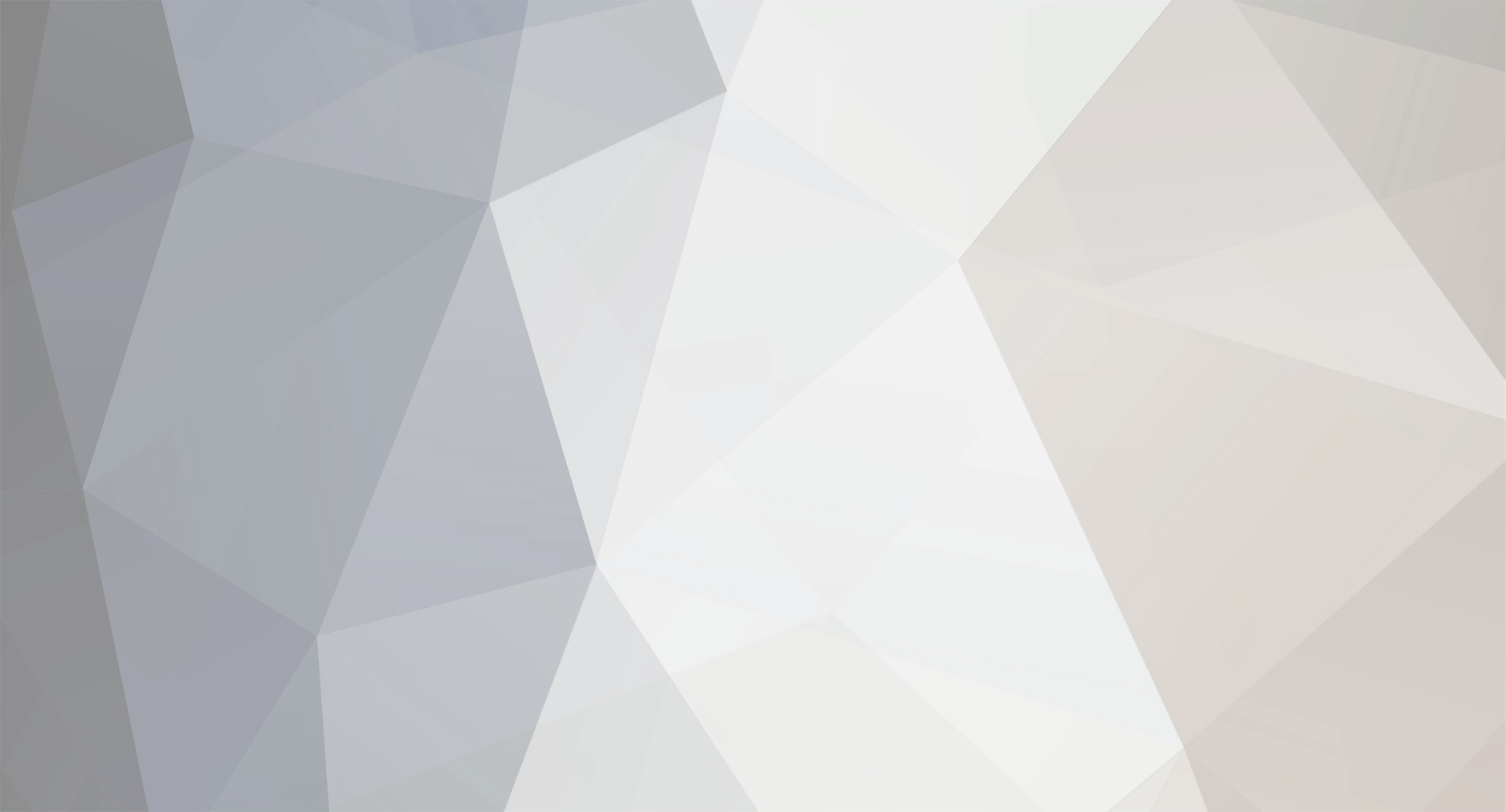
Lumby
Members-
Posts
50 -
Joined
-
Last visited
Everything posted by Lumby
-
It's cutting out the hair plume portion of my texture.
-
I made a helmet with a custom model. The helmet correctly renders the texture and custom model portion designed for it, but has some... extra stuff tacked on. Picture: As the picture shows, the hair plume (mohawk) renders correctly, along with the intended helmet texture. There seems to however be another larger box(that I did not intend) rendered, and it is cutting into the hair plume texture png and rendering parts of said hair plume texture onto the larger box covering the head. Any idea how I can remove it? my model class: public class BestialArmorModel extends ModelBiped { public ModelRenderer head; public ModelRenderer mohawk; public BestialArmorModel(float f) { super(f, 0, 64, 32); textureWidth = 64; textureHeight = 32; mohawk = new ModelRenderer(this, 32, 0); mohawk.setRotationPoint(0F, 0F, 0F); mohawk.addBox(-0.5F, -10.5F, -7.8F, 1, 5, 14, 0.0F); mohawk.setTextureSize(64, 32); mohawk.mirror=true; head = new ModelRenderer(this, 0, 0); head.setRotationPoint(0.0F, 0.0F, 0.0F); head.addBox(-4.0F, -8.0F, -4.0F, 8, 8, 8, 0.0F); head.setTextureSize(64, 32); head.mirror=false; bipedHead.addChild(head); bipedHead.addChild(mohawk); } @Override public void render(Entity entityIn, float limbSwing, float limbSwingAmount, float ageInTicks, float netHeadYaw, float headPitch, float scale) { super.render(entityIn, limbSwing, limbSwingAmount, ageInTicks, netHeadYaw, headPitch, scale); setRotationAngles(limbSwing, limbSwingAmount, ageInTicks, netHeadYaw, headPitch, scale, entityIn); } public void setRotateAngle(ModelRenderer modelRenderer, float x, float y, float z) { modelRenderer.rotateAngleX = x; modelRenderer.rotateAngleY = y; modelRenderer.rotateAngleZ = z; } } getArmorModel method in the armor class: @SideOnly(Side.CLIENT) @Override public ModelBiped getArmorModel(EntityLivingBase entityLiving, ItemStack itemStack, EntityEquipmentSlot armorSlot, ModelBiped _default) { if(!itemStack.isEmpty()) { if(itemStack.getItem() instanceof ItemArmor) { BestialArmorModel armorModel = new BestialArmorModel(0.25f); armorModel.bipedHead.showModel = true; armorModel.isSneak = _default.isSneak; armorModel.isRiding = _default.isRiding; armorModel.isChild = _default.isChild; armorModel.rightArmPose = _default.rightArmPose; armorModel.leftArmPose = _default.leftArmPose; return armorModel; } } return null; } the texture png:
-
[Solved] Attribute Not Removed When Armor is Removed
Lumby replied to Lumby's topic in Modder Support
I've been trying to use the onArmorTick() method but it yielded the same result, being that the player's attribute map remains unchanged. In the end, I traced the call hierarchy of getItemAttributeModifiers() and found that it utilized specific UUIDs to determine whether or not remove the new AttributeModifier passed into the map. Hence, instead of using multimap.put(SharedMonsterAttributes.MOVEMENT_SPEED.getName(), new AttributeModifier(SharedMonsterAttributes.MOVEMENT_SPEED.getName(), this.speedBoost, 1)); I added the armorModifier UUIDs in the ItemArmor class and it did the trick multimap.put(SharedMonsterAttributes.MOVEMENT_SPEED.getName(), new AttributeModifier(ARMOR_MODIFIERS[equipmentSlot.getIndex()],"Daegonite Tank", speedBoost, 1)); -
