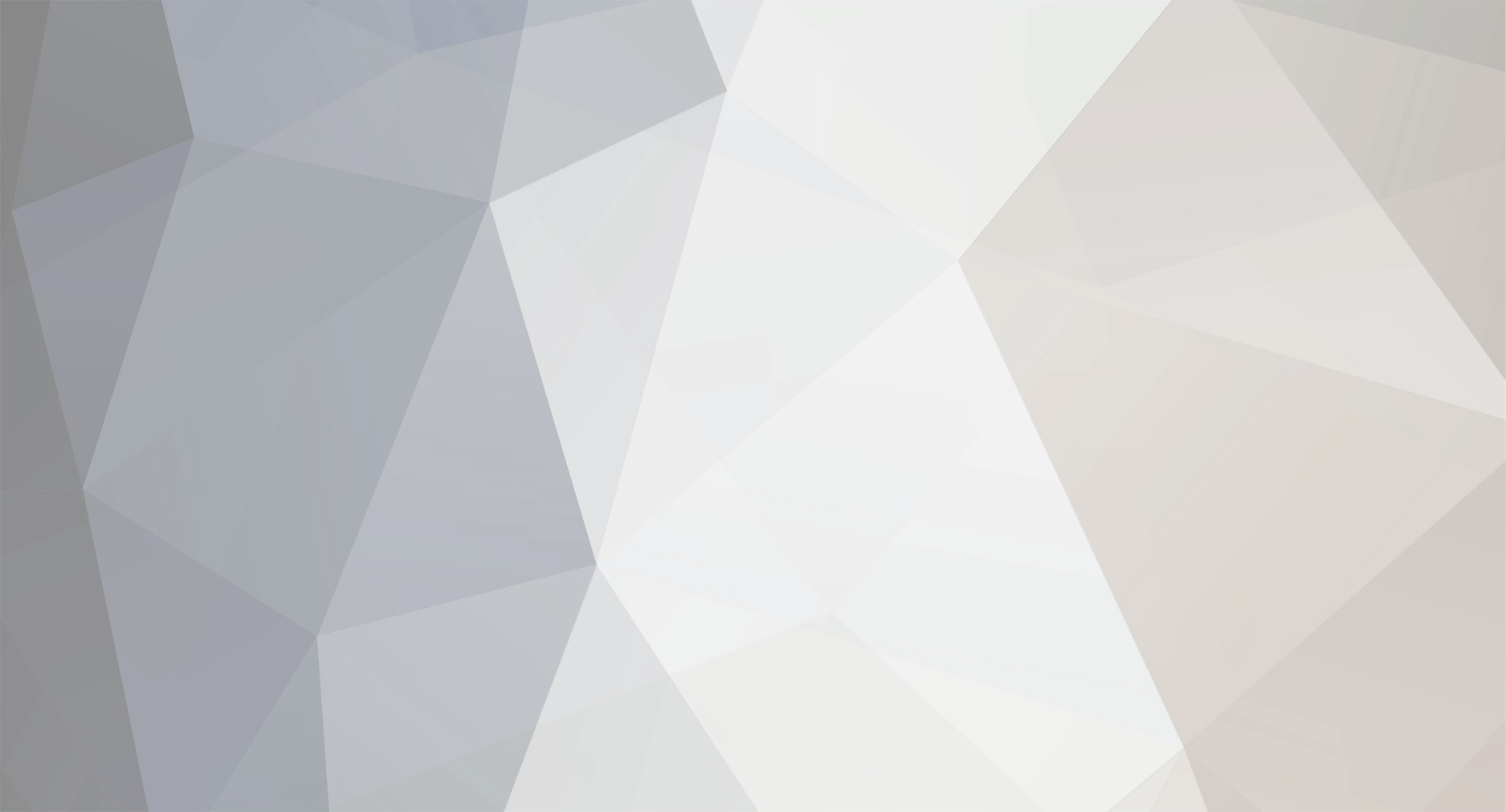
Romejanic
Forge Modder-
Posts
71 -
Joined
-
Last visited
Everything posted by Romejanic
-
Have you registered the entities? You should register them with EntityRegistry.registerModEntity and EntityList.addMapping
-
Hello, I'm making a messenger mod for the client. The person sending/receiving the message are identified by their Mojang UUID. However, I need to find a way to convert the UUID to the person's username to be shown on the GUI. This is client-side, so I'd like to do it without the MinecraftSessionService in the MinecraftServer. I've tried making a query to https://sessionserver.mojang.com/session/minecraft/profile/ and converting it to a GameProfile using Gson. Doesn't work. I've tried using a HttpProfileRepository to get it. It takes to long to respond, usually fails, and is for converting the username to a UUID. It is really important that I do this. It would be easier to use usernames as IDs, but I'd like to be ready for the name changes Here's my user class: package assets.mcmessenger.client.messenger; import java.awt.image.BufferedImage; import java.net.URL; import java.util.UUID; import javax.imageio.ImageIO; import net.minecraft.client.Minecraft; import net.minecraft.client.renderer.texture.DynamicTexture; import net.minecraft.util.ResourceLocation; import assets.mcmessenger.client.messenger.util.GameProfileCreator; import com.mojang.authlib.GameProfile; public class User { private static final String faceFetchUrl = "http://cravatar.eu/helmavatar/%s"; private String uuid; private String name; private ResourceLocation face; public User(String uuid) { this.uuid = uuid; if(this.uuid.equals(Minecraft.getMinecraft().getSession().getUsername())) { this.setName(Minecraft.getMinecraft().getSession().getUsername()); } else { try { GameProfile profile = GameProfileCreator.createGameProfileFromUUID(uuid); setName(profile.getName()); } catch(Exception e) { setName(e.toString()); } } } public String getName() { return this.name; } public void setName(String name) { this.name = name; } public UUID getUUID() { return UUID.fromString(uuid); } public ResourceLocation getFace() { if(this.face == null) { BufferedImage image = null; try { image = ImageIO.read(new URL(String.format(faceFetchUrl, getName()))); } catch(Exception e) { e.printStackTrace(); } DynamicTexture texture = new DynamicTexture(0, 0); if(image != null) { texture = new DynamicTexture(image); } this.face = Minecraft.getMinecraft().getTextureManager().getDynamicTextureLocation("face_" + getName(), texture); } return this.face; } public boolean equals(User user) { return user.uuid == this.uuid; } } Here's my profile creator: package assets.mcmessenger.client.messenger.util; import java.io.InputStreamReader; import java.io.Reader; import java.net.URL; import java.util.UUID; import org.apache.commons.io.IOUtils; import com.google.gson.Gson; import com.google.gson.GsonBuilder; import com.google.gson.stream.JsonReader; import com.mojang.api.profiles.Profile; import com.mojang.authlib.GameProfile; public class GameProfileCreator { private static final String fetchIdUrl = "https://api.mojang.com/users/profiles/minecraft/%s?at=%s"; private static final String fetchNameUrl = "https://sessionserver.mojang.com/session/minecraft/profile/%s"; public static Profile createGameProfileFromUsername(String username) { try { Gson gson = new GsonBuilder().setPrettyPrinting().create(); String url = String.format(fetchIdUrl, username, String.valueOf(System.currentTimeMillis())); JsonReader reader = new JsonReader(new InputStreamReader(new URL(url).openStream())); reader.setLenient(true); return (Profile)gson.fromJson(reader, Profile.class); } catch(Exception e) { e.printStackTrace(); } Profile profile = new Profile(); profile.setName(username); return profile; } public static Profile createGameProfileFromUUID(String uuid) { try { Gson gson = new GsonBuilder().setPrettyPrinting().create(); String url = String.format(fetchNameUrl, uuid); JsonReader reader = new JsonReader(new InputStreamReader(new URL(url).openStream())); reader.setLenient(true); return (Profile)gson.fromJson(reader, Profile.class); } catch(Exception e) { e.printStackTrace(); } Profile profile = new Profile(); profile.setId(uuid); return profile; } } If anyone can help, I would be SO grateful! Thank you very much for your time. - Romejanic
-
[1.7.10]How to I get entityPlayer of my own character?
