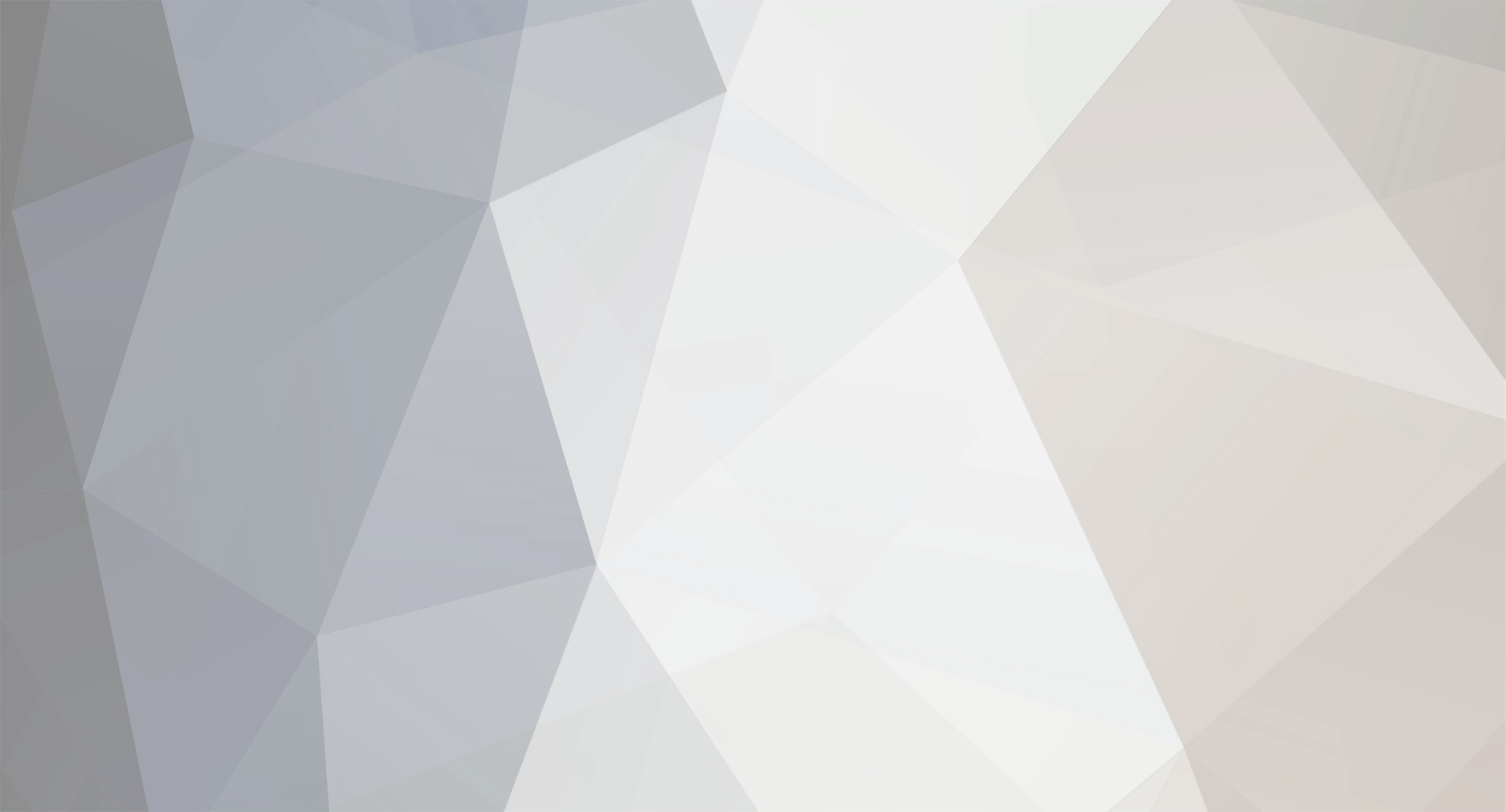
vandy22
-
Posts
224 -
Joined
-
Last visited
Posts posted by vandy22
-
-
So yeah.....
-
Ok I will update. Hopefully nothing modding wise had changed though! And here is my main mod file
Main mod file(mod_tutorial):
package tutorialtest; import tutorial.client.ClientProxy.ClientProxy; import tutorial.common.CommonProxy.CommonProxy; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.Init; import cpw.mods.fml.common.SidedProxy; import cpw.mods.fml.common.event.FMLInitializationEvent; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.item.EnumArmorMaterial; import net.minecraft.item.EnumToolMaterial; import net.minecraft.item.Item; import net.minecraft.item.ItemSword; import cpw.mods.fml.common.network.NetworkMod; import cpw.mods.fml.common.registry.GameRegistry; import net.minecraft.item.ItemStack; import cpw.mods.fml.common.registry.LanguageRegistry; import net.minecraft.src.ModLoader; import net.minecraftforge.client.MinecraftForgeClient; @Mod(modid = "JV's ToolPack", name = "JV's ToolPack", version = "1.4.7") @NetworkMod(clientSideRequired = true, serverSideRequired = false) public class mod_tutorial { //CreativeTabs public static CreativeTabs tabJVCraft = new CreativeTabJVCraft(CreativeTabs.getNextID(), "tabJVCraft"); //Furnace public static Block basicFurnace; public static Block BasicCube; public static final int basicFurnaceGUIid = 0; //blue public static Item TutorialHelmet; public static Item TutorialPlate; public static Item TutorialLegs; public static Item TutorialBoots; public static Item BlueIngot; public static Item BlueDust; public static Item BlueToolFragment; public static Item BlueSword; public static Item BluePickaxe; public static Item BlueSpade; public static Item BlueAxe; public static Block BlueOre; //yellow public static Item YellowHelmet; public static Item YellowPlate; public static Item YellowLegs; public static Item YellowBoots; public static Item YellowToolFragment; public static Item YellowIngot; public static Item YellowDust; public static Item YellowSword; public static Item YellowPickaxe; public static Item YellowSpade; public static Item YellowAxe; public static Block YellowOre; //White/Silver public static Item WhiteHelmet; public static Item WhitePlate; public static Item WhiteLegs; public static Item WhiteBoots; public static Item WhiteIngot; public static Item SilverIngot; public static Item WhitePowder; public static Item SilverDust; public static Block SilverOre; public static Item WhiteSword; public static Item WhitePickaxe; public static Item WhiteSpade; public static Item WhiteAxe; public static Item WhiteToolFragment; //red public static Item RedHelmet; public static Item RedPlate; public static Item RedLegs; public static Item RedBoots; public static Item RedIngot; public static Item RedToolFragment; public static Item RedDust; public static Item RedSword; public static Item RedPickaxe; public static Item RedSpade; public static Item RedAxe; public static Block RedOre; //green public static Item GreenHelmet; public static Item GreenPlate; public static Item GreenLegs; public static Item GreenBoots; public static Item GreenIngot; public static Item GreenToolFragment; public static Item GreenDust; public static Item GreenSword; public static Item GreenPickaxe; public static Item GreenSpade; public static Item GreenAxe; public static Block GreenOre; //Pink public static Item PinkHelmet; public static Item PinkPlate; public static Item PinkLegs; public static Item PinkBoots; public static Item PinkIngot; public static Item PinkToolFragment; public static Item PinkDust; public static Item PinkSword; public static Item PinkPickaxe; public static Item PinkSpade; public static Item PinkAxe; public static Block PinkOre; //Purple public static Item PurpleHelmet; public static Item PurplePlate; public static Item PurpleLegs; public static Item PurpleBoots; public static Item PurpleIngot; public static Item PurpleToolFragment; public static Item PurpleDust; public static Item PurpleSword; public static Item PurplePickaxe; public static Item PurpleSpade; public static Item PurpleAxe; public static Block PurpleOre; //Black public static Item BlackHelmet; public static Item BlackPlate; public static Item BlackLegs; public static Item BlackBoots; public static Item BlackIngot; public static Item BlackToolFragment; public static Item BlackDust; public static Item BlackSword; public static Item BlackPickaxe; public static Item BlackSpade; public static Item BlackAxe; public static Block BlackOre; //Rainbow public static Item RainbowHelmet; public static Item RainbowPlate; public static Item RainbowLegs; public static Item RainbowBoots; public static Item RainbowToolFragment; public static Item RainbowIngot; public static Item RainbowSword; public static Item RainbowPickaxe; public static Item RainbowSpade; public static Item RainbowAxe; public static Item RainbowHoe; public static Item RainbowDust; //Enum Materials static EnumArmorMaterial TutorialEnumArmourMaterial = net.minecraftforge.common.EnumHelper.addArmorMaterial("tutorial", 24, new int[] { 2, 5, 3, 2 }, 16); @SidedProxy(clientSide = "tutorial.client.ClientProxy.ClientProxy", serverSide = "tutorial.common.CommonProxy.CommonProxy") public static CommonProxy proxy; @Init public void load(FMLInitializationEvent event){ MinecraftForgeClient.preloadTexture(ClientProxy.BLOCKS_PNG); MinecraftForgeClient.preloadTexture(ClientProxy.ITEMS_PNG); MinecraftForgeClient.preloadTexture(ClientProxy.FURNACE_PNG); //Creative Tabs //Furnaces basicFurnace = new ModCustomBasicFurnace(1000, 1, false).setBlockName("BF"); BasicCube = new YellowOre(1001, .setHardness(3.0F).setResistance(3.0F).setBlockName("BasicCube").setCreativeTab(tabJVCraft); //Blue //Armor TutorialHelmet = new ItemTutorialHelmet(508,EnumArmorMaterial.BLUE, proxy.addArmor("Tut"),0).setItemName("TutHelm").setIconIndex(0).setCreativeTab(tabJVCraft); TutorialPlate = new ItemTutorialPlate(509,EnumArmorMaterial.BLUE, proxy.addArmor("Tut"),1).setItemName("TutPlate").setIconIndex(1).setCreativeTab(tabJVCraft); TutorialLegs = new ItemTutorialLegs(510,EnumArmorMaterial.BLUE, proxy.addArmor("Tut"),2).setItemName("TutLeg").setIconIndex(2).setCreativeTab(tabJVCraft); TutorialBoots = new ItemTutorialBoots(511,EnumArmorMaterial.BLUE, proxy.addArmor("Tut"),3).setItemName("TutBoot").setIconIndex(3).setCreativeTab(tabJVCraft); //Items BlueIngot = new YellowIngot(532).setItemName("BlueIngot").setIconIndex(24).setCreativeTab(tabJVCraft); BlueToolFragment = new YellowToolFragment(533).setItemName("BlueToolFragment").setIconIndex(25).setCreativeTab(tabJVCraft); BlueSword = new YellowSword(534, EnumToolMaterial.BLUE).setItemName("BlueSword").setIconIndex(26).setCreativeTab(tabJVCraft); BluePickaxe = new YellowPickaxe(535, EnumToolMaterial.BLUE).setItemName("BluePickaxe").setIconIndex(27).setCreativeTab(tabJVCraft); BlueSpade = new YellowSpade(536, EnumToolMaterial.BLUE).setItemName("BlueSpade").setIconIndex(28).setCreativeTab(tabJVCraft); BlueAxe = new YellowAxe(537, EnumToolMaterial.BLUE).setItemName("BlueAxe").setIconIndex(29).setCreativeTab(tabJVCraft); BlueDust = new YellowDust(538).setItemName("BlueDust").setIconIndex(30).setCreativeTab(tabJVCraft); BlueOre = new YellowOre(539, 0).setHardness(3.0F).setResistance(3.0F).setBlockName("BlueOre").setCreativeTab(tabJVCraft); //Yellow YellowHelmet = new ItemYellowHelmet(512,EnumArmorMaterial.YELLOW, proxy.addArmor("Yellow"),0).setItemName("YellowHelm").setIconIndex(4).setCreativeTab(tabJVCraft); YellowPlate = new ItemYellowPlate(513,EnumArmorMaterial.YELLOW, proxy.addArmor("Yellow"),1).setItemName("YellowPlate").setIconIndex(5).setCreativeTab(tabJVCraft); YellowLegs = new ItemYellowLegs(514,EnumArmorMaterial.YELLOW, proxy.addArmor("Yellow"),2).setItemName("YellowLeg").setIconIndex(6).setCreativeTab(tabJVCraft); YellowBoots = new ItemYellowBoots(515,EnumArmorMaterial.YELLOW, proxy.addArmor("Yellow"),3).setItemName("YellowBoot").setIconIndex(7).setCreativeTab(tabJVCraft); YellowIngot = new YellowIngot(540).setItemName("YellowIngot").setIconIndex(31).setCreativeTab(tabJVCraft); YellowToolFragment = new YellowToolFragment(541).setItemName("YellowToolFragment").setIconIndex(32).setCreativeTab(tabJVCraft); YellowSword = new YellowSword(542, EnumToolMaterial.YELLOW).setItemName("YellowSword").setIconIndex(33).setCreativeTab(tabJVCraft); YellowPickaxe = new YellowPickaxe(543, EnumToolMaterial.YELLOW).setItemName("YellowPickaxe").setIconIndex(34).setCreativeTab(tabJVCraft); YellowSpade = new YellowSpade(544, EnumToolMaterial.YELLOW).setItemName("YellowSpade").setIconIndex(35).setCreativeTab(tabJVCraft); YellowAxe = new YellowAxe(545, EnumToolMaterial.YELLOW).setItemName("YellowAxe").setIconIndex(36).setCreativeTab(tabJVCraft); YellowDust = new YellowDust(546).setItemName("YellowDust").setIconIndex(37).setCreativeTab(tabJVCraft); YellowOre = new YellowOre(547, 1).setHardness(3.0F).setResistance(3.0F).setBlockName("YellowOre").setCreativeTab(tabJVCraft); //White WhiteHelmet = new ItemWhiteHelmet(516, EnumArmorMaterial.DIAMOND, proxy.addArmor("White"),0).setItemName("WhiteHelm").setIconIndex(.setCreativeTab(tabJVCraft); WhitePlate = new ItemWhitePlate(517,EnumArmorMaterial.DIAMOND, proxy.addArmor("White"),1).setItemName("WhitePlate").setIconIndex(9).setCreativeTab(tabJVCraft); WhiteLegs = new ItemWhiteLegs(518,EnumArmorMaterial.DIAMOND, proxy.addArmor("White"),2).setItemName("WhiteLeg").setIconIndex(10).setCreativeTab(tabJVCraft); WhiteBoots = new ItemWhiteBoots(519,EnumArmorMaterial.DIAMOND, proxy.addArmor("White"),3).setItemName("WhiteBoot").setIconIndex(11).setCreativeTab(tabJVCraft); WhiteIngot = new YellowIngot(548).setItemName("WhiteIngot").setIconIndex(38).setCreativeTab(tabJVCraft); WhiteToolFragment = new YellowToolFragment(555).setItemName("WhiteToolFragment").setIconIndex(39).setCreativeTab(tabJVCraft); WhiteSword = new YellowSword(549, EnumToolMaterial.EMERALD).setItemName("WhiteSword").setIconIndex(40).setCreativeTab(tabJVCraft); WhitePickaxe = new YellowPickaxe(556, EnumToolMaterial.EMERALD).setItemName("WhitePickaxe").setIconIndex(41).setCreativeTab(tabJVCraft); WhiteSpade = new YellowSpade(550, EnumToolMaterial.EMERALD).setItemName("WhiteSpade").setIconIndex(42).setCreativeTab(tabJVCraft); WhiteAxe = new YellowAxe(551, EnumToolMaterial.EMERALD).setItemName("WhiteAxe").setIconIndex(43).setCreativeTab(tabJVCraft); SilverDust = new YellowDust(552).setItemName("SilverDust").setIconIndex(44).setCreativeTab(tabJVCraft); SilverOre = new YellowOre(553, 