[Solved] Attribute Not Removed When Armor is Removed
Lumby replied to Lumby's topic in Modder Support
Got it, thanks for your help! lmao got it, will go ahead and fix that. Followed some dude's tutorial and he had it in so I thought this was the most efficient way. What does cargo cult mean? -
I made a type of armor that modifies the player speed attribute. The armor successfully adds the value to the player attribute, but when I take off the armor in game, the added value is not removed. How can I make it so that the attribute modifier is only applied while the armor is worn? Thanks in advance. My custom armor class: public class DaegoniteArmor extends ItemArmor implements IHasModel{ public final double speedBoost = 0.5F; public DaegoniteArmor(String name, ArmorMaterial materialIn, int renderIndexIn, EntityEquipmentSlot equipmentSlotIn) { super(materialIn, renderIndexIn, equipmentSlotIn); setUnlocalizedName(name); setRegistryName(name); setCreativeTab(CreativeTabs.COMBAT); ModItems.ITEMS.add(this); } @Override public void registerModels() { Main.proxy.registerItemRenderer(this,0, "inventory"); } @Override public boolean getIsRepairable(ItemStack toRepair, ItemStack repair) { return false; } /** * Gets a map of item attribute modifiers, used by ItemSword to increase hit damage. */ @Override public Multimap<String, AttributeModifier> getItemAttributeModifiers(EntityEquipmentSlot equipmentSlot) { Multimap<String, AttributeModifier> multimap = super.getItemAttributeModifiers(equipmentSlot); if (equipmentSlot == this.armorType) { multimap.put(SharedMonsterAttributes.MOVEMENT_SPEED.getName(), new AttributeModifier(SharedMonsterAttributes.MOVEMENT_SPEED.getName(), this.speedBoost, 1)); } return multimap; } }
-
Can you paste your code on here so we may see what might've gone wrong? It's a pretty common problem but there are several possible reasons for this to occur and we won't be able to know for sure unless we see all your java and json files relating to your block.
-
How do I create custom loot tables for vanilla mobs? 1.12.2
Lumby replied to M1st3rMinecraft's topic in Modder Support
My best guess would be to subscribe to the LivingDropsEvent in an eventHandler and change the item drops there, since you can't modify vanilla functionality. Read https://mcforge.readthedocs.io/en/latest/events/intro/ to get started. -
I've found out the problem. The problem was that my InventoryArkenstoneResult class had an outdated decrStackSize, since I was following a tutorial from older forge versions. The new one can pulled from the InventoryCraftingResult class if anyone else encounters this problem in the future. public ItemStack decrStackSize(int index, int count) { return ItemStackHelper.getAndRemove(this.stackResult, 0); }
-
My initial guess was that since items automatically crafted, when the output slot was clicked it checked the inputInventory again to find nothing and hence reset the output to ItemStack.Empty. After an afternoon in the debug perspective, however, I feel like the problem isn't within the onCraftingMatrixChanged() method, as it isn't called when I take items out of the slot. Any ideas what else may be a possible cause?
