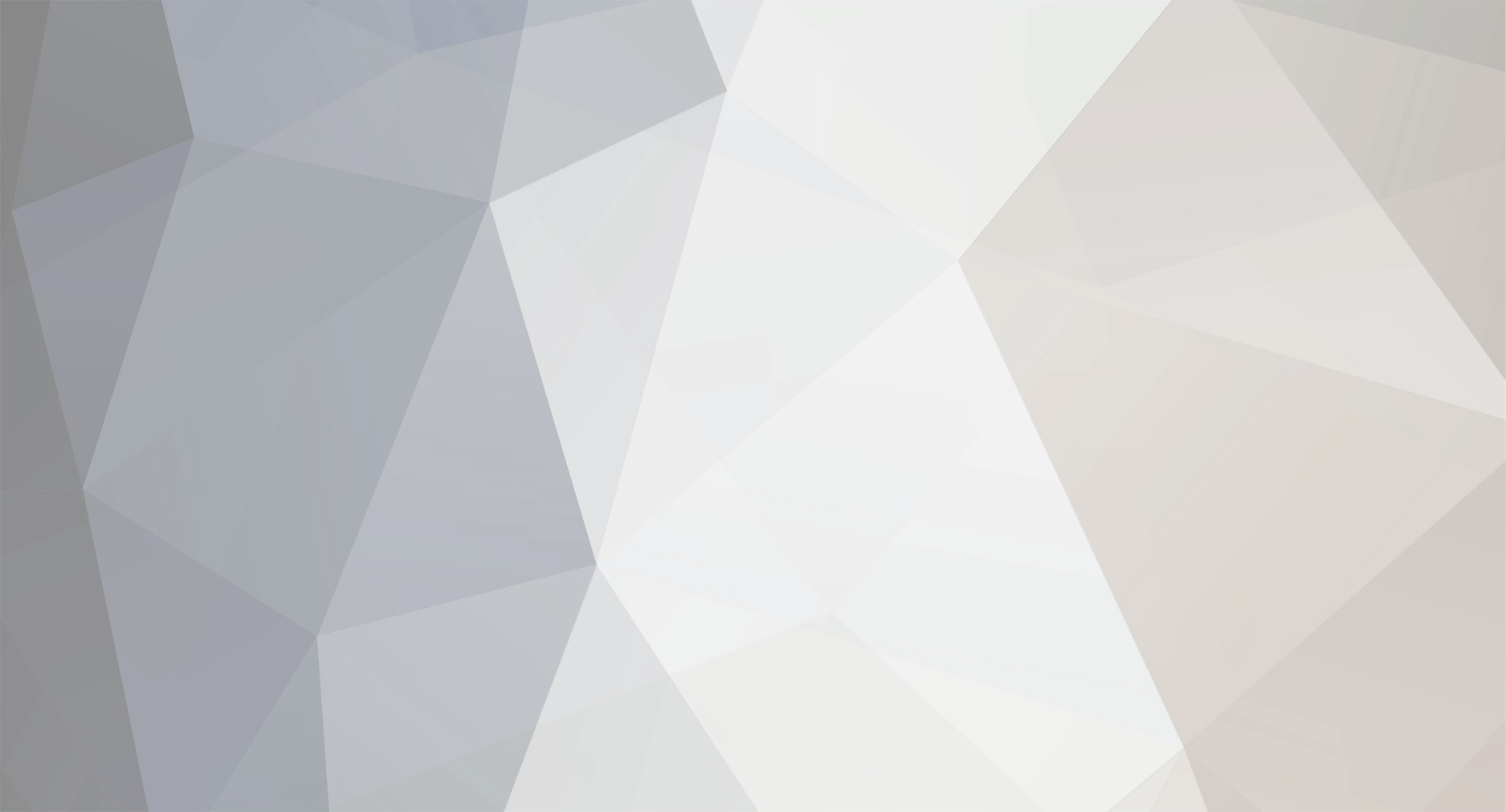
Differentiation
Members-
Posts
606 -
Joined
-
Last visited
-
Days Won
1
Everything posted by Differentiation
-
[1.10.2] How to set a projectile texture?
