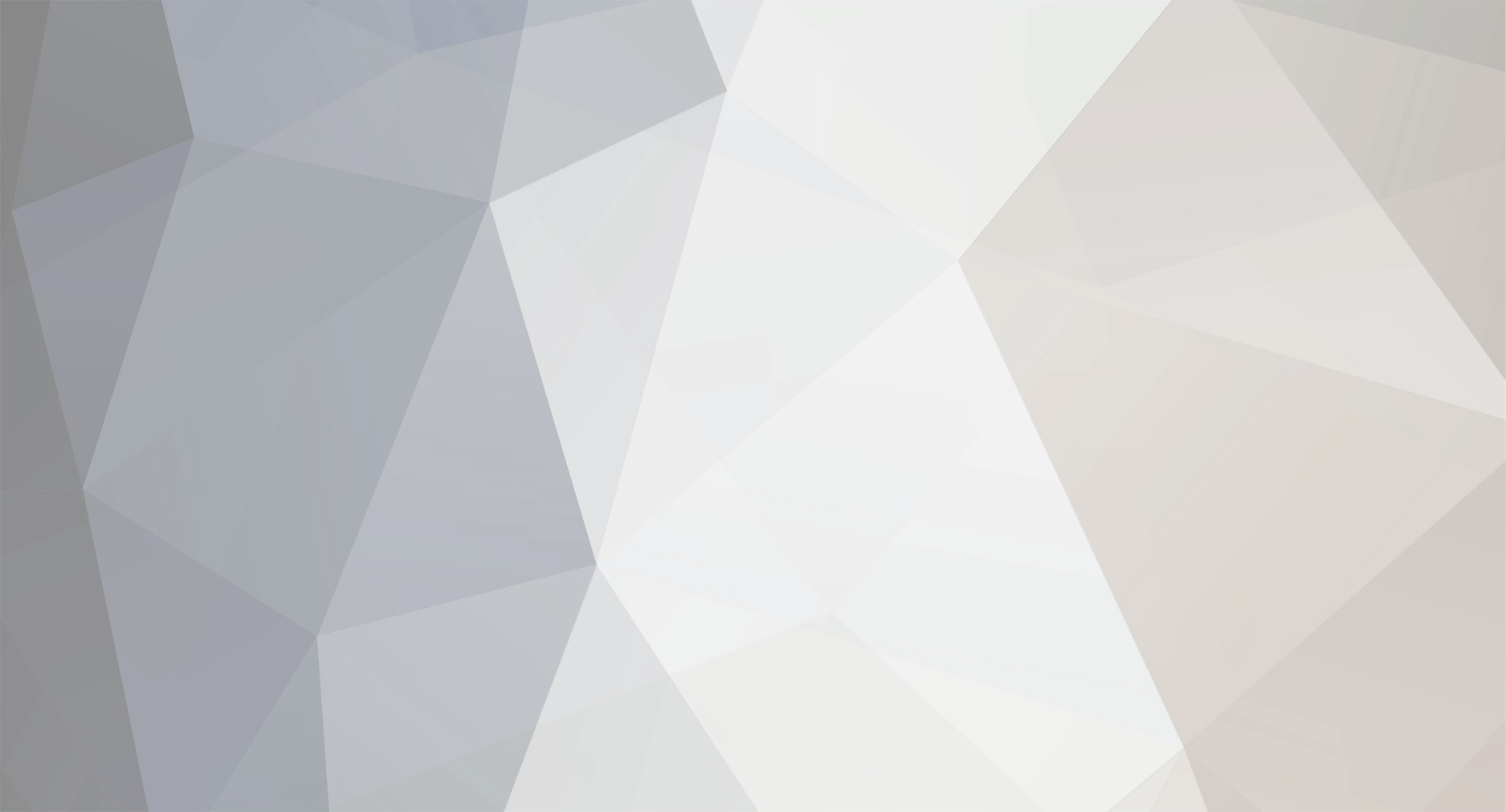
Seroe
Members-
Posts
13 -
Joined
-
Last visited
Everything posted by Seroe
-
Okay, how would I do that?
