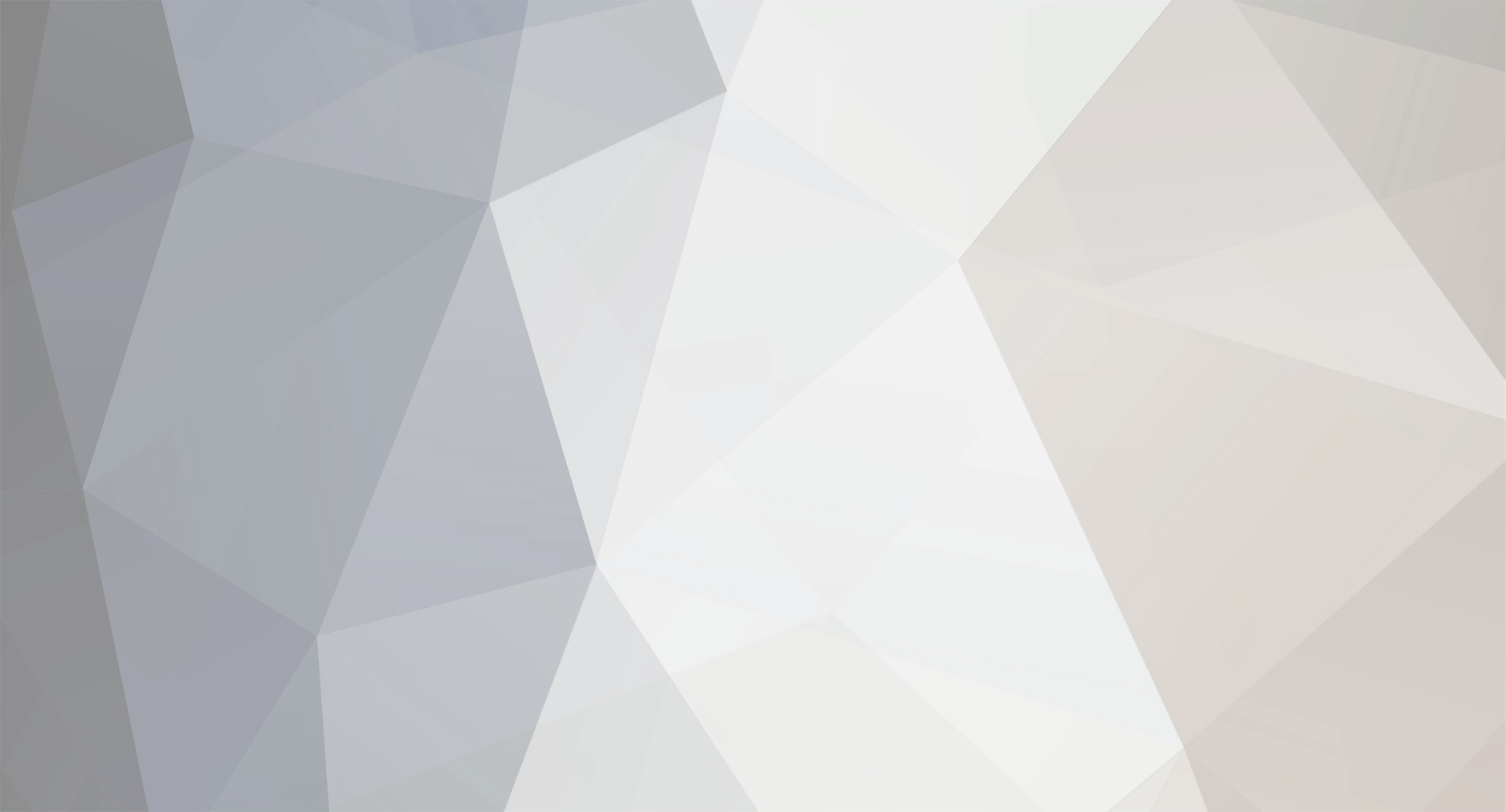
Chydog376
Members-
Posts
5 -
Joined
-
Last visited
Everything posted by Chydog376
-
I figured, I am probably in quite over my head, what I don't get is why I can't even get the java library to load into forge. I can figure out how to code it, but I can't even use Java FX...
