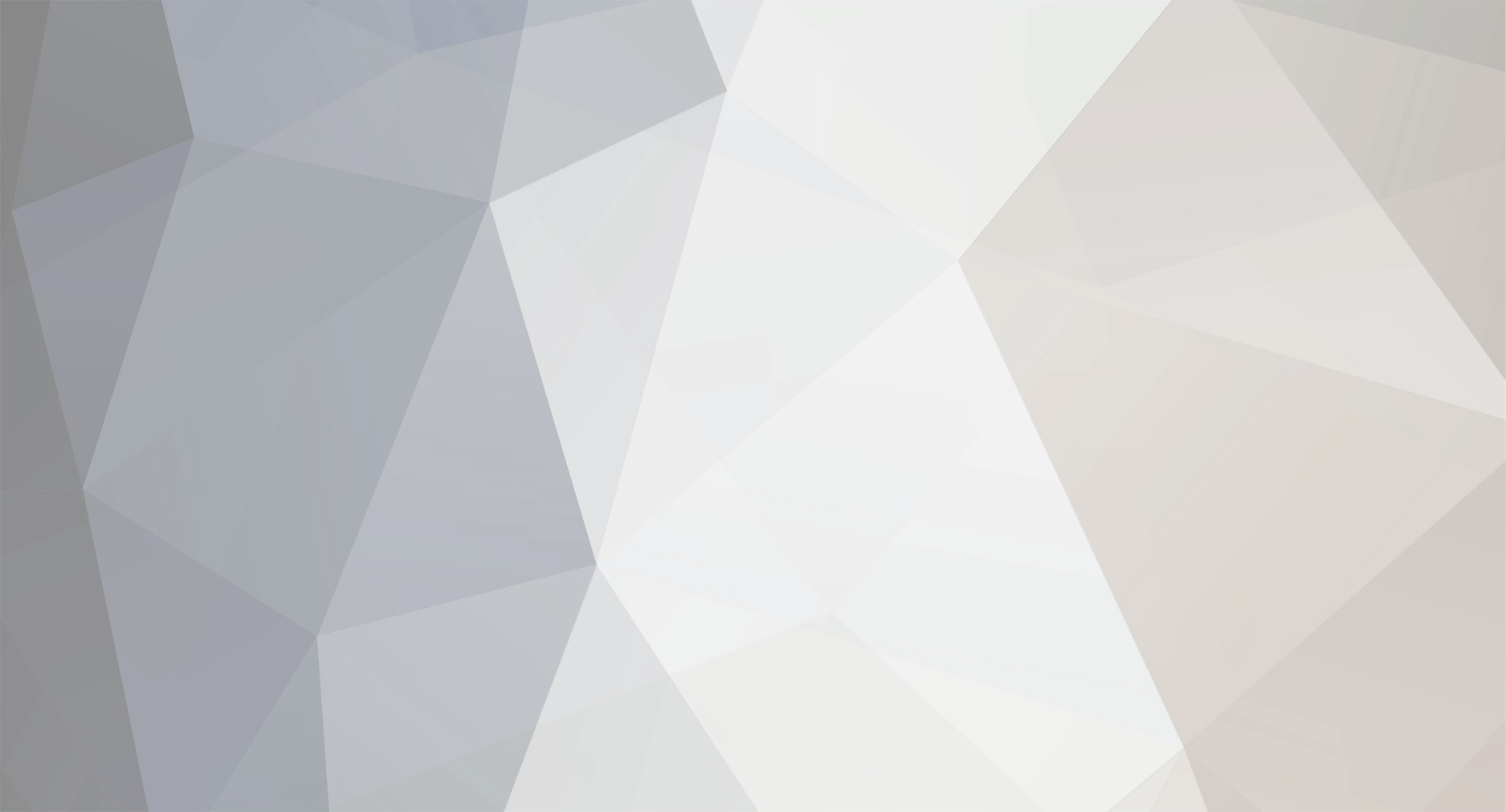
Squander
Members-
Posts
89 -
Joined
-
Last visited
Everything posted by Squander
-
First check if you are on the server side if(!level.isClientSide()). You don't need to check if the item in the player's main hand is the same as your item, you can use the last boolean variable, its name is isSelected. If isSelected is false then flag to false, if true check if(!flag) next add absorptions and set flag to true.