-
There is. Here is the model code: // Date: 10/27/2013 6:09:04 PM // Template version 1.1 // Java generated by Techne // Keep in mind that you still need to fill in some blanks // - ZeuX package Seroemod.Model; import net.minecraft.client.model.ModelBiped; import net.minecraft.client.model.ModelRenderer; import net.minecraft.entity.Entity; public class BasicSith extends ModelBiped { //fields ModelRenderer head; ModelRenderer body; ModelRenderer rightarm; ModelRenderer leftarm; ModelRenderer rightleg; ModelRenderer leftleg; public BasicSith() { textureWidth = 128; textureHeight = 128; head = new ModelRenderer(this, 13, 0); head.addBox(-4F, -8F, -4F, 8, 8, ; head.setRotationPoint(0F, 0F, 0F); head.setTextureSize(128, 128); head.mirror = true; setRotation(head, 0F, 0F, 0F); body = new ModelRenderer(this, 16, 38); body.addBox(-4F, 0F, -2F, 8, 12, 4); body.setRotationPoint(0F, 0F, 0F); body.setTextureSize(128, 128); body.mirror = true; setRotation(body, 0F, 0F, 0F); rightarm = new ModelRenderer(this, 40, 18); rightarm.addBox(-3F, -2F, -2F, 4, 12, 4); rightarm.setRotationPoint(-5F, 2F, 0F); rightarm.setTextureSize(128, 128); rightarm.mirror = true; setRotation(rightarm, -0.4363323F, 0F, 0F); leftarm = new ModelRenderer(this, 0, 18); leftarm.addBox(-1F, -2F, -2F, 4, 12, 4); leftarm.setRotationPoint(5F, 2F, 0F); leftarm.setTextureSize(128, 128); leftarm.mirror = true; setRotation(leftarm, 0F, 0F, 0F); rightleg = new ModelRenderer(this, 40, 60); rightleg.addBox(-2F, 0F, -2F, 4, 12, 4); rightleg.setRotationPoint(-2F, 12F, 0F); rightleg.setTextureSize(128, 128); rightleg.mirror = true; setRotation(rightleg, 0F, 0F, 0F); leftleg = new ModelRenderer(this, 0, 60); leftleg.addBox(-2F, 0F, -2F, 4, 12, 4); leftleg.setRotationPoint(2F, 12F, 0F); leftleg.setTextureSize(128, 128); leftleg.mirror = true; setRotation(leftleg, 0F, 0F, 0F); } public void render(Entity entity, float f, float f1, float f2, float f3, float f4, float f5) { super.render(entity, f, f1, f2, f3, f4, f5); setRotationAngles(f, f1, f2, f3, f4, f5); head.render(f5); body.render(f5); rightarm.render(f5); leftarm.render(f5); rightleg.render(f5); leftleg.render(f5); } private void setRotation(ModelRenderer model, float x, float y, float z) { model.rotateAngleX = x; model.rotateAngleY = y; model.rotateAngleZ = z; } public void setRotationAngles(float f, float f1, float f2, float f3, float f4, float f5) { } } Render: package Seroemod.Render; import Seroemod.SeroeMod; import Seroemod.Model.BasicSith; import Seroemod.entity.EntityBasicSith; import net.minecraft.client.model.ModelBase; import net.minecraft.client.model.ModelBiped; import net.minecraft.client.renderer.entity.Render; import net.minecraft.client.renderer.entity.RenderLiving; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLiving; import net.minecraft.item.ItemStack; import net.minecraft.util.ResourceLocation; public class RenderBasicSith extends RenderLiving { protected BasicSith model; public RenderBasicSith(ModelBiped par1ModelBiped, float par2) { super(par1ModelBiped, par2); model = ((BasicSith) mainModel); } /* public void renderBasicSith(EntityBasicSith entity, double par2, double par3, double par4, double par6, float par8, float par9) { super.doRenderLiving(entity, par2, par4, par6, par8, par9); } public void doRenderLiving(EntityLiving par1EntityLiving, double par2, double par4, double par6, float par8, float par9) { renderBasicSith((EntityBasicSith) par1EntityLiving, par2, par4, par6, par8, par9, par9); } @Override public void doRender(Entity entity, double d0, double d1, double d2, float f, float f1) { renderBasicSith((EntityBasicSith) entity, d0, d1, d2, f, f1, f1); } */ @Override protected ResourceLocation getEntityTexture(Entity entity) { // TODO Auto-generated method stub return new ResourceLocation("seroemod:textures/mobs/BasicSith.png"); } } Entity: package Seroemod.entity; import Seroemod.SeroeMod; import net.minecraft.entity.Entity; import net.minecraft.entity.EnumCreatureAttribute; import net.minecraft.entity.ai.EntityAIAttackOnCollide; import net.minecraft.entity.ai.EntityAIBreakDoor; import net.minecraft.entity.ai.EntityAIHurtByTarget; import net.minecraft.entity.ai.EntityAILookIdle; import net.minecraft.entity.ai.EntityAINearestAttackableTarget; import net.minecraft.entity.ai.EntityAISwimming; import net.minecraft.entity.ai.EntityAIWander; import net.minecraft.entity.ai.EntityAIWatchClosest; import net.minecraft.entity.monster.EntityMob; import net.minecraft.entity.monster.EntityPigZombie; import