2).setHardness(3.0F).setResistance(3.0F).setBlockName("WhiteOre").setCreativeTab(tabJVCraft); WhitePowder = new YellowDust(554).setItemName("WhitePowder").setIconIndex(45).setCreativeTab(tabJVCraft); SilverIngot = new YellowIngot(565).setItemName("SilverIngot").setIconIndex(53).setCreativeTab(tabJVCraft); //Blue RedHelmet = new ItemRedHelmet(520, EnumArmorMaterial.RED, proxy.addArmor("Red"),0).setItemName("RedHelm").setIconIndex(12).setCreativeTab(tabJVCraft); RedPlate = new ItemRedPlate(521,EnumArmorMaterial.RED, proxy.addArmor("Red"),1).setItemName("RedPlate").setIconIndex(13).setCreativeTab(tabJVCraft); RedLegs = new ItemRedLegs(522,EnumArmorMaterial.RED, proxy.addArmor("Red"),2).setItemName("RedLeg").setIconIndex(14).setCreativeTab(tabJVCraft); RedBoots = new ItemRedBoots(523,EnumArmorMaterial.RED, proxy.addArmor("Red"),3).setItemName("RedBoot").setIconIndex(15).setCreativeTab(tabJVCraft); RedIngot = new YellowIngot(564).setItemName("RedIngot").setIconIndex(46); RedToolFragment = new YellowToolFragment(557).setItemName("RedToolFragment").setIconIndex(47).setCreativeTab(tabJVCraft); RedSword = new YellowSword(558, EnumToolMaterial.RED).setItemName("RedSword").setIconIndex(48).setCreativeTab(tabJVCraft); RedPickaxe = new YellowPickaxe(559, EnumToolMaterial.RED).setItemName("RedPickaxe").setIconIndex(49).setCreativeTab(tabJVCraft); RedSpade = new YellowSpade(560, EnumToolMaterial.RED).setItemName("RedSpade").setIconIndex(50).setCreativeTab(tabJVCraft); RedAxe = new YellowAxe(561, EnumToolMaterial.RED).setItemName("RedAxe").setIconIndex(51).setCreativeTab(tabJVCraft); RedDust = new YellowDust(562).setItemName("RedDust").setIconIndex(52).setCreativeTab(tabJVCraft); RedOre = new YellowOre(563, 3).setHardness(3.0F).setResistance(3.0F).setBlockName("RedOre").setCreativeTab(tabJVCraft); //Green GreenHelmet = new ItemGreenHelmet(524, EnumArmorMaterial.GREEN, proxy.addArmor("Green"),0).setItemName("GreenHelm").setIconIndex(16).setCreativeTab(tabJVCraft); GreenPlate = new ItemGreenPlate(525,EnumArmorMaterial.GREEN, proxy.addArmor("Green"),1).setItemName("GreenPlate").setIconIndex(17).setCreativeTab(tabJVCraft); GreenLegs = new ItemGreenLegs(526,EnumArmorMaterial.GREEN, proxy.addArmor("Green"),2).setItemName("GreenLeg").setIconIndex(18).setCreativeTab(tabJVCraft); GreenBoots = new ItemGreenBoots(527,EnumArmorMaterial.GREEN, proxy.addArmor("Green"),3).setItemName("GreenBoot").setIconIndex(19).setCreativeTab(tabJVCraft); GreenIngot = new YellowIngot(566).setItemName("GreenIngot").setIconIndex(54).setCreativeTab(tabJVCraft); GreenToolFragment = new YellowToolFragment(567).setItemName("GreenToolFragment").setIconIndex(55).setCreativeTab(tabJVCraft); GreenSword = new YellowSword(568, EnumToolMaterial.GREEN).setItemName("GreenSword").setIconIndex(56).setCreativeTab(tabJVCraft); GreenPickaxe = new YellowPickaxe(569, EnumToolMaterial.GREEN).setItemName("GreenPickaxe").setIconIndex(57).setCreativeTab(tabJVCraft); GreenSpade = new YellowSpade(570, EnumToolMaterial.GREEN).setItemName("GreenSpade").setIconIndex(58).setCreativeTab(tabJVCraft); GreenAxe = new YellowAxe(571, EnumToolMaterial.GREEN).setItemName("GreenAxe").setIconIndex(59).setCreativeTab(tabJVCraft); GreenDust = new YellowDust(572).setItemName("GreenDust").setIconIndex(60).setCreativeTab(tabJVCraft); GreenOre = new YellowOre(573, 4).setHardness(3.0F).setResistance(3.0F).setBlockName("GreenOre").setCreativeTab(tabJVCraft); //Pink PinkHelmet = new ItemPinkHelmet(528, EnumArmorMaterial.PINK, proxy.addArmor("Pink"),0).setItemName("PinkHelm").setIconIndex(20).setCreativeTab(tabJVCraft); PinkPlate = new ItemPinkPlate(529,EnumArmorMaterial.PINK, proxy.addArmor("Pink"),1).setItemName("PinkPlate").setIconIndex(21).setCreativeTab(tabJVCraft); PinkLegs = new ItemPinkLegs(530,EnumArmorMaterial.PINK, proxy.addArmor("Pink"),2).setItemName("PinkLeg").setIconIndex(22).setCreativeTab(tabJVCraft); PinkBoots = new ItemPinkBoots(531,EnumArmorMaterial.PINK, proxy.addArmor("Pink"),3).setItemName("PinkBoot").setIconIndex(23).setCreativeTab(tabJVCraft); PinkIngot = new YellowIngot(574).setItemName("PinkIngot").setIconIndex(61).setCreativeTab(tabJVCraft); PinkToolFragment = new YellowToolFragment(575).setItemName("PinkToolFragment").setIconIndex(62).setCreativeTab(tabJVCraft); PinkSword = new YellowSword(576, EnumToolMaterial.PINK).setItemName("PinkSword").setIconIndex(63).setCreativeTab(tabJVCraft); PinkPickaxe = new YellowPickaxe(577, EnumToolMaterial.PINK).setItemName("PinkPickaxe").setIconIndex(64).setCreativeTab(tabJVCraft); PinkSpade = new YellowSpade(578, EnumToolMaterial.PINK).setItemName("PinkSpade").setIconIndex(65).setCreativeTab(tabJVCraft); PinkAxe = new YellowAxe(579, EnumToolMaterial.PINK).setItemName("PinkAxe").setIconIndex(66).setCreativeTab(tabJVCraft); PinkDust = new YellowDust(580).setItemName("PinkDust").setIconIndex(67).setCreativeTab(tabJVCraft); PinkOre = new YellowOre(581, 5).setHardness(3.0F).setResistance(3.0F).setBlockName("PinkOre").setCreativeTab(tabJVCraft); //Purple PurpleHelmet = new ItemPurpleHelmet(590, EnumArmorMaterial.PURPLE, proxy.addArmor("Purple"),0).setItemName("PurpleHelm").setIconIndex(75).setCreativeTab(tabJVCraft); PurplePlate = new ItemPurplePlate(591,EnumArmorMaterial.PURPLE, proxy.addArmor("Purple"),1).setItemName("PurplePlate").setIconIndex(76).setCreativeTab(tabJVCraft); PurpleLegs = new ItemPurpleLegs(592,EnumArmorMaterial.PURPLE, proxy.addArmor("Purple"),2).setItemName("PurpleLeg").setIconIndex(77).setCreativeTab(tabJVCraft); PurpleBoots = new ItemPurpleBoots(593,EnumArmorMaterial.PURPLE, proxy.addArmor("Purple"),3).setItemName("PurpleBoot").setIconIndex(78).setCreativeTab(tabJVCraft); PurpleIngot = new YellowIngot(582).setItemName("PurpleIngot").setIconIndex(68).setCreativeTab(tabJVCraft); PurpleToolFragment = new YellowToolFragment(583).setItemName("PurpleToolFragment").setIconIndex(69).setCreativeTab(tabJVCraft); PurpleSword = new YellowSword(584, EnumToolMaterial.PURPLE).setItemName("PurpleSword").setIconIndex(70).setCreativeTab(tabJVCraft); PurplePickaxe = new YellowPickaxe(585, EnumToolMaterial.PURPLE).setItemName("PurplePickaxe").setIconIndex(71).setCreativeTab(tabJVCraft); PurpleSpade = new YellowSpade(586, EnumToolMaterial.PURPLE).setItemName("PurpleSpade").setIconIndex(72).setCreativeTab(tabJVCraft); PurpleAxe = new YellowAxe(587, EnumToolMaterial.PURPLE).setItemName("PurpleAxe").setIconIndex(73).setCreativeTab(tabJVCraft); PurpleDust = new YellowDust(588).setItemName("PurpleDust").setIconIndex(74).setCreativeTab(tabJVCraft); PurpleOre = new YellowOre(589, 6).setHardness(3.0F).setResistance(3.0F).setBlockName("PurpleOre").setCreativeTab(tabJVCraft); //Black BlackHelmet = new ItemBlackHelmet(602, EnumArmorMaterial.BLACK, proxy.addArmor("Black"),0).setItemName("BlackHelm").setIconIndex(79).setCreativeTab(tabJVCraft); BlackPlate = new ItemBlackPlate(603,EnumArmorMaterial.BLACK, proxy.addArmor("Black"),1).setItemName("BlackPlate").setIconIndex(80).setCreativeTab(tabJVCraft); BlackLegs = new ItemBlackLegs(604,EnumArmorMaterial.BLACK, proxy.addArmor("Black"),2).setItemName("BlackLeg").setIconIndex(81).setCreativeTab(tabJVCraft); BlackBoots = new ItemBlackBoots(605,EnumArmorMaterial.BLACK, proxy.addArmor("Black"),3).setItemName("BlackBoot").setIconIndex(82).setCreativeTab(tabJVCraft); BlackIngot = new YellowIngot(594).setItemName("BlackIngot").setIconIndex(83).setCreativeTab(tabJVCraft); BlackToolFragment = new YellowToolFragment(595).setItemName("BlackToolFragment").setIconIndex(84).setCreativeTab(tabJVCraft); BlackSword = new YellowSword(596, EnumToolMaterial.BLACK).setItemName("BlackSword").setIconIndex(85).setCreativeTab(tabJVCraft); BlackPickaxe = new YellowPickaxe(597, EnumToolMaterial.BLACK).setItemName("BlackPickaxe").setIconIndex(86).setCreativeTab(tabJVCraft); BlackSpade = new YellowSpade(598, EnumToolMaterial.BLACK).setItemName("BlackSpade").setIconIndex(87).setCreativeTab(tabJVCraft); BlackAxe = new YellowAxe(599, EnumToolMaterial.BLACK).setItemName("BlackAxe").setIconIndex(88).setCreativeTab(tabJVCraft); BlackDust = new YellowDust(600).setItemName("BlackDust").setIconIndex(89).setCreativeTab(tabJVCraft); BlackOre = new YellowOre(601, 7).setHardness(3.0F).setResistance(3.0F).setBlockName("BlackOre").setCreativeTab(tabJVCraft); //Rainbow RainbowHelmet = new ItemRainbowHelmet(616, EnumArmorMaterial.RAINBOW, proxy.addArmor("Rainbow"),0).setItemName("RainbowHelm").setIconIndex(97).setCreativeTab(tabJVCraft); RainbowPlate = new ItemRainbowPlate(606,EnumArmorMaterial.RAINBOW, proxy.addArmor("Rainbow"),1).setItemName("RainbowPlate").setIconIndex(98).setCreativeTab(tabJVCraft); RainbowLegs = new ItemRainbowLegs(607,EnumArmorMaterial.RAINBOW, proxy.addArmor("Rainbow"),2).setItemName("RainbowLeg").setIconIndex(99).setCreativeTab(tabJVCraft); RainbowBoots = new ItemRainbowBoots(608,EnumArmorMaterial.RAINBOW, proxy.addArmor("Rainbow"),3).setItemName("RainbowBoot").setIconIndex(100).setCreativeTab(tabJVCraft); RainbowIngot = new YellowIngot(609).setItemName("RainbowIngot").setIconIndex(90).setCreativeTab(tabJVCraft); RainbowToolFragment = new YellowToolFragment(610).setItemName("RainbowToolFragment").setIconIndex(91).setCreativeTab(tabJVCraft); RainbowSword = new YellowSword(611, EnumToolMaterial.RAINBOW).setItemName("RainbowSword").setIconIndex(92).setCreativeTab(tabJVCraft); RainbowPickaxe = new YellowPickaxe(612, EnumToolMaterial.RAINBOW).setItemName("RainbowPickaxe").setIconIndex(93).setCreativeTab(tabJVCraft); RainbowSpade = new YellowSpade(613, EnumToolMaterial.RAINBOW).setItemName("RainbowSpade").setIconIndex(94).setCreativeTab(tabJVCraft); RainbowHoe = new RainbowHoe(617, EnumToolMaterial.RAINBOW).setItemName("RainbowHoe").setIconIndex(101).setCreativeTab(tabJVCraft); RainbowAxe = new YellowAxe(614, EnumToolMaterial.RAINBOW).setItemName("RainbowAxe").setIconIndex(95).setCreativeTab(tabJVCraft); RainbowDust = new YellowDust(615).setItemName("RainbowDust").setIconIndex(96).setCreativeTab(tabJVCraft); //Register Blocks GameRegistry.registerBlock(BlueOre, "BlueOre"); GameRegistry.registerBlock(YellowOre, "YellowOre"); GameRegistry.registerBlock(SilverOre, "SilverOre"); GameRegistry.registerBlock(RedOre, "RedOre"); GameRegistry.registerBlock(GreenOre, "GreenOre"); GameRegistry.registerBlock(PinkOre, "PinkOre"); GameRegistry.registerBlock(basicFurnace, "basicFurnace"); GameRegistry.registerBlock(PurpleOre, "PurpleOre"); GameRegistry.registerBlock(BlackOre, "BlackOre"); GameRegistry.registerBlock(BasicCube, "BasicCube"); //Smelting Ore ModLoader.addSmelting(YellowOre.blockID, new