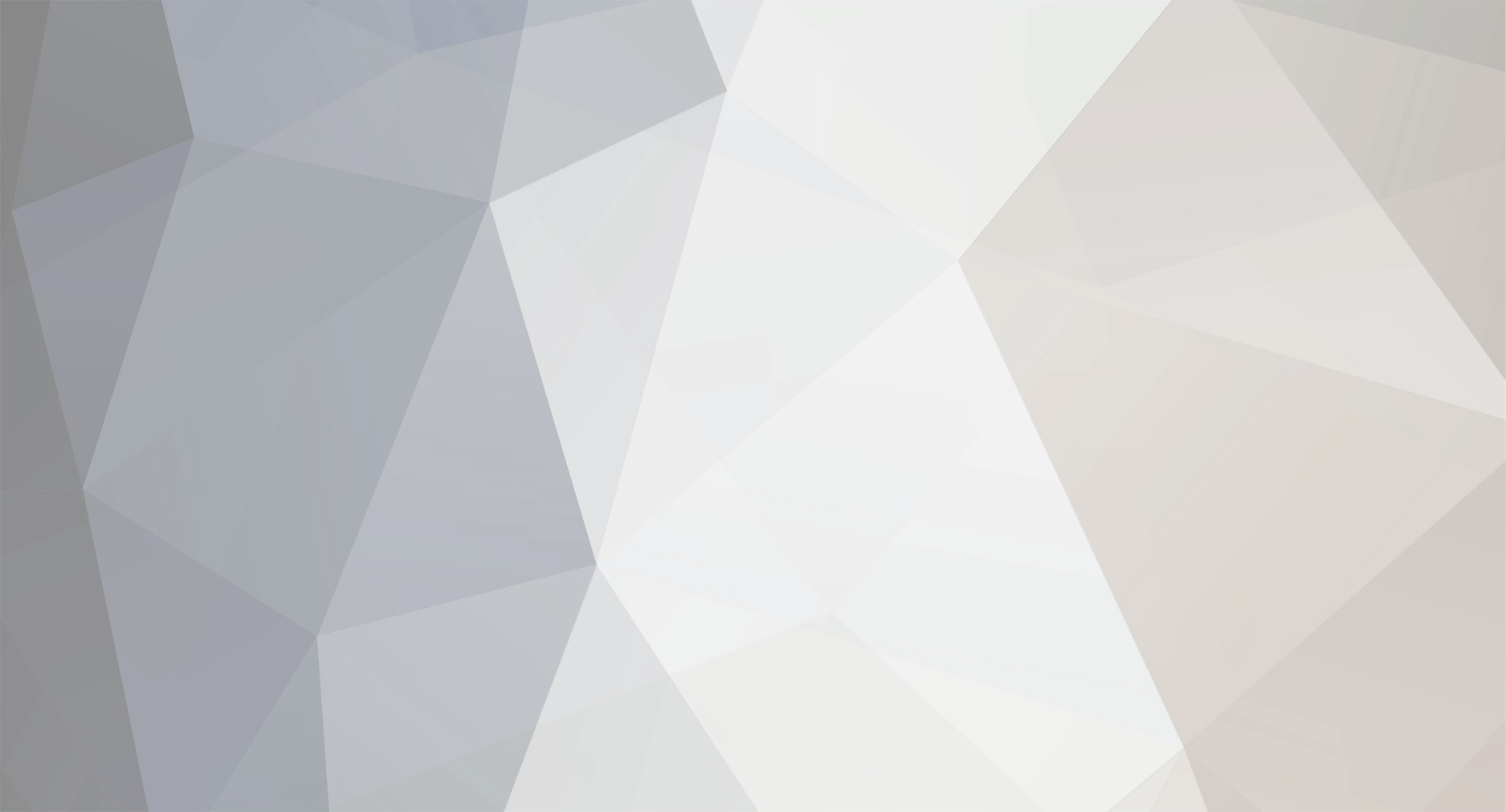
Quarg
Members-
Posts
31 -
Joined
-
Last visited
Everything posted by Quarg
-
Hi; I have been trying to work out a method by which I can build a mod that uses an external library (in this case I am using LuaJ). I have everything running fine from eclipse; however, of course the provided forge build scripts do not know about the referenced library, and as such fail to build the mod. What should I do to build this mod for distribution? **EDIT:** Ok, so having looked through the "build.gradle" file I realised I simply wanted to put the referenced library's jar file into "./libs", which has worked.
-
Yep, that's it, thanks!
