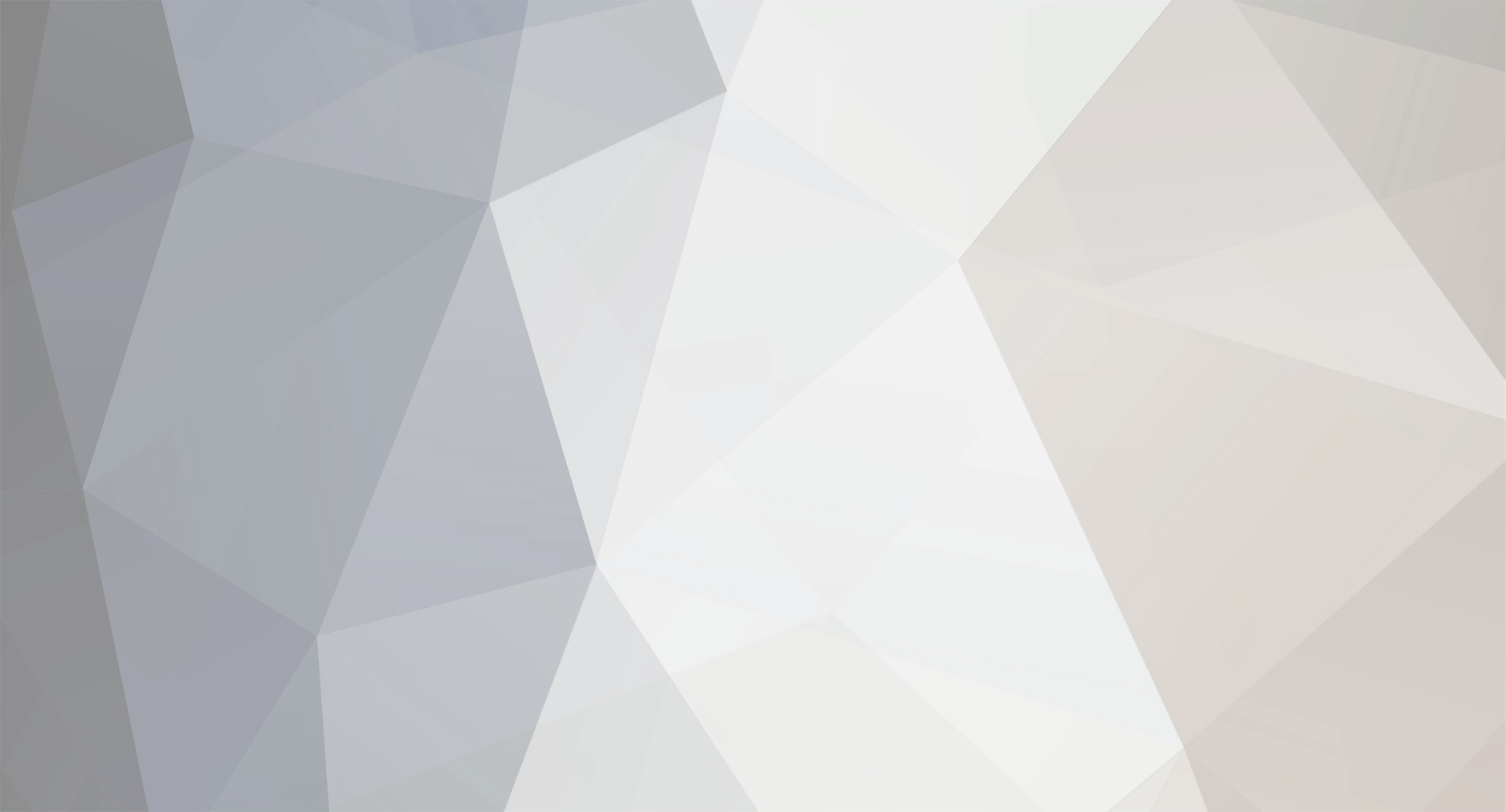
kauan99
Members-
Posts
210 -
Joined
-
Last visited
Everything posted by kauan99
-
also, if you'll only need to differentiate between item, stack size and metadata, and if you won't need to put or get things into the map too often then you could put/get ItemStack.toString. I think it would work, but it's probably slow.
