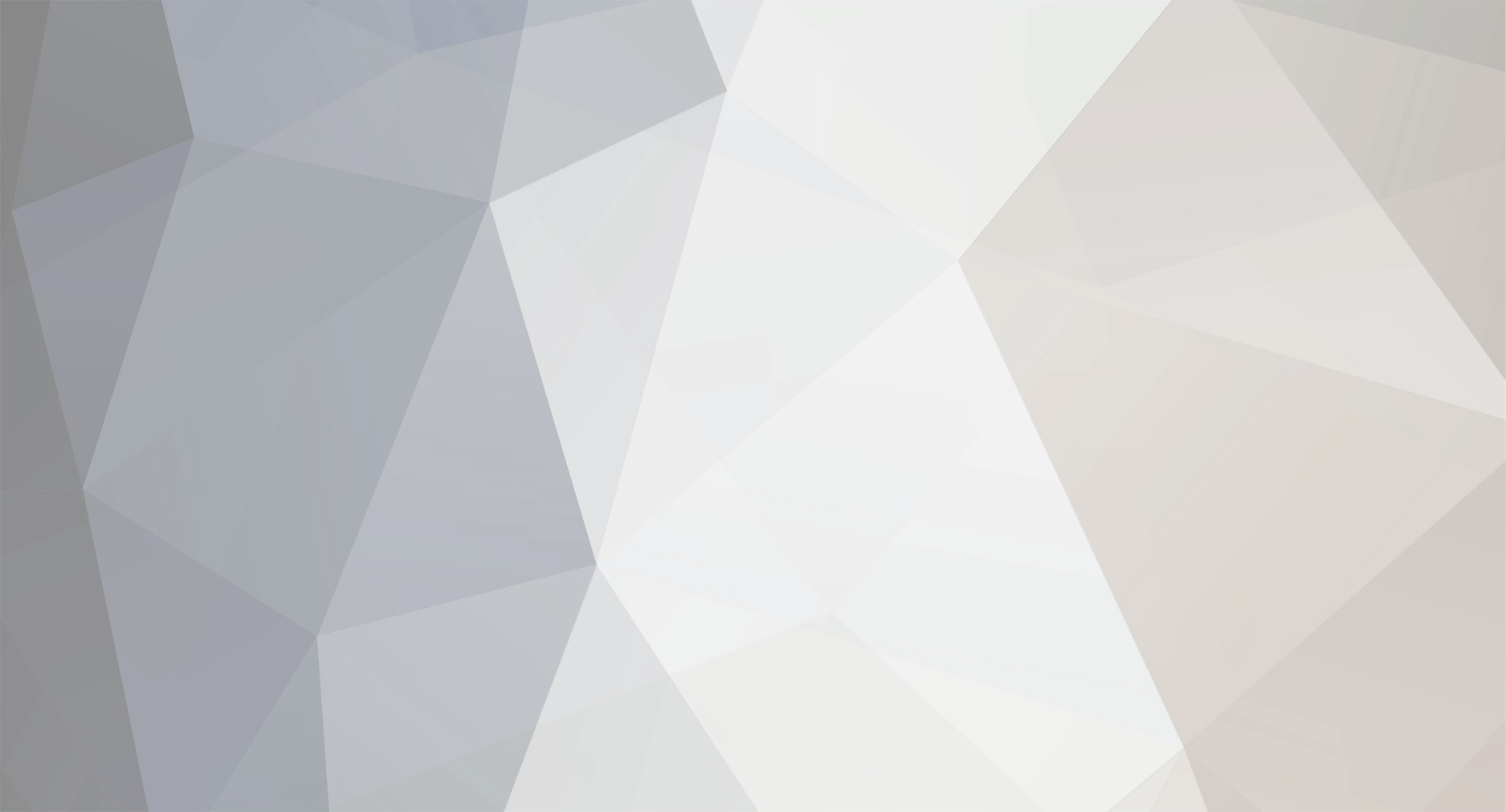
itsonlydan
Members-
Posts
8 -
Joined
-
Last visited
Converted
-
Gender
Undisclosed
itsonlydan's Achievements

Tree Puncher (2/8)
0
Reputation
-
Thank you! That was a stupid oversight on my part. I've got it working now. That wasn't the only step to fixing this, so just in case anyone in the future is having trouble doing a similar thing, my rendering code now looks like this: @Override public void renderTileEntityAt(TileEntity tileEntity, double d0, double d1, double d2, float f) { TileEntityCamouflaged te = (TileEntityCamouflaged)tileEntity; Block block = te.getTextureBlock(); Minecraft.getMinecraft().renderEngine.func_110577_a(TextureMap.field_110575_b); //Disable standard lighting. This is done the same as in TileEntityRendererPiston. RenderHelper.disableStandardItemLighting(); GL11.glBlendFunc(GL11.GL_SRC_ALPHA, GL11.GL_ONE_MINUS_SRC_ALPHA); GL11.glEnable(GL11.GL_BLEND); //GL11.glDisable(GL11.GL_CULL_FACE); This was initially part of the code that was taken from the piston, but it was causing some of the rendering to look of, e.g. with a cobweb (it would look thicker as both sides were rendering due to this being disabled). if (Minecraft.isAmbientOcclusionEnabled()) { GL11.glShadeModel(GL11.GL_SMOOTH); } else { GL11.glShadeModel(GL11.GL_FLAT); } GL11.glPushMatrix(); GL11.glTranslated(d0, d1, d2); Tessellator tessellator = Tessellator.instance; tessellator.startDrawingQuads(); RenderBlocks