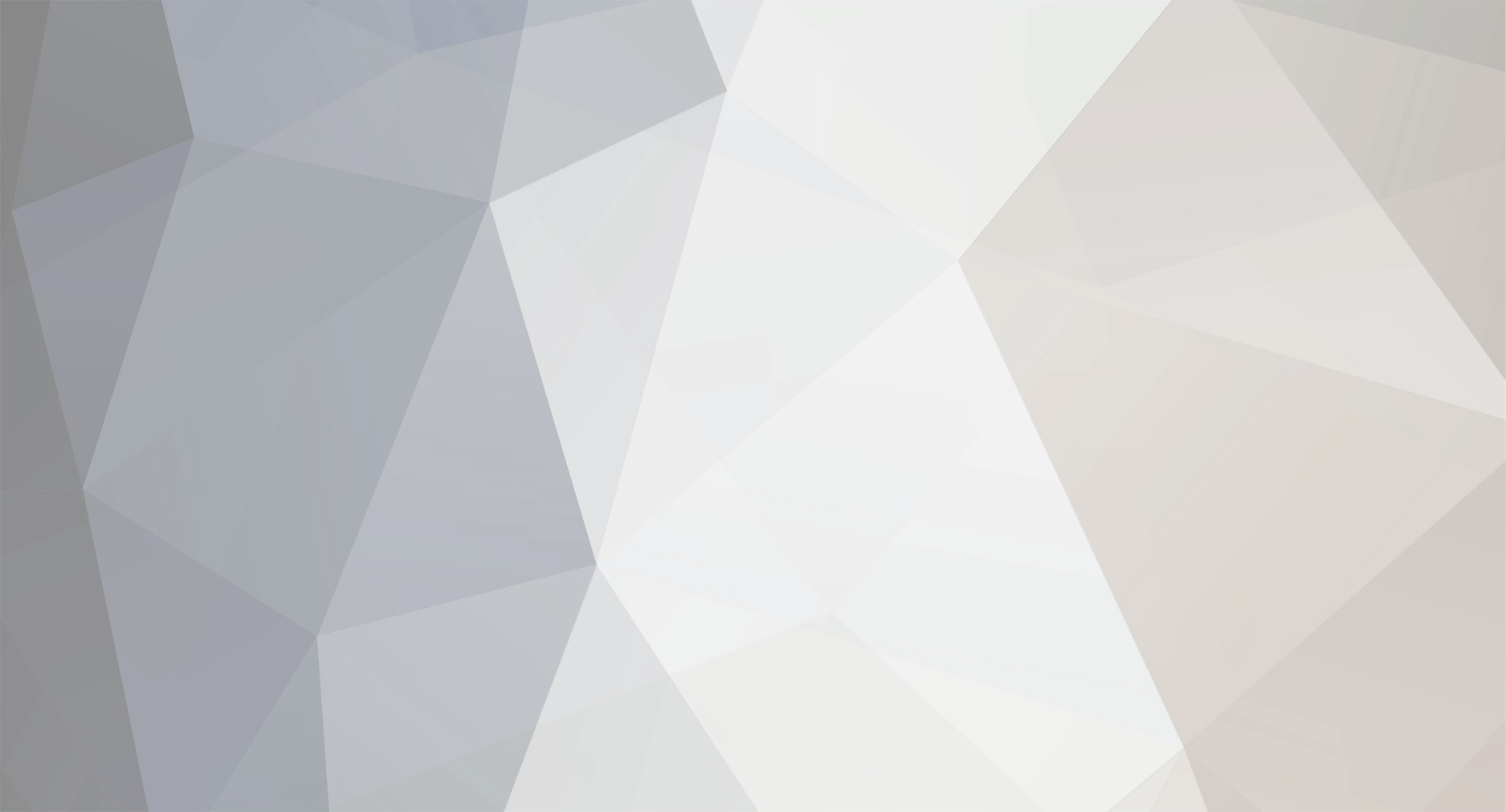
InterdimensionalCat
Members-
Posts
44 -
Joined
-
Last visited
Everything posted by InterdimensionalCat
-
after looking through the debugging logs after running in debug mode, I didn't find anything about getItemAttributeModifiers, the closest thing I found was this line of text: [23:44:32] [main/DEBUG] [FML/]: Unable to lookup btm:copperhoe for public static com.benthom123.test.items.CopperHoe com.benthom123.test.ModItems.copperhoe. This means the object wasn't registered. It's likely just mod options. this same line occurred for all of my items. Other than that I did not see anything pertaining to the item.
-
Ok, that's fine, I am more concerned about changing the attack speed. So far I have tried giving this.speed a finite float value and converted it to a double like this: public CopperHoe(String registryName, ToolMaterial material) { super(material); this.repairItem = ModItems.copperingot; setRegistryName(registryName); setUnlocalizedName(modClass.MODID + "." + registryName); this.setCreativeTab(ModItems.extraTools); this.speed = -3.8F; ... multimap.put(SharedMonsterAttributes.ATTACK_SPEED.getName(), new AttributeModifier(ATTACK_SPEED_MODIFIER, "Weapon modifier", (double)(this.speed) , 0)); I have also tried just putting in a double value like so: multimap.put(SharedMonsterAttributes.ATTACK_SPEED.getName(), new AttributeModifier(ATTACK_SPEED_MODIFIER, "Weapon modifier", -3.8D , 0)); For both of these the Item will load, but the attack speed is not modified.
