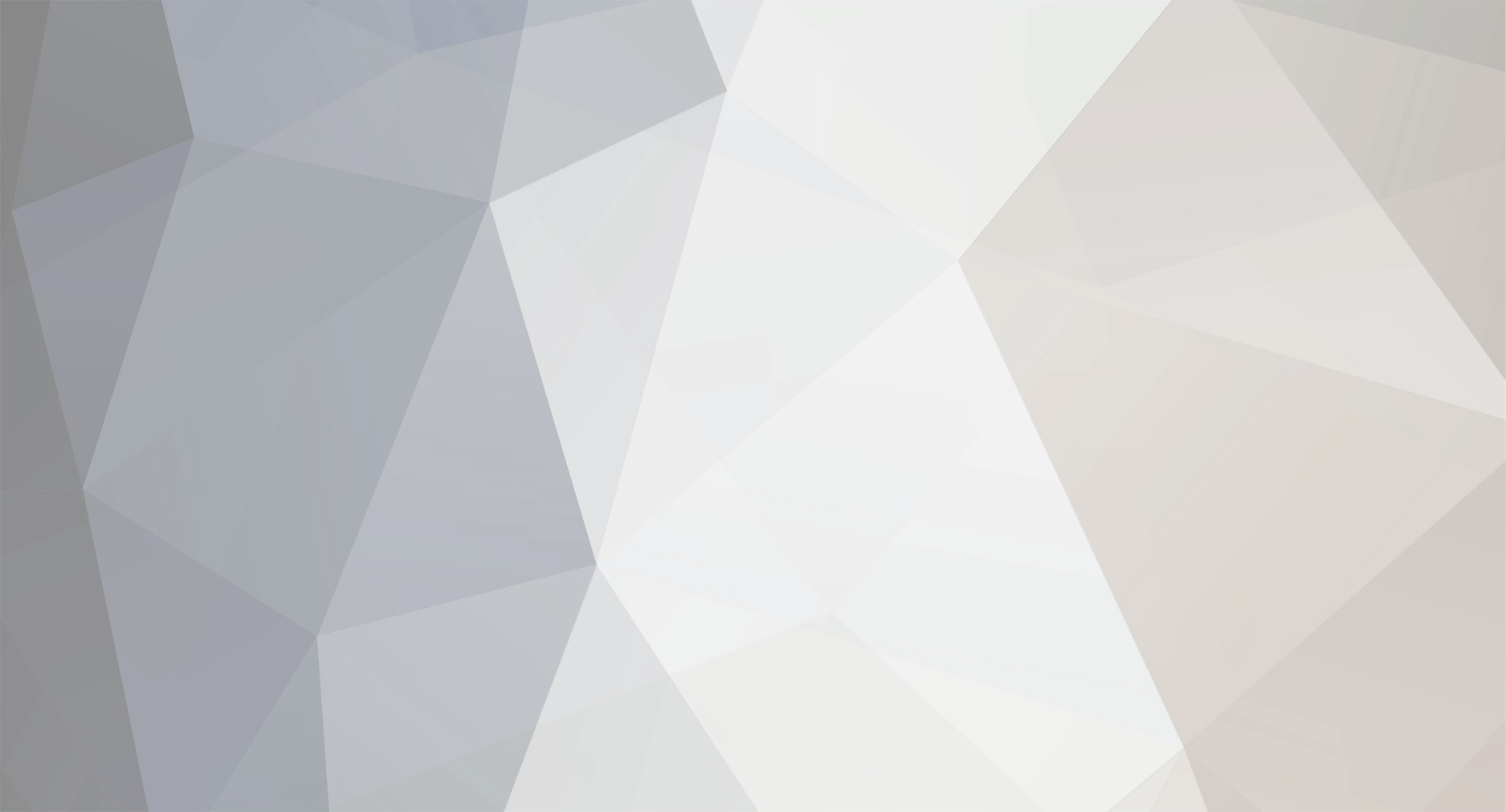
SoLegendary
Members-
Posts
95 -
Joined
-
Last visited
Everything posted by SoLegendary
-
I'm trying to import classes from this mod library in my project: https://www.curseforge.com/minecraft/mc-mods/ftb-library-forge I've downloaded the .jar for 1.18.2, placed it in my project root folder and added it in IntelliJ as a module (by going to file -> project structure -> main -> +JARs or directories -> ftb-library-forge-1802.3.6-build.115.jar) I can import the classes in my code without error and have working autocomplete and inspection: Except whenever I try to build I get: error: package dev.ftb.mods.ftblibrary.ui does not exist import dev.ftb.mods.ftblibrary.ui.BaseScreen; I've also tried adding it to my classpath (I am on Windows) as suggested by some stackoverflow posts, using: javac -cp .;./ftb-library-forge-1802.3.6-build.115.jar dev/ftb/mods/ftblibrary/FTBLibrary.java except this just gives me: error: no source files Is there something wrong with what I'm doing here and/or is there an alternate way to get this dependency into my project?
