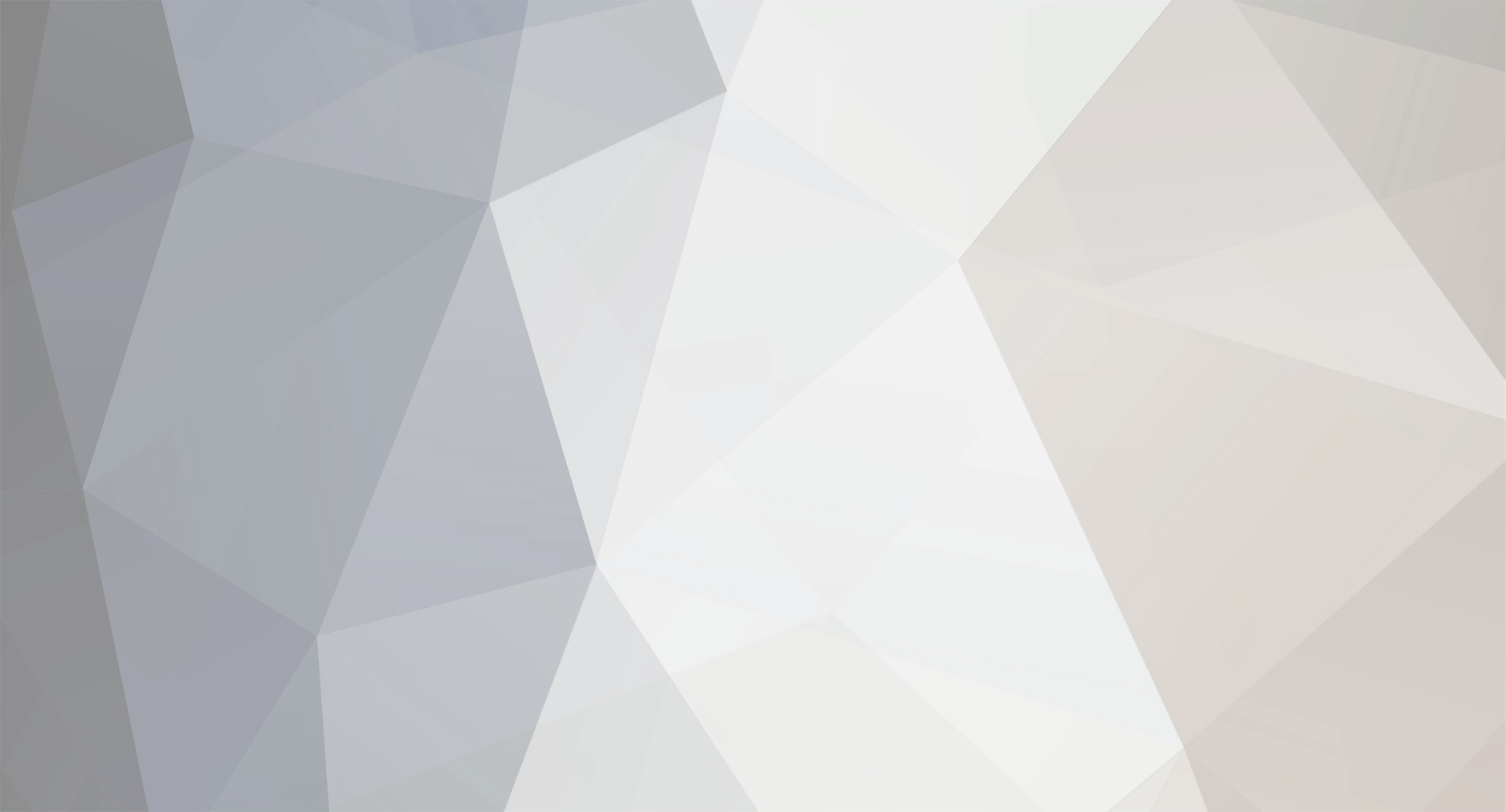
Robo51
Forge Modder-
Posts
53 -
Joined
-
Last visited
Everything posted by Robo51
-
Okay I have the packets working, and now I'm stuck on figuring out the key state. I have the water movement working fine for climbing out but at the moment it stays that way because I haven't figured out how to properly do the unpressed part. Ive tried doing this: KeyHandler: And this is the message recevier: But it always prints out a setting to false, I'm assuming this is because I've done something weird with the logic. Also in the keyhandler I'm using KeyInputEvent but its overwriting vanilla behavior (just the space-bar, I'm not able to jump), should I be using a different event in its place?
