything.
-
15 hours ago, Cadiboo said:
Redownload Forge and set it up in a new folder then paste your “src” folder into it
I've done that 3 times! I even tried it with gradlew intellij (or idea idk), but nothing!
-
For some reason Forge/Intellij isn't finding my mod that I have.
I set it up by extracting forge into my mod folder (EnhancedBows), then running gradlew setupDecompWorkspace, then setupDevWorkspace ideaModule, then imported the build.gradle, then ran genIntellijRuns.
I opened Intellij again, ran Minecraft Client, and no dice.
I tried it 3 times, tried setting up the mod in a forge folder then imported it as a module. Still nothing.
I have no idea what is wrong, I've done this a ton and this has never happened to me.
The 4 Forge default mods load btw.
Console log:
Spoiler"C:\Program Files\Java\jdk1.8.0_161\bin\java.exe" "-javaagent:C:\Program Files\JetBrains\IntelliJ IDEA Community Edition 2018.2.4\lib\idea_rt.jar=52663:C:\Program Files\JetBrains\IntelliJ IDEA Community Edition 2018.2.4\bin" -Dfile.encoding=UTF-8 -classpath "C:\Program Files\Java\jdk1.8.0_161\jre\lib\charsets.jar;C:\Program Files\Java\jdk1.8.0_161\jre\lib\deploy.jar;C:\Program Files\Java\jdk1.8.0_161\jre\lib\ext\access-bridge-64.jar;C:\Program Files\Java\jdk1.8.0_161\jre\lib\ext\cldrdata.jar;C:\Program Files\Java\jdk1.8.0_161\jre\lib\ext\dnsns.jar;C:\Program Files\Java\jdk1.8.0_161\jre\lib\ext\jaccess.jar;C:\Program Files\Java\jdk1.8.0_161\jre\lib\ext\jfxrt.jar;C:\Program Files\Java\jdk1.8.0_161\jre\lib\ext\localedata.jar;C:\Program Files\Java\jdk1.8.0_161\jre\lib\ext\nashorn.jar;C:\Program Files\Java\jdk1.8.0_161\jre\lib\ext\sunec.jar;C:\Program Files\Java\jdk1.8.0_161\jre\lib\ext\sunjce_provider.jar;C:\Program Files\Java\jdk1.8.0_161\jre\lib\ext\sunmscapi.jar;C:\Program Files\Java\jdk1.8.0_161\jre\lib\ext\sunpkcs11.jar;C:\Program Files\Java\jdk1.8.0_161\jre\lib\ext\zipfs.jar;C:\Program Files\Java\jdk1.8.0_161\jre\lib\javaws.jar;C:\Program Files\Java\jdk1.8.0_161\jre\lib\jce.jar;C:\Program Files\Java\jdk1.8.0_161\jre\lib\jfr.jar;C:\Program Files\Java\jdk1.8.0_161\jre\lib\jfxswt.jar;C:\Program Files\Java\jdk1.8.0_161\jre\lib\jsse.jar;C:\Program Files\Java\jdk1.8.0_161\jre\lib\management-agent.jar;C:\Program Files\Java\jdk1.8.0_161\jre\lib\plugin.jar;C:\Program Files\Java\jdk1.8.0_161\jre\lib\resources.jar;C:\Program Files\Java\jdk1.8.0_161\jre\lib\rt.jar;C:\Users\me\Desktop\Minecraft Mods\EnhancedBows\out\production\EnhancedBows_main;C:\Users\me\.gradle\caches\minecraft\deobfedDeps\compileDummy.jar;C:\Users\me\.gradle\caches\minecraft\deobfedDeps\providedDummy.jar;C:\Users\me\.gradle\caches\minecraft\net\minecraftforge\forge\1.12.2-14.23.4.2705\snapshot\20171003\forgeSrc-1.12.2-14.23.4.2705.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\com.google.code.findbugs\jsr305\3.0.1\f7be08ec23c21485b9b5a1cf1654c2ec8c58168d\jsr305-3.0.1.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\com.mojang\patchy\1.1\aef610b34a1be37fa851825f12372b78424d8903\patchy-1.1.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\oshi-project\oshi-core\1.1\9ddf7b048a8d701be231c0f4f95fd986198fd2d8\oshi-core-1.1.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\net.java.dev.jna\jna\4.4.0\cb208278274bf12ebdb56c61bd7407e6f774d65a\jna-4.4.0.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\net.java.dev.jna\platform\3.4.0\e3f70017be8100d3d6923f50b3d2ee17714e9c13\platform-3.4.0.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\com.ibm.icu\icu4j-core-mojang\51.2\63d216a9311cca6be337c1e458e587f99d382b84\icu4j-core-mojang-51.2.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\net.sf.jopt-simple\jopt-simple\5.0.3\cdd846cfc4e0f7eefafc02c0f5dce32b9303aa2a\jopt-simple-5.0.3.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\io.netty\netty-all\4.1.9.Final\97860965d6a0a6b98e7f569f3f966727b8db75\netty-all-4.1.9.Final.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\com.google.guava\guava\21.0\3a3d111be1be1b745edfa7d91678a12d7ed38709\guava-21.0.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.apache.commons\commons-lang3\3.5\6c6c702c89bfff3cd9e80b04d668c5e190d588c6\commons-lang3-3.5.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\commons-io\commons-io\2.5\2852e6e05fbb95076fc091f6d1780f1f8fe35e0f\commons-io-2.5.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\commons-codec\commons-codec\1.10\4b95f4897fa13f2cd904aee711aeafc0c5295cd8\commons-codec-1.10.