-
Hello, I am currently trying to implement Javafx into a minecraft mod. For testing purposes all I am trying to do is make the javafx web browser system open on top of minecraft in the window. I have the code correct as it will work on its own, but as soon as I add it to Minecraft the client loads but then greets me with Caused by: java.lang.ClassNotFoundException: javafx.application.Application. I attached my Build.Gradle, main mod class, and the web browser (main app) class. //Build.Gradle plugins { id 'eclipse' id 'idea' id 'maven-publish' id 'net.minecraftforge.gradle' version '[6.0,6.2)' id 'org.parchmentmc.librarian.forgegradle' version '1.+' id 'application' id 'org.openjfx.javafxplugin' version '0.0.9' } javafx { version = "16" modules = [ 'javafx.controls', 'javafx.fxml', 'javafx.web' ] } version = mod_version group = mod_group_id base { archivesName = mod_id } java.toolchain.languageVersion = JavaLanguageVersion.of(17) println "Java: ${System.getProperty 'java.version'}, JVM: ${System.getProperty 'java.vm.version'} (${System.getProperty 'java.vendor'}), Arch: ${System.getProperty 'os.arch'}" minecraft { mappings channel: mapping_channel, version: mapping_version copyIdeResources = true runs { configureEach { workingDirectory project.file('run') property 'forge.logging.markers', 'REGISTRIES' property 'forge.logging.console.level', 'debug' mods { "${mod_id}" { source sourceSets.main } } } client { property 'forge.enabledGameTestNamespaces', mod_id } server { property 'forge.enabledGameTestNamespaces', mod_id args '--nogui' } gameTestServer { property 'forge.enabledGameTestNamespaces', mod_id } data { workingDirectory project.file('run-data') args '--mod', mod_id, '--all', '--output', file('src/generated/resources/'), '--existing', file('src/main/resources/') } } } sourceSets.main.resources { srcDir 'src/generated/resources' } repositories { mavenCentral() } dependencies { minecraft "net.minecraftforge:forge:${minecraft_version}-${forge_version}" implementation 'org.openjfx:javafx-controls:16' implementation 'org.openjfx:javafx-fxml:16' implementation 'org.openjfx:javafx-web:16' } jar { from { configurations.implementation.collect { it.isDirectory() ? it : zipTree(it) } } } mainClassName = 'net.chydog.webuimod.MainApp' tasks.named('processResources', ProcessResources).configure { var replaceProperties = [ minecraft_version: minecraft_version, minecraft_version_range: minecraft_version_range, forge_version: forge_version, forge_version_range: forge_version_range, loader_version_range: loader_version_range, mod_id: mod_id, mod_name: mod_name, mod_license: mod_license, mod_version: mod_version, mod_authors: mod_authors, mod_description: mod_description, ] inputs.properties replaceProperties filesMatching(['META-INF/mods.toml', 'pack.mcmeta']) { expand replaceProperties + [project: project] } } tasks.named('jar', Jar).configure { manifest { attributes([ 'Specification-Title' : mod_id, 'Specification-Vendor' : mod_authors, 'Specification-Version' : '1', // We are version 1 of ourselves 'Implementation-Title' : project.name, 'Implementation-Version' : project.jar.archiveVersion, 'Implementation-Vendor' : mod_authors, 'Implementation-Timestamp': new Date().format("yyyy-MM-dd'T'HH:mm:ssZ") ]) } } //WebUiMod.java package net.chydog.webuimod; import com.mojang.logging.LogUtils; import net.minecraftforge.api.distmarker.Dist; import net.minecraftforge.common.MinecraftForge; import net.minecraftforge.event.BuildCreativeModeTabContentsEvent; import net.minecraftforge.event.server.ServerStartingEvent; import net.minecraftforge.eventbus.api.IEventBus; import net.minecraftforge.eventbus.api.SubscribeEvent; import net.minecraftforge.fml.ModLoadingContext; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.config.ModConfig; import net.minecraftforge.fml.event.lifecycle.FMLClientSetupEvent; import net.minecraftforge.fml.event.lifecycle.FMLCommonSetupEvent; import net.minecraftforge.fml.javafmlmod.FMLJavaModLoadingContext; import org.slf4j.Logger; import javafx.application.Application; import static net.chydog.webuimod.WebUiMod.ClientModEvents.launchJavaFXApplication; // The value here should match an entry in the META-INF/mods.toml file @Mod(WebUiMod.MODID) public class WebUiMod { // Define mod id in a common place for everything to reference public static final String MODID = "webuimod"; // Directly reference a slf4j logger private static final Logger LOGGER = LogUtils.getLogger(); public WebUiMod() { IEventBus modEventBus = FMLJavaModLoadingContext.get().getModEventBus(); modEventBus.addListener(this::commonSetup); MinecraftForge.EVENT_BUS.register(this); // Register the item to a creative tab