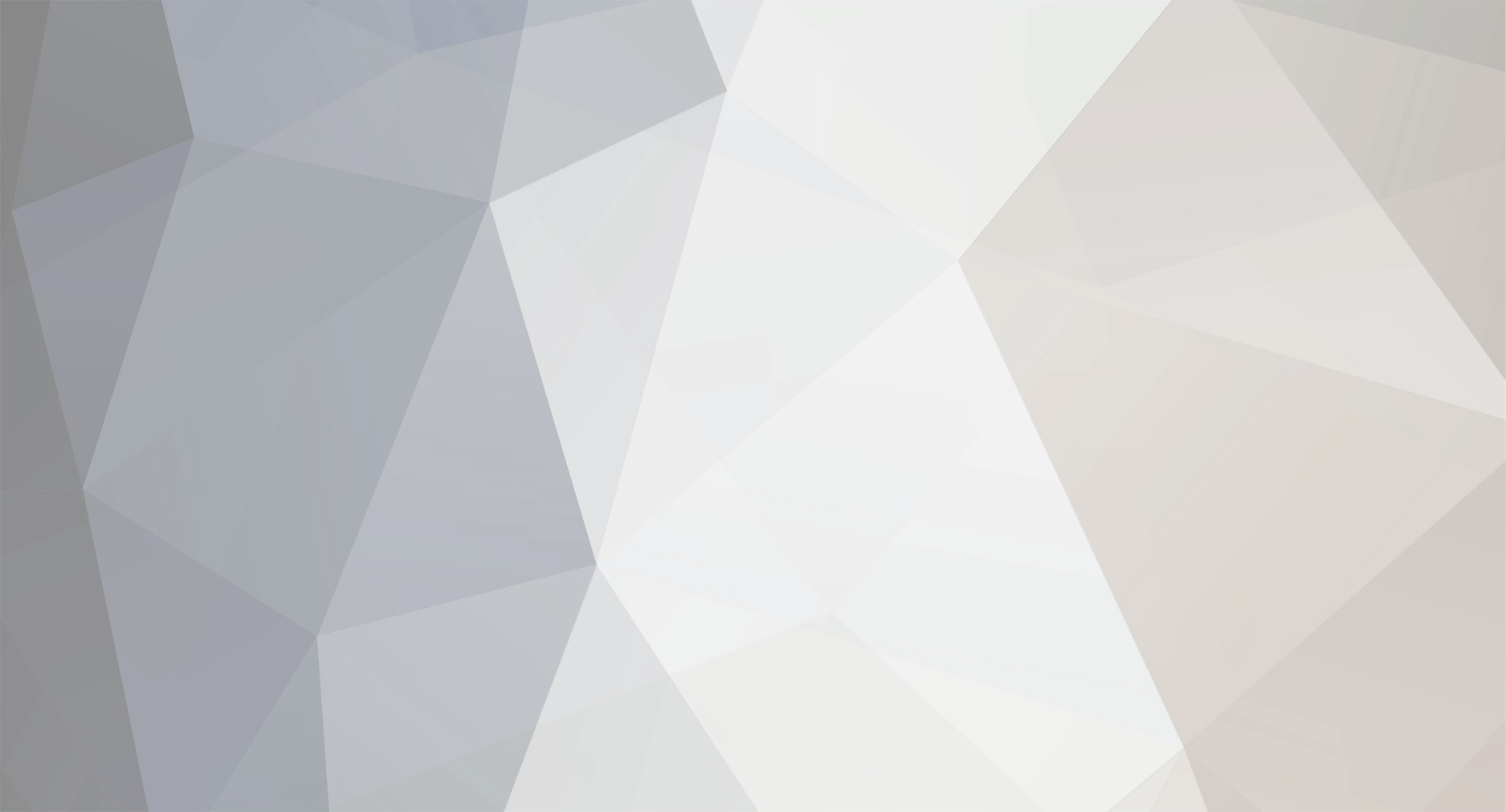
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
:-) Ha yeah I've learnt the value of automated unit testing the hard way... subtle little bugs that made my database lose every 1024th row of data, introducing a memory leak while fixing a different problem that caused my app to crash after running for 3 hours, an object-copying mistake between two classes that made the player disappear everytime he moved left and pushed fire at the same time. Having to re-do 3 hours of extremely boring manual testing, twice, when fixing another bug. Anyway - I got a suggestion in another forum which I'm trying out now: http://objenesis.org/tutorial.html It lets you instantiate classes without calling any of the constructors. The object is in an invalid state of course (all member objects are null), but you can override its methods with your own stubs, pass the instantiated object to your class under test, and it will call them no problem. I've just tested it on EntityPlayerMP and it worked a treat. -TGG
-
Hi If you're still learning IItemRenderer, this link may also be useful http://greyminecraftcoder.blogspot.com.au/p/list-of-topics.html -see the "Item Rendering" topics. -TGG
-
Howdy all I've got a general question about unit and integration testing in Forge. I'm trying to write unit tests for some of my new classes and it's proving almost impossible any time my classes refer to any of the vanilla classes such as EntityPlayerMP or similar because writing stubs or mocks for them is just too complicated. All I really need is to override a couple of the class methods with stubs, but the classes generally don't have a default constructor which makes it a real pain. Anyone got any tricks you can recommend? I'm currently resorting to inserting my test harnesses into the mod code and running it like a normal mod, which is pretty clunky. Surely there's got to be a better way? -TGG
-
Hi GL11.glTranslatef GL11.glRotatef GL11.glScalef A useful guide: http://www.glprogramming.com/red/index.html -TGG
-
TileEntity renderer just renders in black
TheGreyGhost replied to Bedrock_Miner's topic in Modder Support
Hi If you want the face to be opaque, you should turn off BLEND before rendering. i.e. GL11.glDisable(GL11.GL_BLEND); You probably also don't want to disable the depth mask before rendering the face, otherwise entities that are behind the face might render over the top of it if they're drawn afterwards. GL11.glDepthMask(true); -TGG -
[Resolved][1.7.2] Custom Log causing crash when rendered
TheGreyGhost replied to Minothor's topic in Modder Support
Hi Show us an example of your code that doesn't work? -TGG -
Hi Entity.getCollisionBox(), Entity.getCollidingBoundingBox(), Entity.boundingBox, or Entity.canBeCollidedWith(), depending on what you want to do exactly? -TGG
-
Hi As far as I can see Project Red's code is all about logic gates which I don't reckon would help you design a volcano much. I reckon you could have a decent chance of generating volcano shapes and spitting out flames, ash particles etc with some fairly simple math. The only math you need is in a lot of the vanilla already eg most of the particle stuff If you have any particular effect you're trying to achieve, may as well ask us :-) -TGG
