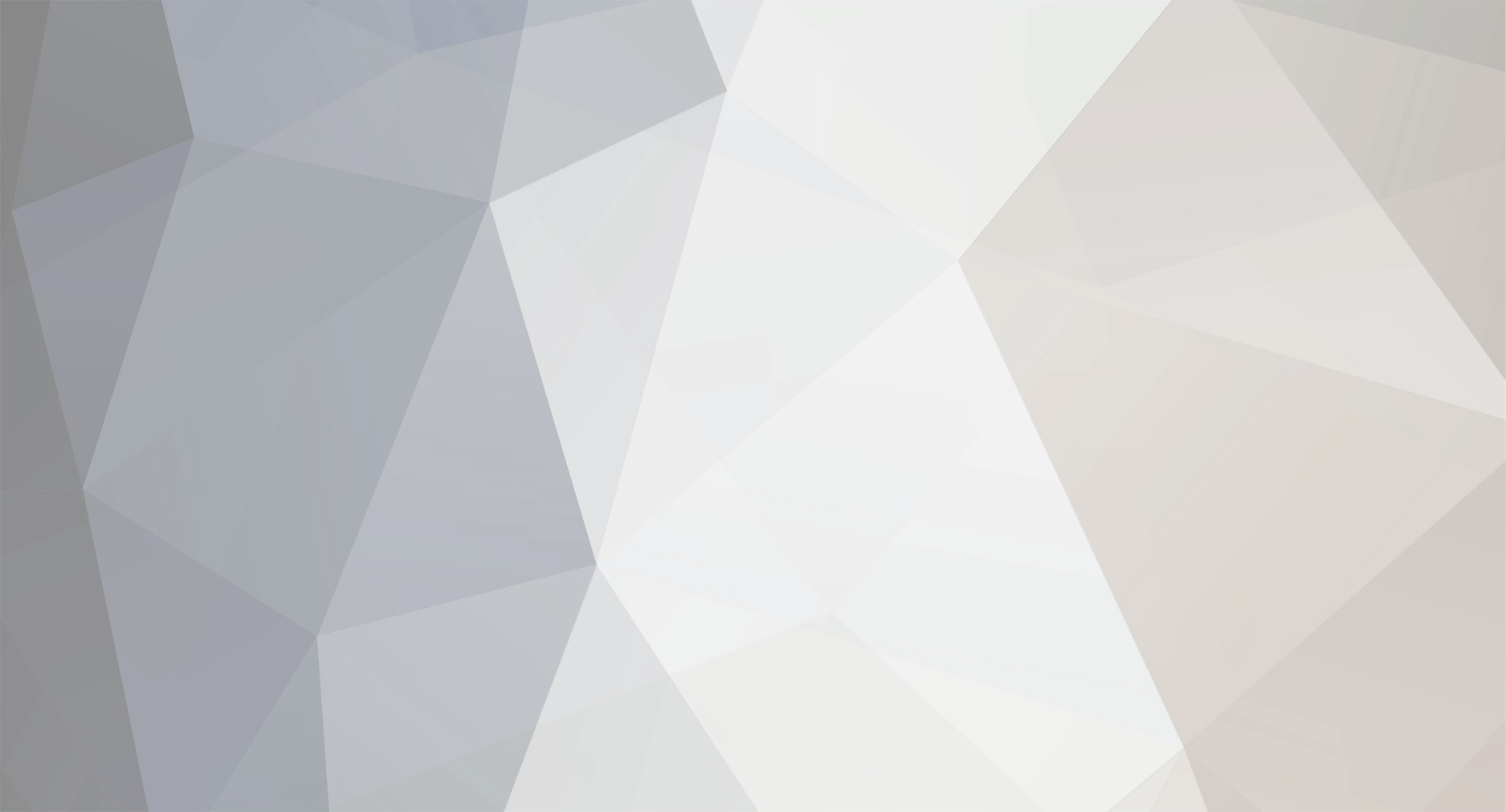
AskHow1248
Members-
Posts
123 -
Joined
-
Last visited
Everything posted by AskHow1248
-
[1.5.2] How to make an item that has an inventory?
AskHow1248 replied to AskHow1248's topic in Modder Support
New problem. I've updated my mcp to 1.6.2, but now I get an error in your ItemStore class. -
[1.5.2] How to make an item that has an inventory?
AskHow1248 replied to AskHow1248's topic in Modder Support
Your common proxy doesn't work with 1.5.2. -
I have overridden getBlockColor like this: public int getBlockColor() { if(blockToCopy != null) { return blockToCopy.getBlockColor(); } return 16777215; } Where blockToCopy is the block whose texture I'm using. Also: Leaves do the same thing as grass. Plus it makes Ice opaque and some blocks (tall grass) use the wrong render type.
-
I want to a keystone like item but I don't have any idea how to make a item have an inventory.
-
I'm trying to make a block that blends in with it's surroundings, like a Ars Magica Illusion Block or a secret Rooms Mod Camo Block. I have absolutely no idea how to go about this. Any help would be appreciated. update: I think it might have something to do with the getBlockTexture method in the Block class.
