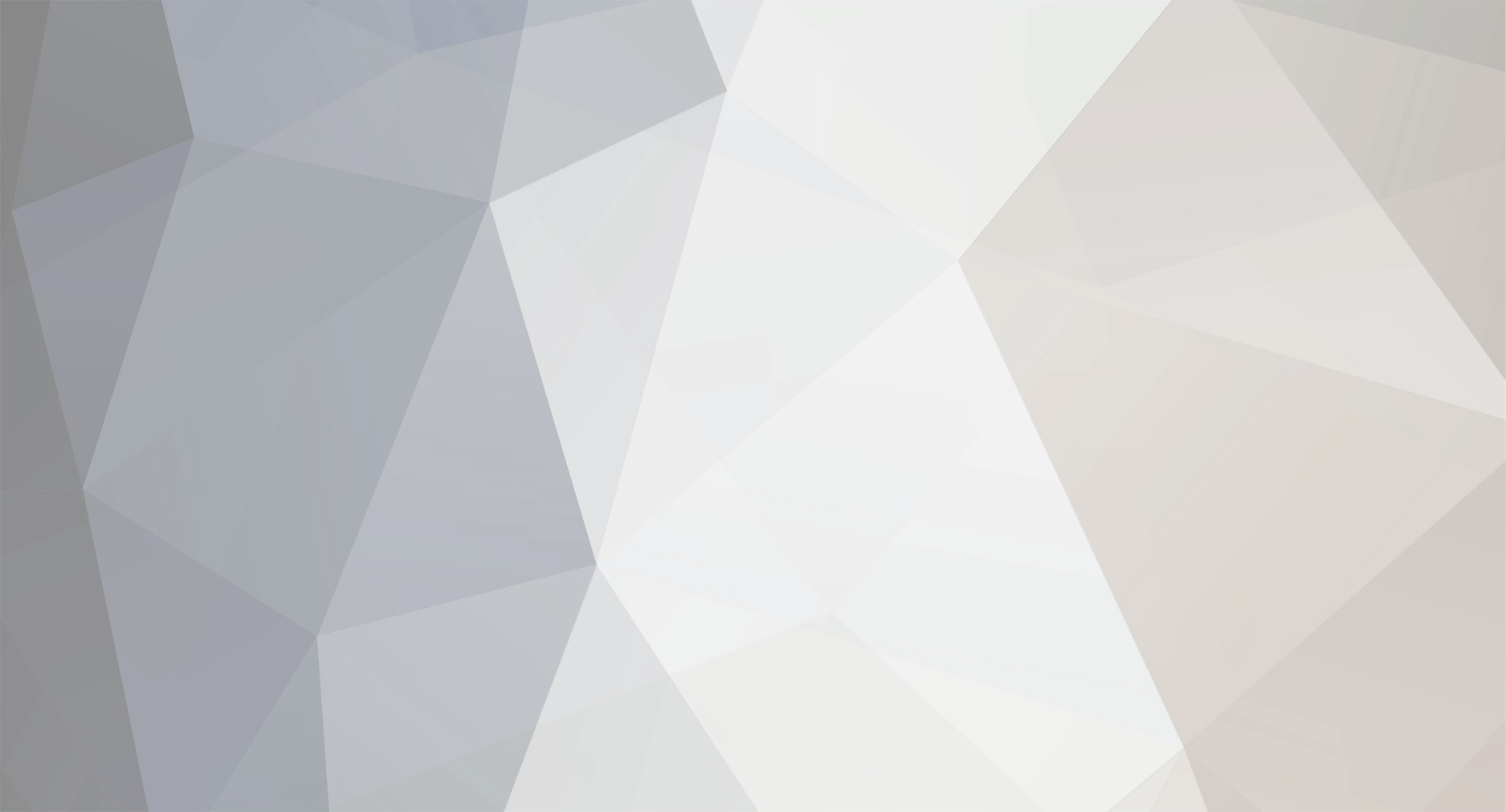
NindyBun
Members-
Posts
88 -
Joined
-
Last visited
Everything posted by NindyBun
-
[1.16.5] Custom Text Overlay not updating when playing on server
NindyBun replied to NindyBun's topic in Modder Support
So I figured out that when I try to get the nbt tag in the RenderGameOverlayEvent it doesn't show the updated values, only the values made when the item was created. Therefore how would I get the current updated tag when playing on the dedicated server? -
[1.16.5] Custom Text Overlay not updating when playing on server
NindyBun replied to NindyBun's topic in Modder Support
It might actually be this line that's causing the problem, but how else do I check if my itemstack is in a players hand so that I knows to draw the text? -
So I have a FontRenderer that displays the Fuel value of my tool, on a singleplayer world the text updates fine but once I go on the server the same text does not update. So I'm assuming that I would have to send the server packet to the client, problem is how would I do that if I need a ServerPlayerEntity. My Font renderer: https://github.com/NindyBun/BurnerGun_1.16.5/blob/master/src/main/java/com/nindybun/burnergun/client/renderer/FuelValueRenderer.java package com.nindybun.burnergun.client.renderer; import com.nindybun.burnergun.common.BurnerGun; import com.nindybun.burnergun.common.items.upgrades.Upgrade; import com.nindybun.burnergun.common.network.PacketHandler; import net.minecraft.client.Minecraft; import net.minecraft.client.gui.FontRenderer; import net.minecraft.entity.player.PlayerEntity; import net.minecraft.item.ItemStack; import net.minecraftforge.api.distmarker.Dist; import net.minecraftforge.client.event.RenderGameOverlayEvent; import net.minecraftforge.eventbus.api.SubscribeEvent; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.items.CapabilityItemHandler; import net.minecraftforge.items.IItemHandler; import org.apache.commons.lang3.StringUtils; import org.apache.logging.log4j.LogManager; import org.apache.logging.log4j.Logger; import javax.annotation.Nonnull; import java.awt.