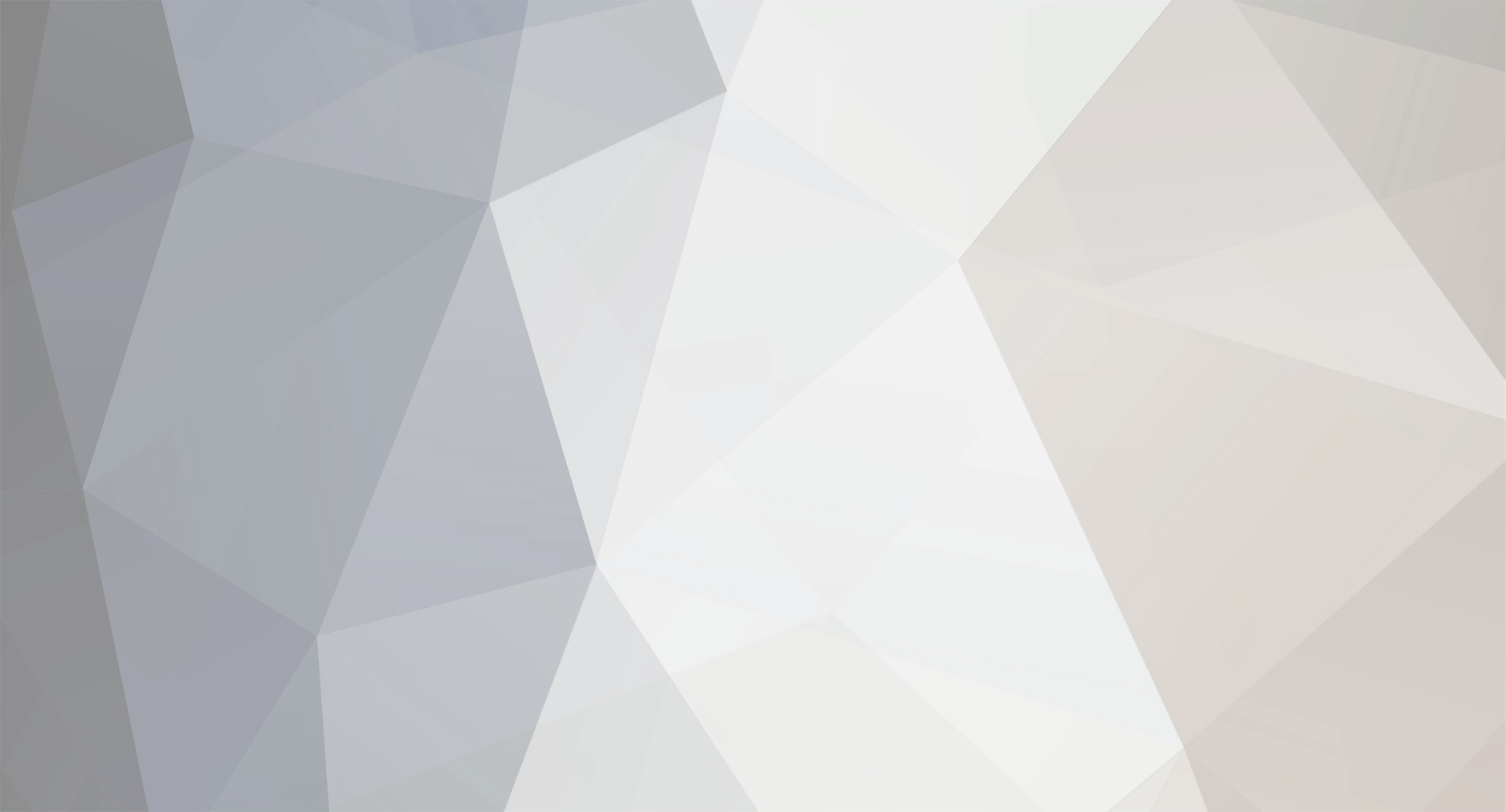
geekles
Members-
Posts
37 -
Joined
-
Last visited
Everything posted by geekles
-
How would I go about creating a connection on a separate thread but then calling a listener synchronous with the main thread with the result? Currently my last issue is synchronously starting my "ProxyResponseReader" class if the connection was successful. new SocketConnection("127.0.0.1", 8080).connect().onConnectionResult(new ConnectionResultEvent() { @Override public void onResult(boolean connected) { if(Thread.currentThread() == Main.t) { System.out.println("onResult: Same Thread!"); } else { System.out.println("onResult: Other Thread!"); //not on the main thread } if (connected) { reader = new ProxyResponseReader(socket); return; } [...] } } private static class SocketConnection { private Socket socket; private final String ip; private final int port; private ConnectionResultEvent event; public SocketConnection(String ip, int port) { this.ip = ip; this.port = port; } // make the connection with the socket private SocketConnection connect() { new Thread() { public void run() { try { SocketConnection.this.socket = new Socket(ip, port); SocketConnection.this.event.onResult(true); } catch (IOException e) { SocketConnection.this.event.onResult(false); } } }.start(); return this; } public void onConnectionResult(ConnectionResultEvent event) { this.event = event; } [...] }
-
I see my problem. I'll try to rewrite my proxy response checker and subscribe to the client's tick instead of running the checks on a new thread.
-
Thank you for the information. I looked into it, and yes, I forgot to consider that I am on a separate thread. This is because the listener I spoke about earlier is called from a separate thread. Is there a client supported asynchronous method that I can use to easily switch back to its main thread?
-
Should I send over my entire project? ? CommandExecutor is an interface I implemented. CommandManager is a class that is responsible for registering these interfaces and setting up a listener for a class responsible for collecting outputs from a node.js proxy connection. Whenever a response is returned, the message is handled and then passed over to the listener that CommandManager uses. It then takes the string, converts it into an array of string command arguments and passes it to all the registered CommandExecutor. I