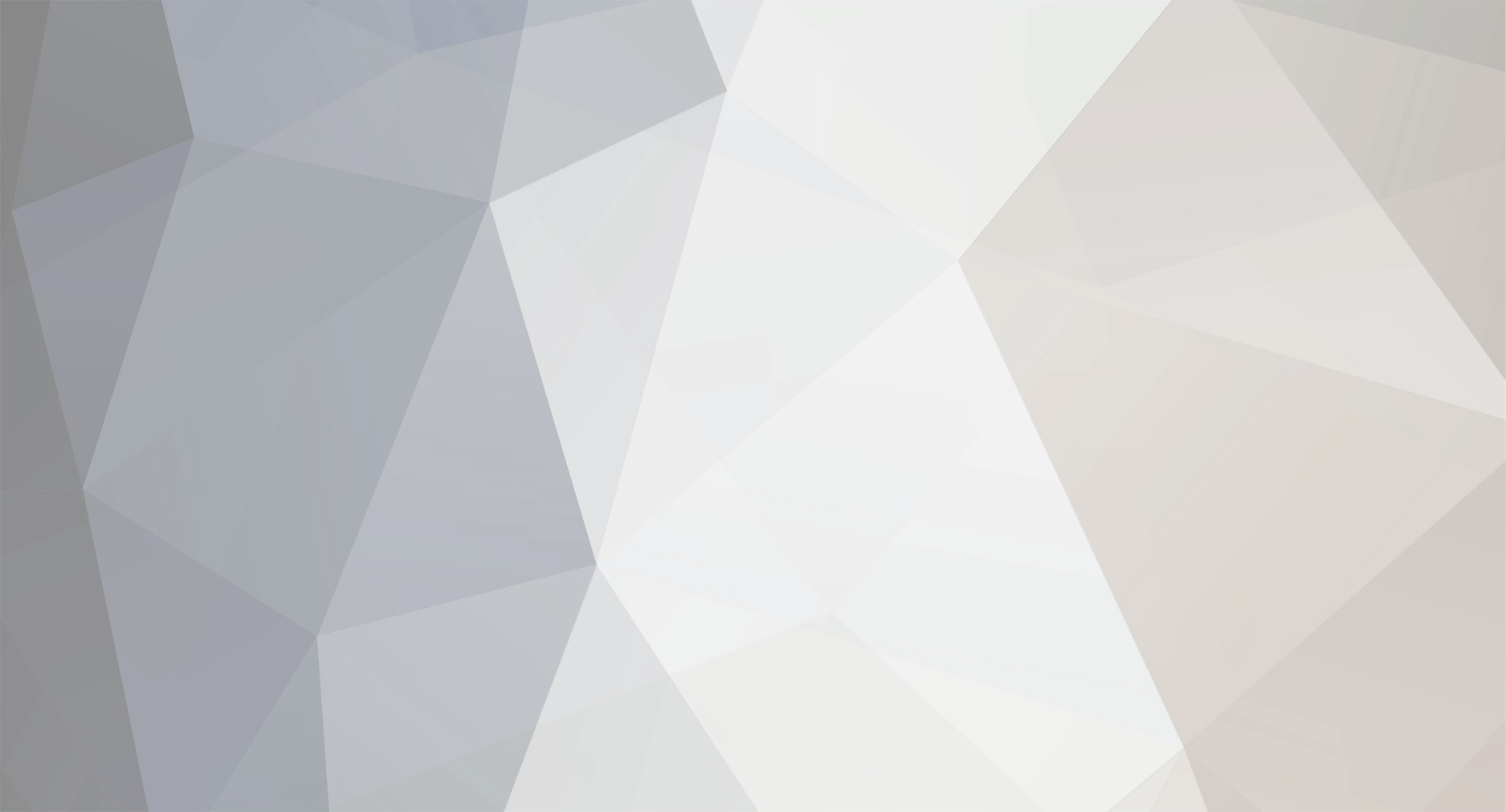
Codemeister88
Members-
Posts
11 -
Joined
-
Last visited
Everything posted by Codemeister88
-
[SOLVED] Modifying Player Movement
Codemeister88 replied to insomniac10102's topic in Modder Support
This is code I did for setting a player to a specific location. Is this what you are looking for? if (!par2World.isRemote) { ChunkCoordinates bedCoords = par3EntityPlayer.getBedLocation(0); if(bedCoords != null) { if(par3EntityPlayer instanceof EntityPlayerMP) { ((EntityPlayerMP)par3EntityPlayer).playerNetServerHandler.setPlayerLocation(bedCoords.posX, bedCoords.posY, bedCoords.posZ, par3EntityPlayer.rotationYaw, par3EntityPlayer.rotationPitch); } } else { par3EntityPlayer.addChatMessage("You haven't slept in a bed."); } } -
So actually it appears that it is working...it spawns the entity; however the spawn location is clear up in the clouds. Inside TileEntityItemLaster.java: Change: entity.setPosition(xCoord, yCoord + i, zCoord); To: entity.setPosition(xCoord, i, zCoord);
-
Yeah...sorry I was having issues getting it to work, but I have gotten past that point. Now I see where it is you are having issues, but my thought would be to use a block as the beam instead of an entity? I would make try to mimic how the redstone works when it is placed. Or is there a specific reason for using an entity?
-
Okay, I have taken a small look at your code. From tracing, I found that it isn't entering the block to spawn the entity. This doesn't work out to true: if(ent.getStackInSlot(0) == null && getStackInSlot(0) != null){ } I ent.getStackInSlot(0) returns true, but getStackInSlot(0) returns false. I'm going to continue to take a look and see where you are adding them into the array.
-
I don't know exactly what your problem is, but here is what I have to render an entity. I was trying to copy and paste your code, but there are some classes that I needed. MagicSpellsMod.java - Main Class package codemeister88.minecraft.src; import net.minecraft.item.Item; import net.minecraftforge.common.Configuration; import codemeister88.minecraft.entity.EntityEarthGolem; import codemeister88.minecraft.item.ItemEarthSpellbook; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.EventHandler; import cpw.mods.fml.common.Mod.Instance; import cpw.mods.fml.common.SidedProxy; import cpw.mods.fml.common.event.FMLInitializationEvent; import cpw.mods.fml.common.event.FMLPostInitializationEvent; import cpw.mods.fml.common.event.FMLPreInitializationEvent; import cpw.mods.fml.common.network.NetworkMod; import cpw.mods.fml.common.registry.EntityRegistry; @Mod(modid="MagicSpellsMod", name="Magic Spells", version="1.2.1") @NetworkMod(clientSideRequired=true, serverSideRequired=false) public class MagicSpellsMod { // The instance of your mod that Forge uses. @Instance("MagicSpellsMod") public static