*; @Mod.EventBusSubscriber(modid = BurnerGun.MOD_ID, value = Dist.CLIENT) public class FuelValueRenderer { private static final Logger LOGGER = LogManager.getLogger(); private static final int base_buffer = com.nindybun.burnergun.common.items.Burner_Gun.BurnerGun.base_use_buffer; private static final int base_heat_buffer = com.nindybun.burnergun.common.items.Burner_Gun.BurnerGun.base_heat_buffer; @SubscribeEvent public static void renderOverlay(@Nonnull RenderGameOverlayEvent.Post event){ if (event.getType() == RenderGameOverlayEvent.ElementType.ALL){ ItemStack stack = ItemStack.EMPTY; PlayerEntity player = Minecraft.getInstance().player; if (player.getMainHandItem().getItem() instanceof com.nindybun.burnergun.common.items.Burner_Gun.BurnerGun) stack = player.getMainHandItem(); else if (player.getOffhandItem().getItem() instanceof com.nindybun.burnergun.common.items.Burner_Gun.BurnerGun) stack = player.getOffhandItem(); if (stack.getItem() instanceof com.nindybun.burnergun.common.items.Burner_Gun.BurnerGun) renderFuel(event, stack); } } public static void renderFuel(RenderGameOverlayEvent event, ItemStack stack){ FontRenderer fontRenderer = Minecraft.getInstance().font; IItemHandler handler = stack.getCapability(CapabilityItemHandler.ITEM_HANDLER_CAPABILITY).orElse(null); int level = stack.getTag().getInt("FuelValue"); int hLevel = stack.getTag().getInt("HeatValue"); Color color; if (level > base_buffer*3/4) color = Color.GREEN; else if (level > base_buffer*1/4 && level <= base_buffer*3/4) color = Color.ORANGE; else color = Color.RED; if (hLevel > base_heat_buffer*3/4) color = Color.RED; else if (hLevel > base_heat_buffer*1/4 && hLevel <= base_heat_buffer*3/4) color = Color.ORANGE; else color = Color.GREEN; //fontRenderer.drawString(event.getMatrixStack(), "Fuel level: "+level, 6, event.getWindow().getScaledHeight()-12, Color.WHITE.getRGB()); if (!handler.getStackInSlot(0).getItem().equals(Upgrade.UNIFUEL.getCard().getItem()) && !handler.getStackInSlot(0).getItem().equals(Upgrade.REACTOR.getCard().getItem())){ fontRenderer.draw(event.getMatrixStack(), "Fuel level: ", 6, event.getWindow().getGuiScaledHeight()-12, Color.WHITE.getRGB()); fontRenderer.draw(event.getMatrixStack(), level+"", 61, event.getWindow().getGuiScaledHeight()-12, color.getRGB()); }else if (handler.getStackInSlot(0).getItem().equals(Upgrade.REACTOR.getCard().getItem())){ double heat = (double)hLevel/base_heat_buffer*100; String heatString = heat+""; int deci = heatString.lastIndexOf("."); heatString = heatString.substring(0, deci+2); fontRenderer.draw(event.getMatrixStack(), "Heat level: ", 