-
Thank you for that. I started from scratch and the keybinds work great now. I move onto the packets and I'm stuck at what I actually send in the packet. This is the KeyHandler: Now in the sendToServer(); bit what do I actually put in the parenthesis? Is it something I choose or does it need to be something specific?
-
How would I go about properly registering the KeyInputHandler in the ClientProxy then?
-
This is the KeyInputHandler code: package robo51.newt.handlers; import net.minecraft.client.settings.KeyBinding; import robo51.newt.proxy.ClientProxy; import cpw.mods.fml.common.eventhandler.EventPriority; import cpw.mods.fml.common.eventhandler.SubscribeEvent; import cpw.mods.fml.common.gameevent.InputEvent.KeyInputEvent; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; public class KeyInputHandler { @SideOnly(Side.CLIENT) @SubscribeEvent(priority=EventPriority.NORMAL, receiveCanceled=true) public void onEvent(KeyInputEvent event) { System.out.println("Key Input Event"); KeyBinding[] keyBindings = ClientProxy.keyBindings; if (keyBindings[0].isPressed()) { //line 19 System.out.println("Key binding ="+keyBindings[0].getKeyDescription()); // do stuff for this key binding here // remember you may need to send packet to server } if (keyBindings[1].isPressed()) { System.out.println("Key binding ="+keyBindings[1].getKeyDescription()); // do stuff for this key binding here // remember you may need to send packet to server } } }
-
I feel like I'm still missing something. I've moved the register KeyInputHandler into the ClientProxy: And still get the same error from the client upon test: Am I just repeating myself at this point?
-
Is: @EventHandler public void fmlLifeCycleEvent(FMLInitializationEvent event) Similar to: @Mod.EventHandler public void init(FMLInitializationEvent event) { Because this is what my main class looks like right now:
-
Okay now I'm kinda confused Ive moved to the ClientProxy: And this is what in now in the KeyInputHandler: And now the client errors the moment I press any key, I feel like its going to smack me in the face when I figure it out. But I've got no idea now.
-
So I've been working through the key binding and hit a small snag. The key binding works fine for the client but the server doesn't like it. I feel like this has to do with which side the key bindings need to be on, but one of the tutorials Ive been reading through mentioned it. This is the KeyBindingHandler: And this is the KeyInputHandler: The server doesn't like this line in the bindinghandler:
-
Okay so I fixed up my playerTickEvent and got that all working. And now I'm working on the next bit that handles water movement and requires a packet. This is what I have so far: Inside of the event handler: And this is in the NewtMessage class: I left the response for the packet as what came out of the tutorial for the moment so I could get the packet working first. However I'm not sure what all needs to be transferred in the packet, I think I need the player? And If I do need the player how do I properly transfer that since what I'm currently using doesn't work.
-
So I decided to redo this little section of code as I always find it easiest to start over. I've started with this: However the player takes no damage when in water or rain. Am I referencing the player wrong here?
-
1. What is the difference between the START and END phase? I can't seem to find anything really explaining them. 2. I do remember the keybind problem I forgot to comment it out in the version I posted as I plan to try really hard to understand packets and how they work with the help of tutorials. 3. Those numbers are somewhat arbitrary at the moment
-
I finally got off my lazy butt and decided to work on my mod again, and I am currently working to fix all the "little" things first. So I have an event that causes the player to take damage in the water if a value is <= 0. Event: However this doesn't seem to do anything now, (it used to work but I remember changing the event a while back). I feel like I'm either using the wrong event for this or not using the current event properly. If someone could help me out with this, maybe point me in the right direction it would be much appreciated.
-
Would anyone be able to help with this?
