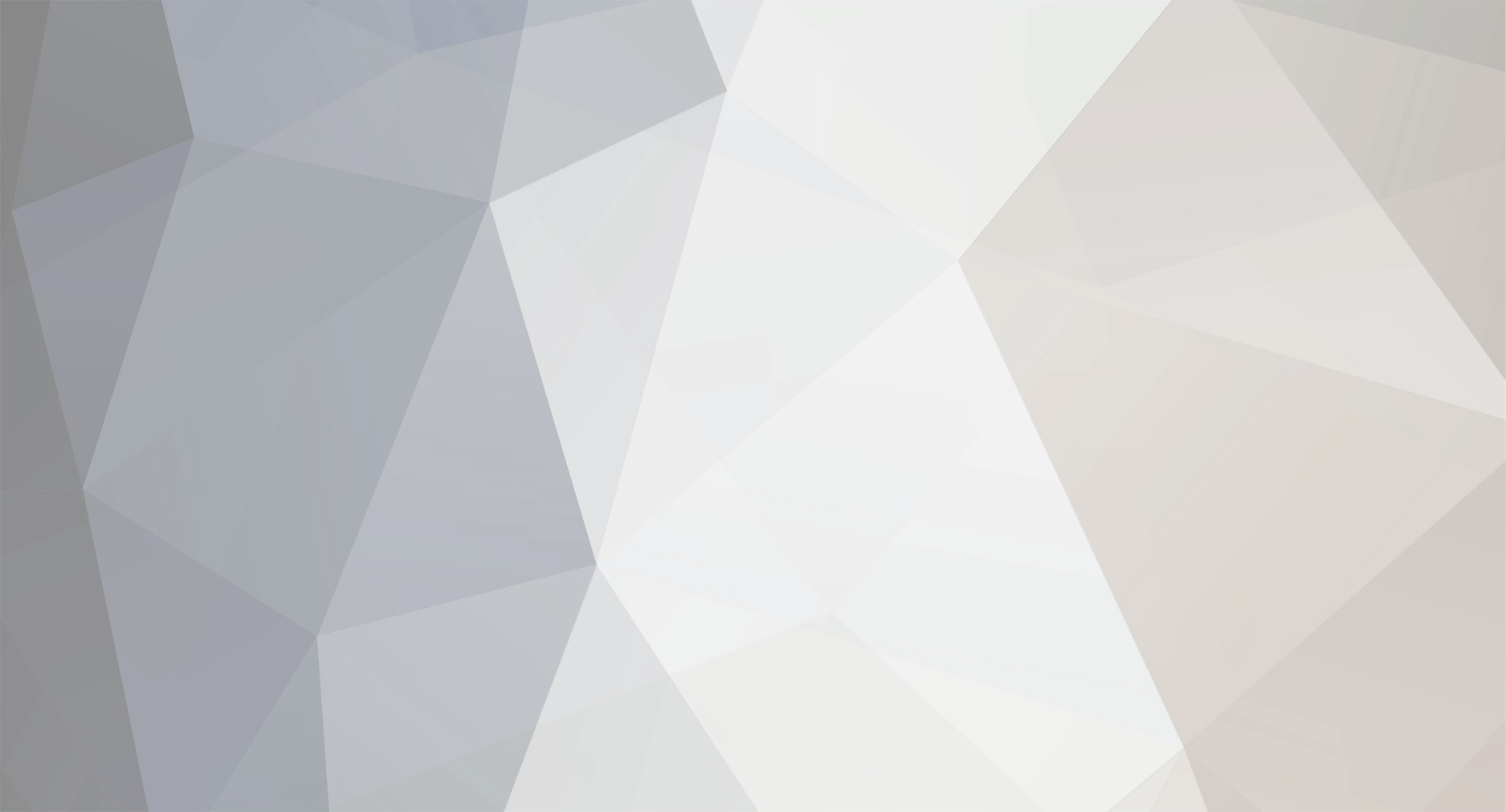
MCArTyR
Members-
Posts
29 -
Joined
-
Last visited
Everything posted by MCArTyR
-
Command class public class BruhCommand { public static void register(CommandDispatcher<CommandSourceStack> dispatcher){ dispatcher.register(Commands.literal("bruh").executes((command) -> { return execute(command); })); } private static int execute(CommandContext<CommandSourceStack> command){ if(command.getSource().getEntity() instanceof Player){ Player player = (Player) command.getSource().getEntity(); player.sendMessage(new TextComponent("bruh"), Util.NIL_UUID); } return Command.SINGLE_SUCCESS; } } Register command in RegisterCommands event @Mod.EventBusSubscriber(modid = ModMain.MODID, bus = Mod.EventBusSubscriber.Bus.FORGE) public class ModEventListener { @SubscribeEvent public static void registerCommands(RegisterCommandsEvent event){ BruhCommand.register(event.getDispatcher()); } }
-
I have this code to overlay block public static void drawOutline(PoseStack poseStack, VoxelShape shape, double x, double y, double z, BlockPos pos){ Matrix4f matrix4f = poseStack.last().pose(); Tesselator tesselator = Tesselator.getInstance(); BufferBuilder bufferIn = tesselator.getBuilder(); RenderSystem.setShader(GameRenderer::getPositionShader); RenderSystem.setShaderColor(0.0F, 0.0F, 0.25F, 1.0F); RenderSystem.disableTexture(); bufferIn.begin(VertexFormat.Mode.QUADS, DefaultVertexFormat.POSITION); shape.forAllEdges((x0, y0, z0, x1, y1, z1) -> { bufferIn.vertex(matrix4f, (float)(x0 + x), (float)(y0 + y), (float)(z0 + z)).color(1.0f, 0.0f, 0.0f, 1.0f).normal(0, 0, 0).endVertex(); bufferIn.vertex(matrix4f, (float)(x1 + x), (float)(y1 + y), (float)(z1 + z)).color(1.0f, 0.0f, 0.0f, 1.0f).normal(0, 0, 0).endVertex(); }); tesselator.end(); RenderSystem.setShaderColor(1.0F, 1.0F, 1.0F, 1.0F); RenderSystem.enableTexture(); } but i'm getting this result
-
Just entity (ItemEntity is here, but can be any) Entity with translucent overlay and white overline
-
Just block Block with overlay
-
overlay like redness during attack
-
I want to outline the entity, but not like the glowing effect. I want to make this outline a static color, not a white to black gradient. Also I want to make 2d overlay for entity. Render a translucent white over the rendered entity. Any ideas how this can be implemented?
-
My file encoding is utf-8, but i keep getting hieroglyphs. I need to do it without TranslatableComponent.
-
-
I'm sending command response But I don't get Russian letters in the chat. I get hieroglyphs.
-
I'm check ChatScreen, it has a "package" property commandSuggestions, it is automatically updated by other classes, but I couldn't figure out how
-
Now I can get the current input, but I still don't understand how to display my suggestion
-
How can i get current user message input (before sending) and display suggestion?
-
May i use ClientChatEvent if i want make command on client side only?
-
How to set custom command prefix (instead of "/") via Mojang brigadier, or using other options?
