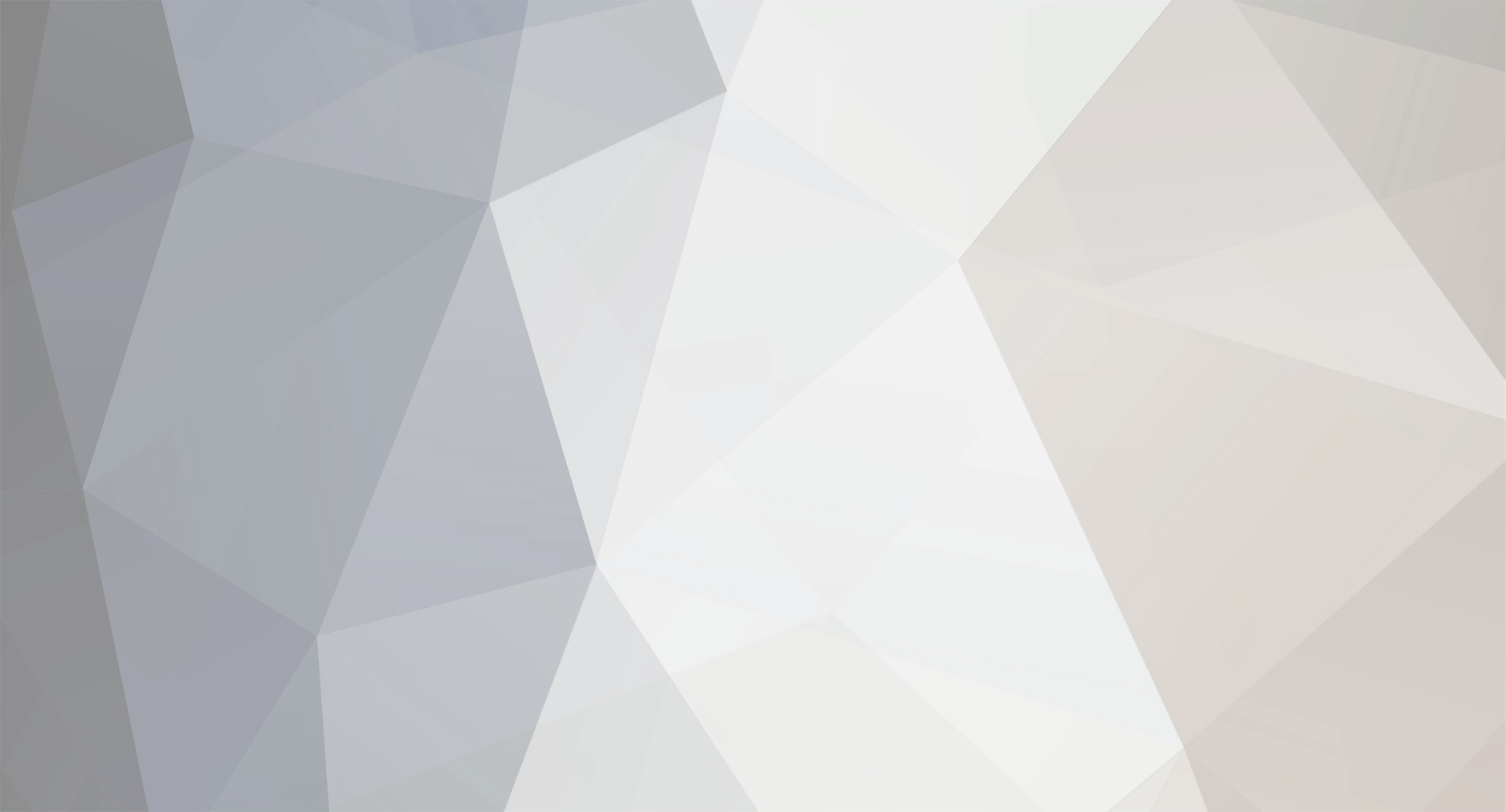
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
> Was trying to find (in MC) where exacly that happens (reading pixels and making cubes) - do you know by any chance? It is in ItemModelGenerator, the whole class is dedicated to doing that. It uses some optimisations so it doesn't actually draw cubes for all sides, only the ones at the edge of the image (i.e. where you have two cubes next to each other, it doesn't create the two quads that are between the cubes.) If your image doesn't have many holes, this will save you up to 2/3 of the quads. But otherwise, yes that's essentially how it does it, for the "edges with thickness" anyway. Once you've got the basic idea working, you can optimise further - eg by drawing the two flat faces with one quad each (with transparent holes) - in which case you are back to stitching your texture into the TextureMap again. This is how vanilla does the flat faces. There is probably also a way to only use the 'thickness' model with first person and third person views, and switch to a simple 2D model for inventory views, which will save a lot of rendering. (But I'm not sure how, you'd need to hunt around for it) -TGG 256x256 is probably too big in either case
-
The way I was thinking, you would need three models only, to define the shape of the hilt, the guard, and the blade. But it sounds like you want the hilt, guard and blade to have different shapes instead of just different colours/patterns. In that case, you will need to generate a model from the .png files, like vanilla does with the vanilla sword. That's trickier stuff, but not impossible. It has the advantage you don't need to mess around with registering your textures in the TextureMap, but is also a lot more complicated. Your five step process above looks about right to me in that case. You will need to read the texel values from the textures, and turn each texel into a cube using 6 quads. So your sword effectively becomes a flat layer of cubes, up to 16x16 in total, one for each texel in your sword's 2D picture. The vanilla code for generating item models from 2D textures is in ItemModelGenerator, but I should warn you it's pretty hard going. If you're doing it this way, you don't need to stitch your textures into the TextureMap, you can read your texture into a BufferedImage instead (see SimpleTexture.loadTexture) and just read out the texels one by one using code, but it does mean a lot more effort in your model baking. I think step 1 for you is probably to master generating a small cube from quads using ISmartItemModel to bake your own- similar to how MBE15 does it for the chessboard pieces, except all 6 faces instead of just the one. Once you've done that, I'd suggest you then add the texel reading from png, with one cube per quad (make the cubes just slightly smaller than 1/16, so they don't overlap) Once that works, figure out how to cache it so you don't have to regenerate up to 256*6 quads for every single sword, for every single frame... yeah learning minecraft rendering had that effect on me too... -TGG
-