-
I have a block that works sort of like a crafting table in that it uses no tileEntities and instantly crafts something out of InventoryCrafting slots. After the crafting result appears, however, although I can shift click the result into my inventory without problems, I am unable to left click the craft result Itemstack as it just vanishes. Is this a result of the code being on the wrong side? If so, should the code in onCraftMatrixChanged() be on the server or client side? Thanks in advance! Container class: public class ContainerArkenstoneTable extends Container{ public InventoryCrafting inputInventory = new InventoryCrafting(this, 3, 1); public int inputSlotNumber; public InventoryArkenstoneResult outputInventory = new InventoryArkenstoneResult(); public ArkenstoneRecipeHandler arkenstoneRecipeHandler; private final World world; private final BlockPos pos; private final InventoryPlayer playerInventory; public ContainerArkenstoneTable(InventoryPlayer playerInventory, World worldIn, BlockPos posIn){ this.world = worldIn; this.pos = posIn; this.playerInventory = playerInventory; arkenstoneRecipeHandler = new ArkenstoneRecipeHandler(); this.addSlotToContainer(new NoInputSlot(outputInventory, 0, 124, 31)); this.addSlotToContainer(new DiamondOnlySlot(inputInventory, 0, 26 + 0 * 18, 9 + 18)); this.addSlotToContainer(new Slot(inputInventory, 1, 44 + 1 * 18, 9 + 18)); this.addSlotToContainer(new Slot(inputInventory, 2, 44 + 1 * 18, 13 + 2*18)); for (int k = 0; k < 3; ++k){ for (int i1 = 0; i1 < 9; ++i1){ this.addSlotToContainer(new Slot(playerInventory, i1 + k * 9 + 9, 8 + i1 * 18, 84 + k * 18)); } } for (int l = 0; l < 9; ++l){ this.addSlotToContainer(new Slot(playerInventory, l, 8 + l * 18, 142)); } } /** * Callback for when the crafting matrix is changed. */ public void onCraftMatrixChanged(IInventory inventoryIn){ //if the inventory selected is actually input inventory if(inventoryIn == inputInventory){ //if this thing is empty, stahp while(!inputInventory.isEmpty()) { ItemStack outputItemStack = arkenstoneRecipeHandler.getArkenstoneResults(inputInventory); //if this input crafts nothing if (outputItemStack == ItemStack.EMPTY ){ return; }else {//if this input can craft something //if something is already lingering in outputInventory from before if(!outputInventory.isEmpty()){ //if what is supposed to be in output is NOT the same as the thing actually in output if(!outputItemStack.isItemEqual(outputInventory.getStackInSlot(0))){ //Add what is supposed to go into output inventory to player inventory //automatically, or drop it on the ground if(!playerInventory.addItemStackToInventory(outputItemStack)){ EntityItem entityItem = playerInventory.player.entityDropItem(outputItemStack, 0.5f); entityItem.posX = playerInventory.player.posX; entityItem.posY = playerInventory.player.posY; entityItem.posZ = playerInventory.player.posZ; } //clear the output inventory outputInventory.setInventorySlotContents(0, ItemStack.EMPTY); } }//at this point output inventory of a different yield should be cleared //get current item stack in case it's the same item causing the segment before to not clear it ItemStack currentStack = outputInventory.getStackInSlot(0); //Test to see if different type of dye by getting item damage int metadata = outputItemStack.getItemDamage(); if(metadata == 32767){ metadata = 0; } ItemStack newStack = ItemStack.EMPTY; //Output Slot already has the item we're trying to craft, just tack on more of it if(!currentStack.isEmpty() && 1 + currentStack.getCount() <= outputItemStack.getMaxStackSize()){ newStack = new ItemStack(outputItemStack.getItem(), 1 + currentStack.getCount(), metadata); }else{ //if output slot is already empty OR output slot can't handle the amount we're crafting //if output slot already has the item we're trying to craft, but just couldn't fit so much if(!currentStack.isEmpty() && !playerInventory.addItemStackToInventory(currentStack)){ EntityItem entityItem = playerInventory.player.entityDropItem(currentStack, 0.5f); entityItem.posX = playerInventory.player.posX; entityItem.posY = playerInventory.player.posY; entityItem.posZ = playerInventory.player.posZ; }else{ newStack = new ItemStack(outputItemStack.getItem(), 1, metadata); } } outputInventory.setInventorySlotContents(0, newStack); //removes the contents from input inventory after crafting is completed inputInventory.decrStackSize(0,1); inputInventory.decrStackSize(1,1); inputInventory.decrStackSize(2,1); } }//while loop ends } } /** * Called when the container is closed. */ @Override public void onContainerClosed(EntityPlayer playerIn) { InventoryPlayer inventoryplayer = playerIn.inventory; if(!inventoryplayer.getItemStack().isEmpty()) { playerIn.dropItem(inventoryplayer.getItemStack(), false); inventoryplayer.setItemStack(ItemStack.EMPTY); } if(!world.isRemote) { ItemStack itemStack = outputInventory.getStackInSlot(0); if(!itemStack.isEmpty()){ if(!inventoryplayer.addItemStackToInventory(itemStack)) { playerIn.dropItem(itemStack, false); } } for(int i = 0; i < inputInventory.getSizeInventory(); i++ ){ itemStack = inputInventory.getStackInSlot(i); if(!itemStack.isEmpty()){ if(!inventoryplayer.addItemStackToInventory(itemStack)) { playerIn.dropItem(itemStack, false); } } } } } /** * Determines whether supplied player can use this container */ @Override public boolean canInteractWith(EntityPlayer playerIn) { if (this.world.getBlockState(this.pos).getBlock() != ModBlocks.ArkenstoneTableBlock) { return false; } else { return playerIn.getDistanceSq((double)this.pos.getX() + 0.5D, (double)this.pos.getY() + 0.5D, (double)this.pos.getZ() + 0.5D) <= 64.0D; } } /** * Handle when the stack in slot {@code index} is shift-clicked. Normally this moves the stack between the player * inventory and the other inventory(s). */ @Override public ItemStack transferStackInSlot(final EntityPlayer player, final int index) { return ModUtils.transferStackInSlot(player, index, this); } /** * Called to determine if the current slot is valid for the stack merging (double-click) code. The stack passed in * is null for the initial slot that was double-clicked. */ public boolean canMergeSlot(ItemStack stack, Slot slotIn){ return slotIn.inventory != this.outputInventory && super.canMergeSlot(stack, slotIn); } } ModUtils class with methods that the above class references: public class ModUtils { public static ItemStack transferStackInSlot(final EntityPlayer player, final int index, final Container container) { ItemStack itemstack = ItemStack.EMPTY; final Slot slot = container.inventorySlots.get(index); if ((slot != null) && slot.getHasStack()) { final ItemStack itemstack1 = slot.getStack(); itemstack = itemstack1.copy(); final int containerSlots = container.inventorySlots.size() - player.inventory.mainInventory.size(); if (index < containerSlots) { if (!mergeItemStack(itemstack1, containerSlots, container.inventorySlots.size(), true, container)) { return