-
Ok cool so I managed to set visibility flags in RenderLivingEvent for any mob I need (note 'Unit' right now consists of Zombies, Skeletons and Creepers in my project): public static void onRenderLivingEntity(RenderLivingEvent.Pre<? extends LivingEntity, ? extends Model> evt) { LivingEntity entity = evt.getEntity(); Model model = evt.getRenderer().getModel(); if (entity instanceof Unit) { if (entity instanceof Creeper) { ((CreeperModel) model).root().visible = false; } else { ((HumanoidModel) model).body.visible = false; } } } Skeletons and zombies work fine and I assume I can extend this to all humanoid mobs with maybe some small edge cases. However, the problem is creepers. They don't seem to have a head, instead only one ModelPart: 'root'. Setting this invisible makes the entire creeper invisible. Is there any way to actually separate the visibility of the creeper's head from the rest of the model?
-
What I can roughly gather from renderEntityInInventory is its grabbing the model rendering data, applying gui-specific configs like disabling shadows and setting a constant rotation, rendering it and then resetting those configs. I don't really understand the matrix and quaternion math here though and I hope that it's not necessary to. I don't see anything inside of EntityRenderDispatcher or the LivingEntity that can directly modify model part visibilities though unless I'm missing something? As for RenderPlayerEvent.Pre, do you mean the event itself has methods/data I need to adjust to control model visibilities or that this is simply the event to call my 'renderHead' function in once written?
-
I want to be able to draw any humanoid mob or player in my custom screen but only the head of it, as opposed to the entire model. I ideally would like to avoid having to create entirely new model resources just for this as I plan to be able to swap out any kind of humanoid mob/player skin easily. I did see that net.minecraft.client.model.HumanoidModel.bodyParts() exists, but I want to exhaust other options before trying to modify existing entity model resources (which could potentially affect in-world rendering too, but I'm not sure).
-
[1.18.1] Workaround for multiple inheritance
SoLegendary replied to SoLegendary's topic in Modder Support
Ah of course, I got used to just accessing directly since they were only ever used privately. Well I suppose this helps out the core issue, would've liked to be able to extend from two classes but I guess this is the best I've got. Thanks for the help. -
[1.18.1] Workaround for multiple inheritance
SoLegendary replied to SoLegendary's topic in Modder Support
That does sound like the way to go, however, many of these helper functions would need to directly modify a lot of new fields specific to 'Units' I've added - I have about 15 of these. I would like to pass in the mob object itself to the functions so that I don't have to keep passing these fields in, but then what comes up is the issue of parameter typing - I want the helper functions to accept any of my three extended mob classes but of course, Java is hard-typed so it's not gonna accept any old generic variable. I could make the parameter something more generic like Monster or even just a plain Object, but then of course we lose the ability to reference my Unit fields. Afaik, interfaces don't let you define non-static fields either, that would have been really helpful for me... -
I've successfully implemented a series of classes that extend vanilla mobs: public class SkeletonUnit extends Skeleton implements Unit public class ZombieUnit extends Zombie implements Unit public class CreeperUnit extends Creeper implements Unit The 'Unit' part of the name denotes that it is a vanilla mob class that has modified AI, fields and methods to allow for RTS-like top-down controls. However, all of these classes' tick() methods are almost identical and need the use of many of the same private helper functions and fields. Just having an interface implemented hardly helps with this as it only enforces that these methods exist and not what kind of code they run or the private fields they rely on. Ideally I would have wanted to have it inherit from both my own original class 'Unit' (where I can define a tick() and series of helper functions that all three of these classes use) as well as the vanilla mob (so that I don't have to recreate all of the vanilla mob's features such as sounds, model and basic AI) but Java does not allow for multiple inheritance. Is there a way that I can have the best of both worlds here without having to duplicate my 'Unit' code across all of these classes?
