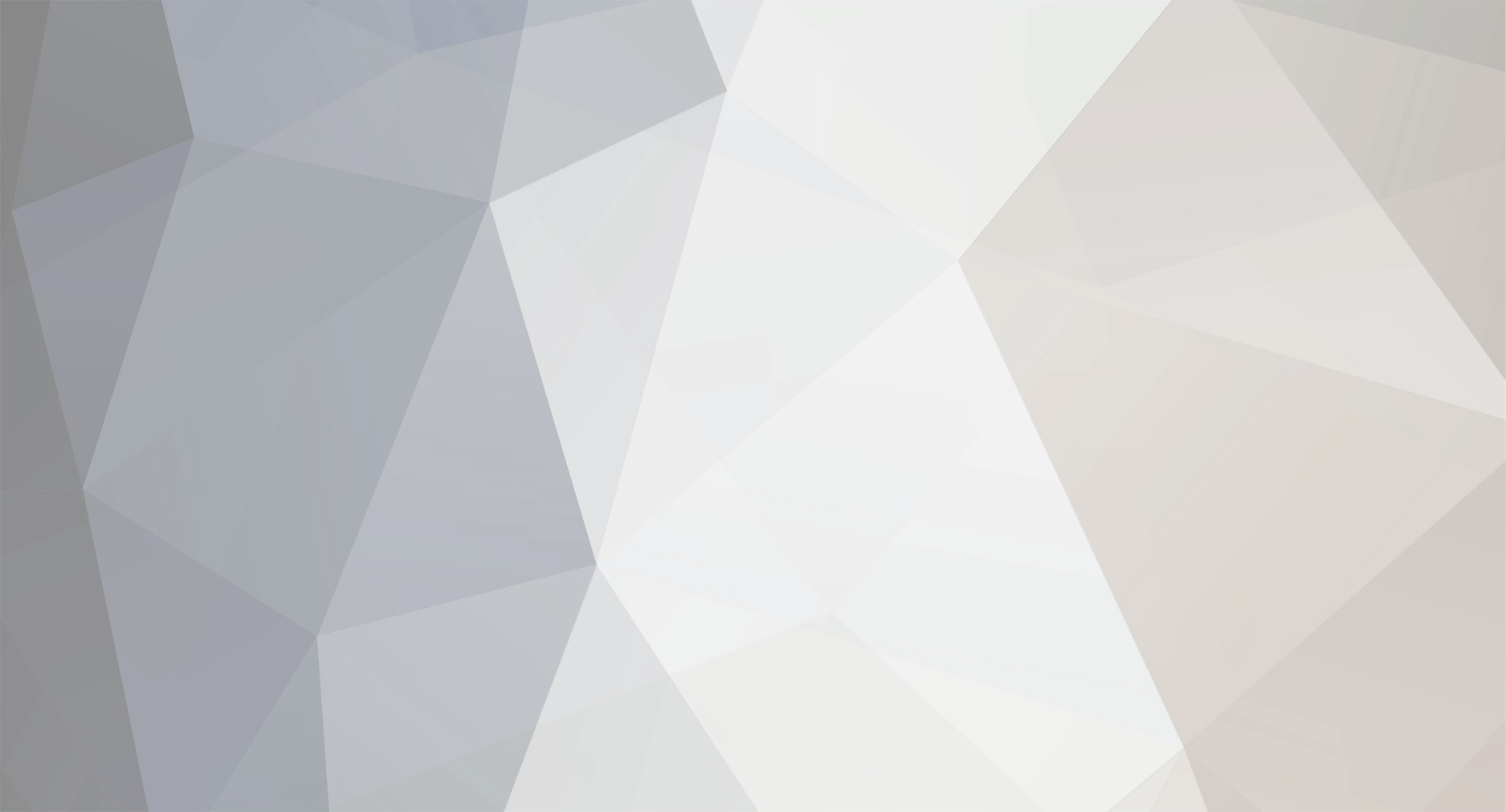
MrCaracal
Members-
Posts
80 -
Joined
-
Last visited
Everything posted by MrCaracal
-
If I'm understanding right, you want an RNG to seed your breathing animation function with so that you don't have every single animal breathing in synchronicity. Instead of telling you how to do this, may I make a fundamental design suggestion? It sounds like you don't need a "random" value, just that you need a "relatively unique" value. Why not make some kind of hash function for the entity's unique ID? Use entity.getUniqueID() as your "seed" so to speak, and have some method work it into the range you need for your "starting breath" value.
