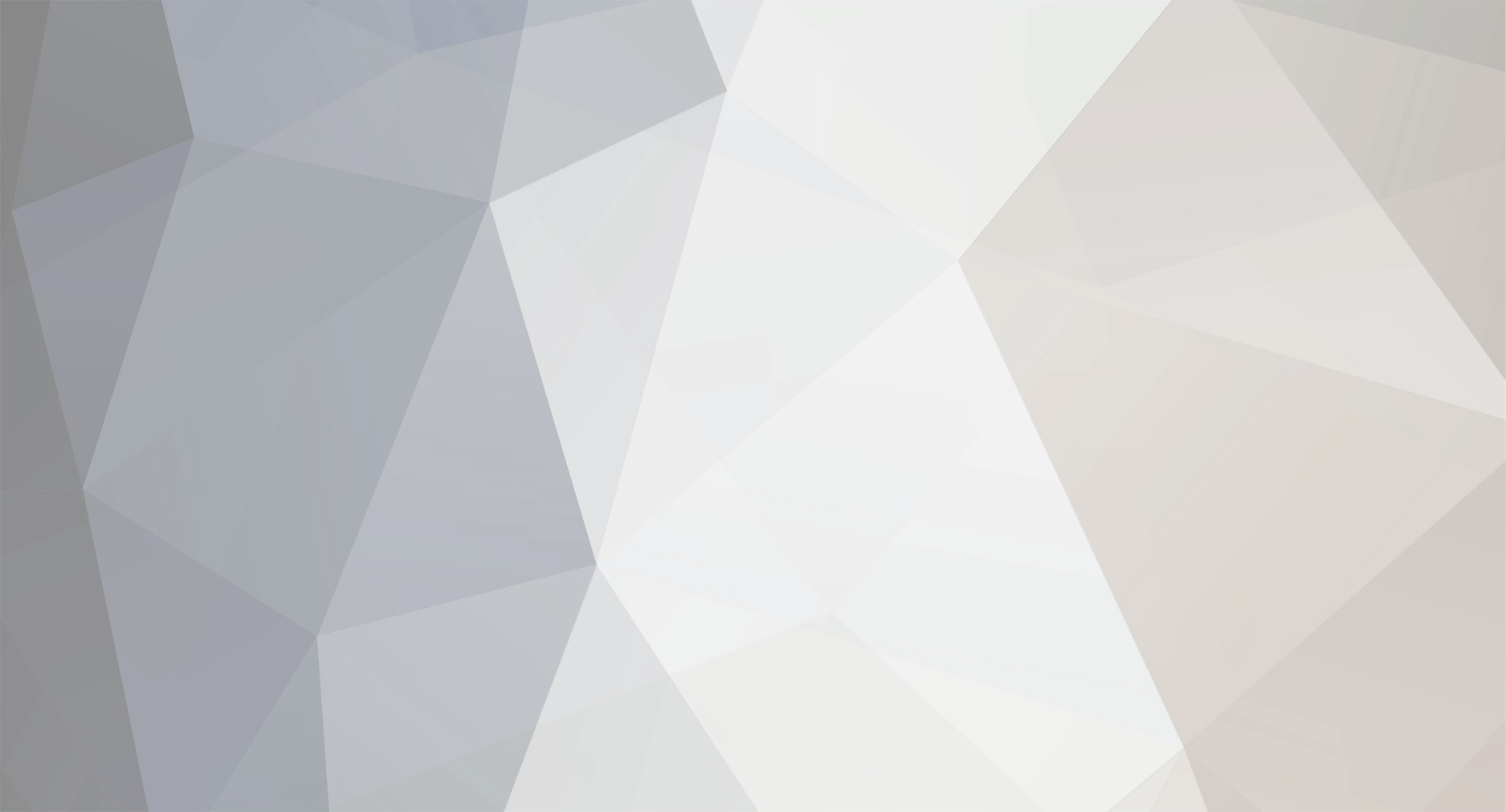
DaBananaboat
Members-
Posts
14 -
Joined
-
Last visited
Everything posted by DaBananaboat
-
[1.7.10] How to update item when right clicking a block?
