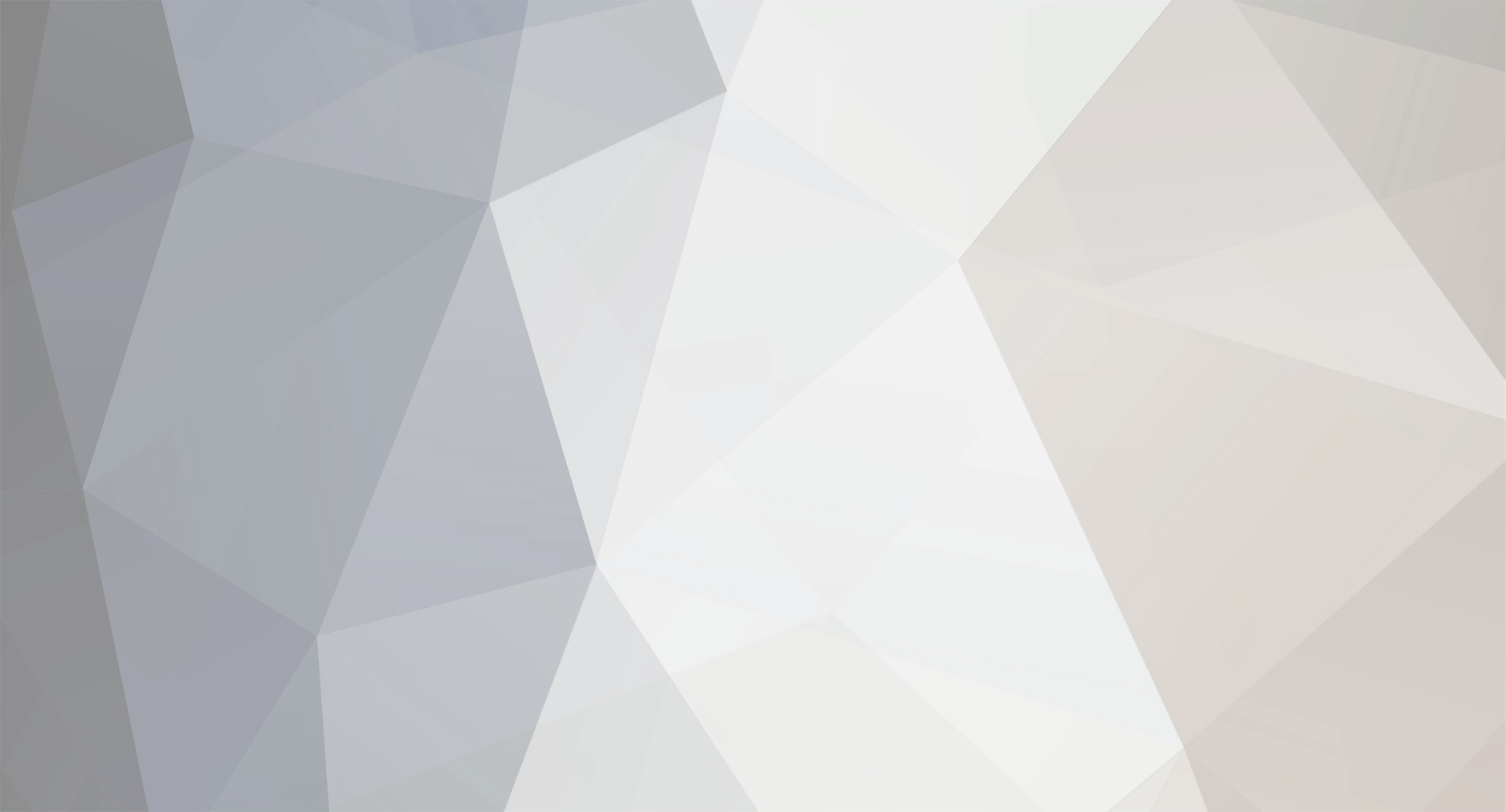
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
Okay, if the list of banned items is short, like it seems to be, I don't think you need an array or list of banned items but can just check them directly. The point of the "recursion" is simply the idea that if you get a random item that is banned you'll want to choose again (and it may again find a banned item). The Java while statement is meant for these sort of situations -- it keeps looping until it finds a condition that is true (in this case until you get a non-banned item. So I suggest something like this (probably needs some debug as I didn't run this, but it should be right idea): 1. create a local boolean variable called something like foundItem and initialize to false. 2. create a local ItemStack variable called something like itemToDrop and initialize to null. 3. have a while loop that continues as long as !foundItem. 4. inside the loop, set itemToDrop to a generated random item and check if it is any of the banned items. If they are not any banned item (probably should also check for null), then set founditem to true. 5. loop should exit with a valid itemToDrop so then you can just drop it. Anyway, hope you get the idea. Main point is that the while loop is intended for such repeated checks.
