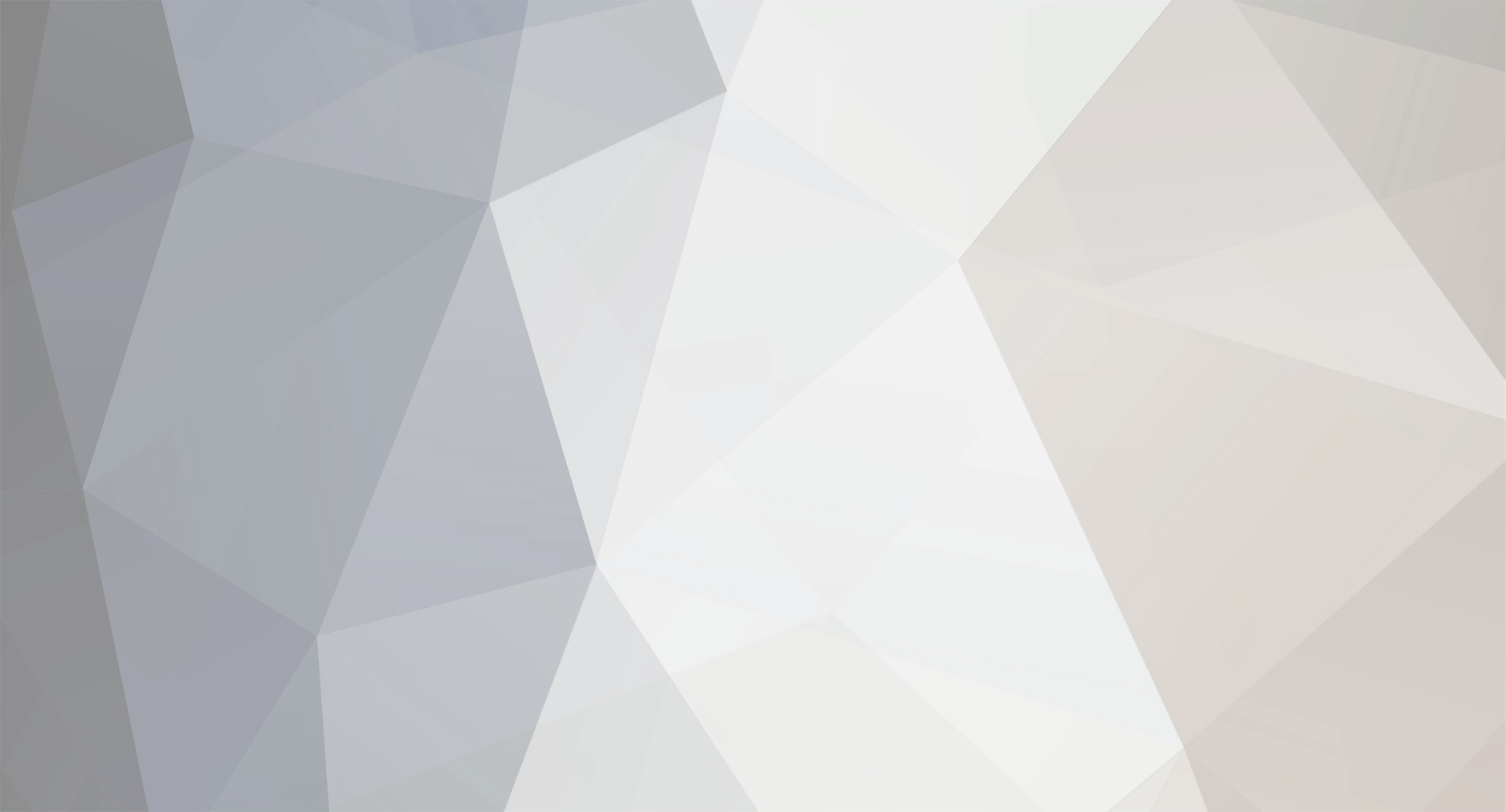
Nethseaar
Members-
Posts
13 -
Joined
-
Last visited
Everything posted by Nethseaar
-
Might you point me toward them? I've searched, to no avail. I've registered my renderer thusly, in Common Proxy: public void registerRenderers() { RenderingRegistry.registerEntityRenderingHandler(Entity.class, new RenderFallingAscender()); } But I haven't registered my entity! Where/how does one do that?