6, event.getWindow().getGuiScaledHeight()-12, Color.WHITE.getRGB()); fontRenderer.draw(event.getMatrixStack(), heatString+"%", 61, event.getWindow().getGuiScaledHeight()-12, color.getRGB()); } } } My packet handler package com.nindybun.burnergun.common.network; import com.nindybun.burnergun.common.BurnerGun; import com.nindybun.burnergun.common.network.packets.PacketOpenAutoFuelGui; import com.nindybun.burnergun.common.network.packets.PacketOpenBurnerGunGui; import com.nindybun.burnergun.common.network.packets.PacketOpenTrashGui; import com.nindybun.burnergun.common.network.packets.PacketOpenUpgradeBagGui; import net.minecraft.entity.player.ServerPlayerEntity; import net.minecraft.util.ResourceLocation; import net.minecraftforge.common.util.FakePlayer; import net.minecraftforge.fml.network.NetworkDirection; import net.minecraftforge.fml.network.NetworkRegistry; import net.minecraftforge.fml.network.simple.SimpleChannel; public class PacketHandler { private static final String PROTOCOL_VERSION = "2"; private static int index = 0; public static final SimpleChannel INSTANCE = NetworkRegistry.ChannelBuilder .named(new ResourceLocation(BurnerGun.MOD_ID, "main")) .clientAcceptedVersions(PROTOCOL_VERSION::equals) .serverAcceptedVersions(PROTOCOL_VERSION::equals) .networkProtocolVersion(() -> PROTOCOL_VERSION) .simpleChannel(); public static void register(){ int id = 0; INSTANCE.registerMessage(id++, PacketOpenBurnerGunGui.class, PacketOpenBurnerGunGui::encode, PacketOpenBurnerGunGui::decode, PacketOpenBurnerGunGui.Handler::handle); INSTANCE.registerMessage(id++, PacketOpenAutoFuelGui.class, PacketOpenAutoFuelGui::encode, PacketOpenAutoFuelGui::decode, PacketOpenAutoFuelGui.Handler::handle); INSTANCE.registerMessage(id++, PacketOpenTrashGui.class, PacketOpenTrashGui::encode, PacketOpenTrashGui::decode, PacketOpenTrashGui.Handler::handle); INSTANCE.registerMessage(id++, PacketOpenUpgradeBagGui.class, PacketOpenUpgradeBagGui::encode, PacketOpenUpgradeBagGui::decode, PacketOpenUpgradeBagGui.Handler::handle); } public static void sendTo(Object msg, ServerPlayerEntity player) { if (!(player instanceof FakePlayer)) INSTANCE.sendTo(msg, player.connection.connection, NetworkDirection.PLAY_TO_CLIENT); } public static void sendToServer(Object msg){ INSTANCE.sendToServer(msg); } } Yes I know, my coding is not the best looking.
