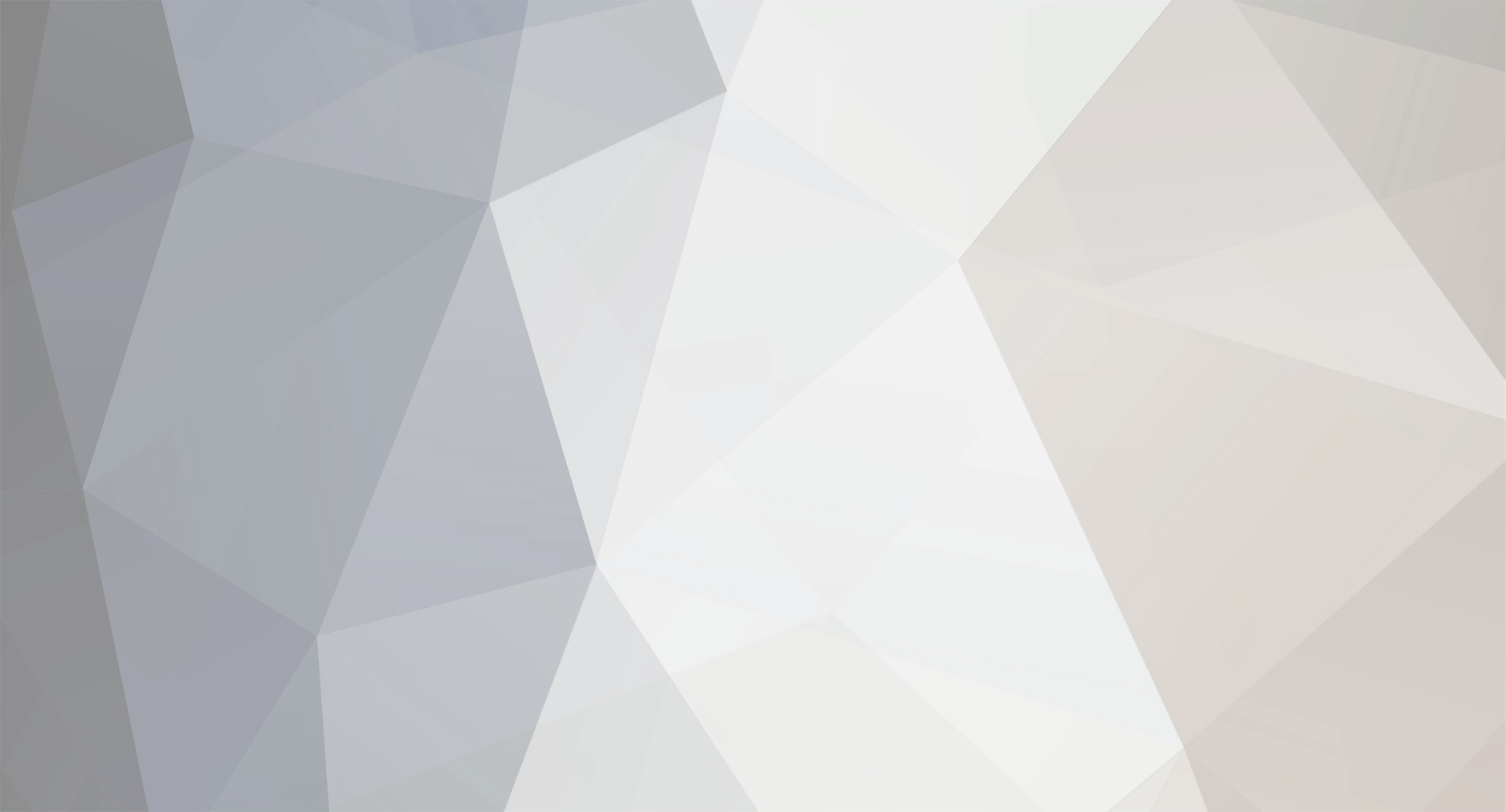
ken2020
Members-
Posts
15 -
Joined
-
Last visited
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
ken2020's Achievements

Tree Puncher (2/8)
0
Reputation
-
Villagers don't recognize special type of door as a door.
