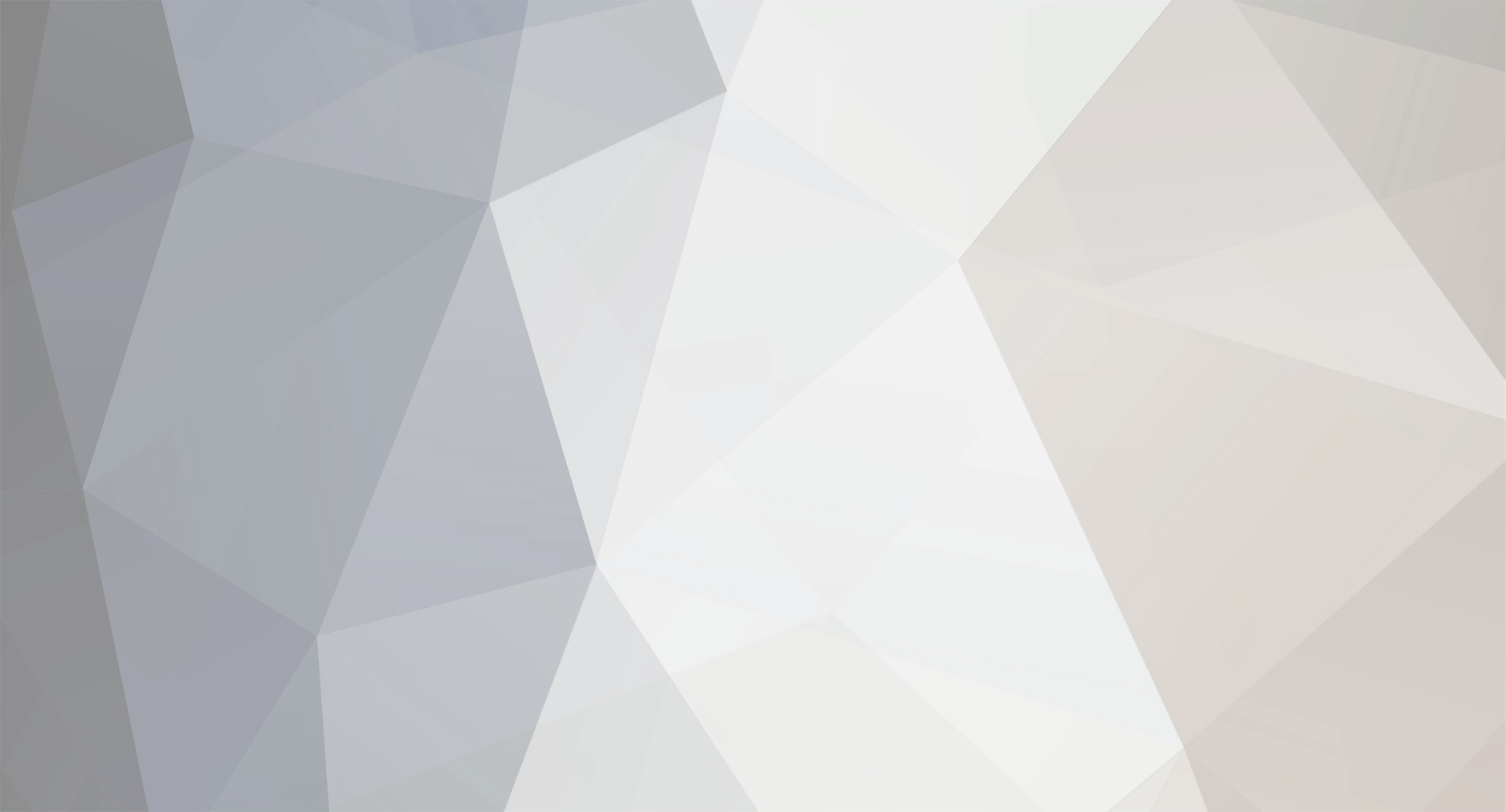
CoddingDirtMC
Members-
Posts
24 -
Joined
-
Last visited
Converted
-
Gender
Male
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
CoddingDirtMC's Achievements

Tree Puncher (2/8)
0
Reputation
-
Here is the full code: package item; import net.minecraft.core.BlockPos; import net.minecraft.world.InteractionResult; import net.minecraft.world.item.Item; import net.minecraft.world.item.context.UseOnContext; import net.minecraft.world.level.block.BaseFireBlock; import net.minecraft.world.level.block.state.BlockState; import net.minecraft.world.level.gameevent.GameEvent; public class FFSS extends Item { public FFSS(Properties p_41383_) { super(p_41383_); } @Override public InteractionResult useOn(UseOnContext context) { BlockPos pos1 = new BlockPos(context.getClickedPos().getX(), context.getClickedPos().getY() + 1, context.getClickedPos().getZ()); BlockPos pos2 = new BlockPos(pos1.getX() + 1, pos1.getY(), pos1.getZ()); BlockPos pos3 = new BlockPos(pos1.getX(), pos1.getY(), pos1.getZ() + 1); BlockPos pos4 = new BlockPos(pos1.getX() + 1, pos1.getY(), pos1.getZ() + 1); BlockState bs1 = BaseFireBlock.getState(context.getLevel(), pos1); BlockState bs2 = BaseFireBlock.getState(context.getLevel(), pos2); BlockState bs3 = BaseFireBlock.getState(context.getLevel(), pos3); BlockState bs4 = BaseFireBlock.getState(context.getLevel(), pos4); context.getLevel().setBlock(pos1, bs1, 1); context.getLevel().setBlock(pos2, bs2, 1); context.getLevel().setBlock(pos3, bs3, 1); context.getLevel().setBlock(pos4, bs4, 1); context.getLevel().gameEvent(context.getPlayer(), GameEvent.BLOCK_PLACE, pos1); context.getLevel().gameEvent(context.getPlayer(), GameEvent.BLOCK_PLACE, pos2); context.getLevel().gameEvent(context.getPlayer(), GameEvent.BLOCK_PLACE, pos3); context.getLevel().gameEvent(context.getPlayer(), GameEvent.BLOCK_PLACE, pos4); return InteractionResult.sidedSuccess(context.getLevel().isClientSide()); } } And here is the register code: package item; import net.minecraft.world.item.CreativeModeTab; import net.minecraft.world.item.Item; import net.minecraftforge.registries.DeferredRegister; import net.minecraftforge.registries.ForgeRegistries; import net.minecraftforge.registries.RegistryObject; import com.elliot.juststeel.*; public class ItemInit { public static final DeferredRegister<Item> ITEMS = DeferredRegister.create(ForgeRegistries.ITEMS, JustSteel.MODID); public static final RegistryObject<Item> STEELSTEEL = ITEMS.register("steelsteel", () -> new Item(new Item.Properties().tab(CreativeModeTab.TAB_TOOLS).stacksTo(1))); public static final RegistryObject<Item> FLINTFLINT = ITEMS.register("flintflint", () -> new FlintFlint(new Item.Properties().tab(CreativeModeTab.TAB_TOOLS).stacksTo(1))); public static final RegistryObject<Item> FLINTFLINTSTEELSTEEL = ITEMS.register("flintflintsteelsteel", () -> new FFSS(new Item.Properties().tab(CreativeModeTab.TAB_TOOLS).stacksTo(1))); } I did look at the FlintAndSteelItem class but most of the difference was sound which I don't really care about.
-
Looking for a modmaker who can give me an hand
CoddingDirtMC replied to VladimirVoid's topic in General Discussion
How experienced are you at mc moding? -