DaBananaboat replied to DaBananaboat's topic in Modder Support
I do, otherwise it'll be fired twice. Once on the client and once on the server. Playing with that, I fixed the issue. The only thing that had to be checked for isRemote was the following block: if(!world.isRemote) { player.addChatMessage(new ChatComponentText("You have rested at this bonfire.")); } Thanks Alexiy -
I have an item that's being refilled when right clicking a block. However on the client it doesn't update until I use the item. How do I update the item on the client? I looked around about TickHandlers, but I doubt that's what I'm looking for. Can anyone help me with this? The block in question: package com.richarda.minesouls.block; import com.richarda.minesouls.common.BonfireContainer; import com.richarda.minesouls.item.ItemEstusFlask; import com.richarda.minesouls.reference.Names; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.ItemStack; import net.minecraft.util.ChatComponentText; import net.minecraft.world.World; public class BlockBonfire extends BlockMineSouls { public BlockBonfire() { super(); this.setBlockName(Names.Blocks.BONFIRE); this.setHarvestLevel("pickaxe", 3); this.blockHardness = 50; } @Override public boolean onBlockActivated(World world, int x, int y, int z, EntityPlayer player, int face, float par7, float par8, float par9) { if(!world.isRemote) { player.addChatMessage(new ChatComponentText("You have rested at this bonfire.")); // Fully heal the player and reset hunger player.heal(player.getMaxHealth()); player.getFoodStats().setFoodLevel(20); BonfireContainer props = BonfireContainer.get(player); props.setBonfireLocation(x, y, z); // Refill estus flask(s) ItemStack[] playerInventory = player.inventory.mainInventory; for(ItemStack itemStack : playerInventory) { if(itemStack != null && itemStack.getItem() instanceof ItemEstusFlask) { ((ItemEstusFlask)itemStack.getItem()).refillEstusFlask(itemStack); } } return true; } return false; } } The item class has this method to refill it: public void refillEstusFlask(ItemStack itemStack) { itemStack.setItemDamage(0); }
-
[1.7.10] LivingDeathEvent is fired either twice or not at all
DaBananaboat replied to DaBananaboat's topic in Modder Support
I feel like an idiot. The EventHander was indeed registered twice. Once in preinit, once in init... -
[1.7.10] LivingDeathEvent is fired either twice or not at all
DaBananaboat replied to DaBananaboat's topic in Modder Support
I assume I don't need it on the clientside. Is it clientside? I'm not very familiar with events like that. This is my first attempt to make an actual mod. Edit: Looking at the debugger, it seems my event listener is registered twice -
I have an event handler which handles the LivingDeathEvent, but it either fires twice or not at all. Why is that? I'm using Forge 1230 for Minecraft 1.7.10 The event handler: public class MobKillHandler { @SubscribeEvent public void onMobDeath(LivingDeathEvent event) { if(!event.entityLiving.worldObj.isRemote) // Removing this line will cause the event to be fired twice, not removing it causes it to not be fired at all { return; } try { Entity killer = event.source.getSourceOfDamage(); Entity killedMob = event.entityLiving; if(killer instanceof EntityPlayer) { int soulsAward = getSoulAward(killedMob); SoulMemoryHandler.addSoulAmount(((EntityPlayer) killer).getDisplayName(), soulsAward); } } // Probably died of fall damage, or a cactus catch(NullPointerException ex) { // NOOP } } private int getSoulAward(Entity mob) { if(mob instanceof EntityZombie) { return Config.soulsPerZombie; } if(mob instanceof EntitySkeleton) { return Config.soulsPerSkeleton; } if(mob instanceof EntityCreeper) { return Config.soulsPerCreeper; } if(mob instanceof EntitySpider) { return Config.soulsPerSpider; } return 0; } } The registry: public class EventHandlers { public static void register() { // Entity death event MinecraftForge.EVENT_BUS.register(new MobKillHandler()); } } The registry is called here: @Mod.EventHandler public void init(FMLInitializationEvent evt) { // Initialize recipes Recipes.init(); // Register event handlers EventHandlers.register(); Logger.info("Init completed!"); } I tried using event.entity.worldObj.isRemote in the same sense, but that gave the same result. EDIT: I tried making the event sided using @SideOnly(Side.CLIENT), same result. Pretty sure that would be a wrong approach anyway.
-
Following tutorials it seemed to work for everyone else. But changing getBlockTextureFromSideAndMetadata() to getIcon() did it for me. Thank you very much and sorry for the bother.
-
That's exactly what I did... Still, I get the block in a single texture and not as the log as I want it to be. Sorry that I just don't understand, sometimes autism and programming don't match.
-
I got it to work-ish. The block is now in the game, but all sides are rendered by the blockIcon After following a few links from that search (thanks for the sarcasm btw, I look all over fyi), I got to this: public class BlockBananaLog extends Block { private Icon[] textures = new Icon[2]; public BlockBananaLog(int id, Material mat) { super(id, mat); this.setCreativeTab(CreativeTabs.tabBlock); this.setStepSound(Block.soundWoodFootstep); this.setUnlocalizedName("BananaLog"); } @SideOnly(Side.CLIENT) @Override public void registerIcons(IconRegister reg) { textures[0] = reg.registerIcon("nl/dabananaboat/projectbanana:" + this.getUnlocalizedName2() + "Bottom"); textures[1] = reg.registerIcon("nl/dabananaboat/projectbanana:" + this.getUnlocalizedName2() + "Top"); this.blockIcon = reg.registerIcon("nl/dabananaboat/projectbanana:" + this.getUnlocalizedName2() + "Sides"); } public Icon getBlockTextureFromSideAndMetadata(int side, int meta) { if(side == 0) return textures[0]; else if(side == 1) return textures[1]; else return this.blockIcon; } } I still don't get what I do wrong.
