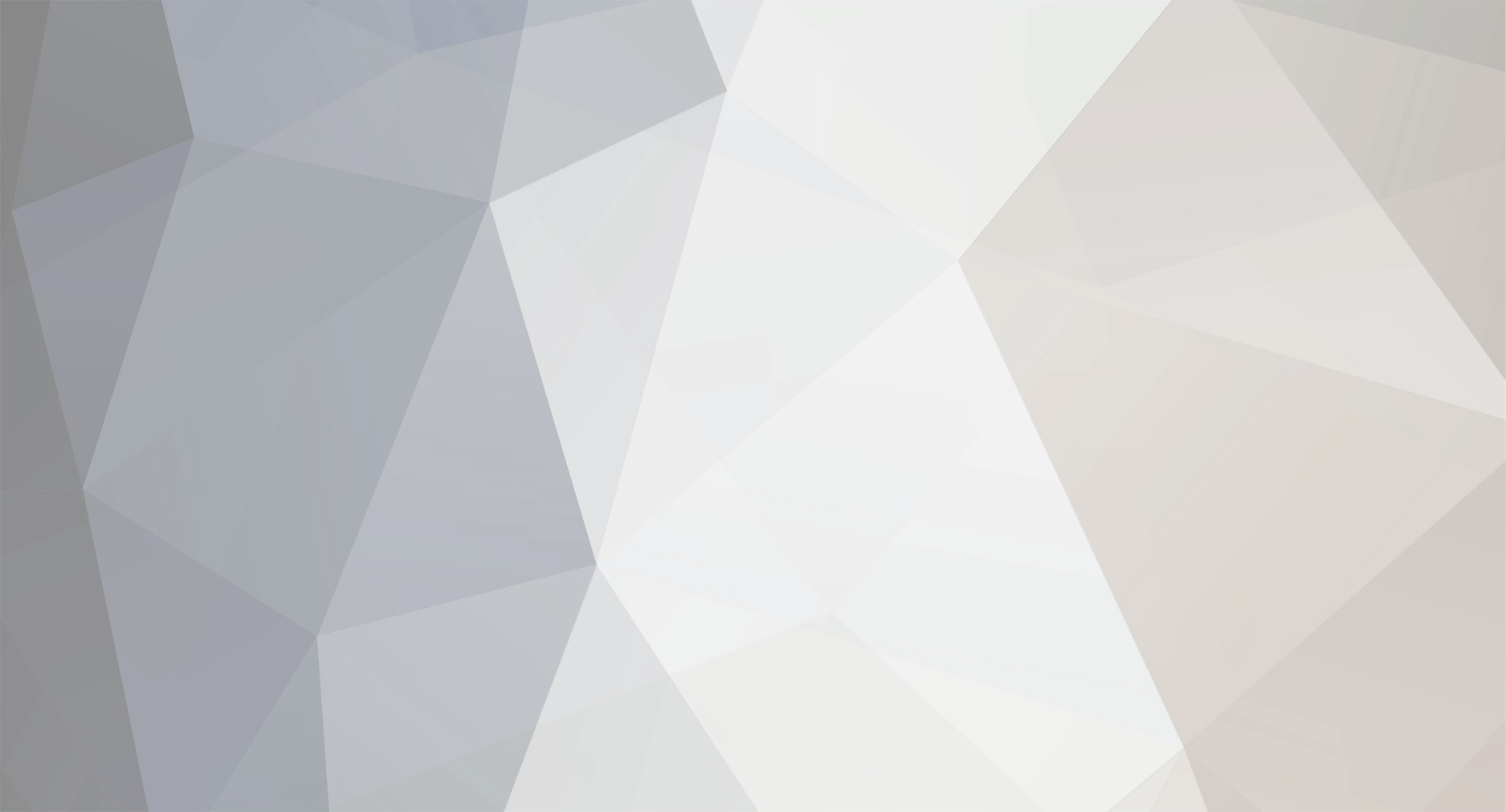
Lea9ue
Members-
Posts
44 -
Joined
-
Last visited
Everything posted by Lea9ue
-
I'm trying to continue some work from previous topic HERE. I'm trying to track the amount of times a diamond is destroyed by fire. I have that working great but need to save the data to world. If I quit after throwing diamonds in fire, the data is saved fine. When I reload the save the data is loaded fine. If I join another save where diamonds have been destroyed by fire already, that data remains fine in that save too. The problem is if I create a new world or load a save that has never seen a diamond destroyed by fire, the data from the last save where diamonds were destroyed by fire is loaded. I hope I'm explaining that in a way everyone can understand. Basically I need to see that 0 diamonds were destroyed when I enter a save where 0 diamonds were destroyed. Any help as always is appreciated. Here is my class that extends WorldSavedData: public class ModSaveData extends WorldSavedData{ private static final String DATA_NAME = Reference.MOD_ID; private static ModSaveData instance; public static int diamondSacrifice; public ModSaveData() { super(DATA_NAME); } public ModSaveData(String name) { super(name); } public static ModSaveData get(World world) { MapStorage storage = world.getMapStorage(); instance = (ModSaveData) storage.getOrLoadData(ModSaveData.class, DATA_NAME); if (instance == null) { instance = new ModSaveData(); storage.setData(DATA_NAME, instance); } return instance; } @Override public void readFromNBT(NBTTagCompound nbt) { diamondSacrifice = nbt.getInteger("diamondSacrifice"); } @Override public NBTTagCompound writeToNBT(NBTTagCompound compound) { compound.setInteger("diamondSacrifice", diamondSacrifice); return compound; } public void setDiamondSacrificeData(int diamondSacrifice) { this.diamondSacrifice = diamondSacrifice; markDirty(); } public int getDiamondSacrifice() { return diamondSacrifice; } } This is my Handler: public class ServerLoader { @SubscribeEvent(priority = EventPriority.NORMAL) public static void playerConnected(PlayerEvent.PlayerLoggedInEvent event) { ModSaveData data = ModSaveData.get(event.player.world); if(data != null) { System.out.println("SACRIFICES " + data.getDiamondSacrifice()); } } @SubscribeEvent(priority = EventPriority.NORMAL) public static void playerDisconnect(PlayerEvent.PlayerLoggedOutEvent event) { ModSaveData data = ModSaveData.get(event.player.world); if(data != null) { data.setDiamondSacrificeData(data.getDiamondSacrifice()); System.out.println("SACRIFICES " + data.getDiamondSacrifice()); } } } My Event for tracking Diamonds destroyed by fire: public static void onItemDeath(EntityItemDeathEvent event){ World world = event.getEntity().world; if (isDiamond(event.getEntityItem()) && event.damageSource.isFireDamage()){ ModSaveData.get(world).setDiamondSacrificeData(ModSaveData.diamondSacrifice + 1); ModSaveData.get(world).isDirty(); System.out.println(ModSaveData.diamondSacrifice); } }