know there's a more efficient method of going about doing this, e.g. associating aliases for each of these classes so that CommandManager can instead pass the parameter to only one of the classes instead of passing it to all of them blindly, however considering that this project is relatively small, and I have not created too many commands yet, but is something I intend to correct soon. But the information you're asking for now is quite redundant since now you're getting into how my entire project is functioning and not staying particularly focused on the subject of why the following line is throwing an error. mc.launchIntegratedServer(handler.getWorldDirectory().getAbsolutePath(), world, settings); I suppose first you can highlight what that error ("No context is current or a function that is not available in the current context was called") means since I'm quite unfamiliar with it and would like a general explanation of why the minecraft client throws it. Secondly, if you really need more information, please explain for what purpose, because at this point, I feel going back and forth like this is quite inefficient, and isn't getting us anywhere. If you have a question about the particular functionality of a class, then please ask and I'll explain.
-
public class WorldCmd implements CommandExecutor { @Override public boolean onCommand(String[] args) { if(!args[0].equalsIgnoreCase("world")) return false; if(args.length == 2) NetworkUtil.joinWorld(args[1]); return true; } }
-
I'm working on a mod (for a personal project of mine) that allows for the game to be controlled wirelessly through commands. It's a challenge for the game where the output from the game will be streamed to connected viewers. The audience may control the game's instance through commands. I currently have it so that the ingame character's movement may be controlled via commands as well as specifying which multiplayer server to join etc. however loading up a singleplayer world is ironically seemingly more tedious and I haven't been able to get far with that part. I would like to give the audience the opportunity to either choose to join a server and try controlling the game through commands only or choose to create or join a pre-existing singleplayer world. Naturally, checks will be made to ensure no one abuses this system and tries spamming servers with connection requests.