-
I think there can be only a couple possible causes. Either you didn't override the function properly, or not all entities call this, or the potion effect isn'w working as expected. It looks like you have the right parameters to override, but you should add the @Override annotation before the method to confirm. It will give you an error if it is not the right parameter template. For the next thing, you can confirm that onEntityWalking() is called by all entities by using your IDE to trace the "call hierarchy". I checked and it seems to be called for all entities during their movement, so it should be being called. To really confirm, I suggest you put a console statement in your method to print out every time there is entity walking on your block. Assuming that you've properly overridden the method, and that all entities actually do call the method, then it must be that the potion effect isn't working as expected. I don't know a lot about potions, so maybe someone else has ideas there.
-
Hmmm, not sure. You might want to try setAvoidsWater(false) instead. Logically swimming and avoiding water are different things, but perhaps there is some conflict in pathfinding if it tries to avoid water while it is in it.
-
Entities with collision box and/or light effect
jabelar replied to Bedrock_Miner's topic in Modder Support
Well, your TileEntity should have a custom block associated with it and blocks are already break-able with a sword depending on hardness . I think in your custom block class you can override the getPlayerRelativeBlockHardness() to check if sword is being held and if it call the super function and if not return 0.0F (i.e. doesn't damage block -- the name of the method is a bit misleading as it returns the damage not the hardness). Something like this I think (I haven't tried this, but just based on what I think should work): @Override public float getPlayerRelativeBlockHardness(EntityPlayer entityPlayer, World world, int x, int y, int z ){ ItemStack heldStack = entityPlayer.getHeldItem(); if( heldStack != null && heldStack.getItem() instanceof ItemSword ){ return super.getPlayerRelativeBlockHardness( entityPlayer, world, x, y, z ); } return 0.0F; } For controlling which entities can walk through it, you can dynamically change the collision box. I explain how to do this in my tutorial (see the bounding box section) at http://jabelarminecraft.blogspot.com/p/minecraft-forge-172-modding-quick-tips.html. Basically you return different collision box depending on the entity checking the collision box. The good thing about this is that entity pathfinding is also affected so entities will know they can move through. -
No offense but I am not sure how the code you posted would possibly work to prevent items. Where do you check if the item is a banned item? You would at least need to create a list or array of the banned items and test the random item against it. Your code just gets a random item and never checks if it is banned. I don't think you really need to create instances of each banned item either, that is what the instanceof operator is for (comparing to see if something is an instance). Also, when you check if select instanceof Item you don't also check if it is null because instanceof won't match if it is null already.
