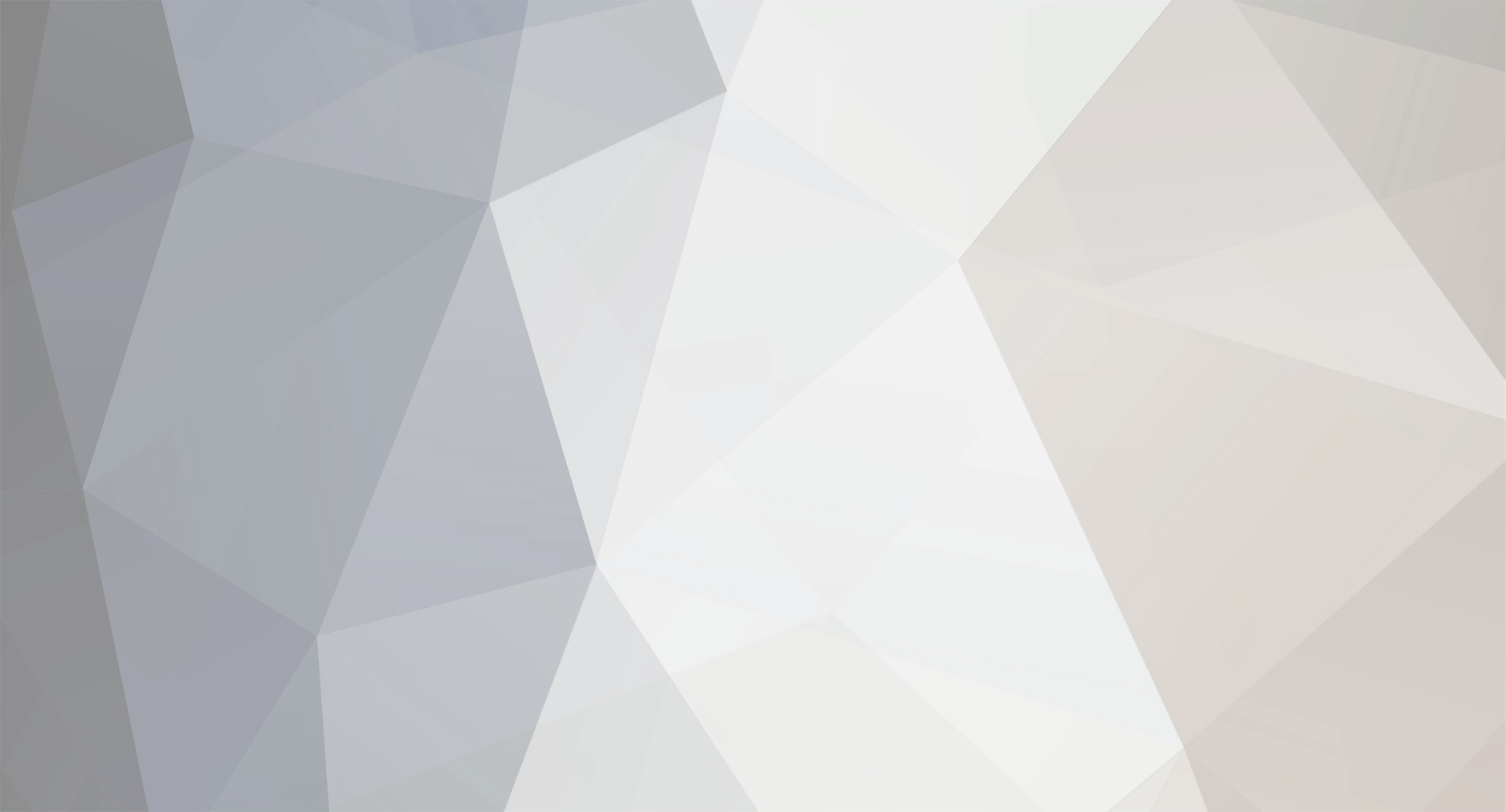
imadnsn
-
Posts
186 -
Joined
-
Last visited
Posts posted by imadnsn
-
-
you should do This in the constructor:
setMaxDamage(0); setHasSubtypes(true);
-
There is no loop, in your stile class override isLadder method. which is:
@Override public boolean isLadder(IBlockAccess world, int x, int y, int z, EntityLivingBase entity)
Since it gives you EntityLivingBase, just check for if entity is instance of EntityPlayer
If (entity != null && entity instanceof EntityPlayer) { return true; } return false;
-
have you tried different values of enchantability? Gold has 22 for example.
The problem is surely not relating to the way the item is registered.
-
And the reason why I tossed .toLowerCase() in is because some of my custom items I use camel case like itemRelic and if I wanted to compare "itemRelic" to "itemrelic" it won't work (or would it?) so I just make them both lowercase to be safe.
You can use .equalsIgnoreCase if you're worried about cases
-
in java, you cannot use == operator for strings, it checks for references, not the hash code of the string. Instead, use:
theitem.equals("diamond");
also remember you are using "substring(5)" so you probably want
if (theitem.toLowerCase().equals("diamo"))
No! substring(5) gives you the string starting from index 5. he used that because getUnlocalizedName prefixes "tile." to blocks' names and "item." to items' names
-
This is forum for people who want support writing their mod in forge, you thread is not in the right place.
