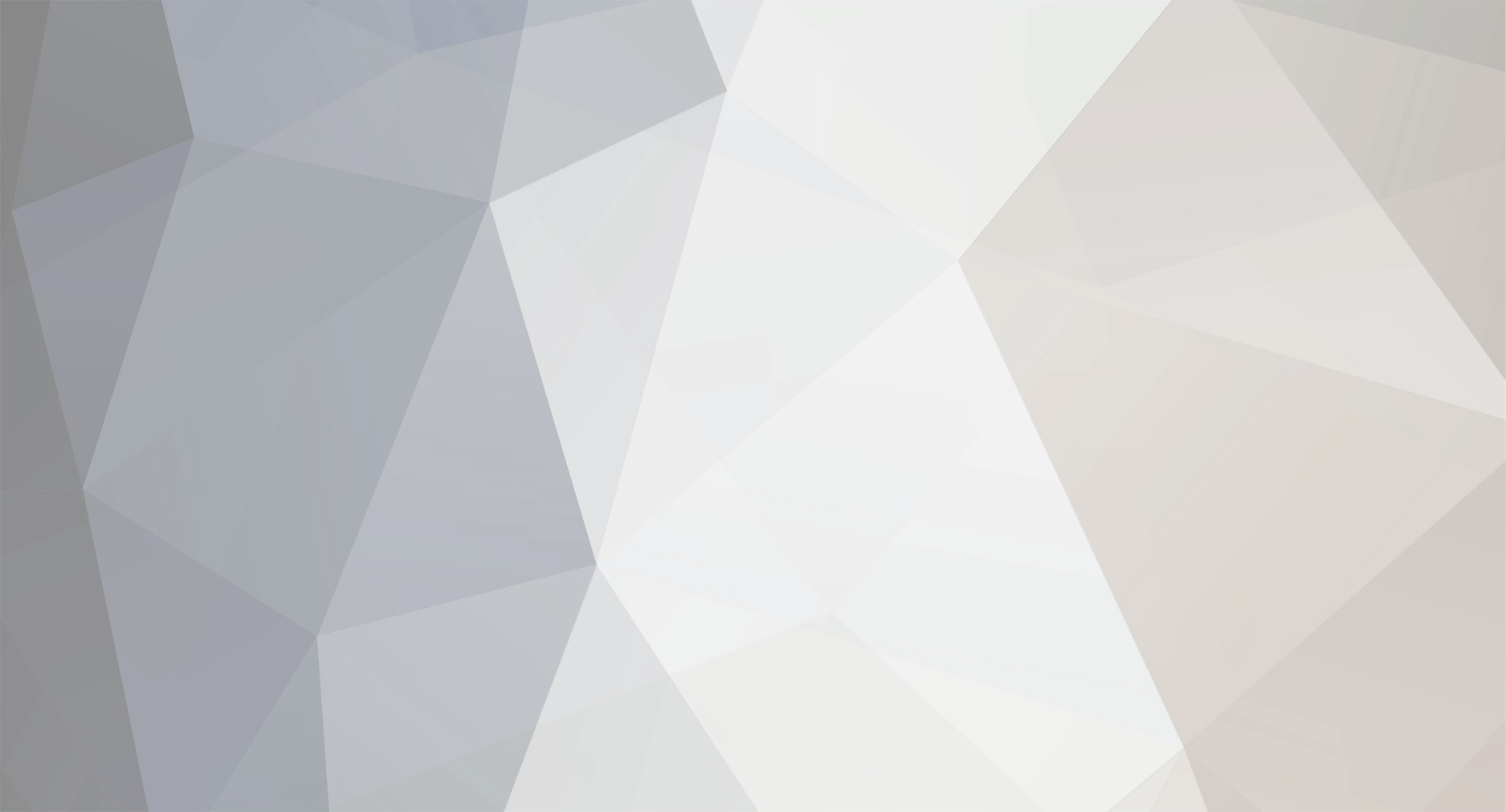
Razzokk
Members-
Posts
17 -
Joined
-
Last visited
Everything posted by Razzokk
-
Ok so I fixed it, thanks to you! Works flawlessly now.
-
Alright thanks for the help. I will try this later.
-
Alright I will do that. But why is that? I don't want to be annoying or anything, I just want to understand things. Is this because of different threads?