Differentiation replied to Differentiation's topic in Modder Support
So I do initRenderers() in the CommonProxy as well? -
[1.10.2] How to set a projectile texture?
Differentiation replied to Differentiation's topic in Modder Support
Isn't this it? I'm not too sure, that's why I kindly ask for a bit of help -
[1.10.2] How to set a projectile texture?
Differentiation replied to Differentiation's topic in Modder Support
Main class (MyCustomMod.java): package me.mycustommod; import me.mycustommod.creativetabs.TabDinocraftBlocks; import me.mycustommod.creativetabs.TabDinocraftItems; import me.mycustommod.entity.RenderVineBall; import me.mycustommod.event.DinocraftFunctionEvents; import me.mycustommod.event.DinocraftItemEvents; import me.mycustommod.handlers.DinocraftSoundEvents; import me.mycustommod.handlers.RecipeHandler; import me.mycustommod.init.DinocraftArmour; import me.mycustommod.init.DinocraftBlocks; import me.mycustommod.init.DinocraftEntities; import me.mycustommod.init.DinocraftItems; import me.mycustommod.init.DinocraftTools; import me.mycustommod.network.NetworkHandler; import me.mycustommod.proxy.CommonProxy; import net.minecraft.creativetab.CreativeTabs; import net.minecraftforge.common.MinecraftForge; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.Mod.EventHandler; import net.minecraftforge.fml.common.SidedProxy; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; @Mod(modid = "dinocraft", name = Reference.NAME, version = Reference.VERSION) public class MyCustomMod { public static final CreativeTabs BLOCKS = new TabDinocraftBlocks(); public static final CreativeTabs ITEMS = new TabDinocraftItems(); me.mycustommod.event.EventHandler eventHandler = new me.mycustommod.event.EventHandler(); @Mod.Instance(Reference.MODID) public static MyCustomMod instance; @SidedProxy(serverSide = Reference.SERVER_PROXY_CLASS, clientSide = Reference.CLIENT_PROXY_CLASS) public static CommonProxy proxy; @EventHandler public void preInit (FMLPreInitializationEvent event) { DinocraftItems.register(); DinocraftBlocks.register(); DinocraftTools.register(); DinocraftArmour.register(); DinocraftEntities.init(); proxy.registerRenders(); } @EventHandler public void init(FMLInitializationEvent event) { proxy.init(); RecipeHandler.registerCraftingRecipes(); RecipeHandler.registerFurnaceRecipes(); eventHandler.registerEvents(); DinocraftSoundEvents.init(); NetworkHandler.init(); } @EventHandler public void postInit(FMLPostInitializationEvent event) { MinecraftForge.EVENT_BUS.register(new DinocraftItemEvents()); MinecraftForge.EVENT_BUS.register(new DinocraftFunctionEvents()); } } CommonProxy Class: package me.mycustommod.proxy; import me.mycustommod.entity.EntityVineBall; import me.mycustommod.worldgen.OreGen; import net.minecraft.entity.Entity; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.world.World; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; import net.minecraftforge.fml.common.network.simpleimpl.MessageContext; import net.minecraftforge.fml.common.registry.GameRegistry; public class CommonProxy implements IProxy { @Override public void preInit(FMLPreInitializationEvent event) {} @Override public void init(FMLInitializationEvent event) {} @Override public void postInit(FMLPostInitializationEvent event) {} public void init() { GameRegistry.registerWorldGenerator(new OreGen(), 0); } public void registerRenders() { } public EntityPlayer getPlayer() { return null; } public World getClientWorld() { return null; } } IProxy Interface: package me.mycustommod.proxy; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; public interface IProxy { void preInit(FMLPreInitializationEvent event); void init(FMLInitializationEvent event); void postInit(FMLPostInitializationEvent event); } That's about it -
[1.10.2] How to set a projectile texture?
