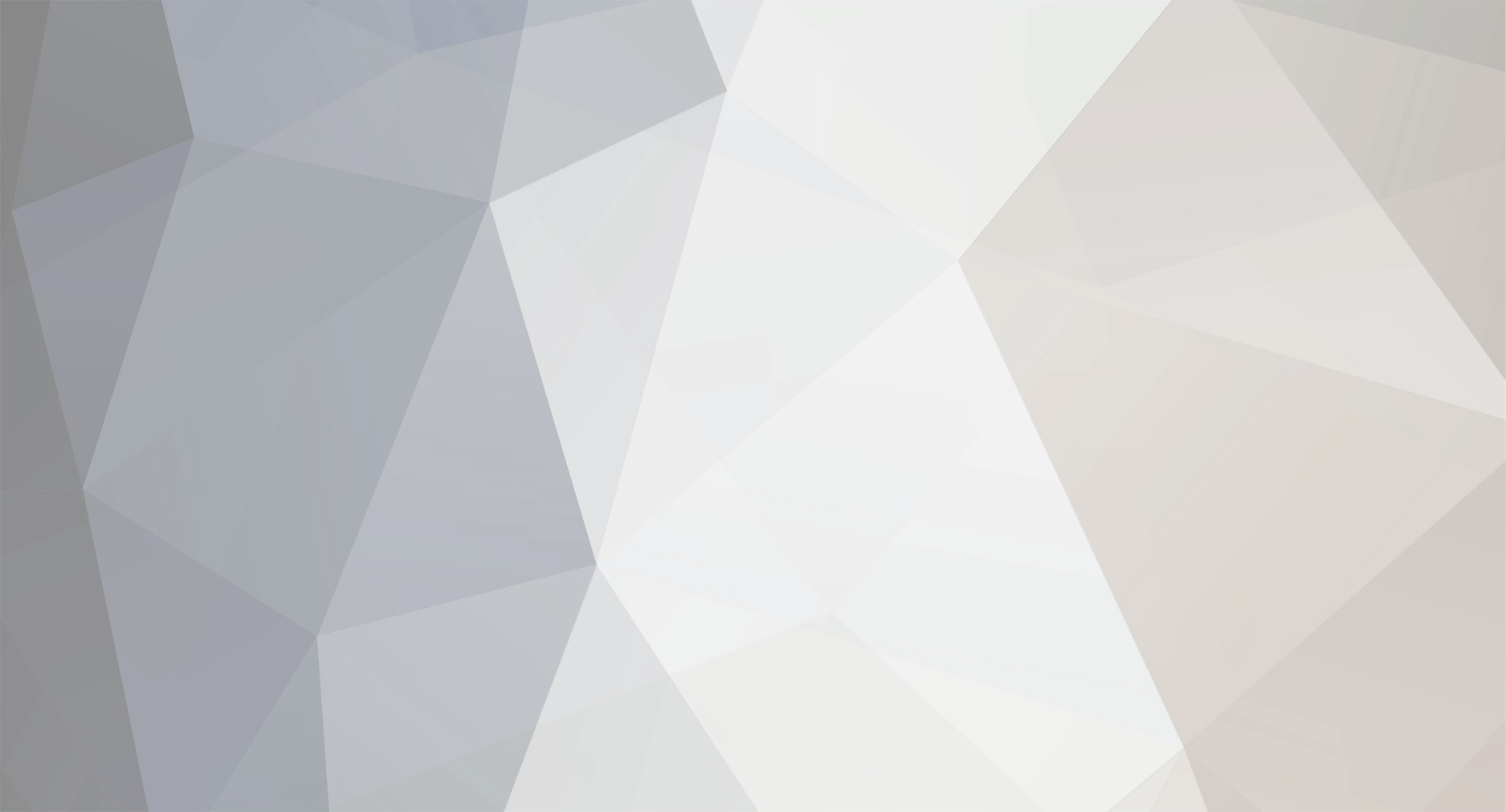
SnakerBone
Members-
Posts
12 -
Joined
-
Last visited
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
SnakerBone's Achievements

Tree Puncher (2/8)
0
Reputation
-
Hi, So I've been wanting to try and find out how to have a custom block renderer without having to use a block entity (for optimization purposes). My actual block does not actually use anything block entity related (like saving and loading data). It's really only a block entity for the custom renderer. If normal blocks can have their own custom renderer without needing a block entity then how would I go about doing this? To be more in depth on what I'm actually doing; I have GLSL shaders and I'm basically wanting to render the shader as simple block (Here is my utility class that draws the block). Thanks
-
Hi! I've been trying to use external libraries like JOML (for animating) and Jsoup (for html parsing), It compiles fine, however at runtime if I call a class that uses one of these external libraries it crashes my game saying "ClassNotFoundException: {external library maven url here}". Here's my relevant code: // build.gradle dependencies { minecraft "net.minecraftforge:forge:${mc_version}-43.2.0" // Other dependencies... implementation "org.joml:joml:${joml_version}" implementation "org.jsoup:jsoup:${jsoup_version}" } // gradle.properties joml_version=1.10.5 jsoup_version=1.16.1 // Class using external joml library (crashes when I call this method) public Quaternionf toQuatf(float x, float y, float z, float w) { return new Quaternionf(x, y, z, w); } If I'm missing something please let me know! I'm not so familiar with Gradle as I originally used maven Poms/XMLs with Java xD
-
[1.19.2] BlockEntity invisible when placed by world gen
SnakerBone replied to SnakerBone's topic in Modder Support
I figured it out! I had to change the buffer size on the render type from 256 to Integer.POSITIVE_INFINITY. This gives it an infinite buffer size with no limit. Though it makes the game EXTREMELY laggy (obviously). So I've scrapped the original idea I had for world gen with shader blocks and moved on because I'm not really the biggest fan of trying to optimise shaders as I hate coding in C++ with a passion lol -
[1.19.2] BlockEntity invisible when placed by world gen
SnakerBone replied to SnakerBone's topic in Modder Support
Bump -
[1.19.2] BlockEntity invisible when placed by world gen
SnakerBone replied to SnakerBone's topic in Modder Support
Bump -
[1.19.2] BlockEntity invisible when placed by world gen
SnakerBone replied to SnakerBone's topic in Modder Support
Bump ,,, -
[1.19.2] BlockEntity invisible when placed by world gen
SnakerBone replied to SnakerBone's topic in Modder Support
Bump -