renderBlocks = new RenderBlocks(tileEntity.worldObj); //To get the block to render in the right place, I had to pass in 0, 0, 0 as the coordinates for renderBlockByRenderType. This however means that it will use the properties of the block at 0, 0, 0 for some things. This translation allows for the actual block coordinates to be passed in. tessellator.setTranslation(-te.xCoord, -te.yCoord, -te.zCoord); renderBlocks.renderBlockByRenderType(block, te.xCoord, te.yCoord, te.zCoord); tessellator.draw(); tessellator.setTranslation(0, 0, 0); GL11.glPopMatrix(); //Reset to standard lighting RenderHelper.enableStandardItemLighting(); }
-
It gets the render type of the block passed in, and then goes on to add vertexes to the tessellator instance based on the block passed in as the first parameter. It does call the right method (e.g. if I pass in a cobweb, then it calls the renderCrossedSquares function), and the methods are being called to add coordinates to the tessellator. I'm still not sure what I'm doing wrong.
-
Check if the icon that you're about to return is null. If it isn't, return that icon, otherwise return the blockIcon
-
Have a look at net.minecraftforge.event.entity.living.LivingDeathEvent, and net.minecraft.util.EntityDamageSource. On a LivingDeathEvent, you could check if the entity is an instance of EntityPlayer, and if the DamageSource is of the type in DamageSource.causePlayerDamage I hope that this helped.