net.minecraft.entity.monster.EntityZombie; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.potion.Potion; import net.minecraft.potion.PotionEffect; import net.minecraft.util.MathHelper; import net.minecraft.world.World; public class EntityBasicSith extends EntityMob { public EntityBasicSith(World par1World) { super(par1World); this.experienceValue = 10; this.getNavigator().setBreakDoors(true); this.tasks.addTask(0, new EntityAISwimming(this)); this.tasks.addTask(1, new EntityAIBreakDoor(this)); this.tasks.addTask(6, new EntityAIWander(this, 0.4D)); this.tasks.addTask(7, new EntityAIWatchClosest(this, EntityPlayer.class, 8.0F)); this.tasks.addTask(7, new EntityAILookIdle(this)); this.targetTasks.addTask(1, new EntityAIHurtByTarget(this, true)); this.targetTasks.addTask(2, new EntityAINearestAttackableTarget(this, EntityPlayer.class, 0, true)); this.tasks.addTask(2, new EntityAIAttackOnCollide(this, EntityPlayer.class, 0.5D, false)); } protected boolean isAIEnabled() { return true; } protected String getHurtSound() { return "mob.zombie.hurt"; } protected String getDeathSound() { return "damage.hit"; } protected void playStepSound(int par1, int par2, int par3, int par4) { this.playSound("mob.zombie.step", 0.15F, 1.0F); } protected int getDropItemId() { return Item.bone.itemID; } protected void dropRareDrop(int par1) { this.dropItem(SeroeMod.hiltRedLightsaber.itemID, 1); } public EnumCreatureAttribute getCreatureAttribute() { return EnumCreatureAttribute.UNDEAD; } @Override protected void addRandomArmor() { super.addRandomArmor(); this.setCurrentItemOrArmor(0, new ItemStack(SeroeMod.redLightsaber)); } public void onLivingUpdate(Entity entity) { if (this.worldObj.isDaytime() && !this.worldObj.isRemote && !this.isChild()) { float f = this.getBrightness(1.0F); EntityBasicSith Player = (EntityBasicSith) entity; Player.addPotionEffect((new PotionEffect(Potion.moveSpeed.getId(), 0, 0, true))); Player.addPotionEffect((new PotionEffect(Potion.jump.getId(), 0, 1, true))); if (f > 0.5F && this.rand.nextFloat() * 30.0F < (f - 0.4F) * 2.0F && this.worldObj.canBlockSeeTheSky(MathHelper.floor_double(this.posX), MathHelper.floor_double(this.posY), MathHelper.floor_double(this.posZ))) { boolean flag = true; ItemStack itemstack = this.getCurrentItemOrArmor(1); if (itemstack != null) { if (itemstack.isItemStackDamageable()) { itemstack.setItemDamage(itemstack.getItemDamageForDisplay() + this.rand.nextInt(2)); if (itemstack.getItemDamageForDisplay() >= itemstack.getMaxDamage()) { this.renderBrokenItemStack(itemstack); this.setCurrentItemOrArmor(4, (ItemStack)null); } } flag = false; } } } super.onLivingUpdate(); } @Override protected Entity findPlayerToAttack() { EntityPlayer entityplayer = this.worldObj.getClosestVulnerablePlayerToEntity(this, 30.0D); return entityplayer != null && this.canEntityBeSeen(entityplayer) ? entityplayer : null; } } Also--in my entity code, I have the following line: @Override protected void addRandomArmor() { super.addRandomArmor(); this.setCurrentItemOrArmor(0, new ItemStack(SeroeMod.redLightsaber)); } This, in theory, should place a lightsaber in the mob's hand. Why does it not work?
-
Update: Got to texture to work
-
Alright, I changed the model and when I extend ModelBiped, this is the result.
-
Lol, after deleting all the methods besides the texture location, the mob rendered somewhat correctly. Now when I use the spawn egg, the mob appears on the ground.
-
Hello: I'm trying to a new mob, the BasicSith, to my mod. I've followed a tutorial and coded almost the exact same way, but I am getting errors that the tutorial maker is not. Here is my main class: (I apologize for the length, and for the fact that I don't know how to use the spoiler thing) package Seroemod; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityEggInfo; import net.minecraft.entity.EntityList; import net.minecraft.entity.EnumCreatureType; import net.minecraft.item.EnumArmorMaterial; import net.minecraft.item.EnumToolMaterial; import net.minecraft.item.Item; import net.minecraft.item.ItemDye; import net.minecraft.item.ItemStack; import net.minecraftforge.common.EnumHelper; import net.minecraftforge.common.MinecraftForge; import Seroemod.Armor.Armor; import Seroemod.Blocks.SapphireBlock; import Seroemod.Bow.SapphireArrow; import Seroemod.Item.SapphireGem; import Seroemod.Item.SapphireShard; import Seroemod.Lightsabers.BlueKyber; import Seroemod.Lightsabers.BlueLightsaber; import Seroemod.Lightsabers.BlueLightsaberHilt; import Seroemod.Lightsabers.GreenKyber; import Seroemod.Lightsabers.GreenLightsaber; import Seroemod.Lightsabers.GreenLightsaberHilt; import