I alredy some experiments, but I have to ask: What do you mean by above? Particulary: "you will need to load in all the textures in advance" - My textures (like one shown in op post) are loaded on startup (are simply all inside assets). E.g.: "weaponpart_shortsword_blade_demonic.png" Whole "weaponpart_shortsword_blade_" is derived from Class, only "demonic" is read from NBT. "If you add an item with a new NBT later, you will need to refresh/restitch the texture sheet" I mean, there will be like hundreds of different guards and blades. What exacly are we talking about here? Isn't .png being in assets folder enough? I have to manually add it in code? It's not enough to just have your png file inside assets, you need to register its ResourceLocation so that it gets stitched into the texture sheet. Basically: the textures for all items and blocks are stitched together into a single big texture, kind of like a patchwork quilt. See the "Texture Coordinates" image on this page for an idea of what it looks like. http://greyminecraftcoder.blogspot.co.at/2014/12/the-tessellator-and-worldrenderer-18.html Once your png file is stitched into the texture sheet you can make BakedQuads which refer to it and render it like a normal item does. -TGG
-
Hi I think there are a couple of key points - you will need to load in all the textures in advance; a custom ModelLoader can do this for you (MBE05 - ModelLoader3DWeb and WebModel) so long as it has all the paths from your NBTs. If you add an item with a new NBT later, you will need to refresh/restitch the texture sheet. This is probably the biggest difference from IItemRenderer, i.e. it's rather difficult to bind your own texture just for the rendering of your item. (I tried, gave up because it was too glitchy for me). - assuming all hilts have the same shape, and all guards the same shape, and all blades the same shape, have a model for each (i.e. 3 models total) and make sure they overlap nicely. - in your ISmartItemModel, use the NBT to pick the three models, work through each BakedQuad to modify the uv coordinates to match the target texture coordinates. MBE15 - ChessboardSmartItemModel does something very similar in createBakedQuadForFace / vertextToInts You can make your weapons appear double size by giving them a larger scale in the model json or by modifying IBakedModel.getItemCameraTransform (similar to how MBE14 does it) -TGG
-
This link might help http://www.minecraftforge.net/forum/index.php/topic,26772.msg136666.html#msg136666 DaBooty has good advice too -TGG
-
Hi The centre of your rendering screen is not a point in the world, of course, it's a line vector that extends perpendicular to the screen. The position of the camera in the world, in first person view at least, is the player's eye position. For third person, from memory the centre of the screen is in the middle of the player's head (the eye position) - so depending on what you want to do, the player's eye position might also be suitable in third person views. -TGG
-
your blockstates file has a syntax error: Encountered an exception when loading model definition of 'sureencore:zombie_spawner_block#age=2' from: 'sureencore:blockstates/zombie_spawner_block.json Unterminated object at line 4 column 10 -TGG
-
ah, I meant this.createBlockState(), but after thinking about it I reckon that probably won't work anyway. Trial and error might be your only chance here mate, it's for sure not leaping out at me. -TGG