Hi, I am trying to make a my own version of a flint and steel but changed up a bit. It is supposed to spawn 4 fire blocks in a 2 by 2 area but it is only spawning the fire that I am looking at. Can you help me? Here is my code. package item; import net.minecraft.core.BlockPos; import net.minecraft.world.InteractionResult; import net.minecraft.world.item.Item; import net.minecraft.world.item.context.UseOnContext; import net.minecraft.world.level.block.BaseFireBlock; import net.minecraft.world.level.block.state.BlockState; import net.minecraft.world.level.gameevent.GameEvent; public class FFSS extends Item { public FFSS(Properties p_41383_) { super(p_41383_); } @Override public InteractionResult useOn(UseOnContext context) { BlockPos pos1 = new BlockPos(context.getClickedPos().getX(), context.getClickedPos().getY() + 1, context.getClickedPos().getZ()); BlockPos pos2 = new BlockPos(pos1.getX() + 1, pos1.getY(), pos1.getZ()); BlockPos pos3 = new BlockPos(pos1.getX(), pos1.getY(), pos1.getZ() + 1); BlockPos pos4 = new BlockPos(pos1.getX() + 1, pos1.getY(), pos1.getZ() + 1); BlockState bs1 = BaseFireBlock.getState(context.getLevel(), pos1); BlockState bs2 = BaseFireBlock.getState(context.getLevel(), pos2); BlockState bs3 = BaseFireBlock.getState(context.getLevel(), pos3); BlockState bs4 = BaseFireBlock.getState(context.getLevel(), pos4); context.getLevel().setBlock(pos1, bs1, 1); context.getLevel().setBlock(pos2, bs2, 1); context.getLevel().setBlock(pos3, bs3, 1); context.getLevel().setBlock(pos4, bs4, 1); context.getLevel().gameEvent(context.getPlayer(), GameEvent.BLOCK_PLACE, pos1); context.getLevel().gameEvent(context.getPlayer(), GameEvent.BLOCK_PLACE, pos2); context.getLevel().gameEvent(context.getPlayer(), GameEvent.BLOCK_PLACE, pos3); context.getLevel().gameEvent(context.getPlayer(), GameEvent.BLOCK_PLACE, pos4); return super.useOn(context); } }
-
ok thank you
-
Im in 1.18.2, where would I put/set the min and max y?
-
what do I put in the Entity parameter?
-
Hello, I was wondering how I could look and see if there was an entity on or near a position. I haven't tried anything yet and I don't know what to do.
-
[1.18.2] RenderType not doing anything
CoddingDirtMC replied to CoddingDirtMC's topic in Modder Support
oh wait uhm I already fixed this afterwards and now I dont know how to delete the topic -
for me, this worked in 1.18 but if you use noOcclusion() in the block properties it should work
-
Hi guys, I've been trying for weeks now. Going to different topics. I was trying to make my teleporter block not cull block sides around it. I've tried many things. None of them work. Can anyone help me
-
you're reading my mind I just did that now and it STILL didnt work package util; import init.BlockInit; import com.example.eitem.ElliotItems; import net.minecraft.client.renderer.ItemBlockRenderTypes; import net.minecraft.client.renderer.RenderType; import net.minecraftforge.eventbus.api.SubscribeEvent; import net.minecraftforge.fml.event.lifecycle.FMLClientSetupEvent; import net.minecraftforge.api.distmarker.Dist; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.Mod.EventBusSubscriber.Bus; @Mod.EventBusSubscriber(modid = ElliotItems.MOD_ID, bus = Bus.MOD, value = Dist.CLIENT) public class ClientEventBusSubscriber { @SubscribeEvent public static void clientSetup(FMLClientSetupEvent event) { ItemBlockRenderTypes.setRenderLayer(BlockInit.TELEPORTER.get(), RenderType.cutout()); } }