-
So far this is what I have, and after trying for a long time I still cannot figure out what is wrong. My Item loads properly but has the default material attack speed and damage. Is there anything I need to do in other classes to make this work right (outside of the normal Item registering)? CopperHoe: package com.benthom123.test.items; import java.util.Collections; import com.benthom123.test.ModItems; import com.benthom123.test.modClass; import com.google.common.collect.Multimap; import net.minecraft.block.Block; import net.minecraft.block.BlockDirt; import net.minecraft.block.material.Material; import net.minecraft.block.state.IBlockState; import net.minecraft.client.renderer.block.model.ModelResourceLocation; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.entity.SharedMonsterAttributes; import net.minecraft.entity.ai.attributes.AttributeModifier; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.Blocks; import net.minecraft.init.SoundEvents; import net.minecraft.inventory.EntityEquipmentSlot; import net.minecraft.item.Item; import net.minecraft.item.Item.ToolMaterial; import net.minecraft.item.ItemHoe; import net.minecraft.item.ItemStack; import net.minecraft.item.ItemTool; import net.minecraft.util.EnumActionResult; import net.minecraft.util.EnumFacing; import net.minecraft.util.EnumHand; import net.minecraft.util.SoundCategory; import net.minecraft.util.math.BlockPos; import net.minecraft.world.World; import net.minecraftforge.client.model.ModelLoader; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.SharedMonsterAttributes; public class CopperHoe extends ItemHoe { private final float speed; private final float attackDamage; public Item repairItem; public CopperHoe(String registryName, ToolMaterial material) { super(material); this.repairItem = ModItems.copperingot; setRegistryName(registryName); setUnlocalizedName(modClass.MODID + "." + registryName); this.setCreativeTab(ModItems.extraTools); this.speed = material.getAttackDamage() + 2.0F; this.attackDamage = 3.0F + material.getAttackDamage(); } @SideOnly(Side.CLIENT) public void initModel() { ModelLoader.setCustomModelResourceLocation(this, 0, new ModelResourceLocation(getRegistryName(), "inventory")); } @Override public boolean getIsRepairable(ItemStack toRepair, ItemStack repair) { return repair.getItem() == ModItems.copperingot ? true : super.getIsRepairable(toRepair, repair); } @Override public Multimap<String, AttributeModifier> getAttributeModifiers(EntityEquipmentSlot slot, ItemStack stack) { Multimap<String, AttributeModifier> multimap = super.getAttributeModifiers(slot, stack); if (slot == EntityEquipmentSlot.MAINHAND) { multimap.put(SharedMonsterAttributes.ATTACK_DAMAGE.getName(), new AttributeModifier(ATTACK_DAMAGE_MODIFIER, "Weapon modifier", (double)(this.attackDamage), 0)); multimap.put(SharedMonsterAttributes.ATTACK_SPEED.getName(), new AttributeModifier(ATTACK_SPEED_MODIFIER, "Weapon modifier", (double)(this.speed) , 0)); } return multimap; } }
-
i have tried this: @Override public Multimap<String, AttributeModifier> getAttributeModifiers(EntityEquipmentSlot slot, ItemStack stack) { Multimap<String, AttributeModifier> multimap = super.getAttributeModifiers(slot, stack); if (slot == EntityEquipmentSlot.MAINHAND) { multimap.put(SharedMonsterAttributes.ATTACK_DAMAGE.getName(), new AttributeModifier(ATTACK_DAMAGE_MODIFIER, "Weapon modifier", 0.0D, 0)); multimap.put(SharedMonsterAttributes.ATTACK_SPEED.getName(), new AttributeModifier(ATTACK_SPEED_MODIFIER, "Weapon modifier", (double)(this.speed), 0)); } return multimap; } } as well as this: @Override public Multimap<String, AttributeModifier> getAttributeModifiers(EntityEquipmentSlot slot, ItemStack stack) { return this.getItemAttributeModifiers(slot); } @Override public Multimap<String, AttributeModifier> getItemAttributeModifiers(EntityEquipmentSlot slot) { Multimap<String, AttributeModifier> multimap = super.getItemAttributeModifiers(slot); if (slot == EntityEquipmentSlot.MAINHAND) { multimap.put(SharedMonsterAttributes.ATTACK_DAMAGE.getName(), new AttributeModifier(ATTACK_DAMAGE_MODIFIER, "Weapon modifier", 0.0D, 0)); multimap.put(SharedMonsterAttributes.ATTACK_SPEED.getName(), new AttributeModifier(ATTACK_SPEED_MODIFIER, "Weapon modifier", (double)(this.speed), 0)); } return multimap; } } I am sure the answer is obvious and I am just being dumb.
-
Hi, thanks for the info. I am using eclipse and yes it is flagging that method as depreciated. I have no idea how I missed that before, or I wouldn't have tried to use it. So from what I understand I need to use an ItemStack sensitive version of the method that looks like this: public Multimap<String, AttributeModifier> getAttributeModifiers(EntityEquipmentSlot slot, ItemStack stack) { return this.getItemAttributeModifiers(slot); } However I am having trouble figuring out how to modify the original line of code I have that uses the depreciated method, when i used this getattributemodifiers method in place of the old one It will not accept the parameters. Sorry if this is kind of a dumb question but I am still a little new to modding Minecraft. Also would this way work similarly for the other tools? (Other than Axes, I already know how to change the attack speed for those).