ItemStack(mod_tutorial.YellowIngot, 1)); //(YellowOre.blockID, new ItemStack(mod_tutorial.YellowIngot, 1), 0.35); ModLoader.addSmelting(mod_tutorial.YellowIngot.itemID, new ItemStack(mod_tutorial.YellowDust, 2)); ModLoader.addSmelting(mod_tutorial.SilverIngot.itemID, new ItemStack(mod_tutorial.SilverDust, 1)); ModLoader.addSmelting(SilverOre.blockID, new ItemStack(SilverIngot, 1)); ModLoader.addSmelting(mod_tutorial.WhitePowder.itemID, new ItemStack(WhiteIngot, 1)); ModLoader.addSmelting(BlueOre.blockID, new ItemStack(BlueIngot, 1)); ModLoader.addSmelting(mod_tutorial.BlueIngot.itemID, new ItemStack(mod_tutorial.BlueDust, 1)); ModLoader.addSmelting(RedOre.blockID, new ItemStack(RedIngot, 1)); ModLoader.addSmelting(mod_tutorial.RedIngot.itemID, new ItemStack(mod_tutorial.RedDust, 1)); ModLoader.addSmelting(GreenOre.blockID, new ItemStack(GreenIngot, 1)); ModLoader.addSmelting(mod_tutorial.GreenIngot.itemID, new ItemStack(mod_tutorial.GreenDust, 1)); ModLoader.addSmelting(PinkOre.blockID, new ItemStack(PinkIngot, 1)); ModLoader.addSmelting(mod_tutorial.PinkIngot.itemID, new ItemStack(PinkDust,1 )); ModLoader.addSmelting(PurpleOre.blockID, new ItemStack(PurpleIngot, 1)); ModLoader.addSmelting(mod_tutorial.PurpleIngot.itemID, new ItemStack(PurpleDust, 1)); ModLoader.addSmelting(BlackOre.blockID, new ItemStack(BlackIngot, 1)); ModLoader.addSmelting(mod_tutorial.BlackIngot.itemID, new ItemStack(BlackDust, 1)); ModLoader.addSmelting(mod_tutorial.RainbowIngot.itemID, new ItemStack(RainbowDust, 1)); //Furnace Stuff GameRegistry.addRecipe(new ItemStack(basicFurnace,1), new Object[]{ " X ", "XTX", " F ", 'X', Item.redstone, 'F', Block.stoneOvenIdle,'T',BasicCube }); GameRegistry.addRecipe(new ItemStack(BasicCube,1), new Object[]{ "YBP", "W E", "LRG", 'Y',YellowIngot, 'B', BlackIngot, 'P', PurpleIngot, 'W', WhiteIngot, 'E', PinkIngot, 'L', BlueIngot, 'R', RedIngot, 'G', GreenIngot }); //blue GameRegistry.addRecipe(new ItemStack(mod_tutorial.TutorialHelmet,1), new Object[]{ "TTT","TXT"," ",'T',BlueDust, 'X',WhiteHelmet }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.TutorialPlate,1), new Object[]{ "TXT","TTT","TTT",'T',BlueDust, 'X', WhitePlate }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.TutorialLegs,1), new Object[]{ "TTT","TXT","T T",'T',BlueDust, 'X', WhiteLegs }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.TutorialBoots,1), new Object[]{ " ","TXT","T T",'T',BlueDust, 'X', WhiteBoots }); //Yellow GameRegistry.addRecipe(new ItemStack(mod_tutorial.YellowHelmet,1), new Object[]{ "TTT","TXT"," ",'T',YellowDust, 'X',Item.helmetSteel }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.YellowPlate,1), new Object[]{ "TXT","TTT","TTT",'T',YellowDust, 'X', Item.plateSteel }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.YellowLegs,1), new Object[]{ "TTT","TXT","T T",'T',YellowDust, 'X', Item.legsSteel }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.YellowBoots,1), new Object[]{ " ","TXT","T T",'T',YellowDust, 'X', Item.bootsSteel }); //white GameRegistry.addRecipe(new ItemStack(mod_tutorial.WhiteHelmet,1), new Object[]{ "TTT","TXT"," ",'T',WhitePowder, 'X',YellowHelmet }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.WhitePlate,1), new Object[]{ "TXT","TTT","TTT",'T',WhitePowder, 'X', YellowPlate }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.WhiteLegs,1), new Object[]{ "TTT","TXT","T T",'T',WhitePowder, 'X', YellowLegs }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.WhiteBoots,1), new Object[]{ " ","TXT","T T",'T',WhitePowder, 'X', YellowBoots }); //red GameRegistry.addRecipe(new ItemStack(mod_tutorial.RedHelmet,1), new Object[]{ "TTT","TXT"," ",'T',RedDust, 'X',TutorialHelmet }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.RedPlate,1), new Object[]{ "TXT","TTT","TTT",'T',RedDust, 'X', TutorialPlate }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.RedLegs,1), new Object[]{ "TTT","TXT","T T",'T',RedDust, 'X', TutorialLegs }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.RedBoots,1), new Object[]{ " ","TXT","T T",'T',RedDust, 'X', TutorialBoots }); //green GameRegistry.addRecipe(new ItemStack(mod_tutorial.GreenHelmet,1), new Object[]{ "TTT","TXT"," ",'T',GreenDust, 'X', RedHelmet }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.GreenPlate,1), new Object[]{ "TXT","TTT","TTT",'T',GreenDust, 'X', RedPlate }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.GreenLegs,1), new Object[]{ "TTT","TXT","T T",'T',GreenDust, 'X', RedLegs }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.GreenBoots,1), new Object[]{ " ","TXT","T T",'T',GreenDust, 'X', RedBoots }); //pink GameRegistry.addRecipe(new ItemStack(mod_tutorial.PinkHelmet,1), new Object[]{ "TTT","TXT"," ",'T',PinkDust, 'X',GreenHelmet }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.PinkPlate,1), new Object[]{ "TXT","TTT","TTT",'T',PinkDust, 'X', GreenPlate }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.PinkLegs,1), new Object[]{ "TTT","TXT","T T",'T',PinkDust, 'X', GreenLegs }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.PinkBoots,1), new Object[]{ " ","TXT","T T",'T',PinkDust, 'X', GreenBoots }); //purple GameRegistry.addRecipe(new ItemStack(mod_tutorial.PurpleHelmet,1), new Object[]{ "TTT","TXT"," ",'T',PurpleDust, 'X',PinkHelmet }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.PurplePlate,1), new Object[]{ "TXT","TTT","TTT",'T',PurpleDust, 'X', PinkPlate }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.PurpleLegs,1), new Object[]{ "TTT","TXT","T T",'T',PurpleDust, 'X', PinkLegs }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.PurpleBoots,1), new Object[]{ " ","TXT","T T",'T',PurpleDust, 'X', PinkBoots }); //black GameRegistry.addRecipe(new ItemStack(mod_tutorial.BlackHelmet,1), new Object[]{ "TTT","TXT"," ",'T',BlackDust, 'X',PurpleHelmet }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.BlackPlate,1), new Object[]{ "TXT","TTT","TTT",'T',BlackDust, 'X', PurplePlate }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.BlackLegs,1), new Object[]{ "TTT","TXT","T T",'T',BlackDust, 'X', PurpleLegs }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.BlackBoots,1), new Object[]{ " ","TXT","T T",'T',BlackDust, 'X', PurpleBoots }); //Rainbow GameRegistry.addRecipe(new ItemStack(mod_tutorial.RainbowHelmet,1), new Object[]{ "TTT","TXT"," ",'T',RainbowDust, 'X',BlackHelmet }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.RainbowPlate,1), new Object[]{ "TXT","TTT","TTT",'T',RainbowDust, 'X', BlackPlate }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.RainbowLegs,1), new Object[]{ "TTT","TXT","T T",'T',RainbowDust, 'X', BlackLegs }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.RainbowBoots,1), new Object[]{ " ","TXT","T T",'T',RainbowDust, 'X', BlackBoots }); //Sword out of YellowIngot GameRegistry.addRecipe(new ItemStack(YellowSword, 1), new Object[]{ " * ", " * ", " X ", 'X', Item.swordSteel, '*', mod_tutorial.YellowToolFragment }); //Pickaxe out of YellowIngot GameRegistry.addRecipe(new ItemStack(YellowPickaxe, 1), new Object[]{ " * ", " X ", 'X', Item.pickaxeSteel, '*', mod_tutorial.YellowToolFragment }); //Axe out of YellowIngot GameRegistry.addRecipe(new ItemStack(YellowAxe, 1), new Object[]{ "** ", "*X ", 'X', Item.axeSteel, '*', mod_tutorial.YellowToolFragment }); //Spade out of Yellow Ingot GameRegistry.addRecipe(new ItemStack(YellowSpade), new Object[]{ " * ", " X ", 'X', Item.shovelSteel, '*', mod_tutorial.YellowToolFragment }); //Yellow Tool Fragment GameRegistry.addRecipe(new ItemStack(YellowToolFragment, 1), new Object[]{ "***", " * ", '*', mod_tutorial.YellowIngot }); //WHITE //WhitePowder out of Dust GameRegistry.addRecipe(new ItemStack(WhitePowder), new Object[]{ "***", "*X*", "***", '*', mod_tutorial.YellowDust, 'X', mod_tutorial.SilverDust }); //Sword out of White Ingot GameRegistry.addRecipe(new ItemStack(WhiteSword, 1), new Object[]{ " * ", " X ", 'X', YellowSword, '*', mod_tutorial.WhiteToolFragment }); //Yellow out of White Ingot GameRegistry.addRecipe(new ItemStack(WhitePickaxe, 1), new Object[]{ " * ", " X ", 'X', YellowPickaxe, '*', mod_tutorial.WhiteToolFragment }); //Axe out of White Ingot GameRegistry.addRecipe(new ItemStack(WhiteAxe, 1), new Object[]{ " * ", " X ", 'X', YellowAxe, '*', mod_tutorial.WhiteToolFragment }); //Spade out of White Ingot GameRegistry.addRecipe(new ItemStack(WhiteSpade, 1), new Object[]{ " * ", " X ", 'X', YellowSpade, '*', mod_tutorial.WhiteToolFragment }); //White Tool Fragment GameRegistry.addRecipe(new ItemStack(WhiteToolFragment, 1), new Object[]{ "***", " * ", '*', mod_tutorial.WhiteIngot }); //BLUE //Sword out of Blue Ingot GameRegistry.addRecipe(new ItemStack(BlueSword, 1), new Object[]{ " * ", " X ", 'X', WhiteSword, '*', mod_tutorial.BlueToolFragment }); //Yellow out of Blue Ingot GameRegistry.addRecipe(new ItemStack(BluePickaxe, 1), new Object[]{ " * ", " X ", 'X', WhitePickaxe, '*', mod_tutorial.BlueToolFragment }); //Spade out of Blue Ingot GameRegistry.addRecipe(new ItemStack(BlueSpade, 1), new Object[]{ " * ", " X ", 'X', WhiteSpade, '*', mod_tutorial.BlueToolFragment });; //Axe out of Blue Ingot GameRegistry.addRecipe(new ItemStack(BlueAxe, 1), new Object[]{ " * ", " X ", 'X', WhiteAxe, '*', mod_tutorial.BlueToolFragment }); //Blue Tool Fragment GameRegistry.addRecipe(new ItemStack(BlueToolFragment, 1), new Object[]{ "***", " * ", '*', mod_tutorial.BlueIngot }); //RED //Axe out of Red Ingot GameRegistry.addRecipe(new ItemStack(RedAxe, 1), new Object[]{ " * ", " X ", 'X', BlueAxe, '*', mod_tutorial.RedToolFragment }); //Yellow out of Red Ingot GameRegistry.addRecipe(new ItemStack(RedPickaxe, 1), new Object[]{ " * ", " X ", 'X', BluePickaxe, '*', mod_tutorial.RedToolFragment }); //Sword out of Red Ingot GameRegistry.addRecipe(new ItemStack(RedSword, 1), new Object[]{ " * ", " X ", 'X', BlueSword, '*', mod_tutorial.RedToolFragment }); //Spade out of Red Ingot GameRegistry.addRecipe(new ItemStack(RedSpade, 1), new Object[]{ " * ", " X ", 'X', BlueSpade, '*', mod_tutorial.RedToolFragment }); //Red Tool Fragment GameRegistry.addRecipe(new ItemStack(RedToolFragment, 1), new Object[]{ "***", " * ", '*', mod_tutorial.RedIngot }); //Green //Axe out of Green Ingot GameRegistry.addRecipe(new ItemStack(GreenAxe, 1), new Object[]{ " * ", " X ", 'X', RedAxe, '*', mod_tutorial.GreenToolFragment }); //Yellow out of Green Ingot GameRegistry.addRecipe(new ItemStack(GreenPickaxe, 1), new Object[]{ " * ", " X ", 'X', RedPickaxe, '*', mod_tutorial.GreenToolFragment }); //Sword out of Green Ingot