-
[1.7.10][Build 1180]Making entities spawn into world
jabelar replied to theOriginalByte's topic in Modder Support
You mean to spawn naturally during world creation, or in response to some event or activity on part of the player? The natural spawns that are associated with the defined biomes, I use something like the following (called by my CommonProxy in the preInit() phase). public void registerEntitySpawns() { // register natural spawns for entities // EntityRegistry.addSpawn(MyEntity.class, spawnProbability, minSpawn, maxSpawn, enumCreatureType, [spawnBiome]); // See the constructor in BiomeGenBase.java to see the rarity of vanilla mobs; Sheep are probability 10 while Endermen are probability 1 // minSpawn and maxSpawn are about how groups of the entity spawn // enumCreatureType represents the "rules" Minecraft uses to determine spawning, based on creature type. By default, you have three choices: // EnumCreatureType.creature uses rules for animals: spawn everywhere it is light out. // EnumCreatureType.monster uses rules for monsters: spawn everywhere it is dark out. // EnumCreatureType.waterCreature uses rules for water creatures: spawn only in water. // [spawnBiome] is an optional parameter of type BiomeGenBase that limits the creature spawn to a single biome type. Without this parameter, it will spawn everywhere. // DEBUG System.out.println("Registering natural spawns"); // For the biome type you can use an list, but unfortunately the built-in biomeList contains // null entries and will crash, so you need to clean up that list. // Diesieben07 suggested the following code to remove the nulls and create list of all biomes BiomeGenBase[] allBiomes = Iterators.toArray(Iterators.filter(Iterators.forArray(BiomeGenBase.getBiomeGenArray()), Predicates.notNull()), BiomeGenBase.class); // savanna EntityRegistry.addSpawn(EntityLion.class, 6, 1, 5, EnumCreatureType.creature, BiomeGenBase.savanna); //change the values to vary the spawn rarity, biome, etc. EntityRegistry.addSpawn(EntityElephant.class, 10, 1, 5, EnumCreatureType.creature, BiomeGenBase.savanna); //change the values to vary the spawn rarity, biome, etc. // savannPlateau EntityRegistry.addSpawn(EntityLion.class, 6, 1, 5, EnumCreatureType.creature, BiomeGenBase.savannaPlateau); //change the values to vary the spawn rarity, biome, etc. EntityRegistry.addSpawn(EntityElephant.class, 10, 1, 5, EnumCreatureType.creature, BiomeGenBase.savannaPlateau); //change the values to vary the spawn rarity, biome, etc. } But if you want to spawn in more specific place that isn't already identified as a biome it sort of depends on your ability to find the conditions then use the standard way of spawning in the world. Basically if you can identify a position to spawn then you can use following to actually spawn: if (!world.isRemote) { System.out.println("Spawning on server side"); String fullEntityName = WildAnimals.MODID+"."+entityName; // put string for your entity's name here if (EntityList.stringToClassMapping.containsKey(fullEntityName)) { Entity conjuredEntity = EntityList.createEntityByName(fullEntityName, world); conjuredEntity.setPosition(locationX, locationY, locationZ); // put the location here that you want world.spawnEntityInWorld(conjuredEntity); } else { System.out.println("Entity not found"); } I'm not really sure how you'd identify a mine shaft as the location though, but maybe others have suggestion. -
[SOLVED] [1.7.2] The Gui needs to read something from the server
jabelar replied to TheDav1311's topic in Modder Support
In Minecraft there are networking packets that go between the client (GUI side) and server. This actually happens even in single-player mode. For the built-in Minecraft and Forge there are several packet systems already in place. TileEntity uses packets to sync information, the data watcher uses packets, containers use packets, chat uses packets, commands use packets, etc. The problem is that if you're not using one of those then you have to send your own custom packets. The currently recommended way to do this is to use the simple network wrapper that has recently been fully implemented in Forge. You should use diesieben07's tutorial to set this up in your mod: http://www.minecraftforge.net/forum/index.php/topic,20135.0.html It is a bit of work, but it is worth doing. You'll find many times in modding when you need to sync client and server with custom packets. -
[1.7.10] Child Parts Of Model Unable To Rotate
jabelar replied to qpwoeiruty's topic in Modder Support
In Java you can rotate both parent and child. But the child rotation is "relative" meaning it adds to the parent. So if parent is rotated 60 degrees and child is rotated 10 degrees the child will actually render as rotated by 70 degrees. This is a good thing because it means that you can move the children while also ensuring they move with the parent. Anyway, your code only shows rotation of the body part which doesn't seem to even be the child, plus youd didn't answer our other questions. -