-
I have two gui's/containers one with 8 slots and the other with 15. My tool opens the former container whenever you press a keybind, but I want to open the latter using the same tool. Essentially, if I pressed a certain keybind, the 15 slot container supersedes the original 8 slot container, therefor my tool it's container gains 7 more slots while retaining whatever's in it's previous inventory. Is there a way to go about this? https://github.com/NindyBun/TestMod
-
Actually, nvm I got it.
-
public class KeyInputHandler { private static final Logger LOGGER = LogManager.getLogger(); @SubscribeEvent public void onKeyInput(InputEvent.KeyInputEvent event) { PlayerEntity player = Minecraft.getInstance().player; if (Keybinds.test_item_gui_key.isPressed() && player.getHeldItemMainhand().getItem() instanceof ItemTestItem){ LOGGER.info("KEY PRESSED"); } } } How can I open my ItemTestItem's GUI when I press the C key? Right Now I am opening it from NetworkHooks in my ItemTestItem class. @Nonnull @Override public ActionResult<ItemStack> onItemRightClick(World world, PlayerEntity player, Hand handIn) { ItemStack stack = player.getHeldItem(handIn).getStack(); if (!world.isRemote){ if (player.isSneaking()){ INamedContainerProvider containerProviderTestItem = new ContainerProviderTestItem(this, stack); final int NUMBER_OF_SLOTS = 8; NetworkHooks.openGui((ServerPlayerEntity) player, containerProviderTestItem, (packetBuffer)-> packetBuffer.writeInt(NUMBER_OF_SLOTS);}); return ActionResult.resultSuccess(stack); } } } Here's the git repo if needed: https://github.com/NindyBun/TestMod/blob/master/src/main/java/com/nindybun/miningtool/KeyInputHandler.java Apologies in advance if my code is crude.
-
https://github.com/NindyBun/TestMod/blob/master/src/main/java/com/nindybun/miningtool/test_item/ItemStackHandlerTestItem.java
-
Basically I want to make an Item Filter that allows the player to set a copy of the held item stack into a slot.
-
So I returned stack.copy() and I am still holding the stack, but when I try to set stack in slot, it doesn't set the ItemStack.
-
My item container works fine, it acts as a normal bag; but I want to turn it into a filter, so how can I make my item container set/remove "ghost" items instead of setting/removing the physical item in a slot? Thanks in advance. Note: I am asleep so I won't be able to answer till I wake up.
-
[1.15.2] How to add block loots into a players inventory?
NindyBun replied to NindyBun's topic in Modder Support
I see it works now but why does checking that do things on the server? -
[1.15.2] How to add block loots into a players inventory?
NindyBun replied to NindyBun's topic in Modder Support
Ah, the null is coming from world.getServer().getWorld(world.getDimension().getType()) where world.getServer() is null -
[1.15.2] How to add block loots into a players inventory?
NindyBun replied to NindyBun's topic in Modder Support
oh...give me a while then, I've never done anything with Intellij Debugger before so I'm wandering about. -
[1.15.2] How to add block loots into a players inventory?
NindyBun replied to NindyBun's topic in Modder Support
So it's saying to insert @NotNull for BlockState and World. -
[1.15.2] How to add block loots into a players inventory?
NindyBun replied to NindyBun's topic in Modder Support
I know it's that line, but why is it wrong? What is making it null. -
[1.15.2] How to add block loots into a players inventory?
NindyBun replied to NindyBun's topic in Modder Support
That's what I'm assuming as well. -
[1.15.2] How to add block loots into a players inventory?
NindyBun replied to NindyBun's topic in Modder Support
I would if I knew why the LootContext#Builder is throwing an error. -
[1.15.2] How to add block loots into a players inventory?
NindyBun replied to NindyBun's topic in Modder Support
I mean, the only thing I seem to be getting is a null pointer exception, and Idk why. It still gives me the result right before throwing an error so...if I just catch the error to prevent my game from crashing then all is fine right? -
[1.15.2] How to add block loots into a players inventory?
NindyBun replied to NindyBun's topic in Modder Support
it do be like that. As long as it works I guess ¯\_(ツ)_/¯ -
[1.15.2] How to add block loots into a players inventory?
