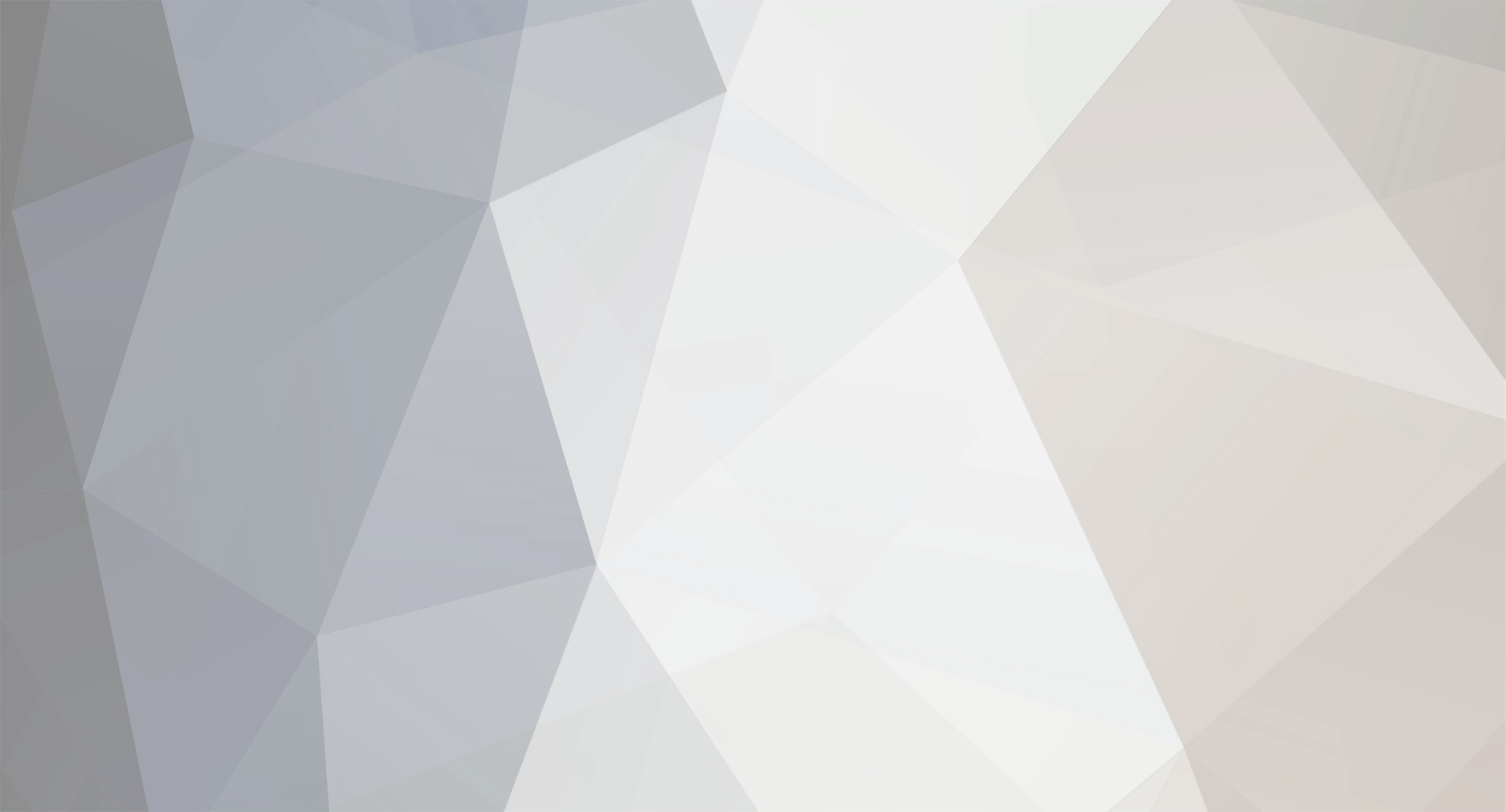
Oscar Ames
Members-
Posts
8 -
Joined
-
Last visited
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
Oscar Ames's Achievements

Tree Puncher (2/8)
0
Reputation
-
how to get the inventory of a chest from the client side [Solved]
Oscar Ames replied to Oscar Ames's topic in Modder Support
thankyou for your input. I grab the GUI object on the GuiOpenEvent and the on the world tick event i keep checking it until the inventory loads. this successfully grabs the chest inventory from the client side. here is my final code. package com.example.examplemod; import java.util.ArrayList; import net.minecraft.client.KeyMapping; import net.minecraft.client.Minecraft; import net.minecraft.client.gui.screens.Screen; import net.minecraft.client.gui.screens.inventory.AbstractContainerScreen; import net.minecraft.client.multiplayer.ClientPacketListener; import net.minecraft.client.player.LocalPlayer; import net.minecraft.core.BlockPos; import net.minecraft.core.Direction; import net.minecraft.core.NonNullList; import net.minecraft.network.chat.Component; import net.minecraft.network.protocol.game.ServerboundContainerClosePacket; import net.minecraft.network.protocol.game.ServerboundMovePlayerPacket; import net.minecraft.network.protocol.game.ServerboundUseItemOnPacket; import net.minecraft.world.InteractionHand; import net.minecraft.world.inventory.Slot; import net.minecraft.world.item.ItemStack; import net.minecraft.world.level.block.entity.*; import net.minecraft.world.phys.BlockHitResult; import net.minecraft.world.phys.Vec3; import net.minecraftforge.client.event.GuiContainerEvent; import net.minecraftforge.client.event.GuiOpenEvent; import net.minecraftforge.event.ForgeEventFactory; import net.minecraftforge.event.TickEvent; import net.minecraftforge.eventbus.api.SubscribeEvent; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.event.lifecycle.FMLClientSetupEvent; import net.minecraftforge.fmlclient.registry.ClientRegistry; @Mod.EventBusSubscriber public class ForgeEventHandler { private static ArrayList<BlockPos> homeBase = new ArrayList<BlockPos>(); private static Boolean pressed = false; public static final KeyMapping keyBinding = new KeyMapping("pam SETUP", 79, "PAM"); private static Screen GUI = null; @SubscribeEvent public static void setup(FMLClientSetupEvent event) { ClientRegistry.registerKeyBinding(keyBinding); } @SubscribeEvent public static void updatePlayer(TickEvent.PlayerTickEvent event) { /*if(event.player.canEat(false)){ for(ItemStack inventoryStack : event.player.getInventory().items){ if (inventoryStack.isEdible()) { ForgeEventFactory.onItemUseStart(event.player, inventoryStack, 0); ItemStack result = inventoryStack.getItem().finishUsingItem(inventoryStack, event.player.getCommandSenderWorld(), event.player); ForgeEventFactory.onItemUseFinish(event.player, inventoryStack, 0, result); if (!event.player.canEat(false)) { break; } } } }*/ if (keyBinding.isDown() && !pressed) { pressed = true; homeBase.add(((BlockHitResult) Minecraft.getInstance().player.pick(20.0D, 