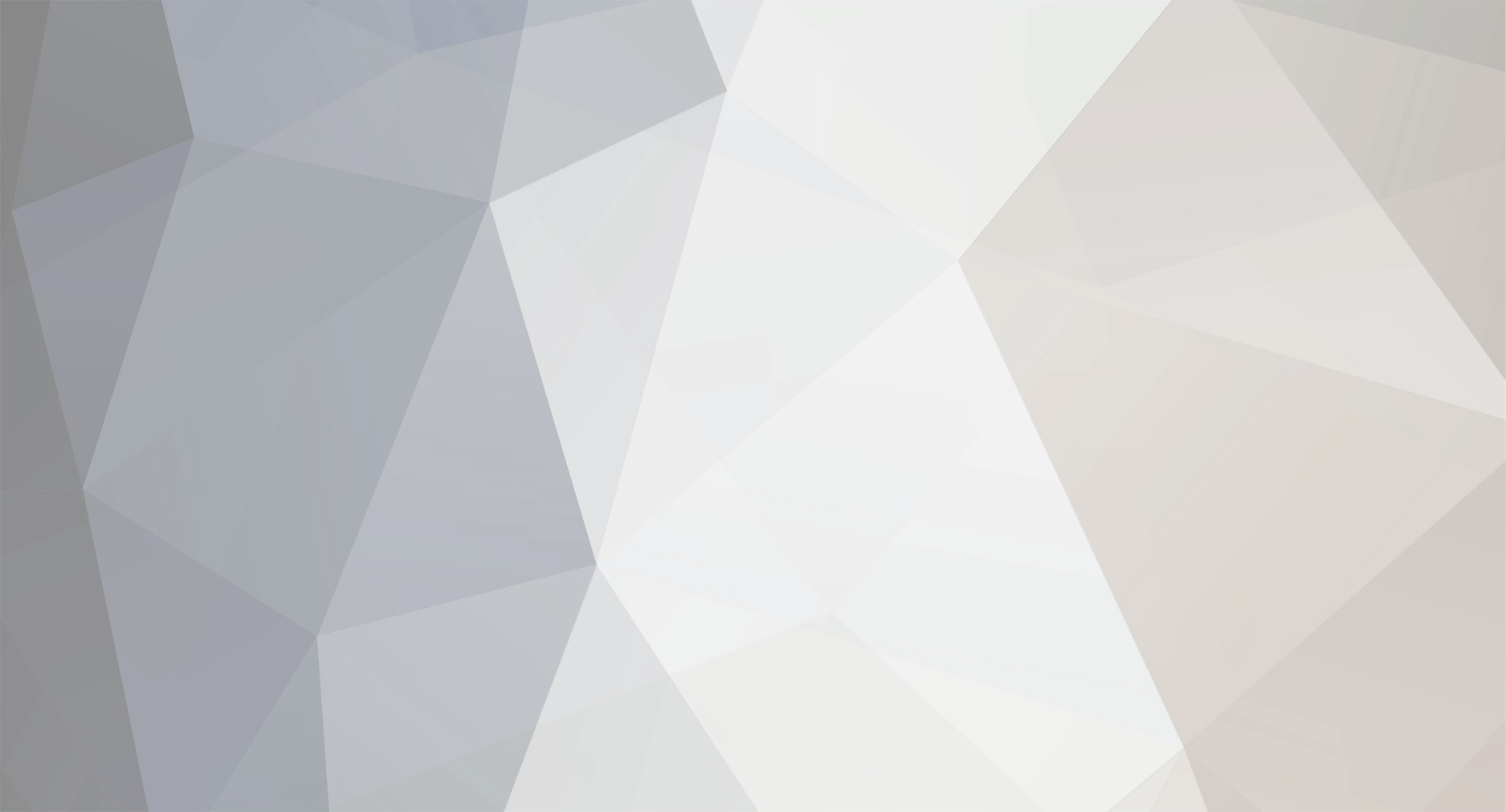
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
I usually open up a browser this way. Create a class like: import java.awt.Desktop; import java.io.IOException; import java.net.URI; import java.net.URISyntaxException; import net.minecraft.client.gui.ScaledResolution; public class Browser { public static void browse(String parUrl) { // Create Desktop object Desktop d=Desktop.getDesktop(); // Browse a URL, say google.com try { d.browse(new URI(parUrl)); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (URISyntaxException e) { // TODO Auto-generated catch block e.printStackTrace(); } ScaledResolution sr; } } Then you can open browser with: Browse.browse("http://www.google.hu");
-
Yes, the GL11 scaling will work fine for this. Note though that it will not increase the collision box for the entity so I usually also adjust that. Lastly, the scaling for the entity happens on the client side and often the server needs to know (i.e. for pathfinding) that the size (and collision box) have changed, so you probably need some sort of packet to sync client and server. I usually have a scaleFactor float field in my entities and then use that (and sync that).