ken2020 replied to ken2020's topic in Modder Support
Ok I did this: { "replace": false, "values": [ "minecraft:oak_door", "minecraft:spruce_door", "minecraft:birch_door", "minecraft:jungle_door", "minecraft:acacia_door", "minecraft:dark_oak_door", "minecraft:crimson_door", "minecraft:warped_door", "kenmod:oak_cabin_door" ] } { "replace": false, "values": [ "minecraft:oak_door", "minecraft:spruce_door", "minecraft:birch_door", "minecraft:jungle_door", "minecraft:acacia_door", "minecraft:dark_oak_door", "minecraft:crimson_door", "minecraft:warped_door", "kenmod:oak_cabin_door" ] } But they still don't open the door. -
Hello, I have tried to update the code to my door. The door is a special type of door where instead of hanging on the edge of a block, it goes through the center. For some reason however, the villagers do not recognize it as a door, and therefore never use it. How can I add to my code to make them use it? package com.kenmod.objects.blocks.doors; import net.minecraft.block.Block; import net.minecraft.block.BlockState; import net.minecraft.block.DoorBlock; import net.minecraft.block.HorizontalBlock; import net.minecraft.state.BooleanProperty; import net.minecraft.state.DirectionProperty; import net.minecraft.state.EnumProperty; import net.minecraft.state.Property; import net.minecraft.state.StateContainer.Builder; import net.minecraft.state.properties.BlockStateProperties; import net.minecraft.state.properties.DoorHingeSide; import net.minecraft.state.properties.DoubleBlockHalf; import net.minecraft.util.Direction; import net.minecraft.util.math.BlockPos; import net.minecraft.util.math.shapes.ISelectionContext; import net.minecraft.util.math.shapes.VoxelShape; import net.minecraft.world.IBlockReader; public class CenteredDoor extends DoorBlock { public static final DirectionProperty FACING = HorizontalBlock.HORIZONTAL_FACING; public static final BooleanProperty OPEN = BlockStateProperties.OPEN; public static final EnumProperty<DoorHingeSide> HINGE = BlockStateProperties.DOOR_HINGE; public static final BooleanProperty POWERED = BlockStateProperties.POWERED; public static final EnumProperty<DoubleBlockHalf> HALF = BlockStateProperties.DOUBLE_BLOCK_HALF; protected static final VoxelShape default_shape = Block.makeCuboidShape(0.0D, 0.0D, 7.0D, 16.0D, 16.0D, 9.0D); protected static final VoxelShape north_south = Block.makeCuboidShape(0.0D, 0.0D, 7.0D, 16.0D, 16.0D, 9.0D); protected static final VoxelShape east_west = Block.makeCuboidShape(7.0D, 0.0D, 0.0D, 9.0D, 16.0D, 16.0D); protected static final VoxelShape south_l = Block.makeCuboidShape(13.0D, 0.0D, 7.0D, 16.0D, 16.0D, 21.0D); protected static final VoxelShape south_r = Block.makeCuboidShape(0.0D, 0.0D, 7.0D, 3.0D, 16.0D, 21.0D); protected static final VoxelShape north_l = Block.makeCuboidShape(0.0D, 0.0D, 9.0D, 3.0D, 16.0D, -5.0D); protected static final VoxelShape north_r = Block.makeCuboidShape(13.0D, 0.0D, 9.0D, 16.0D, 16.0D, -5.0D); protected static final VoxelShape east_l = Block.makeCuboidShape(7.0D, 0.0D, 0.0D, 21.0D, 16.0D, 3.0D); protected static final VoxelShape east_r = Block.makeCuboidShape(7.0D, 0.0D, 13.0D, 21.0D, 16.0D, 16.0D); protected static final VoxelShape west_l = Block.makeCuboidShape(9.0D, 0.0D, 13.0D, -5.0D, 16.0D, 16.0D); protected static final VoxelShape west_r = Block.makeCuboidShape(9.0D, 0.0D, 0.0D, -5.0D, 16.0D, 3.0D); public CenteredDoor (Properties properties) { super(properties); } @Override public VoxelShape getShape(BlockState state, IBlockReader worldIn, BlockPos pos, ISelectionContext context) { Direction dir = (Direction)state.get((Property<?