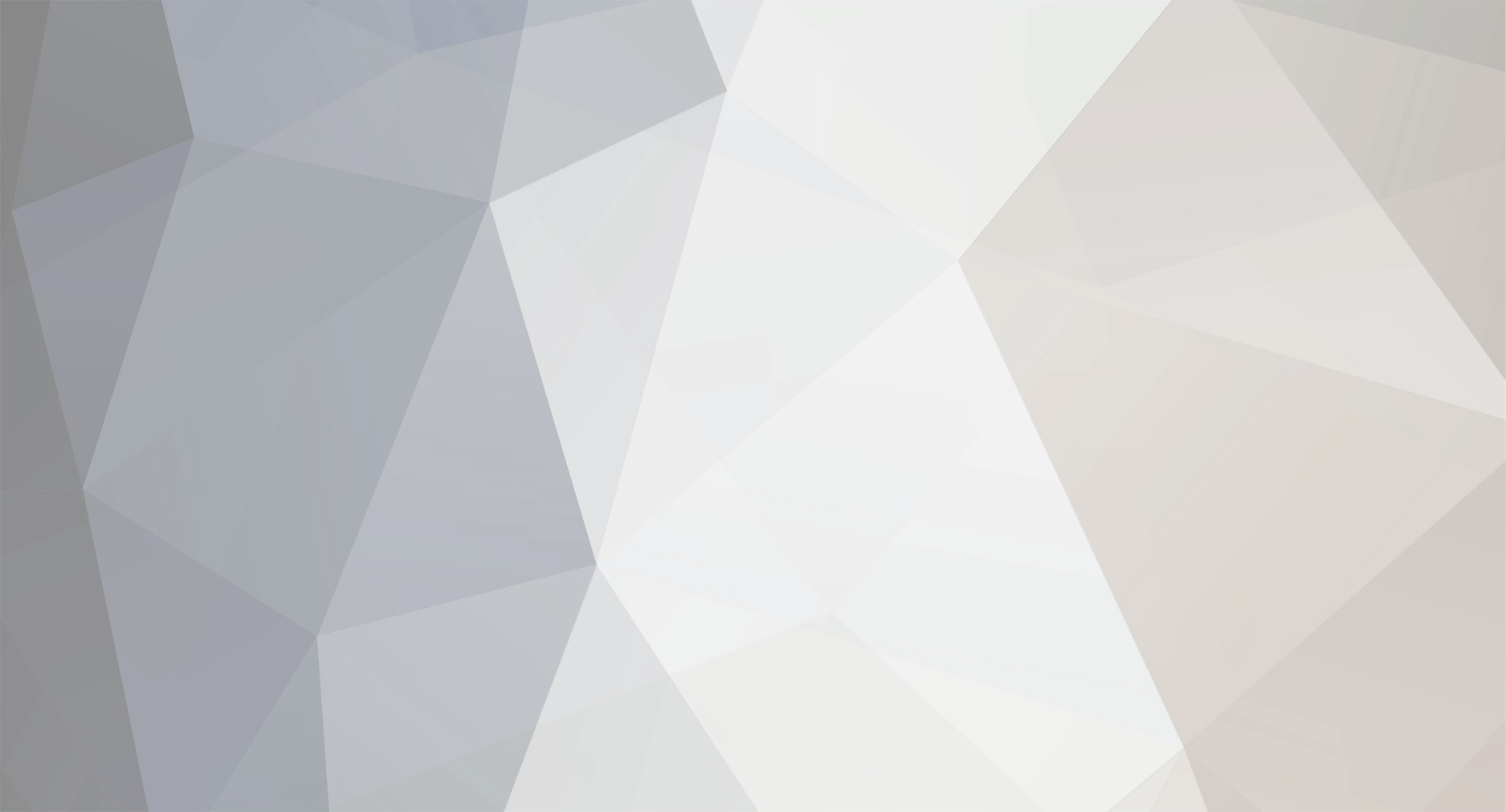
HappyKiller1O1
Members-
Posts
581 -
Joined
-
Last visited
Everything posted by HappyKiller1O1
-
In addition to Choonster's answer: DO NOT use the packet handling method provided in coolAlias's tutorial. It is outdated, and bulky. Use SimpleImpl, docs are here, and a in depth tutorial here.
-
When you loop though your key codes, you set ALL of them to true. So, the game thinks all four keys are pressed down at once. Try doing this: @Override public void run() { int forward = gameSettings.keyBindForward.getKeyCode(); int right = gameSettings.keyBindRight.getKeyCode(); int back = gameSettings.keyBindBack.getKeyCode(); int left = gameSettings.keyBindLeft.getKeyCode(); int[] keyCodes = {forward, right, back, left}; long oldTime = System.currentTimeMillis(); while (System.currentTimeMillis() - oldTime < walkingTime) { KeyBinding.setKeyBindState(keyCodes[0], true); } KeyBinding.setKeyBindState(keyCode, false); try { Thread.sleep(period); } catch (InterruptedException e) { e.printStackTrace(); } } That should only set the forward key to be pressed.