-
My custom DimensionSpecialEffects public class CustomEffects extends DimensionSpecialEffects { public CustomEffects() { super(Float.NaN, true, DimensionSpecialEffects.SkyType.NONE, false, true); } public Vec3 getBrightnessDependentFogColor(Vec3 p_108901_, float p_108902_) { return p_108901_; } public boolean isFoggyAt(int p_108898_, int p_108899_) { return true; } @Nullable public float[] getSunriseColor(float p_108888_, float p_108889_) { return null; } }
-
@SubscribeEvent public static void onAttachToEntity(AttachCapabilitiesEvent<Entity> event){ if(event.getObject() instanceof Player player){ event.addCapability(Helper.createRL("player_data"), new PlayerData(player)); } } public PlayerData(Player player) { this.player = player; this.setCurrentEnergy(this.getMaxEnergy()); } public int getMaxEnergy() { return (int) this.player.getAttributeValue(AttributeInit.MAX_ENERGY.get()); } and console log https://gist.github.com/MatyBotStorage/138473d7c4c7a43ad4b453baa4fda529
-
As in the topic, I am using this code. The button text is obscured by the tooltip but the button itself is not, I don't know how to fix it. public void renderButton(PoseStack poseStack, int pMouseX, int pMouseY, float pPartialTick) { Minecraft mc = Minecraft.getInstance(); int k = this.getYImage(this.isHovered); ScreenUtils.blitWithBorder(poseStack, WIDGETS_LOCATION, this.x, this.y, 0, 46 + k * 20, this.width, this.height, 200, 20, 2, 3, 2, 2, this.getBlitOffset()); this.renderBg(poseStack, mc, pMouseX, pMouseY); Component buttonText = this.getMessage(); int strWidth = mc.font.width(buttonText); int ellipsisWidth = mc.font.width("..."); if (strWidth > width - 6 && strWidth > ellipsisWidth) buttonText = Component.literal(mc.font.substrByWidth(buttonText, width - 6 - ellipsisWidth).getString() + "..."); drawCenteredString(poseStack, mc.font, buttonText, this.x + this.width / 2, this.y + (this.height - 8) / 2, getFGColor()); if(this.isHoveredOrFocused()){ this.renderToolTip(poseStack, pMouseX, pMouseY); } }
-
[1.19.2] Write and read list by FriendyByteBuffer
Squander replied to Squander's topic in Modder Support
Thanks -
As in the topic, I need to send a package and send a list with my object but I don't know how to do it.
-
Oh, ok
-
So what should I do to get the current value
-
Values in init always returning 0, this only applies to menu references
-
Well I created an EnergyWidget in init, in the constructor calls this.menu.getEnergy(), this.menu.getMaxEnergy(), but I don't know why they return 0 all the time, but when I call these methods in renderBg method then they return correct values. @Override protected void init() { super.init(); this.xPos = (width - imageWidth) / 2; this.yPos = (height - imageHeight) / 2; this.addRenderableWidget(new EnergyWidget(this, this.menu.getEnergy(), this.menu.getMaxEnergy(), WIDGETS, this.xPos + 125, this.yPos + 20)); } @Override protected void renderBg(PoseStack poseStack, float pPartialTick, int pMouseX, int pMouseY) { ClientHelper.renderStuff(TEXTURE); this.blit(poseStack, this.xPos, this.yPos, 0, 0, imageWidth, imageHeight); if(this.menu.isWorking()){ int progress = this.menu.getFuelProgress(); this.blit(poseStack, this.xPos + 81, this.yPos + 31 + 12 - progress, 176, 12 - progress, 14, progress + 1); } }
-
Helper.getRc returns a new ResourceLocation instance with mod id
-
My texture path is assets/apocaalypse/textures/entity/chest/norma.png and normal_lef.png and normal_right.png And I changed the military chest render class to vanilla event.registerBlockEntityRenderer(BlockEntityInit.MILITARY_CHEST.get(), ChestRenderer::new);
-
Well i don't see this method but i did something like this and it still doesn't work. public class MilitaryChestRender extends ChestRenderer<MilitaryChestBlockEntity> { public static final Material CHEST_LOCATION = chestMaterial("normal"); public static final Material CHEST_LOCATION_LEFT = chestMaterial("normal_left"); public static final Material CHEST_LOCATION_RIGHT = chestMaterial("normal_right"); public MilitaryChestRender(BlockEntityRendererProvider.Context pContext) { super(pContext); } @Override protected Material getMaterial(MilitaryChestBlockEntity blockEntity, ChestType chestType) { return chooseMaterial(chestType, CHEST_LOCATION, CHEST_LOCATION_LEFT, CHEST_LOCATION_RIGHT); } public static Material chooseMaterial(ChestType pChestType, Material pDoubleMaterial, Material pLeftMaterial, Material pRightMaterial) { return switch (pChestType) { case LEFT -> pLeftMaterial; case RIGHT -> pRightMaterial; default -> pDoubleMaterial; }; } public static Material chestMaterial(String chestName) { return new Material(Sheets.CHEST_SHEET, Helper.getRc("textures/entity/chest/" + chestName)); } }
-
Okay, it worked, but I'm wondering how I can change the texture of the chest.