ItemStack.EMPTY; } } else if (!mergeItemStack(itemstack1, 0, containerSlots, false, container)) { return ItemStack.EMPTY; } if (itemstack1.getCount() == 0) { slot.putStack(ItemStack.EMPTY); } else { slot.onSlotChanged(); } if (itemstack1.getCount() == itemstack.getCount()) { return ItemStack.EMPTY; } slot.onTake(player, itemstack1); } if(container instanceof ContainerArkenstoneTable) { container.onCraftMatrixChanged(((ContainerArkenstoneTable) container).inputInventory); } return itemstack; } public static boolean mergeItemStack(final ItemStack stack, final int startIndex, final int endIndex, final boolean reverseDirection, final Container container) { boolean flag = false; int i = startIndex; if (reverseDirection) { i = endIndex - 1; } if (stack.isStackable()) { while (!stack.isEmpty()) { if (reverseDirection) { if (i < startIndex) { break; } } else if (i >= endIndex) { break; } final Slot slot = container.inventorySlots.get(i); final ItemStack itemstack = slot.getStack(); if (!itemstack.isEmpty() && (itemstack.getItem() == stack.getItem()) && (!stack.getHasSubtypes() || (stack.getMetadata() == itemstack.getMetadata())) && ItemStack.areItemStackTagsEqual(stack, itemstack)) { final int j = itemstack.getCount() + stack.getCount(); final int maxSize = Math.min(slot.getSlotStackLimit(), stack.getMaxStackSize()); if (j <= maxSize) { stack.setCount(0); itemstack.setCount(j); slot.onSlotChanged(); flag = true; } else if (itemstack.getCount() < maxSize) { stack.shrink(maxSize - itemstack.getCount()); itemstack.setCount(maxSize); slot.onSlotChanged(); flag = true; } } if (reverseDirection) { --i; } else { ++i; } } } if (!stack.isEmpty()) { if (reverseDirection) { i = endIndex - 1; } else { i = startIndex; } while (true) { if (reverseDirection) { if (i < startIndex) { break; } } else if (i >= endIndex) { break; } final Slot slot1 = container.inventorySlots.get(i); final ItemStack itemstack1 = slot1.getStack(); if (itemstack1.isEmpty() && slot1.isItemValid(stack)) { if (stack.getCount() > slot1.getSlotStackLimit()) { slot1.putStack(stack.splitStack(slot1.getSlotStackLimit())); } else { slot1.putStack(stack.splitStack(stack.getCount())); } slot1.onSlotChanged(); flag = true; break; } if (reverseDirection) { --i; } else { ++i; } } } return flag; } } ArkenstoneRecipeHandler class package com.crumbletheundead.blocks.machines.ArkenstoneTable; import net.minecraft.init.Items; import net.minecraft.inventory.InventoryCrafting; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; public class ArkenstoneRecipeHandler { //items put into the crafting grid private boolean hasEmpty, hasDiamond, hasBone; public ArkenstoneRecipeHandler() { } public ItemStack getArkenstoneResults(InventoryCrafting inputInventory) { hasEmpty = false; hasDiamond = false; hasBone = false; //test if first slot has diamonds if(inputInventory.getStackInSlot(0).getItem().equals(Items.DIAMOND)) { hasDiamond = true; } //go through the other two slots to see if they have any items required for(int i = 1; i<= inputInventory.getSizeInventory()-1; i++) { if(inputInventory.getStackInSlot(i).isEmpty()) { hasEmpty = true; }else if(inputInventory.getStackInSlot(i).getItem().equals(Items.BONE)) { hasBone = true; } } if(hasDiamond && hasBone && hasEmpty) { return new ItemStack(Items.APPLE); }else { return ItemStack.EMPTY; } } } InventoryArkenstone class package com.crumbletheundead.blocks.machines.ArkenstoneTable; import javax.annotation.Nullable; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.inventory.IInventory; import net.minecraft.inventory.ItemStackHelper; import net.minecraft.item.ItemStack; import net.minecraft.item.crafting.IRecipe; import net.minecraft.util.NonNullList; import net.minecraft.util.text.ITextComponent; import net.minecraft.util.text.TextComponentString; import net.minecraft.util.text.TextComponentTranslation; public class InventoryArkenstoneResult implements IInventory{ private final NonNullList<ItemStack> stackResult = NonNullList.<ItemStack>withSize(1, ItemStack.EMPTY); /** * Returns the number of slots in the inventory. */ @Override public int getSizeInventory() { return 1; } /** * Returns the stack in slot i */ @Override public ItemStack getStackInSlot(int index) { return this.stackResult.get(0); } @Override public boolean isEmpty() { for (ItemStack itemstack : this.stackResult) { if (!itemstack.isEmpty()) { return false; } } return true; } @Override public String getName() { return "Arkenstone Result"; } @Override public boolean hasCustomName() { return false; } @Override public ITextComponent getDisplayName() { return new TextComponentTranslation(this.getName(), new Object[0]); } @Override public ItemStack decrStackSize(int index, int count) { if(this.stackResult.get(index) != null) { ItemStack itemstack = this.stackResult.get(index); this.stackResult.get(index).setCount(0);; return itemstack; } else { return ItemStack.EMPTY; } } @Override public ItemStack removeStackFromSlot(int index) { return ItemStackHelper.getAndRemove(this.stackResult, 0); } @Override public void setInventorySlotContents(int index, ItemStack stack) { this.stackResult.set(0, stack); } @Override public int getInventoryStackLimit() { return 64; } @Override public boolean isUsableByPlayer(EntityPlayer player) { return true; } @Override public void openInventory(EntityPlayer player) { } @Override public void closeInventory(EntityPlayer player) { } @Override public boolean isItemValidForSlot(int index, ItemStack stack) { return false; } public int getField(int id) { return 0; } public void setField(int id, int value) { } public int getFieldCount() { return 0; } public void clear() { this.stackResult.clear(); } @Override public void markDirty() { } }
-
The vanilla ContainerWorkbench class. I was following Jabelar's tutorial: http://jabelarminecraft.blogspot.com/p/blog-page_31.html and it had these methods in his container class, but they were outdated. Hence, I pulled it from the workbench class hoping they'd work.
-
So I'm guessing some method tried to reference the slots by calling inputInventory[3] since the error was arraysOutOfBounds: 3, my question is what method might've called that? And yeah that's just eclipse dark mode haha.
-
Thanks for the help! Sorry this is my first time using an eclipse debugger, but from what I can tell, this means I did indeed create three Slot() objects for my inputInventory. Any idea why minecraft is still crashing? My breakpoint is set right after their creation:
-
Eclipse
-
Sorry, I meant what might've called those inventory slots that I created, so that I can find how it induced the crash. My guess is since it's an out of bounds exception, it might have something to do with the index I created the slots with, so I want to see what methods called the slots and how it called them.
-
Any idea where that request might come from?