MagicSpellsMod instance; /* * Items */ //holds the ids of the items public static int[] itemIDs = new int[1]; //Earth Spell public static Item earthSpellbook; // Says where the client and server 'proxy' code is loaded. @SidedProxy(clientSide="codemeister88.minecraft.client.ClientProxy", serverSide="codemeister88.minecraft.src.CommonProxy") public static CommonProxy proxy; @EventHandler public void preInit(FMLPreInitializationEvent event) { Configuration config = new Configuration(event.getSuggestedConfigurationFile()); config.load(); itemIDs[0] = config.getItem("EarthSpellbook", 5010).getInt(); config.save(); } @EventHandler public void load(FMLInitializationEvent event) { proxy.registerRenderers(); //Earth Spell earthSpellbook = new ItemEarthSpellbook(itemIDs[0], 1); EntityRegistry.registerModEntity(EntityEarthGolem.class, "EarthGolem", 3, instance, 80, 1, true); } @EventHandler public void postInit(FMLPostInitializationEvent event) { } } CommonProxy.java package codemeister88.minecraft.src; import cpw.mods.fml.client.registry.RenderingRegistry; public class CommonProxy { public void registerRenderers() { } } ItemSpellbook.java package codemeister88.minecraft.item; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.EnumAction; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.world.World; public abstract class ItemSpellbook extends Item { protected String spellName; protected int spellLevel; public ItemSpellbook(int par1, String spellName, int spellLevel) { super(par1); this.maxStackSize = 1; this.setMaxDamage(256); this.setCreativeTab(CreativeTabs.tabCombat); this.setUnlocalizedName("spellbook"); this.spellName = spellName; this.spellLevel = spellLevel; setTextureName("book_enchanted"); } public ItemSpellbook setSpellLevel(int spellLevel) { if(spellLevel > 0) { this.spellLevel = spellLevel; } else { this.spellLevel = 1; } return this; } public int getSpellLevel() { return spellLevel; } /** * returns the action that specifies what animation to play when the items is being used */ public EnumAction getItemUseAction(ItemStack par1ItemStack) { return EnumAction.block; } /** * Called whenever this item is equipped and the right mouse button is pressed. Args: itemStack, world, entityPlayer */ public abstract ItemStack onItemRightClick(ItemStack par1ItemStack, World par2World, EntityPlayer par3EntityPlayer); /** * Returns the unlocalized name of this item. This version accepts an ItemStack so different stacks can have * different names based on their damage or NBT. */ public String getUnlocalizedName(ItemStack par1ItemStack) { return super.getUnlocalizedName(par1ItemStack) + "." + spellName + spellLevel; } public String getItemDisplayName(ItemStack par1ItemStack) { return spellName + " Spellbook " + spellLevel; } } ItemEarthSpellbook.java package