-
I'm calling the Minecraft#launchIntegratedServer() function since the CreateWorldScreen class does the same when creating a world. I'm merely trying to replicate the actions used to create/load a new or existing world. CreateWorldScreen#createWorld()
-
I am currently working on creating a method that lets me load a world from the world saves directory, however, after calling launchIntegratedServer to hopefully start loading up the world, I get some kind of *out of context* error that I'm not sure how to handle or resolve. This is my current setup: public static void joinWorld(String world) { Minecraft mc = Minecraft.getInstance(); SaveHandler handler = mc.getSaveLoader().getSaveLoader(world, mc.getIntegratedServer()); WorldInfo info = handler.loadWorldInfo(); if (info != null) { WorldSettings settings = new WorldSettings(info.getSeed(), info.getGameType(), info.isMapFeaturesEnabled(), info.isHardcore(), info.getGenerator()); mc.launchIntegratedServer(handler.getWorldDirectory().getAbsolutePath(), world, settings); LogManager.getLogger().info("World: " + info.getWorldName()); } } Stacktrace: FATAL ERROR in native method: Thread[Thread-15,5,main]: No context is current or a function that is not available in the current context was called. The JVM will abort execution. at org.lwjgl.opengl.GL11.glPushMatrix(Native Method) at com.mojang.blaze3d.platform.GlStateManager.pushMatrix(GlStateManager.java:572) at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:916) at net.minecraft.client.Minecraft.func_213241_c(Minecraft.java:1710) at net.minecraft.client.Minecraft.func_213231_b(Minecraft.java:1683) at net.minecraft.client.Minecraft.func_213254_o(Minecraft.java:1667) at net.minecraft.client.Minecraft.launchIntegratedServer(Minecraft.java:1563)
-
I did a bit more testing and found Optifine's Shaders does nothing to the actual gamma value in the gameSettings (which makes sense). However, the brightness is definitely being altered somehow and knowing how gamma is being used to determine how light darkness actually is would be a start. I'm thinking maybe LightTexture#updateLightmap(float) method may be in charge of this, but I'm not sure and I'm not sure yet how to override such a method for debugging purposes. It is also likely a waste of time to do so since it is very likely such a method is being replaced by Optifine's own way of handling lighting in the world. Optifine also uses a LightMap class which has methods that seem to replace the pre-existing vanilla lighting mechanics, but that could take time to properly debug/override as well. I suppose I could create my own, call it after optifine calls its own, but not knowing when this method is called could be an issue for now. Perhaps I'm overthinking all of this?
-
*bump* Help with original issue.
-
I suspect with reflection I can override some of the basic values, e.g. gamma and fog levels. However, I am still unclear on where these fields exist.
-
I currently developed a mod that drops the gamma levels of the world and modifies the fog levels dynamically based off of several factors occurring in the world. I recently discovered however that when a shader is installed, the gamma levels and fog levels that my mod modifies are overridden by the shaderpacks. This is understandably so, however it would be nice to know if there's a way I could implement compatibility with shader packs instead of potentially having to disable the option completely. I suppose what I am asking, is there an event that Optifine passes when a shader loads so I can ensure the setting my mod plays with are not overridden, or do I need to create a schedular that constantly ensures the gamma levels are where they should be at (obviously a bit overkill but a possibility)?
-
Thank you. I'll give it a try soon.
-
Which one though. Should both handle an unmatched Protocol_Version?
-
How would I listen for when this NetworkRegistry.ABSENT is passed? I assume perhaps there's an event I can cancel and handle it myself instead of having the client by default close the connection.