-
Are there any tutorials for creating and rendering entities like FallingSand? I've copied (and made appropriate changes to) the FallingSand code, and its renderer, but either my entity isn't spawning or it isn't rendering; the sand just disappears when it can fall, and then breaks (drops itself) at some point as it rises. I don't understand how renderers work at all, so I'm blaming the problem on my renderer.
-
Of course! Thanks much! I've got a long way to go before all this is natural to me.
-
I'm trying to get my custom sand block to fall upwards, but changing things has zero effect on anything. I must be missing something obvious, but search as I might, I am not finding it. I've flipped what I thought were the relevant values from negative to positive, and even if that doesn't do what I want, I figure it ought to do something, but the block still acts just like normal sand. Is the normal sand entity being created, instead of mine? Here's my code (red text is altered from sand's code EDIT: Or, ah, I suppose you can't change color in code tags): Sand Block: package nethseaar.bacon.blocks; import java.util.Random; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; import net.minecraft.block.Block; import net.minecraft.block.BlockSand; import net.minecraft.block.material.Material; import net.minecraft.client.renderer.texture.IconRegister; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.entity.item.EntityFallingSand; import net.minecraft.util.Icon; import net.minecraft.world.World; import nethseaar.bacon.entities.EntityFallingAscender; public class BlockUp extends BlockSand { @SideOnly(Side.CLIENT) private Icon upTop; @SideOnly(Side.CLIENT) private Icon upBottom; public BlockUp(int id, Material material) { super(id, material); } /** * If there is space to fall below will start this block falling */ private void tryToFall(World par1World, int par2, int par3, int par4) { [color=red]if (canFallAbove(par1World, par2, par3 + 1, par4) && par3 >= 0)[/color] { byte b0 = 32; if (!fallInstantly && par1World.checkChunksExist(par2 - b0, par3 - b0, par4 - b0, par2 + b0, par3 + b0, par4 + b0)) { if (!par1World.isRemote) { [color=red]EntityFallingAscender entityfallingascender = new EntityFallingAscender(par1World, (double)((float)par2 + 0.5F), (double)((float)par3 + 0.5F), (double)((float)par4 + 0.5F), this.blockID, par1World.getBlockMetadata(par2, par3, par4)); this.onStartFalling(entityfallingascender); par1World.spawnEntityInWorld(entityfallingascender);[/color] } } else { par1World.setBlockToAir(par2, par3, par4); [color=red] while (canFallBelow(par1World, par2, par3 + 1, par4) && par3 > 0) { ++par3; } [/color] if (par3 > 0) { par1World.setBlock(par2, par3, par4, this.blockID); } } } } public static boolean canFallAbove(World par0World, int par1, int par2, int par3) { int l = par0World.getBlockId(par1, par2, par3); if (par0World.isAirBlock(par1, par2, par3)) { return true; } else if (l == Block.fire.blockID) { return true; } else { Material material = Block.blocksList[l].blockMaterial; return material == Material.water ? true : material == Material.lava; } } @SideOnly(Side.CLIENT) public Icon getIcon(int par1, int par2) { return par1 == 0 ? this.upBottom : (par1 == 1 ? this.upTop: this.blockIcon); } @SideOnly(Side.CLIENT) public void registerIcons(IconRegister par1IconRegister) { this.blockIcon = par1IconRegister.registerIcon("upDownSides"); this.upTop = par1IconRegister.registerIcon("upTop"); this.upBottom = par1IconRegister.registerIcon("upBottom"); } } FallingSand Entity: package nethseaar.bacon.entities; import java.util.Iterator; import net.minecraft.block.Block; import net.minecraft.block.BlockSand; import net.minecraft.block.ITileEntityProvider; import net.minecraft.entity.Entity; import net.minecraft.entity.item.EntityFallingSand; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTBase; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.MathHelper; import net.minecraft.world.World; public class EntityFallingAscender extends EntityFallingSand { public int blockID; public int metadata; /** How long the block has been falling for. */ public int fallTime; public boolean shouldDropItem; private boolean isBreakingAnvil; private boolean isAnvil; /** Maximum amount of damage dealt to entities hit by falling block */ private int fallHurtMax; /** Actual damage dealt to entities hit by falling block */ private float fallHurtAmount; public NBTTagCompound fallingBlockTileEntityData; public EntityFallingAscender(World par1World) { super(par1World); this.fallTime = 0; this.shouldDropItem = true; this.isBreakingAnvil = false; this.isAnvil = false; this.fallHurtMax = 40; this.fallHurtAmount = 2.0F; this.fallingBlockTileEntityData = null; } public EntityFallingAscender(World par1World, double par2, double par4, double par6, int par8) { this(par1World, par2, par4, par6, par8, 0); } public EntityFallingAscender(World par1World, double par2, double par4, double par6, int par8, int par9) { super(par1World); this.fallTime = 0; this.shouldDropItem = true; this.isBreakingAnvil = false; this.isAnvil = false; this.fallHurtMax = 40; this.fallHurtAmount = 2.0F; this.fallingBlockTileEntityData = null; this.blockID = par8; this.metadata = par9; this.preventEntitySpawning = true; this.setSize(0.98F, 0.98F); this.yOffset = this.height / 2.0F; this.setPosition(par2, par4, par6); this.motionX = 0.0D; this.motionY = 0.0D; this.motionZ = 0.0D; this.prevPosX = par2; this.prevPosY = par4; this.prevPosZ = par6; } public void onUpdate() { if (this.blockID == 0) { this.setDead(); } else { this.prevPosX = this.posX; this.prevPosY = this.posY; this.prevPosZ = this.posZ; ++this.fallTime; [color=red] this.motionY += 0.03999999910593033D;[/color] this.moveEntity(this.motionX, this.motionY, this.motionZ); this.motionX *= 0.9800000190734863D; this.motionY *= 0.9800000190734863D; this.motionZ *= 0.9800000190734863D; if (!this.worldObj.isRemote) { int i = MathHelper.floor_double(this.posX); int j = MathHelper.floor_double(this.posY); int k = MathHelper.floor_double(this.posZ); if (this.fallTime == 1) { if (this.worldObj.getBlockId(i, j, k) != this.blockID) { this.setDead(); return; } this.worldObj.setBlockToAir(i, j, k); } if (this.onGround) { this.motionX *= 0.699999988079071D; this.motionZ *= 0.699999988079071D; this.motionY *= 0.5D; if (this.worldObj.getBlockId(i, j, k) != Block.pistonMoving.blockID) { this.setDead(); if (!this.isBreakingAnvil && this.worldObj.canPlaceEntityOnSide(this.blockID, i, j, k, true, 1, (Entity)null, (ItemStack)null) && !BlockSand.canFallBelow(this.worldObj, i, j - 1, k) && this.worldObj.setBlock(i, j, k, this.blockID, this.metadata, 3)) { if (Block.blocksList[this.blockID] instanceof BlockSand) { ((BlockSand)Block.blocksList[this.blockID]).onFinishFalling(this.worldObj, i, j, k, this.metadata); } if (this.fallingBlockTileEntityData != null && Block.blocksList[this.blockID] instanceof ITileEntityProvider) { TileEntity tileentity = this.worldObj.getBlockTileEntity(i, j, k); if (tileentity != null) { NBTTagCompound nbttagcompound = new NBTTagCompound(); tileentity.writeToNBT(nbttagcompound); Iterator iterator = this.fallingBlockTileEntityData.getTags().iterator(); while (iterator.hasNext()) { NBTBase nbtbase = (NBTBase)iterator.next(); if (!nbtbase.getName().equals("x") && !nbtbase.getName().equals("y") && !nbtbase.getName().equals("z")) { nbttagcompound.setTag(nbtbase.getName(), nbtbase.copy()); } } tileentity.readFromNBT(nbttagcompound); tileentity.onInventoryChanged(); } } } else if (this.shouldDropItem && !this.isBreakingAnvil) { this.entityDropItem(new ItemStack(this.blockID, 1, Block.blocksList[this.blockID].damageDropped(this.metadata)), 0.0F); } } } else if (this.fallTime > 100 && !this.worldObj.isRemote && (j < 1 || j > 256) || this.fallTime > 600) { if (this.shouldDropItem) { this.entityDropItem(new ItemStack(this.blockID, 1, Block.blocksList[this.blockID].damageDropped(this.metadata)), 0.0F); } this.setDead(); } } } } }
-
[1.5.2] Connected Texture Orientation Without Metadata
Nethseaar replied to Nethseaar's topic in Modder Support
Could anyone point me to an example of connected textures code? Or, alternately, help me to understand this code: package f1rSt1k.blocks; import java.util.List; import java.util.Random; import f1rSt1k.Main; import net.minecraft.block.Block; import net.minecraft.block.BlockGlass; import net.minecraft.block.material.Material; import net.minecraft.client.renderer.texture.IconRegister; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.util.Icon; import net.minecraft.world.IBlockAccess; public class BlockDecorativeGlass extends BlockGlass { public static boolean connectedTexture = true; public static Icon[] textures = new Icon[47]; public static int[] textureRefByID = { 0, 0, 6, 6, 0, 0, 6, 6, 3, 3, 19, 15, 3, 3, 19, 15, 1, 1, 18, 18, 1, 1, 13, 13, 2, 2, 23, 31, 2, 2, 27, 14, 0, 0, 6, 6, 0, 0, 6, 6, 3, 3, 19, 15, 3, 3, 19, 15, 1, 1, 18, 18, 1, 1, 13, 13, 2, 2, 23, 31, 2, 2, 27, 14, 4, 4, 5, 5, 4, 4, 5, 5, 17, 17, 22, 26, 17, 17, 22, 26, 16, 16, 20, 20, 16, 16, 28, 28, 21, 21, 46, 42, 21, 21, 43, 38, 4, 4, 5, 5, 4, 4, 5, 5, 9, 9, 30, 12, 9, 9, 30, 12, 16, 16, 20, 20, 16, 16, 28, 28, 25, 25, 45, 37, 25, 25, 40, 32, 0, 0, 6, 6, 0, 0, 6, 6, 3, 3, 19, 15, 3, 3, 19, 15, 1, 1, 18, 18, 1, 1, 13, 13, 2, 2, 23, 31, 2, 2, 27, 14, 0, 0, 6, 6, 0, 0, 6, 6, 3, 3, 19, 15, 3, 3, 19, 15, 1, 1, 18, 18, 1, 1, 13, 13, 2, 2, 23, 31, 2, 2, 27, 14, 4, 4, 5, 5, 4, 4, 5, 5, 17, 17, 22, 26, 17, 17, 22, 26, 7, 7, 24, 24, 7, 7, 10, 10, 29, 29, 44, 41, 29, 29, 39, 33, 4, 4, 5, 5, 4, 4, 5, 5, 9, 9, 30, 12, 9, 9, 30, 12, 7, 7, 24, 24, 7, 7, 10, 10, 8, 8, 36, 35, 8, 8, 34, 11 }; public BlockDecorativeGlass() { super(502, Material.glass, false); setHardness(0.6F); setResistance(2.0F); setCreativeTab(Main.f1rSt1kCraftCreativeTab); setStepSound(soundGlassFootstep); } public int idDropped(int par1, Random par2Random, int par3) { return Item.stick.itemID; } public int quantityDropped(Random par1Random) { return 1 + par1Random.nextInt(4); } public void registerIcons(IconRegister iconRegistry) { for (int i = 0; i < 47; i++) textures[i] = iconRegistry.registerIcon("f1rst1kcraft:woodGlass_" + (i+1)); } public Icon getBlockTexture(IBlockAccess world, int x, int y, int z, int side) { if(connectedTexture){ boolean[] bitMatrix = new boolean[8]; if (side == 0 || side == 1) { bitMatrix[0] = world.getBlockId(x-1, y, z-1) == this.blockID; bitMatrix[1] = world.getBlockId(x, y, z-1) == this.blockID; bitMatrix[2] = world.getBlockId(x+1, y, z-1) == this.blockID; bitMatrix[3] = world.getBlockId(x-1, y, z) == this.blockID; bitMatrix[4] = world.getBlockId(x+1, y, z) == this.blockID; bitMatrix[5] = world.getBlockId(x-1, y, z+1) == this.blockID; bitMatrix[6] = world.getBlockId(x, y, z+1) == this.blockID; bitMatrix[7] = world.getBlockId(x+1, y, z+1) == this.blockID; } if (side == 2 || side == 3) { bitMatrix[0] = world.getBlockId(x+(side==2?1:-1), y+1, z) == this.blockID; bitMatrix[1] = world.getBlockId(x, y+1, z) == this.blockID; bitMatrix[2] = world.getBlockId(x+(side==3?1:-1), y+1, z) == this.blockID; bitMatrix[3] = world.getBlockId(x+(side==2?1:-1), y, z) == this.blockID; bitMatrix[4] = world.getBlockId(x+(side==3?1:-1), y, z) == this.blockID; bitMatrix[5] = world.getBlockId(x+(side==2?1:-1), y-1, z) == this.blockID; bitMatrix[6] = world.getBlockId(x, y-1, z) == this.blockID; bitMatrix[7] = world.getBlockId(x+(side==3?1:-1), y-1, z) == this.blockID; } if (side == 4 || side == 5) { bitMatrix[0] = world.getBlockId(x, y+1, z+(side==5?1:-1)) == this.blockID; bitMatrix[1] = world.getBlockId(x, y+1, z) == this.blockID; bitMatrix[2] = world.getBlockId(x, y+1, z+(side==4?1:-1)) == this.blockID; bitMatrix[3] = world.getBlockId(x, y, z+(side==5?1:-1)) == this.blockID; bitMatrix[4] = world.getBlockId(x, y, z+(side==4?1:-1)) == this.blockID; bitMatrix[5] = world.getBlockId(x, y-1, z+(side==5?1:-1)) == this.blockID; bitMatrix[6] = world.getBlockId(x, y-1, z) == this.blockID; bitMatrix[7] = world.getBlockId(x, y-1, z+(side==4?1:-1)) == this.blockID; } int idBuilder = 0; for (int i = 0; i <= 7; i++) idBuilder = idBuilder + (bitMatrix[i]?(i==0?1:(i==1?2:(i==2?4:(i==3?8:(i==4?16:(i==5?32:(i==6?64:128))))))):0); return idBuilder>255||idBuilder<0?textures[0]:textures[textureRefByID[idBuilder]]; } return textures[0]; } public Icon getIcon(int side, int meta) { return textures[0]; } } It just occurred to me: the GetBlockTexture lets you get the surrounding block IDs -- I'm going to see whether I can do it there, without metadata. -
I've done it by having different textures for each rotation. It might not be the best way to do it, but it works.
-
PASTEBIN OF CODE I am trying to make a block with connected textures for its top and bottom faces, and I've got it working except for the orientation of the textures. Using metadata, I can get the right type of texture, but not the right orientation of it. There are too many textures for metadata to handle (using separate textures for each orientation), and this cannot be a tile entity, as it is a decorative block. Can I set something other than metadata, without making the block a tile entity, to get the right orientation of the texture? I tried using an instance variable, but of course that doesn't set per instance; every instance of the block changes when one does. I am starting to think what I want is not possible, only I know that others have done connected textures for decorative blocks, like glass. Quite possibly, there's an obvious, better way to do this. I am still learning Java, so I apologize if I seem obtuse. Certainly this code is not elegant or pretty. Many thanks for any help or advice!
-
Thanks! Need to test this, but I found the line. Any way to flip the camera?
