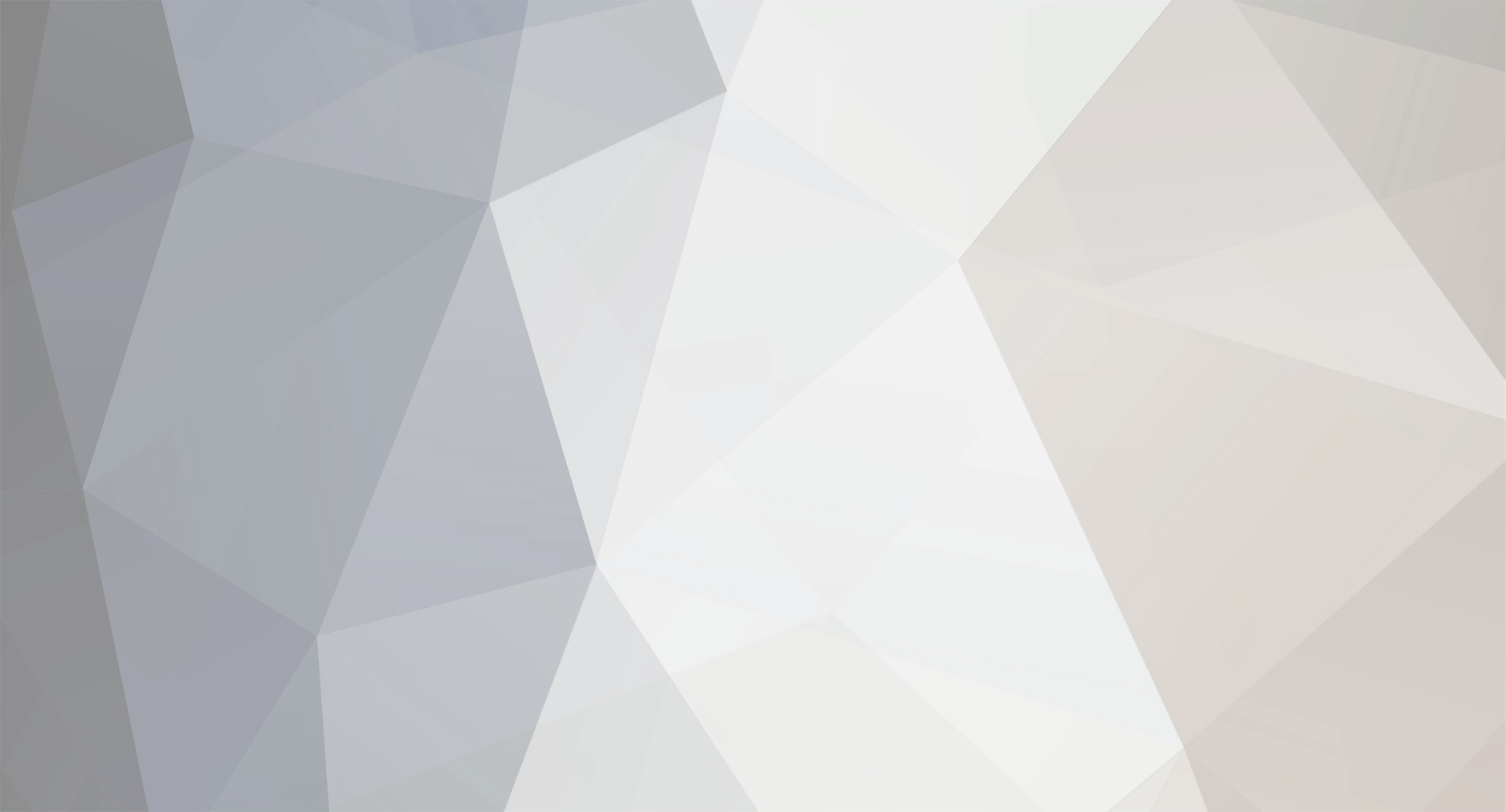
FlashHUN
Members-
Posts
84 -
Joined
-
Last visited
Everything posted by FlashHUN
-
No matter what order I put these in or which class I enable and disable them from (RenderSystem, GlStateManager, GL11), I can't achieve the result I'm looking for...