Differentiation replied to Differentiation's topic in Modder Support
Of course I called it. In the CommonProxy... -
[1.10.2] How to set a projectile texture?
Differentiation replied to Differentiation's topic in Modder Support
So I'm a bit confused, what is it that I'm supposed to add or fix? -
[1.10.2] How to set a projectile texture?
Differentiation replied to Differentiation's topic in Modder Support
Oh in my Proxy! I did, I believe. -
[1.10.2] How to set a projectile texture?
Differentiation replied to Differentiation's topic in Modder Support
I don't have access to it at the moment, that's why I said the only things in the preInit() event is DinocraftEntities#init(); and item init and block init, etc. -
[1.10.2] How to set a projectile texture?
Differentiation replied to Differentiation's topic in Modder Support
No, I meant the only thing having to do with the projectile there is DinocraftEntities#init(); which is present in the FMLPreInitializationEvent. Yes, I do have FMLPreInitializationEvent, FMLInitializationEvent, and FMLPostInitializationEvent. -
[1.10.2] How to set a projectile texture?
Differentiation replied to Differentiation's topic in Modder Support
The only significant thing there is DinocraftEntities#init(); Maybe it's with the texture? package me.mycustommod.entity; import me.mycustommod.Reference; import net.minecraft.client.Minecraft; import net.minecraft.client.renderer.entity.RenderEntity; import net.minecraft.client.renderer.entity.RenderManager; import net.minecraft.entity.Entity; import net.minecraft.util.ResourceLocation; import net.minecraftforge.fml.client.registry.RenderingRegistry; public class RenderVineBall extends RenderEntity { public RenderVineBall(RenderManager r) { super(r); } public static final ResourceLocation VINE_BALL_TEXTURE = new ResourceLocation(Reference.MODID + ":textures/items/vine_ball.png"); protected ResourceLocation getEntityTexture(EntityVineBall e) { return VINE_BALL_TEXTURE; } protected ResourceLocation getEntityTexture(Entity e) { return this.getEntityTexture((EntityVineBall)e); } } Should change it to this?: public class RenderVineBall extends Render<EntityVineBall> { public static final ResourceLocation VINE_BALL_TEXTURE = new ResourceLocation(Reference.MODID, "textures/items/vine_ball.png"); public RenderVineBall(RenderManager r) { super(r); } @Override protected ResourceLocation getEntityTexture(EntityVineBall e) { return VINE_BALL_TEXTURE; } } -
[1.12] Rendering model onto player
Differentiation replied to abused_master's topic in Modder Support
Maybe you can look into the Morph mod classes and see how they did it? -
[1.10.2] How to set a projectile texture?
Differentiation replied to Differentiation's topic in Modder Support
Please help -
Hey, so I made a projectile, similar to the Botania one called Vine Ball, but edited the gravity and effects of it, but I just can't get the item texture to show up. Whenever I throw the Vine Ball, I see a white square as the entity and it REALLY bothers me. I've tried to solve this multiple times but none are working. Please help. Classes: ClientProxy.java package me.mycustommod.proxy; import org.apache.logging.log4j.core.helpers.Constants; import me.mycustommod.entity.EntityVineBall; import me.mycustommod.entity.RenderVineBall; import me.mycustommod.init.DinocraftArmour; import me.mycustommod.init.DinocraftBlocks; import me.mycustommod.init.DinocraftItems; import me.mycustommod.init.DinocraftTools; import net.minecraft.client.Minecraft; import net.minecraft.client.multiplayer.PlayerControllerMP; import net.minecraft.client.network.NetHandlerPlayClient; import net.minecraft.client.renderer.entity.RenderSnowball; import net.minecraft.entity.Entity; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.world.GameType; import net.minecraft.world.World; import net.minecraftforge.fml.client.FMLClientHandler; import net.minecraftforge.fml.client.registry.RenderingRegistry; import net.minecraftforge.fml.common.Mod.EventHandler; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; import net.minecraftforge.fml.relauncher.ReflectionHelper; import