Romejanic replied to TheiTay's topic in Modder Support
If you wanted to cheat it without server compatibility, you would use Minecraft.getMinecraft().thePlayer. Otherwise, you need to send a packet to the server with the username, obtained by using Minecraft.getMinecraft().thePlayer.getCommandSenderName(). The packet will locate a player by their username (matching it to the name in the packet) and move the player like that. Here's a code example: Packet Class public class PacketMovePlayer implements IMessage { public String username; public PacketMovePlayer(String username) { this.username = username; } public PacketMovePlayer() {} @Override public void toBytes(ByteBuf buf) { ByteBufUtils.writeUTF8String(buf, username); } @Override public void fromBytes(ByteBuf buf) { this.username = ByteBufUtils.readUTF8String(buf); } public class Handler implements IMessageHandler<PacketMovePlayer, IMessage> { @Override public IMessage onMessage(PacketMovePlayer message, MessageContext ctx) { for(Object o : MinecraftServer().getServer().getConfigurationManager().playerEntityList) { if(((EntityPlayer)o).getCommandSenderName().equals(message.username)) { // move player return null; } } return null; } } } Mod Class public static SimpleNetworkWrapper network; @EventHandler public void preInit(FMLPreInitializationEvent event) { // other pre-init stuff network = NetworkRegistry.INSTANCE.newSimpleChannel("your_mod_id"); network.registerMessage(PacketMovePlayer.Handler.class, PacketMovePlayer.class, 0, Side.CLIENT); } Teleport Code YourMod.network.sendToServer(new PacketMovePlayer(Minecraft.getMinecraft().thePlayer.getCommandSenderName())); Hope that helps! Just a side note, if you are doing the teleport code in an item, just reference the EntityPlayer parameter in your onItemRightClick method! - Romejanic -
Hye everyone, I am making a mod which replaces the Tall Grass/Dead Bush/Flower/etc rendering to realistic 3D models. I am using the advanced model loader to lead and render the models, but I cannot do anything in the block renderer other than using the RenderBlocks field or drawing it myself with the GL11.glVertex() functions. The tessellator will says it is already drawing when you call tessellator.startDrawing() (which the OBJ model does), and it says it is not drawing when I call tessellator.draw() to reset it. (I also tried to use the vanilla models by drawing ModelPig, and that didn't work either, same problem) Here's the crash report: ---- Minecraft Crash Report ---- // Hey, that tickles! Hehehe! Time: 1/8/15 5:53 PM Description: Unexpected error java.lang.IllegalStateException: Already tesselating! at net.minecraft.client.renderer.Tessellator.startDrawing(Tessellator.java:271) at net.minecraftforge.client.model.obj.WavefrontObject.renderAll(WavefrontObject.java:181) at assets.foliage.client.render.RealisticBlockRenderer.renderWorldBlock(RealisticBlockRenderer.java:48) at cpw.mods.fml.client.registry.RenderingRegistry.renderWorldBlock(RenderingRegistry.java:118) at net.minecraft.src.FMLRenderAccessLibrary.renderWorldBlock(FMLRenderAccessLibrary.java:53) at net.minecraft.client.renderer.RenderBlocks.renderBlockByRenderType(RenderBlocks.java:435) at net.minecraft.client.renderer.WorldRenderer.updateRenderer(WorldRenderer.java:207) at net.minecraft.client.renderer.RenderGlobal.updateRenderers(RenderGlobal.java:1647) at net.minecraft.client.renderer.EntityRenderer.renderWorld(EntityRenderer.java:1271) at net.minecraft.client.renderer.EntityRenderer.updateCameraAndRender(EntityRenderer.java:1095) at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:1067) at net.minecraft.client.Minecraft.run(Minecraft.java:961) at net.minecraft.client.main.Main.main(Main.java:164) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25) at java.lang.reflect.Method.invoke(Method.java:597) at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:78) at GradleStart.main(GradleStart.java:45) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Stacktrace: at net.minecraft.client.renderer.Tessellator.startDrawing(Tessellator.java:271) at net.minecraftforge.client.model.obj.WavefrontObject.renderAll(WavefrontObject.java:181) at assets.foliage.client.render.RealisticBlockRenderer.renderWorldBlock(RealisticBlockRenderer.java:48) at cpw.mods.fml.client.registry.RenderingRegistry.renderWorldBlock(RenderingRegistry.java:118) at net.minecraft.src.FMLRenderAccessLibrary.renderWorldBlock(FMLRenderAccessLibrary.java:53) at net.minecraft.client.renderer.RenderBlocks.renderBlockByRenderType(RenderBlocks.java:435) at net.minecraft.client.renderer.WorldRenderer.updateRenderer(WorldRenderer.java:207) at net.minecraft.client.renderer.RenderGlobal.updateRenderers(RenderGlobal.java:1647) at net.minecraft.client.renderer.EntityRenderer.renderWorld(EntityRenderer.java:1271) -- Affected level -- Details: Level name: MpServer All players: 1 total; [EntityClientPlayerMP['Player362'/164, l='MpServer', x=-323.33, y=67.62, z=-183.30]] Chunk stats: MultiplayerChunkCache: 45, 45 Level seed: 0 Level generator: ID 00 - default, ver 1. Features enabled: false Level generator options: Level spawn location: World: (-317,64,-172), Chunk: (at 3,4,4 in -20,-11; contains blocks -320,0,-176 to -305,255,-161), Region: (-1,-1; contains chunks -32,-32 to -1,-1, blocks -512,0,-512 to -1,255,-1) Level time: 284638 game time, 1200 day time Level dimension: 0 Level storage version: 0x00000 - Unknown? Level weather: Rain time: 0 (now: false), thunder time: 0 (now: false) Level game mode: Game mode: creative (ID 1). Hardcore: false. Cheats: false Forced entities: 64 total; [EntitySquid['Squid'/34, l='MpServer', x=-382.73, y=53.13, z=-217.52], EntitySkeleton['Skeleton'/35, l='MpServer', x=-378.50, y=17.00, z=-195.50], EntitySquid['Squid'/32, l='MpServer', x=-381.22, y=54.34, z=-227.69], EntitySquid['Squid'/33, l='MpServer', x=-376.16, y=56.28, z=-224.31], EntitySquid['Squid'/38, l='MpServer', x=-370.77, y=59.28, z=-205.13], EntityEnderman['Enderman'/39, l='MpServer', x=-379.31, y=20.00, z=-182.22], EntitySkeleton['Skeleton'/36, l='MpServer', x=-378.50, y=17.00, z=-192.50], EntitySkeleton['Skeleton'/37, l='MpServer', x=-378.50, y=17.00, z=-193.50], EntityZombie['Zombie'/42, l='MpServer', x=-381.84, y=13.00, z=-158.50], EntityZombie['Zombie'/43, l='MpServer', x=-375.47, y=16.00, z=-158.97], EntityCreeper['Creeper'/40, l='MpServer', x=-375.50, y=15.00, z=-160.50], EntityZombie['Zombie'/41, l='MpServer', x=-373.39, y=14.00, z=-162.39], EntitySquid['Squid'/46, l='MpServer', x=-367.34, y=56.00, z=-233.81], EntitySkeleton['Skeleton'/47, l='MpServer', x=-366.50, y=13.00, z=-165.50], EntitySkeleton['Skeleton'/44, l='MpServer', x=-369.50, y=15.00, z=-142.50], EntityClientPlayerMP['Player362'/164, l='MpServer', x=-323.33, y=67.62, z=-183.30], EntitySquid['Squid'/51, l='MpServer', x=-361.72, y=45.34, z=-154.47], EntitySquid['Squid'/50, l='MpServer', x=-354.44, y=54.06, z=-167.53], EntitySquid['Squid'/49, l='MpServer', x=-355.50, y=57.19, z=-160.94], EntityBat['Bat'/48, l='MpServer', x=-363.53, y=11.00, z=-170.09], EntityEnderman['Enderman'/55, l='MpServer', x=-353.34, y=17.00, z=-133.34], EntityZombie['Zombie'/54, l='MpServer', x=-362.44, y=18.00, z=-136.50], EntitySkeleton['Skeleton'/53, l='MpServer', x=-365.88, y=17.00, z=-137.50], EntitySkeleton['Skeleton'/52, l='MpServer', x=-361.50, y=18.00, z=-136.50], EntityEnderman['Enderman'/57, l='MpServer', x=-356.50, y=17.00, z=-134.50], EntityEnderman['Enderman'/56, l='MpServer', x=-357.50, y=17.00, z=-130.50], EntitySkeleton['Skeleton'/63, l='MpServer', x=-343.50, y=24.00, z=-192.50], EntitySquid['Squid'/62, l='MpServer', x=-342.03, y=53.38, z=-239.16], EntitySquid['Squid'/68, l='MpServer', x=-340.59, y=47.19, z=-169.19], EntityCreeper['Creeper'/69, l='MpServer', x=-351.50, y=17.00, z=-133.50], EntityCreeper['Creeper'/70, l='MpServer', x=-350.50, y=17.00, z=-132.50], EntityZombie['Zombie'/71, l='MpServer', x=-335.50, y=32.00, z=-201.50], EntitySquid['Squid'/64, l='MpServer', x=-346.75, y=53.19, z=-201.50], EntityBat['Bat'/65, l='MpServer', x=-340.71, y=24.06, z=-186.68], EntityBat['Bat'/66, l='MpServer', x=-339.25, y=23.00, z=-188.06], EntitySquid['Squid'/67, l='MpServer', x=-348.66, y=39.97, z=-191.03], EntitySkeleton['Skeleton'/78, l='MpServer', x=-313.15, y=21.00, z=-214.29], EntityBat['Bat'/79, l='MpServer', x=-317.25, y=44.10, z=-195.25], EntityBat['Bat'/72, l='MpServer', x=-326.13, y=32.00, z=-189.25], EntitySkeleton['Skeleton'/73, l='MpServer', x=-327.47, y=35.00, z=-190.09], EntitySkeleton['Skeleton'/74, l='MpServer', x=-327.00, y=35.00, z=-188.50], EntitySkeleton['Skeleton'/85, l='MpServer', x=-319.13, y=54.00, z=-195.50], EntityCreeper['Creeper'/84, l='MpServer', x=-318.00, y=50.00, z=-196.50], EntitySkeleton['Skeleton'/87, l='MpServer', x=-311.53, y=43.00, z=-188.69], EntitySkeleton['Skeleton'/86, l='MpServer', x=-311.50, y=43.00, z=-186.31], EntityZombie['Zombie'/81, l='MpServer', x=-305.69, y=43.00, z=-199.00], EntityBat['Bat'/80, l='MpServer', x=-316.25, y=42.10, z=-195.25], EntitySkeleton['Skeleton'/83, l='MpServer', x=-305.28, y=43.00, z=-196.00], EntityZombie['Zombie'/82, l='MpServer', x=-305.69, y=43.00, z=-197.00], EntityZombie['Zombie'/89, l='MpServer', x=-312.48, y=43.00, z=-188.70], EntityZombie['Zombie'/88, l='MpServer', x=-314.93, y=43.00, z=-188.70], EntitySkeleton['Skeleton'/102, l='MpServer', x=-295.03, y=14.00, z=-133.56], EntitySkeleton['Skeleton'/103, l='MpServer', x=-291.56, y=17.00, z=-140.06], EntityCreeper['Creeper'/100, l='MpServer', x=-296.63, y=49.00, z=-185.06], EntitySquid['Squid'/101, l='MpServer', x=-294.09, y=45.38, z=-158.94], EntityBat['Bat'/98, l='MpServer', x=-293.31, y=44.10, z=-184.13], EntitySkeleton['Skeleton'/99, l='MpServer', x=-303.50, y=43.00, z=-183.50], EntityZombie['Zombie'/96, l='MpServer', x=-303.50, y=43.00, z=-196.03], EntityBat['Bat'/97, l='MpServer', x=-297.94, y=44.10, z=-184.75], EntityZombie['Zombie'/110, l='MpServer', x=-283.50, y=12.00, z=-139.50], EntityCreeper['Creeper'/108, l='MpServer', x=-278.69, y=11.00, z=-131.56], EntityWitch['Witch'/109, l='MpServer', x=-279.50, y=12.00, z=-137.50], EntitySquid['Squid'/106, l='MpServer', x=-274.53, y=57.97, z=-215.47], EntitySkeleton['Skeleton'/107, l='MpServer', x=-276.34, y=12.00, z=-129.66]] Retry entities: 0 total; [] Server brand: fml,forge Server type: Integrated singleplayer server Stacktrace: at net.minecraft.client.multiplayer.WorldClient.addWorldInfoToCrashReport(WorldClient.java:417) at net.minecraft.client.Minecraft.addGraphicsAndWorldToCrashReport(Minecraft.java:2568) at net.minecraft.client.Minecraft.run(Minecraft.java:990) at net.minecraft.client.main.Main.main(Main.java:164) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25) at java.lang.reflect.Method.invoke(Method.java:597) at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:78) at GradleStart.main(GradleStart.java:45) -- System Details -- Details: Minecraft Version: 1.7.10 Operating System: Mac OS X (x86_64) version 10.10.1 Java Version: 1.6.0_65, Apple Inc. Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Apple Inc. Memory: 934749536 bytes (891 MB) / 1065025536 bytes (1015 MB) up to 1065025536 bytes (1015 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M AABB Pool Size: 0 (0 bytes; 0 MB) allocated, 0 (0 bytes; 0 MB) used IntCache: cache: 0, tcache: 0, allocated: 12, tallocated: 94 FML: MCP v9.05 FML v7.10.18.1180 Minecraft Forge 10.13.0.1180 4 mods loaded, 4 mods active mcp{9.05} [Minecraft Coder Pack] (minecraft.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available FML{7.10.18.1180} [Forge Mod Loader] (forgeSrc-1.7.10-10.13.0.1180.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available Forge{10.13.0.1180} [Minecraft Forge] (forgeSrc-1.7.10-10.13.0.1180.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available foliage{1.0} [Realistic Foliage] (bin) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available Launched Version: 1.7.10 LWJGL: 2.9.1 OpenGL: AMD Radeon HD 6970M OpenGL Engine GL version 2.1 ATI-1.28.29, ATI Technologies Inc. GL Caps: Using GL 1.3 multitexturing. Using framebuffer objects because ARB_framebuffer_object is supported and separate blending is supported. Anisotropic filtering is supported and maximum anisotropy is 16. Shaders are available because OpenGL 2.1 is supported. Is Modded: Definitely; Client brand changed to 'fml,forge' Type: Client (map_client.txt) Resource Packs: [] Current Language: English (US) Profiler Position: N/A (disabled) Vec3 Pool Size: 0 (0 bytes; 0 MB) allocated, 0 (0 bytes; 0 MB) used Anisotropic Filtering: Off (1) And here's my block renderer: package assets.foliage.client.render; import net.minecraft.block.Block; import net.minecraft.client.renderer.RenderBlocks; import net.minecraft.client.renderer.Tessellator; import net.minecraft.init.Blocks; import net.minecraft.util.ResourceLocation; import net.minecraft.world.IBlockAccess; import net.minecraftforge.client.model.AdvancedModelLoader; import net.minecraftforge.client.model.obj.GroupObject; import net.minecraftforge.client.model.obj.Vertex; import net.minecraftforge.client.model.obj.WavefrontObject; import org.lwjgl.opengl.GL11; import cpw.mods.fml.client.registry.ISimpleBlockRenderingHandler; public class RealisticBlockRenderer implements ISimpleBlockRenderingHandler { private WavefrontObject tallGrass; public RealisticBlockRenderer() { tallGrass = (WavefrontObject)AdvancedModelLoader.loadModel(new ResourceLocation("foliage:models/Bush1.obj")); } @Override public void renderInventoryBlock(Block block, int metadata, int modelId, RenderBlocks renderer) { } @Override public boolean renderWorldBlock(IBlockAccess world, int x, int y, int z, Block block, int modelId, RenderBlocks renderer) { Tessellator tessellator = Tessellator.instance; if(block == Blocks.tallgrass) { GL11.glPushMatrix(); GL11.glTranslatef(x, y, z); float scale = 0.5f; GL11.glScalef(scale, scale, scale); tallGrass.renderAll(); GL11.glPopMatrix(); return true; } return false; } @Override public boolean shouldRender3DInInventory(int modelId) { return false; } @Override public int getRenderId() { return Foliage.proxy.renderHandler; } } Thanks for your help, it is greatly appreciated! - Romejanic
-
Yes, I've used that but the problem is that: 1) It's range is too short, it needs to stretch on infinitely 2) It does not change to null if it moves out of range I'll have a look at the Minecraft class and see how it defines objectMouseOver. Thanks for your help. - Romejanic
-
Hey guys! In my gun mod, I have created crosshairs which expand to indicate the bullet spread (like Call of Duty), but I want them to turn red when you look at an entity, to show that you will hit them. I've done the colouring, but there's one problem. The raycasting solution i found on the internet doesn't count for entities. I tried to modify the code with bits from EntityThrowable to make it work, but I doesn't work. Here's the current code: public static MovingObjectPosition raycastBlocksAndEntities(EntityPlayer player, World world) { float f = 1.0F; float f1 = player.prevRotationPitch + (player.rotationPitch - player.prevRotationPitch) * f; float f2 = player.prevRotationYaw + (player.rotationYaw - player.prevRotationYaw) * f; double d = player.prevPosX + (player.posX - player.prevPosX) * (double)f; double d1 = (player.prevPosY + (player.posY - player.prevPosY) * (double)f + 1.6200000000000001D) - (double)player.yOffset; double d2 = player.prevPosZ + (player.posZ - player.prevPosZ) * (double)f; Vec3 vec3d = Vec3.createVectorHelper(d, d1, d2); float f3 = MathHelper.cos(-f2 * 0.01745329F - 3.141593F); float f4 = MathHelper.sin(-f2 * 0.01745329F - 3.141593F); float f5 = -MathHelper.cos(-f1 * 0.01745329F); float f6 = MathHelper.sin(-f1 * 0.01745329F); float f7 = f4 * f5; float f8 = f6; float f9 = f3 * f5; double d3 = 5000D; Vec3 vec3d1 = vec3d.addVector((double)f7 * d3, (double)f8 * d3, (double)f9 * d3); MovingObjectPosition movingobjectposition = world.rayTraceBlocks(vec3d, vec3d1, false); return movingobjectposition; } Here's my modified code (which doesn't work): public static MovingObjectPosition raycastBlocksAndEntities(EntityPlayer player, World world) { float f = 1.0F; float f1 = player.prevRotationPitch + (player.rotationPitch - player.prevRotationPitch) * f; float f2 = player.prevRotationYaw + (player.rotationYaw - player.prevRotationYaw) * f; double d = player.prevPosX + (player.posX - player.prevPosX) * (double)f; double d1 = (player.prevPosY + (player.posY - player.prevPosY) * (double)f + 1.6200000000000001D) - (double)player.yOffset; double d2 = player.prevPosZ + (player.posZ - player.prevPosZ) * (double)f; Vec3 vec3d = Vec3.createVectorHelper(d, d1, d2); float f3 = MathHelper.cos(-f2 * 0.01745329F - 3.141593F); float f4 = MathHelper.sin(-f2 * 0.01745329F - 3.141593F); float f5 = -MathHelper.cos(-f1 * 0.01745329F); float f6 = MathHelper.sin(-f1 * 0.01745329F); float f7 = f4 * f5; float f8 = f6; float f9 = f3 * f5; double d3 = 5000D; Vec3 vec3d1 = vec3d.addVector((double)f7 * d3, (double)f8 * d3, (double)f9 * d3); MovingObjectPosition movingobjectposition = world.rayTraceBlocks(vec3d, vec3d1, false); if(movingobjectposition != null) { Vec3 vec3 = Vec3.createVectorHelper(player.posX, player.posY, player.posZ); Vec3 vec31 = Vec3.createVectorHelper(movingobjectposition.hitVec.xCoord, movingobjectposition.hitVec.yCoord, movingobjectposition.hitVec.zCoord);; if (!player.worldObj.isRemote) { Entity entity = null; List list = player.worldObj.getEntitiesWithinAABBExcludingEntity(player, AxisAlignedBB.getBoundingBox(vec31.xCoord - 1d, vec31.yCoord - 1d, vec31.zCoord - 1d, vec31.xCoord + 1d, vec31.yCoord + 1d, vec31.zCoord + 1d)); double d0 = 0.3D; EntityLivingBase entitylivingbase = player; System.out.println(list); for (int j = 0; j < list.size(); ++j) { Entity entity1 = (Entity)list.get(j); if (entity1.canBeCollidedWith() && !entity1.isEntityEqual(entitylivingbase)) { if(entity1.getDistance(vec31.xCoord, vec31.yCoord, vec31.zCoord) <= d0) { entity = entity1; break; } } } if (entity != null) { return new MovingObjectPosition(entity); } } } return movingobjectposition; } Does anyone have any ideas? Thanks for your help. - Romejanic
-
Thank you so much! Works perfectly! Been looking for a solution to this for ages - Romejanic
-
[1.7.10] RenderPlayerEvent when rendering multiple players
Romejanic replied to Eternaldoom's topic in Modder Support
Who said you can't have capes? Add this line to the render code: if(event.entityPlayer instanceof AbstractClientPlayer) { ((AbstractClientPlayer) event.entityPlayer).func_152121_a(Type.CAPE, new ResourceLocation("yourmod:whatever/the/path/is.png")); } Cheers! - Romejanic -
[1.8] How to make a 3D model item <Not Solved>
Romejanic replied to many231's topic in Modder Support
What do you mean by 3D model item? Like a ItemRenderer (custom model on item in first person, third person, inventory and dropped entity)? - Romejanic -
Hey everyone. I am trying to make a vehicle. A car to be exact. It works pretty smoothly, but the only problem is that I need to get it hopping up blocks. They way I want it to work is that if you collide with a block, and there is a nonsolid/air block above it, it moves up onto the block. If there is however, a solid block above it, it will take crash damage. Any ideas? Here's my code so far: package assets.battlefield.common.entity.vechicle; import assets.battlefield.common.util.EntityUtil; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; import java.util.List; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.Blocks; import net.minecraft.init.Items; import net.minecraft.item.Item; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.util.AxisAlignedBB; import net.minecraft.util.DamageSource; import net.minecraft.util.MathHelper; import net.minecraft.world.World; public class EntityJeep extends Entity implements IBattlefieldVehicle { /** true if no player in boat */ private boolean isBoatEmpty; private double speedMultiplier; private int boatPosRotationIncrements; private double boatX; private double boatY; private double boatZ; private double boatYaw; private double boatPitch; @SideOnly(Side.CLIENT) private double velocityX; @SideOnly(Side.CLIENT) private double velocityY; @SideOnly(Side.CLIENT) private double velocityZ; private static final String __OBFID = "CL_00001667"; public EntityJeep(World p_i1704_1_) { super(p_i1704_1_); this.isBoatEmpty = true; this.speedMultiplier = 0.07D; this.preventEntitySpawning = true; this.setSize(1.5F, 0.6F); this.yOffset = this.height / 2.0F; } /** * returns if this entity triggers Block.onEntityWalking on the