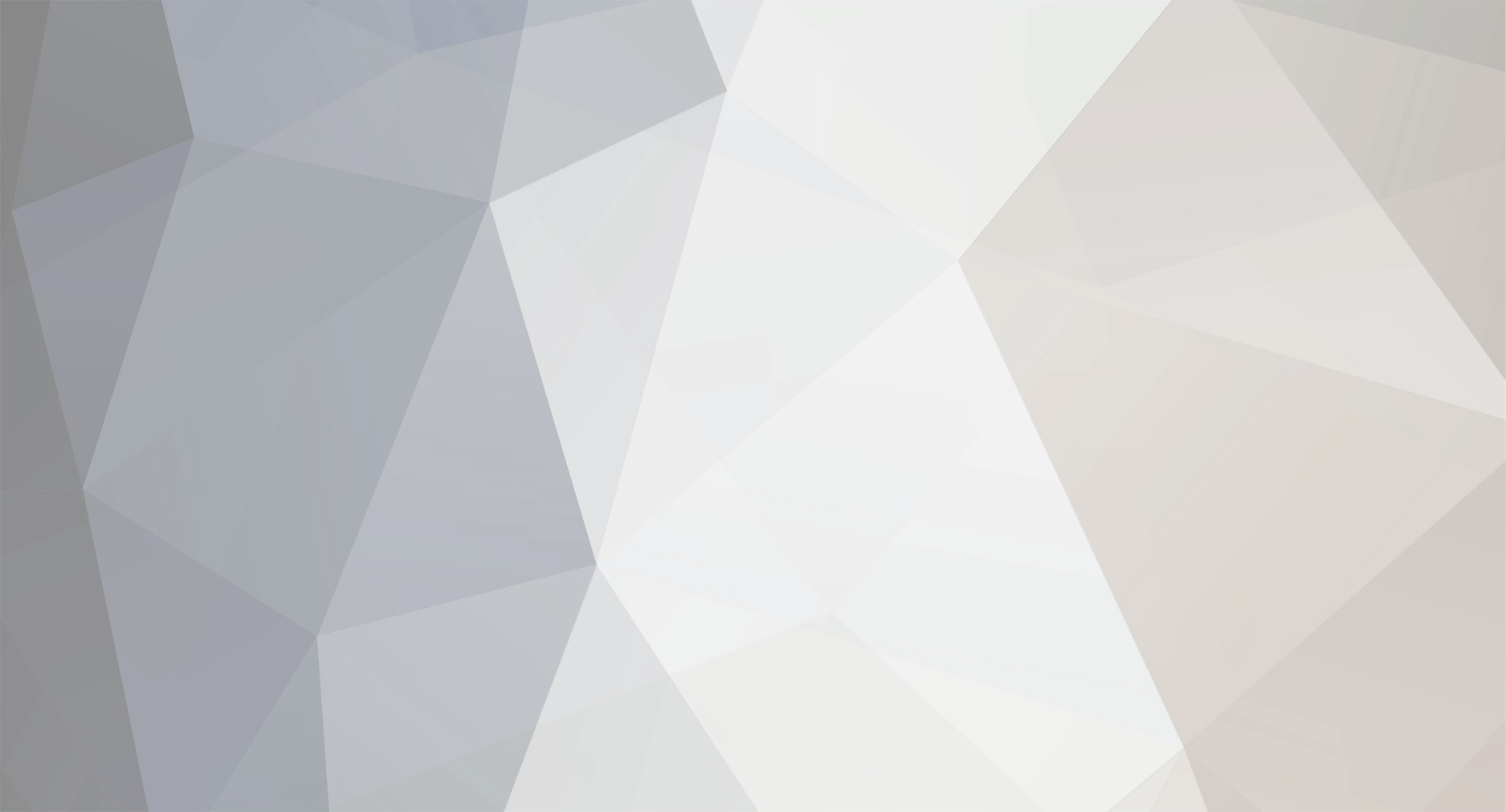
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
Hi The problem is that you're still trying to store information about each block in places that aren't appropriate. There is only one Block. So when you change top in that block, all the blocks change. You have to store the top information in the metadata, in the tile entity, or cheat with the [x,y,z] like dieSieben suggested. If you're having trouble understanding this, these two links might help http://greyminecraftcoder.blogspot.com/2013/10/the-most-important-minecraft-classes_9.html http://greyminecraftcoder.blogspot.com.au/2013/07/blocks.html To show you a proof of concept - try this @SideOnly(Side.CLIENT) public IIcon getIcon(IBlockAccess world, int x, int y, int z, int side) { int meta = world.getBlockMetadata(x, y, z); final int NUMBER_OF_TOP_ICONS = 10; int topIconIndex = (x+y+z) % NUMBER_OF_TOP_ICONS; // "randomly" select a texture for the table top. return side == 1 ? tableIconTop[topIconIndex] : (side == 0 ? tableIconTop[0] : (side != meta ? tableIconSide : tableIconFront)); } -TGG
-
Problem with Packet Causes Crash on World Load
TheGreyGhost replied to kenoba10's topic in Modder Support
Hi My guess is the error is here tileentity.getTankInfo(ForgeDirection.UNKNOWN)[0].fluid. -> probably your tileentity hasn't initialised itself correctly, so that getTankInfo returns a null or the array it returns has null fluid entries Show your TileEntity class? -TGG -
Hi Post your attempt? That method you quoted is the correct one (my getBlockTexture() was from 1.6.4, sorry about that) and it should be really simple to implement. -TGG
-
Hi I would suggest starting with something easy, i.e. just try to draw a single point. Is that what you want to do, i.e. draw a number of dots? Or something more fancy? There are two separate parts to this 1) find the dot location 2) draw the dot Show us an example of your code that worked the best for each part of that? A cut and paste of EntityRenderer.getMouseOver() should do basically everything you need for part 1. In your TileEntitySpecialRenderer.renderTileEntityAt(TileEntity par1TileEntity, double xOffset, double yOffset, double zOffset, float par8) your rendering code should look something like this, where [dx, dy, dz] is the difference between your wall spot and the