-
The "src forge" absolutely does contain source. I just downloaded the 1.6.4 just now to see. It does not, however, contain minecraft's source code (in case that's what you're asking about) as distributing that is illegal. As far as I know you can use ForgeGradle to set up a development environment for 1.6.4 (1.6.2 is the earliest supported version I believe) if you absolutely must for whatever reason. Just set the version you are targeting in the build.gradle file. I would implore you to update your server and target newest builds, but I see you are a necromancer and enjoy playing with dead things.
-
[1.7.10] [Resolved] Help with creating custom tree generation.
MrCaracal replied to Awesome_Spider's topic in Modder Support
I'm going to be blunt here, Awesome_Spider, and I hope you don't find this discouraging. I see a lot of copy-paste going on with little thought given to what the methods you're using actually do or how they function. For instance, I see you directly copy-pasted Kuu's world generation suggestion, which is itself lifted directly from the Forge Wiki's tutorial section. You even preserved the number of iterations in the for-loop and the comment label, erroneously referring to the value 15 as "rarity". If we look at your code however, what it does is call the tree generator 15 times per generateSurface call, which itself is called on every chunk. Is this actually what you want? My advice to you at this point is to set aside a bit of time to go through the code you are using and try to determine how all the pieces fit together. If you must copy-paste, you should at least understand the bits you are using, otherwise, how will you understand what's going on when things don't work? Moreover, how will you know whether the suggestions you are taking are actually what you want? I am certain that if you sit down and sift through your current code and figure out how it is working, the source of the issue you are having will become apparent. You got this far, so I know you can do it! Go forth and conquer! -
Custom EntityItem going random direction when thrown
MrCaracal replied to The_Fireplace's topic in Modder Support
Could it be because you are applying a random velocity to the entity? this.motionX = (double)((float)(Math.random() * 0.20000000298023224D - 0.10000000149011612D)); this.motionY = 0.20000000298023224D; this.motionZ = (double)((float)(Math.random() * 0.20000000298023224D - 0.10000000149011612D)); -
[1.7.10] [SOLVED] custom block step/break sound
MrCaracal replied to Vogner's topic in Modder Support
I think you could probably randomize pitch without any additional code by taking advantage how the existing sound system works. This isn't something I've tried myself, though. Provided you're working with 1.7.2 or newer, of course, sounds are loaded based on a few parameters defined in the Sounds.json file: http://minecraft.gamepedia.com/Sounds.json I don't work with caracals but I sometimes wish I do. They are very cool. -
[1.7.10] Game lags a ton when generating blocks in the sky
MrCaracal replied to Eternaldoom's topic in Modder Support
Thank you, that makes sense, very informative. I am quite new to this stuff in general, so thanks for the link as well. -
[1.7.10] Game lags a ton when generating blocks in the sky
MrCaracal replied to Eternaldoom's topic in Modder Support
Would you mind terribly pointing me towards some literature to that effect, then? I know that this is not "java school" but I have always thought that the "new" keyword will always allocate a new object, and looking it up now I can't find anything that contradicts that. I also just wrote a little for-loop that does nothing but instantiate a new object and print its identity hashcode, and it is most certainly making new allocations when I use the new keyword. Under what conditions does the compiler optimize that away? -
[1.7.10] Game lags a ton when generating blocks in the sky
MrCaracal replied to Eternaldoom's topic in Modder Support