blocks they walk on. used for spiders and wolves to * prevent them from trampling crops */ protected boolean canTriggerWalking() { return false; } protected void entityInit() { this.dataWatcher.addObject(17, new Integer(0)); this.dataWatcher.addObject(18, new Integer(1)); this.dataWatcher.addObject(19, new Float(0.0F)); } /** * Returns a boundingBox used to collide the entity with other entities and blocks. This enables the entity to be * pushable on contact, like boats or minecarts. */ public AxisAlignedBB getCollisionBox(Entity p_70114_1_) { return p_70114_1_.boundingBox; } /** * returns the bounding box for this entity */ public AxisAlignedBB getBoundingBox() { return this.boundingBox; } /** * Returns true if this entity should push and be pushed by other entities when colliding. */ public boolean canBePushed() { return true; } public EntityJeep(World p_i1705_1_, double p_i1705_2_, double p_i1705_4_, double p_i1705_6_) { this(p_i1705_1_); this.setPosition(p_i1705_2_, p_i1705_4_ + (double)this.yOffset, p_i1705_6_); this.motionX = 0.0D; this.motionY = 0.0D; this.motionZ = 0.0D; this.prevPosX = p_i1705_2_; this.prevPosY = p_i1705_4_; this.prevPosZ = p_i1705_6_; } /** * Returns the Y offset from the entity's position for any entity riding this one. */ public double getMountedYOffset() { return (double)this.height * 0.0D - 0.30000001192092896D + 0.6d; } /** * Called when the entity is attacked. */ public boolean attackEntityFrom(DamageSource p_70097_1_, float p_70097_2_) { if (this.isEntityInvulnerable()) { return false; } else if (!this.worldObj.isRemote && !this.isDead) { this.setForwardDirection(-this.getForwardDirection()); this.setTimeSinceHit(10); this.setDamageTaken(this.getDamageTaken() + p_70097_2_ * 10.0F); this.setBeenAttacked(); boolean flag = p_70097_1_.getEntity() instanceof EntityPlayer && ((EntityPlayer)p_70097_1_.getEntity()).capabilities.isCreativeMode; if (flag || this.getDamageTaken() > 40.0F) { if (this.riddenByEntity != null) { this.riddenByEntity.mountEntity(this); } if (!flag) { this.func_145778_a(Items.boat, 1, 0.0F); } this.setDead(); } return true; } else { return true; } } /** * Setups the entity to do the hurt animation. Only used by packets in multiplayer. */ @SideOnly(Side.CLIENT) public void performHurtAnimation() { this.setForwardDirection(-this.getForwardDirection()); this.setTimeSinceHit(10); this.setDamageTaken(this.getDamageTaken() * 11.0F); } /** * Returns true if other Entities should be prevented from moving through this Entity. */ public boolean canBeCollidedWith() { return !this.isDead; } /** * Sets the position and rotation. Only difference from the other one is no bounding on the rotation. Args: posX, * posY, posZ, yaw, pitch */ @SideOnly(Side.CLIENT) public void setPositionAndRotation2(double p_70056_1_, double p_70056_3_, double p_70056_5_, float p_70056_7_, float p_70056_8_, int p_70056_9_) { if (this.isBoatEmpty) { this.boatPosRotationIncrements = p_70056_9_ + 5; } else { double d3 = p_70056_1_ - this.posX; double d4 = p_70056_3_ - this.posY; double d5 = p_70056_5_ - this.posZ; double d6 = d3 * d3 + d4 * d4 + d5 * d5; if (d6 <= 1.0D) { return; } this.boatPosRotationIncrements = 3; } this.boatX = p_70056_1_; this.boatY = p_70056_3_; this.boatZ = p_70056_5_; this.boatYaw = (double)p_70056_7_; this.boatPitch = (double)p_70056_8_; this.motionX = this.velocityX; this.motionY = this.velocityY; this.motionZ = this.velocityZ; } /** * Sets the velocity to the args. Args: x, y, z */ @SideOnly(Side.CLIENT) public void setVelocity(double p_70016_1_, double p_70016_3_, double p_70016_5_) { this.velocityX = this.motionX = p_70016_1_; this.velocityY = this.motionY = p_70016_3_; this.velocityZ = this.motionZ = p_70016_5_; } /** * Called to update the entity's position/logic. */ public void onUpdate() { super.onUpdate(); this.setSize(3f, 2f); if (this.getTimeSinceHit() > 0) { this.setTimeSinceHit(this.getTimeSinceHit() - 1); } if (this.getDamageTaken() > 0.0F) { this.setDamageTaken(this.getDamageTaken() - 1.0F); } this.prevPosX = this.posX; this.prevPosY = this.posY; this.prevPosZ = this.posZ; byte b0 = 5; double d0 = 0.0D; double d10 = Math.sqrt(this.motionX * this.motionX + this.motionZ * this.motionZ); double d2; double d4; int j; if (d10 > 0.26249999999999996D) { d2 = Math.cos((double)this.rotationYaw * Math.PI / 180.0D); d4 = Math.sin((double)this.rotationYaw * Math.PI / 180.0D); for (j = 0; (double)j < 1.0D + d10 * 60.0D; ++j) { double d5 = (double)(this.rand.nextFloat() * 2.0F - 1.0F); double d6 = (double)(this.rand.nextInt(2) * 2 - 1) * 0.7D; double d8; double d9; Block block = EntityUtil.findBlockUnderEntity(this); int meta = EntityUtil.findBlockDataUnderEntity(this); String particle = "blockcrack_" + Block.getIdFromBlock(block) + "_" + meta; if (this.rand.nextBoolean()) { d8 = this.posX - d2 * d5 * 0.8D + d4 * d6; d9 = this.posZ - d4 * d5 * 0.8D - d2 * d6; this.worldObj.spawnParticle(particle, d8, this.posY - 0.125D, d9, this.motionX, this.motionY, this.motionZ); } else { d8 = this.posX + d2 + d4 * d5 * 0.7D; d9 = this.posZ + d4 - d2 * d5 * 0.7D; this.worldObj.spawnParticle(particle, d8, this.posY - 0.125D, d9, this.motionX, this.motionY, this.motionZ); } } } double d11; double d12; if (this.worldObj.isRemote && this.isBoatEmpty) { if (this.boatPosRotationIncrements > 0) { d2 = this.posX + (this.boatX - this.posX) / (double)this.boatPosRotationIncrements; d4 = this.posY + (this.boatY - this.posY) / (double)this.boatPosRotationIncrements; d11 = this.posZ + (this.boatZ - this.posZ) / (double)this.boatPosRotationIncrements; d12 = MathHelper.wrapAngleTo180_double(this.boatYaw - (double)this.rotationYaw); this.rotationYaw = (float)((double)this.rotationYaw + d12 / (double)this.boatPosRotationIncrements); this.rotationPitch = (float)((double)this.rotationPitch + (this.boatPitch - (double)this.rotationPitch) / (double)this.boatPosRotationIncrements); --this.boatPosRotationIncrements; this.setPosition(d2, d4, d11); this.setRotation(this.rotationYaw, this.rotationPitch); } else { d2 = this.posX + this.motionX; d4 = this.posY + this.motionY; d11 = this.posZ + this.motionZ; this.setPosition(d2, d4, d11); if (this.onGround) { this.motionX *= 0.5D; this.motionY *= 0.5D; this.motionZ *= 0.5D; } this.motionX *= 0.9900000095367432D; this.motionY *= 0.949999988079071D; this.motionZ *= 0.9900000095367432D; } } else { if (d0 < 1.0D) { d2 = d0 * 2.0D - 1.0D; this.motionY += 0.03999999910593033D * d2; } else { if (this.motionY < 0.0D) { this.motionY /= 2.0D; } this.motionY += 0.007000000216066837D; } if (this.riddenByEntity != null && this.riddenByEntity instanceof EntityLivingBase) { EntityLivingBase entitylivingbase = (EntityLivingBase)this.riddenByEntity; float f = this.riddenByEntity.rotationYaw + -entitylivingbase.moveStrafing * 90.0F; this.motionX += -Math.sin((double)(f * (float)Math.PI / 180.0F)) * this.speedMultiplier * (double)entitylivingbase.moveForward * 0.05000000074505806D; this.motionZ += Math.cos((double)(f * (float)Math.PI / 180.0F)) * this.speedMultiplier * (double)entitylivingbase.moveForward * 0.05000000074505806D; } d2 = Math.sqrt(this.motionX * this.motionX + this.motionZ * this.motionZ); if (d2 > 0.35D) { d4 = 0.35D / d2; this.motionX *= d4; this.motionZ *= d4; d2 = 0.35D; } if (d2 > d10 && this.speedMultiplier < 0.35D) { this.speedMultiplier += (0.35D - this.speedMultiplier) / 35.0D; if (this.speedMultiplier > 0.35D) { this.speedMultiplier = 0.35D; } } else { this.speedMultiplier -= (this.speedMultiplier - 0.07D) / 35.0D; if (this.speedMultiplier < 0.07D) { this.speedMultiplier = 0.07D; } } int l; for (l = 0; l < 4; ++l) { int i1 = MathHelper.floor_double(this.posX + ((double)(l % 2) - 0.5D) * 0.8D); j = MathHelper.floor_double(this.posZ + ((double)(l / 2) - 0.5D) * 0.8D); for (int j1 = 0; j1 < 2; ++j1) { int k = MathHelper.floor_double(this.posY) + j1; Block block = this.worldObj.getBlock(i1, k, j); if (block == Blocks.snow_layer) { this.worldObj.setBlockToAir(i1, k, j); this.isCollidedHorizontally = false; } else if (block == Blocks.waterlily) { this.worldObj.func_147480_a(i1, k, j, true); this.isCollidedHorizontally = false; } } } if (this.onGround) { this.motionX *= 1D; this.motionY *= 1D; this.motionZ *= 1D; } this.moveEntity(this.motionX, this.motionY, this.motionZ); if (this.isCollidedHorizontally && d10 > 0.2D) { } else { this.motionX *= 0.9900000095367432D; this.motionY *= 0.949999988079071D; this.motionZ *= 0.9900000095367432D; } this.rotationPitch = 0.0F; d4 = (double)this.rotationYaw; d11 = this.prevPosX - this.posX; d12 = this.prevPosZ - this.posZ; if (d11 * d11 + d12 * d12 > 0.001D) { d4 = (double)((float)(Math.atan2(d12, d11) * 180.0D / Math.PI)); } double d7 = MathHelper.wrapAngleTo180_double(d4 - (double)this.rotationYaw); if (d7 > 20.0D) { d7 = 20.0D; } if (d7 < -20.0D) { d7 = -20.0D; } this.rotationYaw = (float)((double)this.rotationYaw + d7); this.setRotation(this.rotationYaw, this.rotationPitch); if (!this.worldObj.isRemote) { List list = this.worldObj.getEntitiesWithinAABBExcludingEntity(this, this.boundingBox.expand(0.20000000298023224D, 0.0D, 0.20000000298023224D)); if (list != null && !list.isEmpty()) { for (int k1 = 0; k1 < list.size(); ++k1) { Entity entity = (Entity)list.get(k1); if (entity != this.riddenByEntity && entity.canBePushed() && entity instanceof EntityJeep) { entity.applyEntityCollision(this); } } } if (this.riddenByEntity != null && this.riddenByEntity.isDead) { this.riddenByEntity = null; } } } } public void updateRiderPosition() { if (this.riddenByEntity != null) { double d0 = Math.cos((double)this.rotationYaw * Math.PI / 180.0D) * 0.1D; double d1 = Math.sin((double)this.rotationYaw * Math.PI / 180.0D) * -0.6D; this.riddenByEntity.setPosition(this.posX + d0, this.posY + this.getMountedYOffset() + this.riddenByEntity.getYOffset(), this.posZ + d1); } } /** * (abstract) Protected helper method to write subclass entity data to NBT. */ protected void writeEntityToNBT(NBTTagCompound p_70014_1_) {} /** * (abstract) Protected helper method to read subclass entity data from NBT. */ protected void readEntityFromNBT(NBTTagCompound p_70037_1_) {} @SideOnly(Side.CLIENT) public float getShadowSize() { return 0.0F; } /** * First layer of player interaction */ public boolean interactFirst(EntityPlayer p_130002_1_) { if (this.riddenByEntity != null && this.riddenByEntity instanceof EntityPlayer && this.riddenByEntity != p_130002_1_) { return true; } else { if (!this.worldObj.isRemote) { p_130002_1_.mountEntity(this); } return true; } } /** * Takes in the distance the entity has fallen this tick and whether its on the ground to update the fall distance * and deal fall damage if landing on the ground. Args: distanceFallenThisTick, onGround */ protected void updateFallState(double p_70064_1_, boolean p_70064_3_) { int i = MathHelper.floor_double(this.posX); int j = MathHelper.floor_double(this.posY); int k = MathHelper.floor_double(this.posZ); if (p_70064_3_) { if (this.fallDistance > 3.0F) { this.fall(this.fallDistance); if (!this.worldObj.isRemote && !this.isDead) { this.setDead(); int l; for (l = 0; l < 3; ++l) { this.func_145778_a(Item.getItemFromBlock(Blocks.planks), 1, 0.0F); } for (l = 0; l < 2; ++l) { this.func_145778_a(Items.stick, 1, 0.0F); } } this.fallDistance = 0.0F; } } else if (this.worldObj.getBlock(i, j - 1, k).getMaterial() != Material.water && p_70064_1_ < 0.0D) { this.fallDistance = (float)((double)this.fallDistance - p_70064_1_); } } /** * Sets the damage taken from the last hit. */ public void setDamageTaken(float p_70266_1_) { this.dataWatcher.updateObject(19, Float.valueOf(p_70266_1_)); } /** * Gets the damage taken from the last hit. */ public float getDamageTaken() { return this.dataWatcher.getWatchableObjectFloat(19); } /** * Sets the time to count down from since the last time entity was hit. */ public void setTimeSinceHit(int p_70265_1_) { this.dataWatcher.updateObject(17, Integer.valueOf(p_70265_1_)); } /** * Gets the time since the last hit. */ public int getTimeSinceHit() { return this.dataWatcher.getWatchableObjectInt(17); } /** * Sets the forward direction of the entity. */ public void setForwardDirection(int p_70269_1_) { this.dataWatcher.updateObject(18, Integer.valueOf(p_70269_1_)); } /** * Gets the forward direction of the entity. */ public int getForwardDirection() { return this.dataWatcher.getWatchableObjectInt(18); } /** * true if no player in boat */ @SideOnly(Side.CLIENT) public void setIsBoatEmpty(boolean p_70270_1_) { this.isBoatEmpty = p_70270_1_; } @Override public Entity[] getRiders() { return new Entity[] { this.riddenByEntity }; } @Override public boolean isRiderDriver(int index) { return this.riddenByEntity.isEntityEqual(getRiders()[index]); } @Override public boolean isRiderGunner(int index) { return isRiderDriver(index); } } Any help is greatly appreciated! Thanks, - Romejanic
-
Instead of calling the setVelocity method (which is client-side only), change the motionX/motionY/motionZ directly. Example: player.motionX += 0d; player.motionY += 1d; player.motionZ += 0d; Hope this helps! - Romejanic
-
Item and block textures are icons, because they are stitched onto a spritesheet at startup, reducing the required memory for texture storage. The Tessellator class uses U and V coordinates to render a texture. The U and V usually translate to the X and Y coordinates of the item texture on the spritesheet. If you are rendering a texture directly with Minecraft.renderEngine.bind(ResourceLocation), the U and V is usually 0 and 1, with 0 being, well 0, and 1 being the width/height of the texture. So the item's U and V is usually between those 2 numbers. If you are using ItemRenderer, you don't need to worry about these methods. You only need to know about them if you are using the Tessellator directly. It's the same as the glTexCoords2() in OpenGL (which Minecraft uses for rendering)
-
Haha sorry, I posted that when I was still using 1.6.4 Just use the item without a constructor. That int was the old itemID, which doesn't exist in 1.7
-
[1.7.10][Fixed] Vehicle bouncing up and down
Romejanic replied to Romejanic's topic in Modder Support
Basically me and my friend copied the code back over from the boat and we were very picky about what code to add and remove. It seems pretty stable now. Thanks anyway, just need help getting it move up blocks. Any ideas? -
Hey everyone! I'm trying to make a vehicle. I've got it moving and the controls work, but it keeps jolting up and down. Sometimes it will stop, before promptly teleporting back to it's original position and starting again. Does anyone have any ideas? This is the first time I've made a vehicle, so i'm not an expert, but i can't find anything causing it. I've tried adding code to stop it, but still nothing. I used code from EntityBoat, and removed some parts I didn't need. package assets.battlefield.common.entity.vechicle; import java.util.List; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.item.EntityBoat; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.Blocks; import net.minecraft.init.Items; import net.minecraft.item.Item; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.util.AxisAlignedBB; import net.minecraft.util.MathHelper; import net.minecraft.world.World; public class EntityTank extends Entity { private double speedMultiplier = 4d; public EntityTank(World p_i1582_1_) { super(p_i1582_1_); this.setSize(1.5F, 0.6F); this.yOffset = this.height / 2.0F; this.preventEntitySpawning = true; } public AxisAlignedBB getCollisionBox(Entity p_70114_1_) { return p_70114_1_.boundingBox; } public AxisAlignedBB getBoundingBox() { return this.boundingBox; } @Override public boolean canBeCollidedWith() { return true; } public void onUpdate() { super.onUpdate(); this.prevPosX = this.posX; this.prevPosY = this.posY; this.prevPosZ = this.posZ; byte b0 = 5; double d0 = 1d / b0; double d10 = Math.sqrt(this.motionX * this.motionX + this.motionZ * this.motionZ); double d2; double d4; int j; if(!this.onGround) { this.motionX *= 0.1d; this.motionZ *= 0.1d; if(this.motionY > -9d) { this.motionY -= 0.9d; } } if (d10 > 0.26249999999999996D) { d2 = Math.cos((double)this.rotationYaw * Math.PI / 180.0D); d4 = Math.sin((double)this.rotationYaw * Math.PI / 180.0D); for (j = 0; (double)j < 1.0D + d10 * 60.0D; ++j) { double d5 = (double)(this.rand.nextFloat() * 2.0F - 1.0F); double d6 = (double)(this.rand.nextInt(2) * 2 - 1) * 0.7D; double d8; double d9; int id = Block.getIdFromBlock(worldObj.getBlock((int)posX, (int)posY - 1, (int)posZ)); int meta = worldObj.getBlockMetadata((int)posX, (int)posY - 1, (int)posZ); if (this.rand.nextBoolean()) { d8 = this.posX - d2 * d5 * 0.8D + d4 * d6; d9 = this.posZ - d4 * d5 * 0.8D - d2 * d6; this.worldObj.spawnParticle("blockcrack_" + id + "_" + meta, d8, this.posY - 0.125D, d9, this.motionX, this.motionY, this.motionZ); } else { d8 = this.posX + d2 + d4 * d5 * 0.7D; d9 = this.posZ + d4 - d2 * d5 * 0.7D; this.worldObj.spawnParticle("blockcrack_" + id + "_" + meta, d8, this.posY - 0.125D, d9, this.motionX, this.motionY, this.motionZ); } } } double d11; double d12; if (this.motionY < 0.0D) { this.motionY /= 2.0D; } if (this.riddenByEntity != null && this.riddenByEntity instanceof EntityLivingBase) { EntityLivingBase entitylivingbase = (EntityLivingBase)this.riddenByEntity; float f = this.riddenByEntity.rotationYaw + -entitylivingbase.moveStrafing * 90.0F; this.motionX += -Math.sin((double)(f * (float)Math.PI / 180.0F)) * this.speedMultiplier * (double)entitylivingbase.moveForward * 0.05000000074505806D; this.motionZ += Math.cos((double)(f * (float)Math.PI / 180.0F)) * this.speedMultiplier * (double)entitylivingbase.moveForward * 0.05000000074505806D; } d2 = Math.sqrt(this.motionX * this.motionX + this.motionZ * this.motionZ); if (d2 > 0.35D) { d4 = 0.35D / d2; this.motionX *= d4; this.motionZ *= d4; d2 = 0.35D; } int l; for (l = 0; l < 4; ++l) { int i1 = MathHelper.floor_double(this.posX + ((double)(l % 2) - 0.5D) * 0.8D); j = MathHelper.floor_double(this.posZ + ((double)(l / 2) - 0.5D) * 0.8D); for (int j1 = 0; j1 < 2; ++j1) { int k = MathHelper.floor_double(this.posY) + j1; Block block = this.worldObj.getBlock(i1, k, j); if (block == Blocks.snow_layer) { this.worldObj.setBlockToAir(i1, k, j); this.isCollidedHorizontally = false; } else if (block == Blocks.waterlily) { this.worldObj.func_147480_a(i1, k, j, true); this.isCollidedHorizontally = false; } } } if (this.onGround) { this.motionX *= 0.5D; this.motionY *= 0.5D; this.motionZ *= 0.5D; } this.moveEntity(this.motionX, this.motionY, this.motionZ); this.motionX *= 0.9900000095367432D; this.motionY *= 0.949999988079071D; this.motionZ *= 0.9900000095367432D; this.rotationPitch = 0.0F; d4 = (double)this.rotationYaw; d11 = this.prevPosX - this.posX; d12 = this.prevPosZ - this.posZ; if (d11 * d11 + d12 * d12 > 0.001D) { d4 = (double)((float)(Math.atan2(d12, d11) * 180.0D / Math.PI)); } double d7 = MathHelper.wrapAngleTo180_double(d4 - (double)this.rotationYaw); if (d7 > 20.0D) { d7 = 20.0D; } if (d7 < -20.0D) { d7 = -20.0D; } this.rotationYaw = (float)((double)this.rotationYaw + d7); this.setRotation(this.rotationYaw, this.rotationPitch); if (!this.worldObj.isRemote) { List list = this.worldObj.getEntitiesWithinAABBExcludingEntity(this, this.boundingBox.expand(0.20000000298023224D, 0.0D, 0.20000000298023224D)); if (list != null && !list.isEmpty()) { for (int k1 = 0; k1 < list.size(); ++k1) { Entity entity = (Entity)list.get(k1); if (entity != this.riddenByEntity && entity.canBePushed() && entity instanceof EntityBoat) { entity.applyEntityCollision(this); } } } if (this.riddenByEntity != null && this.riddenByEntity.isDead) { this.riddenByEntity = null; } } if(this.onGround) { this.motionY = 0d; } } public boolean interactFirst(EntityPlayer player) { if (this.riddenByEntity != null && this.riddenByEntity instanceof EntityPlayer && this.riddenByEntity != player) { return true; } else { if (!this.worldObj.isRemote) { player.mountEntity(this); } return true; } } public void updateRiderPosition() { if (this.riddenByEntity != null) { double d0 = Math.cos((double)this.rotationYaw * Math.PI / 180.0D) * 0.4D; double d1 = Math.sin((double)this.rotationYaw * Math.PI / 180.0D) * 0.4D; this.riddenByEntity.setPosition(this.posX + d0, this.posY + this.getMountedYOffset() + this.riddenByEntity.getYOffset(), this.posZ + d1); } } @Override protected void entityInit() {} @Override protected void readEntityFromNBT(NBTTagCompound p_70037_1_) { // TODO Auto-generated method stub } @Override protected void writeEntityToNBT(NBTTagCompound p_70014_1_) { // TODO Auto-generated method stub } } Thanks, Romejanic.