-
Players can unlock and go into a form that allows them to see other players and mobs through walls in a radius depending on how skilled they are at using said form, and see roughly how much mana those players have left. That's the basic idea.
-
I want to give the player a buff that allows them to see mobs through walls. Instead of using the glowing effect, I actually want to make the mobs render through the walls. How could I achieve that?
-
When I change the player's hitbox with EntityEvent.Size and recalculate it using Entity.recalculateSize() on both the client and server when it should change, the hitbox changes fine, however it is off-center and when the player moves they get teleported into the center of the hitbox. EntityEvent.Size: @SubscribeEvent public void playerSize(EntityEvent.Size event) { if (event.getEntity().isAddedToWorld()) { PlayerEntity player = (PlayerEntity)event.getEntity(); IPlayerCap cap = CapabilityUtil.getPlayerCap(player); EntitySize changeTo = player.getSize(player.getPose()); if (cap.getSusanooActive()) { changeTo = SUSANOO_CAGE; } event.setNewSize(changeTo); } } Entity.recalculateSize() gets called in the packet I sync the capability with when the data changes Edit: the current fix I found for it is after calling recalculateSize() I need to set the bounding box again with player.setBoundingBox(new AxisAlignedBB(player.getPosX()-(double)width/2, player.getPosY(), player.getPosZ()-(double)width/2, player.getPosX()+(double)width/2, player.getPosY()+(double)height, player.getPosZ()+(double)width/2));
-
How can I loop a sound when a condition is met and stop it when needed?
-
Minecraft client = Minecraft.getInstance(); ItemStack stack = new ItemStack(Items.GOLD_INGOT); GL11.glPushMatrix(); GL11.glTranslatef(posX, posY, 0.0F); GL11.glScalef(scale, scale, 0.0F); client.getItemRenderer().renderItemIntoGUI(stack, 0, 0); GL11.glPopMatrix(); Rendering any item in a Screen makes it appear dark. What could cause this and how could I fix it?
-
I looked at how a filled map item gets block colors, tried something similar, but I've ran into a couple of issues. My code: private MaterialColor getBlockColorAt(int x, int z) { Chunk chunk = client.world.getChunkAt(new BlockPos(x, 0, z)); int y = chunk.getTopBlockY(Heightmap.Type.WORLD_SURFACE, x, z); BlockPos pos = new BlockPos(x, y, z); return client.world.getBlockState(pos).getMaterialColor(client.world.getBlockReader(chunk.getPos().x, chunk.getPos().z), pos); } Issues: BlockState#getMaterialColor is deprecated and I can't find any alternatives to use y always returns -1 What am I doing wrong? What do I need to do to get a block's color at a specific position?
-
I have a non-square texture that's larger than 256x256 in size and I want to draw it on the screen without it getting scaled to be a square. What method do I need to use? I know there was a drawModalRectWithCustomTextureSize method in older versions, but I can't find what it was replaced with.
-
This is the error I get when trying to access the layer renderers of a player by getting the player renderer then doing render.layerRenderers, yet some mods like Quark can just simply access it by doing the exact same thing. Why could that be?
-
I have my custom fire block set up, how could I render an overlay when the player is on fire like the vanilla one but with my custom fire's texture?
-
If I have a layer that is rendering a model on top of the player model, how could I render that model in first person view aswell?