codemeister88.minecraft.item; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.ItemStack; import net.minecraft.world.World; import codemeister88.minecraft.entity.EntityEarthGolem; public class ItemEarthSpellbook extends ItemSpellbook { private boolean entitySpawned = false; public ItemEarthSpellbook(int par1, int spellLevel) { super(par1, "Earth", spellLevel); } @Override public ItemStack onItemRightClick(ItemStack par1ItemStack, World par2World, EntityPlayer par3EntityPlayer) { par3EntityPlayer.setItemInUse(par1ItemStack, this.getMaxItemUseDuration(par1ItemStack)); par2World.playSoundAtEntity(par3EntityPlayer, "random.bow", 0.5F, 0.4F / (itemRand.nextFloat() * 0.4F + 0.8F)); if (!par2World.isRemote) { EntityEarthGolem entityEarthGolem = new EntityEarthGolem(par2World); entityEarthGolem.setLocationAndAngles(par3EntityPlayer.posX, par3EntityPlayer.posY, par3EntityPlayer.posZ, 0.0F, 0.0F); System.out.println("Spawn Golem"); par2World.spawnEntityInWorld(entityEarthGolem); entitySpawned = true; } return par1ItemStack; } } EntityEarthGolem.java package codemeister88.minecraft.entity; import net.minecraft.entity.EntityAgeable; import net.minecraft.entity.EntityCreature; import net.minecraft.entity.EntityLiving; import net.minecraft.entity.ai.EntityAIAttackOnCollide; import net.minecraft.entity.ai.EntityAIFollowParent; import net.minecraft.entity.ai.EntityAIHurtByTarget; import net.minecraft.entity.ai.EntityAILookAtVillager; import net.minecraft.entity.ai.EntityAILookIdle; import net.minecraft.entity.ai.EntityAIMoveThroughVillage; import net.minecraft.entity.ai.EntityAIMoveTowardsRestriction; import net.minecraft.entity.ai.EntityAIMoveTowardsTarget; import net.minecraft.entity.ai.EntityAINearestAttackableTarget; import net.minecraft.entity.ai.EntityAIOwnerHurtByTarget; import net.minecraft.entity.ai.EntityAIOwnerHurtTarget; import net.minecraft.entity.ai.EntityAIWander; import net.minecraft.entity.ai.EntityAIWatchClosest; import net.minecraft.entity.monster.IMob; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.world.World; public class EntityEarthGolem extends EntityCreature { public EntityEarthGolem(World par1World) { super(par1World); // getNavigator().setEnterDoors(true); // this.tasks.addTask(1, new EntityAIAttackOnCollide(this, 1.0D, true)); // this.tasks.addTask(2, new EntityAIFollowOwner(this, 1.0D, 10.0F, 2.0F)); // this.tasks.addTask(3, new EntityAIWander(this, 1.0D)); // this.tasks.addTask(4, new EntityAILookIdle(this)); this.tasks.addTask(1, new EntityAIAttackOnCollide(this, 1.0D, true)); this.tasks.addTask(2, new EntityAIMoveTowardsTarget(this, 0.9D, 32.0F)); this.tasks.addTask(3, new EntityAIWander(this, 0.6D)); this.tasks.addTask(4, new EntityAIWatchClosest(this, EntityPlayer.class, 6.0F)); this.tasks.addTask(5, new EntityAILookIdle(this)); // this.targetTasks.addTask(1, new