-
Yes I could, and that works for that scenario. But it doesnt work for other instances. For example take the onDeathEvent, I dont think it will send message to all players. Only player who is trueSource, and let's look at a hypothetical for using INT's. Let's say I want to count skeleton kills. Once 10 skeletons are killed by EntityPlayer, if I want to send a message to everyone playing, then I have a problem. So that's what I need a solution checking a if statement for a static int thats not going thru on client side.
-
In the same class from multiple different ways. For example one reason I would have to do this would be for like this: @SubscribeEvent public static void mobDefeat(LivingDeathEvent event) { World world = event.getEntityLiving().world; if (event.getEntity() instanceof EntitySkeleton) { if(event.getSource().getTrueSource() instanceof EntityPlayerMP) { diamondSacrificeMessage = 1; } } } Obviously for this the true source would only be the player that killed mob so I change diamondSacrificeMessage to 1 and then check with if statement in my original post and then reset to 0 once chat message is sent.
-
So I am having trouble sending chat under certain conditions. From my server handler I can do: @SubscribeEvent public static void sendMessage(LivingUpdateEvent event) { World world = event.getEntity().world; if(!world.isRemote) { if(event.getEntity() instanceof EntityPlayer) { EntityPlayer player = (EntityPlayer) event.getEntity(); player.sendMessage(new TextComponentTranslation("diamondSacrifice" + diamondSacrifice, new Object[0]).setStyle(new Style().setColor(TextFormatting.BLACK))); ....Do Other things and with this everyone receives message as I intend. BUT if I add another if statement: @SubscribeEvent //Send message to player public static void sendMessage(LivingUpdateEvent event) { World world = event.getEntity().world; if(diamondSacrificeMessage == 1) { if(!world.isRemote) { if(event.getEntity() instanceof EntityPlayer) { EntityPlayer player = (EntityPlayer) event.getEntity(); player.sendMessage(new TextComponentTranslation("diamondSacrifice" + diamondSacrifice, new Object[0]).setStyle(new Style().setColor(TextFormatting.BLACK))); Then the client no longer gets messages while testing thru LAN. Can someone explain to me why a check of a public static int would cause problems?
