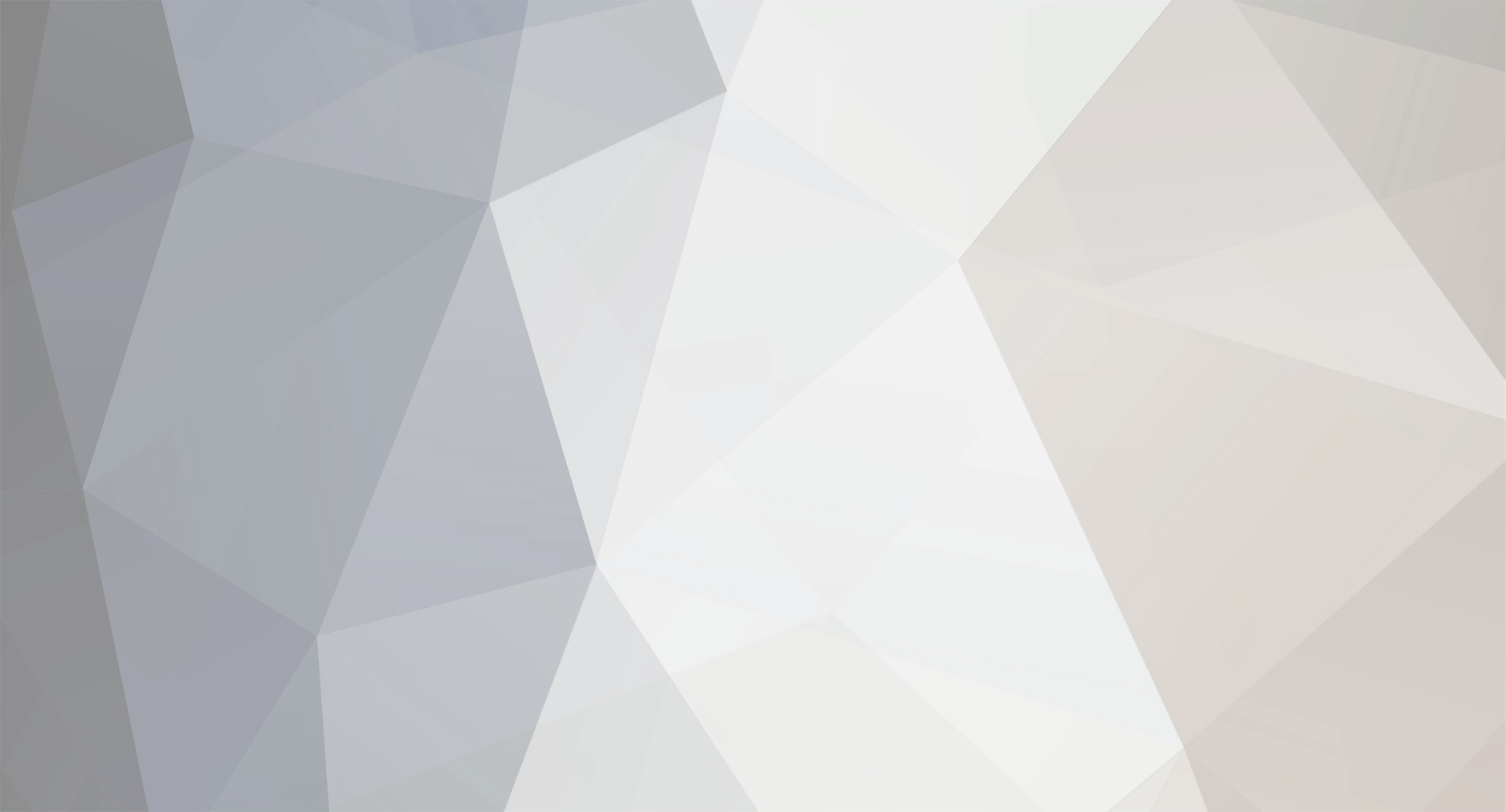
squidlex
Members-
Posts
183 -
Joined
-
Last visited
Everything posted by squidlex
-
[1.15.2] [Solved] Custom arrow entity not rendering
squidlex replied to squidlex's topic in Modder Support
I have seen that some people are able to use the actual entity class itself in registerEntityRenderingHandler RenderingRegistry.registerEntityRenderingHandler(CustomArrowEntity.class, CustomArrowRenderer::new); However I get the error The method registerEntityRenderingHandler(EntityType<T>, IRenderFactory<? super T>) in the type RenderingRegistry is not applicable for the arguments (Class<CustomngArrowEntity>, IRenderFactory<? super T>) When I attempt this. -
[1.15.2] [Solved] Custom arrow entity not rendering
squidlex replied to squidlex's topic in Modder Support
Thanks for all your help, I've made every fix suggested. Unfortunately my arrow still isn't rendering so I will try and look through some source code to see how other people have achieved it. Thanks again. -
I am trying to create a custom arrow however when it is fired from the bow the arrow itself is not rendered. The entity is created however. Here's my code: Combat.java @Mod("combat") public class Combat { public static final Logger LOGGER = LogManager.getLogger(); public static final String MODID = "combat"; public static Combat instance; public static EntityType<CustomArrowEntity> CUSTOM_ARROW_ENTITY_TYPE = EntityType.Builder .<CustomArrowEntity>create(CustomArrowEntity::new, EntityClassification.MISC).size(0.5f, 0.5f) .build("custom_arrow"); public Combat() { final IEventBus modEventBus = FMLJavaModLoadingContext.get().getModEventBus(); modEventBus.addListener(this::setup); modEventBus.addListener(this::doClientStuff); ModEntityTypes.ENTITY_TYPES.register(modEventBus); instance = this; ModLoadingContext.get().registerConfig(net.minecraftforge.fml.config.ModConfig.Type.SERVER, ModConfig.SPEC); ModConfig.init(FMLPaths.CONFIGDIR.get().resolve(MODID + "-server.toml")); MinecraftForge.EVENT_BUS.register(this); } private void setup(final FMLCommonSetupEvent event) { Keybinds.register(); MinecraftForge.EVENT_BUS.register(new CombatGui()); } private void doClientStuff(final FMLClientSetupEvent event) { LOGGER.info("Got game settings {}", event.getMinecraftSupplier().get().gameSettings); } // Register Entities @Mod.EventBusSubscriber(bus = Mod.EventBusSubscriber.Bus.MOD) public static class RegistryEvents { @SubscribeEvent public static void registerEntityTypes(final RegistryEvent.Register<EntityType<?