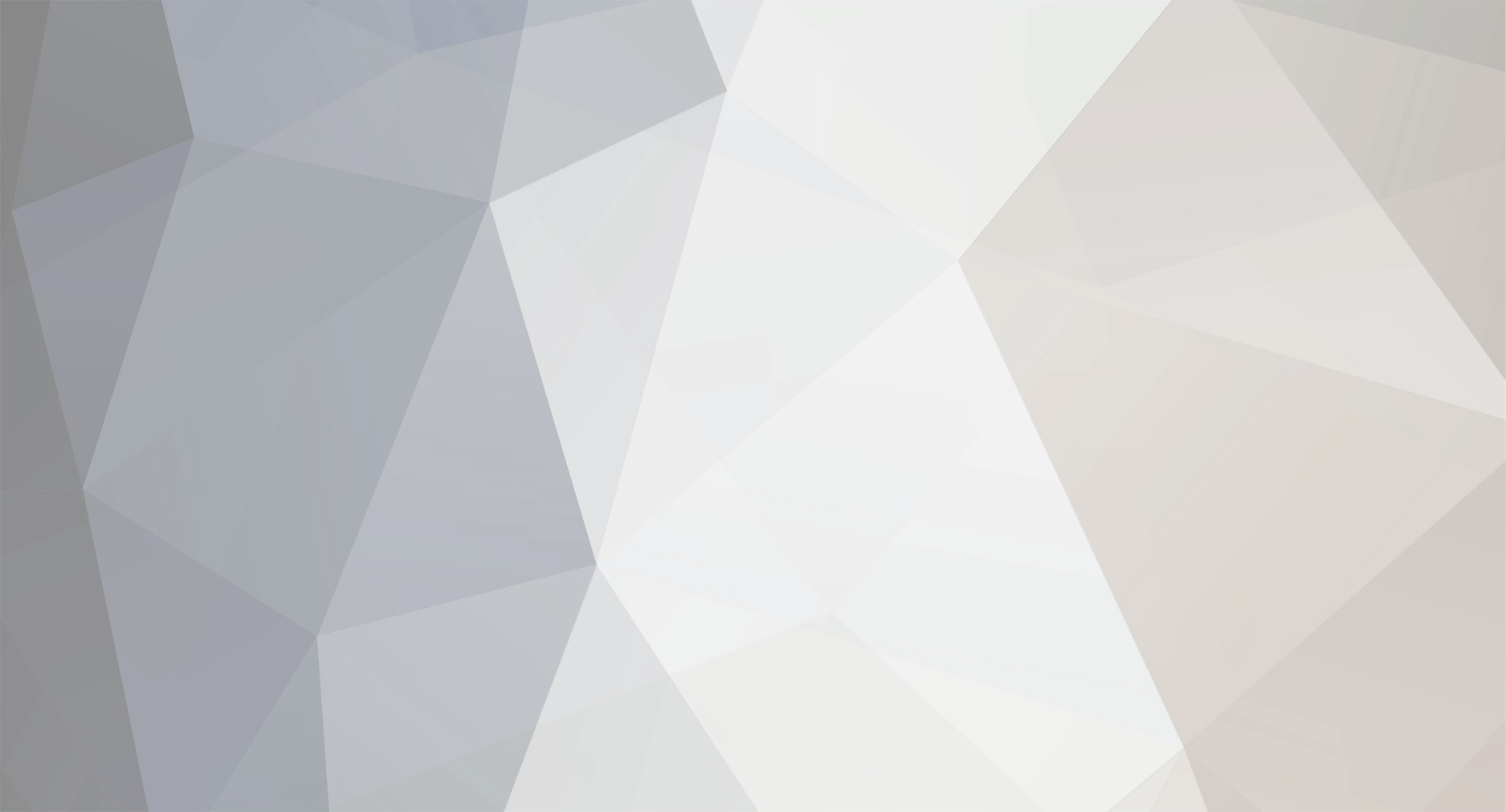
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
[1.7.10] Changing mob stats without using IExtendedProperties
jabelar replied to Thornack's topic in Modder Support
The entity classes have writeEntityToNBT() and readEntityToNBT() methods. So for your own custom entities you can use those to store custom per-entity information. IExtendedEntityProperties works similarly, but is really more useful for adding properties to vanilla enitities since you already have the above methods available in your own custom entities. -
Whenever you want to modify vanilla functionality, I find it is best to consider things in this order: 1) Look for public fields and methods in the class. For example, entity AI list is public so you can totally change the AI of vanilla entities. 2) Use events. Events are exactly for intercepting vanilla processing and there are events for most of the common stuff people like to mod. I have a tutorial on events here: http://jabelarminecraft.blogspot.com/p/minecraft-forge-172-event-handling.html. In your case, this is the way to go -- handle the HarvestBlockDrops event as suggested by diesieben07 3) Use Java reflection to access fields and methods that are not public. Reflection isn't actually that difficult. 4) Consider replacing vanilla classes with ones that extend that class. This can be a bit of work and possibly can affect compatibility with other mods, but sometimes makes sense. 5) Use ASM or other more advanced techniques for actual circumvention of vanilla code.
