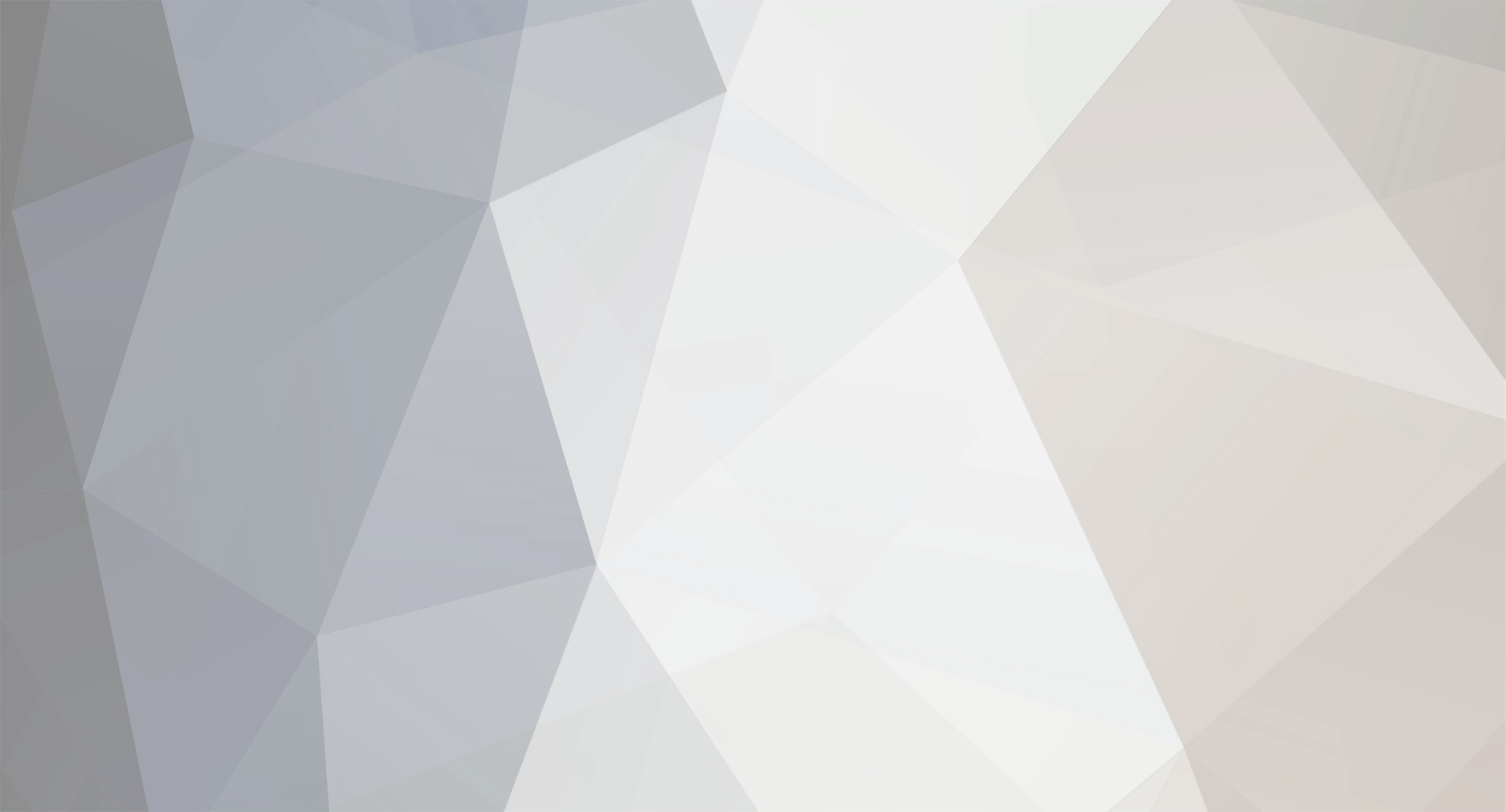
coalbricks
Members-
Posts
12 -
Joined
-
Last visited
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
coalbricks's Achievements

Tree Puncher (2/8)
1
Reputation
-
Oh yeah, you would probably want to store some custom NBT data on itemstacks then. You can figure out exactly what you need to store on the item, but at the very least it sounds like you would want to: 1. Store a custom NBT tag - call it `onKill` or something - that maps to a string. See the `StringTag` class and places where it's used. This string would probably be some command that would be run when the item is used to kill something - look at the `ExecuteCommand` class to see how to run an arbitrary command manually 2. Listen to `LivingDeathEvent` 3. In your event listener, determine if the causing entity is holding an item, if the item was used to kill, and if the item has your `onKill` tag 3. If all of this is true, get the string out of the `onKill` key and run the command. You will need to create an instance of `CommandSourceStack` to run the command, and it sounds like you will need to figure out exactly what the command source should be (i.e., who is executing the command?). You may also need to worry about the permission level of the thing running the command - again, see `ExecuteCommand` for a reference. 4. Get this working, and then add specialized behavior to do specific things as needed - for example, if it would be nontrivial to "add 1 to the sharpness enchantment" via commands, consider making this a custom action that you check for in your `LivingDeathEvent` handler. You should find that most things can be accomplished with vanilla commands - although you may want to extend your `onKill` tag and use a ListTag or an ArrayTag instead, and run commands in sequence. Hint: If you're doing this, you will want to learn about entity selectors, and if normal commands fail you, the /data command will usually save the day. You can do a whole lot with the data command. Another tip: Use the data command when debugging; it can be used to display all the NBT data on an entity. It can also be used to add NBT data to most entities, but the /give command is probably more convenient for you. You may also want to make a custom command that accepts an entity, item id, and greedy string, and gives the entity the item with `onKill` set to the string. Again, look at existing commands, and look at implementations of `ArgumentType` to see all the different kinds of arguments your command can accept. Almost all of what you need to know can be learned by looking through Minecraft's code and seeing how they do things. The rest can be learned by trying it out and seeing what happens. This is all very doable without any client-side modifications, which is great because it means more people will be able to use your cool mod on their servers.
-
Seeking Dedicated Contributors for a HUGE mod
coalbricks replied to IDoTheHax's topic in General Discussion
