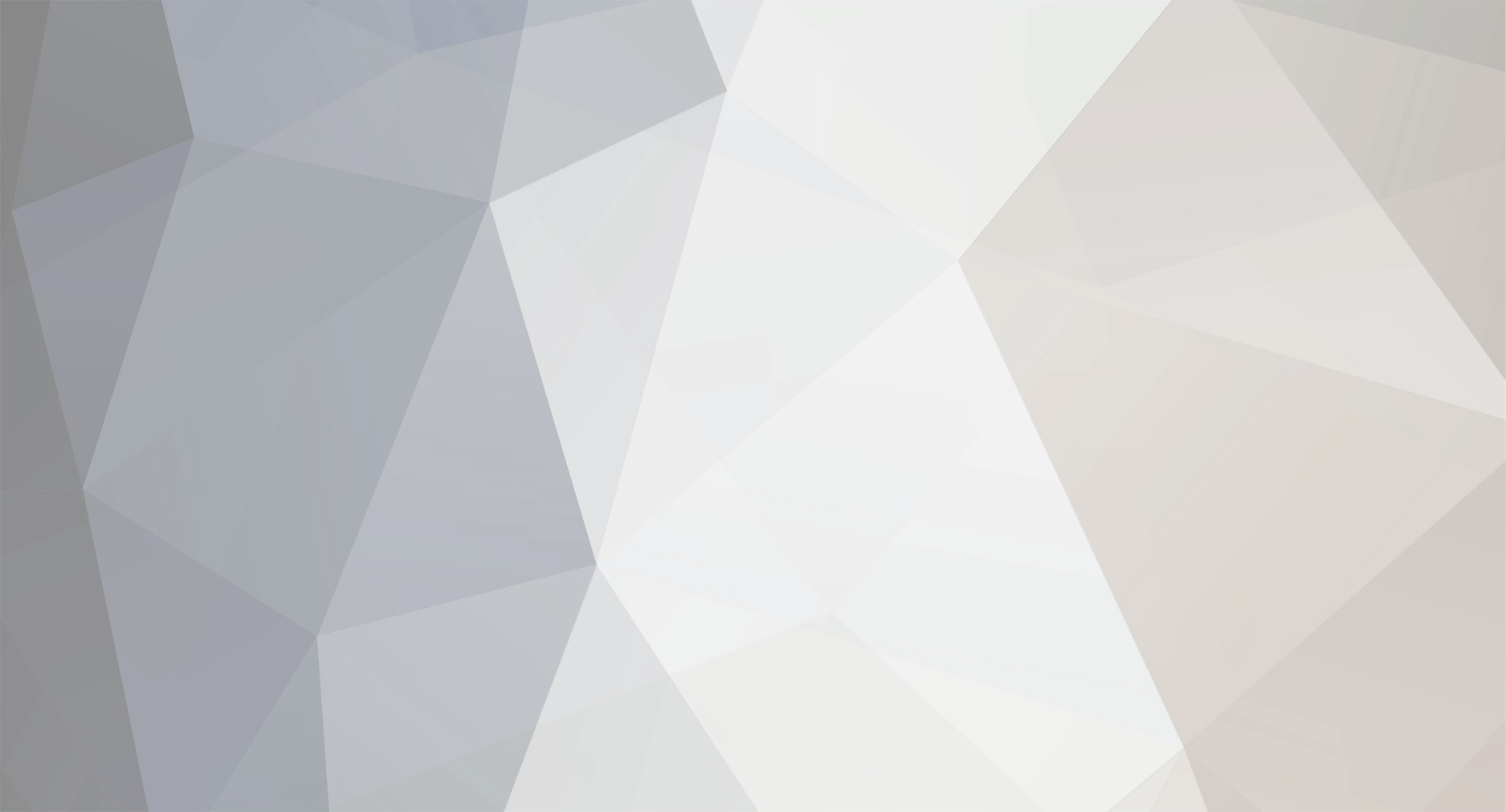
keleko
Members-
Posts
7 -
Joined
-
Last visited
Everything posted by keleko
-
[1.7.2] [Solved] Recipes doesn't work. Crash.
