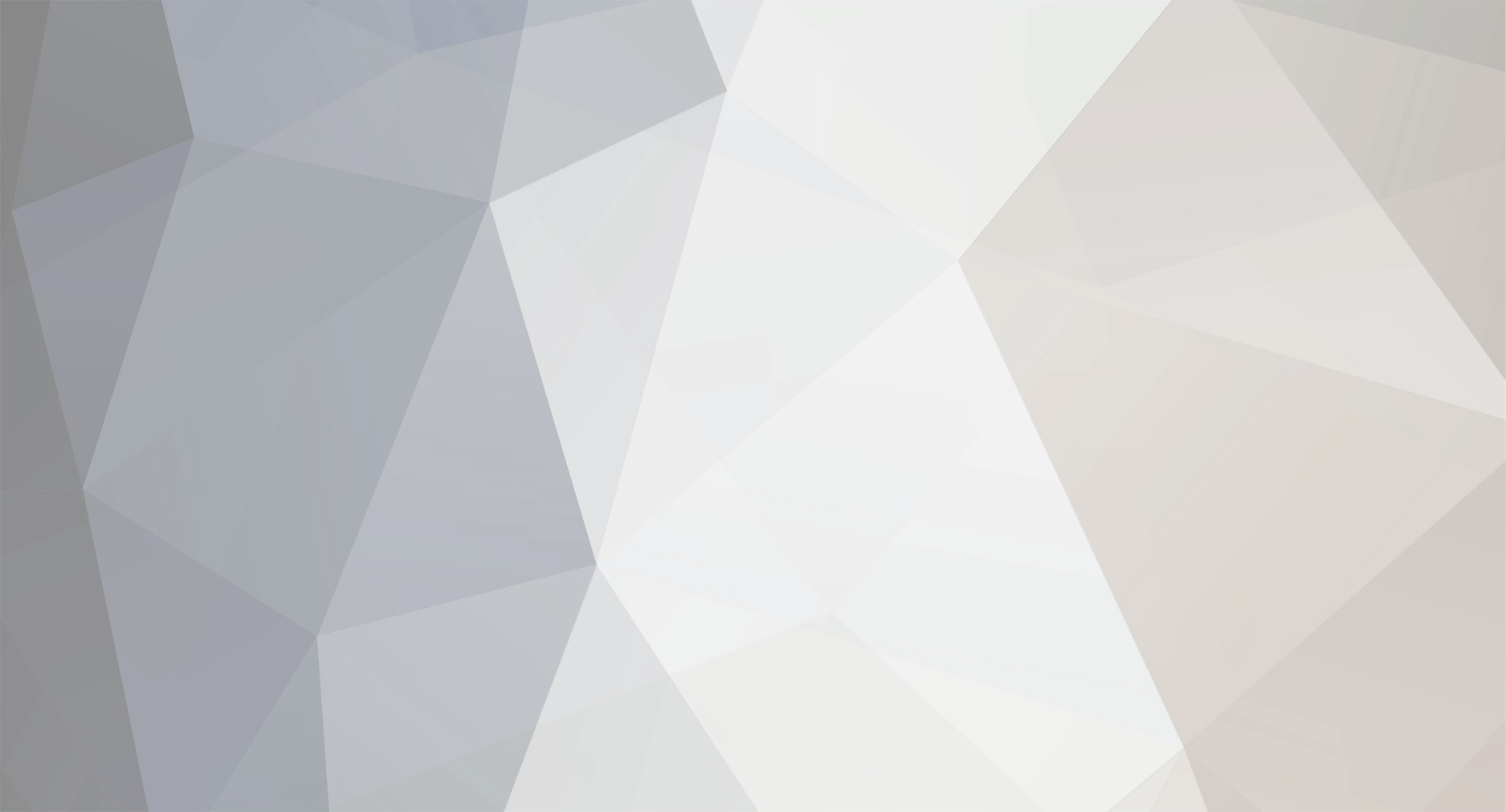
Will Bradley
Members-
Posts
20 -
Joined
-
Last visited
Will Bradley's Achievements

Tree Puncher (2/8)
0
Reputation
-
How to achieve server/client sync for Entity data
Will Bradley replied to Will Bradley's topic in Modder Support
Ok, got it, thanks! -
How to achieve server/client sync for Entity data
Will Bradley replied to Will Bradley's topic in Modder Support
For example, how about this? public class ClientBoundDragonColorPacket { private final Logger LOGGER = LogManager.getLogger(); private final @Nullable String colorName; private final int dragonID; public ClientBoundDragonColorPacket(@NotNull EnderDragon dragon) { this.colorName = dragon.getCapability(MulticoloredDragonMod.COLOR_CAPABILITY).resolve().get() .getColorName(); this.dragonID = dragon.getId(); } public ClientBoundDragonColorPacket(@NotNull FriendlyByteBuf buffer) { boolean colorNameIsNull = buffer.readBoolean(); if (colorNameIsNull) this.colorName = null; else this.colorName = new String(buffer.readByteArray()); this.dragonID = buffer.readVarInt(); } public void write(@NotNull FriendlyByteBuf buffer) { buffer.writeBoolean(this.colorName == null); if (this.colorName != null) buffer.writeByteArray(this.colorName.getBytes()); buffer.writeVarInt(this.dragonID); } public void handle() { Minecraft minecraft = Minecraft.getInstance(); EnderDragon dragon = (EnderDragon) minecraft.level.getEntity(this.dragonID); dragon.getCapability(MulticoloredDragonMod.COLOR_CAPABILITY).resolve().get() .setColorName(this.colorName); } } @SubscribeEvent public void setColorOnDragonSpawnClient(@NotNull PlayerEvent.StartTracking event) { if (event.getTarget() instanceof EnderDragon dragon && event.getPlayer() instanceof ServerPlayer player) { DragonModPacketHandler.INSTANCE.send( // sync with client PacketDistributor.PLAYER.with(() -> player), new ClientBoundDragonColorPacket(dragon) ); } } -
How to achieve server/client sync for Entity data
Will Bradley replied to Will Bradley's topic in Modder Support
Oops, I should've been more vigilant with the PacketDistributor. My bad; that's fixed now. As for the rest, it compiles and seems to work— instead of being rude, would you mind telling me how dynamically registering/unregistering callbacks is a problem, and alternatives? -
How to achieve server/client sync for Entity data
Will Bradley replied to Will Bradley's topic in Modder Support
Here's my structure: I have two event handlers for this stuff, one to add data to the dragon on the server side (called on EntityJoinWorldEvent with type casting and checks for Level::isClientSide(), and one to send message to the client using PlayerEvent.StartTracking, which looks like @SubscribeEvent public void setColorOnDragonSpawnClient(@NotNull PlayerEvent.StartTracking event) { if (event.getTarget() instanceof EnderDragon dragon && event.getPlayer() instanceof ServerPlayer player) { DragonModPacketHandler.INSTANCE.send( // sync with client PacketDistributor.ALL.noArg(), new ClientBoundDragonColorPacket(dragon, player.getId()) ); } } ClientBoundDragonColorPacket looks like import ... public class ClientBoundDragonColorPacket { private final Logger LOGGER = LogManager.getLogger(); private final @Nullable String colorName; private final @Nonnull int playerID; private