Seroemod.Lightsabers.LightsaberHilt; import Seroemod.Lightsabers.PurpleKyber; import Seroemod.Lightsabers.PurpleLightsaber; import Seroemod.Lightsabers.PurpleLightsaberHilt; import Seroemod.Lightsabers.RedKyber; import Seroemod.Lightsabers.RedLightsaber; import Seroemod.Lightsabers.RedLightsaberHilt; import Seroemod.Model.BasicSith; import Seroemod.Ore.SapphireOre; import Seroemod.Ore.SapphireWorldGeneration; import Seroemod.Render.RenderBasicSith; import Seroemod.Tools.ToolSapphireAxe; import Seroemod.Tools.ToolSapphireHoe; import Seroemod.Tools.ToolSapphirePickaxe; import Seroemod.Tools.ToolSapphireShovel; import Seroemod.Tools.ToolSapphireSword; import Seroemod.entity.EntityBasicSith; import cpw.mods.fml.client.registry.RenderingRegistry; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.EventHandler; import cpw.mods.fml.common.event.FMLLoadEvent; import cpw.mods.fml.common.event.FMLPostInitializationEvent; import cpw.mods.fml.common.event.FMLPreInitializationEvent; import cpw.mods.fml.common.event.FMLServerStartingEvent; import cpw.mods.fml.common.network.NetworkMod; import cpw.mods.fml.common.registry.EntityRegistry; import cpw.mods.fml.common.registry.GameRegistry; import cpw.mods.fml.common.registry.LanguageRegistry; @Mod(modid = "seroemod", name = "Seroe's Mod", version = "0.1") @NetworkMod(clientSideRequired = true, serverSideRequired = false) public class SeroeMod { // Mob IDs static int startEntityId = 300; // === Mob Generation === // public static int getUniqueEntityId() { do { startEntityId++; } while(EntityList.getStringFromID(startEntityId) != null); return startEntityId++; } public static void registerEntityEgg(Class<? extends Entity> entity, int primaryColor, int secondaryColor) { int id = getUniqueEntityId(); EntityList.IDtoClassMapping.put(id, entity); EntityList.entityEggs.put(id, new EntityEggInfo(id, primaryColor, secondaryColor)); } // === Blocks === // // Block Section public static Block sapphireBlock, sapphireOre, amethystOre; // Block IDs int sapphireBlockID = 3000; int sapphireOreBlockID = 3001; int amethystOreBlockID = 3002; // === Items === /// // Item Section // Sapphire // Sapphire public static Item sapphireGem, sapphireShard, amethystGem, sapphireSword, sapphireShovel, sapphireAxe, sapphirePickaxe, sapphireHoe; // Lightsaber public static Item hiltLightsaber, blueLightsaber, hiltBlueLightsaber, greenLightsaber, hiltGreenLightsaber, purpleLightsaber, hiltPurpleLightsaber, redLightsaber, hiltRedLightsaber, redKyber, blueKyber, greenKyber, purpleKyber; // Arrow public static Item sapphireArrow; // Armor public static Item sapphireHelmet; public static Item sapphireChest; public static Item sapphireLeggings; public static Item sapphireBoots; // Materials public static EnumToolMaterial Sapphire = EnumHelper.addToolMaterial("Sapphire", 2, 1033, 7.5F, 2.5F, 20); public static EnumToolMaterial Lightsaber = EnumHelper.addToolMaterial("Lightsaber", 0, 200, 0.0F, 3.5F, 1); public static EnumToolMaterial Hilt = EnumHelper.addToolMaterial("Hilt", Lightsaber.getMaxUses(), 0, 0.0F, 0.0F, 1); public static EnumArmorMaterial SapphireArmor = EnumHelper.addArmorMaterial("SapphireArmor", 21, new int[] { 4, 10, 4, 3 }, 5); // Item IDs static int sapphireGemID = 4000 - 256; static int sapphireShardID = 4001 - 256; static int amethystGemID = 4002 - 256; static int sapphireSwordID = 4003 - 256; static int sapphireShovelID = 4004 - 256; static int sapphireAxeID = 4005 - 256; static int sapphirePickaxeID = 4006 - 256; static int sapphireHoeID = 4007 - 256; static int hiltLightsaberID = 4008 - 256; static int blueLightsaberID = 4009 - 256; static int hiltBlueLightsaberID = 4010 - 256; static int sapphireArrowID = 4011 - 256; static int greenLightsaberID = 4012 - 256; static int hiltGreenLightsaberID = 4013 - 256; static int purpleLightsaberID = 4014 - 256; static int hiltPurpleLightsaberID = 4015 - 256; static int redLightsaberID = 4016 - 256; static int hiltRedLightsaberID = 4017 - 256; static int blueKyberID = 4018 - 256; static int greenKyberID = 4019 - 256; static int purpleKyberID = 4020 - 256; static int redKyberID = 4021 - 256; static int sapphireHelmetID = 4022 - 256; static int sapphireChestID = 4023 - 256; static int sapphireLeggingsID = 4024 - 256; static int sapphireBootsID = 4025 - 256; // Instance of our mod public static SeroeMod instance; @EventHandler public void preInit(FMLPreInitializationEvent event) // Contains Block inits, Item inits, Tool inits, World Gen { // Block/Item Registry piRegistry(); // Recipes piRecipes(); // === Sounds === // MinecraftForge.EVENT_BUS.register(new SeroeModSound()); // === World Generation === // GameRegistry.registerWorldGenerator(new SapphireWorldGeneration()); // === Entity Registry === // EntityRegistry.registerGlobalEntityID(EntityBasicSith.class, "Basic Sith", 1); EntityRegistry.addSpawn(EntityBasicSith.class, 15, 5, 10, EnumCreatureType.monster); EntityRegistry.findGlobalUniqueEntityId(); registerEntityEgg(EntityBasicSith.class, 0x3c786c, 0xb50000); RenderingRegistry.registerEntityRenderingHandler(EntityBasicSith.class, new RenderBasicSith (new BasicSith(), 0.3F)); } @EventHandler public void load(FMLLoadEvent event) // Contains itemstacks and recipes { } @EventHandler public void postInit(FMLPostInitializationEvent event) { } @EventHandler public void serverStart(FMLServerStartingEvent event) { } public void piRegistry() { // ==== Blocks ==== // // Sapphire Block Stuff this.sapphireBlock = new SapphireBlock(sapphireBlockID, Material.rock); LanguageRegistry.addName(sapphireBlock, "Sapphire Block"); MinecraftForge.setBlockHarvestLevel(sapphireBlock, "pickaxe", 2); GameRegistry.registerBlock(sapphireBlock, "sapphireBlock"); // Sapphire Ore Stuff this.sapphireOre = new SapphireOre(sapphireOreBlockID); LanguageRegistry.addName(sapphireOre, "Sapphire Ore"); MinecraftForge.setBlockHarvestLevel(sapphireOre, "pickaxe", 2); GameRegistry.registerBlock(sapphireOre, "sapphireOre"); // === Items === // // Sapphire Gem Stuff sapphireGem = new SapphireGem(sapphireGemID); LanguageRegistry.addName(sapphireGem, "Sapphire Gem"); // Sapphire Shard Stuff sapphireShard = new SapphireShard(sapphireShardID); LanguageRegistry.addName(sapphireShard, "Sapphire Shard"); // Sapphire Sword sapphireSword = new ToolSapphireSword(sapphireSwordID, Sapphire); GameRegistry.registerItem(sapphireSword, "sapphireSword"); LanguageRegistry.addName(sapphireSword, "Sapphire Sword"); // Sapphire Axe sapphireAxe = new ToolSapphireAxe(sapphireAxeID, Sapphire); GameRegistry.registerItem(sapphireAxe, "sapphireAxe"); LanguageRegistry.addName(sapphireAxe, "Sapphire Axe"); // Sapphire Hoe sapphireHoe = new ToolSapphireHoe(sapphireHoeID, Sapphire); GameRegistry.registerItem(sapphireHoe, "sapphireHoe"); LanguageRegistry.addName(sapphireHoe, "Sapphire Hoe"); // Sapphire Shovel sapphireShovel = new ToolSapphireShovel(sapphireShovelID, Sapphire); GameRegistry.registerItem(sapphireShovel, "sapphireShovel"); LanguageRegistry.addName(sapphireShovel, "Sapphire Shovel"); // Sapphire Pickaxe sapphirePickaxe = new ToolSapphirePickaxe(sapphirePickaxeID, Sapphire); GameRegistry.registerItem(sapphirePickaxe, "sapphirePickaxe"); LanguageRegistry.addName(sapphirePickaxe, "Sapphire Pickaxe"); // Lightsaber Hilt hiltLightsaber = new LightsaberHilt(hiltLightsaberID); LanguageRegistry.addName(hiltLightsaber, "Lightsaber Hilt"); // Blue Lightsaber blueLightsaber = new BlueLightsaber(blueLightsaberID, Lightsaber); GameRegistry.registerItem(blueLightsaber, "blueLightsaber"); LanguageRegistry.addName(blueLightsaber, "Blue Lightsaber"); // Blue Lightsaber Hilt hiltBlueLightsaber = new BlueLightsaberHilt(hiltBlueLightsaberID, Hilt); LanguageRegistry.addName(hiltBlueLightsaber, "Blue Lightsaber Hilt"); // Green Lightsaber greenLightsaber = new GreenLightsaber(greenLightsaberID, Lightsaber); GameRegistry.registerItem(greenLightsaber, "greenLightsaber"); LanguageRegistry.addName(greenLightsaber, "Green Lightsaber"); // Green Lightsaber Hilt hiltGreenLightsaber = new GreenLightsaberHilt(hiltGreenLightsaberID, Hilt); LanguageRegistry.addName(hiltGreenLightsaber, "Green Lightsaber Hilt"); // Purple Lightsaber purpleLightsaber = new PurpleLightsaber(purpleLightsaberID, Lightsaber); GameRegistry.registerItem(purpleLightsaber, "PurpleLightsaber"); LanguageRegistry.addName(purpleLightsaber, "Purple Lightsaber"); // Purple Lightsaber Hilt hiltPurpleLightsaber = new PurpleLightsaberHilt(hiltPurpleLightsaberID, Hilt); LanguageRegistry.addName(hiltPurpleLightsaber, "Purple Lightsaber Hilt"); // Red Lightsaber redLightsaber = new RedLightsaber(redLightsaberID, Lightsaber); GameRegistry.registerItem(redLightsaber, "redLightsaber"); LanguageRegistry.addName(redLightsaber, "Red Lightsaber"); // Red Lightsaber Hilt hiltRedLightsaber = new RedLightsaberHilt(hiltRedLightsaberID, Hilt); LanguageRegistry.addName(hiltRedLightsaber, "Red Lightsaber Hilt"); // Blue Kyber blueKyber = new BlueKyber(blueKyberID); LanguageRegistry.addName(blueKyber, "Blue Kyber Crystal"); // Green Kyber greenKyber = new GreenKyber(greenKyberID); LanguageRegistry.addName(greenKyber, "Green Kyber