-
So far I have been extending ItemTool whenever I wanted to create an Item with a nonstandard attack speed (other than axes). However while creating the item copperhoe I found a better way to adjust the attack speed while still importing the class ItemHoe. However after overriding the getItemAttributeModifiers method in ItemHoe and replacing the attack speed value with my desired attack speed, the Item still has the speed of a normal hoe. Here is my class CopperHoe: package com.benthom123.test.items; import java.util.Collections; import com.benthom123.test.ModItems; import com.benthom123.test.modClass; import com.google.common.collect.Multimap; import net.minecraft.block.Block; import net.minecraft.block.BlockDirt; import net.minecraft.block.material.Material; import net.minecraft.block.state.IBlockState; import net.minecraft.client.renderer.block.model.ModelResourceLocation; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.entity.SharedMonsterAttributes; import net.minecraft.entity.ai.attributes.AttributeModifier; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.Blocks; import net.minecraft.init.SoundEvents; import net.minecraft.inventory.EntityEquipmentSlot; import net.minecraft.item.Item; import net.minecraft.item.Item.ToolMaterial; import net.minecraft.item.ItemHoe; import net.minecraft.item.ItemPickaxe; import net.minecraft.item.ItemStack; import net.minecraft.item.ItemTool; import net.minecraft.util.EnumActionResult; import net.minecraft.util.EnumFacing; import net.minecraft.util.EnumHand; import net.minecraft.util.SoundCategory; import net.minecraft.util.math.BlockPos; import net.minecraft.world.World; import net.minecraftforge.client.model.ModelLoader; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; public class CopperHoe extends ItemHoe { public Item repairItem; public CopperHoe(String registryName, ToolMaterial material) { super(material); this.repairItem = ModItems.copperingot; setRegistryName(registryName); setUnlocalizedName(modClass.MODID + "." + registryName); this.setCreativeTab(ModItems.extraTools); } @SideOnly(Side.CLIENT) public void initModel() { ModelLoader.setCustomModelResourceLocation(this, 0, new ModelResourceLocation(getRegistryName(), "inventory")); } @Override public boolean getIsRepairable(ItemStack toRepair, ItemStack repair) { return repair.getItem() == ModItems.copperingot ? true : super.getIsRepairable(toRepair, repair); } @Override public Multimap<String, AttributeModifier> getItemAttributeModifiers(EntityEquipmentSlot equipmentSlot) { Multimap<String, AttributeModifier> multimap = super.getItemAttributeModifiers(equipmentSlot); if (equipmentSlot == EntityEquipmentSlot.MAINHAND) { multimap.put(SharedMonsterAttributes.ATTACK_DAMAGE.getName(), new AttributeModifier(ATTACK_DAMAGE_MODIFIER, "Weapon modifier", 0.0D, 0)); multimap.put(SharedMonsterAttributes.ATTACK_SPEED.getName(), new AttributeModifier(ATTACK_SPEED_MODIFIER, "Weapon modifier", -3.8D, 0)); } return multimap; } } If it is necessary to look at other classes here is my project on github: https://github.com/InterdimensionalCat/ModTest/tree/master/java/com/benthom123/test
-
[1.12.2] Modded Custom Block Drops Crash Game
InterdimensionalCat replied to InterdimensionalCat's topic in Modder Support
ok done, that's a whole lot cleaner now, thanks! -
[1.12.2] Modded Custom Block Drops Crash Game
InterdimensionalCat replied to InterdimensionalCat's topic in Modder Support
Thanks for the info, I found a fix that is working and allows me to assign more than 1 drop to the item. As for the @ObjectHolder stuff, I am not sure what way I should do it that is better, I tried putting everything under the same @ObjectHolder annotation but that crashed all of my textures and I didn't know how to fix that. Could you show me an example of what you mean? -
After attempting to set my block obsidianore to drop obsidianingot instead, when I was testing, destroying the block resulted in missing textures spawning, which would crash the game if I picked them up. After repeating this with the block dropping sticks, an Item already in minecraft, It worked perfectly. I think this means that the Item copperingot is not being registered before copperore, but I am not sure. Here is the CopperOre Class: https://github.com/InterdimensionalCat/ModTest/blob/master/java/com/benthom123/test/blocks/CopperOre.java Here is CommonProxy: https://github.com/InterdimensionalCat/ModTest/blob/master/java/com/benthom123/test/proxy/CommonProxy.java Here is modItems: https://github.com/InterdimensionalCat/ModTest/blob/master/java/com/benthom123/test/ModItems.java and here is the crash report: ---- Minecraft Crash Report ---- // Don't be sad. I'll do better next time, I promise! Time: 10/30/17 9:23 PM Description: Ticking player java.lang.ArrayIndexOutOfBoundsException: -1 at net.minecraft.stats.StatList.getObjectsPickedUpStats(StatList.java:123) at net.minecraft.entity.item.EntityItem.onCollideWithPlayer(EntityItem.java:449) at net.minecraft.entity.player.EntityPlayer.collideWithPlayer(EntityPlayer.java:662) at net.minecraft.entity.player.EntityPlayer.onLivingUpdate(EntityPlayer.java:633) at net.minecraft.entity.EntityLivingBase.onUpdate(EntityLivingBase.java:2392) at net.minecraft.entity.player.EntityPlayer.onUpdate(EntityPlayer.java:270) at net.minecraft.entity.player.EntityPlayerMP.onUpdateEntity(EntityPlayerMP.java:423) at net.minecraft.network.NetHandlerPlayServer.update(NetHandlerPlayServer.java:185) at net.minecraftforge.fml.common.network.handshake.NetworkDispatcher$1.update(NetworkDispatcher.java:212) at net.minecraft.network.NetworkManager.processReceivedPackets(NetworkManager.java:307) at net.minecraft.network.NetworkSystem.networkTick(NetworkSystem.java:196) at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:863) at net.minecraft.server.MinecraftServer.tick(MinecraftServer.java:741) at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:192) at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:590) at java.lang.Thread.run(Unknown Source) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Thread: Server thread Stacktrace: at