-
I am making a custom crafting table but whenever I try to shift click something out of an InventoryCrafting slot, minecraft crashes due to java.lang.ArrayIndexOutOfBounds: 3 and java.langIndexOutOfBounds: index40, Size 40 exceptions. The inventoryCrafting slot I used is from vanilla, so I don't know what caused the crash. Any ideas? Container class: public class ContainerArkenstoneTable extends Container{ public InventoryCrafting inputInventory = new InventoryCrafting(this, 3, 1); public int inputSlotNumber; public InventoryArkenstoneResult outputInventory = new InventoryArkenstoneResult(); public ArkenstoneRecipeHandler arkenstoneRecipeHandler; private final World world; private final BlockPos pos; private final InventoryPlayer playerInventory; public ContainerArkenstoneTable(InventoryPlayer playerInventory, World worldIn, BlockPos posIn){ this.world = worldIn; this.pos = posIn; this.playerInventory = playerInventory; arkenstoneRecipeHandler = new ArkenstoneRecipeHandler(); this.addSlotToContainer(new Slot(outputInventory, 0, 124, 35)); this.addSlotToContainer(new Slot(inputInventory, 0, 30 + 0 * 18, 17 + 18)); this.addSlotToContainer(new Slot(inputInventory, 1, 30 + 1 * 18, 17 + 18)); this.addSlotToContainer(new Slot(inputInventory, 2, 30 + 2 * 18, 17 + 18)); for (int k = 0; k < 3; ++k){ for (int i1 = 0; i1 < 9; ++i1){ this.addSlotToContainer(new Slot(playerInventory, i1 + k * 9 + 9, 8 + i1 * 18, 84 + k * 18)); } } for (int l = 0; l < 9; ++l){ this.addSlotToContainer(new Slot(playerInventory, l, 8 + l * 18, 142)); } } /** * Callback for when the crafting matrix is changed. */ public void onCraftMatrixChanged(IInventory inventoryIn){ if (!world.isRemote) { //if the inventory selected is actually input inventory if(inventoryIn == inputInventory){ //if this thing is empty, stahp if(inputInventory.isEmpty()) { return; }else { ItemStack outputItemStack = arkenstoneRecipeHandler.getArkenstoneResults(inputInventory); if (outputItemStack == ItemStack.EMPTY ){ return; }else { outputInventory.setInventorySlotContents(0, new ItemStack(Items.APPLE));; } } } } } /** * Called when the container is closed. */ @Override public void onContainerClosed(EntityPlayer playerIn) { super.onContainerClosed(playerIn); if (!this.world.isRemote) { this.clearContainer(playerIn, this.world, this.inputInventory); } } /** * Determines whether supplied player can use this container */ @Override public boolean canInteractWith(EntityPlayer playerIn) { if (this.world.getBlockState(this.pos).getBlock() != ModBlocks.ArkenstoneTableBlock) { return false; } else { return playerIn.getDistanceSq((double)this.pos.getX() + 0.5D, (double)this.pos.getY() + 0.5D, (double)this.pos.getZ() + 0.5D) <= 64.0D; } } /** * Handle when the stack in slot {@code index} is shift-clicked. Normally this moves the stack between the player * inventory and the other inventory(s). */ public ItemStack transferStackInSlot(EntityPlayer playerIn, int index) { ItemStack itemstack = ItemStack.EMPTY; Slot slot = this.inventorySlots.get(index); if (slot != null && slot.getHasStack()) { ItemStack itemstack1 = slot.getStack(); itemstack = itemstack1.copy(); if (index == 0) { itemstack1.getItem().onCreated(itemstack1, this.world, playerIn); if (!this.mergeItemStack(itemstack1, 10, 46, true)) { return ItemStack.EMPTY; } slot.onSlotChange(itemstack1, itemstack); } else if (index >= 10 && index < 37) { if (!this.mergeItemStack(itemstack1, 37, 46, false)) { return ItemStack.EMPTY; } } else if (index >= 37 && index < 46) { if (!this.mergeItemStack(itemstack1, 10, 37, false)) { return ItemStack.EMPTY; } } else if (!this.mergeItemStack(itemstack1, 10, 46, false)) { return ItemStack.EMPTY; } if (itemstack1.isEmpty()) { slot.putStack(ItemStack.EMPTY); } else { slot.onSlotChanged(); } if (itemstack1.getCount() == itemstack.getCount()) { return ItemStack.EMPTY; } ItemStack itemstack2 = slot.onTake(playerIn, itemstack1); if (index == 0) { playerIn.dropItem(itemstack2, false); } } return itemstack; } /** * Called to determine if the current slot is valid for the stack merging (double-click) code. The stack passed in * is null for the initial slot that was double-clicked. */ public boolean canMergeSlot(ItemStack stack, Slot slotIn){ return slotIn.inventory != this.outputInventory && super.canMergeSlot(stack, slotIn); } @Override public Slot getSlot(int parSlotIndex) { if(parSlotIndex >= inventorySlots.size()) parSlotIndex = inventorySlots.size() - 1; return super.getSlot(parSlotIndex); } }
-
That worked like a charm, thanks for the help!
-
Got it, I'll keep at it for another while before bothering you guys again with the repository. Nonetheless, thank you all very much for the effort.
-
Sorry I don't quite get what this means, I'm assuming you're asking if I put any tests? I tested that the cobblestone if statement fired by having a SoundEvent fire right after it, and it did fire. The above if statement comes right after that cobblestone if statement. I then put the same SoundEvent to fire right after the above if statement, but it never did. I then went into MCEdit to check for TileEntities, and none showed up (other modded tileEntities of mine, however, did show up in MCEdit).
