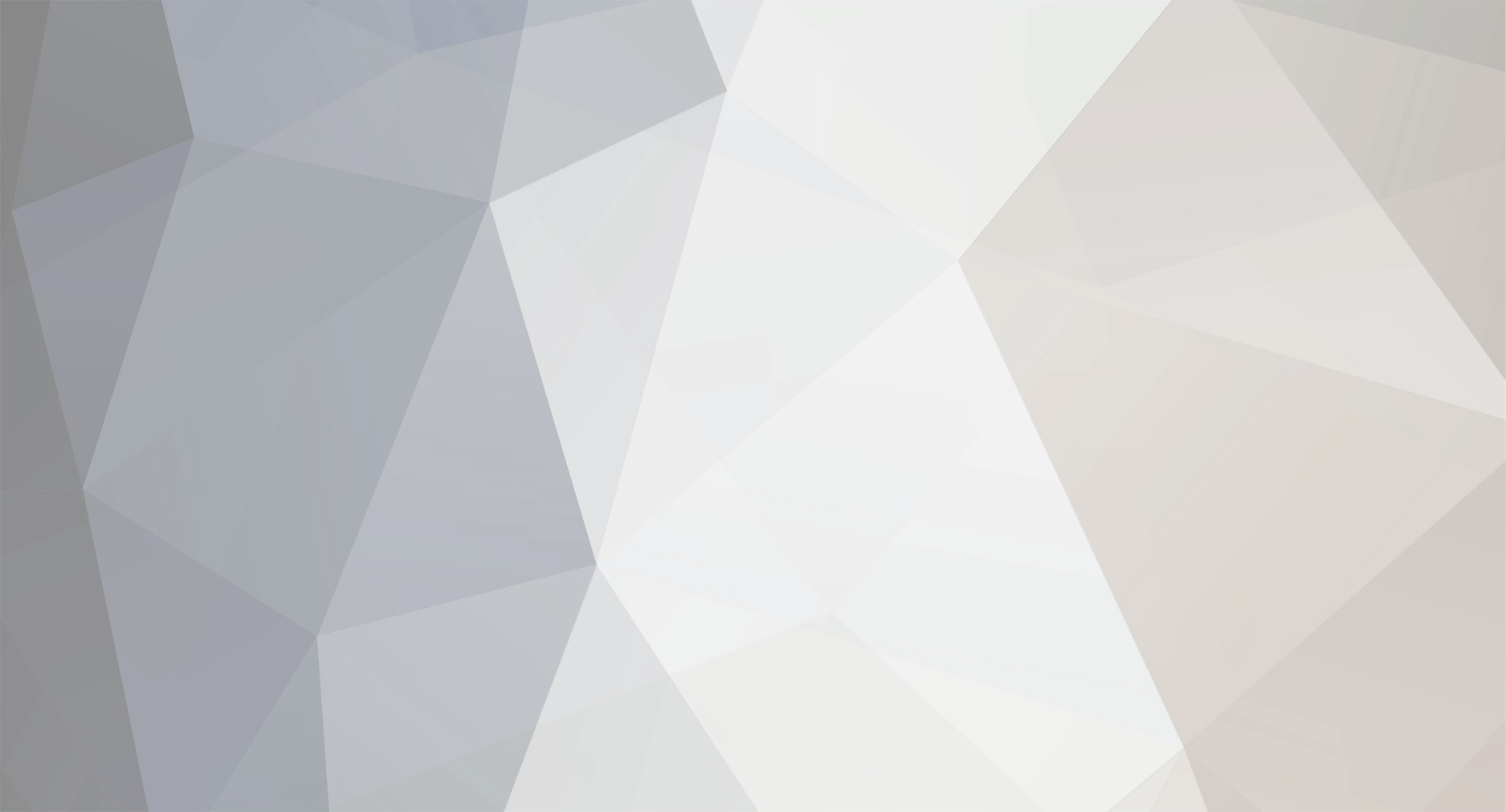
Lycanus Darkbinder
Members-
Posts
118 -
Joined
-
Last visited
Everything posted by Lycanus Darkbinder
-
Forging a Minecraft Mod (video tutorials)
Lycanus Darkbinder replied to Lycanus Darkbinder's topic in Modder Support
Unlocked to ask @TheGreyGhost a question... The link has shown up on the tutorial listing as ForgingAModLectureSeries, I think because there were no underscores used in the page name: "Forging_A_Mod_Lecture_Series" like the other links / pages use. When I tried to edit the list, none of the entries are available in the edit box. Is there any way we can fix the way it appears in the list so it says "Forging a Minecraft Mod - Lecture Series" instead of "ForgingAModLectureSeries"? Edit: The page coolAlias posted also looks that way, all jammed together. -
Forging a Minecraft Mod (video tutorials)
Lycanus Darkbinder replied to Lycanus Darkbinder's topic in Modder Support
@coolAlias Thanks but this wasn't intended to be a thread for collecting links. Locking so it doesn't get out of hand. -
Forging a Minecraft Mod (video tutorials)
Lycanus Darkbinder replied to Lycanus Darkbinder's topic in Modder Support
Thanks, I updated it to include extra info about the version of Minecraft used in the series and slightly more detail about the topics covered. By the way, I added the tag for Beginner and Advanced but I don't see them on the finished page, they showed up in the preview though. PS: I've always had trouble editing wikis on various sites, I think I have a rogue Firefox plugin that doesn't play well with certain API. -
Preface: I apologize if this is not the proper forum however I was unable to create a page on the wiki in the Tutorials subforum (no button of any kind to add topics and info). Details: is a playlist of 12 videos by someone by the name of Vidar Swenning (vswe). Each video runs between 40 minutes to 1 hour and is presented in a "classroom" style format. The playlist starts off with a "Getting Started" video and progresses to advanced topics such as Entities and World Generation. I am new to Forge and started with TileEntities which admittedly was not a beginner topic. I found these videos in my search for information and thought they'd be worth sharing. To the moderator: Again, sorry if this doesn't belong here but I thought it a better fit than "General" since it is related to modding and the videos are very high quality and informative. PS: No, I am not affiliated with the videos or their creator.