-
How to use SimpleNetworkWrapper, IMessage and IMessageHandler
Romejanic replied to Ablaze's topic in User Submitted Tutorials
You can also use the ByteBufUtils class which has some amazing stuff, like ItemStacks and NBT Compounds! -
Here is an example item with the code: package test; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.util.ChunkCoordinates; import net.minecraft.world.World; public class ItemTest extends Item { public ItemTest(int par1) { super(par1); } /** * This is called when the player right-clicks with the item in hand * This is triggered anytime the player right-clicks */ public ItemStack onItemRightClick(ItemStack par1ItemStack, World par2World, EntityPlayer par3EntityPlayer) { ChunkCoordinates spawn = par3EntityPlayer.getBedLocation(); if(spawn == null) { spawn = par3EntityPlayer.worldObj.getSpawnPoint(); } par3EntityPlayer.setPosition(spawn.posX, spawn.posY, spawn.posZ); return par1ItemStack; } /** * This is called when the player right-clicks with the item in hand * This is triggered only when a block is right-clicked */ public boolean onItemUse(ItemStack par1ItemStack, EntityPlayer par2EntityPlayer, World par3World, int par4, int par5, int par6, int par7, float par8, float par9, float par10) { ChunkCoordinates spawn = par2EntityPlayer.getBedLocation(); if(spawn == null) { spawn = par2EntityPlayer.worldObj.getSpawnPoint(); } par2EntityPlayer.setPosition(spawn.posX, spawn.posY, spawn.posZ); return true; } }
-
Using the command would disrupt the gameplay (if they want to use that command) and it would be a little inconvenient. Instead, you can use this: ChunkCoordinates spawn = player.getBedLocation(); if(spawn == null) { spawn = player.worldObj.getSpawnPoint(); } player.setPosition(spawn.posX, spawn.posY, spawn.posZ); It will check if the player has slept in a bed. If they have, teleport them to the bed. Otherwise, teleport the to the world spawn.
-
Hey everyone! I am adding custom achievements to a mod. I have created the achievements and they are unlocking. But there is a small problem... First of all, the game starts up perfectly. If I unlock the achievement and restart the game, it crashes on startup. 2014-04-23 19:10:41 [iNFO] [sTDOUT] ---- Minecraft Crash Report ---- 2014-04-23 19:10:41 [iNFO] [sTDOUT] // Hi. I'm Minecraft, and I'm a crashaholic. 2014-04-23 19:10:41 [iNFO] [sTDOUT] 2014-04-23 19:10:41 [iNFO] [sTDOUT] Time: 4/23/14 7:10 PM 2014-04-23 19:10:41 [iNFO] [sTDOUT] Description: Initializing game 2014-04-23 19:10:41 [iNFO] [sTDOUT] 2014-04-23 19:10:41 [iNFO] [sTDOUT] java.lang.RuntimeException: Duplicate stat id: "Unknown stat" and "achievement.exchicken.buildLauncher" at id 5244883 2014-04-23 19:10:41 [iNFO] [sTDOUT] at net.minecraft.stats.StatBase.registerStat(StatBase.java:58) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at net.minecraft.stats.Achievement.registerAchievement(Achievement.java:116) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at assets.exchicken.common.ExplosiveChickens.init(ExplosiveChickens.java:128) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at java.lang.reflect.Method.invoke(Method.java:597) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at cpw.mods.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:545) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at java.lang.reflect.Method.invoke(Method.java:597) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.post(EventBus.java:267) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at cpw.mods.fml.common.LoadController.sendEventToModContainer(LoadController.java:201) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:181) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at java.lang.reflect.Method.invoke(Method.java:597) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.post(EventBus.java:267) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:112) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at cpw.mods.fml.common.Loader.initializeMods(Loader.java:699) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at cpw.mods.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:249) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at net.minecraft.client.Minecraft.startGame(Minecraft.java:509) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at net.minecraft.client.Minecraft.run(Minecraft.java:808) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at net.minecraft.client.main.Main.main(Main.java:93) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at java.lang.reflect.Method.invoke(Method.java:597) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at net.minecraft.launchwrapper.Launch.launch(Launch.java:131) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at net.minecraft.launchwrapper.Launch.main(Launch.java:27) 2014-04-23 19:10:41 [iNFO] [sTDOUT] 2014-04-23 19:10:41 [iNFO] [sTDOUT] 2014-04-23 19:10:41 [iNFO] [sTDOUT] A detailed walkthrough of the error, its code path and all known details is as follows: 2014-04-23 19:10:41 [iNFO] [sTDOUT] --------------------------------------------------------------------------------------- 2014-04-23 19:10:41 [iNFO] [sTDOUT] 2014-04-23 19:10:41 [iNFO] [sTDOUT] -- Head -- 2014-04-23 19:10:41 [iNFO] [sTDOUT] Stacktrace: 2014-04-23 19:10:41 [iNFO] [sTDOUT] at net.minecraft.stats.StatBase.registerStat(StatBase.java:58) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at net.minecraft.stats.Achievement.registerAchievement(Achievement.java:116) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at assets.exchicken.common.ExplosiveChickens.init(ExplosiveChickens.java:128) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at java.lang.reflect.Method.invoke(Method.java:597) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at cpw.mods.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:545) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at java.lang.reflect.Method.invoke(Method.java:597) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.post(EventBus.java:267) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at cpw.mods.fml.common.LoadController.sendEventToModContainer(LoadController.java:201) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:181) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at java.lang.reflect.Method.invoke(Method.java:597) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.post(EventBus.java:267) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:112) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at cpw.mods.fml.common.Loader.initializeMods(Loader.java:699) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at cpw.mods.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:249) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at net.minecraft.client.Minecraft.startGame(Minecraft.java:509) 2014-04-23 19:10:41 [iNFO] [sTDOUT] 2014-04-23 19:10:41 [iNFO] [sTDOUT] -- Initialization -- 2014-04-23 19:10:41 [iNFO] [sTDOUT] Details: 2014-04-23 19:10:41 [iNFO] [sTDOUT] Stacktrace: 2014-04-23 19:10:41 [iNFO] [sTDOUT] at net.minecraft.client.Minecraft.run(Minecraft.java:808) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at net.minecraft.client.main.Main.main(Main.java:93) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at java.lang.reflect.Method.invoke(Method.java:597) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at net.minecraft.launchwrapper.Launch.launch(Launch.java:131) 2014-04-23 19:10:41 [iNFO] [sTDOUT] at net.minecraft.launchwrapper.Launch.main(Launch.java:27) 2014-04-23 19:10:41 [iNFO] [sTDOUT] 2014-04-23 19:10:41 [iNFO] [sTDOUT] -- System Details -- 2014-04-23 19:10:41 [iNFO] [sTDOUT] Details: 2014-04-23 19:10:41 [iNFO] [sTDOUT] Minecraft Version: 1.6.4 2014-04-23 19:10:41 [iNFO] [sTDOUT] Operating System: Mac OS X (x86_64) version 10.9.2 2014-04-23 19:10:41 [iNFO] [sTDOUT] Java Version: 1.6.0_65, Apple Inc. 2014-04-23 19:10:41 [iNFO] [sTDOUT] Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Apple Inc. 2014-04-23 19:10:41 [iNFO] [sTDOUT] Memory: 980354536 bytes (934 MB) / 1065025536 bytes (1015 MB) up to 1065025536 bytes (1015 MB) 2014-04-23 19:10:41 [iNFO] [sTDOUT] JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M 2014-04-23 19:10:41 [iNFO] [sTDOUT] AABB Pool Size: 0 (0 bytes; 0 MB) allocated, 0 (0 bytes; 0 MB) used 2014-04-23 19:10:41 [iNFO] [sTDOUT] Suspicious classes: FML and Forge are installed 2014-04-23 19:10:41 [iNFO] [sTDOUT] IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 2014-04-23 19:10:41 [iNFO] [sTDOUT] FML: MCP v8.11 FML v6.4.45.953 Minecraft Forge 9.11.1.953 4 mods loaded, 4 mods active 2014-04-23 19:10:41 [iNFO] [sTDOUT] mcp{8.09} [Minecraft Coder Pack] (minecraft.jar) Unloaded->Constructed->Pre-initialized->Initialized 2014-04-23 19:10:41 [iNFO] [sTDOUT] FML{6.4.45.953} [Forge Mod Loader] (bin) Unloaded->Constructed->Pre-initialized->Initialized 2014-04-23 19:10:41 [iNFO] [sTDOUT] Forge{9.11.1.953} [Minecraft Forge] (bin) Unloaded->Constructed->Pre-initialized->Initialized 2014-04-23 19:10:41 [iNFO] [sTDOUT] Romejanic.ExplosiveChickens{1.6} [Explosive Chickens] (bin) Unloaded->Constructed->Pre-initialized->Errored 2014-04-23 19:10:41 [iNFO] [sTDOUT] Launched Version: 1.6 2014-04-23 19:10:41 [iNFO] [sTDOUT] LWJGL: 2.9.0 2014-04-23 19:10:41 [iNFO] [sTDOUT] OpenGL: AMD Radeon HD 6970M OpenGL Engine GL version 2.1 ATI-1.20.11, ATI Technologies Inc. 2014-04-23 19:10:41 [iNFO] [sTDOUT] Is Modded: Definitely; Client brand changed to 'fml,forge' 2014-04-23 19:10:41 [iNFO] [sTDOUT] Type: Client (map_client.txt) 2014-04-23 19:10:41 [iNFO] [sTDOUT] Resource Pack: Default 2014-04-23 19:10:41 [iNFO] [sTDOUT] Current Language: English (US) 2014-04-23 19:10:41 [iNFO] [sTDOUT] Profiler Position: N/A (disabled) 2014-04-23 19:10:41 [iNFO] [sTDOUT] Vec3 Pool Size: ~~ERROR~~ NullPointerException: null 2014-04-23 19:10:41 [iNFO] [sTDOUT] #@!