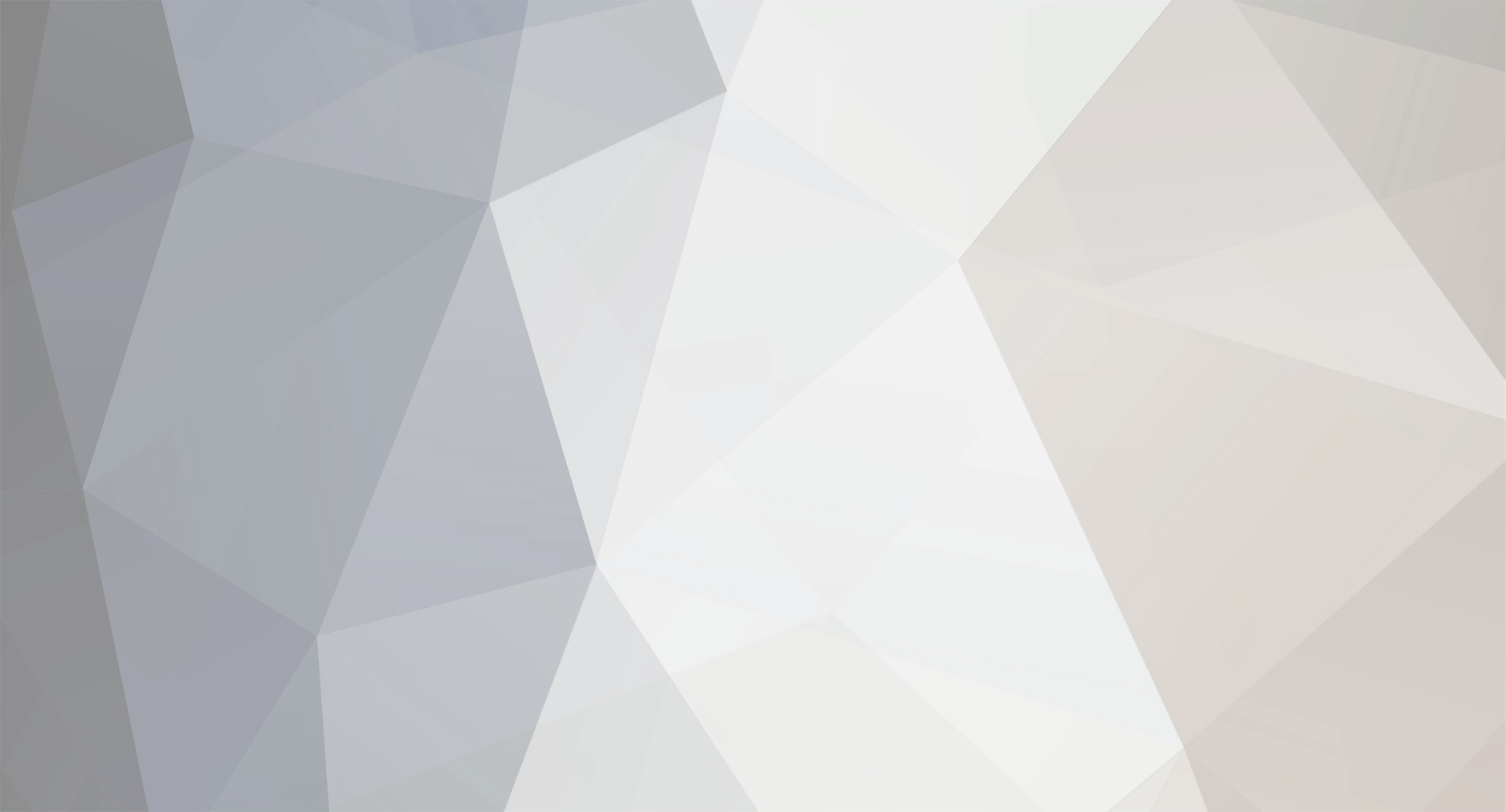
grillo781
-
Posts
12 -
Joined
-
Last visited
Posts posted by grillo781
-
-
fixed, thanks
-
I want to sync itemstacks saved in an entity between server and client when it spawn
package com.grillo78.BeyCraft.entity; import com.grillo78.BeyCraft.BeyRegistry; import net.minecraft.entity.CreatureEntity; import net.minecraft.entity.EntityType; import net.minecraft.entity.SharedMonsterAttributes; import net.minecraft.entity.item.ItemEntity; import net.minecraft.entity.player.PlayerEntity; import net.minecraft.item.ItemStack; import net.minecraft.nbt.CompoundNBT; import net.minecraft.network.PacketBuffer; import net.minecraft.network.datasync.DataParameter; import net.minecraft.network.datasync.DataSerializers; import net.minecraft.network.datasync.EntityDataManager; import net.minecraft.util.ActionResultType; import net.minecraft.util.Hand; import net.minecraft.util.math.Vec3d; import net.minecraft.world.World; import net.minecraftforge.fml.common.registry.IEntityAdditionalSpawnData; import net.minecraftforge.items.ItemStackHandler; /** * @author grillo78 * */ public class EntityBey extends CreatureEntity implements IEntityAdditionalSpawnData { private ItemStackHandler inventory = new ItemStackHandler(3); private int rotationDirection = 1; private static final DataParameter<Float> ROTATIONSPEED = EntityDataManager.createKey(EntityBey.class, DataSerializers.FLOAT); private static final DataParameter<Float> RADIUS = EntityDataManager.createKey(EntityBey.class, DataSerializers.FLOAT); public float angle = 0; private boolean increaseRadius = false; private boolean stoped = false; private float maxRotationSpeed = 5; /** * @param type * @param world */ public EntityBey(EntityType<? extends EntityBey> type, World world) { this(type, world,new ItemStack(BeyRegistry.STRIKEVALTRYEKV3),new ItemStack(BeyRegistry.SIXVDISK),new ItemStack(BeyRegistry.REBOOTDRIVER)); } public EntityBey(EntityType<? extends EntityBey> type, World world,ItemStack layer,ItemStack disk,ItemStack driver) { super(type,world); this.inventory = new ItemStackHandler(3); this.inventory.setStackInSlot(0, layer); this.inventory.setStackInSlot(1, disk); this.inventory.setStackInSlot(2, driver); } @Override