tile entity, i.e. if your tile entity world x = 25 and the spot on the wall is at x = 32, then dx = 7 [umin, vmin] and [umax, vmax] are the texture coordinates you want to draw For the example where the dot is on the west (x negative) wall: GL11.glPushMatrix(); GL11.glTranslatef(xOffset, yOffset, zOffset); double SMALL_OFFSET = 0.001; double DOT_HEIGHT_DIV_2 = 0.1; double DOT_WIDTH_DIV_2 = 0.1; tessellator.startDrawingQuads(); tessellator.setColorRGBA(255, 255, 255, 255); // all white tessellator.addVertexWithUV(dx - SMALL_OFFSET, dy - DOT_HEIGHT_DIV_2, dz +DOT_WIDTH_DIV_2, umax, vmax); tessellator.addVertexWithUV(dx - SMALL_OFFSET, dy + DOT_HEIGHT_DIV_2, dz + DOT_WIDTH_DIV_2, umax, vmin); tessellator.addVertexWithUV(dx - SMALL_OFFSET, dy + DOT_HEIGHT_DIV_2, dz - DOT_WIDTH_DIV_2, umin, vmin); tessellator.addVertexWithUV(dx - SMALL_OFFSET, dy - DOT_HEIGHT_DIV_2, dz - DOT_WIDTH_DIV_2, umin, vmax); tessellator.draw() GL11.glPopMatrix(); I haven't compiled this code but it should be about right. To draw on other wall faces you will need to alter the addVertexWithUV commands to draw parallel to the face. If you don't properly understand the tessellator code, looking at this link might help. http://greyminecraftcoder.blogspot.com.au/2013/08/the-tessellator.html -TGG
-
Hi From memory you need to implement these methods in your custom TileEntity, so that the saved value of facing on the server is communicated to the client upon world reload. /** * Called when you receive a TileEntityData packet for the location this * TileEntity is currently in. On the client, the NetworkManager will always * be the remote server. On the server, it will be whomever is responsible for * sending the packet. * * @param net The NetworkManager the packet originated from * @param pkt The data packet */ public void onDataPacket(INetworkManager net, Packet132TileEntityData pkt) { } public Packet getDescriptionPacket() { } A tutorial on TileEntities should show the way. Unfortunately I don't remember specifics off the top of my head. -TGG
-
[1.7.2][SOLVED] Best way for making an Item with "subsets"?
TheGreyGhost replied to hugo_the_dwarf's topic in Modder Support
Hi Re names: Check out how ItemColored does it... Re crafting: Use ItemStacks with the correct damage value. -TGG -
[SOLVED][1.7.2] How to find the EntityItem I'm looking at?