-
When I hold an item in my hand, the model's texture which I want to be transparent becomes solid. Is there any way to fix this? (Having the texture transparent and rendering it with an alpha of 1.0F has the same result as having it solid and rendering it with a lower alpha) Layer code: @OnlyIn(Dist.CLIENT) public class LayerSusanoo extends LayerRenderer<AbstractClientPlayerEntity, PlayerModel<AbstractClientPlayerEntity>> { public LayerSusanoo( IEntityRenderer<AbstractClientPlayerEntity, PlayerModel<AbstractClientPlayerEntity>> entityRendererIn) { super(entityRendererIn); } private static final ResourceLocation cage = new ResourceLocation(Main.modid, "textures/layers/player/susanoo/cage.png"); @Override public void render(AbstractClientPlayerEntity entityIn, float limbSwing, float limbSwingAmount, float partialTicks, float ageInTicks, float netHeadYaw, float headPitch, float scale) { IPlayerKgCap kgcap = PlayerKgCapStorage.getCapability(entityIn); IPlayerAnimCap animcap = PlayerAnimCapStorage.getCapability(entityIn); if (kgcap.getSusanooActive()) { if (kgcap.getSusanooSize() == 1) { GlStateManager.pushMatrix(); ModelSusanooCage modelSusanooCage = new ModelSusanooCage(); GL11.glColor4f(kgcap.getSusanooColorR()/255F, kgcap.getSusanooColorG()/255F, kgcap.getSusanooColorB()/255F, 0.2F); this.bindTexture(cage); modelSusanooCage.isSitting = this.getEntityModel().isSitting; modelSusanooCage.isChild = this.getEntityModel().isChild; modelSusanooCage.setLivingAnimations(entityIn, limbSwing, limbSwingAmount, partialTicks); modelSusanooCage.render(entityIn, limbSwing, limbSwingAmount, ageInTicks, netHeadYaw, headPitch, scale); GlStateManager.popMatrix(); } } } @Override public boolean shouldCombineTextures() { return false; } }
-
[SOLVED] [1.14.4] Syncing player capabilities to client
FlashHUN replied to FlashHUN's topic in Modder Support
Thank you so much for the solution! -
[SOLVED] [1.14.4] Syncing player capabilities to client
FlashHUN replied to FlashHUN's topic in Modder Support
I added this line in readNBT() in my capability storage, Main.logger.debug("NBT Reading in Storage"); and this is the result: This should mean that it's called before the login event, right? If so, then why is it not sending the data that was saved to the player? -
[SOLVED] [1.14.4] Syncing player capabilities to client
FlashHUN replied to FlashHUN's topic in Modder Support
Not exactly sure what you mean by that, but this is how I set up and registered my capability: Provider: public class PlayerBaseCapProvider implements ICapabilitySerializable<INBT> { @CapabilityInject(IPlayerBaseCap.class) public static Capability<IPlayerBaseCap> PLAYER_BASE_CAP; private LazyOptional<IPlayerBaseCap> instance = LazyOptional.of(PLAYER_BASE_CAP::getDefaultInstance); @Override public <T> LazyOptional<T> getCapability(Capability<T> cap, Direction side) { return cap == PLAYER_BASE_CAP ? instance.cast() : LazyOptional.empty(); } @Override public INBT serializeNBT() { return PLAYER_BASE_CAP.getStorage().writeNBT(PLAYER_BASE_CAP, this.instance.orElseThrow(() -> new IllegalArgumentException("LazyOptional must not be empty!")), null); } @Override public void deserializeNBT(INBT nbt) { PLAYER_BASE_CAP.getStorage().readNBT(PLAYER_BASE_CAP, this.instance.orElseThrow(() -> new IllegalArgumentException("LazyOptional must not be empty!")), null, nbt); } } Storage: public class PlayerBaseCapStorage implements IStorage<IPlayerBaseCap> { @Override public INBT writeNBT(Capability<IPlayerBaseCap> capability, IPlayerBaseCap instance, Direction side) { CompoundNBT tag = new