-
Hi I'd guess that probably one of the OpenGL flags isn't set right. Perhaps GL11.glBlendFunc(GL11.GL_SRC_ALPHA, GL11.GL_ONE_MINUS_SRC_ALPHA); ? I wrote a brief class to print the current OpenGL settings, that might help figure out which flag(s) are different when the mob is in view vs when it isn't in view. Some more info here plus the link http://www.minecraftforge.net/forum/index.php/topic,16562.msg84272.html#msg84272 -TGG
-
Hi Just my guess, since it looks to me like one of the "cylinders" renders correctly and the rest don't: if(player.isSneaking()){ GL11.glPushMatrix(); GL11.glRotatef(-90, 0, 0, 1); Minecraft.getMinecraft().ingameGUI.drawCenteredString(Minecraft.getMinecraft().fontRenderer, Math.round(visA.getAmount(visA.getAspects()[i])/100)+"", -50, 50, 16777215); GL11.glPopMatrix(); } Probably drawCentredString changes one or more of the drawing attributes. You could try looking through it to see what flags get changed, alternatively it might work to use GL11.glPushAttrib(GL11.GL_ENABLE_BIT); and GL11.glPopAttrib(); -TGG
-
[1.7.2]Texture Issue on Custom Rendered Block[Solved]
TheGreyGhost replied to grim3212's topic in Modder Support
Hi Dude, that code sure is hard to follow, couldn't you name the variables something meaningful? :-) What texture do you want it to use? The standard block textures are all stitched together in a big single texture, so if you are using an icon on a different texture sheet which has a different number of items, the boundaries won't match up. Are you sure that the Tessellator is bound to the correct texture sheet? How did you register your texture (icons)? I'm pretty sure you already know about the Tessellator, but just in case you don't this link might be helpful http://greyminecraftcoder.blogspot.com.au/2013/08/the-tessellator.html This one on lighting might be useful too, since I don't think that this code will do what you expect: float f = block.getMixedBrightnessForBlock(world, x, y, z); tessellator.setColorOpaque_F(f, f, f); http://greyminecraftcoder.blogspot.com.au/2013/08/lighting.html -TGG -
Hi Yeah the problem is occurring here: at net.minecraftforge.fluids.FluidRegistry.getFluidID(FluidRegistry.java:119) public static int getFluidID(String fluidName) { return fluidIDs.get(fluidName); // here } static BiMap<String, Integer> fluidIDs = HashBiMap.create(); The only way that this can give NPE is if fluidIDs is null (because it hasn't been initialised yet), or (as I just realised) because the fluid hasn't been registered yet. So I suggest: (1) Create your fluid in the preInit method, not static initialiser (2) register your fluid (3) create your block in the preInit method, not static initialiser (4) register your block -TGG
-
Hi That's curious. I don't see anything obviously wrong. Perhaps the onWorldTick is being invoked too soon after the ChunkLoad. I'd suggest you add some more logging to your world load event, to figure out whether the problem is in your ChunkLoad or onWorldTick. -TGG
-
Hi Just my guess: perhaps it doesn't need to reload all the chunks if you have moved to the ender and back to the overworld. What happens if you save the game after killing the dragon, shut down the game, then restart and reload it? -TGG
-
[1.7.2] [SOLVED] Custom potion effect to armor