net.minecraft.stats.StatList.getObjectsPickedUpStats(StatList.java:123) at net.minecraft.entity.item.EntityItem.onCollideWithPlayer(EntityItem.java:449) at net.minecraft.entity.player.EntityPlayer.collideWithPlayer(EntityPlayer.java:662) at net.minecraft.entity.player.EntityPlayer.onLivingUpdate(EntityPlayer.java:633) at net.minecraft.entity.EntityLivingBase.onUpdate(EntityLivingBase.java:2392) at net.minecraft.entity.player.EntityPlayer.onUpdate(EntityPlayer.java:270) -- Player being ticked -- Details: Entity Type: null (net.minecraft.entity.player.EntityPlayerMP) Entity ID: 194 Entity Name: Player750 Entity's Exact location: 180.51, 47.00, 57.51 Entity's Block location: World: (180,47,57), Chunk: (at 4,2,9 in 11,3; contains blocks 176,0,48 to 191,255,63), Region: (0,0; contains chunks 0,0 to 31,31, blocks 0,0,0 to 511,255,511) Entity's Momentum: 0.00, -0.08, 0.00 Entity's Passengers: [] Entity's Vehicle: ~~ERROR~~ NullPointerException: null Stacktrace: at net.minecraft.entity.player.EntityPlayerMP.onUpdateEntity(EntityPlayerMP.java:423) at net.minecraft.network.NetHandlerPlayServer.update(NetHandlerPlayServer.java:185) at net.minecraftforge.fml.common.network.handshake.NetworkDispatcher$1.update(NetworkDispatcher.java:212) at net.minecraft.network.NetworkManager.processReceivedPackets(NetworkManager.java:307) -- Ticking connection -- Details: Connection: net.minecraft.network.NetworkManager@6fbe3b33 Stacktrace: at net.minecraft.network.NetworkSystem.networkTick(NetworkSystem.java:196) at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:863) at net.minecraft.server.MinecraftServer.tick(MinecraftServer.java:741) at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:192) at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:590) at java.lang.Thread.run(Unknown Source) -- System Details -- Details: Minecraft Version: 1.12.2 Operating System: Windows 10 (amd64) version 10.0 Java Version: 1.8.0_151, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 732016696 bytes (698 MB) / 1037959168 bytes (989 MB) up to 1037959168 bytes (989 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M IntCache: cache: 0, tcache: 0, allocated: 13, tallocated: 95 FML: MCP 9.42 Powered by Forge 14.23.0.2515 5 mods loaded, 5 mods active States: 'U' = Unloaded 'L' = Loaded 'C' = Constructed 'H' = Pre-initialized 'I' = Initialized 'J' = Post-initialized 'A' = Available 'D' = Disabled 'E' = Errored | State | ID | Version | Source | Signature | |:--------- |:--------- |:------------ |:-------------------------------- |:--------- | | UCHIJAAAA | minecraft | 1.12.2 | minecraft.jar | None | | UCHIJAAAA | mcp | 9.42 | minecraft.jar | None | | UCHIJAAAA | FML | 8.0.99.99 | forgeSrc-1.12.2-14.23.0.2515.jar | None | | UCHIJAAAA | forge | 14.23.0.2515 | forgeSrc-1.12.2-14.23.0.2515.jar | None | | UCHIJAAAA | btm | 0.0.1 | bin | None | Loaded coremods (and transformers): GL info: ~~ERROR~~ RuntimeException: No OpenGL context found in the current thread. Profiler Position: N/A (disabled) Player Count: 1 / 8; [EntityPlayerMP['Player750'/194, l='New World', x=180.51, y=47.00, z=57.51]] Type: Integrated Server (map_client.txt) Is Modded: Definitely; Client brand changed to 'fml,forge'
-
I was successfully able to create a tool (deathscythe) that utilized the harvesting characteristics from both an axe and a pickaxe using setHarvestLevel(IE my tool can break stone and Wood as a pickaxe/axe would), however this would not work when I attempted to add sword harvesting functionality. I suspect this is because swords all harvest at the same level (and can only harvest cobwebs as far as I am aware). Is there a way to add this functionality? Here is the current version of deathscythe, if you need to see any other parts of my mod, I can provide them: package com.benthom123.test.items; import java.util.Collections; import java.util.Set; import com.benthom123.test.ModItems; import com.benthom123.test.modClass; import com.google.common.collect.ImmutableSet; import com.google.common.collect.Sets; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.block.state.IBlockState; import net.minecraft.client.renderer.block.model.ModelResourceLocation; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.Blocks; import net.minecraft.init.SoundEvents; import net.minecraft.item.ItemAxe; import net.minecraft.item.ItemPickaxe; import net.minecraft.item.ItemSword; import net.minecraft.item.ItemStack; import net.minecraft.item.ItemTool; import net.minecraft.world.World; import net.minecraft.item.Item; import net.minecraft.item.Item.ToolMaterial; import net.minecraftforge.client.model.ModelLoader; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; public class DeathScythe extends ItemTool { public Item repairItem; public static float attack_speed = -3.7F; public static float base_damage = 20.0F; public DeathScythe(String registryName, ToolMaterial material, Item repairItem) { super(base_damage, attack_speed, material, Collections.emptySet()); this.setHarvestLevel("pickaxe", material.getHarvestLevel()); this.setHarvestLevel("axe", material.getHarvestLevel()); this.repairItem = repairItem; setRegistryName(registryName); setUnlocalizedName(modClass.MODID + "." + registryName); this.setCreativeTab(ModItems.extraTools); } @Override public boolean canHarvestBlock(IBlockState state, ItemStack stack) { if (!(state.getMaterial() == Material.AIR) && !(state.getMaterial() == Material.BARRIER)) { return true; } else { return false; } } @Override public boolean getIsRepairable(ItemStack toRepair, ItemStack repair) { return repair.getItem() == ModItems.ObsidianIngot ? true : super.getIsRepairable(toRepair, repair); } @Override public boolean hitEntity(ItemStack itemStack, EntityLivingBase target, EntityLivingBase attacker) { // Only take one damage like a sword instead of 2 itemStack.damageItem(1, attacker); return true; } @SideOnly(Side.CLIENT) public void initModel() { ModelLoader.setCustomModelResourceLocation(this, 0, new ModelResourceLocation(getRegistryName(), "inventory")); } } EDIT: Nvm I figured out how to do it myself, if anyone comes a cross this wondering how to do it: Swords have an alternate getDestroySpeed method, I just overrided the getDestroySpeed method and replaced it with the one that swords have in the ItemSword class