NindyBun replied to NindyBun's topic in Modder Support
try{ List list = state.getDrops(new LootContext.Builder(world.getServer().getWorld(world.getDimension().getType())) .withParameter(LootParameters.TOOL, stack) .withParameter(LootParameters.POSITION, pos) .withParameter(LootParameters.BLOCK_STATE, state) ); String string = list.get(0).toString(); int count = Integer.parseInt(string.substring(0, string.indexOf(" "))); LOGGER.info(count); ItemStack stackHandler = ItemStackHelper.getAndRemove(list, 0); Item item = stackHandler.getItem(); for (int i = 0; i < count; i++){ player.inventory.addItemStackToInventory(new ItemStack(item.getItem().asItem())); } }catch (Exception e){ } I got it working now -
[1.15.2] How to add block loots into a players inventory?
NindyBun replied to NindyBun's topic in Modder Support
Actually, I just put it into a try catch block and it's fine now. But the number of drops are different from the actual drops in the world. Is that because both statements use a different random? -
[1.15.2] How to add block loots into a players inventory?
NindyBun replied to NindyBun's topic in Modder Support
[10:52:02] [Render thread/INFO] [STDOUT/]: [net.minecraft.util.registry.Bootstrap:printToSYSOUT:110]: ---- Minecraft Crash Report ---- // Hi. I'm Minecraft, and I'm a crashaholic. Time: 3/11/21 10:52 AM Description: Unexpected error java.lang.NullPointerException: Unexpected error at com.nindybun.miningtool.test_item.ItemTestItem.onItemUse(ItemTestItem.java:219) ~[main/:?] {re:classloading} at net.minecraft.item.ItemStack.lambda$onItemUse$0(ItemStack.java:174) ~[forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {re:classloading} at net.minecraft.item.ItemStack.onItemUse(ItemStack.java:189) ~[forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {re:classloading} at net.minecraft.item.ItemStack.onItemUse(ItemStack.java:174) ~[forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {re:classloading} at net.minecraft.client.multiplayer.PlayerController.func_217292_a(PlayerController.java:319) ~[forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {re:classloading,pl:runtimedistcleaner:A} at net.minecraft.client.Minecraft.rightClickMouse(Minecraft.java:1334) ~[forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {re:classloading,pl:accesstransformer:B,pl:runtimedistcleaner:A} at net.minecraft.client.Minecraft.processKeyBinds(Minecraft.java:1601) ~[forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {re:classloading,pl:accesstransformer:B,pl:runtimedistcleaner:A} at net.minecraft.client.Minecraft.runTick(Minecraft.java:1434) ~[forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {re:classloading,pl:accesstransformer:B,pl:runtimedistcleaner:A} at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:942) ~[forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {re:classloading,pl:accesstransformer:B,pl:runtimedistcleaner:A} at net.minecraft.client.Minecraft.run(Minecraft.java:559) ~[forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {re:classloading,pl:accesstransformer:B,pl:runtimedistcleaner:A} at net.minecraft.client.main.Main.main(Main.java:177) ~[forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {re:classloading,pl:runtimedistcleaner:A} at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_251] {} at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_251] {} at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_251] {} at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_251] {} at net.minecraftforge.userdev.FMLUserdevClientLaunchProvider.lambda$launchService$0(FMLUserdevClientLaunchProvider.java:55) ~[forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {} at cpw.mods.modlauncher.LaunchServiceHandlerDecorator.launch(LaunchServiceHandlerDecorator.java:37) [modlauncher-5.0.0-milestone.4.jar:?] {} at