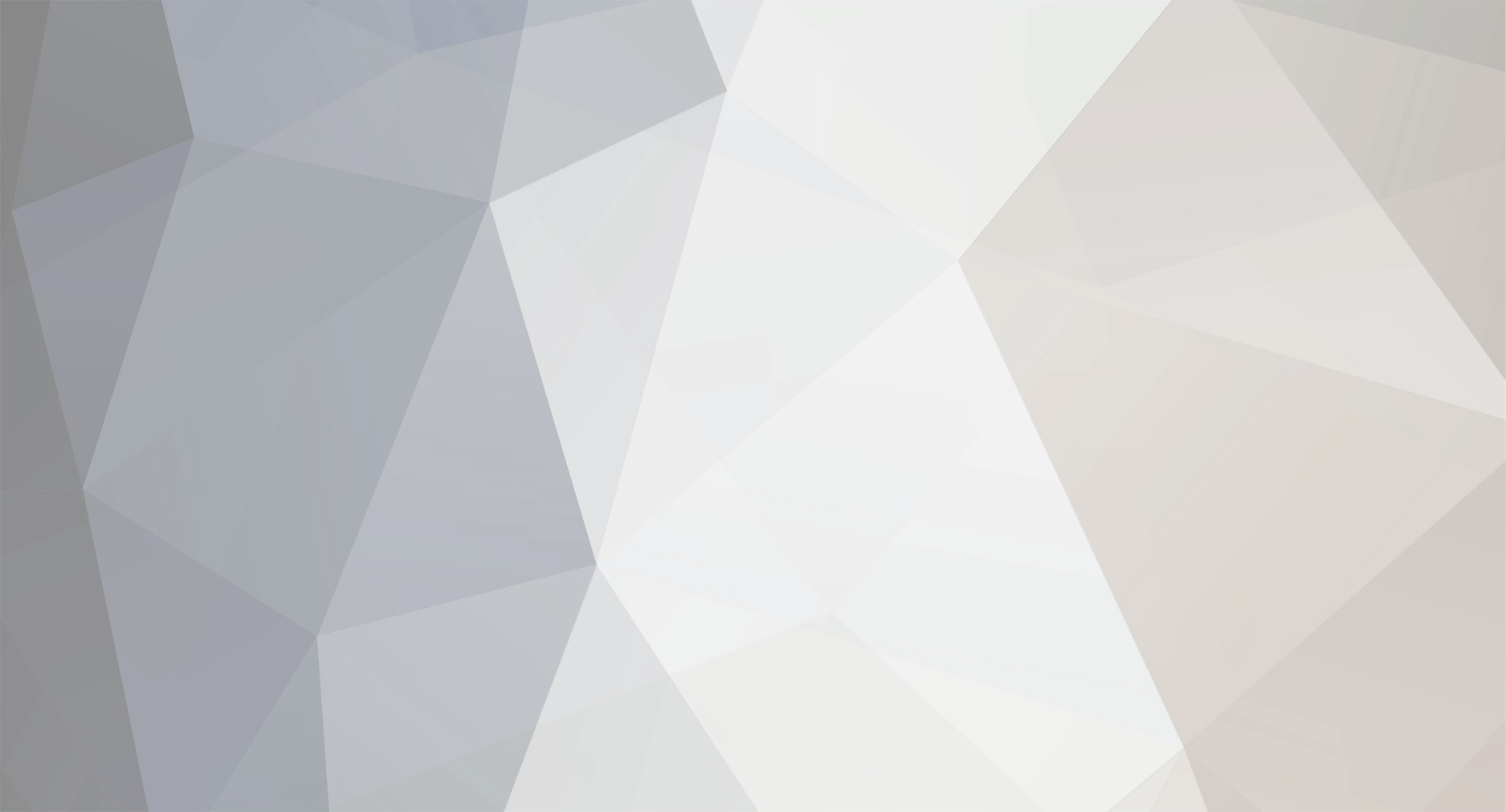
LordMastodon
Forge Modder-
Posts
173 -
Joined
-
Last visited
Everything posted by LordMastodon
-
[solved] [1.8] Render Glitch with TileEntity
LordMastodon replied to LordMastodon's topic in Modder Support
I solved the world holes, but the lighting for the model is still glitched. For some reason everything that is facing the side of a block is completely black. When I break a block next to the model, the lighting glitch on that side disappears, and when I place it again the glitch comes back. As that is the case, I'm thinking it might not be a glitch, but I'd like to know if there's any way to disable the lighting entirely for the model without using a TESR. -
[solved] [1.8] Render Glitch with TileEntity
LordMastodon replied to LordMastodon's topic in Modder Support
UPDATE After adding @Override public int getRenderType() { return 3; } It seems as though it's rendering correctly, but when place in a hole, you can see through the world to the side of the model, as seen in this screenshot: The lighting is also completely screwed up for some reason. -
[solved] [1.8] Render Glitch with TileEntity
LordMastodon replied to LordMastodon's topic in Modder Support
Yes, that's the whole block class. -
[solved] [1.8] Render Glitch with TileEntity
LordMastodon replied to LordMastodon's topic in Modder Support
That seems to work when I place it above ground, but when I place it in a hole, it gives me world holes in the sides and completely screws up the lighting too... -
Why did this: Suddenly start happening to my block when I added a TileEntity? Before that it was working better than fine. It makes no sense to me that once I add a TileEntity to a block suddenly the render starts crapping itself. Here's the main class which registers the TileEntites: package robotsculptures; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.Mod.EventHandler; import net.minecraftforge.fml.common.Mod.Instance; import net.minecraftforge.fml.common.SidedProxy; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; import robotsculptures.block.RSBlocks; import robotsculptures.constants.ModConstants; import robotsculptures.proxy.CommonProxy; import robotsculptures.tileentity.RSTileEntities; @Mod(modid = ModConstants.MOD_ID, name = ModConstants.MOD_NAME, version = ModConstants.MOD_VERSION, acceptedMinecraftVersions = ModConstants.ACCEPTED_MINECRAFT_VERSIONS) public class RobotSculptures { @SidedProxy(clientSide = ModConstants.CLIENT_PROXY_PATH, serverSide = ModConstants.COMMON_PROXY_PATH) public static CommonProxy proxy; @Instance(ModConstants.MOD_ID) public static RobotSculptures instance; @EventHandler public void preInit(FMLPreInitializationEvent event) { RSBlocks.init(); RSBlocks.register(); } @EventHandler public