-
Thanks for the suggestion! I've registered the tileEntity and added the necessary methods and annotations as you mentioned. The world no longer crashes, but no tileEntities are created every time I place a block. Any idea what might cause this? Block class: public class TrebuchetBlock extends BlockBase { public static final PropertyDirection FACING = PropertyDirection.create("facing", EnumFacing.Plane.HORIZONTAL); public TrebuchetBlock(String name, Material material) { super(name, material); setSoundType(SoundType.STONE); setHardness(18.0F); setResistance(18.0F); setHarvestLevel("pickaxe",3); setCreativeTab(CreativeTabs.REDSTONE); } public void updateTick(World worldIn, BlockPos pos, IBlockState state, Random rand){ if (!worldIn.isRemote){ if(worldIn.getTileEntity(pos) instanceof TileEntityTrebuchet) { ((TileEntityTrebuchet)worldIn.getTileEntity(pos)).setCooldown(0); } } } public boolean onBlockActivated(World worldIn, BlockPos pos, IBlockState state, EntityPlayer playerIn, EnumHand hand, EnumFacing facing, float side, float hitX, float hitY){ if(playerIn.getHeldItemMainhand().getItem().equals(Item.getItemFromBlock(Blocks.COBBLESTONE))) { if((worldIn.getTileEntity(pos)) instanceof TileEntityTrebuchet) { worldIn.playSound(playerIn, pos, SoundEvents.BLOCK_CHEST_OPEN, SoundCategory.BLOCKS, 0.5F, 0.4F / (0.2F + 0.8F)); if(((TileEntityTrebuchet)worldIn.getTileEntity(pos)).getCooldown()<=0) { if(!worldIn.isRemote) { EntityTippedArrow entityarrow; if(((EnumFacing)state.getValue(FACING)).getIndex() ==2){ entityarrow = new EntityTippedArrow(worldIn, (double)pos.getX()+0.5, ((double)pos.getY()+0.9), (double)pos.getZ()-0.2); }else if(((EnumFacing)state.getValue(FACING)).getIndex() ==3) { entityarrow = new EntityTippedArrow(worldIn, (double)pos.getX()+0.5, ((double)pos.getY()+0.9), (double)pos.getZ()+1.2); }else if(((EnumFacing)state.getValue(FACING)).getIndex() ==4) { entityarrow = new EntityTippedArrow(worldIn, (double)pos.getX()-0.2, ((double)pos.getY()+0.9), (double)pos.getZ()+0.5); }else{ entityarrow = new EntityTippedArrow(worldIn, (double)pos.getX()+1.2, ((double)pos.getY()+0.9), (double)pos.getZ()+0.5); } entityarrow.setPotionEffect(new ItemStack(Items.ARROW)); float f = -MathHelper.sin(playerIn.rotationYaw * 0.017453292F) * MathHelper.cos(playerIn.rotationPitch * 0.017453292F); float f1 = -MathHelper.sin(playerIn.rotationPitch * 0.017453292F); float f2 = MathHelper.cos(playerIn.rotationYaw * 0.017453292F) * MathHelper.cos(playerIn.rotationPitch * 0.017453292F); entityarrow.setIsCritical(true); entityarrow.setDamage(30.0); entityarrow.setKnockbackStrength(1); entityarrow.shoot((double)f, (double)f1+0.05, (double)f2, 3.0F, 1.0F); worldIn.spawnEntity(entityarrow); ((TileEntityTrebuchet)worldIn.getTileEntity(pos)).setCooldown(1); } if(!playerIn.capabilities.isCreativeMode) { playerIn.getItemStackFromSlot(EntityEquipmentSlot.MAINHAND).shrink(1); } worldIn.playSound(playerIn, pos, SoundEvents.ENTITY_ARROW_SHOOT, SoundCategory.NEUTRAL, 0.5F, 0.4F / (0.2F + 0.8F)); } } worldIn.scheduleUpdate(pos, this, 60); } return true; } protected BlockStateContainer createBlockState() { return new BlockStateContainer(this, new IProperty[] {FACING}); } public IBlockState withRotation(IBlockState state, Rotation rot) { return state.withProperty(FACING, rot.rotate((EnumFacing)state.getValue(FACING))); } public IBlockState withMirror(IBlockState state, Mirror mirrorIn) { return state.withRotation(mirrorIn.toRotation((EnumFacing)state.getValue(FACING))); } public IBlockState getStateForPlacement(World worldIn, BlockPos pos, EnumFacing facing, float hitX, float hitY, float hitZ, int meta, EntityLivingBase placer) { return this.getDefaultState().withProperty(FACING, placer.getHorizontalFacing().getOpposite()); } public int getMetaFromState(IBlockState state) { int i = 0; i = i | ((EnumFacing)state.getValue(FACING)).getHorizontalIndex(); return i; } public IBlockState getStateFromMeta(int meta) { return this.getDefaultState().withProperty(FACING, EnumFacing.getHorizontal(meta)); } @Override public TileEntity createTileEntity(World world, IBlockState state) { TileEntityTrebuchet tileEntityTrebuchet = new TileEntityTrebuchet(); return tileEntityTrebuchet; } @Override public boolean hasTileEntity() { return true; } @Override public void onBlockPlacedBy(World worldIn, BlockPos pos, IBlockState state, EntityLivingBase placer, ItemStack stack) { if(stack.hasDisplayName()) { TileEntity tileentity = worldIn.getTileEntity(pos); if(tileentity instanceof TileEntityTrebuchet){ ((TileEntityTrebuchet)tileentity).setCustomName(stack.getDisplayName()); ((TileEntityTrebuchet)tileentity).setCooldown(0); } } } } TileEntity class: public class TileEntityTrebuchet extends TileEntity{ private String customName; private int cooldown; public TileEntityTrebuchet() { this.cooldown = 0; } public boolean hasCustomName() { return this.customName != null && !this.customName.isEmpty(); } public void setCustomName(String customName) { this.customName = customName; } @Override public void readFromNBT(NBTTagCompound compound) { super.readFromNBT(compound); if (compound.hasKey("cooldown")) { cooldown = compound.getInteger("cooldown"); } if(compound.hasKey("CustomName", 8)) { this.customName = compound.getString("CustomName"); } } @Override public NBTTagCompound writeToNBT(NBTTagCompound compound) { super.writeToNBT(compound); if(compound.hasKey("cooldown")) { compound.setInteger("cooldown", cooldown); } if(compound.hasKey("CustomName", 8)) { compound.setString("CustomName", this.customName); } return compound; } public int getCooldown() { return this.cooldown; } public void setCooldown(int cd) { this.cooldown= cd; } public void shrinkCooldown() { this.cooldown--; } } This is my preInit() method: @EventHandler() public static void PreInit(FMLPreInitializationEvent event) { RegistryHandler.preInitRegistries(); } preInitRegistries() in my RegistryHandler class: public static void preInitRegistries() { TileEntityHandler.registerTileEntities(); Main.network = NetworkRegistry.INSTANCE.newSimpleChannel(Reference.MOD_ID); RegistryHandler.registerPackets(Main.network); } TileEntityHandler class: public class TileEntityHandler { public static void registerTileEntities() { GameRegistry.registerTileEntity(TileEntityTrebuchet.class, new ResourceLocation(Reference.MOD_ID + ":trebuchet")); } }