-
[1.12.2] New Tool Crashing Game on Startup
InterdimensionalCat replied to InterdimensionalCat's topic in Modder Support
Thanks, it is loading without error now. From what I understand this means that axes have set possible attack speed and attack damage values. Is there a way to override these values when making an axe? (IE: an axe with even less attack speed than what is possible in the array) EDIT: nvm the constructor allows for that already and I didn't realize it -
For some reason, I am getting an ArrayIndexOutOfBounds exception from the item obsidianaxe when I load up the game. The other two tools I have added (obsidianpickaxe and obsidiansword) have no problems at all, and I used the same structure to create this item. Here is the GitHub repo: https://github.com/InterdimensionalCat/ModTest The classes effected are: ObsidianAxe: https://github.com/InterdimensionalCat/ModTest/blob/master/java/com/benthom123/test/items/ObsidianAxe.java ModItems: https://github.com/InterdimensionalCat/ModTest/blob/master/java/com/benthom123/test/ModItems.java and CommonProxy: https://github.com/InterdimensionalCat/ModTest/blob/master/java/com/benthom123/test/proxy/CommonProxy.java And here is the crash log: ---- Minecraft Crash Report ---- // Don't be sad. I'll do better next time, I promise! Time: 10/28/17 7:19 PM Description: Initializing game java.lang.ArrayIndexOutOfBoundsException: 5 at net.minecraft.item.ItemAxe.<init>(ItemAxe.java:19) at com.benthom123.test.items.ObsidianAxe.<init>(ObsidianAxe.java:16) at com.benthom123.test.proxy.CommonProxy.registerItems(CommonProxy.java:70) at net.minecraftforge.fml.common.eventhandler.ASMEventHandler_5_CommonProxy_registerItems_Register.invoke(.dynamic) at net.minecraftforge.fml.common.eventhandler.ASMEventHandler.invoke(ASMEventHandler.java:90) at net.minecraftforge.fml.common.eventhandler.EventBus$1.invoke(EventBus.java:143) at net.minecraftforge.fml.common.eventhandler.EventBus.post(EventBus.java:179) at net.minecraftforge.registries.GameData.fireRegistryEvents(GameData.java:741) at net.minecraftforge.fml.common.Loader.preinitializeMods(Loader.java:603) at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:266) at net.minecraft.client.Minecraft.init(Minecraft.java:513) at net.minecraft.client.Minecraft.run(Minecraft.java:421) at net.minecraft.client.main.Main.main(Main.java:118) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) at GradleStart.main(GradleStart.java:26) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Thread: Client thread Stacktrace: at net.minecraft.item.ItemAxe.<init>(ItemAxe.java:19) at com.benthom123.test.items.ObsidianAxe.<init>(ObsidianAxe.java:16) at com.benthom123.test.proxy.CommonProxy.registerItems(CommonProxy.java:70) at net.minecraftforge.fml.common.eventhandler.ASMEventHandler_5_CommonProxy_registerItems_Register.invoke(.dynamic) at net.minecraftforge.fml.common.eventhandler.ASMEventHandler.invoke(ASMEventHandler.java:90) at net.minecraftforge.fml.common.eventhandler.EventBus$1.invoke(EventBus.java:143) at net.minecraftforge.fml.common.eventhandler.EventBus.post(EventBus.java:179) at net.minecraftforge.registries.GameData.fireRegistryEvents(GameData.java:741) at net.minecraftforge.fml.common.Loader.preinitializeMods(Loader.java:603) at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:266) at net.minecraft.client.Minecraft.init(Minecraft.java:513) -- Initialization -- Details: Stacktrace: at net.minecraft.client.Minecraft.run(Minecraft.java:421) at net.minecraft.client.main.Main.main(Main.java:118) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) at GradleStart.main(GradleStart.java:26) -- System Details -- Details: Minecraft Version: 1.12.2 Operating System: Windows 10 (amd64) version 10.0 Java Version: 1.8.0_151, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 809018528 bytes (771 MB) / 1037959168 bytes (989 MB) up to 1037959168 bytes (989 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: MCP 9.42 Powered by Forge 14.23.0.2515 5 mods loaded, 5 mods active States: 'U' = Unloaded 'L' = Loaded 'C' = Constructed 'H' = Pre-initialized 'I' = Initialized 'J' = Post-initialized 'A' = Available 'D' = Disabled 'E' = Errored | State | ID | Version | Source | Signature | |:----- |:--------- |:------------ |:-------------------------------- |:--------- | | UCH | minecraft | 1.12.2 | minecraft.jar | None | | UCH | mcp | 9.42 | minecraft.jar | None | | UCH | FML | 8.0.99.99 | forgeSrc-1.12.2-14.23.0.2515.jar | None | | UCH | forge | 14.23.0.2515 | forgeSrc-1.12.2-14.23.0.2515.jar | None | | UCH | btm | 0.0.1 | bin | None | Loaded coremods (and transformers): GL info: ' Vendor: 'NVIDIA Corporation' Version: '4.5.0 NVIDIA 385.41' Renderer: 'GeForce GTX 1080 Ti/PCIe/SSE2' Launched Version: 1.12.2 LWJGL: 2.9.4 OpenGL: GeForce GTX 1080 Ti/PCIe/SSE2 GL version 4.5.0 NVIDIA 385.41, NVIDIA Corporation GL Caps: Using GL 1.3 multitexturing. Using GL 1.3 texture combiners. Using framebuffer objects because OpenGL 3.0 is supported and separate blending is supported. Shaders are available because OpenGL 2.1 is supported. VBOs are available because OpenGL 1.5 is supported. Using VBOs: Yes Is Modded: Definitely; Client brand changed to 'fml,forge' Type: Client (map_client.txt) Resource Packs: Current Language: English (US) Profiler Position: N/A (disabled) CPU: 8x Intel(R) Core(TM) i7-4770 CPU @ 3.40GHz As the stacktrace indicates an issue assigning the attack value, I tried lowering the attack value of my obsidian material, but that didn't change anything. Since the issue was in registering items, I thought that I might be registering too many items using this method. However looking at other registry for 1.12.2 this does not appear to be the case either. Any help would be greatly appreciated!
-
[1.12.2] Issue loading in textures
InterdimensionalCat replied to InterdimensionalCat's topic in Modder Support