Crystal"); // Purple Kyber purpleKyber = new PurpleKyber(purpleKyberID); LanguageRegistry.addName(purpleKyber, "Purple Kyber Crystal"); // Red Kyber redKyber = new RedKyber(redKyberID); LanguageRegistry.addName(redKyber, "Red Kyber Crystal"); // Arrow sapphireArrow = new SapphireArrow(sapphireArrowID); LanguageRegistry.addName(sapphireArrow, "Sapphire Arrow"); // Sapphire Armor sapphireHelmet = new Armor(sapphireHelmetID, SapphireArmor, 0, 0, "Sapphire"); sapphireChest = new Armor(sapphireChestID, SapphireArmor, 0, 1, "Sapphire"); sapphireLeggings = new Armor(sapphireLeggingsID, SapphireArmor, 0, 2, "Sapphire"); sapphireBoots = new Armor(sapphireBootsID, SapphireArmor, 0, 3, "Sapphire"); LanguageRegistry.addName(sapphireHelmet, "Sapphire Helmet"); LanguageRegistry.addName(sapphireChest, "Sapphire Chestplate"); LanguageRegistry.addName(sapphireLeggings, "Sapphire Leggings"); LanguageRegistry.addName(sapphireBoots, "Sapphire Boots"); } public void piRecipes() { // === RECIPES === // // Item Stacks ItemStack sapphireStack = new ItemStack(sapphireGem, 9); ItemStack sapphireShardStack = new ItemStack(sapphireShard, 9); // === Shaped Crafting Recipes === // // Sapphire Block GameRegistry.addRecipe(new ItemStack(sapphireBlock), "xxx", "xxx", "xxx", 'x', sapphireGem ); // Sapphire Gem GameRegistry.addRecipe(new ItemStack(sapphireGem), "xxx", "xxx", "xxx", 'x', sapphireShard ); // Sapphire Sword GameRegistry.addRecipe(new ItemStack(sapphireSword), " x ", " x ", " y ", 'x', sapphireGem, 'y', Item.stick ); // Sapphire Axe 1 GameRegistry.addRecipe(new ItemStack(sapphireAxe), "xx ", "xy ", " y ", 'x', sapphireGem, 'y', Item.stick ); // Sapphire Axe 2 GameRegistry.addRecipe(new ItemStack(sapphireAxe), " xx", " yx", " y ", 'x', sapphireGem, 'y', Item.stick ); // Sapphire Hoe 1 GameRegistry.addRecipe(new ItemStack(sapphireHoe), "xx ", " y ", " y ", 'x', sapphireGem, 'y', Item.stick ); // Sapphire Hoe 2 GameRegistry.addRecipe(new ItemStack(sapphireHoe), " xx", " y ", " y ", 'x', sapphireGem, 'y', Item.stick ); // Sapphire Shovel GameRegistry.addRecipe(new ItemStack(sapphireShovel), " x ", " y ", " y ", 'y', Item.stick, 'x', sapphireGem ); // Sapphire Pickaxe GameRegistry.addRecipe(new ItemStack(sapphirePickaxe), "xxx", " y ", " y ", 'x', sapphireGem, 'y', Item.stick ); // Lightsaber Hilt GameRegistry.addRecipe(new ItemStack(hiltLightsaber), " x ", " y ", " z ", 'x', Item.diamond, 'y', Item.redstone, 'z', Item.ingotIron ); // Blue Kyber GameRegistry.addRecipe(new ItemStack(blueKyber), " x ", "xyx", " x ", 'x', sapphireShard, 'y', sapphireGem ); // Green Kyber GameRegistry.addRecipe(new ItemStack(greenKyber), " x ", "xyx", " x ", 'x', Item.emerald, 'y', Block.blockEmerald ); // Purple Kyber GameRegistry.addRecipe(new ItemStack(purpleKyber), " x ", "zyz", " x ", 'x', Item.diamond, 'y', sapphireGem, 'z', Item.redstone ); // Red Kyber GameRegistry.addRecipe(new ItemStack(redKyber), " x ", "zyz", " x ", 'x', Item.redstone, 'y', Block.blockRedstone, 'z', Item.ingotIron ); // Blue Lightsaber Hilt GameRegistry.addRecipe(new ItemStack(hiltBlueLightsaber), " x ", " x ", " z ", 'x', blueKyber, 'z', hiltLightsaber ); // Green Lightsaber Hilt GameRegistry.addRecipe(new ItemStack(hiltGreenLightsaber), " x ", " x ", " z ", 'x', greenKyber, 'z', hiltLightsaber ); // Purple Lightsaber Hilt GameRegistry.addRecipe(new ItemStack(hiltPurpleLightsaber), " x ", " x ", " z ", 'x', purpleKyber, 'z', hiltLightsaber ); // Red Lightsaber Hilt GameRegistry.addRecipe(new ItemStack(hiltRedLightsaber), " x ", " x ", " z ", 'x', redKyber, 'z', hiltLightsaber ); // Sapphire Helmet GameRegistry.addRecipe(new ItemStack(sapphireHelmet), "xxx", "x x", 'x', sapphireGem ); // Sapphire Chestplate GameRegistry.addRecipe(new ItemStack(sapphireChest), "x x", "xxx", "xxx", 'x', sapphireGem ); // Sapphire Leggings GameRegistry.addRecipe(new ItemStack(sapphireLeggings), "xxx", "x x", "x x", 'x', sapphireGem ); // Sapphire Boots GameRegistry.addRecipe(new ItemStack(sapphireBoots), " ", "x x", "x x", 'x', sapphireGem ); // === Shapeless Crafting Recipes === // // Sapphires GameRegistry.addShapelessRecipe(sapphireStack, sapphireBlock); // Sapphire Shards GameRegistry.addShapelessRecipe(sapphireShardStack, sapphireGem); } } Model // Date: 10/22/2013 7:56:54 PM // Template version 1.1 // Java generated by Techne // Keep in mind that you still need to fill in some blanks // - ZeuX package Seroemod.Model; import net.minecraft.client.model.ModelBase; import net.minecraft.client.model.ModelRenderer; import net.minecraft.entity.Entity; public class BasicSith