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\net.java.jutils\jutils\1.0.0\e12fe1fda814bd348c1579329c86943d2cd3c6a6\jutils-1.0.0.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\com.google.code.gson\gson\2.8.0\c4ba5371a29ac9b2ad6129b1d39ea38750043eff\gson-2.8.0.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\com.mojang\authlib\1.5.25\9834cdf236c22e84b946bba989e2f94ef5897c3c\authlib-1.5.25.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\com.mojang\realms\1.10.22\bd0dccebdf3744c75f1ca20063f16e8f7d5e663f\realms-1.10.22.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.apache.commons\commons-compress\1.8.1\a698750c16740fd5b3871425f4cb3bbaa87f529d\commons-compress-1.8.1.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.apache.httpcomponents\httpclient\4.3.3\18f4247ff4572a074444572cee34647c43e7c9c7\httpclient-4.3.3.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\commons-logging\commons-logging\1.1.3\f6f66e966c70a83ffbdb6f17a0919eaf7c8aca7f\commons-logging-1.1.3.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.apache.httpcomponents\httpcore\4.3.2\31fbbff1ddbf98f3aa7377c94d33b0447c646b6e\httpcore-4.3.2.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\it.unimi.dsi\fastutil\7.1.0\9835253257524c1be7ab50c057aa2d418fb72082\fastutil-7.1.0.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.apache.logging.log4j\log4j-api\2.8.1\e801d13612e22cad62a3f4f3fe7fdbe6334a8e72\log4j-api-2.8.1.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.apache.logging.log4j\log4j-core\2.8.1\4ac28ff2f1ddf05dae3043a190451e8c46b73c31\log4j-core-2.8.1.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\com.mojang\text2speech\1.10.3\48fd510879dff266c3815947de66e3d4809f8668\text2speech-1.10.3.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\net.minecraft\launchwrapper\1.12\111e7bea9c968cdb3d06ef4632bf7ff0824d0f36\launchwrapper-1.12.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\jline\jline\2.13\2d9530d0a25daffaffda7c35037b046b627bb171\jline-2.13.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.ow2.asm\asm-debug-all\5.2\3354e11e2b34215f06dab629ab88e06aca477c19\asm-debug-all-5.2.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\com.typesafe.akka\akka-actor_2.11\2.3.3\ed62e9fc709ca0f2ff1a3220daa8b70a2870078e\akka-actor_2.11-2.3.3.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\com.typesafe\config\1.2.1\f771f71fdae3df231bcd54d5ca2d57f0bf93f467\config-1.2.1.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.scala-lang\scala-actors-migration_2.11\1.1.0\dfa8bc42b181d5b9f1a5dd147f8ae308b893eb6f\scala-actors-migration_2.11-1.1.0.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.scala-lang\scala-compiler\2.11.1\56ea2e6c025e0821f28d73ca271218b8dd04926a\scala-compiler-2.11.1.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.scala-lang.plugins\scala-continuations-library_2.11\1.0.2\e517c53a7e9acd6b1668c5a35eccbaa3bab9aac\scala-continuations-library_2.11-1.0.2.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.scala-lang.plugins\scala-continuations-plugin_2.11.1\1.0.2\f361a3283452c57fa30c1ee69448995de23c60f7\scala-continuations-plugin_2.11.1-1.0.2.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.scala-lang\scala-library\2.11.1\e11da23da3eabab9f4777b9220e60d44c1aab6a\scala-library-2.11.1.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.scala-lang.modules\scala-parser-combinators_2.11\1.0.1\f05d7345bf5a58924f2837c6c1f4d73a938e1ff0\scala-parser-combinators_2.11-1.0.1.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.scala-lang\scala-reflect\2.11.1\6580347e61cc7f8e802941e7fde40fa83b8badeb\scala-reflect-2.11.1.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.scala-lang.modules\scala-swing_2.11\1.0.1\b1cdd92bd47b1e1837139c1c53020e86bb9112ae\scala-swing_2.11-1.0.1.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.scala-lang.modules\scala-xml_2.11\1.0.2\820fbca7e524b530fdadc594c39d49a21ea0337e\scala-xml_2.11-1.0.2.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\lzma\lzma\0.0.1\521616dc7487b42bef0e803bd2fa3faf668101d7\lzma-0.0.1.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\net.sf.trove4j\trove4j\3.0.3\42ccaf4761f0dfdfa805c9e340d99a755907e2dd\trove4j-3.0.3.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.apache.maven\maven-artifact\3.5.3\7dc72b6d6d8a6dced3d294ed54c2cc3515ade9f4\maven-artifact-3.5.3.