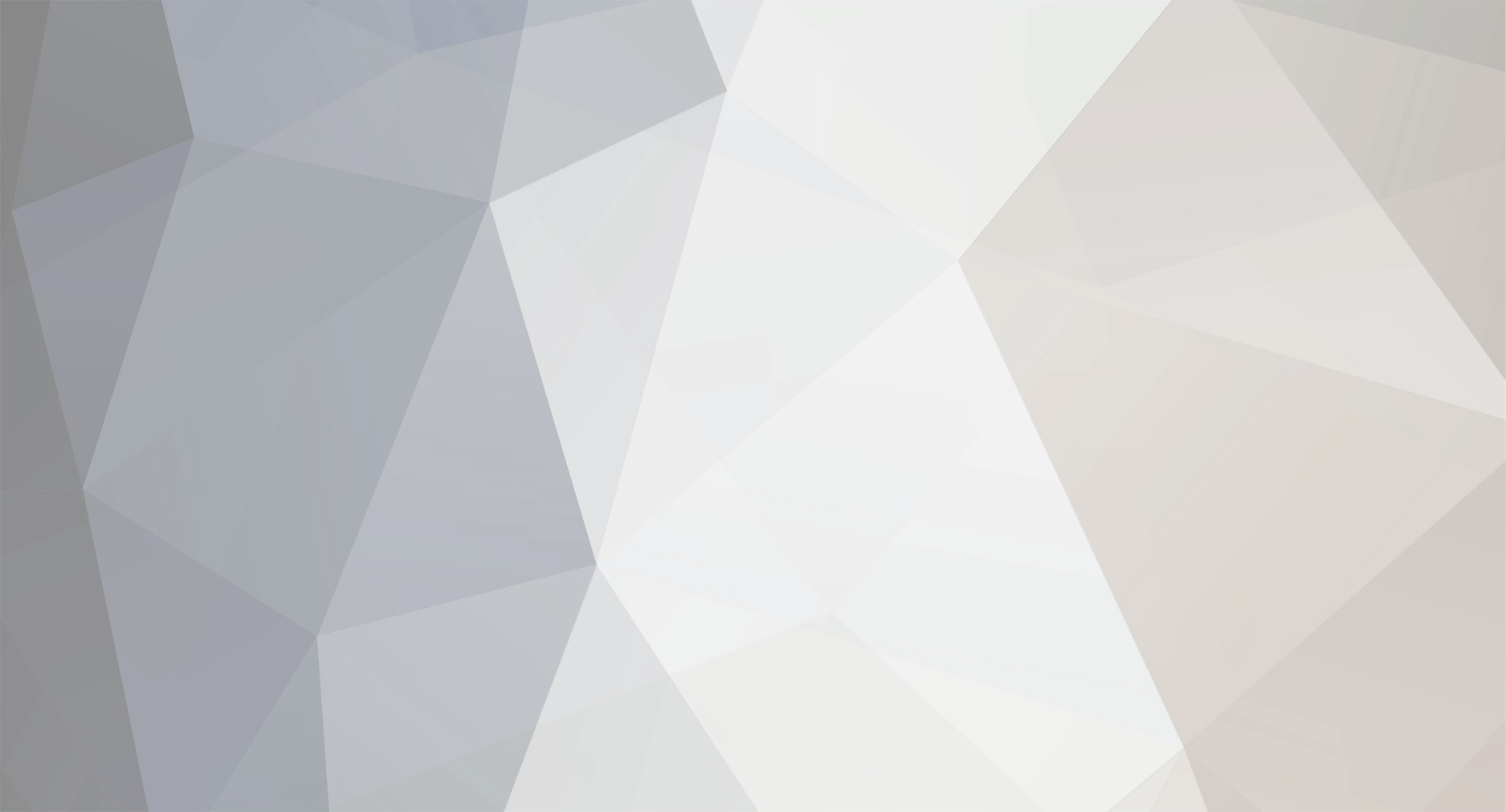
NullDev
Members-
Posts
64 -
Joined
-
Last visited
Everything posted by NullDev
-
I have created a block and added translucence to it using RenderTypeLookup to set its RenderType to RenderType.getTranslucent() but it doesn't render the faces of other blocks it touches. I am doing it like this: private void doClientStuff(final FMLClientSetupEvent event) { RenderType translucent = RenderType.getTranslucent(); RenderTypeLookup.setRenderLayer(RegistryHandler.PINK_SLIME_BLOCK.get(), translucent); } But it looks like this
-
[Solved] Custom Slime Block not working with Pistons
NullDev replied to NullDev's topic in Modder Support
Turns out it was a problem with IntelliJ. Not sure what, but I restarted it and everything is working fine now. -
[Solved] Custom Slime Block not working with Pistons
NullDev replied to NullDev's topic in Modder Support
https://github.com/AyliasTheCoder/The-Secrets-Of-BlocksDrawing -
[Solved] Custom Slime Block not working with Pistons
NullDev replied to NullDev's topic in Modder Support
Does this git repo generated by IntelliJ work? That's the only way I know how to make a git repo of it. .git.zip -
[Solved] Custom Slime Block not working with Pistons
NullDev replied to NullDev's topic in Modder Support
I have overriden both of them and nothing has changed. They both always return true. @Override public boolean isStickyBlock(BlockState state) { return true; } @Override public boolean canStickTo(BlockState state, BlockState other) { return true; } -
I have created a new slime block and it extends the SlimeBlock class, so why doesn't it work with pistons like a normal slime block does? It doesn't pull blocks with it, or bounce the player when it hits them being pushed by the piston. The player bounces fine when landing on it, but that is it. The Class: package com.nulldev.modbase.blocks; import net.minecraft.block.AbstractBlock; import net.minecraft.block.SlimeBlock; import net.minecraft.block.material.Material; import net.minecraft.entity.Entity; import net.minecraft.util.math.BlockPos; import net.minecraft.world.World; public class PinkSlimeBlock extends SlimeBlock { public PinkSlimeBlock() { super(AbstractBlock.Properties.create(Material.SPONGE).hardnessAndResistance(0, 1)); } @Override public void onFallenUpon(World worldIn, BlockPos pos, Entity entityIn, float fallDistance) { double x = entityIn.getMotion().getX(); double y = entityIn.getMotion().getY() * 1.75; double z = entityIn.getMotion().getZ(); entityIn.setMotion(x, y, z); super.onFallenUpon(worldIn, pos, entityIn, fallDistance); } } Edit: I have also tried adding this: @Override public boolean isSlimeBlock(BlockState state) { return true; } It changed nothing
-
How do I override textures?