-
The OP is using that event. I think the problem is that those objects have not been set yet when he / she is trying to access them. It's similar to how you can't access worldObj in the constructor of a TileEntity because it does not get set until after the constructor.
-
getIcon() and getBlockTexture() use sides because of the multi-texture capability of blocks. You can get around this pretty simply though by incrementing the side and storing the result in an array. Note: In your case you really want getBlockTexture() which is called for blocks in the world, getIcon() is called for blocks in your inventory and Creative tabs. Here's some rough code: Icon blockTextures[6]; // All blocks have 6 sides int blockID = 0; // you will need to get this by adapting my hint above for (int side = 0; side < blockTextures.length; side++) { blockTextures[side] = Block.blocksList[blockID].getBlockTexture(IBlockAccess, x, y, z, side); } Then when you override getBlockTexture() for your block you can return an array element based on the side the function is requesting. If you don't want to mess with arrays, you could concievably just return the Icon from the block next to yours by using the opposite side. If the game is asking for (side=1) of your block then it would be (side=0) of the neighboring block that is touching your block so return its Icon instead.
-
Even simpler, you could create a TileEntity when the block is placed and simply store the Icon of the block next to it. Then override getBlockTexture() and return the icon stored in the TileEntity. Hint: int ID = world.getBlockId(x, y+1, z); // change this as needed, maybe x+1, y-1, etc. int meta = world.getBlockMetadata(x, y+1, z); // change this as needed, maybe x+1, y-1, etc. int side = 0; // change this as needed // Icon ico = Block.blocksList[iD].getIcon(side, meta); // Use for blocks in hand / Creative tab Icon ico = Block.blocksList[iD].getBlockTexture(IBlockAccess, x, y, z, side); // Use for blocks placed in world Edit: modified example to include getBlockTexture()
-
[Solved] How to get Object from Itemstack
Lycanus Darkbinder replied to 1n5aN1aC's topic in Modder Support
Don't try to cast the result of getCurrentItemOrArmor(), that's an ItemStack, you want to use getItem() on the ItemStack returned and then cast that... Here it is in long format: ItemStack stack = something.getCurrentItemOrArmor(x); Item tmp = stack.getItem(); MyObject obj = (MyObj)tmp; So to be concise you can simply write: MyObject obj = (MyObj)something.getCurrentItemOrArmor(x).getItem(); if (obj != null) { // do stuff } else { // oops } -
[Solved] How to get Object from Itemstack
Lycanus Darkbinder replied to 1n5aN1aC's topic in Modder Support
Since getCurrentItemOrArmor() returns an ItemStack which has a method called getItem(), you need to cast the result of that into your custom object (which must either extend or implement Item): MyObject obj = (MyObject)object.getCurrentItemOrArmor(X).getItem(); if (obj != null) obj.myCustomFunction(); -
Yes, those two code blocks are essentially the same. Some people prefer to not cast from one class to the other so pick whichever fits your code style. As for the newest crash, you should check if the world is null before trying to use it. It's possible that the world field in EntityJoinWorldEvent() hasn't been set before your override gets called. Also, try using event.entity.worldObj and see if that is null.