-
Modelling, is there anything better than Techne?
jabelar replied to jordsta95's topic in Modder Support
I agree. The Java in the entity models is simple: just a bunch of blocks. So you can actually just draw your entity on graph paper and then code in the positions as quickly and with less aggravation of using Techne. -
It is possible something is just wrong with the rendering or position of the block when placed. However, the built-in crops have some things to consider. Interestingly, other blocks may have a canSustainPlant() method and that checks for the plant type. So you can quickly run into trouble if you make a custom block type that you want to plant on different blocks than usual. In other words, your ability to plant on a block is actually also dependent on the other block. I've recently found that if I want to do any unusual custom crops that I tend to no longer implement IGrowable, but just make my own code to handle it all. Anyway, my only other suggestion is you should trace through the code by putting in useful System.out.println() statements at each point. That way you can tell what code path is being followed and if that matches your expectation.
-
[1.8]Game crashes when I use bonemeal on a custom crop
jabelar replied to The_Fireplace's topic in Modder Support
Is that due to the field being final? If you named it differently and override the createBlockState() method to only work with your property that would be okay, right? -
Are you trying to plant in dirt or grass? or farmland? Can you show your code for registering the item seed and plant block? Generally the code looks like it should work, but it looks like it will only plant on dirt or grass and also with item seeds it is important to construct it to properly reference the plant block. One other possibility is that maybe it is placing the plant but it is not rendering? Do you have a custom texture?
-
I also have a tutorial on custom crops here: http://jabelarminecraft.blogspot.com/p/minecraft-forge-172-creating-custom.html
-
Oh. That isnt what I want.I actually want it at like 10 fps. I could just check whether 12 frames have passed then run my frame, until the end. Would that be right? A tick is already at 20 fps, so like diesieben07 says you should use the tick method for consistency. You can check if 2 ticks have passed if you need 10fps. The frame rate is configurable and will depend on device and user settings, so you don't want to depend on that for timing.
-
It is important to remember that Minecraft and Forge are in Java, so pretty much anything that is possible in Java is possible in a mod. So don't restrict your google searches to Minecraft topics, but look for Java explanations. However, regarding video in mods I would suggest you consider other alternatives, since the video can make the file big and it may be a lot of work to get it working correctly. Why do you want video? If it is for cut-scenes, I'd say that doing more of an animation (series of still images) is a lot easier to code. If you want the video to provide help, maybe just link to a YouTube video instead. But if you really need in-game video for your mod, start by figuring out generally how to do it in Java.
-
[1.7.10] Trying to make water render behind entity.
jabelar replied to Eternaldoom's topic in Modder Support
I ran into a similar issue once. I think I solved it with some combination of the following in the Renderer: GL11.glEnable(GL11.GL_BLEND); GL11.glDepthMask(false); GL11.glEnable(GL11.GL_ALPHA_TEST); -
You don't have to look at the list of all entities in the world. World already has a method called getEntitiesWithinAABBExcludingEntity() that gets only the entities in region you specify. And regarding the original question, why are you looking for a "better" way. Your way is already as simple as it can be -- some code is going to have to execute and modify the motionY for all the entities, so you can't get around that.