GameRegistry.addRecipe(new ItemStack(GreenSword, 1), new Object[]{ " * ", " X ", 'X', RedSword, '*', mod_tutorial.GreenToolFragment }); //Spade out of Green Ingot GameRegistry.addRecipe(new ItemStack(GreenSpade, 1), new Object[]{ " * ", " X ", 'X', RedSpade, '*', mod_tutorial.GreenToolFragment }); //Green Tool Fragment GameRegistry.addRecipe(new ItemStack(GreenToolFragment, 1), new Object[]{ "***", " * ", '*', mod_tutorial.GreenIngot }); //Pink //Axe out of Pink Ingot GameRegistry.addRecipe(new ItemStack(PinkAxe, 1), new Object[]{ " * ", " X ", 'X', GreenAxe, '*', mod_tutorial.PinkToolFragment }); //Yellow out of Pink Ingot GameRegistry.addRecipe(new ItemStack(PinkPickaxe, 1), new Object[]{ " * ", " X ", 'X', GreenPickaxe, '*', mod_tutorial.PinkToolFragment }); //Sword out of Pink Ingot GameRegistry.addRecipe(new ItemStack(PinkSword, 1), new Object[]{ " * ", " X ", 'X', GreenSword, '*', mod_tutorial.PinkToolFragment }); //Spade out of Pink Ingot GameRegistry.addRecipe(new ItemStack(PinkSpade, 1), new Object[]{ " * ", " X ", 'X', GreenSpade, '*', mod_tutorial.PinkToolFragment }); //Pink Tool Fragment GameRegistry.addRecipe(new ItemStack(PinkToolFragment, 1), new Object[]{ "***", " * ", '*', mod_tutorial.PinkIngot }); //Purple //Axe out of Purple Ingot GameRegistry.addRecipe(new ItemStack(PurpleAxe, 1), new Object[]{ " * ", " X ", 'X', PinkAxe, '*', mod_tutorial.PurpleToolFragment }); //Yellow out of Purple Ingot GameRegistry.addRecipe(new ItemStack(PurplePickaxe, 1), new Object[]{ " * ", " X ", 'X', PinkPickaxe, '*', mod_tutorial.PurpleToolFragment }); //Sword out of Purple Ingot GameRegistry.addRecipe(new ItemStack(PurpleSword, 1), new Object[]{ " * ", " X ", 'X', PinkSword, '*', mod_tutorial.PurpleToolFragment }); //Spade out of Purple Ingot GameRegistry.addRecipe(new ItemStack(PurpleSpade, 1), new Object[]{ " * ", " X ", 'X', PinkSpade, '*', mod_tutorial.PurpleToolFragment }); //Purple Tool Fragment GameRegistry.addRecipe(new ItemStack(PurpleToolFragment, 1), new Object[]{ "***", " * ", '*', mod_tutorial.PurpleIngot }); //Black //Axe out of Black Ingot GameRegistry.addRecipe(new ItemStack(BlackAxe, 1), new Object[]{ " * ", " X ", 'X', PurpleAxe, '*', mod_tutorial.BlackToolFragment }); //Yellow out of Black Ingot GameRegistry.addRecipe(new ItemStack(BlackPickaxe, 1), new Object[]{ " * ", " X ", 'X', PurplePickaxe, '*', mod_tutorial.BlackToolFragment }); //Sword out of Black Ingot GameRegistry.addRecipe(new ItemStack(BlackSword, 1), new Object[]{ " * ", " X ", 'X', PurpleSword, '*', mod_tutorial.BlackToolFragment }); //Spade out of Black Ingot GameRegistry.addRecipe(new ItemStack(BlackSpade, 1), new Object[]{ " * ", " X ", 'X', PurpleSpade, '*', mod_tutorial.BlackToolFragment }); //Black Tool Fragment GameRegistry.addRecipe(new ItemStack(BlackToolFragment, 1), new Object[]{ "***", " * ", '*', mod_tutorial.BlackIngot }); //Rainbow //Axe out of Rainbow Ingot GameRegistry.addRecipe(new ItemStack(RainbowAxe, 1), new Object[]{ " * ", " X ", 'X', BlackAxe, '*', mod_tutorial.RainbowToolFragment }); //Yellow out of Rainbow Ingot GameRegistry.addRecipe(new ItemStack(RainbowPickaxe, 1), new Object[]{ " * ", " X ", 'X', BlackPickaxe, '*', mod_tutorial.RainbowToolFragment }); //Sword out of Rainbow Ingot GameRegistry.addRecipe(new ItemStack(RainbowSword, 1), new Object[]{ " * ", " X ", 'X', BlackSword, '*', mod_tutorial.RainbowToolFragment }); //Spade out of Rainbow Ingot GameRegistry.addRecipe(new ItemStack(RainbowSpade, 1), new Object[]{ " * ", " X ", 'X', BlackSpade, '*', mod_tutorial.RainbowToolFragment }); //Rainbow Tool Fragment GameRegistry.addRecipe(new ItemStack(RainbowToolFragment, 1), new Object[]{ "***", " * ", '*', mod_tutorial.RainbowIngot }); //furnace LanguageRegistry.addName(basicFurnace, "Basic Furnace"); LanguageRegistry.addName(BasicCube, "Basic Cube"); //Blue LanguageRegistry.addName(TutorialHelmet, "Blue Helmet"); LanguageRegistry.addName(TutorialPlate, "Blue ChestPlate"); LanguageRegistry.addName(TutorialLegs, "Blue Leggings"); LanguageRegistry.addName(TutorialBoots, "Blue Boots"); LanguageRegistry.addName(BlueIngot, "Blue Ingot"); LanguageRegistry.addName(BlueToolFragment, "Blue-Tool-Fragment"); LanguageRegistry.addName(BlueSword, "Blue Sword"); LanguageRegistry.addName(BluePickaxe, "Blue Pickaxe"); LanguageRegistry.addName(BlueSpade, "Blue Spade"); LanguageRegistry.addName(BlueAxe, "Blue Axe"); LanguageRegistry.addName(BlueDust, "Blue Dust"); LanguageRegistry.addName(BlueOre, "Blue Ore"); //Yellow LanguageRegistry.addName(YellowHelmet, "Orange Helmet"); LanguageRegistry.addName(YellowPlate, "Orange ChestPlate"); LanguageRegistry.addName(YellowLegs, "Orange Leggings"); LanguageRegistry.addName(YellowBoots, "Orange Boots"); LanguageRegistry.addName(YellowIngot, "Orange Ingot"); LanguageRegistry.addName(YellowToolFragment, "Orange-Tool-Fragment"); LanguageRegistry.addName(YellowSword, "Orange Sword"); LanguageRegistry.addName(YellowPickaxe, "Orange Pickaxe"); LanguageRegistry.addName(YellowSpade, "Orange Spade"); LanguageRegistry.addName(YellowAxe, "Orange Axe"); LanguageRegistry.addName(YellowDust, "Orange Dust"); LanguageRegistry.addName(YellowOre, "Orange Ore"); //White LanguageRegistry.addName(WhiteHelmet, "White Helmet"); LanguageRegistry.addName(WhitePlate, "White ChestPlate"); LanguageRegistry.addName(WhiteLegs, "White Leggings"); LanguageRegistry.addName(WhiteBoots, "White Boots"); LanguageRegistry.addName(WhiteIngot, "White Ingot"); LanguageRegistry.addName(WhiteToolFragment, "White-Tool-Fragment"); LanguageRegistry.addName(WhiteSword, "White Sword"); LanguageRegistry.addName(WhitePickaxe, "White Pickaxe"); LanguageRegistry.addName(WhiteSpade, "White Spade"); LanguageRegistry.addName(WhiteAxe, "White Axe"); LanguageRegistry.addName(SilverDust, "Silver Dust"); LanguageRegistry.addName(SilverOre, "Silver Ore"); LanguageRegistry.addName(WhitePowder, "White Powder"); LanguageRegistry.addName(SilverIngot, "SilverIngot"); //Red LanguageRegistry.addName(RedHelmet, "Red Helmet"); LanguageRegistry.addName(RedPlate, "Red ChestPlate"); LanguageRegistry.addName(RedLegs, "Red Leggings"); LanguageRegistry.addName(RedBoots, "Red Boots"); LanguageRegistry.addName(RedToolFragment, "Red-Tool-Fragment"); LanguageRegistry.addName(RedSword, "Red Sword"); LanguageRegistry.addName(RedPickaxe, "Red Pickaxe"); LanguageRegistry.addName(RedSpade, "Red Spade"); LanguageRegistry.addName(RedAxe, "Red Axe"); LanguageRegistry.addName(RedDust, "Red Dust"); LanguageRegistry.addName(RedOre, "Red Ore"); LanguageRegistry.addName(RedIngot, "Red Ingot"); //Green LanguageRegistry.addName(GreenHelmet, "Green Helmet"); LanguageRegistry.addName(GreenPlate, "Green ChestPlate"); LanguageRegistry.addName(GreenLegs, "Green Leggings"); LanguageRegistry.addName(GreenBoots, "Green Boots"); LanguageRegistry.addName(GreenToolFragment, "Green-Tool-Fragment"); LanguageRegistry.addName(GreenSword, "Green Sword"); LanguageRegistry.addName(GreenPickaxe, "Green Pickaxe"); LanguageRegistry.addName(GreenSpade, "Green Spade"); LanguageRegistry.addName(GreenAxe, "Green Axe"); LanguageRegistry.addName(GreenDust, "Green Dust"); LanguageRegistry.addName(GreenOre, "Green Ore"); LanguageRegistry.addName(GreenIngot, "Green Ingot"); //Pink LanguageRegistry.addName(PinkHelmet, "Pink Helmet"); LanguageRegistry.addName(PinkPlate, "Pink ChestPlate"); LanguageRegistry.addName(PinkLegs, "Pink Leggings"); LanguageRegistry.addName(PinkBoots, "Pink Boots"); LanguageRegistry.addName(PinkToolFragment, "Pink-Tool-Fragment"); LanguageRegistry.addName(PinkSword, "Pink Sword"); LanguageRegistry.addName(PinkPickaxe, "Pink Pickaxe"); LanguageRegistry.addName(PinkSpade, "Pink Spade"); LanguageRegistry.addName(PinkAxe, "Pink Axe"); LanguageRegistry.addName(PinkDust, "Pink Dust"); LanguageRegistry.addName(PinkOre, "Pink Ore"); LanguageRegistry.addName(PinkIngot, "Pink Ingot"); //Purple LanguageRegistry.addName(PurpleHelmet, "Purple Helmet"); LanguageRegistry.addName(PurplePlate, "Purple ChestPlate"); LanguageRegistry.addName(PurpleLegs, "Purple Leggings"); LanguageRegistry.addName(PurpleBoots, "Purple Boots"); LanguageRegistry.addName(PurpleToolFragment, "Purple-Tool-Fragment"); LanguageRegistry.addName(PurpleSword, "Purple Sword"); LanguageRegistry.addName(PurplePickaxe, "Purple Pickaxe"); LanguageRegistry.addName(PurpleSpade, "Purple Spade"); LanguageRegistry.addName(PurpleAxe, "Purple Axe"); LanguageRegistry.addName(PurpleDust, "Purple Dust"); LanguageRegistry.addName(PurpleOre, "Purple Ore"); LanguageRegistry.addName(PurpleIngot, "Purple Ingot"); //Black LanguageRegistry.addName(BlackHelmet, "Black Helmet"); LanguageRegistry.addName(BlackPlate, "Black ChestPlate"); LanguageRegistry.addName(BlackLegs, "Black Leggings"); LanguageRegistry.addName(BlackBoots, "Black Boots"); LanguageRegistry.addName(BlackToolFragment, "Black-Tool-Fragment"); LanguageRegistry.addName(BlackSword, "Black Sword"); LanguageRegistry.addName(BlackPickaxe, "Black Pickaxe"); LanguageRegistry.addName(BlackSpade, "Black Spade"); LanguageRegistry.addName(BlackAxe, "Black Axe"); LanguageRegistry.addName(BlackDust, "Black Dust"); LanguageRegistry.addName(BlackOre, "Black Ore"); LanguageRegistry.addName(BlackIngot, "Black Ingot"); //Rainbow LanguageRegistry.addName(RainbowHelmet, "Rainbow Helmet"); LanguageRegistry.addName(RainbowPlate, "Rainbow ChestPlate"); LanguageRegistry.addName(RainbowLegs, "Rainbow Leggings"); LanguageRegistry.addName(RainbowBoots, "Rainbow Boots"); LanguageRegistry.addName(RainbowToolFragment, "Rainbow-Tool-Fragment"); LanguageRegistry.addName(RainbowSword, "Rainbow Sword"); LanguageRegistry.addName(RainbowPickaxe, "Rainbow Pickaxe"); LanguageRegistry.addName(RainbowSpade, "Rainbow Spade"); LanguageRegistry.addName(RainbowAxe, "Rainbow Axe"); LanguageRegistry.addName(RainbowDust, "Rainbow Dust"); LanguageRegistry.addName(RainbowIngot, "Rainbow Ingot"); LanguageRegistry.addName(RainbowHoe, "Rainbow Hoe"); //Ore Generation GameRegistry.registerWorldGenerator(new OreWorldGen()); } }
Also please don't give grief on some things. I know I shouldn't hard code, I know I should have the enums set not coded in. Anyways there it is. Hope you can help. Thanks!
-
Ok duh. I don't need a java thing. Its just in the first post I had the class file for the ItemTutorialHelmet and he said check, when he could have just looked... I didn't know what he ment because nothing in my ItemTutorialHelmet said protected.. So no one knows how to fix this? Ive had this problem for a month now. I cant use my mod!! Please help!
-
How do I see if its protected??