0.0F, false)).getBlockPos()); System.out.println("set postion"); if (homeBase.size()>=2) { System.out.println("opening container"); openBlock(detectContainers(homeBase).get(0)); homeBase.clear(); } if (!keyBinding.isDown()) { pressed=false; } } } @SubscribeEvent public static void updateWorld(TickEvent.WorldTickEvent event) { if (GUI != null) { if (GUI instanceof AbstractContainerScreen) { AbstractContainerScreen container = ((AbstractContainerScreen)GUI); NonNullList<Slot> slots = container.getMenu().slots; for (int i=0; i<slots.size();i++) { ItemStack temp = slots.get(i).getItem(); System.out.print(temp.getDisplayName().getString()+" | "); } System.out.println(""); GUI = null; } } } @SubscribeEvent public static void openChest(GuiOpenEvent event) { GUI = event.getGui(); } private static ArrayList<Vec3> detectContainers(ArrayList<BlockPos> homebase) { ArrayList<Vec3> chests = new ArrayList<Vec3>(); int x = homebase.get(1).getX()-homebase.get(0).getX(); int y = homebase.get(1).getY()-homebase.get(0).getY(); int z = homebase.get(1).getZ()-homebase.get(0).getZ(); for (int i=homebase.get(0).getX();i!=homebase.get(1).getX();i+=Math.signum(x)) { for (int j=homebase.get(0).getY();j!=homebase.get(1).getY();j+=Math.signum(y)) { for (int k=homebase.get(0).getZ();k!=homebase.get(1).getZ();k+=Math.signum(z)) { BlockEntity temp = Minecraft.getInstance().level.getBlockEntity(new BlockPos(i,j,k)); if (temp instanceof RandomizableContainerBlockEntity && !(temp instanceof HopperBlockEntity)) { chests.add(new Vec3(i,j,k)); } } } } return chests; } @SuppressWarnings("resource") private static void openBlock(Vec3 blockPos){ /*BlockHitResult hit = new BlockHitResult(blockPos, Direction.DOWN, new BlockPos(blockPos), false);*/ BlockHitResult hit = (BlockHitResult) Minecraft.getInstance().player.pick(20.0D, 0.0F, false); Minecraft.getInstance().getConnection().send(new ServerboundUseItemOnPacket(InteractionHand.MAIN_HAND,hit)); } } -
how to get the inventory of a chest from the client side [Solved]
Oscar Ames replied to Oscar Ames's topic in Modder Support
Ah I see the problem with the email example. How do I wait for the packet to come in the. I haven't found an event for it do I have to apply an injection or something -
how to get the inventory of a chest from the client side [Solved]
Oscar Ames replied to Oscar Ames's topic in Modder Support
I don't like mining and due to that i want to write a program that will mine for me on my world. i also play on a server with a friend who has allowed this automation. I would also like to use this type of automation to ferry and sort items from farms and such similar to how someone would implement the computercraft turtles or thaumcraft golems to automate a task. i do see the point of view that this could be seen as a cheat to stop player grind on a server but other mods that I would like to make in the future like a item in chest finder for looking through messy bases or a full inventory swapper will need this mechanic as well. I think seeing what items are in a chest while the client has said chest open shouldn't be that hard to accomplish. if it means anything I do not intend for this mod to be a cheat and do not intend on distributing it on any website. -