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\com.paulscode\codecjorbis\20101023\c73b5636faf089d9f00e8732a829577de25237ee\codecjorbis-20101023.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\com.paulscode\codecwav\20101023\12f031cfe88fef5c1dd36c563c0a3a69bd7261da\codecwav-20101023.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\com.paulscode\libraryjavasound\20101123\5c5e304366f75f9eaa2e8cca546a1fb6109348b3\libraryjavasound-20101123.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\com.paulscode\librarylwjglopenal\20100824\73e80d0794c39665aec3f62eee88ca91676674ef\librarylwjglopenal-20100824.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\com.paulscode\soundsystem\20120107\419c05fe9be71f792b2d76cfc9b67f1ed0fec7f6\soundsystem-20120107.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\net.java.jinput\jinput\2.0.5\39c7796b469a600f72380316f6b1f11db6c2c7c4\jinput-2.0.5.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.lwjgl.lwjgl\lwjgl\2.9.4-nightly-20150209\697517568c68e78ae0b4544145af031c81082dfe\lwjgl-2.9.4-nightly-20150209.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.lwjgl.lwjgl\lwjgl_util\2.9.4-nightly-20150209\d51a7c040a721d13efdfbd34f8b257b2df882ad0\lwjgl_util-2.9.4-nightly-20150209.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\java3d\vecmath\1.5.2\79846ba34cbd89e2422d74d53752f993dcc2ccaf\vecmath-1.5.2.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\ca.weblite\java-objc-bridge\1.0.0\6ef160c3133a78de015830860197602ca1c855d3\java-objc-bridge-1.0.0.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.fusesource.jansi\jansi\1.11\655c643309c2f45a56a747fda70e3fadf57e9f11\jansi-1.11.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.scala-lang\scala-actors\2.11.0\8ccfb6541de179bb1c4d45cf414acee069b7f78b\scala-actors-2.11.0.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.codehaus.plexus\plexus-utils\3.1.0\60eecb6f15abdb1c653ad80abaac6fe188b3feaa\plexus-utils-3.1.0.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\net.java.jinput\jinput-platform\2.0.5\7ff832a6eb9ab6a767f1ade2b548092d0fa64795\jinput-platform-2.0.5-natives-linux.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\net.java.jinput\jinput-platform\2.0.5\385ee093e01f587f30ee1c8a2ee7d408fd732e16\jinput-platform-2.0.5-natives-windows.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\net.java.jinput\jinput-platform\2.0.5\53f9c919f34d2ca9de8c51fc4e1e8282029a9232\jinput-platform-2.0.5-natives-osx.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.lwjgl.lwjgl\lwjgl-platform\2.9.4-nightly-20150209\b84d5102b9dbfabfeb5e43c7e2828d98a7fc80e0\lwjgl-platform-2.9.4-nightly-20150209-natives-windows.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.lwjgl.lwjgl\lwjgl-platform\2.9.4-nightly-20150209\931074f46c795d2f7b30ed6395df5715cfd7675b\lwjgl-platform-2.9.4-nightly-20150209-natives-linux.jar;C:\Users\me\.gradle\caches\modules-2\files-2.1\org.lwjgl.lwjgl\lwjgl-platform\2.9.4-nightly-20150209\bcab850f8f487c3f4c4dbabde778bb82bd1a40ed\lwjgl-platform-2.9.4-nightly-20150209-natives-osx.jar;C:\Users\me\.gradle\caches\minecraft\net\minecraftforge\forge\1.12.2-14.23.4.2705\start" GradleStart
[19:14:04] [main/INFO] [GradleStart]: Extra: []
[19:14:04] [main/INFO] [GradleStart]: Running with arguments: [--userProperties, {}, --assetsDir, C:/Users/me/.gradle/caches/minecraft/assets, --assetIndex, 1.12, --accessToken{REDACTED}, --version, 1.12.2, --tweakClass, net.minecraftforge.fml.common.launcher.FMLTweaker, --tweakClass, net.minecraftforge.gradle.tweakers.CoremodTweaker]
[19:14:04] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.FMLTweaker
[19:14:04] [main/INFO] [LaunchWrapper]: Using primary tweak class name net.minecraftforge.fml.common.launcher.FMLTweaker
[19:14:04] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.gradle.tweakers.CoremodTweaker
[19:14:04] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLTweaker
[19:14:04] [main/INFO] [FML]: Forge Mod Loader version 14.23.4.2705 for Minecraft 1.12.2 loading
[19:14:04] [main/INFO] [FML]: Java is Java HotSpot(TM) 64-Bit Server VM, version 1.8.0_161, running on Windows 10:amd64:10.0, installed at C:\Program Files\Java\jdk1.8.0_161\jre
[19:14:05] [main/ERROR] [FML]: Apache Maven library folder was not in the format expected. Using default libraries directory.