-
Hi This site http://greyminecraftcoder.blogspot.com.au/p/list-of-topics.html and this "tutorial by example" project https://github.com/TheGreyGhost/MinecraftByExample might be what you're looking for. -TGG
-
Item Rendering Doesn't Work with Direction
TheGreyGhost replied to Whyneb360's topic in Modder Support
Hi Check out TileEntityChestRenderer, it has a similar example. The key problem is - your TileEntityRenderer is trying to use the TileEntity's direction to figure out which direction to render. That only makes sense if your TileEntity is associated with a block that you've placed in the world. The if statement goes in your renderer before you try to use the world object to retrieve the direction. -TGG -
Item Rendering Doesn't Work with Direction
TheGreyGhost replied to Whyneb360's topic in Modder Support
Hi so... either tileEntity is null or tileEntity.world is null. Set a breakpoint or System.out.println there, to find out which one... In this case tileEntity.world is null. You are trying to pull information about a block from a TileEntity which you created from nothing new ItemRenderPottedPlant(renderPottedPlant, new TileEntityPottedPlant())) Your renderer needs to check for that, and use a default value if it's an item not a block. eg if (tileEntity.hasWorldObj()) { get value from world block } else { use default value } -TGG -
this.getBlockState().getBaseState() should probably be this.getBaseState() -TGG
-
Item Rendering Doesn't Work with Direction
TheGreyGhost replied to Whyneb360's topic in Modder Support
What is the crash message? Which line does it point to in your code? -TGG -
Hi This tutorial project shows how to register a "non-pulling" model properly https://github.com/TheGreyGhost/MinecraftByExample/tree/master/src/main/java/minecraftbyexample/mbe12_item_nbt_animate and in particular https://github.com/TheGreyGhost/MinecraftByExample/blob/master/src/main/java/minecraftbyexample/mbe12_item_nbt_animate/StartupClientOnly.java -TGG
-
Yeah that guide was written for an older build of 1.8. The most recent recommended Forge build very unhelpfully hides all the error messages, in particular the file not founds which give most of the diagnostic information. The problem is here GameRegistry.registerItem(schwarzerdiamantbogen, "schwarzerdiamantbogen"); ModelBakery.addVariantName(schwarzerdiamantbogen, "kriegnesmod:schwarzerbow_standby", "kriegnesmod:schwarzerbow_pulling_0", "kriegnesmod_schwarzerbow_pulling_1", "kriegnesmod:schwarzerbow_pulling_2"); spot the difference kriegnesmod_schwarzerbow_pulling_1 Whenever you have a error like this with three identical objects, where two of them work fine and the other doesn't, a careful comparison will nearly always show you the problem very quickly. -TGG
-
Hi This link might help http://greyminecraftcoder.blogspot.com.au/2015/03/troubleshooting-block-and-item-rendering.html -TGG
-
I would suggest RenderWorldEvent.Post. Find the correct pass for the block you are looking at, when RenderWorldEvent.Post is called for that pass, render the block the player is looking at, slightly expanded so it covers up the already-drawn block faces. -TGG
-