Hi again, I'm having trouble with my block entity being invisible when placed by world gen, It works fine when Its placed by a player. My block also uses a shader for its texture. It looks like this in game: Here's my current code: // Biome import com.mojang.datafixers.util.Pair; import net.minecraft.core.Registry; import net.minecraft.data.worldgen.BiomeDefaultFeatures; import net.minecraft.resources.ResourceKey; import net.minecraft.resources.ResourceLocation; import net.minecraft.sounds.Music; import net.minecraft.util.Mth; import net.minecraft.world.entity.EntityType; import net.minecraft.world.entity.Mob; import net.minecraft.world.entity.MobCategory; import net.minecraft.world.level.biome.*; import net.minecraft.world.level.block.Block; import net.minecraft.world.level.levelgen.SurfaceRules; import net.minecraftforge.registries.RegistryObject; import snaker.snakerbone.registry.SnakerBoneContentRegistry; import snaker.snakerbone.registry.SnakerBoneEntityRegistry; import snaker.snakerbone.registry.SnakerBoneWorldGenRegistry; import terrablender.api.Region; import terrablender.api.RegionType; import javax.annotation.Nullable; import java.util.function.Consumer; import static net.minecraft.world.level.biome.Biomes.PLAINS; import static snaker.snakerbone.util.SnakerBonePolicy.WarningSuppression.CUSTOM_SET; import static snaker.snakerbone.util.SnakerBoneTools.hexToInt; public class ShaderPlains { public static Biome getBiome() { MobSpawnSettings.Builder SpawnBuilder = new MobSpawnSettings.Builder(); BiomeGenerationSettings.Builder BiomeBuilder = new BiomeGenerationSettings.Builder(); addMobSpawn(SpawnBuilder, MobCategory.MONSTER, SnakerBoneEntityRegistry.COSMO, 15, 1, 3); addMobSpawn(SpawnBuilder, MobCategory.MONSTER, SnakerBoneEntityRegistry.FLARE, 15, 1, 3); return generateBiome(Biome.Precipitation.NONE, 0.8F, 0, hexToInt("18ADBD"), hexToInt("58AAB2"), hexToInt("064C53"), SpawnBuilder, BiomeBuilder, AmbientMoodSettings.LEGACY_CAVE_SETTINGS, null); } protected static int calculateSkyColor(float colour) { float hsv = colour / 3; hsv = Mth.clamp(hsv, -1, 1); return Mth.hsvToRgb(0.62222224F - hsv * 0.05F, 0.5F + hsv * 0.1F, 1); } private static Biome generateBiome(Biome.Precipitation precipitation, float temperature, float downfall, int fogColour, int waterColour, int waterFogColour, MobSpawnSettings.Builder spawnBuilder, BiomeGenerationSettings.Builder biomeBuilder, AmbientMoodSettings mood, @Nullable Music music) { return new Biome.BiomeBuilder().precipitation(precipitation).temperature(temperature).downfall(downfall).specialEffects(new BiomeSpecialEffects.Builder().waterColor(waterColour).waterFogColor(waterFogColour).fogColor(fogColour).skyColor(calculateSkyColor(temperature)).ambientMoodSound(mood).backgroundMusic(music).build()).mobSpawnSettings(spawnBuilder.build()).generationSettings(biomeBuilder.build()).build(); } private static <M extends Mob> void addMobSpawn(MobSpawnSettings.Builder builder, MobCategory category, EntityType<M> type, int weight, int