CompoundNBT(); tag.putBoolean("firstspawn", instance.getFirstSpawn()); tag.putInt("village", instance.getVillage()); tag.putInt("clan", instance.getClan()); tag.putInt("rank", instance.getRank()); tag.putInt("level", instance.getLevel()); tag.putInt("exp", instance.getExp()); tag.putInt("chakra", instance.getChakra()); tag.putInt("maxchakra", instance.getMaxChakra()); return tag; } @Override public void readNBT(Capability<IPlayerBaseCap> capability, IPlayerBaseCap instance, Direction side, INBT nbt) { CompoundNBT tag = new CompoundNBT(); instance.setFirstSpawn(tag.getBoolean("firstspawn")); instance.setVillage(tag.getInt("village")); instance.setClan(tag.getInt("clan")); instance.setRank(tag.getInt("rank")); instance.setLevel(tag.getInt("level")); instance.setExp(tag.getInt("exp")); instance.setChakra(tag.getInt("chakra")); instance.setMaxChakra(tag.getInt("maxchakra")); } @Mod.EventBusSubscriber(modid = Main.modid) private static class EventHandler { @SubscribeEvent(priority = EventPriority.HIGHEST) public static void onAttachCapabilities(AttachCapabilitiesEvent<Entity> event) { if (event.getObject() instanceof PlayerEntity) { event.addCapability(new ResourceLocation(Main.modid, "base"), new PlayerBaseCapProvider()); } } @SubscribeEvent public static void playerClone(final PlayerEvent.Clone event) { final IPlayerBaseCap oldBaseCap = event.getOriginal().getCapability(PlayerBaseCapProvider.PLAYER_BASE_CAP).orElseThrow(() -> new RuntimeException("No player capability found!")); final IPlayerBaseCap newBaseCap = event.getPlayer().getCapability(PlayerBaseCapProvider.PLAYER_BASE_CAP).orElseThrow(() -> new RuntimeException("No player capability found!")); if (oldBaseCap != null && newBaseCap != null) { newBaseCap.setFirstSpawn(oldBaseCap.getFirstSpawn()); newBaseCap.setVillage(oldBaseCap.getVillage()); newBaseCap.setClan(oldBaseCap.getClan()); newBaseCap.setRank(oldBaseCap.getRank()); newBaseCap.setExp(oldBaseCap.getExp()); newBaseCap.setLevel(oldBaseCap.getLevel()); newBaseCap.setChakra(oldBaseCap.getChakra()); newBaseCap.setMaxChakra(oldBaseCap.getMaxChakra()); } } @SubscribeEvent public static void serverLoginEvent(final PlayerLoggedInEvent event) { PlayerEntity player = event.getPlayer(); IPlayerBaseCap basecap = player.getCapability(PlayerBaseCapProvider.PLAYER_BASE_CAP).orElseThrow(() -> new RuntimeException("No player capability found!")); if (basecap != null) { Main.logger.info("Clan data sent from Storage: " + basecap.getClan()); PacketDispatcher.INSTANCE.sendTo(new PacketFirstSpawn(basecap.getFirstSpawn()), ((ServerPlayerEntity)player).connection.getNetworkManager(), NetworkDirection.PLAY_TO_CLIENT); PacketDispatcher.INSTANCE.sendTo(new PacketVillageC(basecap.getVillage()), ((ServerPlayerEntity)player).connection.getNetworkManager(), NetworkDirection.PLAY_TO_CLIENT); PacketDispatcher.INSTANCE.sendTo(new PacketClanC(basecap.getClan()), ((ServerPlayerEntity)player).connection.getNetworkManager(), NetworkDirection.PLAY_TO_CLIENT); if (basecap.getFirstSpawn() == true) { basecap.setFirstSpawn(false); PacketDispatcher.INSTANCE.sendTo(new PacketFirstSpawn(basecap.getFirstSpawn()), ((ServerPlayerEntity)player).connection.getNetworkManager(), NetworkDirection.PLAY_TO_CLIENT); player.sendMessage(new TranslationTextComponent("msgs.firstjoin", "")); ItemStack stack = new ItemStack(ItemList.character_creation, 1); player.addItemStackToInventory(stack); } } } @SubscribeEvent public static void changeDimesionEvent(final PlayerChangedDimensionEvent event) { PlayerEntity player = event.getPlayer(); IPlayerBaseCap basecap = player.getCapability(PlayerBaseCapProvider.PLAYER_BASE_CAP).orElseThrow(() -> new RuntimeException("No player capability found!")); PacketDispatcher.INSTANCE.sendTo(new PacketFirstSpawn(basecap.getFirstSpawn()), ((ServerPlayerEntity)player).connection.getNetworkManager(), NetworkDirection.PLAY_TO_CLIENT); PacketDispatcher.INSTANCE.sendTo(new