-
So ive been looking around and posting in many forums and have been getting no responses. For some reason my mod is acting up. I have recompiled and rebofuscated it. I have it in the correct location and everything and this is the error I get:
Minecraft Error(From the actual Client):
Minecraft has crashed! ---------------------- Minecraft has stopped running because it encountered a problem; Failed to start game A full error report has been saved to C:\Users\jacks_000\AppData\Roaming\.minecraft\crash-reports\crash-2013-03-16_10.24.28-client.txt - Please include a copy of that file (Not this screen!) if you report this crash to anyone; without it, they will not be able to help fix the crash --- BEGIN ERROR REPORT 15056c9e -------- Full report at: C:\Users\jacks_000\AppData\Roaming\.minecraft\crash-reports\crash-2013-03-16_10.24.28-client.txt Please show that file to Mojang, NOT just this screen! Generated 3/16/13 10:24 AM -- System Details -- Details: Minecraft Version: 1.4.7 Operating System: Windows 8 (amd64) version 6.2 Java Version: 1.7.0_13, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 367941512 bytes (350 MB) / 514523136 bytes (490 MB) up to 954466304 bytes (910 MB) JVM Flags: 2 total; -Xms512m -Xmx1024m AABB Pool Size: 0 (0 bytes; 0 MB) allocated, 0 (0 bytes; 0 MB) used Suspicious classes: FML and Forge are installed IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: MCP v7.26 FML v4.7.4.520 Minecraft Forge 6.6.0.497 4 mods loaded, 4 mods active mcp [Minecraft Coder Pack] (minecraft.jar) Unloaded->Constructed->Pre-initialized->Initialized FML [Forge Mod Loader] (coremods) Unloaded->Constructed->Pre-initialized->Initialized Forge [Minecraft Forge] (coremods) Unloaded->Constructed->Pre-initialized->Initialized JV's ToolPack [JV's ToolPack] (JV'sToolPack.zip) Unloaded->Constructed->Pre-initialized->Errored LWJGL: 2.4.2 OpenGL: AMD Radeon HD 7570 GL version 4.2.11750 Compatibility Profile Context, ATI Technologies Inc. Is Modded: Definitely; Client brand changed to 'forge,fml' Type: Client (map_client.txt) Texture Pack: Default Profiler Position: N/A (disabled) Vec3 Pool Size: ~~ERROR~~ NullPointerException: null cpw.mods.fml.common.LoaderException: java.lang.VerifyError: (class: tutorialtest/ItemTutorialHelmet, method: getArmorTextureFile signature: (Lur;)Ljava/lang/String;) Bad access to protected data at cpw.mods.fml.common.LoadController.transition(LoadController.java:117) at cpw.mods.fml.common.Loader.initializeMods(Loader.java:658) at cpw.mods.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:207) at net.minecraft.client.Minecraft.a(Minecraft.java:456) at asq.a(SourceFile:56) at net.minecraft.client.Minecraft.run(Minecraft.java:744) at java.lang.Thread.run(Unknown Source) Caused by: java.lang.VerifyError: (class: tutorialtest/ItemTutorialHelmet, method: getArmorTextureFile signature: (Lur;)Ljava/lang/String;) Bad access to protected data at tutorialtest.mod_tutorial.load(mod_tutorial.java:185) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at cpw.mods.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:485) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:69) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:317) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:300) at com.google.common.eventbus.EventBus.post(EventBus.java:268) at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:140) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:69) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:317) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:300) at com.google.common.eventbus.EventBus.post(EventBus.java:268) at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:83) at cpw.mods.fml.common.Loader.initializeMods(Loader.java:657) ... 5 more --- END ERROR REPORT 5263e850 ----------
The game runs from eclipse fine everytime. Its also weird how I have 8 other armor sets and they all contain helmets, all our programed the same way just different icon indexes, textures, and all that jazz. Im not sure what the problem is here. It also refers the the ItemTutorialHelmet class so here it is:
ItemTutorialHelmet class:
package tutorialtest; import tutorial.client.ClientProxy.ClientProxy; import net.minecraft.item.EnumArmorMaterial; import net.minecraft.item.ItemArmor; import net.minecraft.item.ItemStack; import net.minecraftforge.common.IArmorTextureProvider; public class ItemTutorialHelmet extends ItemArmor implements IArmorTextureProvider{ public ItemTutorialHelmet(int par1, EnumArmorMaterial par2EnumArmorMaterial, int par3, int par4) { super(par1, par2EnumArmorMaterial, par3, par4); } public String getTextureFile(){ return ClientProxy.ITEMS_PNG; } public String getArmorTextureFile(ItemStack par1){ if ( par1.itemID==mod_tutorial.TutorialHelmet.iconIndex|| par1.itemID==mod_tutorial.TutorialPlate.iconIndex|| par1.itemID==mod_tutorial.TutorialBoots.iconIndex){ return "/armor/Tut_1.png"; }if(par1.itemID==mod_tutorial.TutorialLegs.iconIndex){ return "/armor/Tut_2.png"; }return "/armor/Tut_1.png"; } }
It also refers to line 185 in my mod_tutorial(Main class file):
TutorialHelmet = new ItemTutorialHelmet(508,EnumArmorMaterial.BLUE, proxy.addArmor("Tut"),0).setItemName("TutHelm").setIconIndex(0).setCreativeTab(tabJVCraft);
If someone could please help I would be very happy. If you need any more information just ask and I will gladly give it to you. Thanks!
-
Ok so its great i figured out how to get the proxy working but now when i export my mod and run it with minecraft i get this error again:
Minecraft Error(From Client):
Minecraft has crashed! ---------------------- Minecraft has stopped running because it encountered a problem; Failed to start game A full error report has been saved to C:\Users\Jackson\AppData\Roaming\.minecraft\crash-reports\crash-2013-03-10_09.45.50-client.txt - Please include a copy of that file (Not this screen!) if you report this crash to anyone; without it, they will not be able to help fix the crash --- BEGIN ERROR REPORT 6be41df6 -------- Full report at: C:\Users\Jackson\AppData\Roaming\.minecraft\crash-reports\crash-2013-03-10_09.45.50-client.txt Please show that file to Mojang, NOT just this screen! Generated 3/10/13 9:45 AM -- System Details -- Details: Minecraft Version: 1.4.7 Operating System: Windows 7 (amd64) version 6.1 Java Version: 1.6.0_20, Sun Microsystems Inc. Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Sun Microsystems Inc. Memory: 377892968 bytes (360 MB) / 514523136 bytes (490 MB) up to 954466304 bytes (910 MB) JVM Flags: 2 total; -Xms512m -Xmx1024m AABB Pool Size: 0 (0 bytes; 0 MB) allocated, 0 (0 bytes; 0 MB) used Suspicious classes: FML and Forge are installed IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: MCP v7.26 FML v4.7.4.520 Minecraft Forge 6.6.0.497 4 mods loaded, 4 mods active mcp [Minecraft Coder Pack] (minecraft.jar) Unloaded->Constructed->Pre-initialized->Initialized FML [Forge Mod Loader] (coremods) Unloaded->Constructed->Pre-initialized->Initialized Forge [Minecraft Forge] (coremods) Unloaded->Constructed->Pre-initialized->Initialized JV's ToolPack [JV's ToolPack] (JV'sArmorMod.zip) Unloaded->Constructed->Pre-initialized->Errored LWJGL: 2.4.2 OpenGL: AMD M880G with ATI Mobility Radeon HD 4250 GL version 3.3.10179 Compatibility Profile Context, ATI Technologies Inc. Is Modded: Definitely; Client brand changed to 'forge,fml' Type: Client (map_client.txt) Texture Pack: Default Profiler Position: N/A (disabled) Vec3 Pool Size: ~~ERROR~~ NullPointerException: null cpw.mods.fml.common.LoaderException: java.lang.VerifyError: (class: tutorialtest/ItemTutorialHelmet, method: getArmorTextureFile signature: (Lur;)Ljava/lang/String;) Bad access to protected data at cpw.mods.fml.common.LoadController.transition(LoadController.java:117) at cpw.mods.fml.common.Loader.initializeMods(Loader.java:658) at cpw.mods.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:207) at net.minecraft.client.Minecraft.a(Minecraft.java:456) at asq.a(SourceFile:56) at net.minecraft.client.Minecraft.run(Minecraft.java:744) at java.lang.Thread.run(Unknown Source) Caused by: java.lang.VerifyError: (class: tutorialtest/ItemTutorialHelmet, method: getArmorTextureFile signature: (Lur;)Ljava/lang/String;) Bad access to protected data at tutorialtest.mod_tutorial.load(mod_tutorial.java:184) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at cpw.mods.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:485) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:69) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:317) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:300) at com.google.common.eventbus.EventBus.post(EventBus.java:268) at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:140) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:69) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:317) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:300) at com.google.common.eventbus.EventBus.post(EventBus.java:268) at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:83) at cpw.mods.fml.common.Loader.initializeMods(Loader.java:657) ... 5 more --- END ERROR REPORT 2cc53ab6 ----------
The funny thing is it launches from eclipse fine everytime. I dont know if im not doing something right or what. I hope you can help! Thanks!
-
woot i figured it out thanks dieieben07! tutorial.client.ClientProxy was just the package name. To actually get the file its tutorial.client.ClientProxy.ClientProxy
The last ClientProxy is the class name. THanks!
-
What do you mean its spelled wrong? This is what I have:
@SidedProxy(clientSide = "tutorial.client.ClientProxy", serverSide = "tutorial.common.CommonProxy")
public static CommonProxy proxy;
Im not sure what you mean. I really need to fix this or im going to need to go back to ModLoader.addArmor which I dont want to. Hope you guys can help, Thanks!
-
Ok so I did what you said and insted of just ClientProxy and CommonProxy i put the location. Still there is an error. I didnt export this time, just ran it from eclipse, but theres still the error. Thats why i had it set as ModLoader.addArmor not proxy.addArmor. Hope you can help! If you need anymore info please tell!! Here is the error:
Eclipse Console Error:
2013-03-07 18:03:53 [iNFO] [ForgeModLoader] Forge Mod Loader version 4.7.32.553 for Minecraft 1.4.7 loading 2013-03-07 18:03:55 [iNFO] [sTDOUT] 27 achievements 2013-03-07 18:03:55 [iNFO] [sTDOUT] 210 recipes 2013-03-07 18:03:55 [iNFO] [sTDOUT] Setting user: Player799, - 2013-03-07 18:03:55 [iNFO] [sTDERR] Client asked for parameter: server 2013-03-07 18:03:55 [iNFO] [sTDOUT] LWJGL Version: 2.4.2 2013-03-07 18:03:56 [iNFO] [MinecraftForge] Attempting early MinecraftForge initialization 2013-03-07 18:03:56 [iNFO] [sTDOUT] MinecraftForge v6.6.1.524 Initialized 2013-03-07 18:03:56 [iNFO] [ForgeModLoader] MinecraftForge v6.6.1.524 Initialized 2013-03-07 18:03:56 [iNFO] [sTDOUT] Replaced 84 ore recipies 2013-03-07 18:03:56 [iNFO] [MinecraftForge] Completed early MinecraftForge initialization 2013-03-07 18:03:56 [iNFO] [ForgeModLoader] Reading custom logging properties from C:\Users\jacks_000\Documents\My Games\Minecraft\Modding\MCP7\jars\config\logging.properties 2013-03-07 18:03:56 [OFF] [ForgeModLoader] Logging level for ForgeModLoader logging is set to ALL 2013-03-07 18:03:56 [iNFO] [ForgeModLoader] Searching C:\Users\jacks_000\Documents\My Games\Minecraft\Modding\MCP7\jars\mods for mods 2013-03-07 18:03:57 [iNFO] [ForgeModLoader] Attempting to reparse the mod container bin 2013-03-07 18:03:58 [iNFO] [ForgeModLoader] Forge Mod Loader has identified 4 mods to load 2013-03-07 18:03:58 [iNFO] [mcp] Activating mod mcp 2013-03-07 18:03:58 [iNFO] [FML] Activating mod FML 2013-03-07 18:03:58 [iNFO] [Forge] Activating mod Forge 2013-03-07 18:03:58 [iNFO] [JV's ToolPack] Activating mod JV's ToolPack 2013-03-07 18:03:58 [sEVERE] [ForgeModLoader] An error occured trying to load a proxy into {clientSide=tutorial.client.ClientProxy, serverSide=tutorial.common.CommonProxy}.tutorialtest.mod_tutorial java.lang.ClassNotFoundException: tutorial.client.ClientProxy at cpw.mods.fml.relauncher.RelaunchClassLoader.findClass(RelaunchClassLoader.java:185) at java.lang.ClassLoader.loadClass(Unknown Source) at java.lang.ClassLoader.loadClass(Unknown Source) at cpw.mods.fml.common.ModClassLoader.loadClass(ModClassLoader.java:57) at java.lang.Class.forName0(Native Method) at java.lang.Class.forName(Unknown Source) at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:52) at cpw.mods.fml.common.FMLModContainer.constructMod(FMLModContainer.java:464) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:69) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:317) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:300) at com.google.common.eventbus.EventBus.post(EventBus.java:268) at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:153) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:69) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:317) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:300) at com.google.common.eventbus.EventBus.post(EventBus.java:268) at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:86) at cpw.mods.fml.common.Loader.loadMods(Loader.java:494) at cpw.mods.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:161) at net.minecraft.client.Minecraft.startGame(Minecraft.java:410) at net.minecraft.client.MinecraftAppletImpl.startGame(MinecraftAppletImpl.java:44) at net.minecraft.client.Minecraft.run(Minecraft.java:744) at java.lang.Thread.run(Unknown Source) Caused by: java.lang.NullPointerException at org.objectweb.asm.ClassReader.<init>(Unknown Source) at net.minecraftforge.transformers.EventTransformer.transform(EventTransformer.java:29) at cpw.mods.fml.relauncher.RelaunchClassLoader.runTransformers(RelaunchClassLoader.java:228) at cpw.mods.fml.relauncher.RelaunchClassLoader.findClass(RelaunchClassLoader.java:173) ... 33 more 2013-03-07 18:03:58 [sEVERE] [ForgeModLoader] Fatal errors were detected during the transition from CONSTRUCTING to PREINITIALIZATION. Loading cannot continue 2013-03-07 18:03:58 [sEVERE] [ForgeModLoader] mcp [Minecraft Coder Pack] (minecraft.jar) Unloaded->Constructed FML [Forge Mod Loader] (coremods) Unloaded->Constructed Forge [Minecraft Forge] (coremods) Unloaded->Constructed JV's ToolPack [JV's ToolPack] (bin) Unloaded->Errored 2013-03-07 18:03:58 [sEVERE] [ForgeModLoader] The following problems were captured during this phase 2013-03-07 18:03:58 [sEVERE] [ForgeModLoader] Caught exception from JV's ToolPack cpw.mods.fml.common.LoaderException: java.lang.ClassNotFoundException: tutorial.client.ClientProxy at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:69) at cpw.mods.fml.common.FMLModContainer.constructMod(FMLModContainer.java:464) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:69) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:317) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:300) at com.google.common.eventbus.EventBus.post(EventBus.java:268) at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:153) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:69) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:317) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:300) at com.google.common.eventbus.EventBus.post(EventBus.java:268) at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:86) at cpw.mods.fml.common.Loader.loadMods(Loader.java:494) at cpw.mods.