-
Thank you so much for your help! Sorry I'm still new to modding so don't really have a clear idea which things should be on server or client. Regarding this, I've made some adjustments to my block class and created a new TileEntity to store the cooldown. Essentially, that cooldown is to prevent spamming the shots and give the block a steady rate of fire. At first it worked fine, but occasionally when I tried to load the world it gives an exception and crashes: Any ideas what this might be? This is my updated block class: public class TrebuchetBlock extends BlockBase implements ITileEntityProvider{ public static final PropertyDirection FACING = PropertyDirection.create("facing", EnumFacing.Plane.HORIZONTAL); public TrebuchetBlock(String name, Material material) { super(name, material); setSoundType(SoundType.STONE); setHardness(18.0F); setResistance(18.0F); setHarvestLevel("pickaxe",3); setCreativeTab(CreativeTabs.REDSTONE); } public void updateTick(World worldIn, BlockPos pos, IBlockState state, Random rand){ if (!worldIn.isRemote){ if(worldIn.getTileEntity(pos) instanceof TileEntityTrebuchet) { ((TileEntityTrebuchet)worldIn.getTileEntity(pos)).setCooldown(0); } } } public boolean onBlockActivated(World worldIn, BlockPos pos, IBlockState state, EntityPlayer playerIn, EnumHand hand, EnumFacing facing, float side, float hitX, float hitY){ if(playerIn.getHeldItemMainhand().getItem().equals(Item.getItemFromBlock(Blocks.COBBLESTONE))) { if(facing == ((EnumFacing)state.getValue(FACING))) { return true; } if((worldIn.getTileEntity(pos)) instanceof TileEntityTrebuchet) { if(((TileEntityTrebuchet)worldIn.getTileEntity(pos)).getCooldown()==0) { if(!worldIn.isRemote) { EntityTippedArrow entityarrow; if(((EnumFacing)state.getValue(FACING)).getIndex() ==2){ entityarrow = new EntityTippedArrow(worldIn, (double)pos.getX()+0.5, ((double)pos.getY()+0.9), (double)pos.getZ()-0.2); }else if(((EnumFacing)state.getValue(FACING)).getIndex() ==3) { entityarrow = new EntityTippedArrow(worldIn, (double)pos.getX()+0.5, ((double)pos.getY()+0.9), (double)pos.getZ()+1.2); }else if(((EnumFacing)state.getValue(FACING)).getIndex() ==4) { entityarrow = new EntityTippedArrow(worldIn, (double)pos.getX()-0.2, ((double)pos.getY()+0.9), (double)pos.getZ()+0.5); }else{ entityarrow = new EntityTippedArrow(worldIn, (double)pos.getX()+1.2, ((double)pos.getY()+0.9), (double)pos.getZ()+0.5); } entityarrow.setPotionEffect(new ItemStack(Items.ARROW)); float f = -MathHelper.sin(playerIn.rotationYaw * 0.017453292F) * MathHelper.cos(playerIn.rotationPitch * 0.017453292F); float f1 = -MathHelper.sin(playerIn.rotationPitch * 0.017453292F); float f2 = MathHelper.cos(playerIn.rotationYaw * 0.017453292F) * MathHelper.cos(playerIn.rotationPitch * 0.017453292F); entityarrow.setIsCritical(true); entityarrow.setDamage(30.0); entityarrow.setKnockbackStrength(1); entityarrow.shoot((double)f, (double)f1+0.05, (double)f2, 3.0F, 1.0F); worldIn.spawnEntity(entityarrow); ((TileEntityTrebuchet)worldIn.getTileEntity(pos)).setCooldown(1); } if(!playerIn.capabilities.isCreativeMode) { playerIn.getItemStackFromSlot(EntityEquipmentSlot.MAINHAND).shrink(1); } worldIn.playSound(playerIn, pos, SoundEvents.ENTITY_ARROW_SHOOT, SoundCategory.NEUTRAL, 0.5F, 0.4F / (0.2F + 0.8F)); } } worldIn.scheduleUpdate(pos, this, 60); } return true; } protected BlockStateContainer createBlockState() { return new BlockStateContainer(this, new IProperty[] {FACING}); } public IBlockState withRotation(IBlockState state, Rotation rot) { return state.withProperty(FACING, rot.rotate((EnumFacing)state.getValue(FACING))); } public IBlockState withMirror(IBlockState state, Mirror mirrorIn) { return state.withRotation(mirrorIn.toRotation((EnumFacing)state.getValue(FACING))); } public IBlockState getStateForPlacement(World worldIn, BlockPos pos, EnumFacing facing, float hitX, float hitY, float hitZ, int meta, EntityLivingBase placer) { return this.getDefaultState().withProperty(FACING, placer.getHorizontalFacing().getOpposite()); } public int getMetaFromState(IBlockState state) { int i = 0; i = i | ((EnumFacing)state.getValue(FACING)).getHorizontalIndex(); return i; } public IBlockState getStateFromMeta(int meta) { return this.getDefaultState().withProperty(FACING, EnumFacing.getHorizontal(meta)); } public TileEntity createNewTileEntity(World worldIn, int meta) { TileEntityTrebuchet tileEntityTrebuchet = new TileEntityTrebuchet(); return tileEntityTrebuchet; } @Override public void onBlockPlacedBy(World worldIn, BlockPos pos, IBlockState state, EntityLivingBase placer, ItemStack stack) { if(stack.hasDisplayName()) { TileEntity tileentity = worldIn.getTileEntity(pos); if(tileentity instanceof TileEntityTrebuchet){ ((TileEntityTrebuchet)tileentity).setCustomName(stack.getDisplayName()); ((TileEntityTrebuchet)tileentity).setCooldown(0); } } } } And TileEntity Class: public class