-
[1.7.10] Does an entity animation reset when you set a new rotation yaw?
jabelar replied to Frag's topic in Modder Support
Most people do their animation in the Model class, so can you post the code for that? Basically you need to look at your code for the rotation of the tail part of the model. I suspect that you forget to add yaw to rotation so it isn't rotating with the model, but maybe I don't quite understand what you're describing. Anyway, I'm sure the answer is in the angle calculations in your Model class. -
[SOLVED][1.8] With built JAR, it crashes with NoClassDefFoundError
jabelar replied to jabelar's topic in Modder Support
Okay, I think I solved it. At least I got it working on a different computer just fine. I think I used the same installer, but some reason on the original computer, the launcher profile references fml-1.8-8.0.20.1023 but with the working computer I just tried the profile references 1.8-Forge11.14.1.1333. Anyway, that explains it -- mismatch with the profile. -
[SOLVED][1.8] With built JAR, it crashes with NoClassDefFoundError
jabelar replied to jabelar's topic in Modder Support
I'm in process of updating my game to 1.8, so there is still some cleanup to do. That field was just the unlocalized name really, but I was doing some conditional stuff on it (wouldn't always just be name of the item) so didn't want to mess with actual unlocalized name. Anyway, I removed that and the NoClassDef problem remains. (Note I really don't think a problem with some string field could be cause of NoClassDef problem for loader, but I appreciate you pointing this field out.) Class now looks like: Note I was also suspicious of the StatCollector class, so I tried the ItemMagicBeans with a simple return ("Magic Beans") and it also fails with NoClassDef error. -
[SOLVED][1.8] With built JAR, it crashes with NoClassDefFoundError
jabelar replied to jabelar's topic in Modder Support
You're welcome to look at the whole codebase here: https://github.com/jabelar/MagicBeans-1.8fixed Here is the ItemMagicBeans class. Nothing special: package com.blogspot.jabelarminecraft.magicbeans.items; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.item.ItemStack; import net.minecraft.util.StatCollector; import com.blogspot.jabelarminecraft.magicbeans.MagicBeans; import com.blogspot.jabelarminecraft.magicbeans.utilities.MagicBeansUtilities; public class ItemMagicBeans extends ItemSeedFoodMagicBeans { private static final ItemStack p_77653_1_ = null; public ItemMagicBeans() { super(1, 0.3F, MagicBeans.blockMagicBeanStalk); setUnlocalizedName("magicbeans"); setCreativeTab(CreativeTabs.tabMaterials); } @Override public String getItemStackDisplayName(ItemStack parItemStack) { return (MagicBeansUtilities.stringToRainbow(StatCollector.translateToLocal(getUnlocalizedNameInefficiently(p_77653_1_) + ".name")).trim()); } } Also note that this mod works when running from Eclipse. I think the NoClassDef error can be related to class path problems, but I don't really know what exactly would be wrong. -
[1.8] Model definition for... not found? (Texture won't load)
jabelar replied to Kander16's topic in Modder Support
that json would make every stage of the growth use the same texture. Is that what you want? Usually for crops you'll have a bunch of images to animate the growth and so your JSON would reference corn_block_0, corn_block_1, and so on. Also, the "age=" part implies that you have Property for the block states that is actually called "age". So make sure you have that in your class. -
[SOLVED][1.8] With built JAR, it crashes with NoClassDefFoundError
jabelar replied to jabelar's topic in Modder Support
Also, the class mentioned com/blogspot/jabelarminecraft/magicbeans/items/ItemMagicBeans is in the JAR: [/img] -
[SOLVED][1.8] With built JAR, it crashes with NoClassDefFoundError
jabelar replied to jabelar's topic in Modder Support
main mod file: -
[SOLVED][1.8] With built JAR, it crashes with NoClassDefFoundError
jabelar replied to jabelar's topic in Modder Support
I'm not using reflection at all in this mod. -
My mod runs great in Eclipse, but if I gradlew build and then use the resulting JAR in the actual Minecraft install it crashes. Using the new launcher, I can play vanilla 1.8 okay, and I can play Forge 1.8 okay (suing profile for fml-1.8-8.0.20.1023) okay. But if I put my JAR in the mods folder it crashes with the following exception. I don't find the console messages that helpful, as it just mentions a NoClassDefFoundError for a class that works fine when running from Eclipse and then gives trace to fairly general classloader methods.
