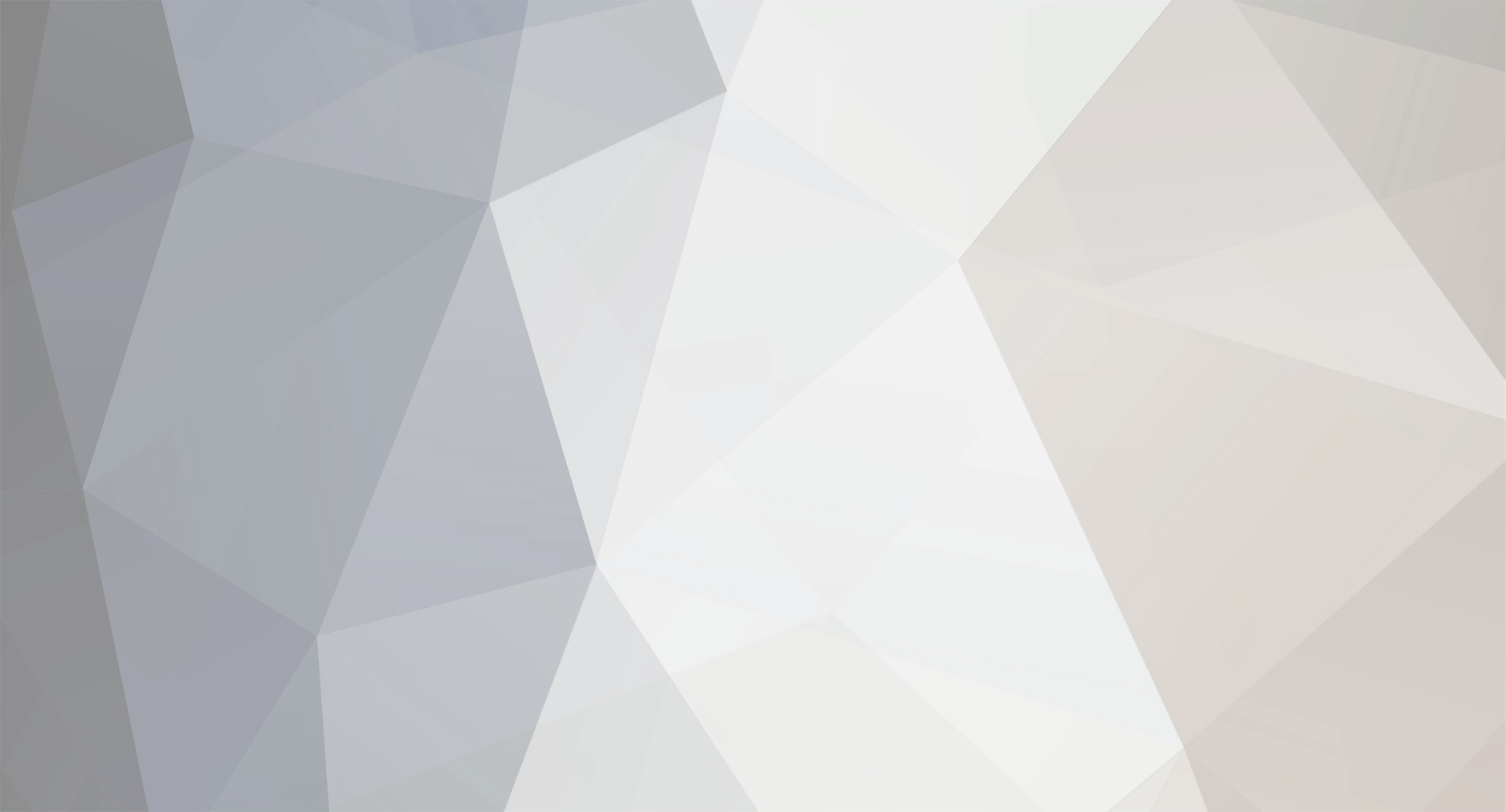
coolAlias
-
Posts
2805 -
Joined
-
Last visited
Posts posted by coolAlias
-
-
Try overriding the getDescriptionPacket() method:
@Override public Packet getDescriptionPacket() { NBTTagCompound tag = new NBTTagCompound(); this.writeToNBT(tag); return new S35PacketUpdateTileEntity(xCoord, yCoord, zCoord, 1, tag); }
-
If that's still not working the only thing I can see that is different is I save the shooter's name to NBT:
// save compound.setString("shooter", getShooter() instanceof EntityPlayer ? ((EntityPlayer) getShooter()).username : ""); // load dataWatcher.updateObject(SHOOTER_DATAWATCHER_INDEX, compound.hasKey("shooter") ? compound.getString("shooter") : "");
-
You can see how I have my lang file set up here. Note that I prefix my names with my shortened modid, which isn't really necessary, but I like it.
-
Thanks, that's all I needed to know!
-
Thanks for this! I was wondering how to go about this. Turns out you can define multiple sounds in one line:
"armorbreak": {"category": "player","sounds": ["armorbreak1", "armorbreak2", "armorbreak3", "armorbreak4"]}
and then play them with just the registered string to get a randomized sound from within. Lots of cool stuff to learn in the vanilla sounds.json file.
-
Use ISimpleBlockRenderingHandler and an ItemBlock.
-
They are still there, but kind of hard to find.
Minecraft
|__Referenced Libraries
|__forgeSrc-1.7.2-(forge version)
|__net.minecraft...
