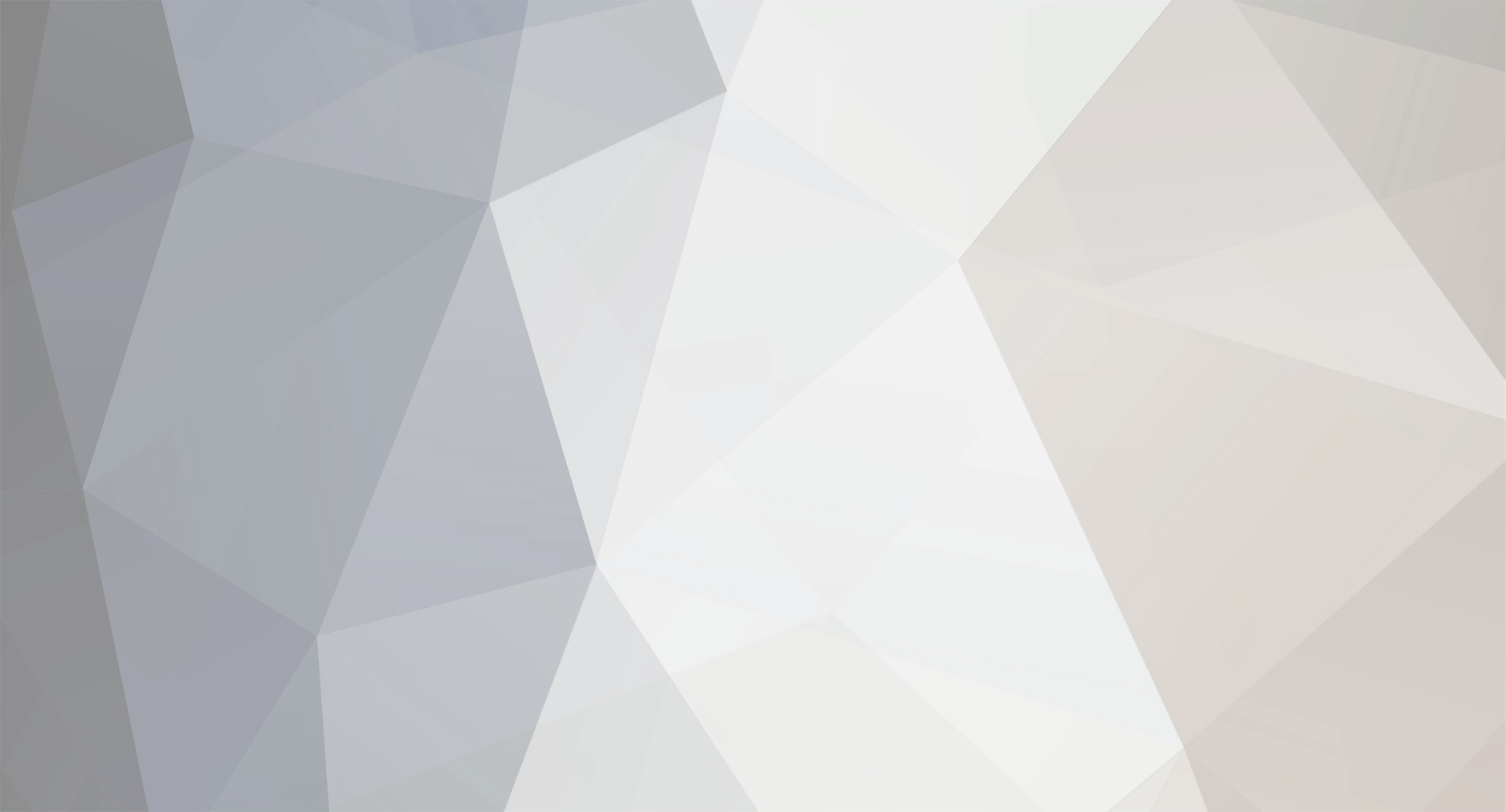
RInventor7
Members-
Posts
87 -
Joined
-
Last visited
Everything posted by RInventor7
-
On regular singleplayer I use function like this to check if player is holding down control key. @OnlyIn(Dist.CLIENT) public static boolean isHoldingControl() { return InputConstants.isKeyDown(Minecraft.getInstance().getWindow().getWindow(), GLFW.GLFW_KEY_LEFT_CONTROL) || InputConstants.isKeyDown(Minecraft.getInstance().getWindow().getWindow(), GLFW.GLFW_KEY_RIGHT_CONTROL); } However, on multiplayer it throws an error, because this is a client-side function and the Minecraft class is also client-side (if I understand correctly). How can I make a function that would work for DEDICATED_SERVER as well? I guess I have to pass in a player as an argument, but I can't figure out how to check weather control is held or not. Thanks.