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:161) at net.minecraft.client.Minecraft.startGame(Minecraft.java:410) at net.minecraft.client.MinecraftAppletImpl.startGame(MinecraftAppletImpl.java:44) at net.minecraft.client.Minecraft.run(Minecraft.java:744) at java.lang.Thread.run(Unknown Source) Caused by: java.lang.ClassNotFoundException: tutorial.client.ClientProxy at cpw.mods.fml.relauncher.RelaunchClassLoader.findClass(RelaunchClassLoader.java:185) at java.lang.ClassLoader.loadClass(Unknown Source) at java.lang.ClassLoader.loadClass(Unknown Source) at cpw.mods.fml.common.ModClassLoader.loadClass(ModClassLoader.java:57) at java.lang.Class.forName0(Native Method) at java.lang.Class.forName(Unknown Source) at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:52) ... 27 more Caused by: java.lang.NullPointerException at org.objectweb.asm.ClassReader.<init>(Unknown Source) at net.minecraftforge.transformers.EventTransformer.transform(EventTransformer.java:29) at cpw.mods.fml.relauncher.RelaunchClassLoader.runTransformers(RelaunchClassLoader.java:228) at cpw.mods.fml.relauncher.RelaunchClassLoader.findClass(RelaunchClassLoader.java:173) ... 33 more 2013-03-07 18:04:08 [iNFO] [sTDERR] cpw.mods.fml.common.LoaderException: java.lang.ClassNotFoundException: tutorial.client.ClientProxy 2013-03-07 18:04:08 [iNFO] [sTDERR] at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:69) 2013-03-07 18:04:08 [iNFO] [sTDERR] at cpw.mods.fml.common.FMLModContainer.constructMod(FMLModContainer.java:464) 2013-03-07 18:04:08 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2013-03-07 18:04:08 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) 2013-03-07 18:04:08 [iNFO] [sTDERR] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) 2013-03-07 18:04:08 [iNFO] [sTDERR] at java.lang.reflect.Method.invoke(Unknown Source) 2013-03-07 18:04:08 [iNFO] [sTDERR] at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:69) 2013-03-07 18:04:08 [iNFO] [sTDERR] at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) 2013-03-07 18:04:08 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:317) 2013-03-07 18:04:08 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:300) 2013-03-07 18:04:08 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.post(EventBus.java:268) 2013-03-07 18:04:08 [iNFO] [sTDERR] at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:153) 2013-03-07 18:04:08 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2013-03-07 18:04:08 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) 2013-03-07 18:04:08 [iNFO] [sTDERR] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) 2013-03-07 18:04:08 [iNFO] [sTDERR] at java.lang.reflect.Method.invoke(Unknown Source) 2013-03-07 18:04:08 [iNFO] [sTDERR] at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:69) 2013-03-07 18:04:08 [iNFO] [sTDERR] at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) 2013-03-07 18:04:08 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:317) 2013-03-07 18:04:08 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:300) 2013-03-07 18:04:08 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.post(EventBus.java:268) 2013-03-07 18:04:08 [iNFO] [sTDERR] at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:86) 2013-03-07 18:04:08 [iNFO] [sTDERR] at cpw.mods.fml.common.Loader.loadMods(Loader.java:494) 2013-03-07 18:04:08 [iNFO] [sTDERR] at cpw.mods.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:161) 2013-03-07 18:04:08 [iNFO] [sTDERR] at net.minecraft.client.Minecraft.startGame(Minecraft.java:410) 2013-03-07 18:04:08 [iNFO] [sTDERR] at net.minecraft.client.MinecraftAppletImpl.startGame(MinecraftAppletImpl.java:44) 2013-03-07 18:04:08 [iNFO] [sTDERR] at net.minecraft.client.Minecraft.run(Minecraft.java:744) 2013-03-07 18:04:08 [iNFO] [sTDERR] at java.lang.Thread.run(Unknown Source) 2013-03-07 18:04:08 [iNFO] [sTDERR] Caused by: java.lang.ClassNotFoundException: tutorial.client.ClientProxy 2013-03-07 18:04:08 [iNFO] [sTDERR] at cpw.mods.fml.relauncher.RelaunchClassLoader.findClass(RelaunchClassLoader.java:185) 2013-03-07 18:04:08 [iNFO] [sTDERR] at java.lang.ClassLoader.loadClass(Unknown Source) 2013-03-07 18:04:08 [iNFO] [sTDERR] at java.lang.ClassLoader.loadClass(Unknown Source) 2013-03-07 18:04:08 [iNFO] [sTDERR] at cpw.mods.fml.common.ModClassLoader.loadClass(ModClassLoader.java:57) 2013-03-07 18:04:08 [iNFO] [sTDERR] at java.lang.Class.forName0(Native Method) 2013-03-07 18:04:08 [iNFO] [sTDERR] at java.lang.Class.forName(Unknown Source) 2013-03-07 18:04:08 [iNFO] [sTDERR] at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:52) 2013-03-07 18:04:08 [iNFO] [sTDERR] ... 27 more 2013-03-07 18:04:08 [iNFO] [sTDERR] Caused by: java.lang.NullPointerException 2013-03-07 18:04:08 [iNFO] [sTDERR] at org.objectweb.asm.ClassReader.<init>(Unknown Source) 2013-03-07 18:04:08 [iNFO] [sTDERR] at net.minecraftforge.transformers.EventTransformer.transform(EventTransformer.java:29) 2013-03-07 18:04:08 [iNFO] [sTDERR] at cpw.mods.fml.relauncher.RelaunchClassLoader.runTransformers(RelaunchClassLoader.java:228) 2013-03-07 18:04:08 [iNFO] [sTDERR] at cpw.mods.fml.relauncher.RelaunchClassLoader.findClass(RelaunchClassLoader.java:173) 2013-03-07 18:04:08 [iNFO] [sTDERR] ... 33 more
-
Ok so maybe you can tell me later about how to not hard code, but it didn't work!
It has something to do with the client proxy, take a look at the error:
Minecraft has crashed! ---------------------- Minecraft has stopped running because it encountered a problem; Failed to start game A full error report has been saved to C:\Users\jacks_000\AppData\Roaming\.minecraft\crash-reports\crash-2013-03-06_18.49.58-client.txt - Please include a copy of that file (Not this screen!) if you report this crash to anyone; without it, they will not be able to help fix the crash --- BEGIN ERROR REPORT 6c1b905f -------- Full report at: C:\Users\jacks_000\AppData\Roaming\.minecraft\crash-reports\crash-2013-03-06_18.49.58-client.txt Please show that file to Mojang, NOT just this screen! Generated 3/6/13 6:49 PM -- System Details -- Details: Minecraft Version: 1.4.7 Operating System: Windows 8 (amd64) version 6.2 Java Version: 1.7.0_13, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 414000320 bytes (394 MB) / 514523136 bytes (490 MB) up to 954466304 bytes (910 MB) JVM Flags: 2 total; -Xms512m -Xmx1024m AABB Pool Size: 0 (0 bytes; 0 MB) allocated, 0 (0 bytes; 0 MB) used Suspicious classes: FML and Forge are installed IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: MCP v7.26 FML v4.7.4.520 Minecraft Forge 6.6.0.497 4 mods loaded, 4 mods active mcp [Minecraft Coder Pack] (minecraft.jar) Unloaded->Constructed FML [Forge Mod Loader] (coremods) Unloaded->Constructed Forge [Minecraft Forge] (coremods) Unloaded->Constructed JV's ToolPack [JV's ToolPack] (JV'sToolPack.zip) Unloaded->Errored LWJGL: 2.4.2 OpenGL: AMD Radeon HD 7570 GL version 4.2.11750 Compatibility Profile Context, ATI Technologies Inc. Is Modded: Definitely; Client brand changed to 'forge,fml' Type: Client (map_client.txt) Texture Pack: Default Profiler Position: N/A (disabled) Vec3 Pool Size: ~~ERROR~~ NullPointerException: null cpw.mods.fml.common.LoaderException: java.lang.ClassNotFoundException: ClientProxy at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:69) at cpw.mods.fml.common.FMLModContainer.constructMod(FMLModContainer.java:462) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:69) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:317) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:300) at com.google.common.eventbus.EventBus.post(EventBus.java:268) at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:140) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:69) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:317) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:300) at com.google.common.eventbus.EventBus.post(EventBus.java:268) at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:83) at cpw.mods.fml.common.Loader.loadMods(Loader.java:479) at cpw.mods.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:161) at net.minecraft.client.Minecraft.a(Minecraft.java:410) at asq.a(SourceFile:56) at net.minecraft.client.Minecraft.run(Minecraft.java:744) at java.lang.Thread.run(Unknown Source) Caused by: java.lang.ClassNotFoundException: ClientProxy at cpw.mods.fml.relauncher.RelaunchClassLoader.findClass(RelaunchClassLoader.java:185) at java.lang.ClassLoader.loadClass(Unknown Source) at java.lang.ClassLoader.loadClass(Unknown Source) at cpw.mods.fml.common.ModClassLoader.loadClass(ModClassLoader.java:57) at java.lang.Class.forName0(Native Method) at java.lang.Class.forName(Unknown Source) at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:52) ... 27 more Caused by: java.lang.NullPointerException at cpw.mods.fml.relauncher.RelaunchClassLoader.findClass(RelaunchClassLoader.java:174) ... 33 more --- END ERROR REPORT 5a1baf51 ----------
Something about proxieingector or something. I hope you can help mnm, thanks!
-
WOW! Amazing scripting man! Hey can you give me a little detail on how to not hard code the ID's? I saw you did and there was ALOT of stuff in your main class its kinda confusing. Also you had all your items have an int. Was that so they could be non hard coded? Honestly I was kinda confused with all that. I managed to get all the proxy stuff done and im recompling as im typing. Hopefully it will work, but besides that I would love to know how to not hard code!
-
Im not sure what you mean? So erase the add Armor? See I have 9 more armor sets not including this one. And there all the same but the only error that comes up is for this ItemTutorialHelmet?!!
-
I recently exported my mod and am now trying to get it to work on the client. I installed everything correctly but there is still an error. Here is the error:
Error Log from Minecraft Client:
Minecraft has crashed! ---------------------- Minecraft has stopped running because it encountered a problem; Failed to start game A full error report has been saved to C:\Users\jacks_000\AppData\Roaming\.minecraft\crash-reports\crash-2013-03-04_21.22.28-client.txt - Please include a copy of that file (Not this screen!) if you report this crash to anyone; without it, they will not be able to help fix the crash --- BEGIN ERROR REPORT 11cf44c1 -------- Full report at: C:\Users\jacks_000\AppData\Roaming\.minecraft\crash-reports\crash-2013-03-04_21.22.28-client.txt Please show that file to Mojang, NOT just this screen! Generated 3/4/13 9:22 PM -- System Details -- Details: Minecraft Version: 1.4.7 Operating System: Windows 8 (amd64) version 6.2 Java Version: 1.7.0_13, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 368774032 bytes (351 MB) / 514523136 bytes (490 MB) up to 954466304 bytes (910 MB) JVM Flags: 2 total; -Xms512m -Xmx1024m AABB Pool Size: 0 (0 bytes; 0 MB) allocated, 0 (0 bytes; 0 MB) used Suspicious classes: FML and Forge are installed IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: MCP v7.26 FML v4.7.4.520 Minecraft Forge 6.6.0.497 4 mods loaded, 4 mods active mcp [Minecraft Coder Pack] (minecraft.jar) Unloaded->Constructed->Pre-initialized->Initialized FML [Forge Mod Loader] (coremods) Unloaded->Constructed->Pre-initialized->Initialized Forge [Minecraft Forge] (coremods) Unloaded->Constructed->Pre-initialized->Initialized JV's ToolPack [JV's ToolPack] (JV'sToolPack.zip) Unloaded->Constructed->Pre-initialized->Errored LWJGL: 2.4.2 OpenGL: AMD Radeon HD 7570 GL version 4.2.11750 Compatibility Profile Context, ATI Technologies Inc. Is Modded: Definitely; Client brand changed to 'forge,fml' Type: Client (map_client.txt) Texture Pack: Default Profiler Position: N/A (disabled) Vec3 Pool Size: ~~ERROR~~ NullPointerException: null cpw.mods.fml.common.LoaderException: java.lang.VerifyError: (class: tutorialtest/ItemTutorialHelmet, method: getArmorTextureFile signature: (Lur;)Ljava/lang/String;) Bad access to protected data at cpw.mods.fml.common.LoadController.transition(LoadController.java:117) at cpw.mods.fml.common.Loader.initializeMods(Loader.java:658) at cpw.mods.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:207) at net.minecraft.client.Minecraft.a(Minecraft.java:456) at asq.a(SourceFile:56) at net.minecraft.client.Minecraft.run(Minecraft.java:744) at java.lang.Thread.run(Unknown Source) Caused by: java.lang.VerifyError: (class: tutorialtest/ItemTutorialHelmet, method: getArmorTextureFile signature: (Lur;)Ljava/lang/String;) Bad access to protected data at tutorialtest.mod_tutorial.load(mod_tutorial.java:182) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at cpw.mods.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:485) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:69) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:317) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:300) at com.google.common.eventbus.EventBus.post(EventBus.java:268) at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:140) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:69) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:317) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:300) at com.google.common.eventbus.EventBus.post(EventBus.java:268) at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:83) at cpw.mods.fml.common.Loader.initializeMods(Loader.java:657) ... 5 more --- END ERROR REPORT 967c111b ----------
Here is the line in my code it is referring to somewhere in the error code (Line 182 in mod_tutorial):
TutorialHelmet = new ItemTutorialHelmet(508,EnumArmorMaterial.BLUE, ModLoader.addArmor("Tut"),0).setItemName("TutHelm").setIconIndex(0).setCreativeTab(tabJVCraft);
Here is my ItemTutorialHelmet class:
package tutorialtest; import net.minecraft.item.EnumArmorMaterial; import net.minecraft.item.ItemArmor; import net.minecraft.item.ItemStack; import net.minecraftforge.common.IArmorTextureProvider; public class ItemTutorialHelmet extends ItemArmor implements IArmorTextureProvider{ public ItemTutorialHelmet(int par1, EnumArmorMaterial par2EnumArmorMaterial, int par3, int par4) { super(par1, par2EnumArmorMaterial, par3, par4); } public String getTextureFile(){ return ClientProxy.ITEMS_PNG; } public String getArmorTextureFile(ItemStack par1){ if ( par1.itemID==mod_tutorial.TutorialHelmet.iconIndex|| par1.itemID==mod_tutorial.TutorialPlate.iconIndex|| par1.itemID==mod_tutorial.TutorialBoots.iconIndex){ return "/armor/Tut_1.png"; }if(par1.itemID==mod_tutorial.TutorialLegs.iconIndex){ return "/armor/Tut_2.png"; }return "/armor/Tut_1.png"; } }
I hope you can help. Im really not sure what the problem is on this one. Any advice would be great. Also, if you need to see any more script ASK! I will gladly give you more!!