[19:14:05] [main/ERROR] [FML]: Full: C:\Users\me\.gradle\caches\modules-2\files-2.1\org.apache.maven\maven-artifact\3.5.3\7dc72b6d6d8a6dced3d294ed54c2cc3515ade9f4\maven-artifact-3.5.3.jar
[19:14:05] [main/ERROR] [FML]: Trimmed: c:/users/me/.gradle/caches/modules-2/files-2.1/org.apache.maven/maven-artifact/3.5.3/
[19:14:05] [main/INFO] [FML]: Managed to load a deobfuscated Minecraft name- we are in a deobfuscated environment. Skipping runtime deobfuscation
[19:14:05] [main/INFO] [FML]: Ignoring missing certificate for coremod FMLCorePlugin (net.minecraftforge.fml.relauncher.FMLCorePlugin), we are in deobf and it's a forge core plugin
[19:14:05] [main/INFO] [FML]: Ignoring missing certificate for coremod FMLForgePlugin (net.minecraftforge.classloading.FMLForgePlugin), we are in deobf and it's a forge core plugin
[19:14:05] [main/INFO] [FML]: Searching C:\Users\me\Desktop\Minecraft Mods\EnhancedBows\run\.\mods for mods
[19:14:05] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.gradle.tweakers.CoremodTweaker
[19:14:05] [main/INFO] [GradleStart]: Injecting location in coremod net.minecraftforge.fml.relauncher.FMLCorePlugin
[19:14:05] [main/INFO] [GradleStart]: Injecting location in coremod net.minecraftforge.classloading.FMLForgePlugin
[19:14:05] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.FMLInjectionAndSortingTweaker
[19:14:05] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.FMLDeobfTweaker
[19:14:05] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.gradle.tweakers.AccessTransformerTweaker
[19:14:05] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLInjectionAndSortingTweaker
[19:14:05] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLInjectionAndSortingTweaker
[19:14:05] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.relauncher.CoreModManager$FMLPluginWrapper
[19:14:09] [main/ERROR] [FML]: FML appears to be missing any signature data. This is not a good thing
[19:14:09] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.relauncher.CoreModManager$FMLPluginWrapper
[19:14:09] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLDeobfTweaker
[19:14:11] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.gradle.tweakers.AccessTransformerTweaker
[19:14:11] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.TerminalTweaker
[19:14:11] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.TerminalTweaker
[19:14:11] [main/INFO] [LaunchWrapper]: Launching wrapped minecraft {net.minecraft.client.main.Main}
[19:14:12] [main/INFO] [net.minecraft.client.Minecraft]: Setting user: Player335
[19:14:21] [main/INFO] [net.minecraft.client.Minecraft]: LWJGL Version: 2.9.4
[19:14:23] [main/INFO] [FML]: -- System Details --
Details:
Minecraft Version: 1.12.2
Operating System: Windows 10 (amd64) version 10.0
Java Version: 1.8.0_161, Oracle Corporation
Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation
Memory: 488539904 bytes (465 MB) / 611319808 bytes (583 MB) up to 3808428032 bytes (3632 MB)
JVM Flags: 0 total;
IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0
FML:
Loaded coremods (and transformers):
GL info: ' Vendor: 'ATI Technologies Inc.' Version: '4.5.13539 Compatibility Profile Context 24.20.13017.5001' Renderer: 'Radeon RX 580 Series'
[19:14:23] [main/INFO] [FML]: MinecraftForge v14.23.4.2705 Initialized
[19:14:23] [main/INFO] [FML]: Starts to replace vanilla recipe ingredients with ore ingredients.