-
Should of noticed that. Thank you so much Choonster! One other, tiny problem: in the picture below, you'll see the backpack fully rendering, but these two little textures at the player's feet are very weird. I was thinking it is because my texture size for my model currently 64x64, but I am not sure.
-
A list of client side mods? (help me plz)
HappyKiller1O1 replied to tavrak's topic in Minecraft General
iChuns hat mod is pretty cool, and Damage Indicators is helpful. Both are client side only. -
Alright, so I have finall figured out how to make layers and such to display my model on the player. Currently, I can not use RenderLivingEvent because of a memory leak. So, I have reverted to adding the layer to the player in my ClientProxy. This would work fine, but my LayerBipedBackpack requires an instance of RendererLivingEntity: (Minecraft.getMinecraft().getRenderManager().getSkinMap().get("default")).addLayer(new LayerBipedBackpack(need RenderLivingEntity instance for the player)); If anyone could provide an answer, it would be very helpful!
-
So, I actually got some progress done on this (looking through the system). And, the model renders, but with two problems: first is MAJOR FPS drop (150 to 3). I am guessing it is because I add the layer in my event constantly, but I am not sure. Secondly, the model scale is incorrect, as shown here (it's rendered too small, just below the body which is where it needs to be): My classes: Layer renderer base (for ease when I need to render different backpack models): package com.happykiller.weightlimit.client.renderer; import com.happykiller.weightlimit.main.ModReference; import com.happykiller.weightlimit.render.models.ModelMediumBackpack; import net.minecraft.client.model.ModelBase; import net.minecraft.client.renderer.GlStateManager; import net.minecraft.client.renderer.entity.RendererLivingEntity; import net.minecraft.client.renderer.entity.layers.LayerRenderer; import net.minecraft.entity.EntityLivingBase; import net.minecraft.util.ResourceLocation; public abstract class LayerBackpackBase<T extends ModelBase> implements LayerRenderer<EntityLivingBase> { protected T field_177186_d; private final RendererLivingEntity<?> renderer; private float alpha = 1.0F; private float colorR = 1.0F; private float colorG = 1.0F; private float colorB = 1.0F; public LayerBackpackBase(RendererLivingEntity<?> rendererIn) { this.renderer = rendererIn; this.initBackpack(); } public void doRenderLayer(EntityLivingBase entitylivingbaseIn, float p_177141_2_, float p_177141_3_, float partialTicks, float p_177141_5_, float p_177141_6_, float p_177141_7_, float scale) { renderLayer(entitylivingbaseIn, p_177141_2_, p_177141_3_, partialTicks, p_177141_5_, p_177141_6_, p_177141_7_, scale); } private void renderLayer(EntityLivingBase entitylivingbaseIn, float f1, float f2, float partialTicks, float p_177182_5_, float p_177182_6_, float p_177182_7_, float scale) { T t = this.field_177186_d; t.setModelAttributes(this.renderer.getMainModel()); t.setLivingAnimations(entitylivingbaseIn, f1, f2, partialTicks); t = (T)new ModelMediumBackpack(6.0F); this.setShownLayer(t); this.renderer.bindTexture(new ResourceLocation(ModReference.MOD_RL + "/models/backpack/MediumBackpack.png")); GlStateManager.color(this.colorR, this.colorG, this.colorB, this.alpha); t.render(entitylivingbaseIn, f1, f2, p_177182_5_, p_177182_6_, p_177182_7_, scale); } public boolean shouldCombineTextures() { return false; } public abstract void initBackpack(); protected abstract void setShownLayer(T model); } LayerBipedBackpack (the layer I add): package com.happykiller.weightlimit.client.renderer; import net.minecraft.client.model.ModelBiped; import net.minecraft.client.renderer.entity.RendererLivingEntity; import net.minecraft.entity.EntityLivingBase; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; @SideOnly(Side.CLIENT) public class LayerBipedBackpack extends LayerBackpackBase<ModelBiped> { public LayerBipedBackpack(RendererLivingEntity<?> rendererIn) { super(rendererIn); } public void initBackpack() { this.field_177186_d = new ModelBiped(0.5F); } protected void setShownLayer(ModelBiped model) { model.bipedBody.showModel = true; } } My Event: @SubscribeEvent public void onRenderEntity(RenderLivingEvent.Post<EntityPlayer> event) { //System.out.println("Render Event Called"); if(event.entity instanceof EntityPlayer) { EntityPlayer player = (EntityPlayer)event.entity; event.renderer.addLayer(new LayerBipedBackpack(event.renderer)); LogHelper.logInfo("Layer Added"); } } I hope someone can help me here, I don't understand this plague of the new layer system. It seems no one ever has a clue on what to do here.