-
[1.7.10] Checking all armor a player is wearing at a given tick
kauan99 replied to onContentStop's topic in Modder Support
what I was suggesting was something like this: an approach to the custom armor class. I don't know how yours is. this is just an idea that would work well with my suggestion public class MyCustomArmorClass extends ItemArmor { public static final int AMETHYST_ID = 0; public static final int SAPPHIRE_ID = 1; public static final int RUBY_ID = 2; public static final int TOPAZ_ID = 3; public static final int[] gemEffects; static { gemEffects = new int[4]; gemEffects[AMETHYST_ID] = Potion.regeneration.id; gemEffects[sAPPHIRE_ID] = Potion.resistance.id; gemEffects[RUBY_ID] = Potion.damageBoost.id; gemEffects[sAPPHIRE_ID] = Potion.digSpeed.id; } public final int ID; public MyCustomArmorClass(ArmorMaterial armorMaterial, int armorType, int renderIndex, int gemID) { super(armorMaterial, armorType, renderIndex); this.ID = gemID; } //and the rest of your code } then the event handler class: public class ArmorEventHandler { public ArmorEventHandler(){ } @SubscribeEvent public void onLivingUpdate(LivingUpdateEvent event) { if(event.entityLiving instanceof EntityPlayer) { EntityPlayer player = (EntityPlayer)event.entityLiving; ItemStack[] armorInv = player.inventory.armorInventory; int[] armorCount = new int[4]; for(ItemStack armorStack : armorInv) { if(armorStack != null && armorStack.getItem() instanceof MyCustomArmorClass) { MyCustomArmorClass armorItem = (MyCustomArmorClass)armorStack.getItem(); armorCount[armorItem.ID]++; } } for(int i = 0; i < 4; ++i) { int count = armorCount[i]; if(count > 0) { player.addPotionEffect(new PotionEffect(MyCustomArmorClass.gemEffects[i], 1, count > 3 ? count : 3)); } } } } } and don't forget to register an instance of your event handler class to the MinecraftForge.EVENT_BUS. i think maybe because of the fact that this would run only once per tick it would be more efficient and less prone to unwanted side effects. -
[1.7.10] Checking all armor a player is wearing at a given tick
kauan99 replied to onContentStop's topic in Modder Support
I think this will run once for every armor piece at every tick, causing it to run 4 times if the player has the item that implements that method in every armor slot. -
[1.7.10] Checking all armor a player is wearing at a given tick
kauan99 replied to onContentStop's topic in Modder Support
you could subscribe to LivingUpdateEvent and, if event.livingEntity is of type EntityPlayer, then you cast it to EntityPlayer, get EntityPlayer.inventory.armorInventory and check each slot. -
but I know it works, I can give whatever enchantment level I want to an item if I do it in code. the thing is, those are called "unsafe" enchantments. and I wanted to know if they are really unsafe. for example, can they corrupt the saved state of an entity/tileentity? if something is known as unsafe, I expect there is some risk involved. I wanted to know if those risks are acceptable or not. corrupting data, causing crashes or any sort of unstability is not acceptable. being too powerful to the point of hit-killing a fully diamond-armored player is.
-
sorry, I'll explain better. what is the worst that can happen if I override Item.onCreated and add an enchantment with a level above that allowed by the enchanting table (for instance, smite 10).
-
if i want to give "smite X" onCreated is it bad?
-
[1.7.10] LivingSpawnEvent.CheckSpawn not working for passive mobs?
kauan99 replied to McJty's topic in Modder Support
this event is not fired for EntityAnimal or any derived class. try using EntityJoinWorld instead -
I think I solved it. I manually removed cauldron and put forge instead (multicraft wanted to charge U$15.00 to do it...). i deleted every file that had my uuid in it. the server runs but a few things seem weird. the problem was not my mod, this I know.
-
your "load" method can't be annotated with @EventHandler because that's not your mod class. you can invoke it from inside the actual load method from your mod class, or move the register code there and delete this method from EventManager. the other thing wrong is that you're registering to the wrong bus. EntityJoinWorld event is fired in MinecraftForge.EVENT_BUS.
-
I'm trying to run my mod on my little multiplayer server and it's not working properly. it's a cauldron server (not by choice... the guys from tech support installed cauldron and spigot and a lot of other weird stuff on it. I had just Forge and I used ForgeEssentials to manage this kind of thing, then I complained about the server being laggy and they told me they were gonna move me to a different panel - whatever that meant. when they were done, my server had all this bukkit stuff on it. everything was working fine though and it has been fine for months, until I intalled my mod's newest version today, with event handling) I believe my character actually broke, and I don't know how to delete my saved data so I can fix it (if anyone knows how to do that, please tell me!). My HP doesn't show. sometimes my character displays the hurt animation forever, even if I exit the game. I have to put myself in god mode to make the hurt animation stop, then when I come back of god mode the animation doesn't restart, thank god, but it goes into a loop again the moment I get hurt again. also I can't die, not even with /kill or or drowning in lava or falling into the void. Even if I revert my mod back to it's past version, when there was no event handling, my character remains broken like this. I want to know what I did wrong but also, if possible, I want to know how to fix my character, because I only have one Minecraft account. I know the problem is the event handling, more specifically the handling of LivingHurtEvent. is this a known issue with cauldron or is my code wrong? my event handling code is bellow http://pastebin.com/jxrF0ae9
-
[1.7.10] how do I know when I need the world to be client or server?