how to get the inventory of a chest from the client side [Solved]
Oscar Ames replied to Oscar Ames's topic in Modder Support
I changed the getBlock function to private static void openBlock(){ BlockHitResult hit = (BlockHitResult) Minecraft.getInstance().player.pick(20.0D, 0.0F, false); Minecraft.getInstance().getConnection().send(new ServerboundUseItemOnPacket(InteractionHand.MAIN_HAND,hit)); try { Thread.sleep(50); } catch (InterruptedException e) { e.printStackTrace(); } BlockEntity entity = Minecraft.getInstance().level.getBlockEntity(hit.getBlockPos()); if (entity != null && entity instanceof RandomizableContainerBlockEntity) { ItemStack item = ((RandomizableContainerBlockEntity)Minecraft.getInstance().level.getBlockEntity(hit.getBlockPos())).getItem(0); System.out.println(item); } } I also removed anything beside getBlock() from within the keybinding.isDown() if statement but I am still unable to get the items from a chest filled with stone -
how to get the inventory of a chest from the client side [Solved]
Oscar Ames replied to Oscar Ames's topic in Modder Support
Thank you I completely forgot about that. I will attempt to put a delay in -
how to get the inventory of a chest from the client side [Solved]
Oscar Ames replied to Oscar Ames's topic in Modder Support
i updated the getBlock function that opens the chest to add an attempt to detect the item in the 0 slot private static void openBlock(){ BlockHitResult hit = (BlockHitResult) Minecraft.getInstance().player.pick(20.0D, 0.0F, false); Minecraft.getInstance().getConnection().send(new ServerboundUseItemOnPacket(InteractionHand.MAIN_HAND,hit)); String item = ((RandomizableContainerBlockEntity)Minecraft.getInstance().level.getBlockEntity(hit.getBlockPos())).getItem(0).toString(); System.out.println(item); } this still returns 1 air -
how to get the inventory of a chest from the client side [Solved]
Oscar Ames replied to Oscar Ames's topic in Modder Support
so if i open the chest then I can read it. i am looking into automating player actions to eventually include mining and chopping trees but I am just working on gearing up at the moment. -
I am working in 1.17.1 and i am trying to read the contents of a chest from a client side mod. I have attempted to read the chest using ((RandomizableContainerBlockEntity)Minecraft.getInstance().level.getBlockEntity(new BlockPos(1,1,1))).getItem(0); this returns null since it is reading from the client side. is there any way to get the inventory within a block without needing any server side code I was thinking of trying to catch the ClientboundContainerSetContentPacket but I have seen that is said not to be the best idea. the overall project is to try to get the inventories within an area and then by sending packets to the server move the