void init(FMLInitializationEvent event) { proxy.registerRenders(); RSTileEntities.register(); } @EventHandler public void postInit(FMLPostInitializationEvent event) { } } Here's the class with the actual register code: package robotsculptures.tileentity; import net.minecraftforge.fml.common.registry.GameRegistry; public class RSTileEntities { public static void register() { GameRegistry.registerTileEntity(KenSculptureTileEntity.class, "KenSculptureTileEntity"); } } Here's the block class: package robotsculptures.blocks; import net.minecraft.block.Block; import net.minecraft.block.BlockContainer; import net.minecraft.block.material.Material; import net.minecraft.block.properties.IProperty; import net.minecraft.block.properties.PropertyDirection; import net.minecraft.block.state.BlockState; import net.minecraft.block.state.IBlockState; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.BlockPos; import net.minecraft.util.ChatComponentText; import net.minecraft.util.EnumChatFormatting; import net.minecraft.util.EnumFacing; import net.minecraft.world.World; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; import robotsculptures.tileentity.KenSculptureTileEntity; public class KenSculptureBlock extends BlockContainer { public static final PropertyDirection FACING = PropertyDirection.create("facing", EnumFacing.Plane.HORIZONTAL); public KenSculptureBlock(Material mat) { super(mat); this.setDefaultState(this.blockState.getBaseState().withProperty(FACING, EnumFacing.NORTH)); } public boolean onBlockActivated(World world, BlockPos pos, IBlockState state, EntityPlayer player, EnumFacing side, float hitX, float hitY, float hitZ) { KenSculptureTileEntity kste = (KenSculptureTileEntity) world.getTileEntity(pos); kste.ignite(); return true; } @Override public void onBlockHarvested(World worldIn, BlockPos pos, IBlockState state, EntityPlayer player) { if (!worldIn.isRemote) { player.addChatMessage(new ChatComponentText(EnumChatFormatting.RED + "You DARE to destroy the sculpture of Ken the Mighty?!")); player.addChatMessage(new ChatComponentText(EnumChatFormatting.DARK_RED + "Be forwarned. Bad things may befall you yet.")); } } public void onBlockAdded(World worldIn, BlockPos pos, IBlockState state) { if (!worldIn.isRemote) { Block block = worldIn.getBlockState(pos.north()).getBlock(); Block block1 = worldIn.getBlockState(pos.south()).getBlock(); Block block2 = worldIn.getBlockState(pos.west()).getBlock(); Block block3 = worldIn.getBlockState(pos.east()).getBlock(); EnumFacing enumFacing = (EnumFacing) state.getValue(FACING); if (enumFacing == EnumFacing.NORTH && block.isFullBlock() && !block1.isFullBlock()) { enumFacing = EnumFacing.SOUTH; } else if (enumFacing == EnumFacing.SOUTH && block1.isFullBlock() && !block.isFullBlock()) { enumFacing = EnumFacing.NORTH; } else if (enumFacing == EnumFacing.WEST && block2.isFullBlock() && !block3.isFullBlock()) { enumFacing = EnumFacing.EAST; } else if (enumFacing == EnumFacing.EAST && block3.isFullBlock() && !block2.isFullBlock()) { enumFacing = EnumFacing.WEST; } worldIn.setBlockState(pos, state.withProperty(FACING, enumFacing), 2); } } public IBlockState onBlockPlaced(World worldIn, BlockPos pos, EnumFacing facing, float hitX, float hitY, float hitZ, int meta, EntityLivingBase placer) { return this.getDefaultState().withProperty(FACING, placer.getHorizontalFacing().getOpposite()); } @SideOnly(Side.CLIENT) public IBlockState getStateForEntityRender(IBlockState state) { return this.getDefaultState().withProperty(FACING, EnumFacing.SOUTH); } public IBlockState getStateFromMeta(int meta) { EnumFacing enumFacing = EnumFacing.getFront(meta); if (enumFacing.getAxis() == EnumFacing.Axis.Y) { enumFacing = EnumFacing.NORTH; } return this.getDefaultState().withProperty(FACING, enumFacing); } public int getMetaFromState(IBlockState state) { return ((EnumFacing) state.getValue(FACING)).getIndex(); } protected BlockState createBlockState() { return new BlockState(this, new IProperty[] {FACING}); } @SideOnly(Side.CLIENT) static final class SwitchEnumFacing { static final int[] FACING_LOOKUP = new int[EnumFacing.values().length]; static { try { FACING_LOOKUP[EnumFacing.WEST.ordinal()] = 1; } catch (NoSuchFieldError var4) { ; } try { FACING_LOOKUP[EnumFacing.EAST.ordinal()] = 2; } catch (NoSuchFieldError var3) { ; } try { FACING_LOOKUP[EnumFacing.NORTH.ordinal()] = 3; } catch (NoSuchFieldError var2) { ; } try { FACING_LOOKUP[EnumFacing.SOUTH.ordinal()] = 4; } catch (NoSuchFieldError var1) { ; } } } @Override public TileEntity createNewTileEntity(World worldIn, int meta) { return new KenSculptureTileEntity(); } } And finally, here's the TileEntity class: package robotsculptures.tileentity; import net.minecraft.server.gui.IUpdatePlayerListBox; import net.minecraft.tileentity.TileEntity; public class KenSculptureTileEntity extends TileEntity implements IUpdatePlayerListBox { private int fuse = 60; private boolean ignited = false; @Override public void update() { if (checkExplosion()) { getWorld().createExplosion(null, pos.getX() + 0.5, pos.getY() + 0.5, pos.getZ() + 0.5, 3.0F, true); ignited = false; fuse = 60; } } public void ignite() { ignited = true; } private boolean checkExplosion() { if (ignited) { if (fuse > 0) { System.out.println(fuse); fuse--; } else { System.out.println("Exploding"); return true; } } return false; } } Sorry if that was a crazy amount of code, but I'm not quite sure why this could be happening at all. Side note: that's not a blue plane in the middle of the block. It's a hole in the world.
-
Got it. Thanks!