>)FACING); DoorHingeSide side = (DoorHingeSide)state.get((Property<DoorHingeSide>)HINGE); Boolean open = (Boolean)state.get((Property<?>)OPEN); if ((dir == Direction.NORTH || dir == Direction.SOUTH) && !open.booleanValue()) { return north_south; } if ((dir == Direction.EAST || dir == Direction.WEST) && !open.booleanValue()) { return east_west; } if (dir == Direction.NORTH && side == DoorHingeSide.LEFT) { return north_l; } if (dir == Direction.NORTH && side == DoorHingeSide.RIGHT) { return north_r; } if (dir == Direction.SOUTH && side == DoorHingeSide.LEFT) { return south_l; } if (dir == Direction.SOUTH && side == DoorHingeSide.RIGHT) { return south_r; } if (dir == Direction.EAST && side == DoorHingeSide.LEFT) { return east_l; } if (dir == Direction.EAST && side == DoorHingeSide.RIGHT) { return east_r; } if (dir == Direction.WEST && side == DoorHingeSide.LEFT) { return west_l; } if (dir == Direction.WEST && side == DoorHingeSide.RIGHT) { return west_r; } return default_shape; } @Override protected void fillStateContainer(Builder<Block, BlockState> builder) { builder.add(new Property[] { HALF, FACING, OPEN, HINGE, POWERED }); } }
-
[1.15.2] How to create 3D item models with attachments?
ken2020 replied to ken2020's topic in Modder Support
Ok so I am trying this: package com.kenmod_main.objects.items.guns.glock; import java.util.ArrayList; import java.util.List; import java.util.Random; import net.minecraft.block.BlockState; import net.minecraft.client.Minecraft; import net.minecraft.client.renderer.BlockRendererDispatcher; import net.minecraft.client.renderer.model.BakedQuad; import net.minecraft.client.renderer.model.IBakedModel; import net.minecraft.client.renderer.model.ItemOverrideList; import net.minecraft.client.renderer.texture.TextureAtlasSprite; import net.minecraft.util.Direction; import net.minecraft.util.ResourceLocation; public class ItemGlockBakedModel implements IBakedModel{ private IBakedModel baseModel; private ResourceLocation barrelLocation = new ResourceLocation("kenmod:item/glock_barrel"); @Override public List<BakedQuad> getQuads(BlockState state, Direction side, Random rand) { throw new AssertionError("IBakedModel::getQuads should never be called, only IForgeBakedModel::getQuads"); } public List<BakedQuad> barrelQuads (boolean hasBarrel){ List<BakedQuad> barrel = new ArrayList<BakedQuad>(); if(hasBarrel) { } return barrel; } @Override public boolean isAmbientOcclusion() { return baseModel.isAmbientOcclusion(); } @Override public boolean isGui3d() { return baseModel.isGui3d(); } @Override public boolean func_230044_c_() { return baseModel.func_230044_c_(); } @Override public boolean isBuiltInRenderer() { return baseModel.isBuiltInRenderer(); } @SuppressWarnings("deprecation") @Override public TextureAtlasSprite getParticleTexture() { return baseModel.getParticleTexture(); } @Override public ItemOverrideList getOverrides() { return baseModel.getOverrides(); } } TGG's code from private List<BakedQuad> getArrowQuads(BlockAltimeter.GPScoordinate gpScoordinate, Direction whichFace) { // we construct the needle from a number of needle models (each needle model is a single cube 1x1x1) // the needle is made up of a central cube plus further cubes radiating out to a 6 texel radius // retrieve the needle model which we previously manually added to the model registry in StartupClientOnly::onModelRegistryEvent Minecraft mc = Minecraft.getInstance(); BlockRendererDispatcher blockRendererDispatcher = mc.getBlockRendererDispatcher(); IBakedModel needleModel = blockRendererDispatcher.getBlockModelShapes().getModelManager().getModel(needleModelRL); // our