-
Hi. I create a custom chest, but neither a block not an inventory item is rendered.
-
i did something like this and it works too. @Override public boolean isItemValid(int slot, @NotNull ItemStack stack) { for (int i = 0; i < getSlots(); i++) { ItemStack stack1 = getStackInSlot(i); if(stack.getItem() == stack1.getItem()){ return false; } } return super.isItemValid(slot, stack); }
-
Ok it works, but i don't know how i can prevent amulet of the same kind from being added to a container
-
I'm not sure if I did it right if(player != null){ if(getStackInSlot(slot).isEmpty()){ ((BaseAmulet)getStackInSlot(slot).getItem()).remove(player); } if(getStackInSlot(slot).getItem() instanceof BaseAmulet amulet){ amulet.apply(player); } }
-
I don't think it will work. I want to do it in a similar way to the inventory when I put my boots on, the attributes from it are added to the player when I take them off they are removed.
-
public class ItemStackHandlerMod extends ItemStackHandler { private Player player; public ItemStackHandlerMod(int size) { super(size); } public ItemStackHandlerMod(int size, Player player) { super(size); this.player = player; } @Override protected void onContentsChanged(int slot) { if(player != null){ if(getStackInSlot(slot).getItem() instanceof BaseAmulet amulet){ amulet.apply(player); } } } } public class HealthAmulet extends BaseAmulet { public static final UUID HEALTH = UUID.fromString("c2d8616a-c58a-4d99-aec9-faad03858302"); public HealthAmulet(Properties pProperties) { super(pProperties); } @Override public void apply(Player player) { AttributeInstance instance = player.getAttribute(Attributes.MAX_HEALTH); instance.addPermanentModifier(new AttributeModifier(HEALTH, "Health Amulet", 5, AttributeModifier.Operation.ADDITION)); } @Override public void remove(Player player) { AttributeInstance instance = player.getAttribute(Attributes.MAX_HEALTH); instance.removeModifier(HEALTH); } }
-
Overrides the onContentChanged method. When I put an item, it calls the apply method, but I would like to call remove when I take item out of the container, but I don't think onContentChanged will let me do that.
-
public class MineSkillSyncPacket { private int level; public MineSkillSyncPacket(int level){ this.level = level; } public MineSkillSyncPacket(FriendlyByteBuf buf){ new MineSkillSyncPacket(buf.readVarInt()); } public void encode(FriendlyByteBuf buf){ buf.writeVarInt(this.level); } public boolean handler(Supplier<NetworkEvent.Context> supplier){ NetworkEvent.Context ctx = supplier.get(); ctx.enqueueWork(() -> { MineSkillData.setLevel(this.level); }); return true; } public static void register(int id){ PacketHandler.INSTANCE.messageBuilder(MineSkillSyncPacket.class, id, NetworkDirection.PLAY_TO_CLIENT) .decoder(MineSkillSyncPacket::new) .encoder(MineSkillSyncPacket::encode) .consumer(MineSkillSyncPacket::handler).add(); } } This is my packet, I have to send it from the server to the client, but I don't know where to call the sending methods.
-
I should send the packet from server to client or client to server and what it should contain?
-
I do not send any packets, what, where and what should be included if should.
-
I get such an error in the console and am using this code. @SubscribeEvent public static void onMineBlock(PlayerEvent.BreakSpeed event){ event.getPlayer().getCapability(Capabilities.PLAYER_SKILLS).ifPresent(skills -> { for(AbstractSkill skill : skills.getSkills()){ if(skill instanceof MineSkill){ event.setNewSpeed(event.getOriginalSpeed() * skill.getLevel() + 0.5f); System.out.println("ORIGINAL: " + event.getOriginalSpeed()); System.out.println("NEW: " + event.getNewSpeed()); } } }); } [Server thread/WARN] [net.minecraft.server.level.ServerPlayerGameMode/]: Mismatch in destroy block pos: BlockPos{x=0, y=0, z=0} BlockPos{x=16, y=94, z=13}