All the Forge source is in there as well.
-
Yeah, your packet / handling is not set up correctly to handle what you are trying to do, and you don't actually send any data with your packet...
props.activeSpellID=1;
That line is setting the value on the client side, when what you want to be doing is writing that data to the packet so it can be sent to the server. You will need to handle this packet separately from your other packet for extended properties, since the data it contains will be different.
-
Rather than using a stored instance of Minecraft, try getting it fresh each time with Minecraft.getMinecraft().thePlayer. I'm sure if that is the source of your problem, though - are you certain that your onActionPerformed method is even being called?
-
In 1.6.4 it was easy to add sounds using the SoundLoadEvent and SoundManager; java docs in 1.7.2 still maintain that SoundLoadEvent is a 'good place for adding your custom sounds to the SoundPool', but there doesn't seem to be any way to access the sound pool using the SoundManager.
Anyone know how it works now?
-
Also, link.
One key point that I haven't yet included in that tutorial is that you need to be aware of which event bus your events are on, so you can register your handlers appropriately. You will need a separate handler for each event bus.
TickEvents are part of cpw.mods.fml.common.gameevents, and have multiple types from which to choose (ClientTickEvent, ServerTickEvent, etc.). FML Events are registered like this:
FMLCommonHandler.instance().bus().register(new YourFMLEventHandler());
Most of the other events are in net.minecraftforge.event, as well as net.minecraftforge.client.event, and these are typically registered as follows:
MinecraftForge.EVENT_BUS.register(new YourEventHandler());
Some are registered instead to the TERRAIN_BUS, and yet others to the ORE_GEN_BUS, but most use the EVENT_BUS.
Again, it's best to have a separate handler for each type that you need, and even to split up your handler into separate ones depending on usage if you find you have a lot of events.
There doesn't seem to be any limit on the number you can register, though if you are listening to the same event in multiple handlers you will need to be extra careful that you are not conflicting with yourself.
-
That is indeed what I was looking for, thank you. It still seems odd to me that it isn't included by default in the event as a class field, as well as either the buttonstate (which can be gotten from Keyboard as well) or something similar to the tick start / tick end.
Guess I'm just spoiled by all the tools handed to us by the Forge team
-
Sounds like IExtendedEntityProperties would work well here.
-
Wherever you are spawning your arrow - e.g. onPlayerStoppedUsing or whatever.
-
If you mean HUD, then check this: http://www.minecraftforge.net/wiki/Gui_Overlay
It's a little outdated, but will work fine if you know just a little bit about what you are doing.
Here also are two tutorials on using NBT, one in an Item, and one in a Block, but you can do the same pretty much anywhere you have access to something that will allow you to save NBT:
http://www.minecraftforge.net/wiki/Item_nbt
http://www.minecraftforge.net/wiki/How_to_use_NBT_Tag_Compound
-
I'm updating my KeyBinding/Handling class using the KeyInputEvent, but the event has no fields for distinguishing which key was pressed or anything else for that matter.
Does anyone know if it's planned to implement those features in the future, like they were previously in the KeyHandler class? Because having access to the KeyBinding pressed as well as tickStart or tickEnd was extremely useful... unless I'm missing the obvious again and it's in there somewhere?
-
I register it in the ClientProxy, and yes, I really do want a render tick handler
I use it to modify the players movement more smoothly for certain effects, such as dodging, locking on to a target, etc.
EDIT: After some more looking around, I found you can use ClientTickEvent as a more specific listener, while of course still checking for the correct type. Should be good enough
EDIT 2: Derp. Just saw RenderTickEvent right under the client one... perfect!
-
You are super helpful, thank you
One more quick question: is there a way to make sure that I only listen to the RENDER type tick, other than the following? Basically what I'm getting at is before I registered my handler only on the client side, and I'm wondering if that is possible to do with the new setup.
Thanks again for sharing your knowledge!
@SubscribeEvent public void onRenderTick(TickEvent event) { if (event.type != Type.RENDER) { return; } // do stuff }
-
Title says it all; I haven't found anything comparable to that class yet in 1.7.2, does anyone happen to know how to achieve a similar functionality?
-
Thanks!!! Worked like a charm!
-
Your mod id and instance names don't match:
public static final String MODID = "ruabmmod";
@Instance("RuabmMod")
Use the modid for your instance name.
-
I get messages like the following spamming my console:
[Client thread/WARN]: Unable to play unknown soundEvent: minecraft:dig.grass
They did not download with the rest of the assets into the assets/minecraft folder. I can add them manually of course, but I'm curious why they don't get downloaded automatically.
Thanks again,
coolAlias
-
Sure:
@Override public void entityInit() { super.entityInit(); dataWatcher.addObject(SHOOTER_DATAWATCHER_INDEX, ""); }
When you create your custom arrow using this method, though, you MUST call the 'setShooter(player)' method from earlier, as that is what updates the arrow's shooter:
// should look something like this: EntityArrowCustom arrow = new EntityArrowCustom(world, player).setShooter(player);
-
Damn it, it's so obvious now that you've pointed it out... thanks!
[SOLVED][1.7.2] Adding sounds with SoundLoadEvent?
in Modder Support
Posted
I agree. I just removed my SoundLoadEvent listener entirely, given that sounds are loaded automatically from a .json file now there seems to no longer be any use for the SoundLoadEvent.