[1.7.10] Child Parts Of Model Unable To Rotate
jabelar replied to qpwoeiruty's topic in Modder Support
To bug this sort of thing, you just need to trace your code. At each important point in the code add your own console debug statement. For example, in your code the rotation of the body seems to be set by the moveTimer. But what is the value of the moveTimer? Are you sure it isn't 0? To check, just put System.out.println("moveTimer ="+moveTimer) inside the check for sitting. Also, are you sure you want par3? And what is its value at that point? Beyond that, you aren't really overriding the setRotationAngles() function. It looks like you're just calling the default super function. Are you sure that will give you the effect you want? And are you sure it isn't overriding your control of the rotationX? Anyway, you need to learn to debug this sort of problem yourself. Just put console statements at each important part of the code and see if the values are what you expect. You will quickly find a place where it isn't what you expect and then the answer is usually pretty clear to see how to fix. -
The problem is that you haven't used a "child" relationship between parts, so they won't stay connected (unless you do a lot of math to re-position them). The vanilla Minecraft biped model has only one part for each arm and each leg so it doesn't have to worry about this. As soon as you make it multiple parts you can't just copy the way Minecraft does it -- instead you have to organize your model into child parts. The ModelRenderer had a method for this called addChild(). You can add children to children as well. The way you should organize it is that the top of the leg should be the child of the body, then the middle of the leg a child to the top of the leg, the bottom of the leg a child to the middle of the let, etc. Then if you rotate the top of the leg, all the other parts will rotate properly with it. I have a tutorial on this: http://jabelarminecraft.blogspot.com/p/complex-entity-models-including.html The difficult part is that the children have a position that is relative to the parent, rather than to the overall model origin. So after you add the child you will need to adjust the rotation point by subtracting the parent's rotation point. Once you have the model properly set up with child parts, animation becomes much easier. You can do things like wave the hand while also raising the arm and it will all work together. Hope that helps.
-
I don't think you need NBT. NBT is important format for custom values you want to save, but for something like cooldown in the middle of a teleport you only need a temporary value (I mean you're not going to save your game right in the middle of teleporting). You just need a boolean field called something like isTeleporting to mask off the further teleporting.
-
[1.7.10] Can't Find the Source Code in Eclipse Package Explorer
jabelar replied to benloz10's topic in Modder Support
The problem is you used setupDevWorkspace. That only provides the method and class names (still useable) but doesn't attach the source. You need to use setupDecompWorkspace if you want to view the source. -
my int is resetted after closing minecraft in eclipse
jabelar replied to knokko's topic in Modder Support
To explain the basics a bit more -- Minecraft will only save the stuff it expects to save (in the save game files) unless you add things to save. A field that you just create in your code will not save automatically since that is never true for programs unless you specifically save and load them to some file. You're not saving your field to a file, so why would you expect it to be saved? Forge has various methods to help you save. For example if you're adding values to any entity, including the player, you can use the extended properties which will be saved and loaded for you (if you set it up right). The tutorial that knokko linked uses this and in fact I think the example used is mana for a player so should be perfect for your case. -
To add to diesieben07's suggestion, note that there is field in the passed event called source that is of type called DamageSource for which DamageSource.FALL represents fall damage. Like many things in programming there are multiple approaches to solving some problems. There are multiple hooks related to damage and you can take the perspective of trying to control it in the "attack", the "fall", or the "hurt" processing. The attack is probably a good point because it happens before the hurt so is a clean hook in this case.