-
Hmm, can you try checking if the player object is valid? EntityPlayer player = (EntityPlayer) event.entity; if (player != null) { // do stuff } else { // oops } Another thing you could try is just using the entity itself since EntityJoinWorldEvent has a public field called world if (event.entity instanceof EntityPlayer) { event.entity.world; } else { // oops }
-
Checking if a vanilla keybind is pressed
Lycanus Darkbinder replied to chimera27's topic in Modder Support
This is what I use in my SmartLights mod that I've been working on, to see if the player is sneaking: int sneak = Minecraft.getMinecraft().gameSettings.keyBindSneak.keyCode; if (Keyboard.isKeyDown(sneak)) { // Do stuff } Note: I use it in onBlockActivated() so I have access to a World object for checking isRemote to avoid crashing. I'm not sure if it's necessary since the Keyboard object is from the library lwjgLinput which should be loaded by the server too. I haven't tested my mod in MP yet. -
Are you sure it's line 30? You're probably getting a NULL for the World World world = player.getEntityWorld(); NBTTagCompound nbt = world.getWorldInfo().getPlayerNBTTagCompound(); By the way, what version of Minecraft are you writing for? Can't you just use player.worldObj?
-
How do I debug a server side mod?
Lycanus Darkbinder replied to Lycanus Darkbinder's topic in Modder Support
Thanks for the advice, will check my code when I get a few minutes. -
These are some utility functions I wrote but have not tested in multiplayer (can't get Eclipse to launch the server). They do work for singleplayer however. public boolean isOp (String playerName) { // Check if multiplayer and OP or singleplayer and commands allowed (cheats) return MinecraftServer.getServer().getConfigurationManager().areCommandsAllowed(playerName); } public boolean isOp (EntityPlayer player) { return isOp(player.getEntityName()); } public boolean isOp (EntityLiving entity) { if (entity instanceof EntityPlayer) return isOp(entity.getEntityName()); else return false; }
-
Note: I didn't post this in Modder support because it isn't a Java "school" as the description says. At any rate, I was trying to set a boolean flag based on the dimension name but found that in this particular code snippet isNether always equals false. // Assuming dimID is -1 String dimName = DimensionManager.getProvider(dimID).getDimensionName().toLowerCase(); boolean isNether = (dimName == "nether"); If I hover over dimName in Eclipse it says nether and the byte array associated with it is 6 characters so there doesn't appear to be any newline character at the end. Note: I am using equalsIgnoreCase("nether") but I'm curious why toLowerCase() doesn't work.
-
[Solved] Overriding getLightValue() has no effect
Lycanus Darkbinder replied to Lycanus Darkbinder's topic in Modder Support
Once I got the TileEntity working properly there was no need to cache the worlds. The client TileEntity contains the same data as the server TileEntity which makes getLightValue() work as expected. -
How do I debug a server side mod?
Lycanus Darkbinder replied to Lycanus Darkbinder's topic in Modder Support
@Axlen Thanks but I was looking to be able to test without having to compile, reobfuscate, etc. @Mew Right, that's when it crashes. As soon as I launch the server from within Eclipse it throws the NoClassDefFoundError about "WorldClient" that I mentioned earlier. It is pointing to my mod's base file at the line that creates the "new BlockSmartLight()". Strange thing is that in my constructor I don't do anything except set the creative tab. -
How do I debug a server side mod?
Lycanus Darkbinder replied to Lycanus Darkbinder's topic in Modder Support
That may be true, not sure myself. I technically don't want to debug the server. What I want to do is debug my mod while connected to a server. Problem is I can't start the server through Eclipse and if I start the server with "startserver.bat" (in the MCP folder) and then run the client through Eclipse it can't connect due to "missing mods". I don't see how I could be missing mods since the MCP server only runs Forge which is also what's running in Eclipse. -
How do I debug a server side mod?
Lycanus Darkbinder replied to Lycanus Darkbinder's topic in Modder Support
I know this is an old topic but it's still relevant since I actually took a break from modding. I'm unable to get the server running in Eclipse because in my modLoad() function it crashes when it tries to create an instance of my block: -
Thanks for the help everyone, this did the trick: @Override public Packet getDescriptionPacket() { // The server calls this function and if we return the NBT filled // with data, this.onDataPacket() will be called for the client with // the server's NBT info NBTTagCompound tag = new NBTTagCompound(); this.writeToNBT(tag); return new Packet132TileEntityData(this.xCoord, this.yCoord, this.zCoord, 1, tag); } @Override public void onDataPacket(INetworkManager net, Packet132TileEntityData pkt) { // PKT contains an NBTTagCompound with the server's TileEntity info. // We can parse that into our local variables so we stay in sync when our various // "get" methods are called to access TileEntity data. this.readFromNBT(pkt.customParam1); }
-
Thanks for that. It's still not clicking in my brain how that all works. createTileEntity() has to be called for the client because it doesn't exist yet. This is where my disconnect comes in. I don't understand how those packet functions tell the server to give me a copy of it's TileEntity so I can stuff the data into the client version. TileEntity.getDescriptionPacket() only gets called for server TileEntities (worldObj.isRemote == false) and TileEntity.onDataPacket() never got called at all. edit: oops, I was returning null from getDescriptionPacket() instead of my actual packet... I'm sure it's one of those "why didn't I think of that" moments when it clicks but right now it's just eluding me.