Huh! I did not know the JIT was clever enough to deal with stuff like that. I just assumed that it would unthinkingly make a new object when explicitly told to in the source, not knowing whether the programmer had special handling in the constructor and would therefore truly want a new object. Never mind then, and thanks for the info, diesieben! -
[1.7.10] Game lags a ton when generating blocks in the sky
MrCaracal replied to Eternaldoom's topic in Modder Support
Well I can tell you one thing that's causing issues, it's a segment like this: for(int i = 0; i < 2; i++) { var12 = 16; var13 = 20; var14 = 16; (new WorldGenMinable(VetheaBlocks.dreamGrass, 16, VetheaBlocks.dreamStone)).generate(this.worldObj, this.rand, var12, var13, var14); } WorldGenMinable is itself a generator, you can reuse it instead of creating a new one every time you need an ore cluster generated. Otherwise, you will be creating new WorldGenMinable objects like crazy, which I would guess is a huge source of your observed lag. Instead, create a single static WorldGenMinable object for a particular type of worldgen and call it at all the appropriate points. This will avoid the massive amount of object allocation-deallocation you would have going on with the above. -
[1.7.10] [SOLVED] custom block step/break sound
MrCaracal replied to Vogner's topic in Modder Support
Did you override the methods in your custom SoundType class to point to the correct resource location? Here is a case of the approach I have suggested in action: https://github.com/Pokefenn/Chisel/blob/master/src/main/java/info/jbcs/minecraft/chisel/block/BlockCarvable.java This is not my code, but it is an open-source example of a mod with custom sound types that uses an extension of SoundType to accomplish custom break and step sounds without the use of a sound event handler. I think you will find that this approach is simpler and with less overhead than a sound event manager. -
Switch statements have a "default" case which handles all cases you don't explicitly specify. int number; ... switch (number) { case 0: // do thing given number == 0 break; case 1: // do thing given number ==1 break; default: // do thing for all unspecified cases break; }
-
[1.7.10] Custom bow animation and behaviour
MrCaracal replied to MuffinMonster's topic in Modder Support
It certainly does seem that way, unfortunately. If you want it to resemble the way the vanilla bow item is scaled and rotated when held by a third-person entity and not just the way a held item is, you will need a special renderer to tell Minecraft to do that. -
What does that mean? We cannot help you solve this issue if you do not tell us what your issue is. "It is broken" provides us with exactly zero insight. Crash reports, descriptions of the problem, what you are expecting to do versus what is happening, code - all of these things will help us help you.
-
[1.7.10] [SOLVED] custom block step/break sound
MrCaracal replied to Vogner's topic in Modder Support
I'm not sure you will need a whole SoundEvent handler just for block step and break sounds. In net.minecraft.block, those sounds are defined by an anonymous class, SoundType. Surely you would only need to create your own SoundType and pass that to the setStepSound method in your block's constructor? For insight as to how this works, check out net.minecraft.block. All the SoundType fields are at the very top of the Block class, the implementation is at the very bottom. I believe the String passed to the SoundType constructor is handled like a ResourceLocation, except the methods in SoundType dumbly prefix the expected sounds with "dig", so you'll need your own class that extends SoundType that doesn't do this. -
[1.7.2][Solved] Can still fly when gravity chestplate is off
MrCaracal replied to skullcrusher1005's topic in Modder Support
This is not what those annotations mean exactly. See here: http://www.minecraftforge.net/forum/index.php/topic,22764 -
[1.7.10] [Resolved] Help with creating custom tree generation.