scala.tools.nsc.doc.model.PrivateInInstance; public class ClientProxy extends CommonProxy { @Override public void preInit(FMLPreInitializationEvent event) { initRenderers(); } private void initRenderers() { RenderingRegistry.registerEntityRenderingHandler(EntityVineBall.class, renderManager -> new RenderSnowball(renderManager, DinocraftItems.VINE_BALL, Minecraft.getMinecraft().getRenderItem())); } @Override public void registerRenders() { DinocraftItems.registerRenders(); DinocraftBlocks.registerRenders(); DinocraftTools.registerRenders(); DinocraftArmour.registerRenders(); } @Override public EntityPlayer getPlayer() { return FMLClientHandler.instance().getClient().thePlayer; } @Override public World getClientWorld() { return FMLClientHandler.instance().getClient().theWorld; } } EntityVineBall.java package me.mycustommod.entity; import java.util.Map; import javax.annotation.Nonnull; import com.google.common.collect.ImmutableMap; import me.mycustommod.Reference; import me.mycustommod.init.DinocraftBlocks; import me.mycustommod.init.DinocraftEntities; import me.mycustommod.init.DinocraftItems; import net.minecraft.block.Block; import net.minecraft.block.BlockVine; import net.minecraft.block.properties.PropertyBool; import net.minecraft.block.state.IBlockState; import net.minecraft.client.entity.EntityPlayerSP; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.monster.EntityBlaze; import net.minecraft.entity.monster.EntityMob; import net.minecraft.entity.projectile.EntityThrowable; import net.minecraft.init.Blocks; import net.minecraft.item.Item; import net.minecraft.network.datasync.DataParameter; import net.minecraft.network.datasync.DataSerializers; import net.minecraft.network.datasync.EntityDataManager; import net.minecraft.potion.Potion; import net.minecraft.potion.PotionEffect; import net.minecraft.util.DamageSource; import net.minecraft.util.EnumFacing; import net.minecraft.util.EnumParticleTypes; import net.minecraft.util.ResourceLocation; import net.minecraft.util.datafix.DataFixer; import net.minecraft.util.math.BlockPos; import net.minecraft.util.math.RayTraceResult; import net.minecraft.world.World; import net.minecraftforge.fml.common.registry.EntityRegistry; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; public class EntityVineBall extends EntityThrowable { private static final DataParameter<Float> GRAVITY = EntityDataManager.createKey(EntityVineBall.class, DataSerializers.FLOAT); private static final Map<EnumFacing, PropertyBool> propMap = ImmutableMap.of(EnumFacing.NORTH, BlockVine.NORTH, EnumFacing.SOUTH, BlockVine.SOUTH, EnumFacing.WEST, BlockVine.WEST, EnumFacing.EAST, BlockVine.EAST); public EntityVineBall(World worldIn) { super(worldIn); } protected ResourceLocation getEntityTexture(EntityVineBall e) { return RenderVineBall.VINE_BALL_TEXTURE; } protected ResourceLocation getEntityTexture(Entity e) { return this.getEntityTexture((EntityVineBall)e); } public EntityVineBall(World worldIn, EntityLivingBase throwerIn) { super(worldIn, throwerIn); } public EntityVineBall(World worldIn, double x, double y, double z) { super(worldIn, x, y, z); } public EntityVineBall(EntityLivingBase thrower, boolean gravity) { super(thrower.worldObj, thrower); dataManager.set(GRAVITY, gravity ? 0.1F : 0F); } @Override protected void entityInit() { super.entityInit(); dataManager.register(GRAVITY, 0F); } @SideOnly(Side.CLIENT) @Override public void handleStatusUpdate(byte id) { if(id == 3) { for(int j = 0; j < 16; j++) { worldObj.spawnParticle(EnumParticleTypes.ITEM_CRACK, posX, posY, posZ, Math.random() * 0.2 - 0.1, Math.random() * 0.25, Math.random() * 0.2 - 0.1, Item.getIdFromItem(DinocraftItems.VINE_BALL)); } } } @Override protected void onImpact(RayTraceResult var1) { if (var1.entityHit != null) { int i = 0; var1.entityHit.attackEntityFrom(DamageSource.causeThrownDamage(this, this.getThrower()), (float)i); if(var1.entityHit instanceof EntityLivingBase) { EntityLivingBase e = (EntityLivingBase) var1.entityHit; e.addPotionEffect(new PotionEffect(Potion.getPotionById(2), 200, 0, false, false)); e.addPotionEffect(new