cpw.mods.modlauncher.LaunchServiceHandler.launch(LaunchServiceHandler.java:54) [modlauncher-5.0.0-milestone.4.jar:?] {} at cpw.mods.modlauncher.LaunchServiceHandler.launch(LaunchServiceHandler.java:72) [modlauncher-5.0.0-milestone.4.jar:?] {} at cpw.mods.modlauncher.Launcher.run(Launcher.java:81) [modlauncher-5.0.0-milestone.4.jar:?] {} at cpw.mods.modlauncher.Launcher.main(Launcher.java:65) [modlauncher-5.0.0-milestone.4.jar:?] {} at net.minecraftforge.userdev.LaunchTesting.main(LaunchTesting.java:102) [forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {} A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Thread: Render thread Stacktrace: at com.nindybun.miningtool.test_item.ItemTestItem.onItemUse(ItemTestItem.java:219) at net.minecraft.item.ItemStack.lambda$onItemUse$0(ItemStack.java:174) at net.minecraft.item.ItemStack.onItemUse(ItemStack.java:189) at net.minecraft.item.ItemStack.onItemUse(ItemStack.java:174) at net.minecraft.client.multiplayer.PlayerController.func_217292_a(PlayerController.java:319) at net.minecraft.client.Minecraft.rightClickMouse(Minecraft.java:1334) at net.minecraft.client.Minecraft.processKeyBinds(Minecraft.java:1601) -- Affected level -- Details: All players: 1 total; [ClientPlayerEntity['Dev'/62, l='MpServer', x=-534.87, y=64.00, z=134.57]] Chunk stats: Client Chunk Cache: 841, 140 Level dimension: DimensionType{minecraft:overworld} Level name: MpServer Level seed: 8179760609708042029 Level generator: ID 00 - default, ver 1. Features enabled: false Level generator options: {} Level spawn location: World: (-160,63,-16), Chunk: (at 0,3,0 in -10,-1; contains blocks -160,0,-16 to -145,255,-1), Region: (-1,-1; contains chunks -32,-32 to -1,-1, blocks -512,0,-512 to -1,255,-1) Level time: 370469 game time, 1144 day time Known server brands: Level was modded: false Level storage version: 0x00000 - Unknown? Level weather: Rain time: 0 (now: true), thunder time: 0 (now: false) Level game mode: Game mode: survival (ID 0). Hardcore: false. Cheats: false Server brand: forge Server type: Integrated singleplayer server Stacktrace: at net.minecraft.client.world.ClientWorld.fillCrashReport(ClientWorld.java:457) at net.minecraft.client.Minecraft.addGraphicsAndWorldToCrashReport(Minecraft.java:1840) at net.minecraft.client.Minecraft.run(Minecraft.java:578) at net.minecraft.client.main.Main.main(Main.java:177) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.userdev.FMLUserdevClientLaunchProvider.lambda$launchService$0(FMLUserdevClientLaunchProvider.java:55) at cpw.mods.modlauncher.LaunchServiceHandlerDecorator.launch(LaunchServiceHandlerDecorator.java:37) at cpw.mods.modlauncher.LaunchServiceHandler.launch(LaunchServiceHandler.java:54) at cpw.mods.modlauncher.LaunchServiceHandler.launch(LaunchServiceHandler.java:72) at cpw.mods.modlauncher.Launcher.run(Launcher.java:81) at cpw.mods.modlauncher.Launcher.main(Launcher.java:65) at net.minecraftforge.userdev.LaunchTesting.main(LaunchTesting.java:102) -- System Details -- Details: Minecraft Version: 1.15.2 Minecraft Version ID: 1.15.2 Operating System: Windows 10 (amd64) version 10.0 Java Version: 1.8.0_251, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 755686792 bytes (720 MB) / 2043674624 bytes (1949 MB) up to 3803185152 bytes (3627 MB) CPUs: 16 JVM Flags: 1 total; -XX:HeapDumpPath=MojangTricksIntelDriversForPerformance_javaw.exe_minecraft.exe.heapdump ModLauncher: 5.0.0-milestone.4+67+b1a340b ModLauncher launch target: fmluserdevclient ModLauncher naming: mcp ModLauncher services: /eventbus-2.0.0-milestone.1-service.jar eventbus PLUGINSERVICE /forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-launcher.jar object_holder_definalize PLUGINSERVICE /forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-launcher.jar runtime_enum_extender PLUGINSERVICE /accesstransformers-2.0.0-milestone.1-shadowed.jar