-
At the moment I ended up with this FrogMoveController: public class FrogMoveController extends MovementController { private float yRot; private int jumpDelay; private final FrogEntity frog; private boolean isAggressive; public FrogMoveController(FrogEntity p_i45821_1_) { super(p_i45821_1_); this.frog = p_i45821_1_; //this.yRot = 180.0F * p_i45821_1_.yRot / (float)Math.PI; } public void setWantedPosition(double p_75642_1_, double p_75642_3_, double p_75642_5_, double p_75642_7_) { this.wantedX = p_75642_1_; this.wantedY = p_75642_3_; this.wantedZ = p_75642_5_; this.speedModifier = p_75642_7_; if (this.operation != MovementController.Action.JUMPING) { this.operation = MovementController.Action.MOVE_TO; } } private boolean isWalkable(float p_234024_1_, float p_234024_2_) { PathNavigator pathnavigator = this.mob.getNavigation(); if (pathnavigator != null) { NodeProcessor nodeprocessor = pathnavigator.getNodeEvaluator(); if (nodeprocessor != null && nodeprocessor.getBlockPathType(this.mob.level, MathHelper.floor(this.mob.getX() + (double)p_234024_1_), MathHelper.floor(this.mob.getY()), MathHelper.floor(this.mob.getZ() + (double)p_234024_2_)) != PathNodeType.WALKABLE) { return false; } } return true; } public void tick() { if (this.operation == MovementController.Action.STRAFE) { float f = (float)this.mob.getAttributeValue(Attributes.MOVEMENT_SPEED); float f1 = (float)this.speedModifier * f; float f2 = this.strafeForwards; float f3 = this.strafeRight; float f4 = MathHelper.sqrt(f2 * f2 + f3 * f3); if (f4 < 1.0F) { f4 = 1.0F; } f4 = f1 / f4; f2 = f2 * f4; f3 = f3 * f4; float f5 = MathHelper.sin(this.mob.yRot * ((float)Math.PI / 180F)); float f6 = MathHelper.cos(this.mob.yRot * ((float)Math.PI / 180F)); float f7 = f2 * f6 - f3 * f5; float f8 = f3 * f6 + f2 * f5; if (!this.isWalkable(f7, f8)) { this.strafeForwards = 1.0F; this.strafeRight = 0.0F; } this.mob.setSpeed(f1); this.mob.setZza(this.strafeForwards); this.mob.setXxa(this.strafeRight); this.operation = MovementController.Action.WAIT; } else if (this.operation == MovementController.Action.MOVE_TO) { this.operation = MovementController.Action.WAIT; double d0 = this.wantedX - this.mob.getX(); double d1 = this.wantedZ - this.mob.getZ(); double d2 = this.wantedY - this.mob.getY(); double d3 = d0 * d0 + d2 * d2 + d1 * d1; float f9 = (float)(MathHelper.atan2(d1, d0) * (double)(180F / (float)Math.PI)) - 90.0F; this.mob.yRot = this.rotlerp(this.mob.yRot, f9, 90.0F); this.mob.setSpeed((float)(this.speedModifier * this.mob.getAttributeValue(Attributes.MOVEMENT_SPEED))); BlockPos blockpos = this.mob.blockPosition(); BlockState blockstate = this.mob.level.getBlockState(blockpos); Block block = blockstate.getBlock(); VoxelShape voxelshape = blockstate.getCollisionShape(this.mob.level, blockpos); if (this.mob.isOnGround()) { this.mob.setSpeed((float)(this.speedModifier * this.mob.getAttributeValue(Attributes.MOVEMENT_SPEED))); if (this.jumpDelay-- <= 0) { this.jumpDelay = this.frog.getJumpDelay(); if (this.isAggressive) { this.jumpDelay /= 3; } this.frog.getJumpControl().jump(); //playSound } else { this.mob.setSpeed(0.0F); } } else { this.mob.setSpeed((float)(this.speedModifier * this.mob.getAttributeValue(Attributes.MOVEMENT_SPEED))); } if (d2 > (double)this.mob.maxUpStep && d0 * d0 + d1 * d1 < (double)Math.max(1.0F, this.mob.getBbWidth()) || !voxelshape.isEmpty() && this.mob.getY() < voxelshape.max(Direction.Axis.Y) + (double)blockpos.getY() && !block.is(BlockTags.DOORS) && !block.is(BlockTags.FENCES)) { this.mob.getJumpControl().jump(); this.operation = MovementController.Action.JUMPING; } } else if (this.operation == MovementController.Action.JUMPING) { this.mob.setSpeed((float)(this.speedModifier * this.mob.getAttributeValue(Attributes.MOVEMENT_SPEED))); if (this.mob.isOnGround()) { this.operation = MovementController.Action.WAIT; } } else { this.mob.setZza(0.0F); } } } But sometimes during the jump, his head rotates 360 or even 720 degrees. And sometimes he jumps in the wrong direction.