-
[1.12.2] Wanting to know if dropped item is gone.
Lea9ue replied to Lea9ue's topic in Modder Support
First off Thanks to @Animefan8888, @DavidM, @Daeruin. This is the code, I feel its good enough. Just wanted to share incase anyone comes across it that needs it. Entity class: package com.lea9ue.mobwars.common.entity; import com.lea9ue.mobwars.event.item.EntityItemDeathEvent; import com.lea9ue.mobwars.event.item.EntityItemHurtEvent; import net.minecraft.entity.item.EntityItem; import net.minecraft.item.ItemStack; import net.minecraft.util.DamageSource; import net.minecraft.world.World; import net.minecraftforge.common.MinecraftForge; public class EntityItemFakeDiamond extends EntityItem { private DamageSource previousDamageSource; public EntityItemFakeDiamond(World world, double x, double y, double z, ItemStack itemStack) { super(world, x, y, z, itemStack); } @Override public boolean attackEntityFrom(DamageSource damageSource, float damage) { boolean returnValue = super.attackEntityFrom(damageSource, damage); this.previousDamageSource = damageSource; if (this.velocityChanged) MinecraftForge.EVENT_BUS.post(new EntityItemHurtEvent(this, damageSource, damage)); if (this.isDead) MinecraftForge.EVENT_BUS.post(new EntityItemDeathEvent(this, damageSource)); return returnValue; } public DamageSource getPreviousDamageSource(){ return this.previousDamageSource; } public static EntityItemFakeDiamond copy(EntityItem oldEntityItem){ EntityItemFakeDiamond newEntityItem = new EntityItemFakeDiamond(oldEntityItem.world, oldEntityItem.posX, oldEntityItem.posY, oldEntityItem.posZ, oldEntityItem.getItem()); newEntityItem.motionX = oldEntityItem.motionX; newEntityItem.motionY = oldEntityItem.motionY; newEntityItem.motionZ = oldEntityItem.motionZ; newEntityItem.hoverStart = oldEntityItem.hoverStart; newEntityItem.lifespan = oldEntityItem.lifespan; return newEntityItem; } } Handler class: package com.lea9ue.mobwars.server; import com.lea9ue.mobwars.common.entity.EntityItemFakeDiamond; import com.lea9ue.mobwars.event.item.EntityItemDeathEvent; import com.lea9ue.mobwars.init.ModItems; import net.minecraft.entity.Entity; import net.minecraft.entity.item.EntityItem; import net.minecraft.init.Items; import net.minecraft.item.ItemStack; import net.minecraft.world.World; import net.minecraftforge.event.entity.EntityJoinWorldEvent; import net.minecraftforge.fml.common.eventhandler.SubscribeEvent; public class DiamondHandler{ @SubscribeEvent public static void onEntityJoinWorld(EntityJoinWorldEvent event){ if (event.getWorld().isRemote) return; if (!(event.getEntity() instanceof EntityItem)) return; if (event.getEntity() instanceof EntityItemFakeDiamond) return; EntityItem entityItem = (EntityItem) event.getEntity(); if (isDiamond(entityItem)){ System.out.println("Diamond has been dropped"); EntityItemFakeDiamond newEntityItem = EntityItemFakeDiamond.copy(entityItem); event.getWorld().spawnEntity(newEntityItem); event.setCanceled(true); newEntityItem.setDefaultPickupDelay(); } } @SubscribeEvent public static void onItemDeath(EntityItemDeathEvent event){ if (isDiamond(event.getEntityItem()) && event.damageSource.isFireDamage()){ System.out.println("Diamond on Died in the fire/lava"); //DO SOMETHING// } } private static boolean isDiamond(EntityItem entityItem){ return entityItem.getItem().getUnlocalizedName().equals(Items.DIAMOND.getUnlocalizedName()); } } Event class: package com.lea9ue.mobwars.event.item; import net.minecraft.entity.item.EntityItem; import net.minecraft.util.DamageSource; import net.minecraftforge.event.entity.item.ItemEvent; public class EntityItemDeathEvent extends ItemEvent{ public final DamageSource damageSource; public EntityItemDeathEvent(EntityItem itemEntity, DamageSource damageSource) { super(itemEntity); this.damageSource = damageSource; } } Event class: package com.lea9ue.mobwars.event.item; import net.minecraft.entity.item.EntityItem; import net.minecraft.util.DamageSource; import net.minecraftforge.event.entity.item.ItemEvent; public class EntityItemHurtEvent extends ItemEvent { public final DamageSource damageSource; public final float amount; public EntityItemHurtEvent(EntityItem entity, DamageSource damageSource, float amount) { super(entity); this.damageSource = damageSource; this.amount = amount; } } -
[1.12.2] Wanting to know if dropped item is gone.