-
I am now registering the colors using that event, but nothing has changed. I even have the event logging to the console to check that it is working. Blockstate: { "variants": { "bites=0": { "model": "tons_of_cakes:block/cake" }, "bites=1": { "model": "tons_of_cakes:block/cake_slice1" }, "bites=2": { "model": "tons_of_cakes:block/cake_slice2" }, "bites=3": { "model": "tons_of_cakes:block/cake_slice3" }, "bites=4": { "model": "tons_of_cakes:block/cake_slice4" }, "bites=5": { "model": "tons_of_cakes:block/cake_slice5" }, "bites=6": { "model": "tons_of_cakes:block/cake_slice6" } } } cake.json: { "parent": "block/cake_slice1", "textures": { "down": "tons_of_cakes:blocks/cake_bottom", "up": "tons_of_cakes:blocks/cake_top", "north": "tons_of_cakes:blocks/cake_side", "south": "tons_of_cakes:blocks/cake_side", "east": "tons_of_cakes:blocks/cake_side", "west": "tons_of_cakes:blocks/cake_inside" } } cake_slice1: { "parent": "block/cake_slice1", "textures": { "down": "tons_of_cakes:blocks/cake_bottom", "up": "tons_of_cakes:blocks/cake_top", "north": "tons_of_cakes:blocks/cake_side", "south": "tons_of_cakes:blocks/cake_side", "east": "tons_of_cakes:blocks/cake_side", "west": "tons_of_cakes:blocks/cake_inside" } } and the textures it is referencing are black and white versions of the vanilla textures, but it doesn't even show as black and white in game. It shows as the original vanilla texture.
-
I register an IBlockColor like this: Minecraft.getInstance().getBlockColors().register(new CakeColor(0xad6b15), block); //Cake color implements IBlockColor and its method The getColor method is setup like this: @Override public int getColor(BlockState p_getColor_1_, @Nullable IBlockDisplayReader p_getColor_2_, @Nullable BlockPos p_getColor_3_, int p_getColor_4_) { return color; // Returns the same color regardless of state } Yet the color of my cake still uses the base texture when placed on the ground. I need the color to change.
-
I am making a mod that adds cake variants, and I have the class and everything set up, but how do I make the new cakes copy the vanilla cake model?
-
I am creating a mod that adds souls to the game, and when you kill mobs, you get soul shards. Every 100 soul shards you get a soul. I want the number of souls a player has collected to be represented in a bar above the xp bar that has the same look, just a different color. How would I go about doing this?
-
I am trying to add new types of melons in 1.16, but I cannot figure out what classes to extend for the seeds, or how to register the stem block. Here's my code for the stem: public class BlueMelonStem extends StemBlock { public BlueMelonStem(Block b, Properties properties) { super((StemGrownBlock) b, properties); } }
-
Thanks that worked!
-
I want to detect when a player left clicks on a block, but onBlockClicked does nothing... Here's my current code: package com.nulldev.extremeskyblock.blocks; import com.nulldev.extremeskyblock.util.RegistryHandler; import net.minecraft.block.Block; import net.minecraft.block.BlockState; import net.minecraft.block.SoundType; import net.minecraft.block.material.Material; import net.minecraft.client.particle.ParticleManager; import net.minecraft.entity.item.ItemEntity; import net.minecraft.entity.player.PlayerEntity; import net.minecraft.item.ItemStack; import net.minecraft.util.math.BlockPos; import net.minecraft.util.math.RayTraceResult; import net.minecraft.world.World; import net.minecraftforge.common.ToolType; public class CompactStoneBlock extends Block { public CompactStoneBlock(Material material, Float hardness, Float resistance, SoundType soundType, int harvestLevel, ToolType toolType) { super(Properties.create(material) .hardnessAndResistance(hardness, resistance) .sound(soundType) .harvestLevel(harvestLevel) .harvestTool(toolType)); } @Override public void onBlockClicked(BlockState state, World worldIn, BlockPos pos, PlayerEntity player) { ItemEntity stonePebble = new ItemEntity(worldIn, pos.getX(), pos.getY() + 2, pos.getZ(), new ItemStack(RegistryHandler.STONE_PEBBLE.get(), 1)); } } But placing the block and clicking on it does nothing, and Intellij says that its deprecated but I cannot find anywhere what to use instead.