-
How do I register them then? I don't understand how to.
-
Okay, so I made the textures and after looking around a bit I found that I needed to use public Icon getBlockTextureFromSideAndMetadata(int side, int meta) It now runs perfectly until I want to move to the block itself, at which point it'll crash. Looking at the console, it said it got a NullPointerException. The error is: java.lang.NullPointerException at net.minecraft.block.BlockLog.getIcon(BlockLog.java:117) at net.minecraft.client.renderer.RenderBlocks.getBlockIconFromSideAndMetadata(RenderBlocks.java:8146) at net.minecraft.client.renderer.RenderBlocks.renderBlockAsItem(RenderBlocks.java:8095) at net.minecraft.client.renderer.entity.RenderItem.renderItemIntoGUI(RenderItem.java:367) at net.minecraft.client.renderer.entity.RenderItem.renderItemAndEffectIntoGUI(RenderItem.java:443) at net.minecraft.client.gui.inventory.GuiContainer.drawSlotInventory(GuiContainer.java:412) at net.minecraft.client.gui.inventory.GuiContainer.drawScreen(GuiContainer.java:128) at net.minecraft.client.renderer.InventoryEffectRenderer.drawScreen(InventoryEffectRenderer.java:43) at net.minecraft.client.gui.inventory.GuiContainerCreative.drawScreen(GuiContainerCreative.java:664) at net.minecraft.client.renderer.EntityRenderer.updateCameraAndRender(EntityRenderer.java:1021) at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:870) at net.minecraft.client.Minecraft.run(Minecraft.java:759) at java.lang.Thread.run(Unknown Source) Here is the full log: https://www.dropbox.com/s/ol9jcqbq8xs0kou/crash-2013-05-17_22.25.46-client.txt My log class is: package mods.nl.dabananaboat.projectbanana.common; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; import net.minecraft.block.BlockLog; import net.minecraft.block.material.Material; import net.minecraft.client.renderer.texture.IconRegister; import net.minecraft.util.Icon; public class BlockBananaLog extends BlockLog { private Icon sides, top, bottom; public BlockBananaLog(int id) { super(id); } @SideOnly(Side.CLIENT) public void registerIcons(IconRegister reg) { this.top = reg.registerIcon("nl/dabananaboat/projectbanana:BananaTreeTop"); this.sides = reg.registerIcon("nl/dabananaboat/projectbanana:BananaTreeSides"); this.bottom = reg.registerIcon("nl/dabananaboat/projectbanana:BananaTreeBottom"); } public Icon getBlockTextureFromSideAndMetadata(int side, int meta) { if(side == 0) return this.bottom; else if(side == 1) return this.top; else return this.sides; } } And to load everything in the main class: // LOAD ITEMS private void loadItems() { banana = new ItemBanana(bananaID, 3, 0.3F, false).setUnlocalizedName("Banana"); bananaPeel = new ItemBananaPeel(bananaPeelID).setUnlocalizedName("BananaPeel"); } // LOAD BLOCKS private void loadBlocks() { bananaLog = new BlockBananaLog(bananaLogID).setUnlocalizedName("BananaLog"); } // Register languages private void registerLanguages() { // Items LanguageRegistry.addName(banana, "Banana"); LanguageRegistry.addName(bananaPeel, "Banana Peel"); // Blocks LanguageRegistry.addName(bananaLog, "Banana Log"); } // Register items for game private void registerGame() { // Items GameRegistry.registerItem(banana, "Banana"); GameRegistry.registerItem(bananaPeel, "BananaPeel"); // Blocks GameRegistry.registerBlock(bananaLog, "BananaLog"); } I truely have no idea what I did wrong.
-
I'm looking at it, assuming I also need to extend my block from it. package mods.nl.dabananaboat.projectbanana.common; import net.minecraft.block.BlockLog; import net.minecraft.block.material.Material; import net.minecraft.client.renderer.texture.IconRegister; public class BlockBananaLog extends BlockLog { public BlockBananaLog(int id) { super(id); } } Do I then use this.getBlockTextureFromSide(par1) to set the textures, seeing there is no setter version of it?
-
Well, as far as making blocks go I'm good. But I don't understand how to add the textures for a log since the top and bottom are different to the sides. I'll look into that class, thank you.
-
Anything really, I couldn't find any recent tutorials on making trees since most of them are made with 1.4.7. Right now I'm trying to make the actual tree items and then I'll read on how to add them into the world gen.
-
I've recently started to mod with Forge and I want to create my own tree. After trying to look up some tutorials on youtube, I found that these are outdated due to the way textures are now stored. I also tried the javadocs, but I can't really make sense of it.. Could anyone here link me an up to date tutorial on making trees or tell me how I could make one? Much appreciated.