-
up
-
My model has body, head and legFrontLeft, legFrontRight..., but legs and body are empty inside. In head alone, there are three elements. After /summon, Frog can only move one way, and cannot turn to go left, right, or back.
-
Now I have a problem like this: java.lang.NullPointerException: Cannot invoke "net.minecraft.client.renderer.entity.EntityRenderer.shouldRender(net.minecraft.entity.Entity, net.minecraft.client.renderer.culling.ClippingHelper, double, double, double)" because "entityrenderer" is null at net.minecraft.client.renderer.entity.EntityRendererManager.shouldRender(EntityRendererManager.java:238) ~[forge-1.16.5-36.1.0_mapped_official_1.16.5-recomp.jar:?] {re:classloading,pl:accesstransformer:B,pl:runtimedistcleaner:A} at net.minecraft.client.renderer.WorldRenderer.renderLevel(WorldRenderer.java:987) ~[forge-1.16.5-36.1.0_mapped_official_1.16.5-recomp.jar:?] {re:classloading,pl:runtimedistcleaner:A} at net.minecraft.client.renderer.GameRenderer.renderLevel(GameRenderer.java:608) ~[forge-1.16.5-36.1.0_mapped_official_1.16.5-recomp.jar:?] {re:classloading,pl:accesstransformer:B,pl:runtimedistcleaner:A} at net.minecraft.client.renderer.GameRenderer.render(GameRenderer.java:425) ~[forge-1.16.5-36.1.0_mapped_official_1.16.5-recomp.jar:?] {re:classloading,pl:accesstransformer:B,pl:runtimedistcleaner:A} at net.minecraft.client.Minecraft.runTick(Minecraft.java:976) ~[forge-1.16.5-36.1.0_mapped_official_1.16.5-recomp.jar:?] {re:classloading,pl:accesstransformer:B,pl:runtimedistcleaner:A} at net.minecraft.client.Minecraft.run(Minecraft.java:607) ~[forge-1.16.5-36.1.0_mapped_official_1.16.5-recomp.jar:?] {re:classloading,pl:accesstransformer:B,pl:runtimedistcleaner:A} at net.minecraft.client.main.Main.main(Main.java:184) ~[forge-1.16.5-36.1.0_mapped_official_1.16.5-recomp.jar:?] {re:classloading,pl:runtimedistcleaner:A} at jdk.internal.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:?] {} at jdk.internal.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:64) ~[?:?] {} at jdk.internal.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:?] {} at java.lang.reflect.Method.invoke(Method.java:564) ~[?:?] {} at net.minecraftforge.userdev.FMLUserdevClientLaunchProvider.lambda$launchService$0(FMLUserdevClientLaunchProvider.java:52) ~[forge-1.16.5-36.1.0_mapped_official_1.16.5-recomp.jar:?] {} at cpw.mods.modlauncher.LaunchServiceHandlerDecorator.launch(LaunchServiceHandlerDecorator.java:37) [modlauncher-8.0.9.jar:?] {} at cpw.mods.modlauncher.LaunchServiceHandler.launch(LaunchServiceHandler.java:54) [modlauncher-8.0.9.jar:?] {} at cpw.mods.modlauncher.LaunchServiceHandler.launch(LaunchServiceHandler.java:72) [modlauncher-8.0.9.jar:?] {} at cpw.mods.modlauncher.Launcher.run(Launcher.java:82) [modlauncher-8.0.9.jar:?] {} at cpw.mods.modlauncher.Launcher.main(Launcher.java:66) [modlauncher-8.0.9.jar:?] {} at net.minecraftforge.userdev.LaunchTesting.main(LaunchTesting.java:105) [forge-1.16.5-36.1.0_mapped_official_1.16.5-recomp.jar:?] {} FrogRenderer: public class FrogRenderer extends MobRenderer<FrogEntity, FrogModel<FrogEntity>> { protected static final ResourceLocation TEXTURE = new ResourceLocation(Main.MOD_ID, "textures/entity/frog"); public FrogRenderer(EntityRendererManager renderManagerIn) { super(renderManagerIn, new FrogModel<>(), 0.7F); } @Override public ResourceLocation getTextureLocation(FrogEntity p_110775_1_) { return TEXTURE; } }