PotionEffect(Potion.getPotionById(15), 200, 0, false, false)); e.addPotionEffect(new PotionEffect(Potion.getPotionById(16), 200, 0, false, false)); } if(!worldObj.isRemote) { if(var1 != null) { EnumFacing dir = var1.sideHit; if(dir != null && dir.getAxis() != EnumFacing.Axis.Y) { BlockPos pos = var1.getBlockPos().offset(dir); while(pos.getY() > 0) { IBlockState state = worldObj.getBlockState(pos); Block block = state.getBlock(); if(block.isAir(state, worldObj, pos)) { IBlockState stateSet = Blocks.VINE.getDefaultState().withProperty(propMap.get(dir.getOpposite()), true); worldObj.setBlockState(pos, stateSet, 1 | 2); worldObj.playEvent(2001, pos, Block.getStateId(stateSet)); pos = pos.down(); } else break; } } } this.worldObj.setEntityState(this, (byte)3); this.setDead(); } } @Override protected float getGravityVelocity() { return dataManager.get(GRAVITY); } } ItemVineBall.java package me.mycustommod.items; import me.mycustommod.Reference; import me.mycustommod.entity.EntityVineBall; import net.minecraft.client.renderer.block.model.ModelResourceLocation; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.SoundEvents; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.stats.StatList; import net.minecraft.util.ActionResult; import net.minecraft.util.EnumActionResult; import net.minecraft.util.EnumHand; import net.minecraft.util.ResourceLocation; import net.minecraft.util.SoundCategory; import net.minecraft.world.World; import net.minecraftforge.client.model.ModelLoader; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; public class ItemVineBall extends Item implements IModelRegister { public ItemVineBall(String unlocalizedName) { this.setUnlocalizedName(unlocalizedName); this.setRegistryName(new ResourceLocation(Reference.MODID, unlocalizedName)); } @SideOnly(Side.CLIENT) @Override public void registerModels() { ModelLoader.setCustomModelResourceLocation(this, 0, new ModelResourceLocation(Reference.MODID + ":textures/items/vine_ball", "inventory")); } public ActionResult<ItemStack> onItemRightClick(ItemStack itemStackIn, World world, EntityPlayer playerIn, EnumHand hand) { if (!playerIn.capabilities.isCreativeMode) { --itemStackIn.stackSize; } world.playSound((EntityPlayer)null, playerIn.posX, playerIn.posY, playerIn.posZ, SoundEvents.ENTITY_SNOWBALL_THROW, SoundCategory.NEUTRAL, 0.5F, 0.4F / (itemRand.nextFloat() * 0.4F + 0.8F)); if (!world.isRemote) { EntityVineBall ball = new EntityVineBall(playerIn, true); ball.setHeadingFromThrower(playerIn, playerIn.rotationPitch, playerIn.rotationYaw, 0.0F, 1.5F, 1.0F); world.spawnEntityInWorld(ball); } playerIn.addStat(StatList.getObjectUseStats(this)); return new ActionResult(EnumActionResult.SUCCESS, itemStackIn); } } RenderVineBall.java package me.mycustommod.entity; import me.mycustommod.Reference; import net.minecraft.client.Minecraft; import net.minecraft.client.renderer.entity.RenderEntity; import net.minecraft.client.renderer.entity.RenderManager; import net.minecraft.entity.Entity; import net.minecraft.util.ResourceLocation; import net.minecraftforge.fml.client.registry.RenderingRegistry; public class RenderVineBall extends RenderEntity { public RenderVineBall(RenderManager r) { super(r); } public static final ResourceLocation VINE_BALL_TEXTURE = new ResourceLocation(Reference.MODID + ":textures/items/vine_ball.png"); protected ResourceLocation getEntityTexture(EntityVineBall e) { return VINE_BALL_TEXTURE; } protected ResourceLocation getEntityTexture(Entity e) { return this.getEntityTexture((EntityVineBall)e); } } DinocraftEntities.java package me.mycustommod.init; import me.mycustommod.MyCustomMod; import me.mycustommod.Reference; import me.mycustommod.entity.EntityVineBall; import net.minecraft.util.ResourceLocation; import net.minecraftforge.fml.common.registry.EntityRegistry; public final class DinocraftEntities { public static void init() { int id = 0; EntityRegistry.registerModEntity(EntityVineBall.class, "vine_ball", id++, MyCustomMod.instance, 64, 10, true); } } I initialized DinocraftEntities in MyCustomMod.java* Please help with this. Thanks!
