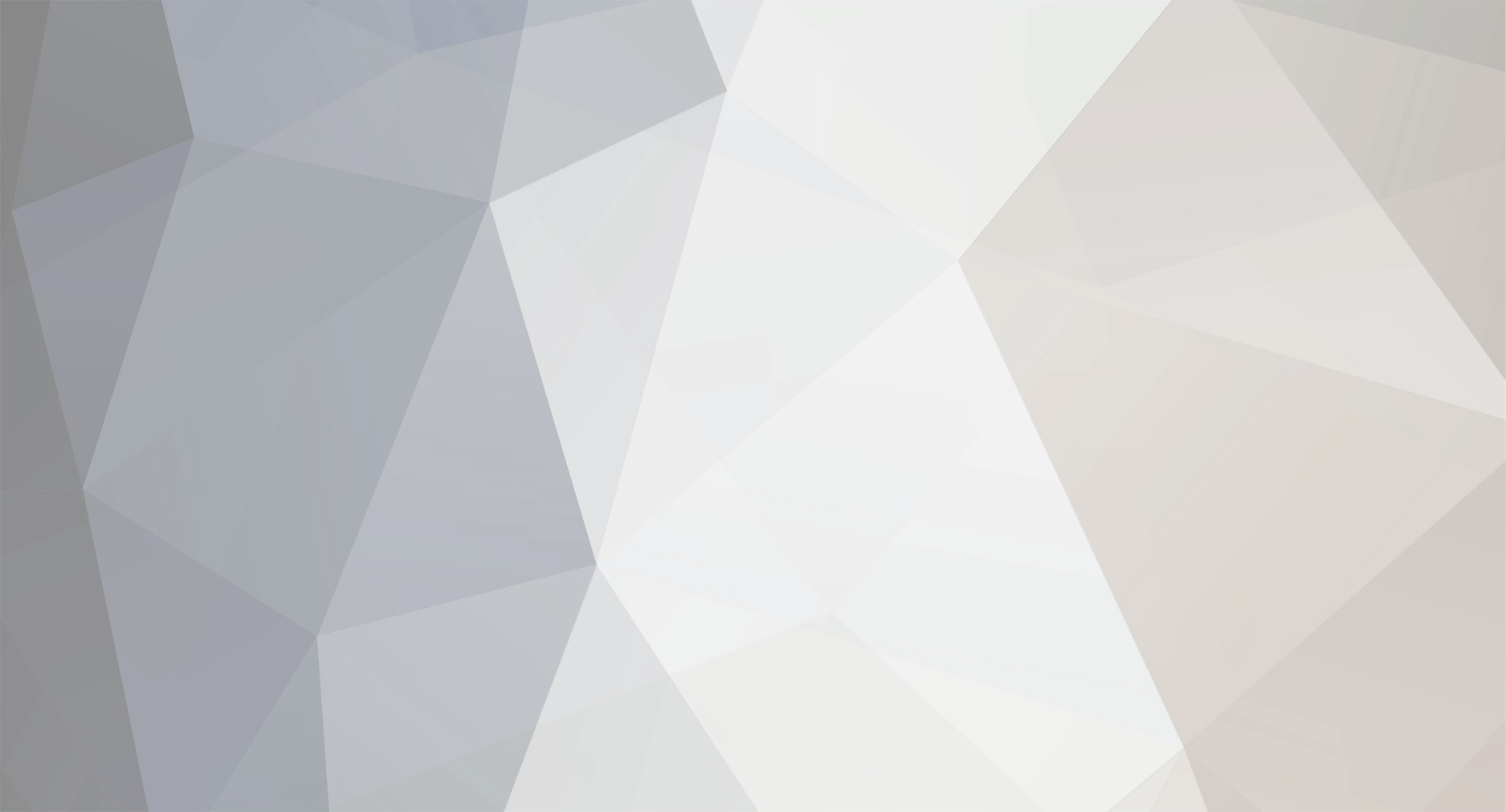
cherrylime
-
Posts
13 -
Joined
-
Last visited
Posts posted by cherrylime
-
-
How would i make a falling block out of this block class? I have tried extending FallingBlock instead of BlockBase, but then the
super(name, material)
gets messed up.
Here is my block class:
Spoilerpackage com.artemaniac.islands.blocks; import net.minecraft.block.BlockFalling; import net.minecraft.block.SoundType; import net.minecraft.block.material.Material; import net.minecraft.init.Blocks; public class BeachSand extends BlockBase { public BeachSand(String name, Material material) { super(name, material); setSoundType(SoundType.SAND); setHardness(0.5F); setResistance(2.5F); setHarvestLevel("spade", 0); } }
Thanks in advance!
-
Thank you! It worked!
-
Quote
There is a code block button looks like this <> and a spoiler block button, please use them.