[19:14:23] [main/INFO] [FML]: Replaced 1036 ore ingredients
[19:14:24] [main/INFO] [FML]: Searching C:\Users\me\Desktop\Minecraft Mods\EnhancedBows\run\.\mods for mods
[19:14:25] [main/INFO] [FML]: Forge Mod Loader has identified 4 mods to load
[19:14:25] [Thread-3/INFO] [FML]: Using sync timing. 200 frames of Display.update took 135660160 nanos
[19:14:26] [main/INFO] [FML]: Attempting connection with missing mods [minecraft, mcp, FML, forge] at CLIENT
[19:14:26] [main/INFO] [FML]: Attempting connection with missing mods [minecraft, mcp, FML, forge] at SERVER
[19:14:28] [main/INFO] [net.minecraft.client.resources.SimpleReloadableResourceManager]: Reloading ResourceManager: Default, FMLFileResourcePack:Forge Mod Loader, FMLFileResourcePack:Minecraft Forge
[19:14:28] [main/INFO] [FML]: Processing ObjectHolder annotations
[19:14:28] [main/INFO] [FML]: Found 1168 ObjectHolder annotations
[19:14:28] [main/INFO] [FML]: Identifying ItemStackHolder annotations
[19:14:28] [main/INFO] [FML]: Found 0 ItemStackHolder annotations
[19:14:28] [main/INFO] [FML]: Configured a dormant chunk cache size of 0
[19:14:28] [Forge Version Check/INFO] [forge.VersionCheck]: [forge] Starting version check at http://files.minecraftforge.net/maven/net/minecraftforge/forge/promotions_slim.json
[19:14:28] [main/INFO] [FML]: Applying holder lookups
[19:14:28] [main/INFO] [FML]: Holder lookups applied
[19:14:28] [main/INFO] [FML]: Applying holder lookups
[19:14:28] [main/INFO] [FML]: Holder lookups applied
[19:14:28] [main/INFO] [FML]: Applying holder lookups
[19:14:28] [main/INFO] [FML]: Holder lookups applied
[19:14:28] [main/INFO] [FML]: Applying holder lookups
[19:14:28] [main/INFO] [FML]: Holder lookups applied
[19:14:28] [main/INFO] [FML]: Injecting itemstacks
[19:14:28] [main/INFO] [FML]: Itemstack injection complete
[19:14:29] [Forge Version Check/INFO] [forge.VersionCheck]: [forge] Found status: UP_TO_DATE Target: null
[19:14:36] [Sound Library Loader/INFO] [net.minecraft.client.audio.SoundManager]: Starting up SoundSystem...
[19:14:36] [Thread-5/INFO] [net.minecraft.client.audio.SoundManager]: Initializing LWJGL OpenAL
[19:14:36] [Thread-5/INFO] [net.minecraft.client.audio.SoundManager]: (The LWJGL binding of OpenAL. For more information, see http://www.lwjgl.org)
[19:14:36] [Thread-5/INFO] [net.minecraft.client.audio.SoundManager]: OpenAL initialized.
[19:14:36] [Sound Library Loader/INFO] [net.minecraft.client.audio.SoundManager]: Sound engine started
[19:14:47] [main/INFO] [FML]: Max texture size: 16384
[19:14:48] [main/INFO] [net.minecraft.client.renderer.texture.TextureMap]: Created: 512x512 textures-atlas
[19:14:51] [main/INFO] [FML]: Applying holder lookups
[19:14:51] [main/INFO] [FML]: Holder lookups applied
[19:14:51] [main/INFO] [FML]: Injecting itemstacks
[19:14:51] [main/INFO] [FML]: Itemstack injection complete
[19:14:52] [main/INFO] [FML]: Forge Mod Loader has successfully loaded 4 mods
[19:14:52] [main/INFO] [com.mojang.text2speech.NarratorWindows]: Narrator library for x64 successfully loaded
[19:14:53] [Realms Notification Availability checker #1/INFO] [com.mojang.realmsclient.client.RealmsClient]: Could not authorize you against Realms server: Invalid session id
[19:14:59] [Server thread/INFO] [net.minecraft.server.integrated.IntegratedServer]: Starting integrated minecraft server version 1.12.2
[19:14:59] [Server thread/INFO] [net.minecraft.server.integrated.IntegratedServer]: Generating keypair
[19:15:00] [Server thread/INFO] [FML]: Injecting existing registry data into this server instance
[19:15:00] [Server thread/INFO] [FML]: Applying holder lookups
[19:15:00] [Server thread/INFO] [FML]: Holder lookups applied
[19:15:01] [Server thread/INFO] [FML]: Loading dimension 0 (Test) (net.minecraft.server.integrated.IntegratedServer@9670c21)