-
I found that in logs [00:27:34] [modloading-worker-2/ERROR] [minecraft/GlobalEntityTypeAttributes]: Entity tutorial:frog has no attributes [00:27:34] [modloading-worker-2/ERROR] [minecraft/GlobalEntityTypeAttributes]: Entity tutorial:frog has no attributes [00:27:34] [modloading-worker-2/ERROR] [minecraft/GlobalEntityTypeAttributes]: Entity tutorial:frog has no attributes [00:27:34] [modloading-worker-2/ERROR] [minecraft/GlobalEntityTypeAttributes]: Entity tutorial:frog has no attributes [00:27:34] [modloading-worker-2/ERROR] [minecraft/GlobalEntityTypeAttributes]: Entity tutorial:frog has no attributes [00:27:34] [modloading-worker-2/ERROR] [minecraft/GlobalEntityTypeAttributes]: Entity tutorial:frog has no attributes [00:27:34] [modloading-worker-2/ERROR] [minecraft/GlobalEntityTypeAttributes]: Entity tutorial:frog has no attributes [00:27:34] [modloading-worker-2/ERROR] [minecraft/GlobalEntityTypeAttributes]: Entity tutorial:frog has no attributes [00:27:34] [modloading-worker-2/ERROR] [minecraft/GlobalEntityTypeAttributes]: Entity tutorial:frog has no attributes [00:27:34] [modloading-worker-2/ERROR] [minecraft/GlobalEntityTypeAttributes]: Entity tutorial:frog has no attributes [00:27:34] [modloading-worker-2/ERROR] [minecraft/GlobalEntityTypeAttributes]: Entity tutorial:frog has no attributes [00:27:34] [modloading-worker-2/ERROR] [minecraft/GlobalEntityTypeAttributes]: Entity tutorial:frog has no attributes [00:27:34] [modloading-worker-2/ERROR] [minecraft/GlobalEntityTypeAttributes]: Entity tutorial:frog has no attributes [00:27:34] [modloading-worker-2/ERROR] [minecraft/GlobalEntityTypeAttributes]: Entity tutorial:frog has no attributes [00:27:34] [modloading-worker-2/ERROR] [minecraft/GlobalEntityTypeAttributes]: Entity tutorial:frog has no attributes [00:27:34] [modloading-worker-2/ERROR] [minecraft/GlobalEntityTypeAttributes]: Entity tutorial:frog has no attributes [00:27:34] [modloading-worker-2/ERROR] [minecraft/GlobalEntityTypeAttributes]: Entity tutorial:frog has no attributes
-
Thats constructor with super() only. Here is the whole code, I removed only irrelevant methods public class FrogEntity extends AnimalEntity { public FrogEntity(EntityType<? extends AnimalEntity> type, World worldIn) { super(type, worldIn); } // << - 31 line public static AttributeModifierMap.MutableAttribute setCustomAttributes() { return MobEntity.createMobAttributes() .add(Attributes.MAX_HEALTH, 10.0) .add(Attributes.MOVEMENT_SPEED, 0.25); } @Nullable @Override public AgeableEntity getBreedOffspring(ServerWorld world, AgeableEntity p_241840_2_) { return RegistryHandler.FROG_ENTITY.get().create(world); } }