PacketVillageC(basecap.getVillage()), ((ServerPlayerEntity)player).connection.getNetworkManager(), NetworkDirection.PLAY_TO_CLIENT); PacketDispatcher.INSTANCE.sendTo(new PacketClanC(basecap.getClan()), ((ServerPlayerEntity)player).connection.getNetworkManager(), NetworkDirection.PLAY_TO_CLIENT); } @SubscribeEvent public static void respawnEvent(final PlayerRespawnEvent event) { PlayerEntity player = event.getPlayer(); IPlayerBaseCap basecap = player.getCapability(PlayerBaseCapProvider.PLAYER_BASE_CAP).orElseThrow(() -> new RuntimeException("No player capability found!")); PacketDispatcher.INSTANCE.sendTo(new PacketFirstSpawn(basecap.getFirstSpawn()), ((ServerPlayerEntity)player).connection.getNetworkManager(), NetworkDirection.PLAY_TO_CLIENT); PacketDispatcher.INSTANCE.sendTo(new PacketVillageC(basecap.getVillage()), ((ServerPlayerEntity)player).connection.getNetworkManager(), NetworkDirection.PLAY_TO_CLIENT); PacketDispatcher.INSTANCE.sendTo(new PacketClanC(basecap.getClan()), ((ServerPlayerEntity)player).connection.getNetworkManager(), NetworkDirection.PLAY_TO_CLIENT); } } } Main: @Mod("naruto") public class Main { public static final String modid = "naruto"; public static final Logger logger = LogManager.getLogger(modid); public Main() { FMLJavaModLoadingContext.get().getModEventBus().addListener(this::setup); MinecraftForge.EVENT_BUS.register(this); } private void setup(final FMLCommonSetupEvent event) { CapabilityManager.INSTANCE.register(IPlayerBaseCap.class, new PlayerBaseCapStorage(), PlayerBaseCap::new); } } Is there anything I'm doing wrong? -
[SOLVED] [1.14.4] Syncing player capabilities to client
FlashHUN replied to FlashHUN's topic in Modder Support
Thanks, I didn't know that. I used a logger to print out the data being sent from the events and received in the packet. In both the respawn and change dimension events the data being sent and received is correct. In the PlayerLoggedInEvent the data being sent and received is 0. -
[SOLVED] [1.14.4] Syncing player capabilities to client
FlashHUN replied to FlashHUN's topic in Modder Support
If it's not static, I'm getting an error at public static PacketFirstSpawn decode(PacketBuffer buf) { return new PacketFirstSpawn(player); } saying The two ways I can solve that error is by either making packets in a different way and send the needed data of the player in the constructor instead of sending the player and then getting the data from the player inside the packet public class PacketClanC { private int data; public PacketClanC() {} public PacketClanC(int data) { this.data = data; } public static void encode(PacketClanC msg, PacketBuffer buf) { buf.writeInt(msg.data); } public static PacketClanC decode(PacketBuffer buf) { int data = buf.readInt(); return new PacketClanC(data); } public static void handle(PacketClanC msg, Supplier<NetworkEvent.Context> ctx) { ctx.get().enqueueWork(() -> { IPlayerBaseCap basecap = Minecraft.getInstance().player.getCapability(PlayerBaseCapProvider.PLAYER_BASE_CAP).orElseThrow(() -> new RuntimeException("No player capability found!")); basecap.setClan(msg.data); }); ctx.get().setPacketHandled(true); } } or make that field static. Please tell me which way you think is better, I'm still new to this whole networking thing and just learning what would be the best way to handle things like this. Both ways work, but the PlayerLoggedInEvent still doesn't send the needed data. By "debugging" I meant that I set the events and packets up in a way where I can see the data being sent from the event and received in the packet by writing it out. I think the problem occours in the PlayerLoggedInEvent, since the data being sent is 0 every time that event runs, but in every other event the data syncing is completely fine. -