TheGreyGhost replied to asaskevich's topic in Modder Support
Hi I think you will probably need to execute your own line-of-sight code, similar to EntityRenderer.getMouseOver(), because the vanilla has this line (entity.canBeCollidedWith()) which ignores any entities you can't collide with, including EntityItem. The RenderWorldLastEvent is a suitable place to execute that code. -TGG -
Hi This link might help with the Tessellator http://greyminecraftcoder.blogspot.com.au/2013/08/the-tessellator.html The block scanning code will look very similar to the vanilla code I mentioned. The basic idea is: 1) start from the middle of your ball 2) choose a direction vector for your ray of light from the ball (in the case of the code I mentioned, this is the direction the player is looking) 3) calculate the intersection of that vector with one of the six faces of your ball, i.e. top, bottom, east, west, north, south 4) Check the block that is touching that face. 4a) if the block is solid, you mark this face as the position of the dot and draw it 4b) if the block is not solid, extend the vector into this block. Figure out which block face it will hit next, i.e. repeat from step 3 until you reach a maximum distance (say, 10 blocks) You will need some basic background in vector maths to understand what is happening in the vanilla code (i.e. enough to understand about vector direction, length, and intersection with a plane). -TGG
-
[SOLVED!] [1.7.2/1.7.10] Bound must be positive Error
TheGreyGhost replied to NovaViper's topic in Modder Support
hi I suggest you place a breakpoint at ChunkProviderNillax.populate(ChunkProviderNillax.java:467 and see why it is trying to generate a random number with an upper bound less than 0. My guess is that you haven't initialised your animal spawning list properly, i.e. maxGroupCount and minGroupCount, assuming my line numbering is the same as yours int i1 = spawnlistentry.minGroupCount + par6Random.nextInt(1 + spawnlistentry.maxGroupCount - spawnlistentry.minGroupCount); Are you familiar with breakpoints and watches? If not, try these http://www.vogella.com/tutorials/EclipseDebugging/article.html http://www.terryanderson.ca/debugging/run.html -TGG -
Hi I think you have mixed up metadata and flags. /** * Sets the block ID and metadata at a given location. Args: X, Y, Z, new block ID, new metadata, flags. Flag 1 will * cause a block update. Flag 2 will send the change to clients (you almost always want this). Flag 4 prevents the * block from being re-rendered, if this is a client world. Flags can be added together. */ public boolean setBlock(int wx, int wy, int wz, Block block, int metadata, int flags) -TGG
-
Hi The problem seems to be that trying to set blocks in dimension B, while you're generating dimension A, causes minecraft to start generating dimension B, which causes your generator to be called again, which asks minecraft to start generating yet another dimension (or the same one again), and so on. Eventually your recursion goes so deep you run out of stack space. I don't know if it's possible for a generator to mess around with other dimensions at the same time like this. You might need to do something a bit tricky, like have the portal appear a couple of seconds later after generation is finished (a scheduled update for the portal), or have a fixed relationship between your portals so that your generator in one dimension automatically knows where the portals are in other dimensions based only on the [x,y,z] coordinate. -TGG
-
Hi easiest way = override Block.getBlockTexture(), which provides [x,y,z], to return one of your textures based on the [x,y,z] as per DieSieben's suggestion. next easiest way = store the texture information in your TileEntity, and render the sides in the TileEntityRenderer not the Block. The bottom line is - if you want each placed container to render a different texture, and you don't want to use the [x,y,z] method, you have to store that texture somewhere for each container. You can't use metadata, so you must use something else. Your texture is changing for all containers at once because you are not storing the necessary information for each placed container. -TGG
-
[1.7.10]How to make two block high plants
TheGreyGhost replied to CroComeT's topic in Modder Support
Step #1: make your icons 16x16 pixels each. If your icons are currently 16x32, split them into two separate 16x16 images. For example corn_0A.png and corn_0B.png Fix that, then try again and post us the result. -TGG -
[1.7.10]How to make two block high plants
TheGreyGhost replied to CroComeT's topic in Modder Support
I don't understand your question. Your initial question was about the black and purple texture. Have you fixed that yet? If not, post your error log again. -TGG -