-
[1.10.2] My projectile does not render in-game. Help
Differentiation replied to Differentiation's topic in Modder Support
Never mind, I figured it out buy watching a quick little rendering tutorial Thanks though! -
[1.10.2] Detecting a Key Press server-side?
Differentiation replied to Differentiation's topic in Modder Support
So I should send a packet to the server saying the player jumped and then execute the events in the Armor Tick Event? -
[1.10.2] Detecting a Key Press server-side?
Differentiation replied to Differentiation's topic in Modder Support
I thought I needed to send the server packets for the particles, etc. but turns out I need to make sure the server knows the player jumped Thank you so much! -
Hey, so I have this class that allows the player to Double Jump, however, I want it to spawn particles. So I created a packet to send particles to all clients but that doesn't work I'm not too sure what I'm doing wrong here. Please help. Class: [SOLVED] Leave feedback below. Thanks!
-
I tried this, but it didn't work @SubscribeEvent (priority = EventPriority.HIGHEST) //Tuskerer's Healing event public void itemPickup(ItemPickupEvent e) { EntityPlayer p = e.player; if (e.pickedUp.getEntityItem().getItem().equals(DinocraftItems.TUSKERERS_HEART)) { p.heal(e.pickedUp.getEntityItem().stackSize); p.inventory.clearMatchingItems(DinocraftItems.TUSKERERS_HEART, 0, 37 * 64, null); } } Am I doing something wrong? Please help. Thanks!
-
Hey, so I have this item, and I want to make it heal the player 1/2 a heart when picked up. However, when the item stacks to a number higher than one, it still heals only half a heart instead of multiple hearts depending on the amount of items in the stack. If you know what I mean... My question is: how do I get the amount of items in a single stack? My code: @SubscribeEvent(priority = EventPriority.HIGHEST) //Tuskerer's Healing event public void itemPickup(ItemPickupEvent e) { EntityPlayer p = e.player; if (e.pickedUp.getEntityItem().getItem() == DinocraftItems.TUSKERERS_HEART) { p.heal(1); //here I need to somehow get the amount of items per stack p.inventory.clearMatchingItems(DinocraftItems.TUSKERERS_HEART, 0, 37 * 64, null); } } Please leave suggestions below. Thanks!
-
[1.10.2] Item durability bug??? Please help.
Differentiation replied to Differentiation's topic in Modder Support
I'll try to do that, thanks! -
Hey, so I have an armour item called Leafy Boots, which allow the player to double jump when hitting space twice in the air. Now, I wanted to make it so that the item gets damaged, or loses durability every time the player double jumps with it. However, when I wrote the script then tested it out, the item got damaged, but when the game updated, the boots remained with full durability. I'm not sure if this is a problem with my code or not. Please help. Item Class: [SOLVED] If you have any suggestions, please leave them down below. Thank you! ~Differentiation