Lea9ue replied to Lea9ue's topic in Modder Support
Once Vahnilla item is in lava. I would like to spawn custom item or maybe custom mob (little undecided) . If I could get block pos and check it, I could probably spawn item. This has issues (eg. mining diamond ore would have a chance to give item if diamond fell in lava) I would rather use ItemTossEvent. I am hoping that I can spawn custom item in on toss event and copy motion of first item. Basically keep both items and with my custom item I should be able to set a pretty short life span and make it so you cant pick it up and if it takes damage, check if damage soure is lava. Then spawn item at players position. Does that sound right? At work for the next 12 hours so cant try anything. My guess is that theres going to be some problems like getting motion from vahnilla item wont be possible with this method. -
[1.12.2] Wanting to know if dropped item is gone.
Lea9ue replied to Lea9ue's topic in Modder Support
So it sounds like the only way to do this is to replace the EntityItem with a custom EntityItem. Don't really want to do that. Is there any way possible to instead get the block location of EntityItem to see if block is lava? -
Trying to do something for when dropped item is destroyed by something, possibly lava . Having trouble getting it. code @SubscribeEvent public static void onEvent(EntityJoinWorldEvent event) { Entity entity = event.getEntity(); World world =event.getWorld(); if(event.getEntity() instanceof EntityItem) { ItemStack stack = (((EntityItem) entity).getItem()); if(stack.getItem() == Items.DIAMOND) { System.out.println("diamond on ground"); if(event.getEntity().isDead) { System.out.println("diamonds gone"); } } } } this prints "diamond on ground" but the next if statement seems to be useless. Is there a solution I'm not thinking of. I don't know if its even possible to get position from an EntityItem to see if block is lava. I also tried to us ItemTossEvent but I don't really think I can do much with item once its tossed. Any help would be appreciated
-
What do you want to happen? blockTNT is everything you need. If you know how to register blocks then it's pretty much copy and paste. Or I maybe just extend
-
Can you verify what version you are programming for. It looks like 1.14. All I have done is in 1.12. I'm guessing though things havent changed to the point that that's not the only code you have. You should have a main class, a entity register class, an entity class, a render class, a model class. You should post all other then model.
-
RegMob(Class<? extends EntityLiving> EntityClass, String entityNameIn, int solidColorIn, int spotColorIn, int prob, int min, int max, Biome... biomes) you should probably post your code if you want help.
-
Everything is working as wanted. Checking for instance of zombie at first is not needed and might of confused. I was only doing that so when I tested and spawned in zombie and skeleton, if skeleton was the victor I would still know right away that code was working up to that point. Hopefully my last question, in this case, do I need to check if world is remote before spawning entity? example: if (!world.isRemote) { world.spawnEntity(zombie); } or is it just redundant at that point in time?
-
Thank you, should of read into that more yesterday. Current code works without crashing here: @SubscribeEvent public static void onDeath(LivingDeathEvent event) { World world = event.getEntityLiving().world; if (event.getEntity() instanceof EntityZombie) { System.out.println("Zombie"); } if (event.getEntity() instanceof EntitySkeleton) { System.out.println("Skeleton"); if (event.getSource().getTrueSource() instanceof EntityZombie) { System.out.println("Skeleton killed by Zombie"); EntityZombie zombie = new EntityZombie(world); zombie.setLocationAndAngles(event.getSource().getTrueSource().posX, event.getSource().getTrueSource().posY, event.getSource().getTrueSource().posZ, 0, 0); world.spawnEntity(zombie); } } } Is there a better way of getting it? Just want to learn.
-
I am aware that this is the problem and that my lack of java knowledge isn't helping the situation. As I said in my 2nd post, I have no idea what to do there. So thanks for the suggestion of not using quick-fixes, is there a direction you can point me in to get me on the right track?