Thank you for all of the help. After doing these fixes, both obsidianingot and smoothobsidian are loading properly. However datablock is still having an issue. It appears with the proper textures in the inventory, but when I place it, it loads the default missing texture. There are no errors in the console when I start up the game or place the block. datablock does have TileEntity data, which I assume is causing the problem. Project main: https://github.com/InterdimensionalCat/ModTest/tree/master/java/com/benthom123/test Project assets: https://github.com/InterdimensionalCat/ModTest/tree/master/resources/assets/btm -
[1.12.2] Issue loading in textures
InterdimensionalCat replied to InterdimensionalCat's topic in Modder Support
Sorry I thought I posted it Here is the code: https://github.com/InterdimensionalCat/ModTest/tree/master/java/com/benthom123/test If you need me to post specific blocks of code into the forums I can do that too, but I am just not sure where the issue is coming from -
Hello, I am new to programming mods for Minecraft. after implementing 2 blocks (DataBlock and SmoothObsidian) and one item (ObsidianIngot) I tried adding basic textures to two of them (DataBlock and ObsidianIngot). The program compiled but the game did not load the textures.\ When running the game, the console logs these errors: [22:43:37] [main/ERROR] [FML]: Exception loading model for variant btm:smoothobsidian#normal for blockstate "btm:smoothobsidian" net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model btm:smoothobsidian#normal with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.registerVariant(ModelLoader.java:233) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadBlock(ModelBakery.java:153) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.loadBlocks(ModelLoader.java:221) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:159) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:559) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:421) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_151] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_151] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_151] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_151] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:83) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1242) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 21 more [22:43:37] [main/ERROR] [FML]: Exception loading blockstate for the variant btm:smoothobsidian#normal: java.lang.Exception: Could not load model definition for variant btm:smoothobsidian at net.minecraftforge.client.model.ModelLoader.getModelBlockDefinition(ModelLoader.java:266) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadBlock(ModelBakery.java:121) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.loadBlocks(ModelLoader.java:221) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:159) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:559) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:421) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_151] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_151] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_151] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_151] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] Caused by: java.lang.RuntimeException: Encountered an exception when loading model definition of model btm:blockstates/smoothobsidian.json at net.minecraft.client.renderer.block.model.ModelBakery.loadMultipartMBD(ModelBakery.java:228) ~[ModelBakery.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.getModelBlockDefinition(ModelBakery.java:208) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.getModelBlockDefinition(ModelLoader.java:262) ~[ModelLoader.class:?] ... 20 more Caused by: java.io.FileNotFoundException: btm:blockstates/smoothobsidian.json at net.minecraft.client.resources.FallbackResourceManager.getAllResources(FallbackResourceManager.java:104) ~[FallbackResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.getAllResources(SimpleReloadableResourceManager.java:79) ~[SimpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadMultipartMBD(ModelBakery.java:221) ~[ModelBakery.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.getModelBlockDefinition(ModelBakery.java:208) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.getModelBlockDefinition(ModelLoader.java:262) ~[ModelLoader.class:?] ... 20 more [22:43:37] [main/ERROR] [FML]: Exception loading model for variant btm:datablock#inventory for items ["btm:datablock", "minecraft:air"], normal location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model btm:item/datablock with loader VanillaLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:297) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:175) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:160) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:559) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:421) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_151] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_151] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_151] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_151] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] Caused by: java.io.FileNotFoundException: btm:models/item/datablock.json at net.minecraft.client.resources.FallbackResourceManager.getResource(FallbackResourceManager.java:69) ~[FallbackResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:65) ~[SimpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadModel(ModelBakery.java:334) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.access$1600(ModelLoader.java:126) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader$VanillaLoader.loadModel(ModelLoader.java:933) ~[ModelLoader$VanillaLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 20 more [22:43:37] [main/ERROR] [FML]: Exception loading model for variant btm:datablock#inventory for items ["btm:datablock", "minecraft:air"], blockstate location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model btm:datablock#inventory