accesstransformer PLUGINSERVICE /forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-launcher.jar capability_inject_definalize PLUGINSERVICE /forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-launcher.jar runtimedistcleaner PLUGINSERVICE /forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-launcher.jar fml TRANSFORMATIONSERVICE FML: 31.1 Forge: net.minecraftforge:31.1.0 FML Language Providers: javafml@31.1 minecraft@1 Mod List: client-extra.jar Minecraft {minecraft@1.15.2 DONE} main Mining Tool {miningtool@1.15.2-1.0.0 DONE} forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar Forge {forge@31.1.0 DONE} Launched Version: MOD_DEV Backend library: LWJGL version 3.2.2 build 10 Backend API: GeForce GTX 1050 Ti/PCIe/SSE2 GL version 4.6.0 NVIDIA 456.71, NVIDIA Corporation GL Caps: Using framebuffer using OpenGL 3.0 Using VBOs: Yes Is Modded: Definitely; Client brand changed to 'forge' Type: Client (map_client.txt) Resource Packs: Current Language: English (US) CPU: 16x AMD Ryzen 7 1700X Eight-Core Processor [10:52:02] [Render thread/INFO] [STDOUT/]: [net.minecraft.util.registry.Bootstrap:printToSYSOUT:110]: #@!@# Game crashed! Crash report saved to: #@!@# C:\Users\ANDY\Desktop\Minecraft Modding\MiningTool\run\.\crash-reports\crash-2021-03-11_10.52.02-client.txt AL lib: (EE) alc_cleanup: 1 device not closed Process finished with exit code -1 -
[1.15.2] How to add block loots into a players inventory?
NindyBun replied to NindyBun's topic in Modder Support
LootContext.Builder lootcontext = (new LootContext.Builder(world.getServer().getWorld(world.getDimension().getType()))).withParameter(LootParameters.POSITION, pos).withParameter(LootParameters.BLOCK_STATE, state).withParameter(LootParameters.TOOL, stack).withNullableParameter(LootParameters.THIS_ENTITY, player).withNullableParameter(LootParameters.BLOCK_ENTITY, world.getTileEntity(pos)); LOGGER.info(state.getDrops(lootcontext)); [10:52:01] [Render thread/FATAL] [minecraft/Minecraft]: Unreported exception thrown! java.lang.NullPointerException: null at com.nindybun.miningtool.test_item.ItemTestItem.onItemUse(ItemTestItem.java:219) ~[main/:?] {re:classloading} at net.minecraft.item.ItemStack.lambda$onItemUse$0(ItemStack.java:174) ~[forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {re:classloading} at net.minecraft.item.ItemStack.onItemUse(ItemStack.java:189) ~[forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {re:classloading} at net.minecraft.item.ItemStack.onItemUse(ItemStack.java:174) ~[forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {re:classloading} at net.minecraft.client.multiplayer.PlayerController.func_217292_a(PlayerController.java:319) ~[forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {re:classloading,pl:runtimedistcleaner:A} at net.minecraft.client.Minecraft.rightClickMouse(Minecraft.java:1334) ~[forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {re:classloading,pl:accesstransformer:B,pl:runtimedistcleaner:A} at net.minecraft.client.Minecraft.processKeyBinds(Minecraft.java:1601) ~[forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {re:classloading,pl:accesstransformer:B,pl:runtimedistcleaner:A} at net.minecraft.client.Minecraft.runTick(Minecraft.java:1434) ~[forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {re:classloading,pl:accesstransformer:B,pl:runtimedistcleaner:A} at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:942) ~[forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {re:classloading,pl:accesstransformer:B,pl:runtimedistcleaner:A} at net.minecraft.client.Minecraft.run(Minecraft.java:559) ~[forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {re:classloading,pl:accesstransformer:B,pl:runtimedistcleaner:A} at