-
I've been doing some stuff with using Lua for making Scriptable item effects, but I've hit a bit of a peculiar issue. I'd thought that it would be sensible to use the "name" format (eg: "minecraft:dirt") for blocks in the Lua scripts, and I have found it easy to obtain the Block from the name using the following: Block.getBlockFromName("minecraft:dirt"); However I cannot find a sensible way to do the reverse; obtaining the "name" from the Block instance. Thanks in advance!
-
After a bit of experimentation, I've found a method that works somewhat. What I have done is put the de-obfuscated mod jar in "/run/mods/" so that the mod is included when running, and the API source in "/src/main/java/" so it shows up in eclipse Unfortunately some APIs (such as the Thaumcraft API) have compile-time missing-reference errors that I am not sure what to do about. Presumably there is a way to make Eclipse ignore these errors, but I have no idea how.
-
Thanks for the reply, but I'm unable to make much use of that tutorial.
-
Hi, All the information that I can find about using APIs for other mods is incredibly unclear, and I was hoping someone could clarify things for me. It is obvious that I need two things: the API, and the De-obfuscated Jar for the mod, but no-where I have looked seems to distinguish between the two, making it unclear as to what I am expected to do with them. EDIT: I am trying to do so for 1.8 if that makes much difference.
-
I've now hit a related issue. Put simply, I don't know how to synchronize variables inside the entity, (which usually would not be an issue unless they are used for rendering, which this is). In this particular case the variable is set when the entity is created and will never be changed.
-
Ok, changing that has worked, thanks a bunch! for the sake of anyone wondering about my final solution: EntityRegistry.registerModEntity(EntityQuantumEssentia.class, "qfthaum_essentia", 0, this, 64, 1, true);
-
Yes I have registered the entity in the init phase (do I need to do it in a specific phase?) EntityRegistry.registerGlobalEntityID(EntityQuantumEssentia.class, "qfthaum_essentia", EntityRegistry.findGlobalUniqueEntityId());
-
At the moment, the entity only exists server-side. Is it that I am entering it into the world wrong? At the moment what I am doing is this: worldObj.spawnEntityInWorld(entity); Which only occurs server-side, and the entity is definitely ticking server-side so it definitely gets this far at the least.
-
So, I am fairly sure that you only need to create/spawn entities server-side (to avoid having client-side "ghost" entities), but I don't know how to then make the client aware of the entities that are spawned server-side. (Note that I am not referring to mobs, just entities that inherit from the base entity class) Thanks in advance for any help!
-
Put simply, the interact and attackEntityFrom methods appear to never be called. public class EntityMagicBuffer extends Entity implements IMagicEnergyProvider { protected float storedEnergy; public EntityMagicBuffer(World world) { super(world); setSize(1, 1); } @Override public boolean doesEntityNotTriggerPressurePlate() { return true; } @Override public boolean canBeCollidedWith() { return false; } @Override public AxisAlignedBB getBoundingBox() { return boundingBox; } @Override public AxisAlignedBB getCollisionBox(Entity par1Entity) { // TODO Auto-generated method stub return boundingBox; } @Override public void onEntityUpdate() { worldObj.spawnParticle("magiccrit", posX, posY, posZ, 0, 0, 0); super.onEntityUpdate(); } @Override public boolean interact(EntityPlayer par1EntityPlayer) { System.out.println("clicked!"); return true; } @Override public boolean attackEntityFrom(DamageSource par1DamageSource, int par2) { System.out.println("poke!"); return super.attackEntityFrom(par1DamageSource, par2); } @Override protected void entityInit() { } [... other code relating purely to my interface ...] } I think it may be a bounding box related issue since arrows fly straight through the entity, although using F3+B still makes it show the bounding box I was expecting. EDIT: as a most likely unrelated problem, the entity appears to sometimes glitch up/down about half a block, (but always remaining in one of the two up/down states, never going any further) EDIT2: making canBeCollidedWith() return true fixed it entirely, the getBoundingBox() and getCollisionBox() functions can freely return null without preventing this.
-
I have a staff item that I want to have drain energy on usage, the current way I have it set up is that this function in the staff class is called via a tickhandler: public float doDrawEnergy(ItemStack item, EntityPlayer player, float energy) { if(item.getTagCompound().getFloat("charge") > energy) { item.setTagInfo("charge", new NBTTagFloat("charge",item.getTagCompound().getFloat("charge") - energy)); return energy; } else { float out = item.getTagCompound().getFloat("charge"); item.setTagInfo("charge", new NBTTagFloat("charge",0)); return out; } } The problem is that the "charge" nbt value doesn't always change, specifically it only that the getFloat function only returns the latest set value after opening a GUI (ie: it only seems to upate the value after opening a GUI), and I have absoluetly no idea why or how this happens.