TileEntityTrebuchet extends TileEntity{ private String customName; public int cooldown; public boolean hasCustomName() { return this.customName != null && !this.customName.isEmpty(); } public void setCustomName(String customName) { this.customName = customName; } @Override public void readFromNBT(NBTTagCompound compound) { super.readFromNBT(compound); if (compound.hasKey("cooldown")) { cooldown = compound.getInteger("cooldown"); } if(compound.hasKey("CustomName", 8)) { this.customName = compound.getString("CustomName"); } } @Override public NBTTagCompound writeToNBT(NBTTagCompound compound) { super.writeToNBT(compound); compound.setInteger("cooldown", cooldown); if(compound.hasKey("CustomName", 8)) { compound.setString("CustomName", this.customName); } return compound; } public int getCooldown() { return this.cooldown; } public void setCooldown(int cd) { this.cooldown= cd; } public boolean isUsableByPlayer(EntityPlayer player) { return this.world.getTileEntity(this.pos) != this ? false : player.getDistanceSq((double)this.pos.getX() + 0.5D, (double)this.pos.getY() + 0.5D, (double)this.pos.getZ() + 0.5D) <= 64.0D; } }
-
I have a block that shoots an arrow every time it is activated by right click whilst holding cobblestone. The arrows it spawn, however, bounce off of any entity it hits. Any idea what might cause this, and possible fixes? Thanks! Code: public boolean onBlockActivated(World worldIn, BlockPos pos, IBlockState state, EntityPlayer playerIn, EnumHand hand, EnumFacing facing, float side, float hitX, float hitY) { if(playerIn.getHeldItemMainhand().getItem().equals(Item.getItemFromBlock(Blocks.COBBLESTONE))) { if(facing == ((EnumFacing)state.getValue(FACING))) { return true; } if(rechargeTimer == 0) { if(worldIn.isRemote) { EntityTippedArrow entityarrow; if(((EnumFacing)state.getValue(FACING)).getIndex() ==2){ entityarrow = new EntityTippedArrow(worldIn, (double)pos.getX()+0.5, ((double)pos.getY()+0.9), (double)pos.getZ()-0.1); }else if(((EnumFacing)state.getValue(FACING)).getIndex() ==3) { entityarrow = new EntityTippedArrow(worldIn, (double)pos.getX()+0.5, ((double)pos.getY()+0.9), (double)pos.getZ()+1.1); }else if(((EnumFacing)state.getValue(FACING)).getIndex() ==4) { entityarrow = new EntityTippedArrow(worldIn, (double)pos.getX()-0.1, ((double)pos.getY()+0.9), (double)pos.getZ()+0.5); }else { entityarrow = new EntityTippedArrow(worldIn, (double)pos.getX()+1.1, ((double)pos.getY()+0.9), (double)pos.getZ()+0.5); } entityarrow.setPotionEffect(new ItemStack(Items.ARROW)); float f = -MathHelper.sin(playerIn.rotationYaw * 0.017453292F) * MathHelper.cos(playerIn.rotationPitch * 0.017453292F); float f1 = -MathHelper.sin(playerIn.rotationPitch * 0.017453292F); float f2 = MathHelper.cos(playerIn.rotationYaw * 0.017453292F) * MathHelper.cos(playerIn.rotationPitch * 0.017453292F); entityarrow.setIsCritical(true); entityarrow.setDamage(30.0); entityarrow.setKnockbackStrength(1); entityarrow.shoot((double)f, (double)f1+0.05, (double)f2, 3.0F, 1.0F); worldIn.spawnEntity(entityarrow); this.rechargeTimer =1; } if(!playerIn.capabilities.isCreativeMode) { playerIn.getActiveItemStack().shrink(1); } worldIn.playSound(playerIn, pos, SoundEvents.ENTITY_ARROW_SHOOT, SoundCategory.NEUTRAL, 0.5F, 0.4F / (0.2F + 0.8F)); } worldIn.scheduleUpdate(pos, this, 10); } return true; }
-
I have a custom block that I want to spawn an arrow projectile on its front face every time a player right clicks said block while holding cobblestone. I've been digging around google and most people who wanted to achieve a similar effect seemed to have used the worldIn.spawnEntityInWorld(). I cannot, however, find said method in the world class, and judging from how the forum posts I've referenced are all 2yrs+ old, I'm guessing this method has been removed. Any ideas how I can achieve this in minecraft 1.12? This is the code for my custom block if it helps: public class TrebuchetBlock extends BlockBase{ public static final PropertyDirection FACING = PropertyDirection.create("facing", EnumFacing.Plane.HORIZONTAL); public TrebuchetBlock(String name, Material material) { super(name, material); setSoundType(SoundType.STONE); setHardness(18.0F); setResistance(18.0F); setHarvestLevel("pickaxe",3); setCreativeTab(CreativeTabs.REDSTONE); } public boolean onBlockActivated(World worldIn, BlockPos pos, IBlockState state, EntityPlayer playerIn, EnumHand hand, EnumFacing facing, float side, float hitX, float hitY){ if(worldIn.isRemote) { if(playerIn.getHeldItemMainhand().getItem().equals(Item.getItemFromBlock(Blocks.COBBLESTONE))) { //Insert solution here return true; }else{ return true; } }else{ return false; } } protected BlockStateContainer createBlockState(){ return new BlockStateContainer(this, new IProperty[] {FACING}); } public IBlockState withRotation(IBlockState state, Rotation rot){ return state.withProperty(FACING, rot.rotate((EnumFacing)state.getValue(FACING))); } public IBlockState withMirror(IBlockState state, Mirror mirrorIn){ return state.withRotation(mirrorIn.toRotation((EnumFacing)state.getValue(FACING))); } public IBlockState getStateForPlacement(World worldIn, BlockPos pos, EnumFacing facing, float hitX, float hitY, float hitZ, int meta, EntityLivingBase placer){ return this.getDefaultState().withProperty(FACING, placer.getHorizontalFacing().getOpposite()); } public int getMetaFromState(IBlockState state){ int i = 0; i = i | ((EnumFacing)state.getValue(FACING)).getHorizontalIndex(); return i; } public IBlockState getStateFromMeta(int meta){ return this.getDefaultState().withProperty(FACING, EnumFacing.getHorizontal(meta)); } }