min, int max) { builder.addSpawn(category, new MobSpawnSettings.SpawnerData(type, weight, min, max)); BiomeDefaultFeatures.commonSpawns(builder); } private static <M extends Mob> void addMobSpawn(MobSpawnSettings.Builder builder, MobCategory category, RegistryObject<EntityType<M>> type, int weight, int min, int max) { builder.addSpawn(category, new MobSpawnSettings.SpawnerData(type.get(), weight, min, max)); BiomeDefaultFeatures.commonSpawns(builder); } private static void addDefaultFeatures(BiomeGenerationSettings.Builder builder) { BiomeDefaultFeatures.addDefaultOres(builder); BiomeDefaultFeatures.addDefaultSoftDisks(builder); BiomeDefaultFeatures.addExtraEmeralds(builder); BiomeDefaultFeatures.addInfestedStone(builder); BiomeDefaultFeatures.addDefaultCarversAndLakes(builder); BiomeDefaultFeatures.addDefaultCrystalFormations(builder); BiomeDefaultFeatures.addDefaultUndergroundVariety(builder); BiomeDefaultFeatures.addDefaultSprings(builder); } public static class RuleData { private static final SurfaceRules.RuleSource SWIRL_BLOCK = makeStateRule(SnakerBoneContentRegistry.SWIRL_BLOCK); private static final SurfaceRules.RuleSource STARS_BLOCK = makeStateRule(SnakerBoneContentRegistry.STARS_BLOCK); private static final SurfaceRules.RuleSource WATERCOLOUR_BLOCK = makeStateRule(SnakerBoneContentRegistry.WATERCOLOUR_BLOCK); public static SurfaceRules.RuleSource getRules() { SurfaceRules.ConditionSource WaterBlockCheck = SurfaceRules.waterBlockCheck(-1, 0); SurfaceRules.RuleSource surface = SurfaceRules.sequence(SurfaceRules.ifTrue(WaterBlockCheck, STARS_BLOCK), SWIRL_BLOCK); return SurfaceRules.sequence(SurfaceRules.ifTrue(SurfaceRules.isBiome(SnakerBoneWorldGenRegistry.SHADER_PLAINS), WATERCOLOUR_BLOCK), SurfaceRules.ifTrue(SurfaceRules.ON_FLOOR, surface)); } private static SurfaceRules.RuleSource makeStateRule(RegistryObject<Block> block) { return SurfaceRules.state(block.get().defaultBlockState()); } private static SurfaceRules.RuleSource makeStateRule(Block block) { return SurfaceRules.state(block.defaultBlockState()); } } public static class BiomeRegion extends Region { public BiomeRegion(ResourceLocation name, int weight) { super(name, RegionType.OVERWORLD, weight); } @Override public void addBiomes(Registry<Biome> registry, Consumer<Pair<Climate.ParameterPoint, ResourceKey<Biome>>> mapper) { this.addModifiedVanillaOverworldBiomes(mapper, builder -> builder.replaceBiome(PLAINS, SnakerBoneWorldGenRegistry.SHADER_PLAINS)); } } } // BlockEntity import net.minecraft.core.BlockPos; import net.minecraft.world.level.block.entity.BlockEntity; import net.minecraft.world.level.block.state.BlockState; import snaker.snakerbone.registry.SnakerBoneContentRegistry; import software.bernie.geckolib3.core.IAnimatable; import software.bernie.geckolib3.core.PlayState; import software.bernie.geckolib3.core.controller.AnimationController; import