[19:15:02] [Server thread/INFO] [net.minecraft.advancements.AdvancementList]: Loaded 488 advancements
[19:15:02] [Server thread/INFO] [FML]: Loading dimension 1 (Test) (net.minecraft.server.integrated.IntegratedServer@9670c21)
[19:15:02] [Server thread/INFO] [FML]: Loading dimension -1 (Test) (net.minecraft.server.integrated.IntegratedServer@9670c21)
[19:15:02] [Server thread/INFO] [net.minecraft.server.MinecraftServer]: Preparing start region for level 0
[19:15:03] [Server thread/INFO] [net.minecraft.server.MinecraftServer]: Preparing spawn area: 0%
[19:15:04] [Server thread/INFO] [net.minecraft.server.MinecraftServer]: Preparing spawn area: 46%
[19:15:06] [Server thread/INFO] [FML]: Unloading dimension -1
[19:15:06] [Server thread/INFO] [FML]: Unloading dimension 1
[19:15:06] [Server thread/INFO] [net.minecraft.server.integrated.IntegratedServer]: Changing view distance to 12, from 10
[19:15:08] [Netty Local Client IO #0/INFO] [FML]: Server protocol version 2
[19:15:08] [Netty Server IO #1/INFO] [FML]: Client protocol version 2
[19:15:08] [Netty Server IO #1/INFO] [FML]: Client attempting to join with 4 mods : [email protected],[email protected],[email protected],[email protected]
[19:15:08] [Netty Local Client IO #0/INFO] [FML]: [Netty Local Client IO #0] Client side modded connection established
[19:15:08] [Server thread/INFO] [FML]: [Server thread] Server side modded connection established
[19:15:08] [Server thread/INFO] [net.minecraft.server.management.PlayerList]: Player335[local:E:49971342] logged in with entity id 496 at (98.5, 79.0, 254.5)
[19:15:08] [Server thread/INFO] [net.minecraft.server.MinecraftServer]: Player335 joined the game
[19:15:11] [Server thread/INFO] [net.minecraft.server.integrated.IntegratedServer]: Saving and pausing game...
[19:15:11] [Server thread/INFO] [net.minecraft.server.MinecraftServer]: Saving chunks for level 'Test'/overworld
[19:15:11] [pool-2-thread-1/WARN] [com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService]: Couldn't look up profile properties for com.mojang.authlib.GameProfile@2c580a75[id=168f5f60-1523-35b7-93b7-01b2c42226b4,name=Player335,properties={},legacy=false]
com.mojang.authlib.exceptions.AuthenticationException: The client has sent too many requests within a certain amount of time
at com.mojang.authlib.yggdrasil.YggdrasilAuthenticationService.makeRequest(YggdrasilAuthenticationService.java:79) ~[YggdrasilAuthenticationService.class:?]
at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService.fillGameProfile(YggdrasilMinecraftSessionService.java:180) [YggdrasilMinecraftSessionService.class:?]
at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService$1.load(YggdrasilMinecraftSessionService.java:60) [YggdrasilMinecraftSessionService$1.class:?]
at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService$1.load(YggdrasilMinecraftSessionService.java:57) [YggdrasilMinecraftSessionService$1.class:?]
at com.google.common.cache.LocalCache$LoadingValueReference.loadFuture(LocalCache.java:3716) [guava-21.0.jar:?]
at com.google.common.cache.LocalCache$Segment.loadSync(LocalCache.java:2424) [guava-21.0.jar:?]
at com.google.common.cache.LocalCache$Segment.lockedGetOrLoad(LocalCache.java:2298) [guava-21.0.jar:?]
at com.google.common.cache.LocalCache$Segment.get(LocalCache.java:2211) [guava-21.0.jar:?]
at com.google.common.cache.LocalCache.get(LocalCache.java:4154) [guava-21.0.jar:?]
at com.google.common.cache.LocalCache.getOrLoad(LocalCache.java:4158) [guava-21.0.jar:?]
at com.google.common.cache.LocalCache$LocalLoadingCache.get(LocalCache.java:5147) [guava-21.0.jar:?]
at com.google.common.cache.LocalCache$LocalLoadingCache.getUnchecked(LocalCache.java:5153) [guava-21.0.jar:?]