-
Indeed. That's what I have done with the Deathstone block -- it has five metadata sub blocks. What I'm trying to do now is make stair blocks out of only three of those sub blocks. Stair blocks with their own IDs. So, there's one ID for all the Deathstone blocks, then one ID each for Deathstone Stairs, Smooth Deathstone Stairs, and Deathstone Brick stairs. But I can't reference the individual sub blocks. So right now each instance of stairs that points toward Deathstone creates 5 stair blocks, all with the same texture.
-
Here's a tutorial you can read. Each block/item does have its own file, and those files must be saved in a Block or Item folder within a Textures folder within your mod folder. The method used to register icons is: public void updateIcons(IconRegister par1iconregister){ this.blockIcon = par1iconregister.registerIcon("[texture name, without .png]"); } An example of different textures on different sides: @SideOnly(Side.CLIENT) private Icon top; @SideOnly(Side.CLIENT) private Icon bottom; @SideOnly(Side.CLIENT) public Icon getIcon(int par1, int par2) { return par1 == 0 ? this.bottom: (par1 == 1 ? this.top: this.blockIcon); } @SideOnly(Side.CLIENT) public void registerIcons(IconRegister par1IconRegister) { this.blockIcon = par1IconRegister.registerIcon("[texture name]"); this.top = par1IconRegister.registerIcon("[texture name]"); this.bottom= par1IconRegister.registerIcon("[texture name]"); }
-
Off-topic question: How would one go about changing the 'gravity' of an entity? (i.e. changing the player so that they fall up, and so that the camera changed to match, or having sand fall a different direction?) Could you give me a place to look, if it's too complicated to be worth your time explaining?
-
Disclaimer: I am new to both Java and Forge. Probably I'm overlooking many things. I thank you for your patience! Is it possible to make stairs out of only specific metadata sub blocks? I have functioning stairs out of metadata sub blocks, but it makes stairs for each sub block, all of them with the first sub block's texture. Is there a way to specify which sub block to make stairs out of, in the same way you specify which sub block to use in crafting recipes? The stairs constructor doesn't call for, and doesn't accept, a metadata value. I tried changing the constructor in my own stair class (the class that extends BlockStairs), but that didn't go over so well. Maybe if I add a constructor that handles metadata? Here's example code with working stairs from metadata blocks, but too many of them, and mostly the wrong texture (Don't mind the bacon; it's a result of being able to put any string I wanted as the mod title, which people should probably not let me do): Block Class: public class BaconBlocks { public static String[] deathstoneNames = { "Deathstone", "Cracked Deathstone", "Condensed Deathstone", "Smooth Deathstone", "Deathstone Brick", "Carved Deathstone" }; //add blocks ----------------------------------------------------------------------------------------------------------------------------------------- public static Block deathstone; public static BlockStairs deathstoneStairs; public static BlockStairs smoothDeathstoneStairs; public static BlockStairs deathstoneBrickStairs; @Init public static void init() { //initialize blocks ------------------------------------------------------------------------------------------------------------------------------- deathstone = new BlockDeathstone(BlockIDs.deathstone, Material.cactus).setUnlocalizedName("deathstone"); deathstoneStairs = new StairBacon(BlockIDs.deathstoneStairs, BaconBlocks.deathstone, 0); smoothDeathstoneStairs = new StairBacon(BlockIDs.smoothDeathstoneStairs, BaconBlocks.deathstone, 0); deathstoneBrickStairs = new StairBacon(BlockIDs.deathstoneBrickStairs, BaconBlocks.deathstone, 0); //register blocks --------------------------------------------------------------------------------------------------------------------------------- GameRegistry.registerBlock(deathstone, ItemBlockBacon.class, "Bacon" + (deathstone.getUnlocalizedName().substring(5))); GameRegistry.registerBlock(deathstoneStairs, "deathstoneStairs"); GameRegistry.registerBlock(smoothDeathstoneStairs, "smoothDeathstoneStairs"); GameRegistry.registerBlock(deathstoneBrickStairs, "deathstoneBrickStairs"); //name blocks ------------------------------------------------------------------------------------------------------------------------------------- for (int i = 0; i< deathstoneNames.length; i++) {LanguageRegistry.addName(new ItemStack(deathstone, 1, i), deathstoneNames[i]); } LanguageRegistry.addName(deathstoneStairs, "Deathstone Stairs"); LanguageRegistry.addName(smoothDeathstoneStairs, "Smooth Deathstone Stairs"); LanguageRegistry.addName(deathstoneBrickStairs, "Deathstone Brick Stairs"); //set block harvest level ------------------------------------------------------------------------------------------------------------------------- MinecraftForge.setBlockHarvestLevel(deathstone, "pickaxe", 3); MinecraftForge.setBlockHarvestLevel(deathstoneStairs, "pickaxe", 3); MinecraftForge.setBlockHarvestLevel(smoothDeathstoneStairs, "pickaxe", 3); MinecraftForge.setBlockHarvestLevel(deathstoneBrickStairs, "pickaxe", 3); } } Deathstone Class: public class BlockDeathstone extends Block { public BlockDeathstone(int id, Material material) { super(id, material); setStepSound(Block.soundStoneFootstep); setHardness(4.0F); setResistance(5.0F); setCreativeTab(CreativeTabs.tabBlock); setLightValue(1.0F); } public AxisAlignedBB getCollisionBoundingBoxFromPool(World par1World, int par2, int par3, int par4) { float f = 0.0625F; return AxisAlignedBB.getAABBPool().getAABB((double)((float)par2 + f), (double)par3, (double)((float)par4 + f), (double)((float)(par2 + 1) - f), (double)((float)(par3 + 1) - f), (double)((float)(par4 + 1) - f)); } public void onEntityCollidedWithBlock(World par1World, int par2, int par3, int par4, Entity par5Entity) { par5Entity.attackEntityFrom(DamageSource.lava, 3); } @SideOnly(Side.CLIENT) private Icon[] icons; @SideOnly(Side.CLIENT) public void registerIcons(IconRegister par1IconRegister) { icons = new Icon[5]; icons[0] = par1IconRegister.registerIcon("deathstone"); icons[1] = par1IconRegister.registerIcon("condensedDeathstone"); icons[2] = par1IconRegister.registerIcon("smoothDeathstone"); icons[3] = par1IconRegister.registerIcon("deathstoneBrick"); icons[4] = par1IconRegister.registerIcon("carvedDeathstone"); } @SideOnly(Side.CLIENT) public Icon getIcon(int par1, int par2) { return icons[par2]; } public int quantityDropped(Random random){ return 1; } public int damageDropped(int metadata) { return metadata; } @SideOnly(Side.CLIENT) public void getSubBlocks(int par1, CreativeTabs par2CreativeTabs, List par3List) { for (int var4 = 0; var4 < 5; ++var4) { par3List.add(new ItemStack(par1, 1, var4)); } } } ItemBlock Class: public class ItemBlockBacon extends ItemBlock { public ItemBlockBacon(int par1) { super(par1); setHasSubtypes(true); } public String getUnlocalizedName(ItemStack itemstack) { String name = ""; switch(itemstack.getItemDamage()) { case 0: { name = "deathstone"; break; } case 1: { name = "crackedDeathstone"; break; } case 2: { name = "condensedDeathstone"; break; } case 3: { name = "smoothDeathstone"; break; } case 4: { name = "deathstoneBrick"; break; } case 6: { name = "carvedDarkstone"; break; } default: name = "broken"; } return getUnlocalizedName() + "." + name; } public int getMetadata(int par1) { return par1; } } Stair Class: public class StairBacon extends BlockStairs { protected StairBacon(int par1, Block par2Block, int par3) { super(par1, par2Block, par3); // TODO Auto-generated constructor stub } public void updateIcons(IconRegister par1iconregister){ this.blockIcon = par1iconregister.registerIcon("Bacon.BLOCK_ID"); } } Have any ideas how I could get this to work? I have way too many blocks for me not to use metadata blocks, but I would really like to have stairs of some of them. I'll keep fiddling with things in the meantime.