EntityAIOwnerHurtByTarget(this)); // this.targetTasks.addTask(2, new EntityAIOwnerHurtTarget(this)); // this.targetTasks.addTask(3, new EntityAIHurtByTarget(this, true)); this.targetTasks.addTask(1, new EntityAIHurtByTarget(this, false)); this.targetTasks.addTask(2, new EntityAINearestAttackableTarget(this, EntityLiving.class, 0, true, true, IMob.mobSelector)); } /** * Plays step sound at given x, y, z for the entity */ protected void playStepSound(int par1, int par2, int par3, int par4) { this.playSound("gravel", 0.15F, 1.0F); } } RenderEarthGolem.java package codemeister88.minecraft.client.renderer.entity; import codemeister88.minecraft.client.model.ModelEarthGolem; import net.minecraft.client.model.ModelBase; import net.minecraft.client.model.ModelIronGolem; import net.minecraft.client.renderer.entity.RenderLiving; import net.minecraft.entity.Entity; import net.minecraft.util.ResourceLocation; public class RenderEarthGolem extends RenderLiving { private static final ResourceLocation earthGolemTextures = new ResourceLocation("codemeister88:textures/entity/earth_golem.png"); public RenderEarthGolem() { super(new ModelEarthGolem(), 0.5F); } @Override protected ResourceLocation getEntityTexture(Entity entity) { return earthGolemTextures; } } ClientProxy.java package codemeister88.minecraft.client; import net.minecraft.src.ModLoader; import codemeister88.minecraft.client.renderer.entity.RenderEarthGolem; import codemeister88.minecraft.entity.EntityEarthGolem; import codemeister88.minecraft.src.CommonProxy; import cpw.mods.fml.client.registry.RenderingRegistry; import cpw.mods.fml.common.registry.EntityRegistry; public class ClientProxy extends CommonProxy { @Override public void registerRenderers() { super.registerRenderers(); EntityRegistry.registerGlobalEntityID(EntityEarthGolem.class, "EarthGolem", ModLoader.getUniqueEntityId()); RenderingRegistry.registerEntityRenderingHandler(EntityEarthGolem.class, new RenderEarthGolem()); } } ModelEarthGolem.java package codemeister88.minecraft.client.model; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; import net.minecraft.client.model.ModelBase; import net.minecraft.client.model.ModelRenderer; import net.minecraft.entity.Entity; import net.minecraft.util.MathHelper; @SideOnly(Side.CLIENT) public class ModelEarthGolem extends ModelBase { /* * Model each part of the entity */ ModelRenderer body; ModelRenderer rightArm; ModelRenderer leftArm; ModelRenderer head; ModelRenderer leftLeg; ModelRenderer rightLeg; public ModelEarthGolem() { head = new ModelRenderer(this, 0, 0); head.addBox(-4F, -8F, -4F, 8, 8, ; head.setRotationPoint(0F, 0F, 0F); body = new ModelRenderer(this, 0, 16); body.addBox(-4F, 0F, -2F, 8, 12, 4); body.setRotationPoint(0F, 0F, 0F); rightArm = new ModelRenderer(this, 48, 0); rightArm.addBox(0F, 0F, -2F, 4, 12, 4); rightArm.setRotationPoint(4F, 0F, 