-
I am currently developing a mod that is an updated version of mods such as "Hardcore Darkness". Being this is my first "real" mod, I was glad to see that I was able to successfully instantiate a new channel for both the server and client to use. This would essentially allow the server to ensure the player has the mod installed before letting them in, which is exactly what I wanted. However, I also want to give the player the option to join a server if they have the mod installed on their client even if the server isn't forge or has the mod installed on the server. However, because of the "forge handshake", it will automatically close the connection from the client to the server if the server doesn't have a channel for it to communicate with my mod with. I want it so that I can disable the client from closing the connection if there is no open channel to communicate with the mod. Not sure how to do this. The mod (at the moment), has no real need to send custom packets to the server since the mechanics simply mess with the visuals (darkness, fog levels, etc.) Therefore there is no need for there to be any information to be synced across all the clients across the server, asides for weather and time which is already sent by the server by default. So far, I know the following lines in the FMLHandshakeHandler is the culprit for the issue: void handleServerModListOnClient(FMLHandshakeMessages.S2CModList serverModList, Supplier<NetworkEvent.Context> c) { LOGGER.debug(FMLHSMARKER, "Logging into server with mod list [{}]", String.join(", ", serverModList.getModList())); boolean accepted = NetworkRegistry.validateClientChannels(serverModList.getChannels()); c.get().setPacketHandled(true); if (!accepted) { LOGGER.error(FMLHSMARKER, "Terminating connection with server, mismatched mod list"); c.get().getNetworkManager().closeChannel(new StringTextComponent("Connection closed - mismatched mod channel list")); return; } [...] I've tried going through the classes to see how it gets from point A (see below) to point B (see above). Too many variables and functions are being called however and it isn't clear how or from what that function gets called. INSTANCE = NetworkRegistry.newSimpleChannel( new ResourceLocation(Blackout.ID, "main"), () -> PROTOCOL_VERSION, PROTOCOL_VERSION::equals, PROTOCOL_VERSION::equals );
-
[Forge 1.14] Optifine Light Modification Conflict
geekles replied to geekles's topic in Modder Support
Hmm, interesting, although I am mildly confused now. This is what comes up in the table for the field name. field_76573_f lightBrightnessTable field_76575_d lightBrightnessTable lightBrightnessTable points to two obfuscated fields. Not sure then if I have to update both of their fields with the value of the lightBrightnessTable field. I'll give it a try, but interested to know if I am wrong. Also how do I find where the location of these fields are? -
[Forge 1.14] Optifine Light Modification Conflict
geekles replied to geekles's topic in Modder Support
So I realized this could be the case after debugging and reading all the fields in the class. I can't tell which field it is and the ObfuscationReflectionHelper throws an exception regarding a field not being found. I also tried manually changing all three of the fields that share the float[] datatype knowing that the lightBrightnessTable shared the same type. Unfortunately, it appears it has had no effect on the world's lighting. Ideas? ObfuscationReflectionHelper.findField(Dimension.class, "lightBrightnessTable") public class CustomBlackoutDimension extends Dimension { [...] /** * Creates the light to brightness table */ @Override protected void generateLightBrightnessTable() { [...] try { Field f1 = Dimension.class.getDeclaredField("field_111203_a"); f1 = DimensionalReflectionUtil.removeFinalModifier(f1); if(Minecraft.getInstance().player != null) { for(float fv : (float[]) f1.get(this)) Minecraft.getInstance().player.sendChatMessage("" + fv); } f1.set(f1, lightBrightnessTable); Field f2 = Dimension.class.getDeclaredField("field_76573_f"); f2 = DimensionalReflectionUtil.removeFinalModifier(f2); f2.set(this, lightBrightnessTable); if(Minecraft.getInstance().player != null) { for(float fv : (float[]) f2.get(this)) Minecraft.getInstance().player.sendChatMessage("" + fv); } Field f3 = Dimension.class.getDeclaredField("field_76580_h"); f3 = DimensionalReflectionUtil.removeFinalModifier(f3); f3.set(this, lightBrightnessTable); if(Minecraft.getInstance().player != null) { for(float fv : (float[]) f3.get(this)) Minecraft.getInstance().player.sendChatMessage("" + fv); } } catch(IllegalAccessException | NoSuchFieldException e) { e.printStackTrace(); } } [...] -
[Forge 1.14] Optifine Light Modification Conflict
geekles replied to geekles's topic in Modder Support
Essentially I created a method that I use to update the World's instance with my custom Dimension instance. public static void injectDimension(World world, Dimension dimension) { try { Field dimensionField = World.class.getDeclaredField("dimension"); dimensionField = removeFinalModifier(dimensionField); dimensionField.set(world, dimension); } catch (Exception ex) { ex.printStackTrace(); } } The custom Dimension class that is passed where I mainly just overrode the Dimension#generateLightBrightnessTable() method and tweaked the equation so that the lightBrightnessTable array is updated with larger light values. In vanilla, this array is often referenced to get the light values. However, this doesn't appear to be the case. I may need to utilize reflection again for debugging purposes to see if any new field names were injected into the instance or were modified that Optifine may perhaps be using. -