final int dragonID; private final Listeners listeners = new Listeners(); public ClientBoundDragonColorPacket(@NotNull EnderDragon dragon, int playerID) { this.colorName = dragon.getCapability(MulticoloredDragonMod.COLOR_CAPABILITY).resolve().get() .getColorName(); this.dragonID = dragon.getId(); this.playerID = playerID; } public ClientBoundDragonColorPacket(@NotNull FriendlyByteBuf buffer) { boolean colorNameIsNull = buffer.readBoolean(); if (colorNameIsNull) this.colorName = null; else this.colorName = new String(buffer.readByteArray()); this.dragonID = buffer.readVarInt(); this.playerID = buffer.readVarInt(); } public void write(@NotNull FriendlyByteBuf buffer) { buffer.writeBoolean(this.colorName == null); if (this.colorName != null) buffer.writeByteArray(this.colorName.getBytes()); buffer.writeVarInt(this.dragonID); buffer.writeVarInt(this.playerID); } public class Listeners { @SubscribeEvent public void changeDragonColorOnceLoadedOnClient(@NotNull EntityJoinWorldEvent event) { ClientBoundDragonColorPacket self = ClientBoundDragonColorPacket.this; if (event.getWorld().isClientSide() && event.getEntity().getId() == self.dragonID) { EnderDragon dragon = (EnderDragon) event.getEntity(); dragon.getCapability(MulticoloredDragonMod.COLOR_CAPABILITY).resolve().get() .setColorName(self.colorName); MinecraftForge.EVENT_BUS.unregister(self.listeners); } } } public void handle() { // 495 MinecraftForge.EVENT_BUS.register(this.listeners); } } -
How to achieve server/client sync for Entity data
Will Bradley replied to Will Bradley's topic in Modder Support
Ok @diesieben07 here's the issue. My entity has a random component, and if I have it join on the client and server, two different random numbers will be generated. This is a problem— how can I send a packet from the server to all clients that would load that entity, telling each client to spawn it with the proper random number? -
FileNotFoundException when loading BlockState
Will Bradley replied to Will Bradley's topic in Modder Support
It's fixed now. -
FileNotFoundException when loading BlockState
Will Bradley replied to Will Bradley's topic in Modder Support
Sorry, I tried to anonymize it by replacing my real names with dummy variables, but I wrote it at 5 AM so it was all screwy. My bad. -
FileNotFoundException when loading BlockState
Will Bradley replied to Will Bradley's topic in Modder Support
-
How to achieve server/client sync for Entity data
Will Bradley replied to Will Bradley's topic in Modder Support
Thanks! -
I have generated a blockstate .json file in src/generated/resources/assets/multicolored_dragon_mod/blockstates/multicolored_enderdragon_egg.json . My registration code in MyMod.java is as follows: public static final DeferredRegister<Block> BLOCKS = DeferredRegister.create(ForgeRegistries.BLOCKS, Constants.MODID); public static final DeferredRegister<Item> BLOCK_ITEMS = DeferredRegister.create(ForgeRegistries.ITEMS, Constants.MODID); public static RegistryObject<Block> DRAGON_EGG_BLOCK = BLOCKS.register(Constants.EGG_ID, ColoredEggBlock::new); public static RegistryObject<Item> DRAGON_EGG_BLOCK_ITEM = BLOCK_ITEMS.register(Constants.EGG_ID, () -> new BlockItem( DRAGON_EGG_BLOCK.get(), new Item.Properties().rarity(Rarity.RARE) ) ); // ... public