Because: 1. No money 2. Not my idea, if there was money offered then this wouldn't matter as much 3. I already have a job (and probably most other people on this forum) I was going to say that it sounds too ambitious but I checked out your repo and it looks like you've got a lot done. I wish you the best and I hope you make an amazing mod -
What are you trying to do exactly? I have a half-finished mod that lets you bind commands to weapons/tools and run them when you right-click with the item. The commands can be triggered by clicking on a block or entity an arbitrary distance away. All of this info is stored in the itemstack's NBT, and the only way to create these custom tools is with /give + NBT. Is that the kind of thing you want to make?
-
[1.12.2]How to send command when player join a game?
coalbricks replied to mlgmxyysd's topic in Modder Support
This is an old discussion, but after reading through it seems you've been unfair to @mlgmxyysd and wrong to assume that there are only two reasons why someone might want an offline mode server. I can give you a couple more valid and legitimate reasons: 1. The local network point is valid, and yes nobody can join from outside, but he could be running his server on a university network in which case custom authentication would be useful. It could be that nobody could join from outside, but 30 thousand people could join from inside. 2. He could be running his server on a network where Minecraft's authentication endpoint is blocked. -
[1.15.2] Change player name in Tab List (Player List)
coalbricks replied to Babatunde's topic in Modder Support
You've already done it, here: new SPlayerListItemPacket(SPlayerListItemPacket.Action.UPDATE_DISPLAY_NAME, (ServerPlayerEntity) player) A packet is just a bit of data that is sent between two or more computers over a network. Usually you don't have to manually send packets because Minecraft takes care of this for you for standard vanilla gameplay, but in this case I think it's the best solution. You can dig around the 'net.minecraft.network' or 'net.minecraft.network.play' packages to look at some example packet implementations; AFAIK there isn't any documentation on them. What IDE are you using? -
[1.15.2] Change player name in Tab List (Player List)
coalbricks replied to Babatunde's topic in Modder Support
It looks like you will need to use reflection twice. After you create the packet object, you will need to access SPlayerListItemPacket#players. SPlayerListItem#getEntries() only exists on the client, so you won't be able to use this. The elements of the list (of type SPlayerListItemPacket.AddPlayerData) contain a private 'displayName' member, which you will also need access to. (I would advise creating the Field objects in a static context instead of every EntityJoinWorldEvent, to save performance). Iterate through all the objects in the list and set their display names accordingly - although from your code it looks like there should only be one object in the list. -
You can use one of the subclasses of net.minecraftforge.event.entity.player.PlayerInteractEvent, some of which are fired both client and server side. The javadoc comments for each of these classes tell you on which sides the event will be fired.