Recently I had the idea of recreating mods such as "Hardcore Darkness" so that they were up to date. I ran into the issue though where the torches' brightness weren't strong enough to counter the darker world that I created by lowering the world's gamma. However, I found out about the Dimension#generateLightBrightnessTable() function which is used to calculate the light levels of surrounding blocks. By modifying a particular constant, I was able to achieve higher levels of brightness from the torches so that more than one didn't need to be used within 3 blocks of one another. Everything was fine until I ran into an issue with Optifine. With optifine, it appears Dimension#generateLightBrightnessTable() is redundant and unused since it is called (shown through debugging) but doesn't produce the brightness output as it would when testing in a development environment without it. Below you will see two images with a side by side comparison of the differences in brightness. Any insight on what Optifine is doing that is preventing me from getting the same brightness result in both scenarios would be great. Some additional details you may find useful: Forge-Version: 1.14.4-28.1.10 Optifine-Version: preview_OptiFine_1.14.4_HD_U_F4_pre4
-
So I gave what I said a try and after quite a bit of tweaking the solution has worked out very well for me. Managed to increase the brightness by modifying the constants used to calculate the lightBrightnessTable. I feared forcefully updating the instance of the Dimension in the World instance for the client would screw something up but the world performs without any errors/bugs (at least from what I am aware of). Naturally now tweaking the gamma has allowed me to get the pitch blackness I was looking for while also being able to brighten up light-emitting objects so that they don't need to be used extensively. Some added bonuses from this solution is a light flickering effect. I suppose at some point it was implemented into the torches and other light-emitting objects however barely noticeable. Because the contrast between the light and pitch blackness is so large, these minor flickering effects are far more apparent however not so much to where they are annoying; so I just found that to be a neat little effect. I'm glad I was able to achieve this effect with such a simple solution. Thank you.
-
Hmm, I like this, however, how would I go about registering my version of the Dimension? I'll try forcefully changing the stored instance of the dimension in the world class via reflection, however I would imagine this is a very hacky solution that will likely either not work at all, or just, in general, be very buggy.
-
No, I am not trying to recreate a dynamic light source. I am simply trying to increase the amount of light torches emit. Read the question carefully, this is something I have already played around with. Yes, I am able to make the world much darker, however, this has made the amount of light torches emit quite minimal and requires at least one torch about every 5 blocks which then makes the mod annoying to use due to the excessive need for torches. Making the torches brighter to counter the reduced gamma would be ideal. Did not consider making a custom shader, however I don't feel this would be the best thing for me to do. Largely since I like to play with shaders and writing one would prevent me from using other shaders. Also this would add the optional ability for someone to add or remove the darkness shader pack when it is intended that everyone on the modded server share the same difficulty. Naturally, the rest of your suggestions are things I feared would be the result, and I would rather deal with placing more torches then rewriting large portions of the game's code. Although I am still quite convinced that a simpler solution exists.
-
Torch is a block? Not sure how your question helps answer my question?
-
I am currently working on creating a mod for myself/group of friends to where we have the added difficulty of where the brightness of the world is much darker (mods like Hardcore-Darkness are currently outdated). It turns out it is very simple to just change the game's gamma and lower it to where the whole world is much darker. For the most part, it has been satisfactory. However, I feel that torches range of brightness is much smaller then I would like it. Obviously it is due to substantially dropped gamma value however if there's a way to either A.) modify the range at which lights can flood a room or B.) adjust the brightness of a torch then this should help counter this effect. Essentially, I want a much darker world however I want torch blocks and other light-emitting blocks to still light up the same range of blocks around it as it did when gamma was at its default value. I am still fairly new to the forge API so perhaps I am missing something obvious? Currently trying to create my own custom torch class however not sure how I can register and replace the existing Torch instance. Perhaps I am overthinking this?