keleko replied to SackCastellon's topic in Modder Support
I'm unclear as the code changes from 1.6 to 1.7 as far as this or if you left code out for just the post but by looking at what you posted I can see You forgot to put this at the top of your load eventhandler proxy.registerRenderers(); Also another change to help you is how your associating your other files functions. try: ItemLoader: package SackCastellon.craftablehorsearmor.loader; import net.minecraft.init.Items; import net.minecraft.item.ItemStack; import cpw.mods.fml.common.registry.GameRegistry; public class ItemLoader { private static ItemStack stirrupStack = new ItemStack(this.Stirrup, 2); private static ItemStack ironStack = new ItemStack(Items.ingot, 1); public static void load() { GameRegistry.addRecipe(stirrupStack ," I ","I I","III", 'I',ironStack); } } Also With that hopefully your already registering the stirrup Item using gameregistry and languageregistry prior to your recipe registry below would be how you bring it into your load event. package SackCastellon.craftablehorsearmor; import java.io.File; import SackCastellon.core.helper.LogHelper; import SackCastellon.craftablehorsearmor.loader.RecipeLoader; import SackCastellon.craftablehorsearmor.proxy.CommonProxy; import SackCastellon.craftablehorsearmor.reference.Reference; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.EventHandler; import cpw.mods.fml.common.Mod.Instance; import cpw.mods.fml.common.SidedProxy; import cpw.mods.fml.common.event.FMLInitializationEvent; import cpw.mods.fml.common.event.FMLPostInitializationEvent; import cpw.mods.fml.common.event.FMLPreInitializationEvent; @Mod(modid=Reference.MODID, name=Reference.NAME, version=Reference.VERSION, dependencies=Reference.DEPENDENCIES) public class CraftableHorseArmor { @Instance(Reference.MODID) public static CraftableHorseArmor instance; @SidedProxy(clientSide=Reference.CLPROXY, serverSide=Reference.CMPROXY) public static CommonProxy proxy; public static ItemLoader registerItems = new ItemLoader (); @EventHandler public void preInit(FMLPreInitializationEvent event) {} @EventHandler public void load(FMLInitializationEvent event) { proxy.registerRenderers(); // Items registerItems.load(); } @EventHandler public void postInit(FMLPostInitializationEvent event) {} } -
Well I was finally able to get back to coding and yep, merely setting the metadata values below 15 worked TY! In the tileentity I now just check for this.getMetadata. which works great! and for the crashing issue I just use this.metaData = 0; inside the tileentity constructor and that resets it to 0 when the game is freshly loaded. Example: Block Class public void onNeighborBlockChange(World world, int i, int j, int k, int l) { System.out.println("neighbor Event ran"); if (world.isBlockIndirectlyGettingPowered(i, j, k)) { world.setBlockMetadataWithNotify(i, j, k, 2,3); } else { world.setBlockMetadataWithNotify(i, j, k, 0,3); } } TileEntityClass public TileEntityGlassLaser() { this.blockMetadata = 0; } @Override public void updateEntity() { if(this.getBlockMetadata() == 2) { if(!worldObj.isRemote){ LaserParticleEffects.spawnParticle("test", this.xCoord+0.5f, this.yCoord-0.1f, this.zCoord+0.5f, 1.5D, -0.1D, 1.5D); } } super.updateEntity(); } TY Everyone!
-
ah ok, that might have been what was happening. I will retry that then.
-
Well I was able to finally solve this but was unable to get it working via metadata(probably due to my lack of knowledge on custom TileEntity Blocks and metadata) My method of solving this was to merely create a second Block that had the particle render in its own tileentity. ex: public void onNeighborBlockChange(World world, int i, int j, int k, int l) { if (world.isBlockIndirectlyGettingPowered(i, j, k)) { world.setBlock(i,j,k,Blocks.blockGlassLaserOn.blockID); } } and then on the other block I merely reversed it. public void onNeighborBlockChange(World world, int i, int j, int k, int l) { if (!world.isBlockIndirectlyGettingPowered(i, j, k)) { world.setBlock(i,j,k,Blocks.blockGlassLaser.blockID); } } though I did not want to do this as it creates issues with if the player left it in the on state and were to restart the client/server it would crash cause it calls the particle render too fast and sees a nullpointer So If anyone knows of a way to fix this? or maybe set a block in the world with an id=blockGlassLaserOn.blockID To the default off state when the server/client is first loaded so it will not crash. I think atm though I can fix it by setting a bool in the load event to false and set it to true when the default block gets power although this is unfortunate as the player will have to turn it off and back on again in the case they left it in the on position when they quit the client/server
-
It seems I must make a separate Block Id For This, I was thinking something like this would be promising: if(world.isBlockIndirectlyGettingPowered(i, j, k)) { if(blockID == CLASS.laserStateOff.blockID) { world.setBlockMetadataWithNotify(i, j, k, CLASS.laserStateOn.blockID); } else { world.setBlockMetadataWithNotify(i, j, k, CLASS.laserStateOff.blockID); } } My other question though with this is whether I might need to use world.setBlockMetadataWithNotify(i, j, k, CLASS.laserStateOff.blockID); for the original constructors as well or if just having it as above should be fine?
-
ok, thanks. I will definitely give that a try.
-
I would like to preemptively say I'm sorry I am newish to forge modding, I seem to have come to a stump and can not find the answer in the forums. But My problem is that when one block gets updated with redstone all blocks of the same Id seem to get updated as well, while I Only want a single one(the one seeing redstone) to be updated. The Block Code is As Follows: public static boolean laserState = false; public void onNeighborBlockChange(World world, int x, int y, int z, int l) { if(BlockGlassBase.BaseHasGlass) { if (world.isBlockIndirectlyGettingPowered(x, y, z)) { this.laserState = true; this.setLightValue(0.8f); } else { this.laserState = false; this.setLightValue(0.0f); } } } The TileEntity Code is As Follows: @Override public void updateEntity() { if(BlockGlassLaser.laserState) { if(!worldObj.isRemote){ LaserParticleEffects.spawnParticle("test", this.xCoord+0.5f, this.yCoord-0.1f, this.zCoord+0.5f, 1.5D, -0.1D, 1.5D); } } super.updateEntity(); } I tried many different things to get this to work from having a incrementing int in the block constructor for an associated id per block but every block was only 1 suggesting all were made at the same time when I logged in() I also tried having the laserState variable in the TileEntity Class and from the block class running a function that was located in the TileEntity Class that set its state but to no avail it also updated all blocks as well. I'm probably noobing it up but please help!