-
The weird thing is I have 8 armor sets all modded the same except for iconindex, texture, id, and name but still. This error only pops up for one item which is weird unless its just covering for all the others so if I were to fix this one the next one in line I need to fix would pop up also I don't know. Heres my client proxy you asked for:
package tutorialtest; import cpw.mods.fml.client.registry.RenderingRegistry; import net.minecraftforge.client.MinecraftForgeClient; public class ClientProxy extends CommonProxy { public static String BLOCKS_PNG = "/tutorial/generic/block.png"; public static String ITEMS_PNG = "/tutorial/generic/items.png"; public static String FURNACE_PNG = "/tutorial/generic/furnace.png"; public static void registerRenderThings() { MinecraftForgeClient.preloadTexture(BLOCKS_PNG); MinecraftForgeClient.preloadTexture(ITEMS_PNG); MinecraftForgeClient.preloadTexture(FURNACE_PNG); } public int addArmor(String armor) { return RenderingRegistry.addNewArmourRendererPrefix(armor); } }
And don't worry I have everything in the right place. If it weren't I would assume there would be more errors unless it only shows one error at a time. Just to make sure I have my tutorial/generic/items.png in my zipped folder(JV'sModPack) in the .minecraft/mods folder. I also have it in the .minecraft just incase it wasent loading from JV'sModPack. Hope you can help Jdb100 or whoever is there to help! Thanks!
-
I recently exported my mod and am now trying to get it to work on the client. I installed everything correctly but there is still an error. Here is the error:
Error Log from Minecraft Client:
Minecraft has crashed! ---------------------- Minecraft has stopped running because it encountered a problem; Failed to start game A full error report has been saved to C:\Users\jacks_000\AppData\Roaming\.minecraft\crash-reports\crash-2013-03-04_21.22.28-client.txt - Please include a copy of that file (Not this screen!) if you report this crash to anyone; without it, they will not be able to help fix the crash --- BEGIN ERROR REPORT 11cf44c1 -------- Full report at: C:\Users\jacks_000\AppData\Roaming\.minecraft\crash-reports\crash-2013-03-04_21.22.28-client.txt Please show that file to Mojang, NOT just this screen! Generated 3/4/13 9:22 PM -- System Details -- Details: Minecraft Version: 1.4.7 Operating System: Windows 8 (amd64) version 6.2 Java Version: 1.7.0_13, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 368774032 bytes (351 MB) / 514523136 bytes (490 MB) up to 954466304 bytes (910 MB) JVM Flags: 2 total; -Xms512m -Xmx1024m AABB Pool Size: 0 (0 bytes; 0 MB) allocated, 0 (0 bytes; 0 MB) used Suspicious classes: FML and Forge are installed IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: MCP v7.26 FML v4.7.4.520 Minecraft Forge 6.6.0.497 4 mods loaded, 4 mods active mcp [Minecraft Coder Pack] (minecraft.jar) Unloaded->Constructed->Pre-initialized->Initialized FML [Forge Mod Loader] (coremods) Unloaded->Constructed->Pre-initialized->Initialized Forge [Minecraft Forge] (coremods) Unloaded->Constructed->Pre-initialized->Initialized JV's ToolPack [JV's ToolPack] (JV'sToolPack.zip) Unloaded->Constructed->Pre-initialized->Errored LWJGL: 2.4.2 OpenGL: AMD Radeon HD 7570 GL version 4.2.11750 Compatibility Profile Context, ATI Technologies Inc. Is Modded: Definitely; Client brand changed to 'forge,fml' Type: Client (map_client.txt) Texture Pack: Default Profiler Position: N/A (disabled) Vec3 Pool Size: ~~ERROR~~ NullPointerException: null cpw.mods.fml.common.LoaderException: java.lang.VerifyError: (class: tutorialtest/ItemTutorialHelmet, method: getArmorTextureFile signature: (Lur;)Ljava/lang/String;) Bad access to protected data at cpw.mods.fml.common.LoadController.transition(LoadController.java:117) at cpw.mods.fml.common.Loader.initializeMods(Loader.java:658) at cpw.mods.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:207) at net.minecraft.client.Minecraft.a(Minecraft.java:456) at asq.a(SourceFile:56) at net.minecraft.client.Minecraft.run(Minecraft.java:744) at java.lang.Thread.run(Unknown Source) Caused by: java.lang.VerifyError: (class: tutorialtest/ItemTutorialHelmet, method: getArmorTextureFile signature: (Lur;)Ljava/lang/String;) Bad access to protected data at tutorialtest.mod_tutorial.load(mod_tutorial.java:182) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at cpw.mods.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:485) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:69) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:317) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:300) at com.google.common.eventbus.EventBus.post(EventBus.java:268) at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:140) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:69) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:317) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:300) at com.google.common.eventbus.EventBus.post(EventBus.java:268) at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:83) at cpw.mods.fml.common.Loader.initializeMods(Loader.java:657) ... 5 more --- END ERROR REPORT 967c111b ----------
Here is the line in my code it is referring to somewhere in the error code (Line 182 in mod_tutorial):
TutorialHelmet = new ItemTutorialHelmet(508,EnumArmorMaterial.BLUE, ModLoader.addArmor("Tut"),0).setItemName("TutHelm").setIconIndex(0).setCreativeTab(tabJVCraft);
Here is my ItemTutorialHelmet class:
package tutorialtest; import net.minecraft.item.EnumArmorMaterial; import net.minecraft.item.ItemArmor; import net.minecraft.item.ItemStack; import net.minecraftforge.common.IArmorTextureProvider; public class ItemTutorialHelmet extends ItemArmor implements IArmorTextureProvider{ public ItemTutorialHelmet(int par1, EnumArmorMaterial par2EnumArmorMaterial, int par3, int par4) { super(par1, par2EnumArmorMaterial, par3, par4); } public String getTextureFile(){ return ClientProxy.ITEMS_PNG; } public String getArmorTextureFile(ItemStack par1){ if ( par1.itemID==mod_tutorial.TutorialHelmet.iconIndex|| par1.itemID==mod_tutorial.TutorialPlate.iconIndex|| par1.itemID==mod_tutorial.TutorialBoots.iconIndex){ return "/armor/Tut_1.png"; }if(par1.itemID==mod_tutorial.TutorialLegs.iconIndex){ return "/armor/Tut_2.png"; }return "/armor/Tut_1.png"; } }
I hope you can help. Im really not sure what the problem is on this one. Any advice would be great. Also, if you need to see any more script ASK! I will gladly give you more!!
-
When I run my mod, everything goes smoothly until I create a world and I get on the world. Then come up the Warnings: Texture /tutorial/generic/block.png not preloaded, will cause render glitches!
Warning Texture /tutorial/generic/furnace.png not preloaded, will cause render glitches!
Here is also a picture of it on the screen: https://www.dropbox.com/s/qi12sgziezbh670/Minecraft%20Preloading%20Error.png?m
Now I have everything preloaded and such in my client proxy. Here it is:
package tutorialtest; import cpw.mods.fml.client.registry.RenderingRegistry; import net.minecraftforge.client.MinecraftForgeClient; public class ClientProxy extends CommonProxy { public static String BLOCKS_PNG = "/tutorial/generic/block.png"; public static String ITEMS_PNG = "/tutorial/generic/items.png"; public static String FURNACE_PNG = "/tutorial/generic/furnace.png"; public static void registerRenderThings() { MinecraftForgeClient.preloadTexture(BLOCKS_PNG); MinecraftForgeClient.preloadTexture(ITEMS_PNG); MinecraftForgeClient.preloadTexture(FURNACE_PNG); } public int addArmor(String armor) { return RenderingRegistry.addNewArmourRendererPrefix(armor); } }
And just in case you need in since my ClientProxy is extending my CommonProxy here is the CommonProxy:
package tutorialtest; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.world.World; import cpw.mods.fml.common.network.IGuiHandler; public class CommonProxy implements IGuiHandler { public static void registerRenderThings() { } @Override public Object getServerGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { return null; } @Override public Object getClientGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { return null; } }
Im not sure if my CommonProxy is doing the wrong thing since my ClientProxy is connected to it or what. Everything I have in there should make it not say the error. If you know the error please help! Ive been getting this error for a while and my ore's are not generating and I have random furnaces generating in the wild. Not sure what the problem is! Hope you can help!
-
Do you need to make more than one set of tool classes for each different set? Or can you just use the one set of tool classes for all different tool sets?
-
Ok so good news I fixed it! In my Armor classes i had the wrong png files connected with it so it screwed it up!
-
Ok let me refresh you on the problem here. All im doing when adding the armor method is just so i dont have to use the modloader. So i can have my own method for adding armor. Thats not the problem, I know thats what the Thread says, but the real problem is whenever i put on my suit of armor only the leggings show. So if anyone knows why that is it would be great thanks!
-
Ok... I'm learning java, but I don't understand what ur point is. I thought learning java takes awhile and can be difficult well guess what it takes awhile. I thought people help people that have problems that's how u learn. Even if I watch all the videos I can I might still have an error I can't figure out. So I might as well ask someone else for help because your not. All I wanted to know is how to fix this simple problem but you guys can't help me unless I learn java.. so I guess I'm just gonna ask someone else and carry on looking for a way to solve this problem..
-
Here is the error when its not static:
Cannot make a static reference to the non-static method addArmor(String) from the type ClientProxy
-
diesieben07 read my main post at the top, it shows why I have to do that. It comes up as an error, like it wont let me put ClientProxy.addArmor unless the method is public static int not public int. Again I explained this in beginning post.
-
Ok.. Way back when, someone told me you want to make a ClientProxy class and CommonProxy class, also known as proxies. Now you would use these for alot of things. They would define the place where your 256x256 items/blocks png is stored. They can also be used for other things. Like in my last thread I had a problem with my armor and it would only show the leggings on my character. So diesieben07 told me one problem may be because in my mod_tutorial(main class) when i was doing the ModLoader.addArmor for all my armor that it had something to do with it. And he told me to make a method in the ClientProxy for the addArmor. Im just running into that problem so thats why i needed to know how to set up a proxy for armor's. Thing is that might not be the error.
Ok so you might be confused about all this. The point is the reason all of this started was because on my character when all the armor was on it only showed him with his leggings. So if you know any way to fix that it would be great.