Yes they would change the hue of the image. Assuming they're just drawing plain dots, they don't use an image. EntityRenderer.getMouseOver() is a good example of this. EntityLivingBase.rayTrace() --> World.rayTraceBlocks_do_do() does all the work for the blocks part of it. The rest of getMouseOver handles collisions with Entities. -TGG
-
Hi If you just want a grey dot, then you don't need an image. Just use the Tessellator.setColorRGBA() and then Tessellator.addVertex() instead of Tessellator.addVertexWithUV() You would need to check every block around the centre emitter. But so long as you don't try and go silly with the range of your dots, or have too many dots, I wouldn't worry about the efficiency. Retrieving blocks is very fast. -TGG
-
Hi You can draw whatever you like in the TileEntity Renderer. The basic idea is: 1) in your tile entity tick, shoot out a ray for every spot and figure out where it hits a nearby wall. (MovingObjectLocation and EntityRenderer.getMouseOver gives you an example of how that can be done). Remember that location. 2) in your tile entity renderer, take the location of every spot and draw a small rectangle using the Tessellator. The hardest part is making sure that the [x,y,z] translation is set up correctly to align with the world [x,y,z] coordinates. Standard tutorials on TileEntitySpecialRenderer will show you how to do that. Look at EntityDropParticleFX and EntityFX.renderParticle for some clues on how to use the tessellator. Remember to move your particle slightly away from the wall so that it renders just on top of the wall and not inside it. -TGG
-
[1.7.10][Solved]Custom ItemTool Renderer in Hand
TheGreyGhost replied to Taji34's topic in Modder Support
Hi The link I sent earlier also has a bit about the Tessellator, which is what most of this code does. I find it helps to copy the class to a new file and use my IDE to rename the variables to something meaningful. In this case, the method is effectivelty converting each pixel in the icon to a cube. It might be more clear if you look at the code which calls it to see what the parameters being passed are, i.e. texturemanager.bindTexture(texturemanager.getResourceLocation(par2ItemStack.getItemSpriteNumber())); Tessellator tessellator = Tessellator.instance; float f = icon.getMinU(); float f1 = icon.getMaxU(); float f2 = icon.getMinV(); float f3 = icon.getMaxV(); // .. some extra stuff not relevant ... renderItemIn2D(tessellator, f1, f2, f, f3, icon.getIconWidth(), icon.getIconHeight(), 0.0625F); -TGG -
cunning, I like it. You could avoid any obvious patterns by using a random number generator with a seed based on your [x,y,z], something like long seed = (x & 255) | ((z & 255) << | (y << 16); Random generator = new Random(seed); int myRandomTexture = generator.nextInt(MY_MAXIMUM_TEXTURE_NUMBER_PLUS_ONE); -TGG
-
[10.13.1188] NetHandlerPlayClient Attribute Bug
TheGreyGhost replied to TLHPoE's topic in Modder Support
Hi It appears that in your ModAttributes.loadAttributes, you have created two new ModAttributes which are being applied to all living creatures by the server. So when the server sends an entity packet to the client, it includes information about those ModAttributes. The Entities themselves don't contain these two new ModAttributes, which are normally added to attributeMap during construction (eg see EntityLivingBase.applyEntityAttributes()). When the client gets the S20 packet, which contains information about your new ModAttributes (eg stepHeight), it looks for your new ModAttributes in the entity's attributeMap, doesn't find them, attempts to create a default object to hold the information, but due to a bug in vanilla, it crashes. I think the answer is to make sure you also register your new attributes during construction of entities on the client side in public void entityConstruct(EntityConstructing event) { -TGG -
[1.7.10][Solved]Custom ItemTool Renderer in Hand
TheGreyGhost replied to Taji34's topic in Modder Support
Hi The Item Rendering sections in this link will probably be useful. It is written for 1.6.4 but the code structure is still the same. http://greyminecraftcoder.blogspot.com.au/p/list-of-topics.html -TGG -
[1.7.10]How to make two block high plants
TheGreyGhost replied to CroComeT's topic in Modder Support
What that error actually means: Minecraft expects your icon to have the same height and width, for example 16 x 16. The only images which it will accept which don't follow this rule are animations. So it is telling you that the height and width of your icon don't match each other (that's what aspect ratio means), because you're not trying to do an animation. -TGG -
[1.7.10] [SOLVED] problems with key bindings.
TheGreyGhost replied to sigurd4's topic in Modder Support
Hi If you're just new to Java, this post might be of interest http://www.minecraftforge.net/forum/index.php/topic,16784.msg84954.html#msg84954 you can compare strings, just use .equals() -TGG