needle model has its minX, minY, minZ at [0,0,0] and its size is [1,1,1], so to put it at the centre of the top // of our altimeter, we need to translate it to [7.5F, 10F, 7.5F] in modelspace coordinates final float CONVERT_MODEL_SPACE_TO_WORLD_SPACE = 1.0F/16.0F; Vector3f centrePos = new Vector3f(7.5F, 10F, 7.5F); centrePos.mul(CONVERT_MODEL_SPACE_TO_WORLD_SPACE); ImmutableList.Builder<BakedQuad> retval = new ImmutableList.Builder<>(); addTranslatedModelQuads(needleModel, centrePos, whichFace, retval); // make a line of needle cubes radiating out from the centre, pointing towards the origin. double bearingToOriginRadians = Math.toRadians(gpScoordinate.bearingToOrigin); // degrees clockwise from north float deltaX = (float)Math.sin(bearingToOriginRadians); float deltaZ = -(float)Math.cos(bearingToOriginRadians); if (Math.abs(deltaX) < Math.abs(deltaZ)) { deltaX /= Math.abs(deltaZ); deltaZ /= Math.abs(deltaZ); } else { deltaZ /= Math.abs(deltaX); deltaX /= Math.abs(deltaX); } float xoffset = 0; float zoffset = 0; final int NUMBER_OF_NEEDLE_BLOCKS = 6; // not including centre for (int i = 0; i < NUMBER_OF_NEEDLE_BLOCKS; ++i) { xoffset += deltaX * CONVERT_MODEL_SPACE_TO_WORLD_SPACE; zoffset += deltaZ * CONVERT_MODEL_SPACE_TO_WORLD_SPACE; Vector3f moveTo = centrePos.copy(); moveTo.add(xoffset, 0, zoffset); addTranslatedModelQuads(needleModel, moveTo, whichFace, retval); } uses something to do with block rendering. What should I change to make it work for items? -
[1.15.2] How to create 3D item models with attachments?
ken2020 replied to ken2020's topic in Modder Support
where in mbe04 does it show how to combine json models? I can't seem to find it. -
[1.15.2] How to create 3D item models with attachments?
ken2020 replied to ken2020's topic in Modder Support
Could you point me to an example of what you are talking about. I get the idea, but I have never tried it before. -
[1.15.2] How to create 3D item models with attachments?
ken2020 replied to ken2020's topic in Modder Support
I dont think you understand what I am getting at... I am asking if the player is just holding the gun, the game renders only the gun. But if the player puts on a scope, the game renders both the gun and scope. What if the scope and gun are two different json models? How would I render both of them on the same Item? -
[1.15.2] How to create 3D item models with attachments?
ken2020 replied to ken2020's topic in Modder Support
The thing is the gun models have interchangable attachments and some can have more than one type of attachment. How do I render the gun and attachments. -
[1.15.2] How to create 3D item models with attachments?
ken2020 replied to ken2020's topic in Modder Support
Can you show me an example? Or point me to some documentation? Edit: What if I wanted to render the model via java? How would that work? -
Hello, I am trying to make gun models with attachments such as scopes. How can I make an item model that can have attachments that can be added/removed?
-
I don't really know how to describe it in words, so I attached an image instead. Can someone please tell me what is going on?
-
I am going to look into the poison affect to base this off of, but am wondering how would I make the player start bleeding if they are hit by a damage value over 5? Any help will be appreciated. Thanks.
-
Thanks for the fast reply. will keep that in mind.
-
I am trying to make 3d gun models for my mod but every search I do leads me to how to create a datapack instead of a mod. Can someone point me to some tutorials / documentation please?