If it's your own custom block: yes. Use getActualBlockState() to figure out if the player is looking at your block. If it's a vanilla block - yes, but it's harder. You will need to re-render the block yourself over the top of the existing block, eg from the render event at the appropriate render pass. Will be tricky to get right. -TGG
-
[1.8] [OpenGL] Using a Framebuffer breaks further rendering
TheGreyGhost replied to Rene8888's topic in Modder Support
Hi >Thanks for your reply and your great blog about Minecraft architecture and informations! No worries, you're welcome Strange symptoms. I don't know, to be honest. Some thoughts: 1) You don't need to call unbindFrameBuffer, it is called in deleteFramebuffer() already 2) Do the symptoms remain if you bind the buffer then unbind again immediately without rendering to it? i.e. perhaps your setColor etc methods are doing something strange - try to narrow it down? 3) If you are changing settings in your setColor , fillCircle etc using the GlStateManager, it caches the settings but doesn't dirty the cache properly, so vanilla can sometimes change the settings through GLStateManager but OpenGL doesn't see it. Solution is to use GL11 calls directly instead of GLstateManager 4) Some rendering settings are not saved by glPushAttrib(GL_ENABLE_BIT). Check out the OpenGL docs to see other settings. You could try doing your setColor, fillRect etc using the Tessellator and worldRenderer - starting simple and building up more complicated. -TGG -
Are you using IntelliJ14? If so you need to add a line to the end of your build.gradle file sourceSets { main { output.resourcesDir = output.classesDir } } or idea { module { inheritOutputDirs = true } } see here http://www.minecraftforge.net/forum/index.php/topic,21354.0.html Otherwise, you could try putting a breakpoint into ModelLoader.VanillaLoader.loadModel to see why it couldn't find your file - the exception e will have more information. try { return loader.new VanillaModelWrapper(modelLocation, loader.loadModel(modelLocation)); } catch(IOException e) { if(loader.isLoading) // breakpoint here { // holding error until onPostBakeEvent } else FMLLog.log(Level.ERROR, e, "Exception loading model %s with vanilla loader, skipping", modelLocation); return loader.getMissingModel(); } -TGG
-
[1.8] [OpenGL] Using a Framebuffer breaks further rendering
TheGreyGhost replied to Rene8888's topic in Modder Support
HI YOu are messing with a pile of the OpenGL settings and not saving/restoring them properly. What's the reason you can't just use the vanilla frame buffer helper? This class is a working example of how you can render to a framebuffer object https://github.com/TheGreyGhost/SpeedyTools/blob/master/src/main/java/speedytools/clientside/selections/SelectionBlockTextures.java start from the updateTextures() method... -TGG -
[1.8] MinecraftByExample sample code project
TheGreyGhost replied to TheGreyGhost's topic in Modder Support
keen yeah that would be awesome if you figure it out. I'll add it to the 'todo' list but probably won't get to it for quite a while... -TGG -
RenderGameOverlayEvent not working with server
TheGreyGhost replied to joecanmakeit's topic in Modder Support
Hi This link might also be helpful for background info on Proxies and the crash-in-dedicated-server problem. http://greyminecraftcoder.blogspot.com.au/2013/11/how-forge-starts-up-your-code.html -TGG