modEventBus.addListener(this::addCreative); ModLoadingContext.get().registerConfig(ModConfig.Type.COMMON, Config.SPEC); } private void commonSetup(final FMLCommonSetupEvent event) { // Launch your JavaFX application when Minecraft initializes launchJavaFXApplication(); } // Add the example block item to the building blocks tab private void addCreative(BuildCreativeModeTabContentsEvent event) { } // You can use SubscribeEvent and let the Event Bus discover methods to call @SubscribeEvent public void onServerStarting(ServerStartingEvent event) { } // You can use EventBusSubscriber to automatically register all static methods in the class annotated with @SubscribeEvent @Mod.EventBusSubscriber(modid = MODID, bus = Mod.EventBusSubscriber.Bus.MOD, value = Dist.CLIENT) public static class ClientModEvents { @SubscribeEvent public static void onClientSetup(FMLClientSetupEvent event) { } static void launchJavaFXApplication() { // Launch your JavaFX application here Application.launch(MainApp.class); } } } //MainApp.java package net.chydog.webuimod; import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.web.WebView; import javafx.stage.Stage; public class MainApp extends Application { @Override public void start(Stage stage) throws Exception { WebView webView = new WebView(); webView.getEngine().load("https://www.abcya.com"); // Set your desired URL here Scene scene = new Scene(webView, 800, 600); stage.setTitle("Web Browser"); stage.setScene(scene); stage.show(); } public static void main(String[] args) { launch(args); } }
-
Hello, I am currently trying to implement Javafx into a minecraft mod. For testing purposes all I am trying to do is make the javafx web browser system open on top of minecraft in the window. I have the code correct as it will work on its own, but as soon as I add it to Minecraft the client loads but then greets me with Caused by: java.lang.ClassNotFoundException: javafx.application.Application. I attached my Build.Gradle, main mod class, and the web browser (main app) class. //Build.Gradle plugins { id 'eclipse' id 'idea' id 'maven-publish' id 'net.minecraftforge.gradle' version '[6.0,6.2)' id 'org.parchmentmc.librarian.forgegradle' version '1.+' id 'application' id 'org.openjfx.javafxplugin' version '0.0.9' } javafx { version = "16" modules = [ 'javafx.controls', 'javafx.fxml', 'javafx.web' ] } version = mod_version group = mod_group_id base { archivesName = mod_id } java.toolchain.languageVersion = JavaLanguageVersion.of(17) println "Java: ${System.getProperty 'java.version'}, JVM: ${System.getProperty 'java.vm.version'} (${System.getProperty 'java.vendor'}), Arch: ${System.getProperty 'os.arch'}" minecraft { mappings channel: mapping_channel, version: mapping_version copyIdeResources = true runs { configureEach { workingDirectory project.file('run') property 'forge.logging.markers', 'REGISTRIES' property 'forge.logging.console.level', 'debug' mods { "${mod_id}" { source sourceSets.main } } } client { property 'forge.enabledGameTestNamespaces', mod_id } server { property 'forge.enabledGameTestNamespaces', mod_id args '--nogui' } gameTestServer { property 'forge.enabledGameTestNamespaces', mod_id } data { workingDirectory project.file('run-data') args '--mod', mod_id, '--all', '--output', file('src/generated/resources/'), '--existing', file('src/main/resources/') } } } sourceSets.main.resources { srcDir 'src/generated/resources' } repositories { mavenCentral() } dependencies { minecraft "net.minecraftforge:forge:${minecraft_version}-${forge_version}" implementation 'org.openjfx:javafx-controls:16' implementation 'org.openjfx:javafx-fxml:16' implementation 'org.openjfx:javafx-web:16' } jar { from { configurations.implementation.collect { it.isDirectory() ? it : zipTree(it) } } } mainClassName = 'net.chydog.webuimod.MainApp' tasks.named('processResources', ProcessResources).configure { var replaceProperties = [ minecraft_version: minecraft_version, minecraft_version_range: minecraft_version_range, forge_version: forge_version, forge_version_range: forge_version_range, loader_version_range: loader_version_range, mod_id: mod_id, mod_name: mod_name, mod_license: mod_license, mod_version: mod_version, mod_authors: mod_authors, mod_description: mod_description, ] inputs.properties replaceProperties filesMatching(['META-INF/mods.toml', 'pack.mcmeta']) { expand replaceProperties + [project: project] } } tasks.named('jar', Jar).configure { manifest { attributes([ 'Specification-Title' : mod_id, 'Specification-Vendor' : mod_authors, 'Specification-Version' : '1', // We are version 1 of ourselves 'Implementation-Title' : project.name, 'Implementation-Version' : project.jar.archiveVersion, 'Implementation-Vendor' : mod_authors, 'Implementation-Timestamp': new Date().format("yyyy-MM-dd'T'HH:mm:ssZ") ]) } } //WebUiMod.java