>> event) { event.getRegistry().register( CUSTOM_ARROW_ENTITY_TYPE.setRegistryName(new ResourceLocation("combat", "custom_arrow"))); } } @SubscribeEvent public void onServerStarting(FMLServerStartingEvent event) { LOGGER.info("HELLO from server starting"); } } CustomArrowEntity.java public class CustomArrowEntity extends AbstractArrowEntity { public CustomArrowEntity(EntityType<? extends CustomArrowEntity> p_i50172_1_, World p_i50172_2_) { super(p_i50172_1_, p_i50172_2_); } public CustomArrowEntity(World worldIn, double x, double y, double z) { super(Combat.CUSTOM_ARROW_ENTITY_TYPE, x, y, z, worldIn); } public CustomArrowEntity(World worldIn, LivingEntity shooter) { super(Combat.CUSTOM_ARROW_ENTITY_TYPE, shooter, worldIn); } protected void arrowHit(LivingEntity living) { super.arrowHit(living); } protected ItemStack getArrowStack() { return new ItemStack(ItemInit.custom_arrow); } } ModelHandler.java @EventBusSubscriber(value = Dist.CLIENT, modid = Combat.MODID, bus = EventBusSubscriber.Bus.MOD) public class ModelHandler { @SubscribeEvent public static void registerModels(ModelRegistryEvent event) { RenderingRegistry.registerEntityRenderingHandler(Combat.CUSTOM_ARROW_ENTITY_TYPE, CustomArrowRenderer::new); } } CustomArrowRenderer.java @OnlyIn(Dist.CLIENT) public class CustomArrowRenderer<T extends CustomArrowEntity> extends ArrowRenderer<T> { public static final ResourceLocation CUSTOM_ARROW_RL = new ResourceLocation(Combat.MODID, "textures/entity/projectiles/custom_arrow.png"); public CustomArrowRenderer(EntityRendererManager renderManagerIn) { super(renderManagerIn); } @Override public ResourceLocation getEntityTexture(CustomArrowEntity entity) { return CUSTOM_ARROW_RL; } } Apologies for the long question. Thank you for your help!
-
[1.15.2] how to use LivingKnockbackEvent with projectiles
squidlex replied to squidlex's topic in Modder Support
Turns out my damage source in my EntityClass was wrong, I'd put in my values backwards. entity.attackEntityFrom(DamageSource.causeThrownDamage(this.getThrower(), this), 1f); Is correct I think. -
I wan't to disable knockback if an entity is hit by my throwable item, however if (event.getAttacker() instanceof MyThrowableEntity) { //DO SOMETHING } Isn't working, as when I throw my item it is read as the player dealing damage, not the thrown entity. Is there anyway I can detect if an entity was hit by my thrown item entity? I've been stuck on this for a while, thanks for your help.
-
Figured it out for my needs at least, here's my code should anyone need it: @EventBusSubscriber(modid = Combat.MODID) public class ModParticleTypes { public static final IParticleData SHURIKEN_PARTICLE = new BlockParticleData(ParticleTypes.BLOCK, Blocks.IRON_BLOCK.getDefaultState()); }
-
How would I add a custom particle to the game? I've looked at Minecraft's ParticleTypes class but I'm confused. Thank you!