protected void registerData() { this.dataManager.register(ROTATIONSPEED, 100F); this.dataManager.register(RADIUS, 1.5F); super.registerData(); } @Override protected void registerAttributes() { super.registerAttributes(); this.getAttribute(SharedMonsterAttributes.MAX_HEALTH).setBaseValue(16.0D); } @Override public void deserializeNBT(CompoundNBT nbt) { rotationDirection = nbt.getInt("RotationDirection"); setRotationSpeed(nbt.getFloat("RotationSpeed")); maxRotationSpeed = nbt.getFloat("MaxRotationSpeed"); inventory.deserializeNBT(nbt.getCompound("inventory")); super.deserializeNBT(nbt); } @Override public CompoundNBT serializeNBT() { CompoundNBT compound = new CompoundNBT(); compound.put("inventory", inventory.serializeNBT()); compound.putInt("RotationDirection", rotationDirection); compound.putFloat("MaxRotationSpeed", maxRotationSpeed); compound.putFloat("RotationSpeed", getRotationSpeed()); return compound; } @Override public void writeSpawnData(PacketBuffer buffer) { for (int i = 0; i < 3; i++) { buffer.writeItemStack(inventory.getStackInSlot(i)); } } @Override public void readSpawnData(PacketBuffer additionalData) { for (int i = 0; i < 3; i++) { this.inventory.setStackInSlot(i, additionalData.readItemStack()); } } @Override public void writeAdditional(CompoundNBT compound) { compound.put("inventory", inventory.serializeNBT()); compound.putInt("RotationDirection", rotationDirection); compound.putFloat("MaxRotationSpeed", maxRotationSpeed); compound.putFloat("RotationSpeed", getRotationSpeed()); super.writeAdditional(compound); } @Override public void readAdditional(CompoundNBT compound) { rotationDirection = compound.getInt("RotationDirection"); setRotationSpeed(compound.getFloat("RotationSpeed")); maxRotationSpeed = compound.getFloat("MaxRotationSpeed"); inventory.deserializeNBT(compound.getCompound("inventory")); super.readAdditional(compound); } @Override public ActionResultType applyPlayerInteraction(PlayerEntity player, Vec3d vec, Hand hand) { if (!world.isRemote && hand == Hand.MAIN_HAND) { world.addEntity(new ItemEntity(world, getPosition().getX(), getPosition().getY(), getPosition().getZ(), inventory.getStackInSlot(0))); world.addEntity(new