-
I have a block and I want to display text on the block. At the moment I just have the text in the block texture. I looked Minecraft's SignBlock and SignBlockEntity classes, but didn't find a place where it actually deals with the text drawing part. How can I draw the text over the block texture in game?
-
In 1.18 creating biomes is bit different. I'm using DeferredRegister and everything was almost done but got stuck at the end. The biome object class is fine, but I have problems registring? (or I think its something to do with world generation?) the biomes. There ware some fields and methods that as the compiler said ware 'not visible'. I don't know very well how to use reflection, so I used AccessTransformers. Managed to make all necessary fields/methods visible apart from one constructor. How can I change this constructor visible? Or should I do this in some different way? In my main class: @SubscribeEvent public static void onServerAboutToStart(ServerAboutToStartEvent event) { MinecraftServer server = event.getServer(); Registry<DimensionType> dimensionTypeRegistry = server.registryAccess().registryOrThrow(Registry.DIMENSION_TYPE_REGISTRY); Registry<Biome> biomeRegistry = server.registryAccess().registryOrThrow(Registry.BIOME_REGISTRY); WorldGenSettings worldGenSettings = server.getWorldData().worldGenSettings(); for (Map.Entry<ResourceKey<LevelStem>, LevelStem> entry : worldGenSettings.dimensions().entrySet()) { DimensionType dimensionType = entry.getValue().typeHolder().value(); if (dimensionType == dimensionTypeRegistry.getOrThrow(DimensionType.OVERWORLD_LOCATION)) { ChunkGenerator chunkGenerator = entry.getValue().generator(); // Inject biomes to biome source if (chunkGenerator.getBiomeSource() instanceof MultiNoiseBiomeSource noiseSource) { List<Pair<Climate.ParameterPoint, Holder<Biome>>> parameters = new ArrayList<>(noiseSource.parameters.values()); parameters.add(new Pair<>(AirportBiome.PARAMETER_POINT, biomeRegistry.getOrCreateHolder(ResourceKey.create(Registry.BIOME_REGISTRY, ModBiomes.AIRPORT_BIOME.getId())))); //Next line gives error: The constructor 'MultiNoiseBiomeSource' is not visible. MultiNoiseBiomeSource moddedNoiseSource = new MultiNoiseBiomeSource(new Climate.ParameterList<>(parameters), noiseSource.preset); chunkGenerator.biomeSource = moddedNoiseSource; chunkGenerator.runtimeBiomeSource = moddedNoiseSource; } // Inject surface rules if (chunkGenerator instanceof NoiseBasedChunkGenerator noiseGenerator) { NoiseGeneratorSettings noiseGeneratorSettings = noiseGenerator.settings.value(); SurfaceRules.RuleSource currentRuleSource = noiseGeneratorSettings.surfaceRule(); if (currentRuleSource instanceof SurfaceRules.SequenceRuleSource sequenceRuleSource) { List<SurfaceRules.RuleSource> surfaceRules = new ArrayList<>(sequenceRuleSource.sequence()); surfaceRules.add(1, preliminarySurfaceRule(ResourceKey.create(Registry.BIOME_REGISTRY, ModBiomes.AIRPORT_BIOME.getId()), Blocks.LIGHT_GRAY_CONCRETE.defaultBlockState(), Blocks.SMOOTH_STONE.defaultBlockState(), Blocks.STONE.defaultBlockState())); NoiseGeneratorSettings moddedNoiseGeneratorSettings = new NoiseGeneratorSettings(noiseGeneratorSettings.noiseSettings(), noiseGeneratorSettings.defaultBlock(), noiseGeneratorSettings.defaultFluid(), noiseGeneratorSettings.noiseRouter(), SurfaceRules.sequence(surfaceRules.toArray(i -> new SurfaceRules.RuleSource[i])), noiseGeneratorSettings.seaLevel(), noiseGeneratorSettings.disableMobGeneration(), noiseGeneratorSettings.aquifersEnabled(), noiseGeneratorSettings.oreVeinsEnabled(), noiseGeneratorSettings.useLegacyRandomSource()); noiseGenerator.settings = new Holder.Direct(moddedNoiseGeneratorSettings); } } } } } private static SurfaceRules.RuleSource preliminarySurfaceRule(ResourceKey<Biome> biomeKey, BlockState groundBlock, BlockState undergroundBlock, BlockState underwaterBlock) { return SurfaceRules.ifTrue(SurfaceRules.isBiome(biomeKey), SurfaceRules.ifTrue(SurfaceRules.abovePreliminarySurface(), SurfaceRules.sequence( SurfaceRules.ifTrue(SurfaceRules.stoneDepthCheck(0, false, 0, CaveSurface.FLOOR), SurfaceRules.sequence(SurfaceRules.ifTrue(SurfaceRules.waterBlockCheck(-1, 0), SurfaceRules.state(groundBlock)), SurfaceRules.state(underwaterBlock))), SurfaceRules.ifTrue(SurfaceRules.stoneDepthCheck(0, true, 0, CaveSurface.FLOOR), SurfaceRules.state(undergroundBlock))))); }
-
I'm using RenderGameOverlayEvent to display text on a screen. I'm reading the text in from a file using a BufferedReader. The text file is in UTF-8 encoding, I specify for the reader to be in UTF-8 but when the text is displayed some characters (öÖäÄõÕüÜ) are displayed as displayed as question marks (????????). If I set the readers charset to StandardCharsets.ISO_8859_1, then everything is displayed correctly. However, when I then export my mod and run it in real Minecraft those letters are replaced with two other weird characters making the string double its actual size. EDIT: In the real game one character is displayed as two different other characters, then if printed out to Minecraft's debug.txt, in there those two characters are also different to those displayed in the game. How can I make those characters (öÖäÄõÕüÜ) to be displayed correctly not only in the development environment but in the real Minecraft as well?