TheGreyGhost replied to GravityBurger's topic in Modder Support
Hi The TickRegistry and TickHandler have been replaced by TickEvent. You need to register them on the FMLCommonHandler.bus(), not the Forge bus. -TGG -
Hi In that case, I would suggest either you get someone else to run a client in a debugger, or you write to a disk file, or you change the player name from Reika to the name of one of the other players. So you see will the weird bodypart rendering that they see when looking at you, and you can debug it. -TGG
-
Hi This link gives a bit of background information about preInit and all the rest. http://greyminecraftcoder.blogspot.com.au/2013/11/how-forge-starts-up-your-code.html It's a little bit outdated now but the concepts are the same. The reason to use preInit and the others is to make sure that your objects are created at the correct time. I suspect this might be the problem in your case. Re the brewing stand: the answer is almost certainly yes, but I haven't done anything with Brewing Stands myself so I'm not sure. PotionBrewedEvent might be a good place to start; http://www.minecraftforum.net/topic/1419836-forge-4x-events-howto/ -TGG
-
Hi It looks to me like FluidRegistry hasn't initialised yet. Perhaps try moving the static initialisation of these public static Fluid FluidHydrofluoricAcid_ = new Fluid("Hydrofluoric Acid"); public static Material MaterialHydrofluoricAcid_ = new MaterialLiquid(MapColor.clothColor); public static Block HydrofluoricAcid_ = new HydrofluoricAcid(FluidHydrofluoricAcid_, MaterialHydrofluoricAcid_); to your preInit method, eg public static Fluid fluidHydrofluoricAcid; PreInit method: fluidHydrofluoricAcid = new Fluid("Hydrofluoric Acid"); // etc ... I didn't really understand your question about the brewing stand. -TGG
-
[1.7.2] - No damage when spawning lightning
TheGreyGhost replied to AppliedOnce's topic in Modder Support
No worries :-) I tried this code in 1.6.4 and it seems to work fine: @Override public ItemStack onItemRightClick(ItemStack itemStack, World world, EntityPlayer player) { if (world.isRemote) { System.out.println("Client: player y= " + player.getPosition(1.0F).yCoord); } else { System.out.println("Server: player y=" + player.getPosition(1.0F).yCoord); } final double EYE_HEIGHT = 1.62; // this is a guess... need to confirm it final double reachDistance = 300; Vec3 startPos = player.getPosition(1.0F); if (!world.isRemote) startPos = startPos.addVector(0, EYE_HEIGHT, 0); Vec3 look = player.getLook(1.0F); Vec3 endPos = startPos.addVector(look.xCoord * reachDistance, look.yCoord * reachDistance, look.zCoord * reachDistance); MovingObjectPosition mop = world.clip(startPos, endPos); System.out.println((world.isRemote ? "Client-" : "Server-") + " HitVec:[" + mop.hitVec.xCoord + ", " + mop.hitVec.yCoord + ", " + mop.hitVec.zCoord + "]"); if (mop != null && mop.typeOfHit == EnumMovingObjectType.TILE) { int i = mop.blockX; int j = mop.blockY; int k = mop.blockZ; System.out.println((world.isRemote ? "Client-" : "Server-") + "HitBlock:[" + i + ", " + j + ", " + k + "]"); world.spawnEntityInWorld(new EntityLightningBolt(world, i, j, k)); } return itemStack; } Right-click gives the following output, i.e. client and server hitvec and hitblock match, and are equal