MyMod() { // ... EntityRenderers.register(EntityType.ENDER_DRAGON, ColoredDragonRenderer::new); MinecraftForge.EVENT_BUS.register(this); BLOCKS.register(FMLJavaModLoadingContext.get().getModEventBus()); BLOCK_ITEMS.register(FMLJavaModLoadingContext.get().getModEventBus()); FMLJavaModLoadingContext.get().getModEventBus() .addListener(ModPacketHandler::register); } Yet I get the following FileNotFoundException warning on load: [05:21:54] [Worker-Main-14/WARN]: Exception loading blockstate definition: multicolored_dragon_mod:blockstates/multicolored_dragon_egg.json: java.io.FileNotFoundException: multicolored_dragon_mod:blockstates/multicolored_dragon_egg.json Looking with a debugger, I found that in SimpleReloadableResourceManager::getResources, this.namespacedPacks doesn't contain multicolored_dragon_mod, which is very strange... any help would be appreciated.
-
I am extending a vanilla entity, and for reasons beyond the scope of this post I chose to use reflection instead of subclassing my mob (EnderDragon, if it matters). At any rate, I have one event listener in my main mod class structured as follows: @SubscribeEvent public void setColorOnDragonSpawn(EntityJoinWorldEvent event) { if (!event.getWorld().isClientSide() && event.getEntity() instanceof EnderDragon dragon) { // ... more logic doThingOnServerSide(); DragonModPacketHandler.INSTANCE.send( // sync with client PacketDistributor.ALL.noArg(), new DoSameThingButOnClientSidePacket(dragon, otherArgs) ); } } For DoSameThingButOnClientSidePacket#handle, I have public void handle() { Minecraft minecraft = Minecraft.getInstance(); EnderDragon dragon = (EnderDragon) minecraft.level.getEntity(this.dragonID); // null because the client level hasn't loaded yet doStuffWithDragon(dragon); // throws NPE } What's the best way to tell DoSameThingButOnClientSidePacket#handle to run doStuffWithDragon only once the dragon is spawned in on the client? I've thought of two approaches: A package-private static final ConcurrentMap<Integer, Foo> in my main mod class, where the keys are dragon IDs (see Entity#getId) and Foo is additional metadata I want to store (a name, or color, or something. Not relevant). DoSameThingButOnClientSidePacket#handle would write this.dragonID into the map and another EntityJoinWorldEvent listener in the mod class (configured to only run when event.getWorld().isClientSide()) would pop from it and execute at the appropriate time. Can I dynamically register and unregister events? Then I could have a helper @SubscribeEvent in DoSameThingButOnClientSidePacket that is pushed onto the event bus for each instance of the packet, executes on the client side (see option #1), and then removes itself from the event bus. A built-in approach?
-
How do I make a single-player mod able to run on a server?
Will Bradley replied to Will Bradley's topic in Modder Support
Got it, thanks! And is it correct to assume most operations (say, Snowball#shoot) can be called without worrying about Level#isClientSide ? -
How do I make a single-player mod able to run on a server?
Will Bradley replied to Will Bradley's topic in Modder Support
Could you elaborate more? Specifically, is "distributions" referring to physical client vs. physical server? And what are the use cases for either method (@EventBusSubscriber vs Level#isClientSide)?