-
I've created a custom entity named VillagerUnit which I want to look exactly like a base villager but with my own custom AI. I was following this guide here for how to make said custom entity but he uses BlockBench to make a custom model renderer class instead of a vanilla model: https://www.youtube.com/watch?v=iMJ1kWNWQBQ When I try to apply the same registerRenderers code he does at 37:40 but instead on the existing vanilla renderer VillagerRenderer I get this error: I assume I don't need to register the layer since that had no reference the example entity made in the video (and I assume vanilla registers the layer itself for use by the actual villager mob) Unit class: https://pastebin.com/Sa7PJLJT VillagerUnit class (extends Unit): https://pastebin.com/RvhjXL5t Entity registration class: https://pastebin.com/aTqAuxcN Attributes registration class: https://pastebin.com/iU0xFALg
-
I'm able to successfully find the first block along a ray by using this line: HitResult hitResult = MC.level.clip(new ClipContext(vectorNear, vectorFar, ClipContext.Block.OUTLINE, ClipContext.Fluid.NONE, null)); However, while there is an option to include ClipContext.Fluid.NONE to ignore fluid blocks like water and lava, there isn't a similar one to ignore non-solid blocks such as grass, flowers, etc. Is there a way to achieve the same outcome but ignore these blocks? I am able to tell if a particular block is solid or not using this line: Minecraft.level.getBlockState(blockPos).getMaterial().isSolidBlocking() EDIT: to clarify, I want to get the next solid block BEHIND the non-solid one, using Fluid.NONE instead causes it to return nothing
-
For 1.16.5 you can check out this example code here: https://github.com/TheGreyGhost/MinecraftByExample/blob/master/src/main/java/minecraftbyexample/usefultools/debugging/DebugBlockVoxelShapeHighlighter.java From what I understand it basically cancels DrawBlockHighlightEvent and then goes on to write what the event would do in the first place so that you can adjust its parameters. If you want to change the block to be highlighted for example, you can change the BlockPos variable to whatever coordinate you want. Keep it mind it only ever highlights the wireframe, not the block faces themselves. If you ever manage to get this working in 1.17.1 please tell me! I've been stuck on this issue for a while
-
I've managed to convert the example over to 1.17.1 and get the two variables I needed, but now the issue is that as soon as the vertex building code is executed (vertexConsumer.vertex()) my game immediately crashes and throws an exception: java.lang.IllegalStateException: Not filled all elements of the vertex Crash logs: https://pastebin.com/Bibb7MBM My 1.17.1 code: https://pastebin.com/qZLdpTA5 Note that I did still just run this in the HighlightBlock event for the time being just to rule out that the crash didn't happen because I ran it in an unusual event. I tested this code in 1.16.5 and it ran fine, even when forcing the blockpos to a constant with testBlockPos.
-
I want to be able to draw the typical block outline that you get when you mouse over a block in first person on any block given I already have its BlockPos. I've found the specifics of how to draw an outline here (I know this is 1.16, I can deal with the class remappings later, assuming the logic has not significantly changed in 1.17): https://github.com/TheGreyGhost/MinecraftByExample/blob/master/src/main/java/minecraftbyexample/usefultools/debugging/DebugBlockVoxelShapeHighlighter.java However, this code does the drawing logic inside the DrawHighlightEvent.HighlightBlock event, and so is a bit redundant since this event is usually fired when a block looked at is being highlighted anyway (I want to outline blocks in events that don't involve the player looking at things). While I get that the point of the code is to demonstrate the inner workings of the event, it does automatically get access to important data that other events wouldn't, namely renderTypeBuffers and matrixStack (which I'm guessing are specific to the block to be outlined). If I wanted to run this code inside, for example, GuiScreenEvent.DrawScreenEvent, how could I get access to or recreate these two variables for the BlockPos that I have?