@# Game crashed! Crash report saved to: #@!@# /Users/jammer365/Desktop/Explosive Chickens/workspaces/1.6.4/mcp/jars/./crash-reports/crash-2014-04-23_19.10.41-client.txt Here's my config file with the IDs package assets.exchicken.common.core; import java.io.File; import cpw.mods.fml.common.FMLCommonHandler; import cpw.mods.fml.relauncher.Side; import net.minecraftforge.common.Configuration; public class ConfigHandler { public int entity1ID; public int entity2ID; public int entity3ID; public int trickEggID; public int launcherID; public int trickChickenRawID; public int trickChickenCookedID; public int eatChickenID; public int throwEggID; public int buildLauncherID; public int directHitID; public boolean useAdvancedSkin = true; public boolean useLauncherModel = true; public boolean allowChainReactions = true; public ConfigHandler(File targetFile) { final String itemIDs = "Item IDs"; final String achievementIDs = "Achievement IDs"; final String entityIDs = "Entity IDs"; final String set = "Mod Settings"; Side side = FMLCommonHandler.instance().getEffectiveSide(); Configuration config = new Configuration(targetFile); config.load(); config.addCustomCategoryComment(entityIDs, "The IDs for mobs and other entities in the mod"); config.addCustomCategoryComment(set, "Certain user-defined controls of how the mod operates"); config.addCustomCategoryComment(itemIDs, "The IDs for items in the mod"); config.addCustomCategoryComment(achievementIDs, "The in-code IDs for every echievement in the mod"); entity1ID = config.get(entityIDs, "Explosive Chicken Mob", 101).getInt(); entity2ID = config.get(entityIDs, "Launched Explosive Chicken Entity", 102).getInt(); entity3ID = config.get(entityIDs, "Explosive Chicken Pet", 103).getInt(); trickEggID = config.get(itemIDs, "Trick Egg (Egg)", 530).getInt(); launcherID = config.get(itemIDs, "Chicken Launcher", 531).getInt(); trickChickenRawID = config.get(itemIDs, "Trick Chicken Raw", 532).getInt(); trickChickenCookedID = config.get(itemIDs, "Trick Chicken Cooked", 533).getInt(); // here are the achievement IDs throwEggID = config.get(achievementIDs, "Throw Egg ID", 2001).getInt(); eatChickenID = config.get(achievementIDs, "Eat Chicken ID", 2002).getInt(); buildLauncherID = config.get(achievementIDs, "Build Launcher ID", 2003).getInt(); directHitID = config.get(achievementIDs, "Direct Hit ID", 2004).getInt(); if(side == side.CLIENT) { useAdvancedSkin = config.get(set, "Advanced Chicken Texture", true, "Does the chicken mob use the advanced skin rendering?").getBoolean(true); useLauncherModel = config.get(set, "Handheld Launcher Model", true, "Does the explosive chicken launcher have a custom handheld model?").getBoolean(true); } allowChainReactions = config.get(set, "Allow Chain Reactions", true, "When a chicken explodes, will it cause a chain reaction with other chickens?\nIf you have a slow computer, i would recommend turning this off!").getBoolean(true); config.save(); } } Here's my main class package assets.exchicken.common; import java.io.File; import java.io.IOException; import org.apache.commons.io.FileUtils; import net.minecraft.block.Block; import net.minecraft.client.Minecraft; import net.minecraft.entity.EnumCreatureType; import net.minecraft.item.Item; import net.minecraft.item.ItemFood; import net.minecraft.item.ItemStack; import net.minecraft.logging.ILogAgent; import net.minecraft.logging.LogAgent; import net.minecraft.stats.Achievement; import net.minecraft.stats.AchievementList; import net.minecraft.world.biome.BiomeGenBase; import net.minecraftforge.common.MinecraftForge; import assets.exchicken.common.core.CommonProxy; import assets.exchicken.common.core.ConfigHandler; import assets.exchicken.common.core.CraftingHandler; import assets.exchicken.common.core.EventListener; import assets.exchicken.common.entity.EntityChickenFired; import assets.exchicken.common.entity.EntityExplosiveChicken; import assets.exchicken.common.item.ItemFoodExplosive; import assets.exchicken.common.item.ItemLauncher; import assets.exchicken.common.item.ItemTrickEgg; import assets.exchicken.common.network.ConnectionHandler; import cpw.mods.fml.common.FMLCommonHandler; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.EventHandler; import cpw.mods.fml.common.Mod.Instance; import cpw.mods.fml.common.SidedProxy; import cpw.mods.fml.common.event.FMLInitializationEvent; import cpw.mods.fml.common.event.FMLPreInitializationEvent; import cpw.mods.fml.common.network.NetworkMod; import cpw.mods.fml.common.network.NetworkRegistry; import cpw.mods.fml.common.registry.EntityRegistry; import cpw.mods.fml.common.registry.GameRegistry; import cpw.mods.fml.relauncher.Side; @Mod(modid="Romejanic.ExplosiveChickens", name="Explosive Chickens", version=ExplosiveChickens.currentVersion) @NetworkMod(clientSideRequired=true, serverSideRequired=false) public class ExplosiveChickens { public static final String currentVersion = "1.6"; public Item trickEgg; public Item launcher; public Item trickChickenRaw; public Item trickChickenCooked; public Achievement throwEgg; public Achievement eatChicken; public Achievement buildLauncher; public Achievement directHit; public File workingDirectory = new File(new File(".", "romejanic"), "Explosive Chickens"); public ConfigHandler config; private ILogAgent logAgent; public final String updateURL = "https://dl.dropboxusercontent.com/s/fqnx8u5scbhxp6s/UpToDate.txt?dl=1&token_hash=AAF0_aIbX-m76ip3Hz1VFN2iVUt5zLgESRkphoGMBBO-Tw"; public boolean checkedForUpdates = false; public boolean updateAvaliable = false; @Instance public static ExplosiveChickens instance; @SidedProxy(clientSide="assets.exchicken.client.core.ClientProxy", serverSide="assets.exchicken.common.core.CommonProxy") public static CommonProxy proxy; @EventHandler public void preInit(FMLPreInitializationEvent event) { File romejanicFolder = new File(".", "romejanic"); if(FMLCommonHandler.instance().getEffectiveSide() == Side.CLIENT) { romejanicFolder = new File(Minecraft.getMinecraft().mcDataDir, "romejanic"); workingDirectory = new File(romejanicFolder, "Explosive Chickens"); } if(!workingDirectory.exists()) { workingDirectory.mkdirs(); } File configFile = new File(workingDirectory, "config.txt"); if(new File(romejanicFolder, "Explosive Chickens.txt").exists()) { try { FileUtils.writeStringToFile(new File(romejanicFolder, "Explosive Chickens.txt"), "KEEP READING! IT IS IMPORTANT!\n\nSince the mod's update in 1.6.4, the config file has moved to:\n\n" + configFile + "\n\nThanks and sorry for any inconveniences!"); } catch (IOException e) { } } config = new ConfigHandler(configFile); logAgent = new LogAgent("EXCHICKENS", " - Explosive Chickens by Romejanic", new File(workingDirectory, "output.log").getAbsolutePath()); } @EventHandler public void init(FMLInitializationEvent event) { getLogger().logInfo("Setting up the mod!"); proxy.registerLang(); trickEgg = new ItemTrickEgg(config.trickEggID).setUnlocalizedName("romejanic.trickegg"); launcher = new ItemLauncher(config.launcherID).setUnlocalizedName("romejanic.chicklauncher"); trickChickenRaw = new ItemFoodExplosive(config.trickChickenRawID, (ItemFood) Item.chickenRaw).setUnlocalizedName("romejanic.chickenraw"); trickChickenCooked = new ItemFoodExplosive(config.trickChickenCookedID, (ItemFood) Item.chickenCooked).setUnlocalizedName("romejanic.chickencooked"); // here's where the achievements are registered throwEgg = new Achievement(config.throwEggID, "exchicken.throwEgg", 3, -4, Item.egg, (Achievement)null).setIndependent().registerAchievement(); eatChicken = new Achievement(config.eatChickenID, "exchicken.eatChicken", 4, -4, Item.chickenRaw, throwEgg).registerAchievement(); buildLauncher = new Achievement(config.buildLauncherID, "exchicken.buildLauncher", 3, -5, launcher, AchievementList.buildWorkBench).registerAchievement(); directHit = new Achievement(config.directHitID, "exchicken.directHit", 4, -5, Item.bow, buildLauncher).setSpecial().registerAchievement(); EntityRegistry.registerGlobalEntityID(EntityExplosiveChicken.class, "romejanic.exchick", config.entity1ID, 0xcccccc, 0xff3300); EntityRegistry.registerGlobalEntityID(EntityChickenFired.class, "romejanic.firedchicken", config.entity2ID); EntityRegistry.addSpawn(EntityExplosiveChicken.class, 10, 1, 1000, EnumCreatureType.creature, BiomeGenBase.beach, BiomeGenBase.extremeHills, BiomeGenBase.extremeHillsEdge, BiomeGenBase.forest, BiomeGenBase.plains, BiomeGenBase.taiga); GameRegistry.addRecipe(new ItemStack(launcher, 1), "SFS", "SIS", "SRS", 'S', Block.stone, 'F', Item.flint, 'I', Item.ingotIron, 'R', Item.redstone); GameRegistry.addSmelting(trickChickenRaw.itemID, new ItemStack(trickChickenCooked, 1), 100); GameRegistry.registerCraftingHandler(new CraftingHandler()); NetworkRegistry.instance().registerConnectionHandler(new ConnectionHandler()); MinecraftForge.EVENT_BUS.register(new EventListener()); proxy.registerRenderers(); getLogger().logInfo("Finished setting up!"); } public ILogAgent getLogger() { if(logAgent == null) { logAgent = new LogAgent("EXCHICKENS", " - Explosive Chickens by Romejanic", new File(workingDirectory, "output.log").getAbsolutePath()); } return logAgent; } } If anyone can help me out, thank you so much! From what I understand, it think's i am registering under an ID that is already taken, although I am not. Thanks! Romejanic
-
I downloaded the latest forge source for 1.6.4 and set it up but it's still crashing! It's the same errors! Any ideas?