0F); leftArm = new ModelRenderer(this, 32, 0); leftArm.addBox(-4F, 0F, -2F, 4, 12, 4); leftArm.setRotationPoint(-4F, 0F, 0F); leftLeg = new ModelRenderer(this, 24, 16); leftLeg.addBox(-2F, 0F, -2F, 4, 12, 4); leftLeg.setRotationPoint(-2F, 12F, 0F); rightLeg = new ModelRenderer(this, 40, 16); rightLeg.addBox(-2F, 0F, -2F, 4, 12, 4); rightLeg.setRotationPoint(2F, 12F, 0F); } @Override public void render(Entity entity, float f, float f1, float f2, float f3, float f4, float f5) { setRotationAngles(f, f1, f2, f3, f4, f5, entity); body.render(f5); rightArm.render(f5); leftArm.render(f5); head.render(f5); leftLeg.render(f5); rightLeg.render(f5); } @Override public void setRotationAngles(float par1, float par2, float par3, float par4, float par5, float par6, Entity entity) { head.rotateAngleY = par4 / (180F / (float)Math.PI); head.rotateAngleX = par5 / (180F / (float)Math.PI); rightArm.rotateAngleX = MathHelper.cos(par1 * 0.6662F + (float)Math.PI) * 2.0F * par2 * 0.5F; rightArm.rotateAngleZ = 0.0F; leftArm.rotateAngleX = MathHelper.cos(par1 * 0.6662F) * 2.0F * par2 * 0.5F; leftArm.rotateAngleZ = 0.0F; rightLeg.rotateAngleX = MathHelper.cos(par1 * 0.6662F) * 1.4F * par2; rightLeg.rotateAngleY = 0.0F; leftLeg.rotateAngleX = MathHelper.cos(par1 * 0.6662F + (float)Math.PI) * 1.4F * par2; leftLeg.rotateAngleY = 0.0F; } }
-
I don't know if you figured this out, but here is what I have found that seems to work. Output: 2013-11-22 17:48:37 [iNFO] [sTDOUT] Location: C:\Users\cody\forge_hardcore_mod\mcp\jars\.\hello_world.txt
-
Hardcore Mode in multiplayer server
Codemeister88 replied to Codemeister88's topic in Modder Support
Thanks...I'll give this a try. -
Hardcore Mode in multiplayer server
Codemeister88 replied to Codemeister88's topic in Modder Support
The current hardcore mode on multiplayer just bans the player. I want it to work the same way it does on single player for multiplayer. Stopping the server isn't my problem. I call the same code that is already in the MinecraftServer class; however, I would like it to start it again. -
Hardcore Mode in multiplayer server
Codemeister88 replied to Codemeister88's topic in Modder Support
I have tried to do this, but because they System.exit() it kills my thread as well. I need to stop the System.exit() from occurring. -
Hi, I am in the progress on trying to create a hardcore mode mod that works like the single player. I have everything working except the ability to restart the server; instead, the server is shutdown. I have read through and find where I believe I could modify the code, but it exists inside the core of minecraft. I know that forge has events that indicate when the server is shutting down. Would it be possible to have an event that could be cancelled which would prevent the System.exit() and start the server? Thanks in advance for any advice.