[SOLVED] [1.14.4] Syncing player capabilities to client
FlashHUN replied to FlashHUN's topic in Modder Support
Did some debugging, apparently the data I'm trying to send from the CapabilityStorage is the default value (0) for the village and clan tags, but only in the login event. Why could that be? -
Hey. I need to sync the player's capabilities to them when they log in, respawn, etc., but the events that worked for this purpose in 1.12.2 for some reason no longer work. The capabilities just don't sync when those events happen. CapabilityStorage: public class PlayerBaseCapStorage implements IStorage<IPlayerBaseCap> { @Override public INBT writeNBT(Capability<IPlayerBaseCap> capability, IPlayerBaseCap instance, Direction side) { CompoundNBT tag = new CompoundNBT(); tag.putBoolean("firstspawn", instance.getFirstSpawn()); tag.putInt("village", instance.getVillage()); tag.putInt("clan", instance.getClan()); tag.putInt("rank", instance.getRank()); tag.putInt("level", instance.getLevel()); tag.putInt("exp", instance.getExp()); tag.putInt("chakra", instance.getChakra()); tag.putInt("maxchakra", instance.getMaxChakra()); return tag; } @Override public void readNBT(Capability<IPlayerBaseCap> capability, IPlayerBaseCap instance, Direction side, INBT nbt) { CompoundNBT tag = new CompoundNBT(); instance.setFirstSpawn(tag.getBoolean("firstspawn")); instance.setVillage(tag.getInt("village")); instance.setClan(tag.getInt("clan")); instance.setRank(tag.getInt("rank")); instance.setLevel(tag.getInt("level")); instance.setExp(tag.getInt("exp")); instance.setChakra(tag.getInt("chakra")); instance.setMaxChakra(tag.getInt("maxchakra")); } @Mod.EventBusSubscriber(modid = Main.modid) private static class EventHandler { @SubscribeEvent public static void onAttachCapabilities(AttachCapabilitiesEvent<Entity> event) { if (event.getObject() instanceof PlayerEntity) { event.addCapability(new ResourceLocation(Main.modid, "base"), new PlayerBaseCapProvider()); } } @SubscribeEvent public static void playerClone(final PlayerEvent.Clone event) { final IPlayerBaseCap oldBaseCap = event.getOriginal().getCapability(PlayerBaseCapProvider.PLAYER_BASE_CAP).orElseThrow(() -> new RuntimeException("No player capability found!")); final IPlayerBaseCap newBaseCap = event.getPlayer().getCapability(PlayerBaseCapProvider.PLAYER_BASE_CAP).orElseThrow(() -> new RuntimeException("No player capability found!")); if (oldBaseCap != null && newBaseCap != null) { newBaseCap.setFirstSpawn(oldBaseCap.getFirstSpawn()); newBaseCap.setVillage(oldBaseCap.getVillage()); newBaseCap.setClan(oldBaseCap.getClan()); newBaseCap.setRank(oldBaseCap.getRank()); newBaseCap.setExp(oldBaseCap.getExp()); newBaseCap.setLevel(oldBaseCap.getLevel()); newBaseCap.setChakra(oldBaseCap.getChakra()); newBaseCap.setMaxChakra(oldBaseCap.getMaxChakra()); } } @SubscribeEvent public static void serverLoginEvent(final PlayerLoggedInEvent event) { PlayerEntity player = event.getPlayer(); PacketDispatcher.INSTANCE.sendTo(new PacketFirstSpawn(player), ((ServerPlayerEntity)player).connection.getNetworkManager(), NetworkDirection.PLAY_TO_CLIENT); PacketDispatcher.INSTANCE.sendTo(new PacketVillageC(player), ((ServerPlayerEntity)player).connection.getNetworkManager(), NetworkDirection.PLAY_TO_CLIENT); PacketDispatcher.INSTANCE.sendTo(new PacketClanC(player), ((ServerPlayerEntity)player).connection.getNetworkManager(), NetworkDirection.PLAY_TO_CLIENT); if (player.getCapability(PlayerBaseCapProvider.PLAYER_BASE_CAP).orElseThrow(() -> new RuntimeException("No player capability found!")).getFirstSpawn() == true) { player.getCapability(PlayerBaseCapProvider.PLAYER_BASE_CAP).orElseThrow(() -> new