-
Given a block at position x,y,z how could I go about writing code in order to highlight it, for example by raising its apparently brightness (graphical brightness, not in-game minecraft brightness)? For some context I have created a mod to allow for free cursor movement while still being able to pan and rotate the camera, and want to also be able to select blocks by clicking. I've already gotten the actual selection working but I can't see the block that has been selected until I implement some kind of highlighting. EDIT: and the same for entities too EDIT2: I'll also settle for just dynamically changing the model on runtime. If altering the model is too complex, I can just create a simple block model defined as a 'marker' block for this purpose. EDIT3: I asked a much better question here
-
Following creating a top-down view mod of the game, I want to also be able to pan the camera in the X-Z plane by moving the mouse to the edges of the screen, like a classic top-down strategy game or MOBA. This works fine in full screen, but in windowed mode, there is nothing keeping the mouse inside the window so it's very easy to just move off of it out to the desktop and subsequently lost. Since mouse coordinates are only tracked on every tick, moving the mouse to the edges really fast also means it's last known coordinates may be too far from the edge to begin panning. As a bonus, if this is possible, could it also be made active only for certain GUI screens so that the user can still get the mouse out without having to alt-tab? I know what I'm trying to do might be out of scope of what Forge can do since it deals with the application window itself, I still want to make sure there isn't any in-forge options I'm missing.
-
Resurrecting this thread to ask: Is there a way to enable keybindings while in a GUI? I've been able to use GuiScreenEvent.KeyboardKeyEvent but for detecting held buttons it has a delay after the initial press, just like when holding down a button to repeat the same character while typing. The same is true for getGui().keyPressed(). However, keybindings do not have this issue and are guaranteed to execute code smoothly when a key is held. I've noticed that some GUI screens do in fact allow keybindings when open, like the player inventory screen. Is there a way to also allow use of keybindings on custom GUIs?
-
Actually I solved it a different way by just setting the camera entity to face downward above the ground and hard-setting the FOV to 180 to effectively render everything in range, then just manually offsetting the camera angle up by 45deg. That said, this method would definitely improve performance a lot. Thanks!
-
Do you know another method for editing the view frustum or disabling frustum culling entirely? I've tried looking at Minecraft.levelRenderer as it has quite a few references to view frustums like capturedFrustum, viewArea, frustumPoints, frustumPos, except when I use the access transformer to make these public in order to read them, they all seem to be null or 0. For some context, I've implemented an orthographic camera, but when it's zoomed out, you can see behind the player, which isn't rendered due to frustum culling: Increasing FOV helps somewhat, but I can only get it to a maximum of 180 (ie. a semicircle rendering cone) using the FOV modifier event before it just wraps around again. If there's a way to get it to 360 somehow that would also solve the issue.
-
I have only recently started modding using Forge, but I found very quickly that I was able to modify the vanilla source code directly by simply right clicking a classname -> Go to Declarations or Usages in IntelliJ. This would open up the source .jar file at the path: \build\fg_cache\net\minecraftforge\forge\1.16.5-36.2.0_mapped_official_1.16.5\forge-1.16.5-36.2.0_mapped_official_source_1.16.5.jar which I could easily pull out of the .jar archive, edit with a notepad and throw back in. I was doing this to disable frustum culling in ClippingHelper.java (ie. rendering outside of the player's usual view) for a camera mod I was working on. However, as of today making these changes seemed to suddenly have no effect. I saved the changes, deleted my .gradle and build folders, invalidated my caches and reran the gradlew genIntellijRuns to rebuild them and suddenly that source jar no longer exists and I cannot find any vanilla .java files anymore, only .class files which are not editable by text editor. My mod still runs perfectly fine, minus the changes to the ClippingHelper class I made of course. So my questions are: Where did this source .jar go? Is there an alternative way to edit Vanilla .java source files like I was doing? Is there an alternative to editing Vanilla classes in the first place? As proof I'm not crazy, I've attached the original ClippingHelper.java class below that I was previously able to find under that path. https://pastebin.com/MvFtQaTH