with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:305) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:175) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:160) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:559) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:421) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_151] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_151] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_151] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_151] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:83) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1242) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 20 more [22:43:37] [main/ERROR] [FML]: Exception loading model for variant btm:smoothobsidian#inventory for item "btm:smoothobsidian", normal location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model btm:item/smoothobsidian with loader VanillaLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:297) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:175) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:160) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:559) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:421) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_151] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_151] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_151] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_151] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] Caused by: java.io.FileNotFoundException: btm:models/item/smoothobsidian.json at net.minecraft.client.resources.FallbackResourceManager.getResource(FallbackResourceManager.java:69) ~[FallbackResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:65) ~[SimpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadModel(ModelBakery.java:334) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.access$1600(ModelLoader.java:126) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader$VanillaLoader.loadModel(ModelLoader.java:933) ~[ModelLoader$VanillaLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 20 more [22:43:37] [main/ERROR] [FML]: Exception loading model for variant btm:smoothobsidian#inventory for item "btm:smoothobsidian", blockstate location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model btm:smoothobsidian#inventory with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:305) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:175) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:160) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:559) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:421) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_151] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_151] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_151] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_151] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:83) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1242) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 20 more [22:43:37] [main/ERROR] [FML]: Exception loading model for variant btm:obsidianingot#inventory for item "btm:obsidianingot", normal location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model btm:item/obsidianingot with loader VanillaLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:297) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:175) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:160) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:559) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:421) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_151] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_151] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_151] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_151] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] Caused by: java.io.FileNotFoundException: btm:models/item/obsidianingot.json at net.minecraft.client.resources.FallbackResourceManager.getResource(FallbackResourceManager.java:69) ~[FallbackResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:65) ~[SimpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadModel(ModelBakery.java:334) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.access$1600(ModelLoader.java:126) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader$VanillaLoader.loadModel(ModelLoader.java:933) ~[ModelLoader$VanillaLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 20 more [22:43:37] [main/ERROR] [FML]: Exception loading model for variant btm:obsidianingot#inventory for item "btm:obsidianingot", blockstate location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model btm:obsidianingot#inventory with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:305) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:175) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:160) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:559) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:421) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_151] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_151] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_151] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_151] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_151] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:83) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1242) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 20 more Any help figuring out what is wrong would be great! Keep in mind I am still very new so the mistake might be something really obvious. Alternatively if there is another way to add models to blocks and/or items that is more friendly to beginners that would work too. Project on GitHub: https://github.com/InterdimensionalCat/ModTest/tree/master/java/com/benthom123/test
-