Sorry, they aren't showing up in my reply screen, only in my post screen. I'm new to forge forums
-
Here is the FireGen code:
package com.cherrylime.minecraftimproved.gen;
import java.util.Random;
import com.cherrylime.minecraftimproved.blocks.BlockBase;
import com.cherrylime.minecraftimproved.init.ModBlocks;import net.minecraft.util.math.BlockPos;
import net.minecraft.world.World;
import net.minecraft.world.chunk.IChunkProvider;
import net.minecraft.world.gen.IChunkGenerator;
import net.minecraft.world.gen.feature.WorldGenMinable;
import net.minecraft.world.gen.feature.WorldGenerator;
import net.minecraftforge.fml.common.IWorldGenerator;public class FireOreGen implements IWorldGenerator
{
private WorldGenerator fire_ore;
public FireOreGen()
{
fire_ore = new WorldGenMinable(ModBlocks.FIRE_ORE.getDefaultState(), 9);
}
@Override
public void generate(Random random, int chunkX, int chunkZ, World world, IChunkGenerator chunkGenerator,
IChunkProvider chunkProvider) {
switch(world.provider.getDimension())
{
case 0:
runGenerator(fire_ore, world, random, chunkX, chunkZ, 10, 0, 50);
break;
case 1:
break;
case -1:
break;
}
}
private void runGenerator(WorldGenerator gen, World world, Random rand, int chunkX, int chunkZ, int chance, int minHeight, int maxHeight)
{
if(minHeight > maxHeight || minHeight < 0 || maxHeight > 256) throw new IllegalArgumentException("Ore generated off limits");
int heightDiff = maxHeight - minHeight + 1;
for(int i = 0; i < chance; i++)
{
int x = chunkX * 16 + rand.nextInt(16);
int y = minHeight + rand.nextInt(heightDiff);
int z = chunkZ * 16 + rand.nextInt(16);
gen.generate(world, rand, new BlockPos(x, y, z));
}
}
}
And here is the WaterGen code:
package com.cherrylime.minecraftimproved.gen;
import java.util.Random;
import com.cherrylime.minecraftimproved.blocks.BlockBase;
import com.cherrylime.minecraftimproved.init.ModBlocks;import net.minecraft.util.math.BlockPos;
import net.minecraft.world.World;
import net.minecraft.world.chunk.IChunkProvider;
import net.minecraft.world.gen.IChunkGenerator;
import net.minecraft.world.gen.feature.WorldGenMinable;
import net.minecraft.world.gen.feature.WorldGenerator;
import net.minecraftforge.fml.common.IWorldGenerator;public class WaterOreGen implements IWorldGenerator
{
private WorldGenerator water_ore;
public WaterOreGen()
{
water_ore = new WorldGenMinable(ModBlocks.WATER_ORE.getDefaultState(), 9);
}
@Override
public void generate(Random random, int chunkX, int chunkZ, World world, IChunkGenerator chunkGenerator,
IChunkProvider chunkProvider) {
switch(world.provider.getDimension())
{
case 0:
runGenerator(water_ore, world, random, chunkX, chunkZ, 10, 0, 50);
break;
case 1:
break;
case -1:
break;
}
}
private void runGenerator(WorldGenerator gen, World world, Random rand, int chunkX, int chunkZ, int chance, int minHeight, int maxHeight)
{
if(minHeight > maxHeight || minHeight < 0 || maxHeight > 256) throw new IllegalArgumentException("Ore generated off limits");
int heightDiff = maxHeight - minHeight + 1;
for(int i = 0; i < chance; i++)
{
int x = chunkX * 16 + rand.nextInt(16);
int y = minHeight + rand.nextInt(heightDiff);
int z = chunkZ * 16 + rand.nextInt(16);
gen.generate(world, rand, new BlockPos(x, y, z));
}
}
}
-
In my main mod file with my ore generation order, this is what the code looks like.
@EventHandler public static void init(FMLInitializationEvent event) { GameRegistry.registerWorldGenerator(new FireOreGen(), 0); GameRegistry.registerWorldGenerator(new WaterOreGen(), 0); }
The problem is, only the WaterOreGen works. When i put the FireOreGen line after the WaterOreGen line, only the FireOreGen works.
Am I supposed to somehow generate multiple types of ores in one Generator? Or am I doing something wrong in my main mod file? Thanks in advance!
-
When I am creating a new world in the Eclipse client, it gets stuck on Loading World, Building Terrain. I have two ores that should be generating in my world. Is the problem happening because the two ores have the same chance? Thanks in advance!
-
Thank you so much! It works now!
-
I have changed my code to this:
{
"type": "minecraft:crafting_shaped",
"pattern": [
" A ",
"ASA",
" A "
],
"key": {
"S": {
"item": "minecraft:bone"
},
"A": {
"item": "minecraft:cooked_beef"
}
}
"result": {
"item": "im:pet_treat",
"data": 0
}
}However no result shows up. Do I need to register this crafting recipe on a different class or interface?
-
I'm sorry, I don't know much about modding. How do I do that?
-
I changed the code to this to make the JSON valid, but it still is not working in the game.
{
"type": "minecraft:crafting_shaped",
"pattern": [
" A ",
"ASA",
" A "
],
"key": {
"S": {
"item": "minecraft:bone"
},
"A": {
"item": "minecraft:cooked_beef"
},
"result": {
"item": "im:pet_treat",
"data": 0
}
}
} -
Here is my new code. It still does not work. Do I have to do anything to register the crafting recipe?
{
"type": "minecraft:crafting_shaped",
"pattern": [
" A ",
"ASA",
" A "
],
"key": {
"S": {
"item": "minecraft:bone"
},
"A": [
{
"item": "minecraft:cooked_beef"
},
]
},
"result": {
"item": "im:pet_treat",
"data": 0
}
} -
When I Run the client, and put the items in the correct order in the crafting table, nothing comes out at all! Here is my json file:
{ "type": "minecraft:crafting_shaped", "pattern": [ " A ", "ASA", " A " ], "key": { "S": { "item": "minecraft:bone" }, "A": [ { "item": "minecraft:cooked_beef" }, ] }, "result": { "item": "im:pet_treat" } }
im:pet_treat is an item id in the game. I tested this with other items and blocks and even changed the result but it still didn't work. Can anyone help?
[SOLVED] Falling Blocks?
in Modder Support
Posted · Edited by cherrylime
I just don't know what class I should extend or what I should do. This is not a problem with not knowing java, it's just not knowing forge, which is what this forum is about.