-
Well, if the recipe isn't working, it must not be registering. If the enchanting isn't working, you'll have to set the NBT manually.
-
Call "CraftingRecipes.addRecipe" in your main mod init, not your proxy. Also, try doing "ItemStack(ModItem.eff1, 1, 0)". The 1 is the amount required, the 0 is the metadata.
-
I said look at how armor is rendered on the player, not armor itself. You could use that system to render a cube above an entities head.
-
Try just adding the recipe in the init of your main mod class. Or, make sure your CommonProxy is registered correctly.
-
Could you show where you're registering it? This should work just fine.
-
Please take a look at the ItemEnchantedBook class (net.minecraft.item.ItemEnchantedBook). With this, you will see that enchantments are saved via NBT to the ItemStack. When creating the book in crafting, you need to set the correct NBT.
-
So, I need to render my custom model (a backpack) on the player when my item is in my custom slot. I know how to check for my item there, I am just not sure how to render it. I know it requires me to use this new system in 1.8, but I have no clue where to start. If someone could point me to a tutorial, some docs, or give me some examples on how to accomplish this, it would be great.
-
@gummby8 There is your answer.
-
I'd take a look at how armor is rendered, then just use that to produce the cube over the entities head. I know as of 1.8, Minecraft uses a "layered" armor system. But, I have not been able to figure out how to utilize it correctly.
-
There ya go! Should of mentioned that in your post.
-
The problem with that is: when you need to reset the sensitivity, you won't have the sensitivity the player may have set his game to. Thus, they would have to constantly change their sensitivity back (if they changed it before) every time it is affected.
-
[1.8] Rendering a different model on the player
HappyKiller1O1 replied to HappyKiller1O1's topic in Modder Support
So, looking into it more I found the class that renders armor. Except, the methods are all "func_..." which is quite annoying. Perhaps someone could explain how I would go about using that class, or how it renders things on the player, to allow me to render my custom model on the player? -
[1.8] Rendering a different model on the player
HappyKiller1O1 replied to HappyKiller1O1's topic in Modder Support
Actually, found getArmorModel within the Item class. My problem is, I am trying to render a custom model (backpack) on the players back. I made the model, set the class, and made my base model of it as a child for the ModelBiped body. But, how would I go about rendering this on the player when my item is in my custom slot? I feel like I should do this in #setInventorySlotContents, as to not constantly be checking for it in a tick handler. Still, I have no clue how to render things like this in 1.8. -
So, I was following a tutorial on adding some custom models to a mod, but it was for 1.7.10. With this, ItemArmor#getArmorModel() was used to get the actual model. Sadly, this method is gone from 1.8. I read that 1.8 uses layers for models being rendered on the player, but I'm not sure where to find the place to render these. If anyone could help, I would be very appreciative!
-
[1.8] Can't iterate through a keySet
HappyKiller1O1 replied to HappyKiller1O1's topic in Modder Support
I'm sorry! I've never used Iterator before, so I wasn't sure how to do it, and just went off some basic Java sites. Anyway, got that working, so no problems in the packet handling. My only problem now is, file overwriting. Every time I load up the server, or client, the original json file that may have been changed gets overwritten. I've tried using BufferedReader to read the line and check if it was null. And I've tried doing File#length; nothing seems to work. I'll be looking through some Java sites for a solution, but if any of you have one, feel free to post it! -
So, I am attempting to iterate through an NBTTagCompound in order to send a Map through a packet. Problem is, I can't seem to iterate correctly though it. Here is my code: public IMessage onMessage(final PacketServerToClientWeightUpdate message, MessageContext ctx) { System.out.println("onMessage Ran!"); IThreadListener mainThread = Minecraft.getMinecraft(); final Map<String, Float> clientWeightValues = ModReference.weightMap; mainThread.addScheduledTask(new Runnable() { public void run() { Iterator it = message.mapData.getKeySet().iterator(); while(it.hasNext()) { Map.Entry<String, Float> pair = (Map.Entry<String, Float>)it.next(); System.out.println("Pair is: " + pair.getKey() + "/" + pair.getValue()); clientWeightValues.put(pair.getKey(), pair.getValue()); it.remove(); } System.out.println("FINSHED SERVER PACKET"); } }); return null; } When I attempt to send the packet, I get a "String cannot be cast to Map.Entry". When looking online, it says I need an entrySet to iterate; but I can't get one from NBTTagCompound. Any ideas on how to do this?
-
[1.7.10] help with client not running in eclipse
HappyKiller1O1 replied to iceblu008's topic in Modder Support
For one, you should be using 1.8, as 1.7.10 will be quite out of date in a few months. Secondly, how did you setup your workspace?