Hello, I am new to modding in Minecraft. After creating a few test blocks and a TileEntity I wanted to test my mod to see if things were working alright. However when I loaded up the game in Eclipse the game crashed and I got this error, and I cannot figure out what the problem is: ---- Minecraft Crash Report ---- // Hey, that tickles! Hehehe! Time: 10/24/17 8:31 PM Description: Initializing game java.lang.NullPointerException: Initializing game at com.benthom123.test.proxy.CommonProxy.registerItems(CommonProxy.java:52) at net.minecraftforge.fml.common.eventhandler.ASMEventHandler_5_CommonProxy_registerItems_Register.invoke(.dynamic) at net.minecraftforge.fml.common.eventhandler.ASMEventHandler.invoke(ASMEventHandler.java:90) at net.minecraftforge.fml.common.eventhandler.EventBus$1.invoke(EventBus.java:143) at net.minecraftforge.fml.common.eventhandler.EventBus.post(EventBus.java:179) at net.minecraftforge.registries.GameData.fireRegistryEvents(GameData.java:741) at net.minecraftforge.fml.common.Loader.preinitializeMods(Loader.java:603) at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:266) at net.minecraft.client.Minecraft.init(Minecraft.java:513) at net.minecraft.client.Minecraft.run(Minecraft.java:421) at net.minecraft.client.main.Main.main(Main.java:118) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) at GradleStart.main(GradleStart.java:26) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Thread: Client thread Stacktrace: at com.benthom123.test.proxy.CommonProxy.registerItems(CommonProxy.java:52) at net.minecraftforge.fml.common.eventhandler.ASMEventHandler_5_CommonProxy_registerItems_Register.invoke(.dynamic) at net.minecraftforge.fml.common.eventhandler.ASMEventHandler.invoke(ASMEventHandler.java:90) at net.minecraftforge.fml.common.eventhandler.EventBus$1.invoke(EventBus.java:143) at net.minecraftforge.fml.common.eventhandler.EventBus.post(EventBus.java:179) at net.minecraftforge.registries.GameData.fireRegistryEvents(GameData.java:741) at net.minecraftforge.fml.common.Loader.preinitializeMods(Loader.java:603) at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:266) at net.minecraft.client.Minecraft.init(Minecraft.java:513) -- Initialization -- Details: Stacktrace: at net.minecraft.client.Minecraft.run(Minecraft.java:421) at net.minecraft.client.main.Main.main(Main.java:118) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) at GradleStart.main(GradleStart.java:26) -- System Details -- Details: Minecraft Version: 1.12.2 Operating System: Windows 10 (amd64) version 10.0 Java Version: 1.8.0_151, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 805540112 bytes (768 MB) / 1037959168 bytes (989 MB) up to 1037959168 bytes (989 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: MCP 9.42 Powered by Forge 14.23.0.2515 5 mods loaded, 5 mods active States: 'U' = Unloaded 'L' = Loaded 'C' = Constructed 'H' = Pre-initialized 'I' = Initialized 'J' = Post-initialized 'A' = Available 'D' = Disabled 'E' = Errored | State | ID | Version | Source | Signature | |:----- |:--------- |:------------ |:-------------------------------- |:--------- | | UCH | minecraft | 1.12.2 | minecraft.jar | None | | UCH | mcp | 9.42 | minecraft.jar | None | | UCH | FML | 8.0.99.99 | forgeSrc-1.12.2-14.23.0.2515.jar | None | | UCH | forge | 14.23.0.2515 | forgeSrc-1.12.2-14.23.0.2515.jar | None | | UCH | btm | 0.0.1 | bin | None | Loaded coremods (and transformers): GL info: ' Vendor: 'NVIDIA Corporation' Version: '4.5.0 NVIDIA 385.41' Renderer: 'GeForce GTX 1080 Ti/PCIe/SSE2' Launched Version: 1.12.2 LWJGL: 2.9.4 OpenGL: GeForce GTX 1080 Ti/PCIe/SSE2 GL version 4.5.0 NVIDIA 385.41, NVIDIA Corporation GL Caps: Using GL 1.3 multitexturing. Using GL 1.3 texture combiners. Using framebuffer objects because OpenGL 3.0 is supported and separate blending is supported. Shaders are available because OpenGL 2.1 is supported. VBOs are available because OpenGL 1.5 is supported. Using VBOs: Yes Is Modded: Definitely; Client brand changed to 'fml,forge' Type: Client (map_client.txt) Resource Packs: Current Language: English (US) Profiler Position: N/A (disabled) CPU: 8x Intel(R) Core(TM) i7-4770 CPU @ 3.40GHz It should be noted that I am following this tutorial to learn to mod: https://wiki.mcjty.eu/modding/index.php/Main_Page I believe the problem is in CommonProxy, so here is what that looks like: package com.benthom123.test.proxy; import java.io.File; import com.benthom123.test.Config; import com.benthom123.test.ModBlocks; import com.benthom123.test.ModItems; import com.benthom123.test.modClass; import com.benthom123.test.blocks.FirstBlock; import com.benthom123.test.blocks.datablock.DataTileEntity; import com.benthom123.test.items.FirstItem; import net.minecraft.block.Block; import net.minecraft.item.Item; import net.minecraft.item.ItemBlock; import net.minecraftforge.common.config.Configuration; import net.minecraftforge.event.RegistryEvent; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; import net.minecraftforge.fml.common.eventhandler.SubscribeEvent; import net.minecraftforge.fml.common.registry.GameRegistry; @Mod.EventBusSubscriber public class CommonProxy { // Config instance public static Configuration config; public void preInit(FMLPreInitializationEvent e) { File directory = e.getModConfigurationDirectory(); config = new Configuration(new File(directory.getPath(), "modtut.cfg")); Config.readConfig(); } public void init(FMLInitializationEvent e) { if (config.hasChanged()) { config.save(); } } public void postInit(FMLPostInitializationEvent e) { } @SubscribeEvent public static void registerBlocks(RegistryEvent.Register<Block> event) { event.getRegistry().register(new FirstBlock()); GameRegistry.registerTileEntity(DataTileEntity.class, modClass.MODID + "_datablock"); } @SubscribeEvent public static void registerItems(RegistryEvent.Register<Item> event) { event.getRegistry().register(new ItemBlock(ModBlocks.SmoothObsidian).setRegistryName(ModBlocks.SmoothObsidian.getRegistryName())); event.getRegistry().register(new FirstItem()); event.getRegistry().register(new ItemBlock(ModBlocks.dataBlock).setRegistryName(ModBlocks.dataBlock.getRegistryName())); } } if another class is the issue, here is the entire project on GitHub: https://github.com/InterdimensionalCat/src Any help would be appreciated! EDIT: It looks like this line of code specifically in CommonProxy is the issue: event.getRegistry().register(new ItemBlock(ModBlocks.SmoothObsidian).setRegistryName(ModBlocks.SmoothObsidian.getRegistryName()));