player in order to reequip with items from said chests. here is my code so far I haven't implemented movement of the player yet i am just trying to move items between inventories. ForgeEventHandeler.java @Mod.EventBusSubscriber public class ForgeEventHandler { private static ArrayList<BlockPos> homeBase = new ArrayList<BlockPos>(); private static Boolean pressed = false; public static final KeyMapping keyBinding = new KeyMapping("pam SETUP", 79, "PAM"); @SubscribeEvent public static void updatePlayer(TickEvent.PlayerTickEvent event) { if(event.player.canEat(false)){ for(ItemStack inventoryStack : event.player.getInventory().items){ if (inventoryStack.isEdible()) { ForgeEventFactory.onItemUseStart(event.player, inventoryStack, 0); ItemStack result = inventoryStack.getItem().finishUsingItem(inventoryStack, event.player.getCommandSenderWorld(), event.player); ForgeEventFactory.onItemUseFinish(event.player, inventoryStack, 0, result); if (!event.player.canEat(false)) { break; } } } } if (keyBinding.isDown() && !pressed) { pressed = true; openBlock(); if(homeBase.size()==2) { homeBase.clear(); } HitResult block = Minecraft.getInstance().player.pick(20.0D, 0.0F, false); if(block.getType() == BlockHitResult.Type.BLOCK){ BlockPos blockpos = ((BlockHitResult)block).getBlockPos(); homeBase.add(blockpos); Minecraft.getInstance().getConnection().send(new ServerboundChatPacket("position set")); } if(homeBase.size()==2) { int[] start ={(int)homeBase.get(0).get(Direction.Axis.X),(int)homeBase.get(0).get(Direction.Axis.Y),(int)homeBase.get(0).get(Direction.Axis.Z)}; int[] end ={ (int) homeBase.get(1).get(Direction.Axis.X),(int)homeBase.get(1).get(Direction.Axis.Y),(int)homeBase.get(1).get(Direction.Axis.Z)}; int[] diff = {end[0]-start[0],end[1]-start[1],end[2]-start[2]}; for (int i=start[0]; i!=(end[0]+Integer.signum(diff[0])); i+=Integer.signum(diff[0])){ for (int j=start[1]; j!=(end[1]+Integer.signum(diff[1])); j+=Integer.signum(diff[1])){ for (int k=start[2]; k!=(end[2]+Integer.signum(diff[2])); k+=Integer.signum(diff[2])){ System.out.println(i+","+j+","+k); BlockPos posTemp = new BlockPos(i,j,k); BlockEntity blockEntity = Minecraft.getInstance().level.getBlockEntity(posTemp); if (blockEntity != null && blockEntity instanceof RandomizableContainerBlockEntity) { /*add chest to a category of chests that best describes what is in it E.G tools, weapons, food*/ } } } } } } if (!keyBinding.isDown()) { pressed=false; } } @SubscribeEvent public static void setup(FMLClientSetupEvent event) { ClientRegistry.registerKeyBinding(keyBinding); } @SuppressWarnings("resource") private static void openBlock(){ BlockHitResult hit = (BlockHitResult) Minecraft.getInstance().player.pick(20.0D, 0.0F, false); Minecraft.getInstance().getConnection().send(new ServerboundUseItemOnPacket(InteractionHand.MAIN_HAND,hit)); } } exampemod.java // The value here should match an entry in the META-INF/mods.toml file @Mod("examplemod") public class ExampleMod { // Directly reference a log4j logger. public ExampleMod() { } }