kauan99 replied to kauan99's topic in Modder Support
I see. sometimes the client displays the effect ran out, even though it's probably there, because I'm adding it before it can run out. that's because the client haven't received the packet yet right? in those cases, is the potion effect actually active? if such effect was to reduce damage amount from a specific source during OnLivingHurt event, then it would, no matter what the client would think, since that event is only fired on server side. is this correct? -
some methods get invoked by both client and server. The ones I'm struggling with at the moment is ItemSword.onUpdate and ArmorItem.onArmorTick. I want to add potion effects to the player while he's holding/wearing an item. But I don't want to do that every tick so I set the potion effect to last a fixed amount of ticks and I perform the check again after that same amount of ticks. Basically, I do entity.addPotionEffect for every Potion on every armor item the player is wearing and also the weapon they're holding, setting the duration of the effect to the next time I will tick the item. Is this supposed to be done server side or client side? If I do it on both sides I get a few weird behaviors like effects lasting longer than they should. I'm currently doing it only when world.isRemote == false (i.e. server side). Is this right?
-
[1.7.10] [SOLVED] How to properly extend Potion?
kauan99 replied to kauan99's topic in Modder Support
Everything works great now. -
[1.7.10] event when item gets equiped/unequiped?
kauan99 replied to kauan99's topic in Modder Support
You're right. It wouldn't work If I got those packets. And even if Forge had an event for it, it still wouldn't work, precisely because of what you said about the nature of PotionEffects being combined with each other when added. I loved world.getWorldTime(). I will use it to avoid adding the effects on every tick. -
[1.7.10] event when item gets equiped/unequiped?
kauan99 replied to kauan99's topic in Modder Support
yes that's what I've been using, but I don't think it's a good pattern.every tick I have to add several PotionEffects to each entity wearing my items (really a lot, I created a whole bunch of new potions). the fact that there are packets sent specifically for the 2 cases I need gives me hope of designing a better implementation -
Is there an event when an armor piece is equiped and unequiped? Also, when the held item is changed? I know minecraft sends a packet when the armorInventory gets changed (S04PacketEntityEquipment) and when the held item gets changed (C09PacketHeldItemChange and S09PacketHeldItemChange), Is there a way to react to those packets? I want to be able to add PotionEffects to players while they wear/wield my items My current approach is to override the ItemSword.onUpdate and the ItemArmor.onArmorTick and add all the effects to the player, but I feel like adding a bunch of potion effects to everyone that's wearing my items every tick is not a good design. I would also like to make check if a certain armor set is complete in order to give a special custom effect, but that would be too much overhead.
-
[1.7.10] [SOLVED] How to properly extend Potion?
kauan99 replied to kauan99's topic in Modder Support
So my way (doing that thing inside performEffect) is the only way? I thought something invoked from server side only would end up updating the client. -
[1.7.10] [SOLVED] How to properly extend Potion?
kauan99 replied to kauan99's topic in Modder Support
I can't understand Attribute modifiers at all. What I'm trying to do is a potion of flight. This is what I do: @Override public void performEffect(EntityLivingBase target, int amplifier) { if(target instanceof EntityPlayer) { EntityPlayer player = (EntityPlayer)target; if(!player.capabilities.isCreativeMode) { PotionEffect effect = player.getActivePotionEffect(ModPotions.flight); if(effect != null) { if(effect.getDuration() <= 20)//strangely, it doesn't work with 1 { player.capabilities.allowFlying = false; player.capabilities.isFlying = false; } else { player.capabilities.allowFlying = true; } } else { player.capabilities.allowFlying = false; } } } } @Override public boolean isReady(int duration, int amplifier) { return duration == 1 || duration % 20 == 0; //returns true every 20 ticks, then performEffect is invoked } it works but I can't help but feel this is an ugly hack. I was wondering if there was a better way of doing that. I tryed overloading applyAttributesModifiersToEntity and removeAttributesModifiersFromEntity (I would just invoke the super class version of the method and then set the player.capabilities to allow or disallow flight depending on the method) but setting the player's capabilities from inside those methods has no effect, probably something to do with the Side from which those methods are invoked (Server/Client). Is there a better way of doing that? -
[1.7.10] [SOLVED] How to properly extend Potion?
kauan99 replied to kauan99's topic in Modder Support
Is there an event I can subscribe to handle when a potion effect is added and when it's ended? If there isn't, maybe Forge devs could give Potions some attention if I may ask. There should be a way to register them into the GameRegistry instead of having to hack the potionTypes array and all that. and there should also be events related to them. performEffect is executed too often and doesn't fit certain cases very well, the most clear example of that being a potion of flight. -
[1.7.10] [SOLVED] How to properly extend Potion?
kauan99 replied to kauan99's topic in Modder Support
thanks again, diesieben07. you're a living wiki!