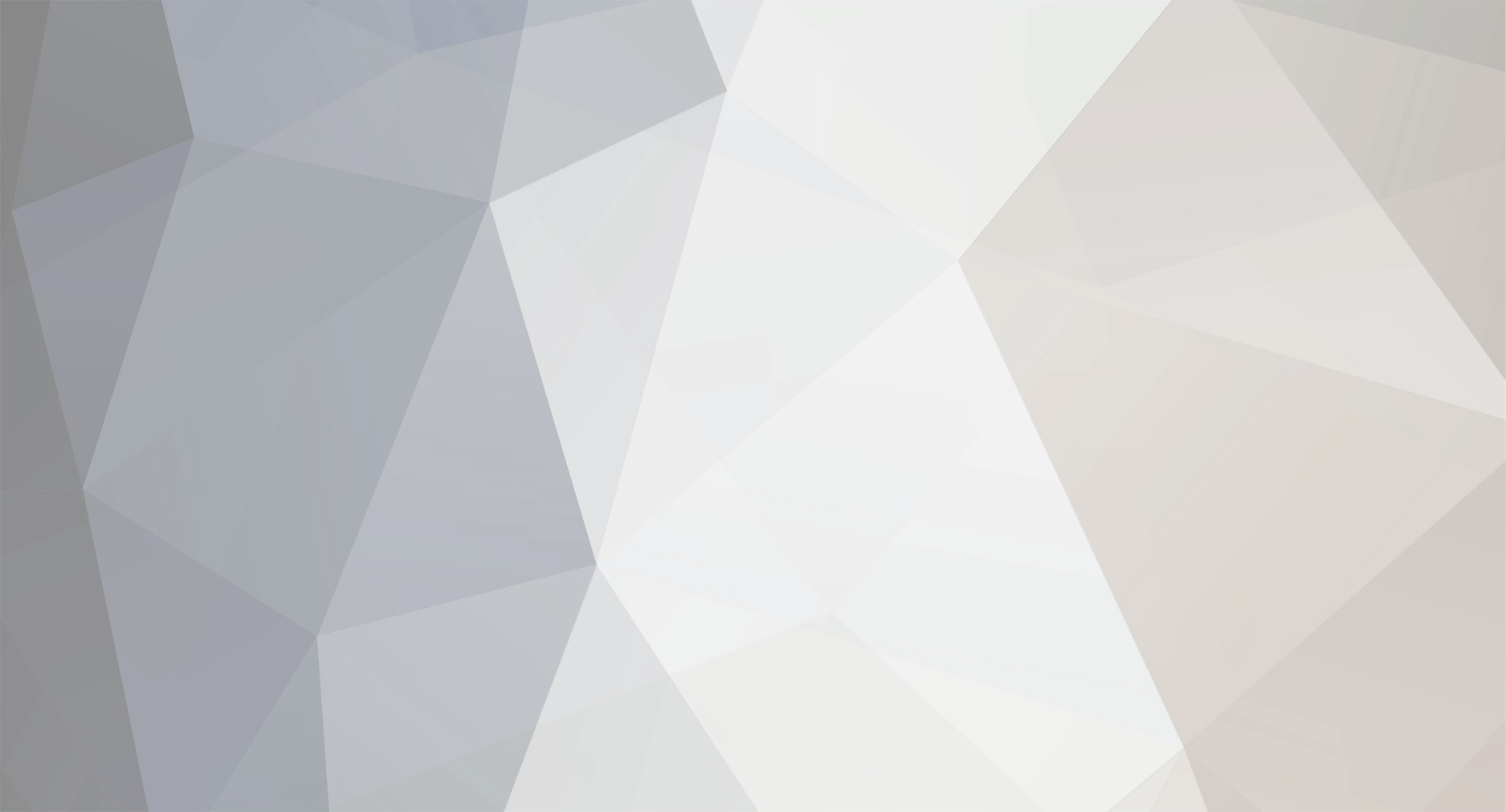
NuclearLavaLamp
Members-
Posts
8 -
Joined
-
Last visited
Everything posted by NuclearLavaLamp
-
Perfect! Thank you!
-
Good morning - Trying to determine if a block is made of wood. I've been referencing blocks by their exact type (e.g. Blocks.ACACIA_DOOR. The purpose is to have entities interact with a block if it's made of wood. However, this has two problems: - There are a lot of blocks to loop through, because I'm checking by type. This has overhead and leads to unclean code. - Mods with custom blocks wouldn't be included. Is there a generic class or tag that all wooden items (blocks, doors, etc.) use? Thanks!