-
I am attempting to make a block that will appear as another block in the game, depending on the id passed into it. To do this I am using a TileEntitySpecialRenderer, and calling RenderBlocks.renderBlockByRenderType, as shown below. This works perfectly for all 1mx1mx1m blocks, but it renders everything else at that size. For items that are not full blocks, but are using textures that are from full block (e.g. half slabs and fences), this just renders a full block using that texture (so a fence appears as oak planks). For other blocks (such as a cactus), there are just gaps between the textures. I don't have much experience with custom rendering in minecraft/forge, and was hoping that somebody could help. From my TileEntitySpecialRenderer subclass: @Override public void renderTileEntityAt(TileEntity tileEntity, double d0, double d1, double d2, float f) { TileEntityCamouflaged te = (TileEntityCamouflaged)tileEntity; Block block = te.getTextureBlock(); RenderBlocks renderBlocks = new RenderBlocks(tileEntity.worldObj); renderBlocks.renderBlockByRenderType(block, te.xCoord, te.yCoord, te.zCoord); } Methods overwritten in my block class: @Override public boolean renderAsNormalBlock() { return false; } @Override public boolean isOpaqueCube() { return false; } @Override public boolean shouldSideBeRendered(IBlockAccess blockAccess, int x, int y, int z, int side) { System.out.println("shouldSideBeRendered at " + x + ", " + y + ", " + z + " on side " + side); int newX = x; int newY = y; int newZ = z; //The x, y and z coordinates appear to change because of the side switch (side) { case 0: ++newY; break; case 1: --newY; break; case 2: ++newZ; break; case 3: --newZ; break; case 4: ++newX; break; case 5: --newX; break; } TileEntityCamouflaged tileEntity = (TileEntityCamouflaged)blockAccess.getBlockTileEntity(newX, newY, newZ); if (tileEntity == null) return false; return tileEntity.getTextureBlock().shouldSideBeRendered(blockAccess, x, y, z, side); } @Override public Icon getBlockTexture(IBlockAccess blockAccess, int x, int y, int z, int side) { TileEntityCamouflaged tileEntity = (TileEntityCamouflaged)blockAccess.getBlockTileEntity(x, y, z); return tileEntity.getTextureBlock().getBlockTexture(blockAccess, x, y, z, side); } @Override public boolean isBlockSolid(IBlockAccess blockAccess, int x, int y, int z, int side) { TileEntityCamouflaged tileEntity = (TileEntityCamouflaged)blockAccess.getBlockTileEntity(x, y, z); return tileEntity.getTextureBlock().isBlockSolid(blockAccess, x, y, z, side); } @Override public int colorMultiplier(IBlockAccess blockAccess, int x, int y, int z) { System.out.println("colorMultiplier at " + x + ", " + y + ", " + z); TileEntityCamouflaged tileEntity = (TileEntityCamouflaged)blockAccess.getBlockTileEntity(x, y, z); return tileEntity.getTextureBlock().colorMultiplier(blockAccess, x, y, z); } @Override public void setBlockBoundsBasedOnState(IBlockAccess blockAccess, int x, int y, int z) { TileEntityCamouflaged tileEntity = (TileEntityCamouflaged)blockAccess.getBlockTileEntity(x, y, z); tileEntity.getTextureBlock().setBlockBoundsBasedOnState(blockAccess, x, y, z); } @Override public AxisAlignedBB getCollisionBoundingBoxFromPool(World world, int x, int y, int z) { Block block = ((TileEntityCamouflaged)world.getBlockTileEntity(x, y, z)).getTextureBlock(); return block.getCollisionBoundingBoxFromPool(world, x, y, z); } @Override public int getLightOpacity(World world, int x, int y, int z) { Block block = ((TileEntityCamouflaged)world.getBlockTileEntity(x, y, z)).getTextureBlock(); return block.getLightOpacity(world, x, y, z); } @Override public AxisAlignedBB getSelectedBoundingBoxFromPool(World world, int x, int y, int z) { Block block = ((TileEntityCamouflaged)world.getBlockTileEntity(x, y, z)).getTextureBlock(); return block.getSelectedBoundingBoxFromPool(world, x, y, z); } @Override public boolean isBlockNormalCube(World world, int x, int y, int z) { Block block = ((TileEntityCamouflaged)world.getBlockTileEntity(x, y, z)).getTextureBlock(); return block.isBlockNormalCube(world, x, y, z); } @Override public boolean isBlockSolidOnSide(World world, int x, int y, int z, ForgeDirection side) { Block block = ((TileEntityCamouflaged)world.getBlockTileEntity(x, y, z)).getTextureBlock(); return block.isBlockSolidOnSide(world, x, y, z, side); } //I am not sure about this one, though it fixes the rendering of ice, and doesn't appear to cause problems with anything that already worked. @Override public int getRenderBlockPass() { return 1; }