-
Replying for future modders. net.minecraftforge.event.entity.player.PlayerInteractEvent does what you need. As of 1.16.1 there are several subclasses that the server fires when a player right or left clicks; the one you're looking for is probably PlayerInteractEvent.RightClickItem.
-
How to use Reflection to change vanilla values
coalbricks replied to JimiIT92's topic in Modder Support
1. It's a basic example. And that exception handling is fine for the purposes of being a basic example. It's also fine in production code in certain cases which I don't want to debate here, although I will admit the doStuff() function should have a null guard. 2. Yes while it would be better to store it in a static final field, the JVM optimizations obtained by making the member final are minimal at best, unless the field is being used to perform reflective gets/sets frequently. This pattern works perfectly fine in MOST cases but if you really care you can separate the static initializer logic into a separate function and make the field final; in most cases it's just not worth the trouble. -
[1.16.4] How do I make a block connect with adjacent blocks?
coalbricks replied to Linky132's topic in Modder Support
I took a look at RedstoneWireBlock and placed some dust in Minecraft to see what's up (you can press F3 and look at the dust to see properties). There's a RedstoneSide enum that can be either NONE, SIDE, or UP. Each side of the block (north, south, east, west) has an associated RedstoneSide value. These side properties are mapped to these enum values in a BlockState (field_235543_o_). Consider a block of redstone dust, Block A. If Block A was not surrounded by any blocks and the dust was pointing in all directions, the NORTH, SOUTH, EAST, and WEST properties would all be SIDE. If there was another redstone dust to the north of Block A, then Block A's NORTH and SOUTH properties would be SIDE, and its EAST and WEST properties would be NONE. If there was a solid block to the north of Block A with a dust on top, then Block A's NORTH property would be UP, its SOUTH property would be SIDE, and its EAST and WEST properties would be NONE. From a quick look it seems like func_235554_l_ builds a shape for each valid block state. You can probably look at the blocks adjacent to yours and set these properties accordingly, then use the properties to change the block state. -
How do I Update My mod From 1.16.3 To 1.16.4
coalbricks replied to OwenC9999's topic in Modder Support
Can you provide some details? What problems are you facing, what have you tried to fix them, etc... -
coalbricks joined the community
-
How to use Reflection to change vanilla values
coalbricks replied to JimiIT92's topic in Modder Support
Replying for future modders. ObfuscationReflectionHelper is perfectly fine if you use it efficiently. You can use it to get a Field object, which you can store in a static member. Example: public class Example { private static Field someField = null; static { try { someField = ObfuscationReflectionHelper.findField(SomeMinecraftClass.class, "field_12345_a"); // You need to pass the SRG name someField.setAccessible(true); } catch (Exception e) { e.printStackTrace(); } } public void doStuff(SomeMinecraftClass minecraftThing) { try { Object o = someField.get(minecraftThing); } catch (Exception e) { e.printStackTrace(); } } } I would also advise against passing the fully qualified class name as a String if you can help it. It's better to use the Class object directly if you can, and in most cases you can. You can find SRG names at http://mcpbot.bspk.rs/