-
[1.16.5] How to sync player custom persistent data?
RInventor7 replied to RInventor7's topic in Modder Support
It works. Thank you very much! I didn't think of that simple solution -
Hi! I add a value (e.g. 10) to player's custom persistent data variable and print it out. It's the same on server and client until I exit the world. If I reopen the game (or load the world) the value is still there on server, but on client it's 0. My TileEntity screen gets the value from the player and displays it. But it gets the value only on client which is not the correct value. How to sync this value/variable after I reopen the game/world? I have tried to add a DataParameter to PlayerEntity and use the entityDataManager in the PlayerTickEvent function, but it crashed the game. It's probably not the right way to do it. Any ideas? Thanks.
-
[1.16.5] How to sync slots in custom GUI?
RInventor7 replied to RInventor7's topic in Modder Support
There happened to be a few simple logic mistakes. I now get the correct sound out and a correct value is printed out on server Edit: Client it is still 0 for some reason. Because the functions checking the item count in a slot ware running only on server. -
[1.16.5] How to sync slots in custom GUI?
RInventor7 replied to RInventor7's topic in Modder Support
ATMButtonMessage https://pastebin.com/iPtCZ4jM I play the sound in ATMEvents lines 34 and 38 The printed output in ATMEvents line 26 shouldn't still be 0 when there are 64 items in the slot?? -
[1.16.5] How to sync slots in custom GUI?
RInventor7 replied to RInventor7's topic in Modder Support
Menu https://pastebin.com/aZ0u23rw Screen https://pastebin.com/wPEg6wRE Events https://pastebin.com/BbAeBGNw Block https://pastebin.com/SCA22h6i TileEntity https://pastebin.com/nBsb7AKS Screen functions https://pastebin.com/V92Qn8zr -
Hi! I have created a custom TileEntity. The container has 1 slot and 1 button. I enter any item to that slot. If I press the button, it should play a sound and print out how many items are in the slot (if the slot is not empty). Doesn't matter which items/blocks I put into the slot or how many of them I put into the slot, after pressing the button the sound is NOT played and the count printed out is always 0. Why is that so? And how to fix this? Thanks.
-
Hi! I have created a block that runs a check every second. In that check it should find certain particles (e.g. cloud particles) for example in 6 block radius around the block and if they exist then play a sound. I know how to check if certain entity exists in specified range, but how to check if a certain particle exists? Are particles also entities? Thanks.
-
How to sync custom entity NBT data [1.16.5]?
RInventor7 replied to RInventor7's topic in Modder Support
Thank you very much! -
Hi! My custom entity has a number variable stored in its NBT data. When I print out the values I see that it is showing me on server thread correct output (10 for example) but on the client/render thread it is always showing 0. If the value gets set on render thread I send the serverbound packet to server and set the value on server as well. Sadly, all the logic (variable value setting included) happens mostly on server thread. Or the value is 0 on the client thread and the correct server value is overwritten with the wrong client value. Is there an easy way to sync client and server values? And if not, then how to send clientbound packets? And would it even work?
-
In 1.16.5 I used AbstractGui.blit function to draw textures on the screen during the RenderGameOverlayEvent. In 1.17.1 the class is missing. It's probably just renamed. Does anyone know the new name of that class? Thanks.
-
Displaying text on screen without stopping the game [1.16.5]
RInventor7 replied to RInventor7's topic in Modder Support
Thank you very much. It was just the thing I needed :) -
When you press F3 bunch of information is displayed on the screen. I'd like to do something similar. I tried using GUI window with text labels on it, but when it is open the game runs in the background but the player can't move and the background has this gray semitransparent overlay that I do not want. Is it possible to create something like that and how? Thanks.
-
This was the only thing I forgot. Thanks very much!
-
I have updated Forge to the latest version, but for some reason it shows in the console that it is still using the old forge. build.gradle and .classpath files reference to the new forge. I can't seem to find the mistake or the reference to old forge version.
-
Debug.log-2
-
Updated Forge, but I'm still getting exactly the same error.