net.minecraft.client.main.Main.main(Main.java:177) ~[forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {re:classloading,pl:runtimedistcleaner:A} at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_251] {} at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_251] {} at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_251] {} at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_251] {} at net.minecraftforge.userdev.FMLUserdevClientLaunchProvider.lambda$launchService$0(FMLUserdevClientLaunchProvider.java:55) ~[forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {} at cpw.mods.modlauncher.LaunchServiceHandlerDecorator.launch(LaunchServiceHandlerDecorator.java:37) [modlauncher-5.0.0-milestone.4.jar:?] {} at cpw.mods.modlauncher.LaunchServiceHandler.launch(LaunchServiceHandler.java:54) [modlauncher-5.0.0-milestone.4.jar:?] {} at cpw.mods.modlauncher.LaunchServiceHandler.launch(LaunchServiceHandler.java:72) [modlauncher-5.0.0-milestone.4.jar:?] {} at cpw.mods.modlauncher.Launcher.run(Launcher.java:81) [modlauncher-5.0.0-milestone.4.jar:?] {} at cpw.mods.modlauncher.Launcher.main(Launcher.java:65) [modlauncher-5.0.0-milestone.4.jar:?] {} at net.minecraftforge.userdev.LaunchTesting.main(LaunchTesting.java:102) [forge-1.15.2-31.1.0_mapped_snapshot_20200507-1.15.1-recomp.jar:?] {} -
[1.15.2] How to add block loots into a players inventory?
NindyBun replied to NindyBun's topic in Modder Support
So I practically took the LootContext#Builder from vanilla but I get a Null pointer exception from it @Override public ActionResultType onItemUse(ItemUseContext context) { refuel(context.getItem(), context.getPlayer()); int fuelValue = 0; int fortune = 0; int silkTouch = 0; if (context.getItem().hasTag()) { if (context.getItem().getTag().contains("FuelValue")) { fuelValue = context.getItem().getTag().getInt("FuelValue"); } if (context.getItem().getTag().contains("SilkTouch")) { silkTouch = context.getItem().getTag().getInt("SilkTouch"); }else if (context.getItem().getTag().contains("Fortune")) { fortune = context.getItem().getTag().getInt("Fortune"); } } World world = context.getWorld(); ItemStack stack = context.getItem(); BlockPos pos = context.getPos(); BlockState state = context.getWorld().getBlockState(pos); PlayerEntity player = context.getPlayer(); Block block = world.getBlockState(pos).getBlock(); stack.addEnchantment(Enchantments.FORTUNE, 3); if (fuelValue >= use){ //context.getWorld().destroyBlock(context.getPos(), true); if (block.removedByPlayer(state, world, pos, player, true, state.getFluidState())){ //BlockEvent.BreakEvent event = new BlockEvent.BreakEvent(world, pos, state, player); block.dropXpOnBlockBreak(world, pos, block.getExpDrop(state, world, pos, fortune, silkTouch)); block.harvestBlock(world, player, pos, state, world.getTileEntity(pos), stack); LootContext.Builder lootcontext = (new LootContext.Builder(world.getServer().getWorld(world.getDimension().getType()))).withRandom(world.rand).withParameter(LootParameters.POSITION, pos).withParameter(LootParameters.TOOL, stack).withNullableParameter(LootParameters.THIS_ENTITY, player).withNullableParameter(LootParameters.BLOCK_ENTITY, world.getTileEntity(pos)); LOGGER.info(state.getDrops(lootcontext)); //event.getWorld().destroyBlock(pos, false); //event.getWorld().playSound(context.getPlayer(), event.getPos(), event.getState().getSoundType().getBreakSound(), SoundCategory.BLOCKS, event.getState().getSoundType().getVolume(), event.getState().getSoundType().getPitch()); //context.getWorld().setBlockState(context.getPos(), Blocks.AIR.getDefaultState()); } fuelValue -= use; context.getItem().getTag().putInt("FuelValue", context.getItem().getTag().getInt("FuelValue")-use); } stack.getEnchantmentTagList().clear(); return ActionResultType.SUCCESS; } -
[1.15.2] How to add block loots into a players inventory?
NindyBun replied to NindyBun's topic in Modder Support
The Block#getDrop has a parameter of Builder, what would go into that?