at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService.fillProfileProperties(YggdrasilMinecraftSessionService.java:170) [YggdrasilMinecraftSessionService.class:?]
at net.minecraft.client.Minecraft.getProfileProperties(Minecraft.java:3178) [Minecraft.class:?]
at net.minecraft.client.resources.SkinManager$3.run(SkinManager.java:138) [SkinManager$3.class:?]
at java.util.concurrent.Executors$RunnableAdapter.call(Executors.java:511) [?:1.8.0_161]
at java.util.concurrent.FutureTask.run(FutureTask.java:266) [?:1.8.0_161]
at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1149) [?:1.8.0_161]
at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:624) [?:1.8.0_161]
at java.lang.Thread.run(Thread.java:748) [?:1.8.0_161]
[19:15:12] [Server thread/WARN] [net.minecraft.server.MinecraftServer]: Can't keep up! Did the system time change, or is the server overloaded? Running 2877ms behind, skipping 57 tick(s)
[19:15:20] [Server thread/INFO] [net.minecraft.server.integrated.IntegratedServer]: Saving and pausing game...
[19:15:20] [Server thread/INFO] [net.minecraft.server.MinecraftServer]: Saving chunks for level 'Test'/overworld
[19:36:53] [main/INFO] [net.minecraft.client.Minecraft]: Stopping!
[19:36:53] [Server thread/INFO] [net.minecraft.server.MinecraftServer]: Stopping server
[19:36:53] [Server thread/INFO] [net.minecraft.server.MinecraftServer]: Saving players
[19:36:53] [Server thread/INFO] [net.minecraft.server.MinecraftServer]: Saving worlds
[19:36:53] [Server thread/INFO] [net.minecraft.server.MinecraftServer]: Saving chunks for level 'Test'/overworld
[19:36:53] [Server thread/INFO] [FML]: Unloading dimension 0
[19:36:53] [Server thread/INFO] [FML]: Applying holder lookups
[19:36:53] [Server thread/INFO] [FML]: Holder lookups applied
[19:36:53] [main/INFO] [net.minecraft.client.audio.SoundManager]: SoundSystem shutting down...
[19:36:54] [main/WARN] [net.minecraft.client.audio.SoundManager]: Author: Paul Lamb, www.paulscode.comLog:
http://www.mediafire.com/file/mx4lnaw19ha3wpc/debug.log
-
6 hours ago, Spaceboy Ross said:
I had the same problem with my mod but deleting the pack.mcmeta file fixed it for me.
That was probaly my problem, but I was lazy and copy pasted the files from another set up mod and gradle was hating me for it.
-
4 minutes ago, V0idWa1k3r said:
Ahem.
"Please bind my texture and then draw it at position [0,0] with a size of [0,0]"
I don't think that this is the behaviour you wanted.
Also you do not need to do this
The game already does that for you.
I just had a placeholder for the draw to see if the gui actually opened, saw the error, and didn't fix the placeholder.
Thanks for telling me the buttons draw themself, didn't know.
-
1 minute ago, Animefan8888 said:
Sometimes there is an extra "clause" that gives more information. I was mainly looking for the exact file path it was looking in. But my next logical check would be, did you refresh eclipses/ideas files.
I forgot to refresh, but still didn't change anything.
Nothing else, but I think I know what the problem is. Thanks for the help.
-
Just now, Animefan8888 said:
Is that the whole error or is there a part that says caused by below that part.
What do you mean a caused by part? Its a FileNotFoundException, look in the spoiler.
-
8 minutes ago, Animefan8888 said:
Did it say anything in the console about a missing texture? Also are you sure that your draw method is being called?
Yes, I said it can't find the texture. The method is being called bc the drawDefaultBackground() method is being called
Spoiler[09:54:24] [main/WARN] [net.minecraft.client.renderer.texture.TextureManager]: Failed to load texture: enhancedbows:textures\gui\workbench_gui.png
java.io.FileNotFoundException: enhancedbows:textures\gui\workbench_gui.png
at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:69) ~[SimpleReloadableResourceManager.class:?]
at net.minecraft.client.renderer.texture.SimpleTexture.loadTexture(SimpleTexture.java:34) ~[SimpleTexture.class:?]
at net.minecraft.client.renderer.texture.TextureManager.loadTexture(TextureManager.java:69) [TextureManager.class:?]
at net.minecraft.client.renderer.texture.TextureManager.bindTexture(TextureManager.java:44) [TextureManager.class:?]
at bigbade.enhancedbows.guis.bowworkbench.BWGui.drawGuiContainerBackgroundLayer(BWGui.java:47) [BWGui.class:?]
at net.minecraft.client.gui.inventory.GuiContainer.drawScreen(GuiContainer.java:93) [GuiContainer.class:?]
at net.minecraftforge.client.ForgeHooksClient.drawScreen(ForgeHooksClient.java:368) [ForgeHooksClient.class:?]
at net.minecraft.client.renderer.EntityRenderer.updateCameraAndRender(EntityRenderer.java:1177) [EntityRenderer.class:?]
at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:1207) [Minecraft.class:?]
at net.minecraft.client.Minecraft.run(Minecraft.java:441) [Minecraft.class:?]
at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?]