-
I have made some magic armor (gravitite) that allows you to fly, but when I take it off, I can still fly. I want it to be able to work with other ways of flight though (like Thaumcraft's Thermostatic Harness or Ars Magica's Flight Spell). Edit: it works properly if I take the gravitite chestplate off and put the Earth chestplate on instead. My code is: Tick Handler: -------------------------------------------------------------------------------------------------------------------------------------------------------- import java.util.EnumSet; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.ItemStack; import net.minecraft.potion.Potion; import net.minecraft.potion.PotionEffect; import net.minecraftforge.event.ForgeSubscribe; import net.minecraftforge.event.entity.living.LivingEvent.LivingUpdateEvent; import cpw.mods.fml.common.ITickHandler; import cpw.mods.fml.common.TickType; public class ServerTickHandler implements ITickHandler { private boolean isWearingGravitite; private boolean isWearingEarthChest; //you keep flying once you take the armor off private void onPlayerTick(EntityPlayer player) { if (player != null && player.getCurrentItemOrArmor(4) != null && player.getCurrentItemOrArmor(3) != null && player.getCurrentItemOrArmor(2) != null && player.getCurrentItemOrArmor(1) != null) { ItemStack Helmet = player.getCurrentItemOrArmor(4); ItemStack Chestplate = player.getCurrentItemOrArmor(3); ItemStack Leggings = player.getCurrentItemOrArmor(2); ItemStack Boots = player.getCurrentItemOrArmor(1); ItemStack EarthChestplate = new ItemStack(MagicOres.EarthChestplate); isWearingGravitite = (Helmet.getItem() == MagicOres.GravititeHelmet && Chestplate.getItem() == MagicOres.GravititeChestplate && Leggings.getItem() == MagicOres.GravititeLeggings && Boots.getItem() == MagicOres.GravititeBoots); isWearingEarthChest = (Chestplate.getItem() == MagicOres.EarthChestplate || Chestplate == EarthChestplate); if (isWearingGravitite == true) { player.capabilities.allowFlying = true; player.sendPlayerAbilities(); player.setFlying(true); } if(player.capabilities.isCreativeMode == false && (isWearingGravitite == false || player.getCurrentItemOrArmor(4).getItem() != MagicOres.GravititeHelmet || player.getCurrentItemOrArmor(3).getItem() != MagicOres.GravititeChestplate || player.getCurrentItemOrArmor(2).getItem() != MagicOres.GravititeLeggings || player.getCurrentItemOrArmor(1).getItem() != MagicOres.GravititeBoots)) { player.capabilities.allowFlying = false; player.capabilities.isFlying = false; player.isAirBorne = true; player.onGround = false; player.sendPlayerAbilities(); } //won't work if (player.getCurrentItemOrArmor(3) == EarthChestplate) { player.addPotionEffect(new PotionEffect(Potion.resistance.getId(), 20, 1)); player.addPotionEffect(new PotionEffect(Potion.damageBoost.getId(), 20, 1)); player.addPotionEffect(new PotionEffect(Potion.digSpeed.getId(), 20, 1)); player.addPotionEffect(new PotionEffect(Potion.moveSlowdown.getId(), 20, 0)); } if (isWearingEarthChest) { player.addPotionEffect(new PotionEffect(Potion.resistance.getId(), 20, 1)); player.addPotionEffect(new PotionEffect(Potion.damageBoost.getId(), 20, 1)); player.addPotionEffect(new PotionEffect(Potion.digSpeed.getId(), 20, 1)); player.addPotionEffect(new PotionEffect(Potion.moveSlowdown.getId(), 20, 0)); } } } @Override public void tickStart(EnumSet<TickType> type, Object... tickData) { if (type.equals(EnumSet.of(TickType.PLAYER))) { onPlayerTick((EntityPlayer) tickData[0]); } } @Override public void tickEnd(EnumSet<TickType> type, Object... tickData) { } @Override public EnumSet<TickType> ticks() { return EnumSet.of(TickType.PLAYER, TickType.SERVER); } @Override public String getLabel() { return null; } } -------------------------------------------------------------------------------------------------------------------------------------------------------- Armor Class (chestplate): -------------------------------------------------------------------------------------------------------------------------------------------------------- import RickyRyan.mod.MagicOres.common.MagicOres; import net.minecraft.client.renderer.texture.IconRegister; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.EnumArmorMaterial; import net.minecraft.item.ItemArmor; import net.minecraft.item.ItemStack; import net.minecraft.world.World; public class ItemGravititeChestplate extends ItemArmor { public ItemGravititeChestplate(int par1, EnumArmorMaterial par2EnumArmorMaterial, int par3, int par4) { super(par1, par2EnumArmorMaterial, par3, par4); } public void registerIcons(IconRegister iconRegister) { itemIcon = iconRegister.registerIcon("MagicOres:GravititeChestplate"); } } -------------------------------------------------------------------------------------------------------------------------------------------------------- Any help will be greatly appreciated.