-
By the way, if you're feeling limited with the metadata due to small number of bits to work with you can create your own sort of extended metadata if you want. In your main class you can just make a public byte array with index being position of the block for example and just make sure to update it when blocks are placed or destroyed.
If you mean a public array to store one block's coordinates, which I don't think you do, I don't think that's a good idea, since all what the metadata's about is the inability to have a field that applies to one block, as a field ingame is like a static field because you use one Block instance.
If you mean a list with coordinates as key, then that's not good idea either, unless you're sure your block won't exist a lot in a world. It will have some instabilities too, such as if piston pushes a block.
The way Minecraft handle coordinate specific things to blocks is known to everyone, tile entities.
-
How will you get the coords only from the block class?
They meant overriding methods in Block class such as onBlockPlaced, those methods have the coordinates as there parameters
-
Firstly, the package should be assets.modid.textures.entity.bork, make sure everything is lowercase or it won't work.
Secondly use either ResourceLocation("modid:textures/entity/bork/bork.png") or ResourceLocation("modid", "textures/entity/bork/bork.png")
Thirdly, it is better practice to define your modid as a "static final String" in you mod's base class in case you want to use it again (you will!), if you do so, replace the (modid = "McFarms") to (modid = McFarms.modid (or whatever you named your variable)) in the @Mod annotation
-
return false in isLadder method (override if you didn't) if entity isn't instance of EntityPlayer
-
Did it crash or just nothing happened
-
Try extending Item in your ItemFB class and overriding getItemEnchantability in your bow class. Although I haven't tried that, but I think something more that 0 will let your item be enchantable.
-
You should note that sometimes, durability reduces by two, such as when you break a block with the sword, you can overcome this by overriding onBlockDestroyed with the same code in ItemSword, but you replace the item once the durability hits getMaxDurability - 2 instead of damaging the ItemStack. Like so:
@Override public boolean onBlockDestroyed(ItemStack stack, World world, Block block, int x, int y, int z, EntityLivingBase entity) { if ((double)block.getBlockHardness(world, x, y, z) != 0.0D) { if (stack.getItemDamage() == stack.getMaxDamage() - 2) { // replace item and return true } stack.damageItem(2, entity); } return true; }
-
Metadata have always been used for reduced code repetition, for example, you won't make 6 different stair blocks all the same but different orientation, metadata only reduced id usage in some cases.
Actually, reduced code repetition is kind of what efficient programming is. Object-oriented programming was made to reduce the amount of code you use to make the program by having some kind of structure, i.e. class, that has all the relevant code, and use instances whenever needed.
This means metadata is only another thing to use so you won't make blocks for everything, not only because you don't want to use up all the ids.
-
If I will extandened the ItemBow class, what will I do with my Item class? there are methods there that I want all of my items to use so should I copy the methods from there? What should I do with those 2 classes?
ItemBow extends Item, if you don't know how that makes sense then learn java before modding
-
If the number of textures is less than 5, you can also use metadata with bit-shifting since there are only four states of a block with front texture.
-
You can also use getDrops to return a list of ItemStack with metadata using the block's metadata. I use this in my ore which drops different kind of gems depending on block's metadata.
You should note that damageDropped is also called on silk touch and pick block. So if the metadata of the block is important, let it always return the metadata.
-
That makes the use of server proxies only for multiplayer, but it does make sense to me since the proxy instance has to be something and Minecraft processes with integrated server are clients too and need the client-side code.
-
So basically, I should register renderers and keybindings and client-side configs in client proxies?
-
I have got what proxies are for, I know how they should be made and used, but one question persists, when?
All I want is to get an idea of when I should start to think about putting things, and what things, in my proxy classes. Can you give me examples to get the idea?
-
Ok, but what if some blocks give off light, and others shouldn't give off light?
ItemBlock doesn't have anything to do with the Block's functionality, it is just an item that represents the block. What I meant by "custom functionality" is that if, for example, you do something with your mod when player right-clicks with the ItemBlock (or the item that represents the block) in his hand.
Other than that, custom ItemBlock classes aren't needed.
-
Firstly, ItemBlock class isn't necessary for every block, if it doesn't have custom functionality or use of metadata then not using it is fine.
Secondly, if all the ItemBlock classes do exactly the same thing then you can put that thing in one class and use metadata for distinguishing between different blocks.
-
Then that's not the case of block updating.
What you should do is make the portal when you put the fluid, this happens in onBlockAdded event of the fluid's class, simply override it, remember to keep the super call, since the block liquid uses it.
-
I extanded my items class but copied the bow's code so it will automatically have some methods that all of my items are using, is there any difrence? and btw, can I extanded both my items class and the bow class?
Yes, there's difference, ItemBow is accepted by enchantment table and can be enchanted.
No, java doesn't support multiple base classes
[1.7.10] Managing textures for custom gui
in Modder Support
Posted
Edit: never mind all the nonsense I wrote, I was literally saying what wasn't the problem... The "thing", LOL
what was I thinking? 