-
I think you can cancel the LivingFallEvent if you see that the livingEntity is the player. The hurt effect is implemented together with the attackEntityFrom() method that checks for things like invulnerability and such and I think there is a field called the hurtTimer or something like that. I'm not clear from your question if you actually want to hurt the entity, or just make it look hurt. If you just want it to look hurt then you'd have to handle the render event and blend in red while keeping track of a timer to control how many ticks.
-
I'll still try to help you in the PM messages since you've been trying to follow my tutorial. I've already sent you the first replies there. I just wanted to set your expectations that you need to get strong in Java to continue further.
-
I have an explanation of this in my entity animation tutorial: http://jabelarminecraft.blogspot.com/p/complex-entity-models-including.html I also give a recommendation on how you can use arrays (containing the part rotations) to manage complex animations. Short answer though is that whenever the parent part is rotated or moved, all the children (and children of the children, etc.) are moved with it. If you rotate or move the child, it is *relative* to the parent. So if the parent is rotated 90 degrees and you rotate the child 10, then the child will actually be rotated 100 degrees. This is a good thing because it means you don't have to do any trigonometry to keep all the children attached. For example, if you have an arm with a hand and the hand has fingers, you'd make the hand the child of the arm and the fingers the child of the hand. Then if you just move the arm you don't have to do anything to move the hand and fingers with it (they'll all stay connected), but if you decide to wiggle the fingers you can just rotate them. The only other important point is that if you render the parent it will render all the children. You should *not* render the children or it will create very weird duplication.
-
It is okay to be a beginner in Java, but you're too much a beginner to try modding. The difficulty with modding is that you're trying to modify someone else's code (Minecraft and Forge) and so you have to know Java quite well to do anything significant. If you don't know how to even initialize instances, how to use the static denotation, how constructors work, and so on, you simply don't know enough Java to even get started. Even people like me, who is more intermediate in Java, can still get stuck very often because modding is also about figuring out the code that already exists. If I wrote the whole code myself, then I probably wouldn't have trouble, but you have to figure out all the weird ways that Minecraft and Forge work. Things like the lifestyle events (like "preInit" that diesieben07 is talking about), or the sided proxy system, or the way blocks are tiled without being individually instances, or the way metadata works, and so on aren't related to learning Java but are about learning how Minecraft and Forge works. if I'm trying to figure out how to make a "key up" event I had to spend a couple days reviewing the code, trying different things, asking questions and then eventually giving up because even the experts said that the solution required modification to the Forge code source. So we're not trying to be mean when we tell you you need to learn more before modding, just practical. To learn Java, I recommend actually reading a real book (not online) because most people that try online programming only pay attention to the topics they think are important but a book will ensure you cover all the topics. There are so many details in a programming language including differences from other programming languages you may know (like in Java it is a mistake to use == to compare Strings, or in Java enum can't be used as a array, or in Java a multi-dimensional array can be jagged, or that object parameters passed to a method access the actual object instead of a copy, and so on). The really big programming books like Java For Dummies are a little scary because they are so big. I recommend Java in Easy Steps because it is a slim little book but covers all the basic topics in a easy to read manner. If you do want to continue with modding, I suggest you stick with the following things that don't require much programming skill: - making new recipes (that use vanilla items) - making very simple extensions of vanilla items (e.g. make a custom entity that extends a vanilla one and only change a couple simple things like the health, sounds and texture). A crop isn't quite simple because it has interaction between a custom item (the seed) and custom block (the plant) which can be harvested for a custom item (the fruit). Note you can also have a lot of fun modifying Minecraft without doing any programming. My kids spend a lot of time making texture packs and skins that radically change the look of Minecraft. You can also use command blocks to create some really interesting things including minigames.
-
I think the ServerConfigurationManager has this information. You should be able to check via a method in MinecraftServer.getServer().getConfigurationManager(). However, the method has changed over various versions of Forge. For a while it was isOp(), then it was isPlayerOpped(). For 1.7.10 it seems to be func_152607_e(). Anyway, I'm not sure on 1.7.2 since I'm not running it at the moment, but just look through all the methods in the ServerConfigurationManager class to find the right one.