-
Got it. The problem is, they have to be static, unless you can find a way to make an instance of a TileEntity with using the new keyword. Here's the class that accesses them: package <modname>.blocks; import net.minecraft.block.Block; import net.minecraft.block.BlockContainer; import net.minecraft.block.material.Material; import net.minecraft.block.properties.IProperty; import net.minecraft.block.properties.PropertyDirection; import net.minecraft.block.state.BlockState; import net.minecraft.block.state.IBlockState; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.BlockPos; import net.minecraft.util.ChatComponentText; import net.minecraft.util.EnumChatFormatting; import net.minecraft.util.EnumFacing; import net.minecraft.world.World; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; import <modname>.tileentity.<tileentity>TileEntity; public class <blockname> extends BlockContainer { public static final PropertyDirection FACING = PropertyDirection.create("facing", EnumFacing.Plane.HORIZONTAL); public <blockName>(Material mat) { super(mat); this.setDefaultState(this.blockState.getBaseState().withProperty(FACING, EnumFacing.NORTH)); } public boolean onBlockActivated(World world, BlockPos pos, IBlockState state, EntityPlayer player, EnumFacing side, float hitX, float hitY, float hitZ) { <tileentityname>TileEntity.ignite(); return true; } @Override public void onBlockHarvested(World worldIn, BlockPos pos, IBlockState state, EntityPlayer player) { if (!worldIn.isRemote) { player.addChatMessage(new ChatComponentText(EnumChatFormatting.RED + "You DARE to destroy the sculpture of <sculpturename>?!")); player.addChatMessage(new ChatComponentText(EnumChatFormatting.DARK_RED + "Be forwarned. Bad things may befall you yet.")); } } public void onBlockAdded(World worldIn, BlockPos pos, IBlockState state) { if (!worldIn.isRemote) { Block block = worldIn.getBlockState(pos.north()).getBlock(); Block block1 = worldIn.getBlockState(pos.south()).getBlock(); Block block2 = worldIn.getBlockState(pos.west()).getBlock(); Block block3 = worldIn.getBlockState(pos.east()).getBlock(); EnumFacing enumFacing = (EnumFacing) state.getValue(FACING); if (enumFacing == EnumFacing.NORTH && block.isFullBlock() && !block1.isFullBlock()) { enumFacing = EnumFacing.SOUTH; } else if (enumFacing == EnumFacing.SOUTH && block1.isFullBlock() && !block.isFullBlock()) { enumFacing = EnumFacing.NORTH; } else if (enumFacing == EnumFacing.WEST && block2.isFullBlock() && !block3.isFullBlock()) { enumFacing = EnumFacing.EAST; } else if (enumFacing == EnumFacing.EAST && block3.isFullBlock() && !block2.isFullBlock()) { enumFacing = EnumFacing.WEST; } worldIn.setBlockState(pos, state.withProperty(FACING, enumFacing), 2); } } public IBlockState onBlockPlaced(World worldIn, BlockPos pos, EnumFacing facing, float hitX, float hitY, float hitZ, int meta, EntityLivingBase placer) { return this.getDefaultState().withProperty(FACING, placer.getHorizontalFacing().getOpposite()); } @SideOnly(Side.CLIENT) public IBlockState getStateForEntityRender(IBlockState state) { return this.getDefaultState().withProperty(FACING, EnumFacing.SOUTH); } public IBlockState getStateFromMeta(int meta) { EnumFacing enumFacing = EnumFacing.getFront(meta); if (enumFacing.getAxis() == EnumFacing.Axis.Y) { enumFacing = EnumFacing.NORTH; } return this.getDefaultState().withProperty(FACING, enumFacing); } public int getMetaFromState(IBlockState state) { return ((EnumFacing) state.getValue(FACING)).getIndex(); } protected BlockState createBlockState() { return new BlockState(this, new IProperty[] {FACING}); } @SideOnly(Side.CLIENT) static final class SwitchEnumFacing { static final int[] FACING_LOOKUP = new int[EnumFacing.values().length]; static { try { FACING_LOOKUP[EnumFacing.WEST.ordinal()] = 1; } catch (NoSuchFieldError var4) { ; } try { FACING_LOOKUP[EnumFacing.EAST.ordinal()] = 2; } catch (NoSuchFieldError var3) { ; } try { FACING_LOOKUP[EnumFacing.NORTH.ordinal()] = 3; } catch (NoSuchFieldError var2) { ; } try { FACING_LOOKUP[EnumFacing.SOUTH.ordinal()] = 4; } catch (NoSuchFieldError var1) { ; } } } @Override public TileEntity createNewTileEntity(World worldIn, int meta) { return new <tileentityname>TileEntity(); } }
-
So I've set it up so that I've got a block, and if I right-click that block, 60 update()s later, it explodes. Now the bug here is that if I place two of the blocks, and right-click the one I placed last, the one I placed first explodes, instead of the one I placed last. Here's the TileEntity (necessary for the update() function) code: package <modpackage>.tileentity; import net.minecraft.server.gui.IUpdatePlayerListBox; import net.minecraft.tileentity.TileEntity; public class <blockname>TileEntity extends TileEntity implements IUpdatePlayerListBox { private static int fuse = 60; private static boolean ignited = false; @Override public void update() { if (checkExplosion()) { getWorld().createExplosion(null, pos.getX() + 0.5, pos.getY() + 0.5, pos.getZ() + 0.5, 3.0F, true); ignited = false; fuse = 60; } } public static void ignite() { ignited = true; } private static boolean checkExplosion() { if (ignited) { if (fuse > 0) { System.out.println(fuse); fuse--; } else { System.out.println("Exploding"); return true; } } return false; } } It's nothing special, but it does its job well. Any help? Thanks in advance.