extends ModelBase { //fields ModelRenderer Body; ModelRenderer Right_Leg; ModelRenderer Left_Leg_; ModelRenderer Right_Arm; ModelRenderer Left_Arm; ModelRenderer Head; public BasicSith() { textureWidth = 64; textureHeight = 32; Body = new ModelRenderer(this, 40, 0); Body.addBox(0F, 0F, 0F, 8, 12, 4); Body.setRotationPoint(0F, 0F, 0F); Body.setTextureSize(64, 32); Body.mirror = true; setRotation(Body, 0F, 0F, 0F); Right_Leg = new ModelRenderer(this, 48, 16); Right_Leg.addBox(-2F, 0F, -2F, 4, 12, 4); Right_Leg.setRotationPoint(2F, 12F, 2F); Right_Leg.setTextureSize(64, 32); Right_Leg.mirror = true; setRotation(Right_Leg, 0F, 0F, 0F); Left_Leg_ = new ModelRenderer(this, 32, 16); Left_Leg_.addBox(-2F, 0F, -2F, 4, 12, 4); Left_Leg_.setRotationPoint(6F, 12F, 2F); Left_Leg_.setTextureSize(64, 32); Left_Leg_.mirror = true; setRotation(Left_Leg_, 0F, 0F, 0F); Right_Arm = new ModelRenderer(this, 16, 16); Right_Arm.addBox(-4F, -2F, -2F, 4, 12, 4); Right_Arm.setRotationPoint(0F, 2F, 2F); Right_Arm.setTextureSize(64, 32); Right_Arm.mirror = true; setRotation(Right_Arm, 0F, 0F, 0F); Left_Arm = new ModelRenderer(this, 0, 16); Left_Arm.addBox(0F, -2F, -2F, 4, 12, 4); Left_Arm.setRotationPoint(8F, 2F, 2F); Left_Arm.setTextureSize(64, 32); Left_Arm.mirror = true; setRotation(Left_Arm, 0F, 0F, 0F); Head = new ModelRenderer(this, 0, 0); Head.addBox(-4F, -8F, -4F, 8, 8, ; Head.setRotationPoint(4F, 0F, 2F); Head.setTextureSize(64, 32); Head.mirror = true; setRotation(Head, 0F, 0F, 0F); } public void render(Entity entity, float f, float f1, float f2, float f3, float f4, float f5) { super.render(entity, f, f1, f2, f3, f4, f5); setRotationAngles(f, f1, f2, f3, f4, f5, entity); Body.render(f5); Right_Leg.render(f5); Left_Leg_.render(f5); Right_Arm.render(f5); Left_Arm.render(f5); Head.render(f5); } private void setRotation(ModelRenderer model, float x, float y, float z) { model.rotateAngleX = x; model.rotateAngleY = y; model.rotateAngleZ = z; } public void setRotationAngles(float f, float f1, float f2, float f3, float f4, float f5, Entity par6Entity) { } } Entity package Seroemod.entity; import Seroemod.SeroeMod; import net.minecraft.entity.EnumCreatureAttribute; import net.minecraft.entity.ai.EntityAIAttackOnCollide; import net.minecraft.entity.ai.EntityAIBreakDoor; import net.minecraft.entity.ai.EntityAIHurtByTarget; import net.minecraft.entity.ai.EntityAILookIdle; import net.minecraft.entity.ai.EntityAINearestAttackableTarget; import net.minecraft.entity.ai.EntityAISwimming; import net.minecraft.entity.ai.EntityAIWander; import net.minecraft.entity.ai.EntityAIWatchClosest; import net.minecraft.entity.monster.EntityMob; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.util.MathHelper; import net.minecraft.world.World; public class EntityBasicSith extends EntityMob { public ItemStack getHeldItem() { return defaultHeldItem; } private static final ItemStack defaultHeldItem; static { defaultHeldItem = new ItemStack(SeroeMod.redLightsaber, 1); } public EntityBasicSith(World par1World) { super(par1World); this.experienceValue = 10; this.getNavigator().setBreakDoors(true); this.tasks.addTask(0, new EntityAISwimming(this)); this.tasks.addTask(1, new EntityAIBreakDoor(this)); this.tasks.addTask(6, new EntityAIWander(this, 0.5D)); this.tasks.addTask(7, new EntityAIWatchClosest(this, EntityPlayer.class, 8.0F)); this.tasks.addTask(7, new EntityAILookIdle(this)); this.targetTasks.addTask(1, new EntityAIHurtByTarget(this, true)); this.targetTasks.addTask(2, new EntityAINearestAttackableTarget(this, EntityPlayer.class, 0, true)); this.tasks.addTask(2, new EntityAIAttackOnCollide(this, EntityPlayer.class, 0.5D, false)); } protected boolean isAIEnabled() { return true; } protected String getHurtSound() { return "mob.zombie.hurt"; } protected String getDeathSound() { return "damage.hit"; } protected void playStepSound(int par1, int par2, int par3, int par4) { this.playSound("mob.zombie.step", 0.15F, 1.0F); } protected int getDropItemId() { return Item.bone.itemID; } protected void dropRareDrop(int par1) { switch (this.rand.nextInt(3)) { case 0: this.dropItem(SeroeMod.hiltRedLightsaber.itemID, 1); break; } } public EnumCreatureAttribute getCreatureAttribute() { return EnumCreatureAttribute.UNDEAD; } public void onLivingUpdate() { if (this.worldObj.isDaytime() && !this.worldObj.isRemote && !this.isChild()) { float f = this.getBrightness(1.0F); if (f > 0.5F && this.rand.nextFloat() * 30.0F < (f - 0.4F) * 2.0F && this.worldObj.canBlockSeeTheSky(MathHelper.floor_double(this.posX), MathHelper.floor_double(this.posY), MathHelper.floor_double(this.posZ))) { boolean