-
Not sure if it helps but there is also the LivingFallEvent which can be canceled to set fallDistance to 0. Not sure if that also prevents the hurt animation, but I think it will because of the following. The hurt animation is related to the attackEntityFrom() method. If you look in EntityLivingBase there is method called fall() and that is where it triggers the LivingFallEvent (and passes fall distance), plays the hurt sound and also invokes the attackEntityFrom() with a DamageSource.FALL. If you cancel the event, it won't play the sound or invoke the damage.
-
Is this with 1.7.2 or with 1.7.10 (note the latest forge versions are 1.7.10)? For 1.7.10 you need to add some more arguments to the run configuration "--userProperties={} --assetIndex 1.7.10 --assetsDir C:/Users/Aaron/.gradle/caches/minecraft/assets" otherwise you'll get a sound error like what you're describing.
-
[1.7.2] KeyHandler to KeyEvents, how to replace keyUP() method?
jabelar replied to AXELTOPOLINO's topic in Modder Support
yeah, I could tell I was getting into dangerous territory. I think I'll try polling the keybindings instead and see if I can fire a useful key up event from there. But out of interests' sake: is there a way to do reflection on fields in a non-instantiated class? -
[1.7.2] KeyHandler to KeyEvents, how to replace keyUP() method?
jabelar replied to AXELTOPOLINO's topic in Modder Support
Okay, I'm stuck on the reflection already -- I'm not sure what the instance of the Keyboard class really is. It seems that there is no constructor or any call that instantiates it. Instead it creates its own "implementation" instance of InputImplementation but that is also a private static field within the class. Diesieben07, you're good at reflection -- is there any way to use reflection on this readBuffer field in Keyboard class? Without an instance to reference I'm not sure how to use reflection... -
[1.7.2] KeyHandler to KeyEvents, how to replace keyUP() method?
jabelar replied to AXELTOPOLINO's topic in Modder Support
Okay, so I just tried the following: @SubscribeEvent(priority=EventPriority.NORMAL, receiveCanceled=true) public void onEvent(ClientTickEvent event) { while (Keyboard.next()) { System.out.println("Keyboard event detected"); FMLCommonHandler.instance().fireKeyInput(); } } And it "nicely" detects both key down and key up events. However, it seems to entirely override any other keyboard processing by Minecraft (even ESC no longer works!). Basically, (like I suspected when asking my first question) that iterating through the Keyboard KeyEvents removes them from the queue and so they no longer get processed by rest of Minecraft. So the problem with creating your own key up hook is figuring out how to do it without blocking vanilla keyboard processing. One way might be to put the stuff that is read from the Keyboard.readBuffer back. There is a readNext() method that is private that is called by the public next() method, and readNext() just reads out the values from the buffer. You could probably use reflection (right) to access readBuffer and put back what was just read out. I suspect that would allow vanilla keyboard processing to continue. The other method would be to recreate all of the Minecraft class' keyboard processing but I suspect that would be difficult as it would likely run into issues with private fields/method calls and also potentially would change the sequence of processing in a way that might muck up actual game. I think I'll play around with using reflection to restore the readBuffer as it seems like a fairly possible way to make the KeyInputEvent hook extend to include key up. -
[1.7.2] KeyHandler to KeyEvents, how to replace keyUP() method?
jabelar replied to AXELTOPOLINO's topic in Modder Support
My point about polling and creating own events was about augmenting/replacing the FML hook event. In other words, by accessing Keyboard class KeyEvent directly you can detect both key down (including repeat it seems) and key up. So can't you poll and then fire your own events for KeyUp? A question about polling performance. On the one hand doing something every tick seems like a performance burden, but of course the built-in code is already doing a lot of such polling. Is the built-in code performance optimized in some way? It just seems to me that polling, while a bit heavy, is something that computer should still be able to handle fairly well -- just poll KeyBoard.next() and fire off a KeyUp custom event as needed.