-
[solved] [1.8] How to get EntityPlayer from world parameter
LordMastodon replied to LordMastodon's topic in Modder Support
Got it. Thanks. -
[solved] [1.8] How to get EntityPlayer from world parameter
LordMastodon replied to LordMastodon's topic in Modder Support
Ah. Ok. Thanks. Also, side question: how could I check from a TileEntity if a block has been right-clicked? Thanks! EDIT 1 (unrelated to side question) player.sendChatMessage does not seem to exist. EDIT 2 Stupid me. It's addChatMessage, not send. -
[solved] [1.8] How to get EntityPlayer from world parameter
LordMastodon replied to LordMastodon's topic in Modder Support
Is there a way that I can send it client-side using onBlockHarvested? Because I want the message to be visible only to the player that destroyed the block, not everyone on the server. -
[solved] [1.8] How to get EntityPlayer from world parameter
LordMastodon replied to LordMastodon's topic in Modder Support
Got it. So I should write @SideOnly(Side.CLIENT) and then below override onBlockDestroyedByPlayer. Makes sense. EDIT 1 There is no EntityPlayer parameter for onBlockDestroyedByPlayer. It's got the same parameters as breakBlock. I suppose it makes sense, for instance a block could be destroyed by an explosion and I suppose they wanted another method that specifies that this should only happen when the block is destroyed by a player. However, there's no EntityPlayer parameter, meaning this helps me the same amount as breakBlock. However, looking again, there seems to be 3 functions that could be correct and DO have the EntityPlayer parameter: onBlockHarvested, removedByPlayer and harvestBlock. Any one of those could potentially be it, but I'm not sure which one or if any at all. -
Is there way to get the player from a world parameter? I want to have my block send a chat message to the player when it is broken, and the breakBlock function does not supply a player parameter. However, it does supply a world parameter and I'm wondering if it's possible to get the player from the world class, or indeed from any of the parameters for the breakBlock function (world seems most likely)? If not, is there any other way to send a chat message using the breakBlock function?
-
[Solved] [1.8] variants: #normal set to null
LordMastodon replied to LordMastodon's topic in Modder Support
I'm using MrCrayfish's Model Creator. That has no cube or size limit (*cough cough* Cubik Lite *cough*), and it exports pretty well. -
[Solved] [1.8] variants: #normal set to null
LordMastodon replied to LordMastodon's topic in Modder Support
Cubik DOES cost money though... -
[Solved] [1.8] variants: #normal set to null
LordMastodon replied to LordMastodon's topic in Modder Support
Is that so? In 1.7 I used it all the time for anything and everything that need a custom model. What's the best free .json model creator out there in your opinion (I REALLY don't want to go and write the code myself, as it seems like that would take on the order of x3 the time it would take in a model creator)? -
So, I'm trying to make a custom model using TileEntitySpecialRenderer (and succeeding, so far). Yes, I am not in fact an idiot, and know that the .json system was added for that very purpose, but so far I'm finding it much easier to use TileEntitySpecialRenderer than that system. It's probably because it's new, and I'm guessing it'll grow on me at some point, but I'd just rather use what I know as long as works (probably not for long, considering they just implemented the .json point). Anyhow, my problem is that while I DO want to render the inventory version of the block, I don't want to render the normal version. So what I'm asking is if there's a way, in the blockstates file, to set the normal variant to null, meaning that it doesn't pass anything to the renderer when it asks for the normal variant. I don't want to pass an empty cube, as that would simply render the invisible cube instead of the TileEntitySpecialRenderer. Thanks in advance!
-
Thank you for providing that. I've decided to switch to Eclipse now, and that has completely fixed my problem. *grumble grumble forge recommending eclipse grumble*. A sad state of events, but it had to be done.