-
[SOLVED] GUI Items in player inventory behaving awkwardly
Quarg replied to intermediateuser's topic in Modder Support
I decided to use a few break points to see what actually happens when I open the gui, and I found that it wasn't opening the gui handler server-side because my @Mod file didn't have an @NetworkMod annotation, simply adding @NetworkMod after the @Mod annotation and it's parameters seems to have fixed it for me. -
[SOLVED] GUI Items in player inventory behaving awkwardly
Quarg replied to intermediateuser's topic in Modder Support
I just updated my latest post in the other thread, it appears that (with my mod at least) the server thinks that the player has their regular inventory open while the client thinks that the tile inventory is open. (which explains all of these oddities, since the player inventory has nine additional slots, 4 armour, 4 crafting, and the crafting output, and adding more slots in the custom container class will move the slots that the client closer to those the server uses) As such; I guess there must be something we are both missing for the opening of the GUI. EDIT: here is the code used for opening the GUI for me: @Override public boolean onBlockActivated(World world, int i, int j, int k, EntityPlayer player, int a, float b, float c, float d) { TileEntity tileEntity = world.getBlockTileEntity(i, j, k); if (tileEntity == null || player.isSneaking()) { return false; } player.openGui(Alchemagic.instance, 0, world, i, j, k); return true; } -
[SOLVED] GUI Items in player inventory behaving awkwardly
Quarg replied to intermediateuser's topic in Modder Support
I had realised much the same thing, except adding additional slots *before* the inventory results in offsetting the slot taken from, (see my post here which goes into a bit more detail about it and some other odd behaviour.) -
Having noticed that the slots were always offset by the ammount of tile slots minus nine, I tried making it so I had nine tile slots (though some were duplicates) like so: addSlotToContainer(new Slot(tile,0,17,17)); //Water In addSlotToContainer(new Slot(tile,1,17,49)); //Water Out addSlotToContainer(new Slot(tile,2,143,49)); //Output Crystals for(int i = 0; i< 6;i++) { addSlotToContainer(new Slot(tile,2,-18,18*i)); //THESE ARE THE DUMMY SLOTS } for (j = 0; j < 3; ++j) { for (k = 0; k < 9; ++k) { this.addSlotToContainer(new Slot(player, k + j * 9 + 9, 8 + k * 18, 84 + j * 18)); } } for (j = 0; j < 9; ++j) { this.addSlotToContainer(new Slot(player, j, 8 + j * 18, 142)); } at which point interacting with the player's inventory was exactly as it should be (or is as far as I know, I've yet to experience a problem with it). though the interaction with my tile's inventory is still quite wonkey, though I expecty that is an issue with the IInventory functions' implementations EDIT: I've just found something very strange, I appear to be able to craft using my tile's inventory, in that slot 0 of the tile is the output and slots 1 and 2 are horisontally adjacent slots in the craft matrix, despite the fact I have no crafting logic stuff in it... (though the 'output' slot doesn't show the crafted item, but clicking on it does cause it to craft) EDIT2: I thought I'd post my IGuiHandler's code since it's relevant here: @Override public Object getServerGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { TileEntity tileEntity = world.getBlockTileEntity(x, y, z); if(tileEntity instanceof TileCrystaliser){ return new ContainerCrystaliser((TileCrystaliser) tileEntity, player.inventory); } return null; } @Override public Object getClientGuiElement(int id, EntityPlayer player, World world, int x, int y, int z) { TileEntity tileEntity = world.getBlockTileEntity(x, y, z); if(tileEntity instanceof TileCrystaliser){ return new GuiCrystaliser(player.inventory, (TileCrystaliser) tileEntity); } return null; } EDIT3: after noticing the ability to use the slots to craft I decided to do a test and expant the tile's inventory size to nine items and add a slot to the container for each of them, and I found that clicking on four of those slots drops the current worn armour, which makes me think that the server thinks that the player has their normal inventory open while the client has the tile's inventory open.
-
or better yet: if (this.kegItemStacks[0] != null && this.kegItemStacks[0].isItemEqual(new ItemStack(EatMe.yeast))) would work fine.