ItemEntity(world, getPosition().getX(), getPosition().getY(), getPosition().getZ(), inventory.getStackInSlot(1))); world.addEntity(new ItemEntity(world, getPosition().getX(), getPosition().getY(), getPosition().getZ(), inventory.getStackInSlot(2))); this.remove(); return ActionResultType.SUCCESS; } return super.applyPlayerInteraction(player, vec, hand); } @Override public void tick() { if(getRotationSpeed()>0) { setRotationSpeed(getRotationSpeed() - 0.1f); } super.tick(); } public ItemStack getLayer() { return inventory.getStackInSlot(0); } public void setLayer(ItemStack layer) { inventory.setStackInSlot(0, layer); } public ItemStack getDisk() { return inventory.getStackInSlot(1); } public void setDisk(ItemStack disk) { inventory.setStackInSlot(1, disk); } public ItemStack getDriver() { return inventory.getStackInSlot(2); } public void setDriver(ItemStack driver) { inventory.setStackInSlot(2, driver); } public int getRotationDirection() { return rotationDirection; } public void setRotationDirection(int rotationDirection) { this.rotationDirection = rotationDirection; } public final float getRadius() { return ((Float) this.dataManager.get(RADIUS)).floatValue(); } public void setRadius(float radius) { this.dataManager.set(RADIUS, Float.valueOf(radius)); } public void setRotationSpeed(float speed) { this.dataManager.set(ROTATIONSPEED, Float.valueOf(speed)); } public final float getRotationSpeed() { return ((Float) this.dataManager.get(ROTATIONSPEED)).floatValue(); } }
-
I have a block with custom collision that have a floor and walls, I want to know then an entity collide with a wall to change the entity rotationYaw.
How can I do to check the wall collision only?
-
and are some tutorial that I can follow to do a collision similar to the stair collision but horizontal? I try to understand the stair collision but I wasn't able
-
Okay, thanks for the replies
-
-
2 hours ago, diesieben07 said:
The client-side entity will always use the default constructor. If you need additional data to be synced to the client you can use IEntityAdditionalSpawnData (sent only once on spawning) or EntityDataManager (kept in sync all the time).