-
UPDATE: I've had a look at http://greyminecraftcoder.blogspot.com/2015/05/symptoms-of-block-and-item-model-errors_24.html and while he/she has a very detailed website, everything that was stated as could be causing the issue was not, in fact, a problem, and every single one of my names is set correctly, and every single one of my tags is set correctly. I've also created a block, but it seems to be having the same problem as the item, with the addition of "[22:04:46] [Client thread/ERROR] [FML]: Model definition for location techschmech:dual_smeltery#normal not found". Help is very much required, thank you.
-
So, I'm trying to create a custom item for my mod, having followed MrCrayfish's tutorial, and Minecraft doesn't seem to want to recognize that my JSON file actually exists. As the "image" feature of the forums seems to be broken, meaning that I can not share a screenshot of my path, I'll simply tell you that my path is exactly as follows: src/main/resources/assets/techschmech/models/item/dualSmeltingCalculationCPU.json. This is exactly the path specified by the tutorial, and tutorial does seem to have got it right. Here is the code inside the JSON: { "parent": "builtin/generated", "textures": { "layer0": "techschmech:items/dualSmeltingCalculationCPU" }, "display": { "thirdperson": { "rotation": [ -90, 0, 0 ], "translation": [ 0, 1, -3 ], "scale": [ 0.55, 0.55, 0.55 ] }, "firstperson": { "rotation": [ 0, -135, 25 ], "translation": [ 0, 4, 2 ], "scale": [ 1.7, 1.7, 1.7 ] } } } It was copied over exactly from apple.json, so there should be no syntactical errors. In fact, the console error itself is: "[19:27:22] [Client thread/ERROR] [FML]: Model definition for location techschmech:dualSmeltingCalculationCPU#inventory not found" Here is the code for my Items class that retrieves and registers renders: package techschmech.registry; import net.minecraft.client.Minecraft; import net.minecraft.client.resources.model.ModelResourceLocation; import net.minecraft.item.Item; import net.minecraftforge.fml.common.registry.GameRegistry; import techschmech.constants.ModConstants; public class TSItems { public static Item dualSmeltingCalculationCPU; public static void init() { dualSmeltingCalculationCPU = new Item().setUnlocalizedName("dualSmeltingCalculationCPU"); } public static void register() { GameRegistry.registerItem(dualSmeltingCalculationCPU, dualSmeltingCalculationCPU.getUnlocalizedName().substring(5)); } public static void registerRenders() { registerRender(dualSmeltingCalculationCPU); } public static void registerRender(Item item) { Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(item, 0, new ModelResourceLocation(ModConstants.ID + ":" + item.getUnlocalizedName().substring(5), "inventory")); } } And finally, here is the code for the ClientProxy which actually calls the function in TSItems: package techschmech.proxy; import techschmech.registry.TSItems; public class ClientProxy extends CommonProxy { @Override public void registerRenders() { TSItems.registerRenders(); } } Please let me know if you require any other information. Thank you in advance EDIT 1 I've changed all the names of the files and variables and such to dual_smelting_calculation_cpu as opposed to dualSmeltingCalculationCPU, as per the recommendation of MrCrayfish in his video, and this does not seem to have helped one tiny bit.
-
[1.8] acceptedMinecraftVersions documentation
LordMastodon replied to LordMastodon's topic in Modder Support
I have looked at that code, and that's the reason I even knew to specify the acceptedMinecraftVersions, but I'm not quite sure what you're trying to convey with that message. Some clarification would be nice. Thank you, that helped tremendously. -
I've been searching, but I can't find any documentation for acceptedMinecraftVersions in the @Mod @interface. I read in the @Mod class that it's recommended to set it, so I looked at the code for Thermal Foundation to see if and how they set it, seeing as how I've got great coding respect for TeamCOFH, but they've set it for 1.7.10 and I'm not sure if it's changed in between 1.7.10 and 1.8. Any help would be greatly appreciated.
-
Thanks !
-
If that is the case, than I'm afraid I'm not quite sure what you're talking about. If you could provide a screenshot highlighting what you are talking about, that would be very helpful in solving this problem. EDIT 1 Never mind. It seems that after downloading the absolute latest version of Src on files.minecraftforge.net and using that, it's worked. I'm not quite sure as to why it wouldn't be working with the latest version of 1.8, but I don't think it quite matters. Thank you all for your help, and I'd like to apologize to your faces about any facepalms that may have been executed as a result of my actions.
-
I'm not quite sure what you mean. I've gone to files.minecraftforge.net and downloaded the latest Src build, unpacked everything and run the commands, and that gets me this error message. If there's something else I need, then it wasn't mentioned in the official tutorial.