package net.chydog.webuimod; import com.mojang.logging.LogUtils; import net.minecraftforge.api.distmarker.Dist; import net.minecraftforge.common.MinecraftForge; import net.minecraftforge.event.BuildCreativeModeTabContentsEvent; import net.minecraftforge.event.server.ServerStartingEvent; import net.minecraftforge.eventbus.api.IEventBus; import net.minecraftforge.eventbus.api.SubscribeEvent; import net.minecraftforge.fml.ModLoadingContext; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.config.ModConfig; import net.minecraftforge.fml.event.lifecycle.FMLClientSetupEvent; import net.minecraftforge.fml.event.lifecycle.FMLCommonSetupEvent; import net.minecraftforge.fml.javafmlmod.FMLJavaModLoadingContext; import org.slf4j.Logger; import javafx.application.Application; import static net.chydog.webuimod.WebUiMod.ClientModEvents.launchJavaFXApplication; // The value here should match an entry in the META-INF/mods.toml file @Mod(WebUiMod.MODID) public class WebUiMod { // Define mod id in a common place for everything to reference public static final String MODID = "webuimod"; // Directly reference a slf4j logger private static final Logger LOGGER = LogUtils.getLogger(); public WebUiMod() { IEventBus modEventBus = FMLJavaModLoadingContext.get().getModEventBus(); modEventBus.addListener(this::commonSetup); MinecraftForge.EVENT_BUS.register(this); // Register the item to a creative tab modEventBus.addListener(this::addCreative); ModLoadingContext.get().registerConfig(ModConfig.Type.COMMON, Config.SPEC); } private void commonSetup(final FMLCommonSetupEvent event) { // Launch your JavaFX application when Minecraft initializes launchJavaFXApplication(); } // Add the example block item to the building blocks tab private void addCreative(BuildCreativeModeTabContentsEvent event) { } // You can use SubscribeEvent and let the Event Bus discover methods to call @SubscribeEvent public void onServerStarting(ServerStartingEvent event) { } // You can use EventBusSubscriber to automatically register all static methods in the class annotated with @SubscribeEvent @Mod.EventBusSubscriber(modid = MODID, bus = Mod.EventBusSubscriber.Bus.MOD, value = Dist.CLIENT) public static class ClientModEvents { @SubscribeEvent public static void onClientSetup(FMLClientSetupEvent event) { } static void launchJavaFXApplication() { // Launch your JavaFX application here Application.launch(MainApp.class); } } } //MainApp.java package net.chydog.webuimod; import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.web.WebView; import javafx.stage.Stage; public class MainApp extends Application { @Override public void start(Stage stage) throws Exception { WebView webView = new WebView(); webView.getEngine().load("https://www.abcya.com"); // Set your desired URL here Scene scene = new Scene(webView, 800, 600); stage.setTitle("Web Browser"); stage.setScene(scene); stage.show(); } public static void main(String[] args) { launch(args); } }
-
I changed it to public static void but I can't find anyway to get level from the event even if it isn't a listener parameter. @JimiIT92
-
Hello, I am trying to make a drowned transform into a custom mod I made (called Hot Drowned) when it goes through a magma bubble column. I already have the mob fully working in game, but the code for the transformation is giving me trouble. Since I can't edit the drowned code, I tried to make it check on Living Tick Event for a drowned that is doing the ticking and check its position from there in relation to a magma block but its not working. My code is below, thank you in advance. @SubscribeEvent(priority = EventPriority.HIGHEST) public void entityJoinWorld(LivingEvent.LivingTickEvent event, ServerLevel pServerLevel) { System.out.println("Entity Ticked"); Entity entity = event.getEntity(); if (entity.isAlive() && entity instanceof Drowned) { Drowned drowned = (Drowned) entity; BlockPos bPos = BlockPos.of(drowned.blockPosition().get(Direction.Axis.Y)); BlockState bPosBlock = pServerLevel.getBlockState(bPos); int dHieght = bPos.getY(); if (bPosBlock == Blocks.WATER.defaultBlockState() || bPosBlock == Blocks.MAGMA_BLOCK.defaultBlockState()){ if (bPosBlock == Blocks.MAGMA_BLOCK.defaultBlockState()){ ModEntities.HOT_DROWN.get().spawn(pServerLevel, BlockPos.of(drowned.blockPosition().get(Direction.Axis.Y)), NATURAL); }else { for (int x = dHieght + 64; x > 0; x--) { bPos = BlockPos.of(bPos.below().get(Direction.Axis.Y)); bPosBlock = pServerLevel.getBlockState(bPos); System.out.println(bPosBlock); if (bPosBlock == Blocks.MAGMA_BLOCK.defaultBlockState()) { ModEntities.HOT_DROWN.get().spawn(pServerLevel, BlockPos.of(drowned.blockPosition().get(Direction.Axis.Y)), NATURAL); } else { return; } } } } } } }