Fixed, thx u
-
when I spawn an entity with custom constructor using an item it use the values of the default constructor, this is the entity class:
public class EntityBey extends EntityCreature{ public ItemStack layer; public ItemStack disk; public ItemStack driver; public EntityBey(World worldIn) { this(worldIn, new ItemStack(BeyRegistry.ACHILLESA4), new ItemStack(BeyRegistry.ELEVENDISK), new ItemStack(BeyRegistry.XTENDDRIVER)); } public EntityBey(World worldIn, ItemStack layerIn, ItemStack diskIn, ItemStack driverIn) { super(worldIn); this.setSize(0.25F, 0.25F); this.height = 0.253F; layer = layerIn; disk = diskIn; driver = driverIn; } @Override public IEntityLivingData onInitialSpawn(DifficultyInstance difficulty, IEntityLivingData livingdata) { this.setEquipmentBasedOnDifficulty(difficulty); return super.onInitialSpawn(difficulty, livingdata); } @Override protected void applyEntityAttributes() { super.applyEntityAttributes(); this.getEntityAttribute(SharedMonsterAttributes.MAX_HEALTH).setBaseValue(100); this.getEntityAttribute(SharedMonsterAttributes.MOVEMENT_SPEED).setBaseValue(1D); } @Override protected void initEntityAI() { this.tasks.addTask(0, new EntityAIWander(this, 1.0D)); // this.tasks.addTask(0, new EntityAIAttackMelee(this, 1, true)); super.initEntityAI(); } public float getEyeHeight() { return this.height-0.1F; } @Override public void onRemovedFromWorld() { BeyCraft.logger.info("Bey die"); super.onRemovedFromWorld(); } @Override protected boolean canDespawn() { return false; } @Override protected SoundEvent getDeathSound() { return SoundHandler.BEY_HIT; } @Override protected SoundEvent getHurtSound(DamageSource damageSourceIn) { return SoundHandler.BEY_HIT; } protected void setEquipmentBasedOnDifficulty(DifficultyInstance difficulty) { this.setItemStackToSlot(EntityEquipmentSlot.MAINHAND, new ItemStack(Items.STONE_SWORD)); } @Override public void readEntityFromNBT(NBTTagCompound compound) { layer = new ItemStack(compound.getCompoundTag("Layer")); disk = new ItemStack(compound.getCompoundTag("Disk")); driver = new ItemStack(compound.getCompoundTag("Driver")); super.readEntityFromNBT(compound); } @Override public void writeEntityToNBT(NBTTagCompound compound) { compound.setTag("Layer", layer.writeToNBT(new NBTTagCompound())); compound.setTag("Disk", disk.writeToNBT(new NBTTagCompound())); compound.setTag("Driver", driver.writeToNBT(new NBTTagCompound())); super.writeEntityToNBT(compound); } }
and this the item class:
public class ItemLauncher extends Item{ public ItemLauncher(String name) { setCreativeTab(BeyCraft.beyCraftTab); setRegistryName(name); setUnlocalizedName(name); setMaxStackSize(1); BeyRegistry.ITEMS.add(this); } @Override public ICapabilityProvider initCapabilities(ItemStack stack, NBTTagCompound nbt) { if( stack.getItem() == this ) { return new BeyBladeProvider(); } return null; } @Override public ActionResult<ItemStack> onItemRightClick(World worldIn, EntityPlayer playerIn, EnumHand handIn) { if (!worldIn.isRemote) { if (playerIn.isSneaking()) { playerIn.openGui(BeyCraft.instance, 0, worldIn, 0, 0, 0); } else { EntityBey beyEntity = new EntityBey(worldIn, new ItemStack(BeyRegistry.ACHILLESA4), new ItemStack(BeyRegistry.BOOSTDISK), new ItemStack(BeyRegistry.VOLCANICDRIVER)); beyEntity.setLocationAndAngles(playerIn.posX, playerIn.posY, playerIn.posZ, 0, 0); worldIn.spawnEntity(beyEntity); } } return super.onItemRightClick(worldIn, playerIn, handIn); } }
-
finally it works fine, thanks you @DavidM
-
8 minutes ago, DavidM said:
1. Stop using IHasModel.
2. What is this NBT even for? You are not doing anything with it.
3. You never serialized the ItemStackHandler to NBT in your BeyBladeProvider, thus the content is not saved.
2. I will delete, I forgot it.
3. How do I need serialize it?
-
I'm having this issue: https://youtu.be/Uxr60WOhEOE
here my classes:
I want that the inventory doesn't be empty after drop it.
How to set a ISTER[1.15.2]
in Modder Support
Posted
I want to know how can I use a ISTER because the documentation in forge page is outdated
Item class:
https://github.com/grillo78/BeyCraft/blob/master/src/main/java/com/grillo78/BeyCraft/items/ItemBeyLayer.java
ISTER class:
https://github.com/grillo78/BeyCraft/blob/master/src/main/java/com/grillo78/BeyCraft/items/render/BeyItemStackRendererTileEntity.java
Json:
https://github.com/grillo78/BeyCraft/blob/master/src/main/resources/assets/beycraft/models/item/valtryekv2.json