RuntimeException("No player capability found!")).setFirstSpawn(false); PacketDispatcher.INSTANCE.sendTo(new PacketFirstSpawn(player), ((ServerPlayerEntity)player).connection.getNetworkManager(), NetworkDirection.PLAY_TO_CLIENT); player.sendMessage(new TranslationTextComponent("msgs.firstjoin", "")); ItemStack stack = new ItemStack(ItemList.character_creation, 1); player.addItemStackToInventory(stack); } } @SubscribeEvent public static void changeDimesionEvent(final PlayerChangedDimensionEvent event) { PlayerEntity player = event.getPlayer(); PacketDispatcher.INSTANCE.sendTo(new PacketFirstSpawn(player), ((ServerPlayerEntity)player).connection.getNetworkManager(), NetworkDirection.PLAY_TO_CLIENT); PacketDispatcher.INSTANCE.sendTo(new PacketVillageC(player), ((ServerPlayerEntity)player).connection.getNetworkManager(), NetworkDirection.PLAY_TO_CLIENT); PacketDispatcher.INSTANCE.sendTo(new PacketClanC(player), ((ServerPlayerEntity)player).connection.getNetworkManager(), NetworkDirection.PLAY_TO_CLIENT); } @SubscribeEvent public static void respawnEvent(final PlayerRespawnEvent event) { PlayerEntity player = event.getPlayer(); PacketDispatcher.INSTANCE.sendTo(new PacketFirstSpawn(player), ((ServerPlayerEntity)player).connection.getNetworkManager(), NetworkDirection.PLAY_TO_CLIENT); PacketDispatcher.INSTANCE.sendTo(new PacketVillageC(player), ((ServerPlayerEntity)player).connection.getNetworkManager(), NetworkDirection.PLAY_TO_CLIENT); PacketDispatcher.INSTANCE.sendTo(new PacketClanC(player), ((ServerPlayerEntity)player).connection.getNetworkManager(), NetworkDirection.PLAY_TO_CLIENT); } } } Packet: public class PacketFirstSpawn { private boolean data; private static PlayerEntity player; public PacketFirstSpawn() {} public PacketFirstSpawn(PlayerEntity player) { PacketFirstSpawn.player = player; IPlayerBaseCap playercap = player.getCapability(PlayerBaseCapProvider.PLAYER_BASE_CAP).orElseThrow(() -> new RuntimeException("No player capability found!")); data = playercap.getFirstSpawn(); } public static void encode(PacketFirstSpawn msg, PacketBuffer buf) { buf.writeBoolean(msg.data); } public static PacketFirstSpawn decode(PacketBuffer buf) { return new PacketFirstSpawn(player); } public static void handle(PacketFirstSpawn msg, Supplier<NetworkEvent.Context> ctx) { ctx.get().enqueueWork(() -> { Main.proxy.handleClientBooleanPackets(0, msg.data); }); ctx.get().setPacketHandled(true); } } Proxy: public class ClientProxy extends CommonProxy { public void handleClientBooleanPackets(int id, boolean b) { IPlayerBaseCap basecap = Minecraft.getInstance().player.getCapability(PlayerBaseCapProvider.PLAYER_BASE_CAP).orElseThrow(() -> new RuntimeException("No player capability found!")); switch(id) { case 0: basecap.setFirstSpawn(b); } } public void handleClientIntPackets(int id, int i) { IPlayerBaseCap basecap = Minecraft.getInstance().player.getCapability(PlayerBaseCapProvider.PLAYER_BASE_CAP).orElseThrow(() -> new RuntimeException("No player capability found!")); switch(id) { case 0: basecap.setVillage(i); case 1: basecap.setClan(i); } } } What am I doing wrong? Also, oddly enough firstspawn doesn't even need syncing for some reason, its default value is true but even without syncing it on those events it stays false after it gets set to false.
-
I need to make all entities glow on the client side if the player has specific capabilities, however I do not want the client player to glow too. How could I do this? LivingUpdateEvent @SubscribeEvent public void onUpdate(LivingUpdateEvent event) { if (this.shouldGlow) { event.getEntityLiving().setGlowing(true); } else if (!event.getEntityLiving().isPotionActive(MobEffects.GLOWING)){ event.getEntityLiving().setGlowing(false); } }