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_161]
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_161]
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_161]
at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_161]
at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?]
at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?]
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_161]
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_161]
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_161]
at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_161]
at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?]
at GradleStart.main(GradleStart.java:25) [start/:?] -
1 hour ago, DiamondMiner88 said:
First of all im using Idea, and i already found a method so im testing with it.
Idea still shows you all the methods, and maybe try the method named getItem().
-
I have a TileEntity and a GUIContainer and a Container. WHen I right click on the Block, the GUI opens, and there is no background.
Code:
public class BWGui extends GuiContainer { private final int BUTTON_X = 20; private final int BUTTON_Y = 20; private static final ResourceLocation GUI_BACKGROUND = new ResourceLocation("enhancedbows", "textures/gui/workbench_gui.png"); private static final ResourceLocation GUI_WIDGETS = new ResourceLocation("enhancedbows", "textures/gui/workbench_widgets.png"); BWContainer container; public BWGui(Container inventorySlotsIn) { super(inventorySlotsIn); container = (BWContainer) inventorySlotsIn; } @Override public void initGui() { super.initGui(); buttonList.clear(); int id = 1; for(int i = 0; i > 1; i++) { buttonList.add(new GuiButtonImage(id, BUTTON_X*((id-1)/8), BUTTON_Y*((id-1)%8), 64, 64, 64*((id-1)/8), 64*((id-1)%8), 0, GUI_WIDGETS)); id += 1; } container.updateType(); } @Override protected void actionPerformed(GuiButton button) { BowTypes type = null; for(BowTypes type1 : BowTypes.values()) if(type1.ordinal() == button.id) type = type1; container.type = type; } @Override protected void drawGuiContainerBackgroundLayer(float partialTicks, int mouseX, int mouseY) { drawDefaultBackground(); Minecraft.getMinecraft().getTextureManager().bindTexture(GUI_BACKGROUND); this.drawTexturedModalRect(Minecraft.getMinecraft().displayWidth-256, 0f,0, 0, 256, 256); } @Override protected void drawGuiContainerForegroundLayer(int mouseX, int mouseY) { } }
The background is grayed out, the inventory works, but apparently it can't find my background texture.
Never mind, found my problem. Thanks for the help everyone. (It was the pack.mcmeta I think)
File layout:
src\main\resources\assets\enhancedbows\textures\gui\workbench_gui.png
Also the button (A GuiButtonImage) doesn't draw, I have no idea why.
-
I have no idea what's wrong, help is appreciated (I can't respond rn but I will tomorrow, also no errors)
-
Updated my code, when I place the block it shows the missing block model texture, when I refresh it shows the model with the missing texture texture.
-
16 minutes ago, Draco18s said:
An array of 1 is not the same as an object. Yes you do need them.
Well it worked with no error so forgive my ignorance.
-
BLockState code:
@Override @MethodsReturnNonnullByDefault public BlockStateContainer createBlockState() { CrystalColor color = new CrystalColor(CrystalColor.colors[rand.nextInt(5)]); BlockStateContainer.Builder container = new BlockStateContainer.Builder(this).add(color); return container.build(); } @Override public void onBlockPlacedBy(World worldIn, BlockPos pos, IBlockState state, EntityLivingBase placer, ItemStack stack) { worldIn.setBlockState(pos, createBlockState().getBaseState()); worldIn.getBlockState(pos).getProperties().forEach((prop, value) -> System.out.println(prop.getName() + ", " + value + ", " + value.toString())); System.out.println("test"); }
Test gets outprinted twice. Also no properties are outprinted of course.
Why does it get outprinted twice and what am I doing wrong with my properties?
I am supposed to use a property map?
Also, I don't really need the brackets cause it is just one item.
-
45 minutes ago, Draco18s said:
If you're getting an error, then it's wrong.
Your code:
"normal": { "model": "crystalweapons:crystalore" }
What's expected:
"normal": [{ "model": "crystalweapons:crystalore" }]
Fixed it, but I don't get an error for that so, idk what's wrong
Help with Slot Texture
in Modder Support
Posted
5 hour bump.