-
My Fault Crash Report: ---- Minecraft Crash Report ---- // Don't be sad, have a hug! <3 Time: 8/22/19 5:30 PM Description: Ticking entity java.lang.NullPointerException: Ticking entity at com.lea9ue.mobwars.server.ServerEventWalkerKill.onDeath(ServerEventWalkerKill.java:42) at net.minecraftforge.fml.common.eventhandler.ASMEventHandler_13_ServerEventWalkerKill_onDeath_LivingDeathEvent.invoke(.dynamic) at net.minecraftforge.fml.common.eventhandler.ASMEventHandler.invoke(ASMEventHandler.java:90) at net.minecraftforge.fml.common.eventhandler.EventBus.post(EventBus.java:182) at net.minecraftforge.common.ForgeHooks.onLivingDeath(ForgeHooks.java:601) at net.minecraft.entity.EntityLivingBase.onDeath(EntityLivingBase.java:1284) at net.minecraft.entity.monster.EntitySkeleton.onDeath(EntitySkeleton.java:62) at net.minecraft.entity.EntityLivingBase.attackEntityFrom(EntityLivingBase.java:1129) at net.minecraft.entity.monster.EntityMob.attackEntityFrom(EntityMob.java:80) at net.minecraft.entity.monster.EntityMob.attackEntityAsMob(EntityMob.java:109) at net.minecraft.entity.monster.EntityZombie.attackEntityAsMob(EntityZombie.java:320) at net.minecraft.entity.ai.EntityAIAttackMelee.checkAndPerformAttack(EntityAIAttackMelee.java:197) at net.minecraft.entity.ai.EntityAIAttackMelee.updateTask(EntityAIAttackMelee.java:186) at net.minecraft.entity.ai.EntityAIZombieAttack.updateTask(EntityAIZombieAttack.java:39) at net.minecraft.entity.ai.EntityAITasks.onUpdateTasks(EntityAITasks.java:114) at net.minecraft.entity.EntityLiving.updateEntityActionState(EntityLiving.java:843) at net.minecraft.entity.EntityLivingBase.onLivingUpdate(EntityLivingBase.java:2582) at net.minecraft.entity.EntityLiving.onLivingUpdate(EntityLiving.java:647) at net.minecraft.entity.monster.EntityMob.onLivingUpdate(EntityMob.java:49) at net.minecraft.entity.monster.EntityZombie.onLivingUpdate(EntityZombie.java:246) at net.minecraft.entity.EntityLivingBase.onUpdate(EntityLivingBase.java:2398) at net.minecraft.entity.EntityLiving.onUpdate(EntityLiving.java:346) at net.minecraft.entity.monster.EntityMob.onUpdate(EntityMob.java:57) at net.minecraft.world.World.updateEntityWithOptionalForce(World.java:2171) at net.minecraft.world.WorldServer.updateEntityWithOptionalForce(WorldServer.java:871) at net.minecraft.world.World.updateEntity(World.java:2130) at net.minecraft.world.World.updateEntities(World.java:1931) at net.minecraft.world.WorldServer.updateEntities(WorldServer.java:643) at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:842) at net.minecraft.server.MinecraftServer.tick(MinecraftServer.java:743) at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:192) at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:592) at java.lang.Thread.run(Unknown Source) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Thread: Server thread Stacktrace: at com.lea9ue.mobwars.server.ServerEventWalkerKill.onDeath(ServerEventWalkerKill.java:42) at net.minecraftforge.fml.common.eventhandler.ASMEventHandler_13_ServerEventWalkerKill_onDeath_LivingDeathEvent.invoke(.dynamic) at net.minecraftforge.fml.common.eventhandler.ASMEventHandler.invoke(ASMEventHandler.java:90) at net.minecraftforge.fml.common.eventhandler.EventBus.post(EventBus.java:182) at net.minecraftforge.common.ForgeHooks.onLivingDeath(ForgeHooks.java:601) at net.minecraft.entity.EntityLivingBase.onDeath(EntityLivingBase.java:1284) at net.minecraft.entity.monster.EntitySkeleton.onDeath(EntitySkeleton.java:62) at net.minecraft.entity.EntityLivingBase.attackEntityFrom(EntityLivingBase.java:1129) at net.minecraft.entity.monster.EntityMob.attackEntityFrom(EntityMob.java:80) at net.minecraft.entity.monster.EntityMob.attackEntityAsMob(EntityMob.java:109) at net.minecraft.entity.monster.EntityZombie.attackEntityAsMob(EntityZombie.java:320) at net.minecraft.entity.ai.EntityAIAttackMelee.checkAndPerformAttack(EntityAIAttackMelee.java:197) at