-
Ok got the thing with @OnlyIn. But the Packet Instance is created when opening the gui (I have to refactor this, I made this really crappy I just realized). @Override public ActionResultType onBlockActivated(BlockState state, World world, BlockPos pos, PlayerEntity player, Hand hand, BlockRayTraceResult hit) { if (world.isRemote) WorldUtils.ifTilePresent(world, pos, TileFrequency.class, tile -> WirelessRedstone.proxy.openFrequencyGui(tile.getFrequency(), new PacketFrequencyBlock(pos))); return ActionResultType.SUCCESS; }
-
Yep I noticed that, it should be formatted now.
-
public class PacketHandler { private static int id = 0; private static final String PROTOCOL_VERSION = "1.0"; public static final SimpleChannel INSTANCE = NetworkRegistry.newSimpleChannel( new ResourceLocation(WirelessRedstone.MODID, "main_channel"), () -> PROTOCOL_VERSION, PROTOCOL_VERSION::equals, PROTOCOL_VERSION::equals ); public static void registerMessages() { registerMessage(PacketFrequencyBlock.class, PacketFrequencyBlock::new); registerMessage(PacketFrequencyItem.class, PacketFrequencyItem::new); } public static <P extends Packet> void registerMessage(Class<P> packetType, Function<PacketBuffer, P> decoder) { INSTANCE.registerMessage(id++, packetType, Packet::toBytes, decoder, Packet::handle); } } public abstract class Packet { public Packet() {} public Packet(PacketBuffer buffer) {} public abstract void toBytes(PacketBuffer buffer); public abstract void handle(Supplier<NetworkEvent.Context> ctx); } public abstract class PacketFrequency extends Packet { private int frequency; public PacketFrequency(int frequency) { this.frequency = frequency; } public PacketFrequency(PacketBuffer buffer) { super(buffer); frequency = buffer.readInt(); } @Override public void toBytes(PacketBuffer buffer) { buffer.writeInt(frequency); } public void setFrequency(int frequency) { this.frequency = frequency; } public int getFrequency() { return frequency; } } public class PacketFrequencyBlock extends PacketFrequency { private final BlockPos pos; public PacketFrequencyBlock(BlockPos pos) { super(-1); this.pos = pos; } public PacketFrequencyBlock(PacketBuffer buffer) { super(buffer); pos = BlockPos.fromLong(buffer.readLong()); } @Override public void toBytes(PacketBuffer buffer) { super.toBytes(buffer); buffer.writeLong(pos.toLong()); } @Override public void handle(Supplier<NetworkEvent.Context> ctx) { ctx.get().enqueueWork(() -> { ServerPlayerEntity player = ctx.get().getSender(); ServerWorld world = player != null ? player.getServerWorld() : null; if (world != null && world.isAreaLoaded(pos, 0)) WorldUtils.ifTilePresent(world, pos, TileFrequency.class, tile -> tile.setFrequency(getFrequency())); }); ctx.get().setPacketHandled(true); } } @OnlyIn(Dist.CLIENT) public class GuiFrequency extends Screen { public static final ResourceLocation GUI_TEXTURE_NORMAL = new ResourceLocation(WirelessRedstone.MODID, "textures/gui/frequency.png"); public static final ResourceLocation GUI_TEXTURE_EXTENDED = new ResourceLocation(WirelessRedstone.MODID, "textures/gui/frequency_extended.png"); private ResourceLocation gui_texture = GUI_TEXTURE_NORMAL; private int guiLeft; private int guiTop; private int xSize; private int ySize; private int frequency; private PacketFrequency frequencyPacket; // Standard GUI private Button close; private TextFieldWidget frequencyField; private Button buttonSubtract_1; private Button buttonSubtract_10; private Button buttonAdd_1; private Button buttonAdd_10; private Button done; // Extended GUI private boolean extended; private Button buttonExtend; private TextFieldWidget frequencyName; private Button buttonAddName; private TextFieldWidget searchbar; public GuiFrequency(int frequency, PacketFrequency frequencyPacket) { super(new TranslationTextComponent(LangKeys.Gui.FREQUENCY)); this.frequency = frequency; this.frequencyPacket = frequencyPacket; } @Override protected void init() { xSize = 192; ySize = 96; guiLeft = (width - xSize) / 2; guiTop = (height - ySize) / 2 - 40; // Standard GUI addButton(close = new SizedButton(guiLeft + xSize - 