to where my cursor is pointing 2014-03-10 10:22:10 [iNFO] [sTDOUT] Client: player y= 5.620000004768372 2014-03-10 10:22:10 [iNFO] [sTDOUT] Client- HitVec:[-809.4795666515165, 4.0, -1480.5625485323928] 2014-03-10 10:22:10 [iNFO] [sTDOUT] Client-HitBlock:[-810, 3, -1481] 2014-03-10 10:22:10 [iNFO] [sTDOUT] Server: player y=4.0 2014-03-10 10:22:10 [iNFO] [sTDOUT] Server- HitVec:[-809.4795666515766, 4.0, -1480.5625485380956] 2014-03-10 10:22:10 [iNFO] [sTDOUT] Server-HitBlock:[-810, 3, -1481] If I move my mouse to move the look to the next block along in the x direction, it gives the expected result: 2014-03-10 10:23:17 [iNFO] [sTDOUT] Client: player y= 5.620000004768372 2014-03-10 10:23:17 [iNFO] [sTDOUT] Client- HitVec:[-810.474262374682, 4.0, -1480.5878117751354] 2014-03-10 10:23:17 [iNFO] [sTDOUT] Client-HitBlock:[-811, 3, -1481] 2014-03-10 10:23:17 [iNFO] [sTDOUT] Server: player y=4.0 2014-03-10 10:23:17 [iNFO] [sTDOUT] Server- HitVec:[-810.4742623718143, 4.0, -1480.587811780764] 2014-03-10 10:23:17 [iNFO] [sTDOUT] Server-HitBlock:[-811, 3, -1481] -TGG -
Hi Sorry dude, I'm way out of my depth there... never done a coremod. -TGG
-
[1.7.2] - No damage when spawning lightning
TheGreyGhost replied to AppliedOnce's topic in Modder Support
Hi I'd suggest using the code out of rayTrace instead of fiddling with the player object (and actually I don't think modifying player.eyeHeight will work, rayTrace doesn't seem to use it) final double EYE_HEIGHT = 1.6; // this is a guess... need to confirm it Vec3 startPos = this.getPosition(partialTick); if (!world.isRemote) startPos = startPos.addVector(0, EYE_HEIGHT, 0); Vec3 look = this.getLook(partialTick); Vec3 endPos = vec3.addVector(look.xCoord * reachDistance, look.yCoord * reachDistance, look.zCoord * reachDistance); MovingObjectPosition mop = world.func_147447_a(startPos, endPos, false, false, true); System.out.println("HitVec:[" + mop.hitVec.xCoord + ", " + mop.hitVec.yCoord + ", " + mop.hitVec.zCoord + "]"); -TGG -
Hi So I guess that's what prints out on your client, which seems to work as expected. What prints out on the clients of other users looking at you? @ForgeSubscribe public void addReikaModel(RenderPlayerEvent.Pre evt) { RenderPlayer render = evt.renderer; EntityPlayer ep = evt.entityPlayer; // add System.out.println here float tick = evt.partialRenderTick; //... if ("Reika_Kalseki".equals(ep.getEntityName())) { // add System.out.println here -TGG
-
[1.7.2] - No damage when spawning lightning
TheGreyGhost replied to AppliedOnce's topic in Modder Support
Hi I reckon when you try to run this on a server there's a good chance it will crash. In fact, in might occasionally crash on single player as well when you get unlucky (the client thread and the server thread try to both access Minecraft object at the same time) Minecraft mc = Minecraft.getMinecraft(); This only exists on the client side and if you try to access it from the server side it will cause problems MinecraftServer.getServer is what you need on the server side. Which will leave you back with your "feet height vs eye height" problem from before. You can correct for that by adding the difference on the server, 1.6 or something like that (use your println(ypos) from before to figure it out exactly...) -TGG