mod_tutorial.class
package tutorialtest; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.Init; import cpw.mods.fml.common.event.FMLInitializationEvent; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.item.EnumArmorMaterial; import net.minecraft.item.EnumToolMaterial; import net.minecraft.item.Item; import net.minecraft.item.ItemSword; import cpw.mods.fml.common.network.NetworkMod; import cpw.mods.fml.common.registry.GameRegistry; import net.minecraft.item.ItemStack; import cpw.mods.fml.common.registry.LanguageRegistry; import net.minecraft.src.ModLoader; @Mod(modid = "ArmorTest-Tutorial", name = "Tutorial", version = "1.4.7") @NetworkMod(clientSideRequired = true, serverSideRequired = false) public class mod_tutorial { //blue public static Item TutorialHelmet; public static Item TutorialPlate; public static Item TutorialLegs; public static Item TutorialBoots; public static Item BlueIngot; public static Item BlueDust; public static Item BlueToolFragment; public static Item BlueSword; public static Item BluePickaxe; public static Item BlueSpade; public static Item BlueAxe; public static Block BlueOre; //yellow public static Item YellowHelmet; public static Item YellowPlate; public static Item YellowLegs; public static Item YellowBoots; public static Item YellowToolFragment; public static Item YellowIngot; public static Item YellowDust; public static Item YellowSword; public static Item YellowPickaxe; public static Item YellowSpade; public static Item YellowAxe; public static Block YellowOre; //White/Silver public static Item WhiteHelmet; public static Item WhitePlate; public static Item WhiteLegs; public static Item WhiteBoots; public static Item WhiteIngot; public static Item SilverIngot; public static Item WhitePowder; public static Item SilverDust; //red public static Item RedHelmet; public static Item RedPlate; public static Item RedLegs; public static Item RedBoots; public static Item RedIngot; public static Item RedToolFragment; public static Item RedDust; //green public static Item GreenHelmet; public static Item GreenPlate; public static Item GreenLegs; public static Item GreenBoots; public static Item GreenIngot; public static Item GreenToolFragment; public static Item GreenDust; //Pink public static Item PinkHelmet; public static Item PinkPlate; public static Item PinkLegs; public static Item PinkBoots; public static Item PinkIngot; public static Item PinkToolFragment; public static Item PinkDust; //Enum Materials //static EnumArmorMaterial TutorialEnumArmourMaterial = EnumHelper.addArmorMaterial("tutorial", 24, new int[] { 2, 5, 3, 2 }, 16); @Init public void load(FMLInitializationEvent event){ //Blue TutorialHelmet = new ItemTutorialHelmet(508,EnumArmorMaterial.BLUE, ClientProxy.addArmor("Tut"),0).setItemName("TutHelm").setIconIndex(0); TutorialPlate = new ItemTutorialPlate(509,EnumArmorMaterial.BLUE, ClientProxy.addArmor("Tut"),1).setItemName("TutPlate").setIconIndex(1); TutorialLegs = new ItemTutorialLegs(510,EnumArmorMaterial.BLUE, ClientProxy.addArmor("Tut"),2).setItemName("TutLeg").setIconIndex(2); TutorialBoots = new ItemTutorialBoots(511,EnumArmorMaterial.BLUE, ClientProxy.addArmor("Tut"),3).setItemName("TutBoot").setIconIndex(3); BlueIngot = new BlueIngot(532).setItemName("BlueIngot").setIconIndex(24); BlueToolFragment = new BlueToolFragment(533).setItemName("BlueToolFragment").setIconIndex(25); BlueSword = new BlueSword(534, EnumToolMaterial.BLUE).setItemName("BlueSword").setIconIndex(26); BluePickaxe = new BluePickaxe(535, EnumToolMaterial.BLUE).setItemName("BluePickaxe").setIconIndex(27); BlueSpade = new BlueSpade(536, EnumToolMaterial.BLUE).setItemName("BlueSpade").setIconIndex(28); BlueAxe = new BlueAxe(537, EnumToolMaterial.BLUE).setItemName("BlueAxe").setIconIndex(29); BlueDust = new BlueDust(538).setItemName("BlueDust").setIconIndex(30); BlueOre = new BlueOre(539, 0).setHardness(3.0F).setResistance(3.0F).setBlockName("BlueOre"); //Yellow YellowHelmet = new ItemYellowHelmet(512,EnumArmorMaterial.YELLOW, ModLoader.addArmor("Tutorial"),0).setItemName("YellowHelm").setIconIndex(4); YellowPlate = new ItemYellowPlate(513,EnumArmorMaterial.YELLOW, ModLoader.addArmor("Tutorial"),1).setItemName("YellowPlate").setIconIndex(5); YellowLegs = new ItemYellowLegs(514,EnumArmorMaterial.YELLOW, ModLoader.addArmor("Tutorial"),2).setItemName("YellowLeg").setIconIndex(6); YellowBoots = new ItemYellowBoots(515,EnumArmorMaterial.YELLOW, ModLoader.addArmor("Tutorial"),3).setItemName("YellowBoot").setIconIndex(7); YellowIngot = new YellowIngot(540).setItemName("YellowIngot").setIconIndex(31); YellowToolFragment = new YellowToolFragment(541).setItemName("YellowToolFragment").setIconIndex(32); YellowSword = new YellowSword(542, EnumToolMaterial.YELLOW).setItemName("YellowSword").setIconIndex(33); YellowPickaxe = new YellowPickaxe(543, EnumToolMaterial.YELLOW).setItemName("YellowPickaxe").setIconIndex(34); YellowSpade = new YellowSpade(544, EnumToolMaterial.YELLOW).setItemName("YellowSpade").setIconIndex(35); YellowAxe = new YellowAxe(545, EnumToolMaterial.YELLOW).setItemName("YellowAxe").setIconIndex(36); YellowDust = new YellowDust(546).setItemName("YellowDust").setIconIndex(37); YellowOre = new YellowOre(547, 1).setHardness(3.0F).setResistance(3.0F).setBlockName("YellowOre"); //White WhiteHelmet = new ItemWhiteHelmet(516, EnumArmorMaterial.DIAMOND, ModLoader.addArmor("Tutorial"),0).setItemName("WhiteHelm").setIconIndex(; WhitePlate = new ItemWhitePlate(517,EnumArmorMaterial.DIAMOND, ModLoader.addArmor("Tutorial"),1).setItemName("WhitePlate").setIconIndex(9); WhiteLegs = new ItemWhiteLegs(518,EnumArmorMaterial.DIAMOND, ModLoader.addArmor("Tutorial"),2).setItemName("WhiteLeg").setIconIndex(10); WhiteBoots = new ItemWhiteBoots(519,EnumArmorMaterial.DIAMOND, ModLoader.addArmor("Tutorial"),3).setItemName("WhiteBoot").setIconIndex(11); //Blue RedHelmet = new ItemRedHelmet(520, EnumArmorMaterial.RED, ModLoader.addArmor("Tutorial"),0).setItemName("RedHelm").setIconIndex(12); RedPlate = new ItemRedPlate(521,EnumArmorMaterial.RED, ModLoader.addArmor("Tutorial"),1).setItemName("RedPlate").setIconIndex(13); RedLegs = new ItemRedLegs(522,EnumArmorMaterial.RED, ModLoader.addArmor("Tutorial"),2).setItemName("RedLeg").setIconIndex(14); RedBoots = new ItemRedBoots(523,EnumArmorMaterial.RED, ModLoader.addArmor("Tutorial"),3).setItemName("RedBoot").setIconIndex(15); //Green GreenHelmet = new ItemGreenHelmet(524, EnumArmorMaterial.GREEN, ModLoader.addArmor("Tutorial"),0).setItemName("GreenHelm").setIconIndex(16); GreenPlate = new ItemGreenPlate(525,EnumArmorMaterial.GREEN, ModLoader.addArmor("Tutorial"),1).setItemName("GreenPlate").setIconIndex(17); GreenLegs = new ItemGreenLegs(526,EnumArmorMaterial.GREEN, ModLoader.addArmor("Tutorial"),2).setItemName("GreenLeg").setIconIndex(18); GreenBoots = new ItemGreenBoots(527,EnumArmorMaterial.GREEN, ModLoader.addArmor("Tutorial"),3).setItemName("GreenBoot").setIconIndex(19); //Pink PinkHelmet = new ItemPinkHelmet(528, EnumArmorMaterial.PINK, ModLoader.addArmor("Tutorial"),0).setItemName("PinkHelm").setIconIndex(20); PinkPlate = new ItemPinkPlate(529,EnumArmorMaterial.PINK, ModLoader.addArmor("Tutorial"),1).setItemName("PinkPlate").setIconIndex(21); PinkLegs = new ItemPinkLegs(530,EnumArmorMaterial.PINK, ModLoader.addArmor("Tutorial"),2).setItemName("PinkLeg").setIconIndex(22); PinkBoots = new ItemPinkBoots(531,EnumArmorMaterial.PINK, ModLoader.addArmor("Tutorial"),3).setItemName("PinkBoot").setIconIndex(23); //Register Blocks GameRegistry.registerBlock(BlueOre, "BlueOre"); GameRegistry.registerBlock(YellowOre, "YellowOre"); //blue GameRegistry.addRecipe(new ItemStack(mod_tutorial.TutorialHelmet,1), new Object[]{ "TTT","T T"," ",'T',Block.dirt }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.TutorialPlate,1), new Object[]{ "TTT","TTT","T T",'T',Block.dirt }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.TutorialLegs,1), new Object[]{ "TTT","T T","T T",'T',Block.dirt }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.TutorialBoots,1), new Object[]{ " ","T T","T T",'T',Block.dirt }); //Yellow GameRegistry.addRecipe(new ItemStack(mod_tutorial.YellowHelmet,1), new Object[]{ "TTT","T T"," ",'T',Block.dirt }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.YellowPlate,1), new Object[]{ "TTT","TTT","T T",'T',Block.dirt }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.YellowLegs,1), new Object[]{ "TTT","T T","T T",'T',Block.dirt }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.YellowBoots,1), new Object[]{ " ","T T","T T",'T',Block.dirt }); //white GameRegistry.addRecipe(new ItemStack(mod_tutorial.WhiteHelmet,1), new Object[]{ "TTT","T T"," ",'T',Block.dirt }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.WhitePlate,1), new Object[]{ "TTT","TTT","T T",'T',Block.dirt }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.WhiteLegs,1), new Object[]{ "TTT","T T","T T",'T',Block.dirt }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.WhiteBoots,1), new Object[]{ " ","T T","T T",'T',Block.dirt }); //red GameRegistry.addRecipe(new ItemStack(mod_tutorial.RedHelmet,1), new Object[]{ "TTT","T T"," ",'T',Block.dirt }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.RedPlate,1), new Object[]{ "TTT","TTT","T T",'T',Block.dirt }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.RedLegs,1), new Object[]{ "TTT","T T","T T",'T',Block.dirt }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.RedBoots,1), new Object[]{ " ","T T","T T",'T',Block.dirt }); //green GameRegistry.addRecipe(new ItemStack(mod_tutorial.GreenHelmet,1), new Object[]{ "TTT","T T"," ",'T',Block.dirt }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.GreenPlate,1), new Object[]{ "TTT","TTT","T T",'T',Block.dirt }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.GreenLegs,1), new Object[]{ "TTT","T T","T T",'T',Block.dirt }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.GreenBoots,1), new Object[]{ " ","T T","T T",'T',Block.dirt }); //pink GameRegistry.addRecipe(new ItemStack(mod_tutorial.PinkHelmet,1), new Object[]{ "TTT","T T"," ",'T',Block.dirt }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.PinkPlate,1), new Object[]{ "TTT","TTT","T T",'T',Block.dirt }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.PinkLegs,1), new Object[]{ "TTT","T T","T T",'T',Block.dirt }); GameRegistry.addRecipe(new ItemStack(mod_tutorial.PinkBoots,1), new Object[]{ " ","T T","T T",'T',Block.dirt }); //Blue LanguageRegistry.addName(TutorialHelmet, "Blue Helmet"); LanguageRegistry.addName(TutorialPlate, "Blue ChestPlate"); LanguageRegistry.addName(TutorialLegs, "Blue Leggings"); LanguageRegistry.addName(TutorialBoots, "Blue Boots"); LanguageRegistry.addName(BlueIngot, "Blue Ingot"); LanguageRegistry.addName(BlueToolFragment, "Blue-Tool-Fragment"); LanguageRegistry.addName(BlueSword, "Blue Sword"); LanguageRegistry.addName(BluePickaxe, "Blue Pickaxe"); LanguageRegistry.addName(BlueSpade, "Blue Spade"); LanguageRegistry.addName(BlueAxe, "Blue Axe"); LanguageRegistry.addName(BlueDust, "Blue Dust"); LanguageRegistry.addName(BlueOre, "Blue Ore"); //Yellow LanguageRegistry.addName(YellowHelmet, "Yellow Helmet"); LanguageRegistry.addName(YellowPlate, "Yellow ChestPlate"); LanguageRegistry.addName(YellowLegs, "Yellow Leggings"); LanguageRegistry.addName(YellowBoots, "Yellow Boots"); LanguageRegistry.addName(YellowIngot, "Yellow Ingot"); LanguageRegistry.addName(YellowToolFragment, "Yellow-Tool-Fragment"); LanguageRegistry.addName(YellowSword, "Yellow Sword"); LanguageRegistry.addName(YellowPickaxe, "Yellow Pickaxe"); LanguageRegistry.addName(YellowSpade, "Yellow Spade"); LanguageRegistry.addName(YellowAxe, "Yellow Axe"); LanguageRegistry.addName(YellowDust, "Yellow Dust"); LanguageRegistry.addName(YellowOre, "Yellow Ore"); //White LanguageRegistry.addName(WhiteHelmet, "White Helmet"); LanguageRegistry.addName(WhitePlate, "White ChestPlate"); LanguageRegistry.addName(WhiteLegs, "White Leggings"); LanguageRegistry.addName(WhiteBoots, "White Boots"); //Red LanguageRegistry.addName(RedHelmet, "Red Helmet"); LanguageRegistry.addName(RedPlate, "Red ChestPlate"); LanguageRegistry.addName(RedLegs, "Red Leggings"); LanguageRegistry.addName(RedBoots, "Red Boots"); //Green LanguageRegistry.addName(GreenHelmet, "Green Helmet"); LanguageRegistry.addName(GreenPlate, "Green ChestPlate"); LanguageRegistry.addName(GreenLegs, "Green Leggings"); LanguageRegistry.addName(GreenBoots, "Green Boots"); //Pink LanguageRegistry.addName(PinkHelmet, "Pink Helmet"); LanguageRegistry.addName(PinkPlate, "Pink ChestPlate"); LanguageRegistry.addName(PinkLegs, "Pink Leggings"); LanguageRegistry.addName(PinkBoots, "Green Boots"); //Ore Generation GameRegistry.registerWorldGenerator(new OreWorldGen()); } }
ClientProxy
package tutorialtest; import cpw.mods.fml.client.registry.RenderingRegistry; import net.minecraftforge.client.MinecraftForgeClient; public class ClientProxy extends CommonProxy { public static String BLOCKS_PNG = "/tutorial/generic/block.png"; public static String ITEMS_PNG = "/tutorial/generic/items.png"; public static void registerRenderThings() { MinecraftForgeClient.preloadTexture(BLOCKS_PNG); MinecraftForgeClient.preloadTexture(ITEMS_PNG); } public static int addArmor(String armor) { return RenderingRegistry.addNewArmourRendererPrefix(armor); } }
CommonProxy
package tutorialtest; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.world.World; import cpw.mods.fml.common.network.IGuiHandler; public class CommonProxy implements IGuiHandler { public static void registerRenderThings() { } @Override public Object getServerGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { return null; } @Override public Object getClientGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { return null; } public int addArmour(String armour) { return 0; } }
Picture of problem
Hope you can help Mazetar. If you dont understand im not sure if i can explain it any better, but still ask (basic)questions if needed
. Thanks!
-
I don't know what to write for my Armor Proxies. I need to make a method/string in the proxies so i can replace my modloader.addArmor with my proxies. I just dont know how to write them. If you need any current proxy script information just ask and ill give it to you. Hope you can help! Thanks!
Need Help Quickly!!
in Modder Support
Posted
diesieben07 the thing you wanted is right there ^^^^^^^!