18, guiTop + 6, 12, 12, new StringTextComponent("x"), 0, -1, button -> minecraft.player.closeScreen())); addButton(buttonSubtract_1 = new SizedButton(guiLeft + 28, guiTop + 24, 36, 16, new StringTextComponent("-1"), this::buttonPressed)); addButton(buttonSubtract_10 = new SizedButton(guiLeft + 28, guiTop + 44, 36, 16, new StringTextComponent("-10"), this::buttonPressed)); addButton(buttonAdd_1 = new SizedButton(guiLeft + 128, guiTop + 24, 36, 16, new StringTextComponent("+1"), this::buttonPressed)); addButton(buttonAdd_10 = new SizedButton(guiLeft + 128, guiTop + 44, 36, 16, new StringTextComponent("+10"), this::buttonPressed)); addButton(done = new SizedButton(guiLeft + 78, guiTop + 64, 36, 18, new TranslationTextComponent(LangKeys.Gui.DONE), onPress -> sendPacket())); frequencyField = new TextFieldWidget(font, guiLeft + 76, guiTop + 35, 38, 14, new TranslationTextComponent(LangKeys.Gui.FREQUENCY)) { @Override public void writeText(String textToWrite) { StringBuilder stringbuilder = new StringBuilder(); for (char c0 : textToWrite.toCharArray()) if (c0 >= 48 && c0 <= 57) stringbuilder.append(c0); super.writeText(stringbuilder.toString()); } }; frequencyField.setMaxStringLength(5); frequencyField.setText(String.valueOf(frequency)); frequencyField.setResponder(text -> { if (text == null || text.isEmpty()) { done.active = false; setFrequency(0); } else { done.active = true; setFrequency(Integer.parseInt(text)); } }); children.add(frequencyField); // Extended GUI addButton(buttonExtend = new SizedButton(guiLeft + xSize - 48, guiTop + ySize - 22, 42, 16, new TranslationTextComponent(LangKeys.Gui.EXTEND), this::extend)); frequencyName = new TextFieldWidget(font, guiLeft + 7, guiTop + 100, 144, 14, new TranslationTextComponent(LangKeys.Gui.FREQUENCY_NAME)); addButton(buttonAddName = new SizedButton(guiLeft + 154, guiTop + 99, 32, 16, new TranslationTextComponent(LangKeys.Gui.ADD), onPress -> System.out.println("add to list"))); buttonAddName.visible = extended; searchbar = new TextFieldWidget(font, guiLeft + 7, guiTop + 130, 178, 14, new TranslationTextComponent(LangKeys.Gui.SEARCHBAR)); children.add(frequencyName); children.add(searchbar); } private void setFrequency(int frequency) { this.frequency = MathUtils.constrain(frequency, 0, 99999); } private void buttonPressed(Button button) { setFrequency(frequency + Integer.parseInt(button.getMessage().getString())); frequencyField.setText(String.valueOf(frequency)); } private void extend(Button button) { extended = !extended; if (extended) { ySize = 176; buttonExtend.setMessage(new TranslationTextComponent(LangKeys.Gui.REDUCE)); gui_texture = GUI_TEXTURE_EXTENDED; } else { ySize = 96; buttonExtend.setMessage(new TranslationTextComponent(LangKeys.Gui.EXTEND)); gui_texture = GUI_TEXTURE_NORMAL; } buttonAddName.visible = extended; } @Override public boolean keyPressed(int keyCode, int scanCode, int modifiers) { switch (keyCode) { case GLFW.GLFW_KEY_LEFT_SHIFT: case GLFW.GLFW_KEY_RIGHT_SHIFT: buttonSubtract_1.setMessage(new StringTextComponent("-100")); buttonSubtract_10.setMessage(new StringTextComponent("-1000")); buttonAdd_1.setMessage(new StringTextComponent("+100")); buttonAdd_10.setMessage(new StringTextComponent("+1000")); break; } return frequencyField.keyPressed(keyCode, scanCode, modifiers) || frequencyField.canWrite() || super.keyPressed(keyCode, scanCode, modifiers); } @Override public boolean keyReleased(int keyCode, int scanCode, int modifiers) { switch (keyCode) { case GLFW.GLFW_KEY_LEFT_SHIFT: case GLFW.GLFW_KEY_RIGHT_SHIFT: buttonSubtract_1.setMessage(new StringTextComponent("-1")); buttonSubtract_10.setMessage(new StringTextComponent("-10")); buttonAdd_1.setMessage(new StringTextComponent("+1")); buttonAdd_10.setMessage(new StringTextComponent("+10")); break; } return super.keyReleased(keyCode, scanCode, modifiers); } @Override public void render(MatrixStack matrixStack, int mouseX, int mouseY, float partialTicks) { renderBackground(matrixStack); drawGuiBackgroundTexture(matrixStack, mouseX, mouseY, partialTicks); font.drawString(matrixStack, title.getString(), guiLeft + (xSize - font.getStringWidth(title.getString())) / 2, guiTop + 7, 0x404040); frequencyField.render(matrixStack, mouseX, mouseY, partialTicks); if (extended) { font.drawString(matrixStack, new TranslationTextComponent(LangKeys.Gui.FREQUENCY_NAME).getString(), guiLeft + 6, guiTop + 80, 0x404040); frequencyName.render(matrixStack, mouseX, mouseY, partialTicks); searchbar.render(matrixStack, mouseX, mouseY, partialTicks); } super.render(matrixStack, mouseX, mouseY, partialTicks); } private void drawGuiBackgroundTexture(MatrixStack matrixStack, int mouseX, int mouseY, float partialTicks) { RenderSystem.color4f(1.0F, 1.0F, 1.0F, 1.0F); minecraft.getTextureManager().bindTexture(gui_texture); blit(matrixStack, guiLeft, guiTop, 0, 0, xSize, ySize); } private void sendPacket() { frequencyPacket.setFrequency(frequency); PacketHandler.INSTANCE.sendToServer(frequencyPacket); minecraft.player.closeScreen(); } @Override public boolean isPauseScreen() { return false; } }
-
Exception thrown: [Server thread/FATAL] [minecraft/ThreadTaskExecutor]: Error executing task on Server java.lang.ArrayIndexOutOfBoundsException: null I get this exception when playing on the server. I open a client-side only gui and when pressing a button I am sending a packet that sets an integer in the blocks TileEntity but because of this error, the integer is not actually set. Any ideas what that could be? I do not get any errors on the client. It works correctly when playing on singleplayer. debug.log: https://pastebin.pl/view/6c5c281a
-
To any moderator that reads this, please close this. I accidentally posted this in the wrong channel (I re-post this in Modder Support). Sry for that.
-
That is the problem. I do not get any additional information out of the logs. It just tells me about the ArraysIndexOutOfBoundsException: null. How is it even possible to get null in a ArraysIndexOutOfBoundsException?
-
Exception thrown: [Server thread/FATAL] [minecraft/ThreadTaskExecutor]: Error executing task on Server java.lang.ArrayIndexOutOfBoundsException: null I get this exception when playing on the server. I open a client-side only gui and when pressing a button I am sending a packet that sets an integer in the blocks TileEntity but because of this error, the integer is not actually set. Any ideas what that could be? I do not get any errors on the client. It works correctly when playing on singleplayer.
-
Ahh, that's a bummer.
-
Is it possible to detect when an ItemStack is getting destroyed or changed? For example: I want to detect if my Item is getting deleted when I am in the creative inventory and I drag the Item somewhere into a creative tab so that it will get destroyed.
-
[1.15.2] Block Rendering based on TileEntity Inventory’s ItemStack
Razzokk replied to Razzokk's topic in Modder Support
Thanks for the advice, I'll take a look at those example, and then gonna try it out. -
[1.15.2] Block Rendering based on TileEntity Inventory’s ItemStack
Razzokk replied to Razzokk's topic in Modder Support
Hmm maybe I‘ve just written it in a not understandable way ^^. Anyways thanks for the suggestion, I‘ll take a look at it. -
[1.15.2] Block Rendering based on TileEntity Inventory’s ItemStack
Razzokk replied to Razzokk's topic in Modder Support
Thank you, yeah that is why I am asking for 1.15 now cause I would like to know an opinion/how others would approach this problem. -
Sorry for posting this as outdated 1.12.2 content but since I’m going to update sooner or later to 1.15.2 this is still relevant. I have a block with an inventory that consists of 6 slots, each slot for a face of the block. Now depending on what ItemStack is in a Slot I want to render an overlay on the corresponding face of the block. What would be the best approach to accomplish this? Thanks in advance.
-
I have a block with an inventory that consists of 6 slots, each slot for a face of the block. Now depending on what ItemStack is in a Slot I want to render an overlay on the corresponding face of the block. What would be the best approach to accomplish this? Thanks in advance.