MrCaracal replied to Awesome_Spider's topic in Modder Support
What does your world generator look like, Awesome_Spider? Not the tree bit, the one that implements IWorldGenerator. -
I suppose I wasn't overt enough before. I apologize if this comes off as rude. -You are applying a velocity to things that have an acceleration. This is why you're seeing a "slowing" effect but not a "stopping" effect, you're only removing one dimension of the object's displacement. Acceleration is displacement over time squared, if you remove one dimension of this, you're left with a displacement over time, a velocity. -By adding universal "set velocity of this entity" rule, you may encounter odd behavior in the future if ever your mod is installed alongside another that has different movement rules for their entities. There is a nonzero chance of this happening, because Forge provides ways for modders to accomplish precisely this. Instead, I would suggest picking around in the net.minecraft.entity superclass, which is the point from which all the objects you care about extend. There are a few functions in there that may catch your eye, namely setPosition, setVelocity (as brandon3055 suggested), moveEntity, and even some public fields dealing with this stuff such as isCollided (and the ones you've found, motionX, motionY, motionZ). You might be able to trick the entity into thinking it has collided with something while it is in your AABB, thereby halting it's movement, or just repeatedly reset the position of the entity, etc. Failing all that, you could even try to write your own EntityItem that doesn't move, and have that object temporarily replace all the ones in your AABB. What's the scope of "all entities movement", if I might ask? Does it include blocklike entities such piston heads, blocks moved by pistons, TNT and other moving entities like arrows, snowballs, particles, etc? Movement-like block state changes, like lever flips, button pushes, doors being closed? Unrelated: I noticed you're using a List, and lists are iterable. Have you yet opened your heart to the Church of the For-Each-Loop? I love them. for (Entity iteratedEntity : entitiesToFreeze) { // For each element iteratedEntity in the list entitiesToFreeze, iteratedEntity.extinguish(); // call the extinguish method on it. }
-
If you're afraid of losing your files, back them up first. Your source is located in /src/main/java/. Your assets are nearby in /src/main/resources. Just put them somewhere else while you update, and put them back when you're done. MuffinMonster was right about what you had to do about updating, but there's another detail. You have to edit build.gradle: In build.gradle there is a field that should look something like this: minecraft { version = [version number] assetDir = "eclipse/assets" } You must change that version to the full version number of whatever Forge build you would like to use. Then, run the commands that MuffinMonster specified again.
-
You could certainly try that, but would it work in every case? For instance, what would happen to an entityItem within your bounding box that has a different onUpdate method?
-
[1.7.2][Solved] Can still fly when gravity chestplate is off
MrCaracal replied to skullcrusher1005's topic in Modder Support
OnUpdate is only called on items in the inventory. If the armor is not in the inventory, that method is not called. Three's the charm? -
A quick glance at EntityItem's class reveals the following line (118), in the onUpdate method: this.motionY -= 0.03999999910593033D; I believe this is to simulate gravity. This is the reason why items fall "slower" in your bounds - their acceleration is being effectively zeroed by your code setting their Y motion to zero. They are not stopped because EntityItem has it's own ideas and sometime after you set the entity's velocity to zero, the onUpdate method is called and suddenly they're moving again. Fortunately, EntityItem does check the noClip bounding box before actually moving the items, perhaps you could take advantage of this?
-
[1.7.2][Solved] Can still fly when gravity chestplate is off
MrCaracal replied to skullcrusher1005's topic in Modder Support
onPlayerStoppedUsing is for held items. -
[1.7.10] [Resolved] Help with creating custom tree generation.
MrCaracal replied to Awesome_Spider's topic in Modder Support
To answer the question in your edit, WorldGenCanopyTree is the "big" oak trees' generation algorithm. -
[1.6.4] Changing the chance of a custom villager trade
MrCaracal replied to Da9L's topic in Modder Support
Well, I don't know that there is a good way of accomplishing that without crowding other mods' trades in return. There are quite a few methods in the VillagerRegistry that your mod can use to fiddle with the trade manifests. You're probably interested in manipulateTradesForVillager, which would let you directly manipulate the MerchantRecipeList of a given villager, or manageVillagerTrades which is a callback you could use for dealing with them in aggregate. If you want a guaranteed way of accessing your custom trades, would you be willing to consider a custom villager? I understand that this isn't in the original scope of your mod's functionality but I feel it would be a better solution (in terms of compatibility, which is the root of your problem here) than just budging other mods' trades to the side. There are handy methods in the VillagerRegistry for this too, including ones that set the skin and whatnot. TL;DR - Poke through VillagerRegistry.class, the answers are therein! EDIT: Also, 1.6.4?