software.bernie.geckolib3.core.event.predicate.AnimationEvent; import software.bernie.geckolib3.core.manager.AnimationData; import software.bernie.geckolib3.core.manager.AnimationFactory; import software.bernie.geckolib3.util.GeckoLibUtil; import static snaker.snakerbone.util.SnakerBonePolicy.WarningSuppression.CUSTOM_SET; public class WaterColourBlockEntity extends BlockEntity implements IAnimatable { private final AnimationFactory FACTORY = GeckoLibUtil.createFactory(this); public WaterColourBlockEntity(BlockPos pos, BlockState state) { super(SnakerBoneContentRegistry.WATERCOLOUR_BE.get(), pos, state); } private <E extends IAnimatable> PlayState predicate(AnimationEvent<E> entity) { return PlayState.CONTINUE; } @Override public void registerControllers(AnimationData data) { data.addAnimationController(new AnimationController<>(this, "controller", 0, this::predicate)); } @Override public AnimationFactory getFactory() { return FACTORY; } } // Block import net.minecraft.core.BlockPos; import net.minecraft.world.level.block.BaseEntityBlock; import net.minecraft.world.level.block.RenderShape; import net.minecraft.world.level.block.entity.BlockEntity; import net.minecraft.world.level.block.state.BlockState; import org.jetbrains.annotations.Nullable; import snaker.snakerbone.registry.SnakerBoneContentRegistry; import static net.minecraft.world.level.material.Material.METAL; import static snaker.snakerbone.util.SnakerBonePolicy.WarningSuppression.CUSTOM_SET; public class WaterColourBlock extends BaseEntityBlock { public WaterColourBlock() { super(Properties.of(METAL).requiresCorrectToolForDrops().strength(5)); } @Nullable @Override public BlockEntity newBlockEntity(BlockPos pos, BlockState state) { return SnakerBoneContentRegistry.WATERCOLOUR_BE.get().create(pos, state); } @Override public RenderShape getRenderShape(BlockState pState) { return RenderShape.ENTITYBLOCK_ANIMATED; } }
-
[1.19.2] Custom shader not rendering on BlockItem
SnakerBone replied to SnakerBone's topic in Modder Support
Figured it out. I had to put "parent": "builtin/entity" in the block models json. My bad XD -
[1.19.2] Custom shader not rendering on BlockItem
SnakerBone replied to SnakerBone's topic in Modder Support
Hi, thanks for your reply. I have done this however I am still having the same problem. Here's my new code: I'm think I might be missing something. Please let me know if that's the case -
Hi, so I recently made a shader with OpenGL and I'm able to get it to render on the block in the world but I'm having trouble trying to get the actual BlockItem to render. I'm also using GeckoLib & CodeChickenLib, heres what it looks like in game: Here's my code: I'm also not missing any block/item JSON's so I'm kind of confused. I have been able to get this working in 1.16 but I cant seem to get it working in 1.19.2 Any help appreciated. Thanks ๐