-
Maybe I'll explain what I'm tying to accomplish better. I'm changing how the player reacts with water, in my EventsHandler: This block of code: if(event.player.isWet()) { int watersealing = ExtendedPlayerWater.getCurrentWaterSealing(); if(watersealing <= 0) { event.player.attackEntityFrom(DamageSource.drown, 1.0F); } else { --watersealing; ExtendedPlayerWater.newCurrentWaterSealing(watersealing); if(watersealing == 200) { if(!event.player.worldObj.isRemote) { player.addChatMessage(new ChatComponentText("5 Seconds left of WaterSealing.")); } } if(watersealing == 50) { if(!event.player.worldObj.isRemote) { player.addChatMessage(new ChatComponentText("1 Second left of WaterSealing!")); } } } } I'm using to check if the player is in contact with water. (I have an item that adds water sealing to the player via an item.) If they have no water sealing they take damage, otherwise watersealing ticks down, when the sealing hits a certain level a chat message is sent to the player to warn them of imminent failure. In this block: if(event.entity.isInWater()) { event.entity.setAir(10); if(Keyboard.isKeyDown(57)) { event.entity.motionY = 0.005D; if(event.entity.isCollidedHorizontally) { event.entity.motionY = 0.2D; } } else { event.entity.motionY = -1.0D; } } I'm modifying the players movement in water making them sink straight to the bottom. They can swim up but it's slow unless pressed up against a block for leverage. And that's where the problem came from. The server not liking the isKeyDown part. I apologize for not knowing very much about packets and the like. I'm new to java coding and I'm trying to learn through modding. Also if it helps I have a github for this, it can be found here: https://github.com/Robo51/Newt
-
Thank you very much for the world help, It's much appreciated Would you perhaps be able to help me with the packet? I'm having trouble understanding where I would need to break off in this code: I know the server doesn't handle key-binds, does that mean the packet should contain the isKeyDown or would it be everything after?
-
So I been working on this again a bit and worked through a few things. Now I'm having trouble figuring out how to properly implement the packet sending into what I have already. This is my eventshandler: This is the message that i'm trying to use: I also added a KeyHandler for later, not sure if I need something else in this: I've also been having trouble with the chat components in the EventsHandler, I believe it has to to with the world parameter that I added to prevent double chat messages. How would I properly implement the packet? I'm just not sure what the server and the client need to handle. And some help with the world/chat problem would be much appreciated. Also, Happy Halloween!
-
So after a few busy days I had a chance to sit down and try and work this out, and I feel like I have hit a wall with my face. I ended up using Ablaze's How to use SimpleNetworkWrapper, IMessage and IMessageHandler tutorial. I have these two classes from that: NewtMessageHandler NewtMessage But I'm confused as to what I am supposed to put in these. I think I need to send a packet from my event handler using these to the server? But what do I need to send? Do I send the Keyboard.isKeyDown? And then does my event handler get somethings back to execute the code or is that supposed to happen somewhere else? I feel like these are easy questions to answer, but I'm not capable of answering them.
-
I see, I have no idea what that would entail.
-
I've been working through and learning how to use events and I've run into a problem. This is the code in question: This works fine in single player but throws and error in multiplayer: Now after doing a little looking around I've figured out that I could register a key binder to help fix this. But I'm wondering it there is an easier way since I'm just trying to check for the space-bar (or jump key) press.
-
Thank you that's much appreciated.
-
I've started to use variables to store information about players. Example: However the problem with this code is that it's obviously a global variable that applies to everyone. And I would like each player to have their own value for this variable. What would I need to do make this individual to each player? And what is the best way to store info for this kind of thing? Would I use the player file for this or is there a way to write a file of my own?(I plan on eventually storing a fairly good chunk of info.)
-
Any help on this would be appreciated.
-
Thank you. Using what you gave me and some examples from a tutorial it works now. Much appreciated.
-
So after some fiddling I came up with this: However I realize that this is not an event and won't actually work. I'm confused on the event to use and how to use it. Would I use this event? And if so I'm still a little confused on how to use it properly:
-
I'm trying to make the player take damage when they come in contact with water, however I'm not entirely sure what I'm doing wrong. I've registered an EventsHandler for my mod and I believe I need to use onPlayerTick to do what I need to do. (Total shot in the dark) This is the code I'm using: However this does not work,which I figured it wouldn't. I think I need it to ?reference? the entity class for the .iswet and .attackEntityFrom methods. But I'm not sure how to do so. Am I headed in the right direction or am I backasswards?