-
[1.7.2] KeyHandler to KeyEvents, how to replace keyUP() method?
jabelar replied to AXELTOPOLINO's topic in Modder Support
Yeah, I was looking at this too last night. Furthermore the FML KeyInputEvent event parameter passed could have more useful fields. It seems that polling Keyboard directly and maybe firing custom events (or just processing at the time) is more generally useful, although ideally you'd want to implement the key binding as well to allow user mapping. One question about the Keyboard.next() is: does invoking this method clear the list of key events from Keyboard? I mean if you call next() do you then have to fully process it or will it still work as expected in addition to anything you might do? Another question: Regarding the "key up" detection, doesn't the getEventKeyState() method do this? From the comment description for the method, it seems that if Keyboard has a key event where getEventKeyState() returns false that means that the key was released. -
[1.7.2] looking for event when NetherPortal is Used
jabelar replied to Jacky2611's topic in Modder Support
In the event handler you could possibly check more information to figure out what caused the change. You could also have your teleporter set a flag to indicate it was activated. -
[1.7.2] looking for event when NetherPortal is Used
jabelar replied to Jacky2611's topic in Modder Support
I think the PlayerChangedDimensionEvent might be useful for this. -
[1.7.10] Printing Custom Name To Chat Problem
jabelar replied to qpwoeiruty's topic in Modder Support
A couple comments: First of all, if you have an else statement you don't need to test the condition again (you shouldn't need the second if because that is what else already does). Secondly, I don't think that the custom name will be null if there is no name, it might be an empty string instead "". So you might have to test for empty string, or test if the length of the name is 0. I believe this is your problem (that the name isn't actually null when there is no name). Thirdly, I don't think you need the empty string in your chat messages. I could be wrong, but I don't think you need the "". Lastly, with string values you usually shouldn't use == to test them in Java. Instead you should use the equals() method for strings. Anyway, try something more like this: if(this.func_152114_e(entityPlayer) && entityPlayer.isSneaking() && !this.worldObj.isRemote) { if(this.getCustomNameTag().length > 0) { entityPlayer.addChatMessage(new ChatComponentText(EnumChatFormatting.GOLD + this.getCustomNameTag())); entityPlayer.addChatMessage(new ChatComponentText(EnumChatFormatting.GOLD + this.currentXP)); } else { entityPlayer.addChatMessage(new ChatComponentText(EnumChatFormatting.GOLD + this.currentXP)); } } -
WTF dude. Learn some java, then you don't have to do shit like this. Diesieben07, actually that comment is mine (from my tutorial). It isn't a comment related to Java, I'm just saying you don't have to create animation textures for all 8 stages. Basically it is to save effort on the artwork of the textures. For example I found that having 4 textures still give fine effect of growth (i.e. you put bonemeal on and it grows).
-
[1.7.2] KeyHandler to KeyEvents, how to replace keyUP() method?
jabelar replied to AXELTOPOLINO's topic in Modder Support
While it is good practice to avoid any extra processing every tick (due to the processing burden), the reality is that the code is already doing tons of stuff every tick and a simple event handler that is updating some boolean flag isn't going to break a modern computer. I agree it would be better to have an built-in (i.e. performance optimized) keyUp type event but I wouldn't be so loathe to implement it yourself. A pain yes but hardly a performance killer. -
Entities with collision box and/or light effect
jabelar replied to Bedrock_Miner's topic in Modder Support
Is this entity supposed to move positions at all? If not, then I think what you want is an actual block with a TileEntity attached. You can use the TileEntity to handle any cool stuff like animation, damage and even counter attacks, I guess the question is whether you're creating something more block-like (i.e. pathfinding considers it an obstacle and it can emit light) or more entity like (it moves around). -
My tutorial isn't advanced. And you have to do all the steps in my tutorial, you cant really simplify further. Lastly text tutorials are good because they have code examples. Anyway to make a crop you need a seed item and a plant block.