net.minecraft.entity.ai.EntityAIAttackMelee.updateTask(EntityAIAttackMelee.java:186) at net.minecraft.entity.ai.EntityAIZombieAttack.updateTask(EntityAIZombieAttack.java:39) at net.minecraft.entity.ai.EntityAITasks.onUpdateTasks(EntityAITasks.java:114) at net.minecraft.entity.EntityLiving.updateEntityActionState(EntityLiving.java:843) at net.minecraft.entity.EntityLivingBase.onLivingUpdate(EntityLivingBase.java:2582) at net.minecraft.entity.EntityLiving.onLivingUpdate(EntityLiving.java:647) at net.minecraft.entity.monster.EntityMob.onLivingUpdate(EntityMob.java:49) at net.minecraft.entity.monster.EntityZombie.onLivingUpdate(EntityZombie.java:246) at net.minecraft.entity.EntityLivingBase.onUpdate(EntityLivingBase.java:2398) at net.minecraft.entity.EntityLiving.onUpdate(EntityLiving.java:346) at net.minecraft.entity.monster.EntityMob.onUpdate(EntityMob.java:57) at net.minecraft.world.World.updateEntityWithOptionalForce(World.java:2171) at net.minecraft.world.WorldServer.updateEntityWithOptionalForce(WorldServer.java:871) at net.minecraft.world.World.updateEntity(World.java:2130) -- Entity being ticked -- Details: Entity Type: minecraft:zombie (net.minecraft.entity.monster.EntityZombie) Entity ID: 24633 Entity Name: Zombie Entity's Exact location: -62.46, 202.00, -38.73 Entity's Block location: World: (-63,202,-39), Chunk: (at 1,12,9 in -4,-3; contains blocks -64,0,-48 to -49,255,-33), Region: (-1,-1; contains chunks -32,-32 to -1,-1, blocks -512,0,-512 to -1,255,-1) Entity's Momentum: 0.00, -0.08, -0.06 Entity's Passengers: [] Entity's Vehicle: ~~ERROR~~ NullPointerException: null Stacktrace: at net.minecraft.world.World.updateEntities(World.java:1931) at net.minecraft.world.WorldServer.updateEntities(WorldServer.java:643) -- Affected level -- Details: Level name: New World All players: 1 total; [EntityPlayerMP['Player517'/598, l='New World', x=-58.45, y=203.04, z=-38.26]] Chunk stats: ServerChunkCache: 767 Drop: 0 Level seed: 6659549246155252085 Level generator: ID 00 - default, ver 1. Features enabled: true Level generator options: Level spawn location: World: (116,64,88), Chunk: (at 4,4,8 in 7,5; contains blocks 112,0,80 to 127,255,95), Region: (0,0; contains chunks 0,0 to 31,31, blocks 0,0,0 to 511,255,511) Level time: 98358 game time, 21320 day time Level dimension: 0 Level storage version: 0x04ABD - Anvil Level weather: Rain time: 39332 (now: false), thunder time: 70297 (now: false) Level game mode: Game mode: creative (ID 1). Hardcore: false. Cheats: true Stacktrace: at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:842) at net.minecraft.server.MinecraftServer.tick(MinecraftServer.java:743) at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:192) at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:592) at java.lang.Thread.run(Unknown Source) -- System Details -- Details: Minecraft Version: 1.12.2 Operating System: Windows 10 (amd64) version 10.0 Java Version: 1.8.0_221, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 728052120 bytes (694 MB) / 1037959168 bytes (989 MB) up to 1037959168 bytes (989 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M IntCache: cache: 0, tcache: 0, allocated: 12, tallocated: 94 FML: MCP 9.42 Powered by Forge 14.23.5.2768 5 mods loaded, 5 mods active States: 'U' = Unloaded 'L' = Loaded 'C' = Constructed 'H' = Pre-initialized 'I' = Initialized 'J' = Post-initialized 'A' = Available 'D' = Disabled 'E' = Errored | State | ID | Version | Source | Signature | |:--------- |:--------- |:------------ |:-------------------------------- |:--------- | | UCHIJAAAA | minecraft | 1.12.2 | minecraft.jar | None | | UCHIJAAAA | mcp | 9.42 | minecraft.jar | None | | UCHIJAAAA | FML | 8.0.99.99 | forgeSrc-1.12.2-14.23.5.2768.jar | None | | UCHIJAAAA | forge | 14.23.5.2768 | forgeSrc-1.12.2-14.23.5.2768.jar | None | | UCHIJAAAA | mobwars | 0.1.0 | bin | None | Loaded coremods (and transformers): GL info: ~~ERROR~~ RuntimeException: No OpenGL context found in the current thread. Profiler Position: N/A (disabled) Player Count: 1 / 8; [EntityPlayerMP['Player517'/598, l='New World', x=-58.45, y=203.04, z=-38.26]] Type: Integrated Server (map_client.txt) Is Modded: Definitely; Client brand changed to 'fml,forge'