-
you can't simply compare two itemstacks using the standard == or !=, it will always consider them not equal since they arn't the same object, you should either manually compare their item IDs and Metadata/Damage OR use item1.equals(item2)
-
[SOLVED] GUI Items in player inventory behaving awkwardly
Quarg replied to intermediateuser's topic in Modder Support
Considering that I am having the same problem, and that my tile entity does have the read and write functions (as well as simple packet code), and that their container doesn't actually create any slots for the tile, I would be quite surprised if that were the root of the problem. here is my tile code to be clear: package com.quargos.am.blocks.tiles; import net.minecraft.block.Block; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.inventory.IInventory; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.network.INetworkManager; import net.minecraft.network.packet.Packet; import net.minecraft.network.packet.Packet132TileEntityData; import net.minecraft.tileentity.TileEntity; import net.minecraftforge.liquids.ILiquidTank; import net.minecraftforge.liquids.LiquidContainerRegistry; import net.minecraftforge.liquids.LiquidStack; public class TileCrystaliser extends TileEntity implements IInventory, ILiquidTank { public LiquidStack liquid = new LiquidStack(Block.waterStill, 0); public ItemStack[] contents = new ItemStack[3]; @Override public void updateEntity() { super.updateEntity(); } public Packet getDescriptionPacket() { NBTTagCompound nbtTag = new NBTTagCompound(); this.writeToNBT(nbtTag); return new Packet132TileEntityData(this.xCoord, this.yCoord, this.zCoord, 1, nbtTag); } public void onDataPacket(INetworkManager net, Packet132TileEntityData packet) { readFromNBT(packet.customParam1); } @Override public void readFromNBT(NBTTagCompound nbt) { for(int i = 0; i < contents.length; i++) { contents[i] = ItemStack.loadItemStackFromNBT(nbt.getCompoundTag("content_" + i)); } liquid.amount = nbt.getInteger("waterLevel"); super.readFromNBT(nbt); } @Override public void writeToNBT(NBTTagCompound nbt) { for(int i = 0; i < contents.length; i++) { if(contents[i] != null) contents[i].writeToNBT(nbt.getCompoundTag("content_" + i)); //Ensure works! } nbt.setInteger("waterLevel", liquid.amount); super.writeToNBT(nbt); } //ILiquidTank @Override public LiquidStack getLiquid() { return liquid; } @Override public int getCapacity() { return 8*LiquidContainerRegistry.BUCKET_VOLUME; } @Override public int fill(LiquidStack resource, boolean doFill) { if(doFill) { if(resource.itemID == Block.waterStill.blockID) { int amount = liquid.amount + resource.amount; if(amount > getCapacity()) { amount -= getCapacity(); liquid.amount = getCapacity(); return resource.amount - amount; } liquid.amount += resource.amount; return resource.amount; } } return 0; } @Override public LiquidStack drain(int maxDrain, boolean doDrain) { if(doDrain) { liquid.amount -= maxDrain; if(liquid.amount < 0) { LiquidStack out = new LiquidStack(liquid.itemID, -liquid.amount); liquid.amount = 0; return out; } return new LiquidStack(liquid.itemID, maxDrain); } return null; } @Override public int getTankPressure() { return 0; } //IInventory @Override public int getSizeInventory() { return contents.length; } @Override public ItemStack getStackInSlot(int i) { if(i >= 0 && i < contents.length) return contents[i]; return null; } @Override public ItemStack decrStackSize(int i, int j) { if(i >= 0 && i < contents.length) { if(contents[i].stackSize > j) { contents[i].stackSize -= j; return new ItemStack(contents[i].itemID,j,contents[i].getItemDamage()); } ItemStack out = contents[i]; contents[i] = null; return out; } return null; } @Override public ItemStack getStackInSlotOnClosing(int i) { return null; } @Override public void setInventorySlotContents(int i, ItemStack itemstack) { contents[i] = itemstack; } @Override public String getInvName() { return "Soul Crystaliser"; } @Override public boolean isInvNameLocalized() { return true; //should change when implementing localisation stuff. } @Override public int getInventoryStackLimit() { return 64; } @Override public boolean isUseableByPlayer(EntityPlayer entityplayer) { return true; } @Override public void openChest() { } @Override public void closeChest() { } @Override public boolean isStackValidForSlot(int i, ItemStack itemstack) { return true; } } -
I'm already following (and have posted in) that thread.
-
doing that appears to have made absolutely no difference.
-
You've most likely got the mod files in a subfolder in the .zip file at a guess. If you could give us some idea of the structure of the files in the zip (so much as a screenshot would be good) then we might be able to give some more help.
-
[SOLVED] GUI Items in player inventory behaving awkwardly
Quarg replied to intermediateuser's topic in Modder Support
I appear to be having the same problem, and changing the equivilant code didn't work for me either.