-
[1.7.10] What are my prospects for learning to mod?
jabelar replied to ravingmadlunatic's topic in Modder Support
You need an IQ of 129 to mod, so sorry no. Seriously, I think modding is not necessarily the best way to learn programming and you definitely need to learn programming to mod. The reason I say modding isn't great way to learn programming is that it is very difficult to follow other people's code, especially when it is not documented. Modding is even worse because the code we're trying to work with was sort of reverse-engineered -- it is not even properly published for sharing. Also, Minecraft is already a full-fledged game with a lot of complexity. Secondly, there are a lot of very specific concepts in programming that need a detailed understanding that comes from directed learning (i.e. a course). Just figuring out difference between an int, double, and float takes some discussion, let alone how loops, conditional statements, etc. work. Only grounds up programming as part of directed study can really give you a strong confidence in such topics. However, it sort of depends on your ambitions. As long as your expectations are in line with your skill, then go ahead and play around. Any smart person can make a simple mod -- like make a new variation of an item or armor. But I often find people with no programming skill want to do major modifications that would be a struggle for even more experienced modders. So just set your sights modestly and you will have some satisfaction. Lastly is personality. You need tons of patience and ability for very deep concentration. The tone of your question actually makes me think you DO have the right personality for programming. So my suggestion is (a) get a good book on learning Java programming, (b) play around with a modest mod attempt. By the way, I highly suggest an actual book instead of online learning. The reason is that with online learning people tend to skip around and jump ahead. A good book will lead you more specifically through the progression. I recommend Java in Easy Steps by Mike McGrath because it isn't too scary (many programming books are like encyclopedias and can be daunting, but this book is a friendly introduction to Java that covers all the important stuff). -
[1.8] The kind of change that can really mess up porting mod to 1.8
jabelar replied to jabelar's topic in Modder Support
To me this is always the risk with a pseudo-API that exposes its internals, you can't rely on the contract remaining stable (often can't even tell what the contract is). Personally I tend to assume that, if a Forge method has JavaDoc, it will probably remain stable. Otherwise, better not touch it, or at least make sure your automated test cases verify it hasn't changed. Like the Minecraft code really. -TGG Yeah, this is a better way of stating what the point of my warning is: many of us are relying on an "API" that isn't really an actual supported/stable API so watch out and verify at least a couple levels into the call hierarchy to make sure it still does what you think it does. -
[1.8] The kind of change that can really mess up porting mod to 1.8
jabelar replied to jabelar's topic in Modder Support
In a custom entity I am overriding the attackEntityFrom() method. In order to be compatible with other mods (or even other stuff I might do later in the mod) I want to fire the event just like vanilla entities do and check if the event EventLivingAttack has been canceled. Basically something like this is the first few lines of the method: @Override public boolean attackEntityFrom(DamageSource par1DamageSource, float parDamageAmount) { // allow event cancellation if (ForgeHooks.onLivingAttack(this, par1DamageSource, parDamageAmount)) return false; I thought it was good practice to post events like vanilla classes do, and also check for cancellation. And I guess I mistakenly thought the idea of forge "hooks" was that they were there to use. So should I just call MinecraftForge.EVENT_BUS.post() directly? Usually when I'm modding I look at the way the vanilla classes implement things and copy it where it works for me. Other entities call onLivingAttack() so I did too. Is there any real problem with doing so? -
Just a warning to those porting there mods: there are cases where the behavior of functions has been changed substantially, possibly as new bug. Beware the onLivingAttack hook for posting the living attack event now returns false if event is canceled (instead of true in 1.7.10). Here is the 1.7.10 code from ForgeHooks class: public static boolean onLivingAttack(EntityLivingBase entity, DamageSource src, float amount) { return MinecraftForge.EVENT_BUS.post(new LivingAttackEvent(entity, src, amount)); } Here is the 1.8 code from ForgeHooks class (note the return value is negated relative to 1.7.10): public static boolean onLivingAttack(EntityLivingBase entity, DamageSource src, float amount) { return !MinecraftForge.EVENT_BUS.post(new LivingAttackEvent(entity, src, amount)); } I think this is a bug really, since all the other event hooks still return true if the event is canceled. Anyway, I'm not so much telling you about this specific change but rather a general warning to really double-check all the functions you're calling to see that they're behaving as you've previously expected.
-
I think breaking it up is probably what you have to do. Otherwise I think you'd have to get into the low level networking and create a streaming connection socket or something.