-
I don't know. Yes I get that the entities have access to the world they are In . I'm just not smart enough to implement it. Heres what code looks like but it crashes when entity spawns private static World worldIn; @SubscribeEvent public static void onDeath(LivingDeathEvent event) { if (event.getEntity() instanceof EntityZombie) { System.out.println("Zombie"); } if (event.getEntity() instanceof EntitySkeleton) { System.out.println("Skeleton"); if (event.getSource().getTrueSource() instanceof EntityZombie) { System.out.println("Skeleton killed by Zombie"); EntityZombie zombie = new EntityZombie(worldIn); zombie.setLocationAndAngles(event.getSource().getTrueSource().posX,event.getSource().getTrueSource().posY,event.getSource().getTrueSource().posZ,0,0); worldIn.spawnEntity(zombie); } } }
-
In my first code example I can get it to work only if I use public static void. Public void I'm assuming doesn't work since I'm not getting messages in Console (eclipse). The spawning code EntityZombie zombie = new EntityZombie(this.world); I used in the third example is typically what I would use when spawning a entity but I cant use (this.world) in a static class (public static void). For setting Location of spawn I'm trying to use zombie.setLocationAndAngles(event.getSource().getTrueSource().posX, event.getSource().getTrueSource().posY, event.getSource().getTrueSource().posZ, 0, 0); which I believe should work fine. Then for the actual spawning typically I would use this.world.spawnEntity(zombie); So the over all code that I'm stuck with is @SubscribeEvent public void onDeath(LivingDeathEvent event) { if (event.getEntity() instanceof EntitySkeleton) { if (event.getSource().getTrueSource() instanceof EntityZombie) { EntityZombie zombie = new EntityZombie(//NO IDEA WHAT TO PUT HERE//); zombie.setLocationAndAngles(event.getSource().getTrueSource().posX, event.getSource().getTrueSource().posY, event.getSource().getTrueSource().posZ,0,0); (///NO IDEA WHAT TO PUT HERE///).spawnEntity(walker); } } } So as you can see, my amateur java brain can't seem to grasp what to do. I think I'm atleast close. Any advice would be appreciated.
-
Code works as is, but cant get a Entity to spawn in a static event. Am I missing something? What I am trying to achieve is when an entity dies, it checks to see what entity was killed and then what entity did the killing. If it was Zombie that did the killing, I would like to spawn another ZombieEntity in the place of the dead entity. Any other way of doing this that im not thinking of? ServerEventZombieKill.class: { @SubscribeEvent public static void onDeath(LivingDeathEvent event) { if (event.getEntity() instanceof EntityZombie) { System.out.println("Zombie"); } if (event.getEntity() instanceof EntitySkeleton) { System.out.println("Skeleton"); if (event.getSource().getTrueSource() instanceof EntityZombie) { System.out.println("Skeleton killed by Zombie"); } } } Main: @EventHandler public void init2(FMLPreInitializationEvent event) { MinecraftForge.EVENT_BUS.register(ServerEventZombieKill.class); I can get the outcome I want in my custom entity class like this: @Override public void onKillEntity(EntityLivingBase entityLivingIn) { if (entityLivingIn instanceof IMob) { System.out.println("Mob"); EntityWalker walker = new EntityWalker(this.world); walker.setLocationAndAngles(entityLivingIn.posX, entityLivingIn.posY, entityLivingIn.posZ, 0, 0); if (!entityLivingIn.world.isRemote) { this.world.spawnEntity(walker); } } } ^^^^ But obviously I cant do that method to Vanilla Entities.