-
Getting Spawner (Dungeon) Block and Position
NuclearLavaLamp replied to NuclearLavaLamp's topic in Modder Support
Thanks! I'm trying to identify if a monster spawner is placed in the world, and, where it is. I've been playing around with BiomeLoadingEvent with no luck, likely due to, as you said, the world not being loaded yet. Next, I was trying to see if I could monitor for the spawn of the spinning entity inside the spawner, hoping I could get its block position on a SpecialSpawn event. It would have been something like: public void onEntitySpawn(SpecialSpawn e) { Entity Entity_Class = e.getEntity(); if(Entity_Class.getType() == EntityType.SPINNING_SPAWNER_ENTITY) { BlockPos Block_Position = Entity_Class.Entity_Class.blockPosition(); } } What I'm trying to achieve: Find / identify where a monster spawner is if it spawns naturally in the world, like, in a dungeon. I'm not actually sure if this is possible, as I could see obvious performance issues with finding blocks through something like an x,y,z loop. I was hoping there was a built-in way or hack to find spawner blocks as they spawn in the world, but haven't found any code yet that would help. -
Sorry if this has been asked - I've been searching for a while and couldn't find an answer. Is there a performance-friendly way to grab a block if it exists in a chunk? I would assume I would use a BiomeLoadingEvent to do so? Here's pseudocode based on what I've generally been trying so far: @SubscribeEvent public void ChunkBuilt(BiomeLoadingEvent e) { //Block BLOCK_SPAWNER = GET BLOCK BY TYPE (SPAWNER) // BLOCK POSITION = BLOCK_SPAWNER.BlockPos } Basically, I'm looking to determine if a dungeon spawner has spawned, and identify its world position. Thanks!
-
Thank you! This is what I was looking for, I believe.
-
Hi guys - I'm able to duplicate entities through an EntityJoinWorldEvent. However, I'm trying to find a way to mark the duplicated entity as a clone so that it isn't clone itself when it spawns. Essentially: 1 - Entity spawns naturally 2 - Event fires to duplicate this entity. 3 - Entity Clone spawns through clone PROBLEM: 4 - The EntityJoinWorldEvent fires for the clone, causing an infinite cloning loop. Seems like EntityJoinWorldEvent fires right when the entity is cloned, and, I don't have a good way of differentiating the original entity from its clones. The current code is bad because entities duplicate on save and reload. Any idea on how to go about this? Thanks! @SubscribeEvent public void entitySpawned(EntityJoinWorldEvent e) { // Try to duplicate this monster DuplicateMonster Duplicate_Monster = new DuplicateMonster(M); } } //Causes an entity to be duplicated public class DuplicateMonster { // Directly reference a log4j logger. private static final Logger LOGGER = LogManager.getLogger(); // WIthin how many blocks should swarming entity be placed? int Swarm_Placement_Proximity = 2; // Change that entity will be duplicated float Duplication_Chance = 15; // Points where an entity was already placed so that entities are not placed on // top of each other when spawned. ArrayList<BlockPos> Used_Points = new ArrayList<BlockPos>(); // These monsters are allowed to be duplicated. EntityType[] Duplication_Whitelist = { EntityType.BLAZE, EntityType.CAVE_SPIDER, EntityType.DROWNED, EntityType.ENDERMAN, EntityType.EVOKER, EntityType.GHAST, EntityType.HOGLIN, EntityType.HUSK, EntityType.PIGLIN, EntityType.PIGLIN_BRUTE, EntityType.PILLAGER, EntityType.SILVERFISH, EntityType.SKELETON, EntityType.SPIDER, EntityType.RAVAGER, EntityType.VEX, EntityType.VINDICATOR, EntityType.WITCH, EntityType.WITHER_SKELETON, EntityType.ZOGLIN, EntityType.ZOMBIE, EntityType.ZOMBIE_VILLAGER, EntityType.ZOMBIFIED_PIGLIN }; // Duplicate the given monster // M - the monster to duplicate public DuplicateMonster(Monster M) { // If this monster is on the duplication whitelist, then if (isWhitelisted(M)) { RNG RNGVal = new RNG(0, 100); if(Duplication_Chance > RNGVal.Value) { LOGGER.info("ADDING CLONES "); // For number of cloning iterations, clone Clone(M); } } } // Clone the given monster // M - Monster to clone. void Clone(Monster M) { // Current Minecraft server MinecraftServer Curr_Server = ServerLifecycleHooks.getCurrentServer(); ServerLevel Lvl = Curr_Server.getLevel(Level.OVERWORLD); // Get entity block position BlockPos pos = M.blockPosition(); LOGGER.info("CREATE NEW CLONE..."); Entity New_Entity = M.getType().spawn(Lvl, new ItemStack(Items.BIRCH_WOOD), (Player) null, pos, MobSpawnType.MOB_SUMMONED, true, false); } // Is the given monster allowed to be duplicated? // M: Check if M is on the white listed of monsters that can be duplicated boolean isWhitelisted(Monster M) { for (int i = 0; i < Duplication_Whitelist.length; i++) { if (Duplication_Whitelist[i] == M.getType()) { // Monster is on the white list return true; } } // This monster is not on the white list return false; } }
-
Apologies if this is a common topic. I've searched through topics on this forum and on Google, and the code I've found is mostly deprecated. I'm currently trying to get the server instance using the below code: I'm guessing this is the incorrect way to grab the server instance, as it's mostly null? What would be a better way, instead? Any documentation that might help on this? This class is invoked from a LivingEvent event, and "Attacker" is always a spawned Monster. Thanks!