-
I cd'd to the forge folder and typed python install.py. The workspace already had the assets, versions and mcl in it
-
Hi, I just set up a forge workspace. Every time I try to run it, this happens: 2014-02-26 17:56:31 [iNFO] [ForgeModLoader] Forge Mod Loader version 6.4.45.953 for Minecraft 1.6.4 loading 2014-02-26 17:56:31 [iNFO] [ForgeModLoader] Java is Java HotSpot(TM) 64-Bit Server VM, version 1.6.0_65, running on Mac OS X:x86_64:10.9.1, installed at /System/Library/Java/JavaVirtualMachines/1.6.0.jdk/Contents/Home 2014-02-26 17:56:31 [FINE] [ForgeModLoader] Java classpath at launch is /Users/130102/Desktop/ /HFZ/Mods/Flyyyyy!/workspaces/forge 1.6.4/mcp/eclipse/Minecraft/bin:/Users/130102/Desktop/ /HFZ/Mods/Flyyyyy!/workspaces/forge 1.6.4/mcp/jars/libraries/org/ow2/asm/asm-debug-all/4.1/asm-debug-all-4.1.jar:/Users/130102/Desktop/ /HFZ/Mods/Flyyyyy!/workspaces/forge 1.6.4/mcp/jars/libraries/net/minecraft/launchwrapper/1.8/launchwrapper-1.8.jar:/Users/130102/Desktop/ /HFZ/Mods/Flyyyyy!/workspaces/forge 1.6.4/mcp/jars/versions/1.6.4/1.6.4.jar:/Users/130102/Desktop/ /HFZ/Mods/Flyyyyy!/workspaces/forge 1.6.4/mcp/jars/libraries/lzma/lzma/0.0.1/lzma-0.0.1.jar:/Users/130102/Desktop/ /HFZ/Mods/Flyyyyy!/workspaces/forge 1.6.4/mcp/jars/libraries/net/sf/jopt-simple/jopt-simple/4.5/jopt-simple-4.5.jar:/Users/130102/Desktop/ /HFZ/Mods/Flyyyyy!/workspaces/forge 1.6.4/mcp/jars/libraries/com/paulscode/codecjorbis/20101023/codecjorbis-20101023.jar:/Users/130102/Desktop/ /HFZ/Mods/Flyyyyy!/workspaces/forge 1.6.4/mcp/jars/libraries/com/paulscode/codecwav/20101023/codecwav-20101023.jar:/Users/130102/Desktop/ /HFZ/Mods/Flyyyyy!/workspaces/forge 1.6.4/mcp/jars/libraries/com/paulscode/libraryjavasound/20101123/libraryjavasound-20101123.jar:/Users/130102/Desktop/ /HFZ/Mods/Flyyyyy!/workspaces/forge 1.6.4/mcp/jars/libraries/com/paulscode/librarylwjglopenal/20100824/librarylwjglopenal-20100824.jar:/Users/130102/Desktop/ /HFZ/Mods/Flyyyyy!/workspaces/forge 1.6.4/mcp/jars/libraries/com/paulscode/soundsystem/20120107/soundsystem-20120107.jar:/Users/130102/Desktop/ /HFZ/Mods/Flyyyyy!/workspaces/forge 1.6.4/mcp/jars/libraries/argo/argo/2.25_fixed/argo-2.25_fixed.jar:/Users/130102/Desktop/ /HFZ/Mods/Flyyyyy!/workspaces/forge 1.6.4/mcp/jars/libraries/org/bouncycastle/bcprov-jdk15on/1.47/bcprov-jdk15on-1.47.jar:/Users/130102/Desktop/ /HFZ/Mods/Flyyyyy!/workspaces/forge 1.6.4/mcp/jars/libraries/com/google/guava/guava/14.0/guava-14.0.jar:/Users/130102/Desktop/ /HFZ/Mods/Flyyyyy!/workspaces/forge 1.6.4/mcp/jars/libraries/org/apache/commons/commons-lang3/3.1/commons-lang3-3.1.jar:/Users/130102/Desktop/ /HFZ/Mods/Flyyyyy!/workspaces/forge 1.6.4/mcp/jars/libraries/commons-io/commons-io/2.4/commons-io-2.4.jar:/Users/130102/Desktop/ /HFZ/Mods/Flyyyyy!/workspaces/forge 1.6.4/mcp/jars/libraries/net/java/jutils/jutils/1.0.0/jutils-1.0.0.jar:/Users/130102/Desktop/ /HFZ/Mods/Flyyyyy!/workspaces/forge 1.6.4/mcp/jars/libraries/com/google/code/gson/gson/2.2.2/gson-2.2.2.jar:/Users/130102/Desktop/ /HFZ/Mods/Flyyyyy!/workspaces/forge 1.6.4/mcp/jars/libraries/net/java/jinput/jinput/2.0.5/jinput-2.0.5.jar:/Users/130102/Desktop/ /HFZ/Mods/Flyyyyy!/workspaces/forge 1.6.4/mcp/jars/libraries/org/lwjgl/lwjgl/lwjgl/2.9.0/lwjgl-2.9.0.jar:/Users/130102/Desktop/ /HFZ/Mods/Flyyyyy!/workspaces/forge 1.6.4/mcp/jars/libraries/org/lwjgl/lwjgl/lwjgl_util/2.9.0/lwjgl_util-2.9.0.jar 2014-02-26 17:56:31 [FINE] [ForgeModLoader] Java library path at launch is /Users/130102/Desktop/ /HFZ/Mods/Flyyyyy!/workspaces/forge 1.6.4/mcp/jars/versions/1.6.4/1.6.4-natives 2014-02-26 17:56:31 [iNFO] [ForgeModLoader] Managed to load a deobfuscated Minecraft name- we are in a deobfuscated environment. Skipping runtime deobfuscation 2014-02-26 17:56:31 [FINE] [ForgeModLoader] Instantiating coremod class FMLCorePlugin 2014-02-26 17:56:31 [FINE] [ForgeModLoader] Enqueued coremod FMLCorePlugin 2014-02-26 17:56:31 [FINE] [ForgeModLoader] Instantiating coremod class FMLForgePlugin 2014-02-26 17:56:31 [FINE] [ForgeModLoader] Enqueued coremod FMLForgePlugin 2014-02-26 17:56:31 [FINE] [ForgeModLoader] All fundamental core mods are successfully located 2014-02-26 17:56:31 [FINE] [ForgeModLoader] Discovering coremods 2014-02-26 17:56:31 [iNFO] [ForgeModLoader] Loading tweak class name cpw.mods.fml.common.launcher.FMLInjectionAndSortingTweaker 2014-02-26 17:56:31 [iNFO] [ForgeModLoader] Loading tweak class name cpw.mods.fml.common.launcher.FMLDeobfTweaker 2014-02-26 17:56:31 [iNFO] [ForgeModLoader] Calling tweak class cpw.mods.fml.common.launcher.FMLInjectionAndSortingTweaker 2014-02-26 17:56:31 [iNFO] [ForgeModLoader] Calling tweak class cpw.mods.fml.common.launcher.FMLInjectionAndSortingTweaker 2014-02-26 17:56:31 [iNFO] [ForgeModLoader] Calling tweak class cpw.mods.fml.relauncher.CoreModManager$FMLPluginWrapper 2014-02-26 17:56:31 [FINE] [ForgeModLoader] Injecting coremod FMLCorePlugin {cpw.mods.fml.relauncher.FMLCorePlugin} class transformers 2014-02-26 17:56:31 [FINEST] [ForgeModLoader] Registering transformer cpw.mods.fml.common.asm.transformers.AccessTransformer 2014-02-26 17:56:31 [iNFO] [sTDOUT] Loaded 40 rules from AccessTransformer config file fml_at.cfg 2014-02-26 17:56:31 [FINEST] [ForgeModLoader] Registering transformer cpw.mods.fml.common.asm.transformers.MarkerTransformer 2014-02-26 17:56:31 [FINEST] [ForgeModLoader] Registering transformer cpw.mods.fml.common.asm.transformers.SideTransformer 2014-02-26 17:56:31 [FINE] [ForgeModLoader] Injection complete 2014-02-26 17:56:31 [FINE] [ForgeModLoader] Running coremod plugin for FMLCorePlugin {cpw.mods.fml.relauncher.FMLCorePlugin} 2014-02-26 17:56:31 [FINE] [ForgeModLoader] Running coremod plugin FMLCorePlugin 2014-02-26 17:56:31 [sEVERE] [ForgeModLoader] The binary patch set is missing. Either you are in a development environment, or things are not going to work! 2014-02-26 17:56:32 [sEVERE] [ForgeModLoader] A critical exception occured reading a class file a java.util.zip.ZipException: error in opening zip file at java.util.zip.ZipFile.open(Native Method) at java.util.zip.ZipFile.<init>(ZipFile.java:128) at java.util.jar.JarFile.<init>(JarFile.java:136) at java.util.jar.JarFile.<init>(JarFile.java:73) at sun.net.www.protocol.jar.URLJarFile.<init>(URLJarFile.java:72) at sun.net.www.protocol.jar.URLJarFile.getJarFile(URLJarFile.java:48) at sun.net.www.protocol.jar.JarFileFactory.get(JarFileFactory.java:55) at sun.net.www.protocol.jar.JarURLConnection.connect(JarURLConnection.java:104) at sun.net.www.protocol.jar.JarURLConnection.getInputStream(JarURLConnection.java:132) at java.net.URL.openStream(URL.java:1010) at net.minecraft.launchwrapper.LaunchClassLoader.getClassBytes(LaunchClassLoader.java:365) at cpw.mods.fml.common.patcher.ClassPatchManager.getPatchedResource(ClassPatchManager.java:65) at cpw.mods.fml.common.asm.transformers.deobf.FMLDeobfuscatingRemapper.getFieldType(FMLDeobfuscatingRemapper.java:225) at cpw.mods.fml.common.asm.transformers.deobf.FMLDeobfuscatingRemapper.parseField(FMLDeobfuscatingRemapper.java:202) at cpw.mods.fml.common.asm.transformers.deobf.FMLDeobfuscatingRemapper.setup(FMLDeobfuscatingRemapper.java:163) at cpw.mods.fml.common.asm.FMLSanityChecker.injectData(FMLSanityChecker.java:245) at cpw.mods.fml.relauncher.CoreModManager$FMLPluginWrapper.injectIntoClassLoader(CoreModManager.java:123) at net.minecraft.launchwrapper.Launch.launch(Launch.java:111) at net.minecraft.launchwrapper.Launch.main(Launch.java:27) Any ideas as to what's happening? Thanks - Romejanic
-
For making a GUI, make a new class extending the GuiScreen class. You can look in the net.minecraft.client.gui packages for help. When it comes to displaying it, you just say Minecraft.getMinecraft().displayGuiScreen(new MyGuiScreen()); But make sure this code is only executed in a @SideOnly(Side.CLIENT) method or inside a if(FMLCommonHandler.instance().getEffectiveSide == Side.CLIENT) statement to stop your mod crashing on servers. Hope I helped! Romejanic
-
You can't be serious! I won't update my mods until that is fixed