flag = true; ItemStack itemstack = this.getCurrentItemOrArmor(4); if (itemstack != null) { if (itemstack.isItemStackDamageable()) { itemstack.setItemDamage(itemstack.getItemDamageForDisplay() + this.rand.nextInt(2)); if (itemstack.getItemDamageForDisplay() >= itemstack.getMaxDamage()) { this.renderBrokenItemStack(itemstack); this.setCurrentItemOrArmor(4, (ItemStack)null); } } flag = false; } } } super.onLivingUpdate(); } } Render package Seroemod.Render; import Seroemod.Model.BasicSith; import Seroemod.entity.EntityBasicSith; import net.minecraft.client.model.ModelBase; import net.minecraft.client.renderer.entity.Render; import net.minecraft.client.renderer.entity.RenderLiving; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLiving; import net.minecraft.util.ResourceLocation; public class RenderBasicSith extends RenderLiving { protected BasicSith model; public RenderBasicSith(ModelBase par1ModelBase, float par2) { super(par1ModelBase, par2); model = ((BasicSith) mainModel); } public void renderBasicSith(EntityBasicSith entity, double par2, double par3, double par4, double par6, float par8, float par9) { super.doRenderLiving(entity, par2, par4, par6, par8, par9); } public void doRenderLiving(EntityLiving par1EntityLiving, double par2, double par4, double par6, float par8, float par9) { renderBasicSith((EntityBasicSith) par1EntityLiving, par2, par4, par6, par8, par9); // ERROR HERE } @Override public void doRender(Entity entity, double d0, double d1, double d2, float f, float f1) { renderBasicSith((EntityBasicSith) entity, d0, d1, d2, f, f1); // ERROR HERE ^ } @Override protected ResourceLocation getEntityTexture(Entity entity) { // TODO Auto-generated method stub return new ResourceLocation("seroemod:textures/mobs/test.png"); } } However this is the result. The mobs appear to be a circular shadow on the group, and then become a pink and black shape when they fly into the air (they aren't suppose to fly). Help?
-
Hello I am trying to add a potion effect when my item is held that lasts until the player selects a different item. public void onUpdate(ItemStack par1ItemStack, World par2World, Entity par3Entity, int par4, boolean par5, EntityPlayer par6) { if(par6.getCurrentEquippedItem().equals(getUnlocalizedName())) { par6.addPotionEffect(new PotionEffect(Potion.moveSpeed.id, 100, 3, true)); } else { par6.curePotionEffects(par1ItemStack); } } For some reason this doesn't work. How can I get it to work? Here is the link to the full item's class: http://pastebin.com/R3EHXvif Thanks in advance for the help.
-
Why does Minecraft crash when I add this line of code?
Seroe replied to Seroe's topic in Modder Support
Okay, I fixed that. I also want to add durability to my item, so I extended ItemSword. When you have 2 open lightsabers in your inventory, damage applies to both of them. How might one fix that? Also, the lightsaber now will not close when it has damage. Also follow this link to see the update of my situation: http://www.minecraftforum.net/topic/2045112-why-does-minecraft-crash-when-i-add-this-line-of-code/ -
Whenever I add this line of code: ItemStack hilt = new ItemStack(SeroeMod.hiltBlueLightsaber); Minecraft won't start. Here is the link to the error: http://pastebin.com/EgxhkeHd Here is the link to my main class: http://pastebin.com/3xbeLpQ5 Here is a link to my hiltBlueLightsaber class: http://pastebin.com/YaCwndfC Here is a link to my BlueLightsaber class, where I have the "ItemStack hilt = new ItemStack(SeroeMod.hiltBlueLightsaber);" code: http://pastebin.com/5CDC8v1B Also, in my BlueLightsaber class, why doesn't this code work: @Override public boolean onItemUse(ItemStack par1ItemStack, EntityPlayer par2EntityPlayer, World par3World, int par4, int par5, int par6, int par7, float par8, float par9, float par10) { par3World.playSoundAtEntity(par2EntityPlayer, "seroemod:swing", 500.0F, 1.0F); return true; } My intention is to play a sound every time the user left-clicks. Thanks in advance
-
Okay, makes sense... But what exactly do I enter to do that? List of commands I have tried: chmod +x (dragged in install.sh) sh (dragged in install.sh) python (dragged in install.py) (dragged in install.sh) None of these have yielded a satisfying result.
-
Hello. I installed Minecraft forge on Eclipse. (I posted my process here http://www.minecraftforum.net/topic/2008856-why-does-installing-minecraftforge-to-eclipse-work-differently-for-me/). I am getting an error when I try to import net.minecraftforge.common.MinecraftForge. Why is this?