-
I have a tutorial on custom crops at: http://jabelarminecraft.blogspot.com/p/minecraft-forge-172-creating-custom.html?m=1
-
You have to spawn the entity on the server side. I think the chat event is probably on client side.
-
Modifying tooltips not working server sided
jabelar replied to 61352151511's topic in Modder Support
Can you explain why you want to do tooltips on server side? Tooltips are related to GUI because it is about rendering something graphical. So by definition it is pretty much a client only thing. -
To animate you need to be able to control the rotation and location of pieces within the model. In the Java models each block is named and can be controlled independently. Furthermore you can create a hierarchical model that allows for relative rotation and positioning. For obj and wavefront I don't know if you have that control. It is probably possible to create similar effect with multiple models (eg you could have one obj model for body, one for each limb, etc.
-
[SOLVED][1.7.x] Bug in FML, or am I just not understanding?
jabelar replied to coolAlias's topic in Modder Support
I was going to ask same question as coolalias but am on my phone so couldn't write up examples as nicely. At what point does Java hang up on missing (ie due to sided proxy) classes. I guess the import itself might fail? Or just when you try to access the class? I thought that just checking isRemote (or otherwise being sure that code was only being called on one side) could protect you from running client only code on server. Or does Java runtime try to resolve it all even for paths it might not execute? -
Overriding vanilla minecraft armor textures in a mod
jabelar replied to theOriginalByte's topic in Modder Support
I think that if you're just changing the texture you don't need to make a mod at all. Minecraft textures are kept in accessible folders and you can just replace the assets with your own without any coding. Look up how to make a texture pack (also called resource pack). -
Some things in the log that got my attention...
jabelar replied to latias1290's topic in Modder Support
These are normal and just due to the "hacking" nature of mod development -- you're not using a legit minecraft when you're in the development environment. So the minecraft that runs in dev environment throws some errors because it is skipping the actual player login and such. When you actually publish your mod it will run against a legit minecraft jar. -
Latias, the point is that it sounds like forge IS working for you. Your grade recognizes previous install and skips unnecessary steps, you have the skeleton example mod, you have the mcp deobfuscation data and you have the referenced libraries for minecraft and forge. Not sure why you're maintaining that it isn't working.
-
[1.7.2] How to check if player is in creative mode
jabelar replied to Electrobob99's topic in Modder Support
How do you know it isn't working? Is it crashing with an error? If you're debugging an if statement just add console statements before and after to print out the value of the expression you're testing then confirm that the code is taking the path you expect. If it is crashing then post the error messages. Otherwise just trace the execution with console statements and you should quickly find any problem. -
For the static entity data i think there is an interface called something like IEntityAdditionalSpawnData you may want to check out. For dynamic entity data you should use a custom packet to sync. The key is to pass the entity id as an integer in the message payload. I send a sync from server to client that works like this: you send the packet from the entity class whenever a custom field you want to sync changes. you get the entity ID by simply using the getEntityID() method and write that int into message payload. Then on client side when you receive the packet read that int and use Entity foundEntity = getEntityByID(entityID, theWorld); That id should work to identify the right entity on client based on ID sent by server in the sync packet. Hope that helps.
-
He didn't ask for shortest distance, he asked for shortest path. That isn't necessary the same thing. For example in a dungeon the closest could be behind a wall but needs long path to get to.
-
Java is bit weird as it treats primitive types different from objects. In this case the difference is that for object fields the memory isn't allocated until the object is instantiated (I. E. By using new keyword. Since you passed the field before you instantiated it then the block pointed to in your seed was pointing nowhere. When you later instantiate the block it doesn't update the seed because that references different memory location. In other words the block gets updated someplace the seed isn't pointing to. So yes it tried to plant a null object because the seed was pointing to null.
-
It isn't hard to make a custom AI class. Just copy the one that is closest and change what you need and add it to the list instead.
-
I want to make sure you understand why the crash didn't happen during the initialization -- basically the seed constructor doesn't prevent you from passing a "null" block. That type of "null" passed as parameter is legitimate (not a null pointer exception) at the time it is passed. So only becomes a null pointer exception later when you actually try to plant (place that null block).
-
This event was just added to Forge, so your friend probably didn't have the correct release of Forge. I needs to be something like 1133 or later (if I remember correctly, you can check the release notes)